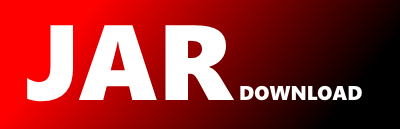
org.glassfish.jersey.jettison.internal.entity.JettisonJaxbElementProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jersey-media-json-jettison Show documentation
Show all versions of jersey-media-json-jettison Show documentation
Jersey JSON Jettison entity providers support module.
The newest version!
/*
* Copyright (c) 2010, 2023 Oracle and/or its affiliates. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package org.glassfish.jersey.jettison.internal.entity;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.lang.annotation.Annotation;
import java.lang.reflect.Type;
import java.nio.charset.Charset;
import jakarta.inject.Inject;
import jakarta.ws.rs.Consumes;
import jakarta.ws.rs.Produces;
import jakarta.ws.rs.core.Configuration;
import jakarta.ws.rs.core.Context;
import jakarta.ws.rs.core.MediaType;
import jakarta.ws.rs.ext.Providers;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.JAXBException;
import jakarta.xml.bind.Marshaller;
import jakarta.xml.bind.Unmarshaller;
import org.glassfish.jersey.jaxb.internal.AbstractJaxbElementProvider;
import org.glassfish.jersey.jettison.JettisonJaxbContext;
import org.glassfish.jersey.jettison.JettisonMarshaller;
import org.glassfish.jersey.message.internal.ReaderWriter;
/**
* JSON message entity media type provider (reader & writer) for {@link jakarta.xml.bind.JAXBElement}
* type.
*
* @author Jakub Podlesak
*/
public class JettisonJaxbElementProvider extends AbstractJaxbElementProvider {
JettisonJaxbElementProvider(Providers ps, Configuration config) {
super(ps, config);
}
JettisonJaxbElementProvider(Providers ps, MediaType mt, Configuration config) {
super(ps, mt, config);
}
@Override
public boolean isReadable(Class> type, Type genericType, Annotation[] annotations, MediaType mediaType) {
return super.isReadable(type, genericType, annotations, mediaType);
}
@Override
public boolean isWriteable(Class> type, Type genericType, Annotation[] annotations, MediaType mediaType) {
return super.isWriteable(type, genericType, annotations, mediaType);
}
@Produces("application/json")
@Consumes("application/json")
public static final class App extends JettisonJaxbElementProvider {
@Inject
public App(@Context Providers ps, @Context Configuration config) {
super(ps, MediaType.APPLICATION_JSON_TYPE, config);
}
}
@Produces("*/*")
@Consumes("*/*")
public static final class General extends JettisonJaxbElementProvider {
@Inject
public General(@Context Providers ps, @Context Configuration config) {
super(ps, config);
}
@Override
protected boolean isSupported(MediaType m) {
return m.getSubtype().endsWith("+json");
}
}
@Override
protected final JAXBElement> readFrom(Class> type, MediaType mediaType, Unmarshaller unmarshaller,
InputStream entityStream) throws JAXBException {
final Charset c = ReaderWriter.getCharset(mediaType);
return JettisonJaxbContext.getJSONUnmarshaller(unmarshaller)
.unmarshalJAXBElementFromJSON(new InputStreamReader(entityStream, c), type);
}
@Override
protected final void writeTo(JAXBElement> t, MediaType mediaType, Charset c, Marshaller m,
OutputStream entityStream) throws JAXBException {
JettisonMarshaller jsonMarshaller = JettisonJaxbContext.getJSONMarshaller(m);
if (isFormattedOutput()) {
jsonMarshaller.setProperty(JettisonMarshaller.FORMATTED, true);
}
jsonMarshaller.marshallToJSON(t, new OutputStreamWriter(entityStream, c));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy