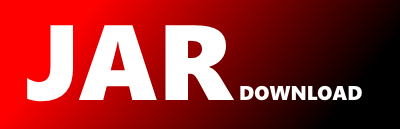
org.glassfish.jersey.ext.cdi1x.internal.InjecteeSkippingAnalyzer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jersey-cdi1x Show documentation
Show all versions of jersey-cdi1x Show documentation
Jersey CDI 1.1 integration
The newest version!
/*
* Copyright (c) 2013, 2020 Oracle and/or its affiliates. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package org.glassfish.jersey.ext.cdi1x.internal;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.Member;
import java.lang.reflect.Method;
import java.util.HashSet;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import org.glassfish.jersey.internal.util.collection.Views;
import org.glassfish.hk2.api.ClassAnalyzer;
import org.glassfish.hk2.api.MultiException;
import jakarta.enterprise.inject.spi.BeanManager;
import jakarta.inject.Inject;
/**
* Class analyzer that ignores given injection points.
* Used for CDI integration, where we need to avoid HK2 replacing CDI injected entities.
*
* @author Jakub Podlesak
*/
public final class InjecteeSkippingAnalyzer implements ClassAnalyzer {
private final ClassAnalyzer defaultAnalyzer;
private final Map, Set> methodsToSkip;
private final Map, Set> fieldsToSkip;
private final BeanManager beanManager;
private final CdiComponentProvider cdiComponentProvider;
public InjecteeSkippingAnalyzer(ClassAnalyzer defaultAnalyzer,
Map, Set> methodsToSkip,
Map, Set> fieldsToSkip,
BeanManager beanManager) {
this.defaultAnalyzer = defaultAnalyzer;
this.methodsToSkip = methodsToSkip;
this.fieldsToSkip = fieldsToSkip;
this.beanManager = beanManager;
this.cdiComponentProvider = beanManager.getExtension(CdiComponentProvider.class);
}
@Override
public Constructor getConstructor(Class type) throws MultiException, NoSuchMethodException {
throw new IllegalStateException(LocalizationMessages.CDI_CLASS_ANALYZER_MISUSED());
}
@Override
public Set getInitializerMethods(Class type) throws MultiException {
final Set originalMethods = defaultAnalyzer.getInitializerMethods(type);
final Set skippedMethods = getMembersToSkip(type, methodsToSkip);
return Views.setDiffView(originalMethods, skippedMethods);
}
@Override
public Set getFields(Class type) throws MultiException {
final Set originalFields = defaultAnalyzer.getFields(type);
final Set skippedFields = getMembersToSkip(type, fieldsToSkip);
addCdiInjectedFieldsToSkip(skippedFields, originalFields);
return Views.setDiffView(originalFields, skippedFields);
}
@Override
public Method getPostConstructMethod(Class type) throws MultiException {
throw new IllegalStateException(LocalizationMessages.CDI_CLASS_ANALYZER_MISUSED());
}
@Override
public Method getPreDestroyMethod(Class type) throws MultiException {
throw new IllegalStateException(LocalizationMessages.CDI_CLASS_ANALYZER_MISUSED());
}
private Set getMembersToSkip(final Class> type, final Map, Set> skippedMembers) {
final Set directResult = skippedMembers.get(type);
if (directResult != null) {
return directResult;
}
// fallback for GLASSFISH-20255
final Set compositeResult = new HashSet<>();
for (Entry, Set> type2Method : skippedMembers.entrySet()) {
if (type2Method.getKey().isAssignableFrom(type)) {
compositeResult.addAll(type2Method.getValue());
}
}
return compositeResult;
}
private void addCdiInjectedFieldsToSkip(Set skippedFields, Set originalFields) {
for (Field field : originalFields) {
if (field.getAnnotation(Inject.class) != null && !cdiComponentProvider.isHk2ProvidedType(field.getType())) {
skippedFields.add(field);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy