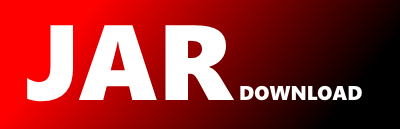
com.sun.jdo.api.persistence.enhancer.classfile.ConstantPool Maven / Gradle / Ivy
/*
* Copyright (c) 1997, 2018 Oracle and/or its affiliates. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package com.sun.jdo.api.persistence.enhancer.classfile;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.PrintStream;
import java.util.Hashtable;
import java.util.Vector;
/**
* Constant Pool implementation - this represents the constant pool
* of a class in a class file.
*/
public class ConstantPool implements VMConstants {
/* The actual pool */
private Vector pool = new Vector();
/* uniqifier tables */
private boolean hashed = false;
private Hashtable utfTable = new Hashtable(11);
private Hashtable unicodeTable = new Hashtable(3);
private Hashtable stringTable = new Hashtable(11);
private Hashtable classTable = new Hashtable(11);
private Hashtable intTable = new Hashtable(3);
private Hashtable floatTable = new Hashtable(3);
private Hashtable longTable = new Hashtable(3);
private Hashtable doubleTable = new Hashtable(3);
private Vector methodRefTable = new Vector();
private Vector fieldRefTable = new Vector();
private Vector ifaceMethodRefTable = new Vector();
private Vector nameAndTypeTable = new Vector();
/* public accessors */
/**
* Return the number of pool entries.
*/
public int nEntries() {
return pool.size();
}
/**
* Return the constant in the pool at the specified entry index
*/
public ConstBasic constantAt (int index) {
return (ConstBasic) pool.elementAt(index);
}
/**
* Find or create a class constant in the pool
*/
public ConstClass addClass (String className) {
hashConstants();
ConstClass c = (ConstClass) classTable.get(className);
if (c == null) {
c = new ConstClass(addUtf8(className));
internConstant(c);
}
return c;
}
/**
* Find or create a field constant in the pool
*/
public ConstFieldRef addFieldRef (String className, String fieldName,
String type) {
hashConstants();
ConstFieldRef f = (ConstFieldRef)
searchTable(fieldRefTable, className, fieldName, type);
if (f == null) {
f = new ConstFieldRef (addClass(className),
addNameAndType(fieldName, type));
internConstant(f);
}
return f;
}
/**
* Find or create a method constant in the pool
*/
public ConstMethodRef addMethodRef (String className, String methodName,
String type) {
hashConstants();
ConstMethodRef m = (ConstMethodRef)
searchTable(methodRefTable, className, methodName, type);
if (m == null) {
m = new ConstMethodRef (addClass(className),
addNameAndType(methodName, type));
internConstant(m);
}
return m;
}
/**
* Find or create an interface method constant in the pool
*/
public ConstInterfaceMethodRef addInterfaceMethodRef (String className,
String methodName, String type) {
hashConstants();
ConstInterfaceMethodRef m = (ConstInterfaceMethodRef)
searchTable(ifaceMethodRefTable, className, methodName, type);
if (m == null) {
m = new ConstInterfaceMethodRef (addClass(className),
addNameAndType(methodName, type));
internConstant(m);
}
return m;
}
/**
* Find or create a string constant in the pool
*/
public ConstString addString (String s) {
hashConstants();
ConstString cs = (ConstString) stringTable.get(s);
if (cs == null) {
cs = new ConstString(addUtf8(s));
internConstant(cs);
}
return cs;
}
/**
* Find or create an integer constant in the pool
*/
public ConstInteger addInteger (int i) {
hashConstants();
Integer io = new Integer(i);
ConstInteger ci = (ConstInteger) intTable.get(io);
if (ci == null) {
ci = new ConstInteger(i);
internConstant(ci);
}
return ci;
}
/**
* Find or create a float constant in the pool
*/
public ConstFloat addFloat (float f) {
hashConstants();
Float fo = new Float(f);
ConstFloat cf = (ConstFloat) floatTable.get(fo);
if (cf == null) {
cf = new ConstFloat(f);
internConstant(cf);
}
return cf;
}
/**
* Find or create a long constant in the pool
*/
public ConstLong addLong (long l) {
hashConstants();
Long lo = new Long(l);
ConstLong cl = (ConstLong) longTable.get(lo);
if (cl == null) {
cl = new ConstLong(l);
internConstant(cl);
internConstant(null);
}
return cl;
}
/**
* Find or create a double constant in the pool
*/
public ConstDouble addDouble (double d) {
hashConstants();
Double dobj = new Double(d);
ConstDouble cd = (ConstDouble) doubleTable.get(dobj);
if (cd == null) {
cd = new ConstDouble(d);
internConstant(cd);
internConstant(null);
}
return cd;
}
/**
* Find or create a name/type constant in the pool
*/
public ConstNameAndType addNameAndType (String name, String type) {
hashConstants();
for (int i=0; i 0)
nconstants -= readConstant(input);
resolvePool();
}
void print (PrintStream out) {
for (int i=0; i 1)
pool.addElement(null);
return slots;
}
private void internConstant (ConstBasic c) {
if (c != null) {
c.setIndex(pool.size());
recordConstant(c);
}
pool.addElement(c);
}
private void recordConstant (ConstBasic c) {
if (c != null) {
switch (c.tag()) {
case CONSTANTUtf8:
utfTable.put(((ConstUtf8)c).asString(), c);
break;
case CONSTANTUnicode:
unicodeTable.put(((ConstUnicode)c).asString(), c);
break;
case CONSTANTInteger:
intTable.put(new Integer(((ConstInteger)c).value()), c);
break;
case CONSTANTFloat:
floatTable.put(new Float(((ConstFloat)c).value()), c);
break;
case CONSTANTLong:
longTable.put(new Long(((ConstLong)c).value()), c);
break;
case CONSTANTDouble:
doubleTable.put(new Double(((ConstDouble)c).value()), c);
break;
case CONSTANTClass:
classTable.put(((ConstClass)c).asString(), c);
break;
case CONSTANTString:
stringTable.put(((ConstString)c).value().asString(), c);
break;
case CONSTANTFieldRef:
fieldRefTable.addElement(c);
break;
case CONSTANTMethodRef:
methodRefTable.addElement(c);
break;
case CONSTANTInterfaceMethodRef:
ifaceMethodRefTable.addElement(c);
break;
case CONSTANTNameAndType:
nameAndTypeTable.addElement(c);
break;
}
}
}
private ConstBasicMemberRef searchTable(Vector table, String cname,
String mname, String sig) {
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy