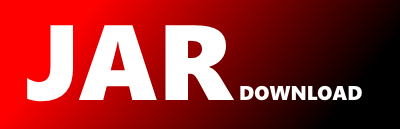
org.glassfish.jersey.jettison.internal.JettisonJaxbUnmarshaller Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jersey-media-json-jettison Show documentation
Show all versions of jersey-media-json-jettison Show documentation
Jersey JSON Jettison entity providers support module.
The newest version!
/*
* Copyright (c) 2010, 2022 Oracle and/or its affiliates. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package org.glassfish.jersey.jettison.internal;
import java.io.File;
import java.io.InputStream;
import java.io.Reader;
import java.net.URL;
import jakarta.xml.bind.JAXBContext;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.JAXBException;
import jakarta.xml.bind.PropertyException;
import jakarta.xml.bind.Unmarshaller;
import jakarta.xml.bind.UnmarshallerHandler;
import jakarta.xml.bind.ValidationEventHandler;
import jakarta.xml.bind.annotation.adapters.XmlAdapter;
import jakarta.xml.bind.attachment.AttachmentUnmarshaller;
import javax.xml.stream.XMLEventReader;
import javax.xml.stream.XMLStreamReader;
import javax.xml.transform.Source;
import javax.xml.validation.Schema;
import org.glassfish.jersey.jettison.JettisonConfig;
import org.w3c.dom.Node;
import org.xml.sax.InputSource;
/**
* JSON JAXB unmarshaller.
*
* @author Jakub Podlesak
*/
public class JettisonJaxbUnmarshaller extends BaseJsonUnmarshaller implements Unmarshaller {
public JettisonJaxbUnmarshaller(JAXBContext jaxbContext, JettisonConfig jsonConfig) throws JAXBException {
super(jaxbContext, jsonConfig);
}
// Unmarshaller
@Override
public Object unmarshal(File file) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(file);
}
@Override
public Object unmarshal(InputStream inputStream) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(inputStream);
}
@Override
public Object unmarshal(Reader reader) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(reader);
}
@Override
public Object unmarshal(URL url) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(url);
}
@Override
public Object unmarshal(InputSource inputSource) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(inputSource);
}
@Override
public Object unmarshal(Node node) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(node);
}
@Override
public JAXBElement unmarshal(Node node, Class type) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(node, type);
}
@Override
public Object unmarshal(Source source) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(source);
}
@Override
public JAXBElement unmarshal(Source source, Class type) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(source, type);
}
@Override
public Object unmarshal(XMLStreamReader xmlStreamReader) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(xmlStreamReader);
}
@Override
public JAXBElement unmarshal(XMLStreamReader xmlStreamReader, Class type) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(xmlStreamReader, type);
}
@Override
public Object unmarshal(XMLEventReader xmlEventReader) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(xmlEventReader);
}
@Override
public JAXBElement unmarshal(XMLEventReader xmlEventReader, Class type) throws JAXBException {
return this.jaxbUnmarshaller.unmarshal(xmlEventReader, type);
}
@Override
public UnmarshallerHandler getUnmarshallerHandler() {
return this.jaxbUnmarshaller.getUnmarshallerHandler();
}
@Override
public void setEventHandler(ValidationEventHandler validationEventHandler) throws JAXBException {
this.jaxbUnmarshaller.setEventHandler(validationEventHandler);
}
@Override
public ValidationEventHandler getEventHandler() throws JAXBException {
return this.jaxbUnmarshaller.getEventHandler();
}
@Override
public void setProperty(String key, Object value) throws PropertyException {
this.jaxbUnmarshaller.setProperty(key, value);
}
@Override
public Object getProperty(String key) throws PropertyException {
return this.jaxbUnmarshaller.getProperty(key);
}
@Override
public void setSchema(Schema schema) {
this.jaxbUnmarshaller.setSchema(schema);
}
@Override
public Schema getSchema() {
return this.jaxbUnmarshaller.getSchema();
}
@Override
public > void setAdapter(A xmlAdapter) {
this.jaxbUnmarshaller.setAdapter(xmlAdapter);
}
@Override
public > void setAdapter(Class type, A adapter) {
this.jaxbUnmarshaller.setAdapter(type, adapter);
}
@Override
public > A getAdapter(Class type) {
return this.jaxbUnmarshaller.getAdapter(type);
}
@Override
public void setAttachmentUnmarshaller(AttachmentUnmarshaller attachmentUnmarshaller) {
this.jaxbUnmarshaller.setAttachmentUnmarshaller(attachmentUnmarshaller);
}
@Override
public AttachmentUnmarshaller getAttachmentUnmarshaller() {
return this.jaxbUnmarshaller.getAttachmentUnmarshaller();
}
@Override
public void setListener(Listener listener) {
this.jaxbUnmarshaller.setListener(listener);
}
@Override
public Listener getListener() {
return this.jaxbUnmarshaller.getListener();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy