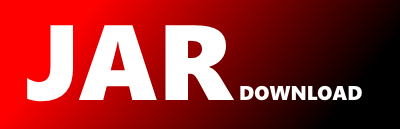
com.sun.messaging.ConnectionConfiguration Maven / Gradle / Ivy
/*
* Copyright (c) 2000, 2020 Oracle and/or its affiliates. All rights reserved.
* Copyright (c) 2021, 2024 Contributors to the Eclipse Foundation
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package com.sun.messaging;
/**
* The ConnectionConfiguration
class contains OpenMQ specific connection configuration property names and
* special values.
*
* @see com.sun.messaging.ConnectionFactory com.sun.messaging.ConnectionFactory
*/
public class ConnectionConfiguration {
/* No public constructor needed */
private ConnectionConfiguration() {
}
/**
* This property holds the address list that will be used to connect to the OpenMQ Message Service.
*
* Message Server Address Syntax
*
* The syntax for specifying a message server address is as follows:
*
*
* scheme://address_syntax
*
*
*
* where the scheme
and address_syntax
are described in the folowing table.
*
*
* scheme and address_syntax for a message server address
*
* Scheme
* Connection Service
* Description
* Syntax
*
*
*
* mq
*
* jms
* and
* ssljms
*
* The MQ Port Mapper at the specified host and port will handle the connection request, dynamically
* assigning a port based on the specified connection service. Once the port number is known, MQ makes the
* connection.
*
* [hostName][:port][/serviceName]
* Defaults (for jms service only):
* hostName = localhost
* port = 7676
* serviceName = jms
*
*
*
*
* mqtcp
*
* jms
*
* MQ makes a direct tcp connection to the specified host and port to establish a connection.
*
* hostName:port/jms
*
*
*
*
*
* mqssl
*
* ssljms
*
* MQ makes a direct, secure ssl connection to the specified host and port to establish a
* connection.
*
* hostName:port/ssljms
*
*
*
*
*
* htttp
*
* httpjms
*
* MQ makes a direct HTTP connection to the specified MQ tunnel servlet URL. (The broker must be
* configured to access the tunnel servlet.)
*
* HTTPtunnelServletURL
*
*
*
*
*
* htttps
*
* httpsjms
*
* MQ makes a direct HTTPS connection to the specified MQ tunnel servlet URL. (The broker must be
* configured to access the tunnel servlet.)
*
* HTTPStunnelServletURL
*
*
*
*
*
* mqws
*
* wsjms
*
* MQ makes a direct WebSocket connection to the specified host and port to establish a connection.
*
*
* hostName:port/wsjms
*
*
*
*
*
* mqwss
*
* wssjms
*
* MQ makes a direct WebSocket secure connection to the specified host and port to establish a
* connection.
*
* hostName:port/wssjms
*
*
*
*
*
*
*
* The following table shows how the message server address syntax applies in some typical cases.
*
*
* typical message server address syntax applications
*
* Connection Service
* Broker Host
* Port
* Example Address
*
*
*
*
* Unspecified
*
* Unspecified
*
* Unspecified
*
* Default
* (mq://localHost:7676/jms)
*
*
*
*
* Unspecified
*
* Specified Host
*
* Unspecified
*
* myBkrHost
* (mq://myBkrHost:7676/jms)
*
*
*
*
* Unspecified
*
* Unspecified
*
* Specified Portmapper Port
*
* 1012
* (mq://localHost:1012/jms)
*
*
*
*
* ssljms
*
* Local Host
*
* Default Portmapper Port
*
* mq://localHost:7676/ssljms
*
*
*
* ssljms
*
* Specified Host
*
* Default Portmapper Port
*
* mq://myBkrHost:7676/ssljms
*
*
* ssljms
*
* Specified Host
*
* Specified Portmapper Port
*
* mq://myBkrHost:1012/ssljms
*
*
*
*
* jms
*
* Local Host
*
* Specified Service Port
*
* mqtcp://localhost:1032/jms
*
*
*
*
* ssljms
*
* Specified Host
*
* Specified Service Port
*
* mqssl://myBkrHost:1034/ssljms
*
*
*
*
* httpjms
*
* N/A
*
* N/A
*
* http://websrvr1:8085/imq/tunnel
*
*
*
*
* httpsjms
*
* N/A
*
* N/A
*
* https://websrvr2:8090/imq/tunnel
*
*
*
*
* wsjms
*
* Specified Host
*
* Specified Service Port
*
* mqws://websrvr1:7670/wsjms
*
*
*
*
* wssjms
*
* Specified Host
*
* Specified Service Port
*
* mqwss://websrvr2:7671/wssjms
*
*
*
*
*
*
* The default value of this property is empty
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqAddressListBehavior
* @see com.sun.messaging.ConnectionConfiguration#imqAddressListIterations
*/
public static final String imqAddressList = "imqAddressList";
/**
* This property holds the number of times that OpenMQ will iterate through imqAddressList
when
* connecting to the OpenmMQ Service.
*
* The default value of this property is 1
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqAddressList
* @see com.sun.messaging.ConnectionConfiguration#imqAddressListBehavior
*/
public static final String imqAddressListIterations = "imqAddressListIterations";
/**
* This property determines how OpenMQ will select entries from the imqAddressList
property to use
* when making a connection to the OpenMQ Message Service.
*
* The acceptable values for this property are PRIORITY
and RANDOM
.
*
* When PRIORITY
is used, OpenMQ will start with the firstentry in
* imqAddressList
when attempting to make the first connection to the OpenMQ Message Service.
*
* Subsequently, when OpenMQ is attempting to re-connect to the Message Service, it will use successive entries from
* imqAddressList
in the order they are specified.
*
* When RANDOM
is used, OpenMQ will start with a random entry in
* imqAddressList
when attempting to make the first connection to the OpenMQ Message Service.
*
* Subsequently, when OpenMQ is attempting to re-connect to the Message Service, it will use entries in the order they
* are specified in imqAddressList
starting with the initial randomly chosen entry.
*
* The default value of this property is PRIORITY
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqAddressList
* @see com.sun.messaging.ConnectionConfiguration#imqAddressListIterations
*/
public static final String imqAddressListBehavior = "imqAddressListBehavior";
/**
* This property holds the connection type used to connect to the OpenMQ Message Service.
*
* Note that this property is superseded by the imqAddressList
property and is present only for
* compatibility with MQ 3.0 clients. This property may be removed in the next major version of MQ.
*
* The default value of this property is TCP
*
* The allowable values are TCP,TLS,HTTP,and the special value - ...
*
* When the special value of ...
is used, the classname specified in the property
* imqConnectionHandler
is required to handle the connection to the OpenMQ Message Service.
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqAddressList
*/
public static final String imqConnectionType = "imqConnectionType";
/**
* This property holds the name of the class that will be used to handle the connection to the OpenMQ Message Service
* and is required when the value of the imqConnectionType
property is set to
* ...
*
* This property is not normally specified and will assume the classname handling the ConnectionType specified in
* imqConnectionType
. However, if specified, this property overrides
* imqConnectionType
.
*
* The default value of this property is
* com.sun.messaging.jmq.jmsclient.protocol.tcp.TCPStreamHandler
*/
public static final String imqConnectionHandler = "imqConnectionHandler";
/**
* This property holds the default username that will be used to authenticate with the OpenMQ Message Service.
*
* The default value of this property is guest
*/
public static final String imqDefaultUsername = "imqDefaultUsername";
/**
* This property holds the default password that will be used to authenticate with the OpenMQ Message Service.
*
* The default value of this property is guest
*/
public static final String imqDefaultPassword = "imqDefaultPassword";
/**
* This property holds the the maximum time, in milliseconds, that a OpenMQ Client Application will wait before throwing
* a JMSException when awaiting an acknowledgement from the OpenMQ Message Service.
*
* A value of 0
indicates that it will wait indefinitely.
*
* The default value for this property is 0
i.e. indefinitely.
*/
public static final String imqAckTimeout = "imqAckTimeout";
/**
* This property holds the the minimum time, in milliseconds, that the MQ client runtime will wait for an asynchronous
* send to complete before calling the CompletionListener's onException method with timed out exception
*
* A value of 0
is not allowed.
*
* The default value for this property is 180000
*/
public static final String imqAsyncSendCompletionWaitTimeout = "imqAsyncSendCompletionWaitTimeout";
/**
* This property indicates whether the OpenMQ Client Application will attempt to reconnect to the OpenMQ Message Service
* upon losing its connection.
*
* The default value for this property is false
*/
public static final String imqReconnectEnabled = "imqReconnectEnabled";
/**
* This property specifies the interval, in milliseconds, between successive reconnect attempts made by the MQ Client
* Application to the OpenMQ Message Service.
*
* The default value for this property is 30000
milliseconds.
*/
public static final String imqReconnectInterval = "imqReconnectInterval";
/**
* This property holds the number of reconnect attempts for each address in the imqAddressList
property
* that the OpenMQ Client Application will make before moving on the the next address in imqAddressList
.
* will make in trying to reconnect to the OpenMQ Message Service. A value of -1
indicates that the
* MQ Client Application will make an unlimited number of reconnect attempts.
*
* Note that this property is only applicable when imqReconnectEnabled
is set to
* true
.
*
* The default value for this property is 0
- i.e. No reconnect attempts.
*/
public static final String imqReconnectAttempts = "imqReconnectAttempts";
/**
* This property specifies the 'ping' interval, in seconds, between successive attempts made by an MQ Connection
* to verify that its physical connection to the MQ broker is still functioning properly. The MQ Client Runtime uses
* this value to set up the frequency of 'pings' made by MQ Connections that originate from this ConnectionFactory.
* Valid values include all positive integers indicating the number of seconds to delay between successive 'pings'. A
* value of 0
, or -1
indicates that the MQ Connection will disable this 'ping'
* functionality.
*
* The default value for this property is 30
- i.e. 30 seconds between 'pings'.
*/
public static final String imqPingInterval = "imqPingInterval";
/**
* When imqAbortOnPingAckTimeout
is true, this property specifies maximum time, in milliseconds, that MQ
* Client Runtime will wait for a ping reply or any data sent from the MQ broker since last 'ping'. This property is
* ignored if imqAbortOnPingAckTimeout
is false.
*
* A value of 0
indicates no timeout
*
* The default value for this property is 0
*
* @see com.sun.messaging.ConnectionConfiguration#imqAbortOnPingAckTimeout
*/
public static final String imqPingAckTimeout = "imqPingAckTimeout";
/**
* This property defines the socket timeout, in milliseconds, used when a TCP connection is made to the broker. A
* timeout of zero is interpreted as an infinite timeout. The connection will then block until established or an error
* occurs. This property is used when connecting to the port mapper as well as when connecting to the required service.
*/
public static final String imqSocketConnectTimeout = "imqSocketConnectTimeout";
/**
* This property defines port mapper client socket read timeout, in milliseconds.
*
* A value of 0 indicates no timeout
*
* The value of this property is set to 60000 by default.
*
*/
public static final String imqPortMapperSoTimeout = "imqPortMapperSoTimeout";
/**
* This property specifies the key store location
*
* The value of this property is not set by default.
*
*/
public static final String imqKeyStore = "imqKeyStore";
/**
* This property specifies the key store password. Set this property to a non-null value will make the connection
* capable of SSL client authenication if requested by broker. If it is set to empty string, Java system property
* javax.net.ssl.keyStorePassword will be used if set
*
* The value of this property is not set by default.
*
*/
public static final String imqKeyStorePassword = "imqKeyStorePassword";
/**
* If this property is set to true, the MQ Client Runtime will abort the connection to the MQ broker when timeout, as
* specified by
* imqPingAckTimeout
, in waiting for a 'ping' reply or any data sent from the MQ broker since last 'ping', then
* the MQ Client Runtime will perform actions as if the connection is broken.
*
* @see com.sun.messaging.ConnectionConfiguration#imqPingAckTimeout
*/
public static final String imqAbortOnPingAckTimeout = "imqAbortOnPingAckTimeout";
/**
* This property indicates whether OpenMQ should set the JMSXAppID property on produced messages.
*
* The default value of this property is false
*/
public static final String imqSetJMSXAppID = "imqSetJMSXAppID";
/**
* This property indicates whether OpenMQ should set the JMSXUserID property on produced messages.
*
* The default value of this property is false
*/
public static final String imqSetJMSXUserID = "imqSetJMSXUserID";
/**
* This property indicates whether OpenMQ should set the JMSXProducerTXID property on produced messages.
*
* The default value of this property is false
*/
public static final String imqSetJMSXProducerTXID = "imqSetJMSXProducerTXID";
/**
* This property indicates whether OpenMQ should set the JMSXConsumerTXID property on consumed messages.
*
* The default value of this property is false
*/
public static final String imqSetJMSXConsumerTXID = "imqSetJMSXConsumerTXID";
/**
* This property indicates whether OpenMQ should set the JMSXRcvTimestamp property on consumed messages.
*
* The default value of this property is false
*/
public static final String imqSetJMSXRcvTimestamp = "imqSetJMSXRcvTimestamp";
/**
* This property holds the hostname that will be used to connect to the OpenMQ Message Service using the TCP and TLS
* ConnectionHandler classes provided with OpenMQ.
*
* Note that this property is superseded by the imqAddressList
property and is present only for
* compatibility with MQ 3.0 clients. This property may be removed in the next major version of MQ.
*
* The default value of this property is localhost
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqAddressList
*/
public static final String imqBrokerHostName = "imqBrokerHostName";
/**
* This property holds the Primary Port number that will be used to connect to the OpenMQ Message Service using
* the TCP and TLS ConnectionHandler classes provided with OpenMQ.
*
* Note that this property is superseded by the imqAddressList
property and is present only for
* compatibility with MQ 3.0 clients. This property may be removed in the next major version of MQ.
*
* The OpenMQ Client uses this port to communicate with the Port Mapper Service to determine the actual port
* number of the connection service that it wishes to use.
*
* The default value of this property is 7676
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqAddressList
*/
public static final String imqBrokerHostPort = "imqBrokerHostPort";
/**
* This property holds the connection service name that will be used to connect to the OpenMQ Message Service using the
* TCP and TLS ConnectionHandler classes provided with OpenMQ.
*
* Note that this property is superseded by the imqAddressList
property and is present only for
* compatibility with MQ 3.0 clients. This property may be removed in the next major version of MQ.
*
* By default, when the value of this property is null
, the MQ Client uses the Primary Port
* to connect to the MQ Message Service and subsequently uses the first connection service that corresponds to
* imqConnnectionType.
*
* When the value of this property is non-null, the MQ Client will first connect to the Port Mapper Service and
* then use the connection service that matches imqBrokerServiceName
ensuring that it corresponds to
* the right imqConnectionType
.
*
* The default value of this property is null
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqAddressList
*/
public static final String imqBrokerServiceName = "imqBrokerServiceName";
/**
* This property holds the actual port number that will be used to connect to the OpenMQ Message Service using the TCP
* and TLS ConnectionHandler classes provided with OpenMQ.
*
* Note that this property is superseded by the imqAddressList
property and is present only for
* compatibility with MQ 3.0 clients. This property may be removed in the next major version of MQ.
*
* By default, when the value of this property is 0
, the MQ Client uses the Primary Port to
* connect to the MQ Message Service and subsequently determines the actual port number of the connection service that
* it wishes to use.
*
* When the value of this property is non-zero, the MQ Client will use this port number to directly connect to the
* connection service that it wishes to use without first communicating with the Port Mapper Service
*
* The default value of this property is 0
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqAddressList
*/
public static final String imqBrokerServicePort = "imqBrokerServicePort";
/**
* This property holds the TLS Provider Classname that will be used when connecting to the OpenMQ Message Service using
* the TLS ConnectionHandler class provided with OpenMQ.
*
* The default value of this property is com.sun.net.ssl.internal.ssl.Provider
*/
public static final String imqSSLProviderClassname = "imqSSLProviderClassname";
/**
* This property indicates whether the host is trusted when connecting to the OpenMQ Message Service using the TLS
* ConnectionHandler class provided with OpenMQ.
*
* If this value is set to false
, then the Root Certificate provided by the Certificate Authority must be
* available to the MQ Client Application when it connects to the MQ Message Service.
*
* The default value of this property is false
*/
public static final String imqSSLIsHostTrusted = "imqSSLIsHostTrusted";
/**
* This property holds the URL that will be used when connecting to the OpenMQ Message Service using the HTTP
* ConnectionHandler class provided with OpenMQ.
*
* Note that this property is superseded by the imqAddressList
property and is present only for
* compatibility with MQ 3.0 clients. This property may be removed in the next major version of MQ.
*
* Since the URL may be shared by multiple message service instances, the optional ServerName
attribute
* can be used to identify a specific message service address. The ServerName
attribute is specified
* using the standard query string syntax. For example -
* http://localhost/imq/tunnel?ServerName=localhost:imqbroker
*
* The default value of this property is http://localhost/imq/tunnel
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqAddressList
*/
public static final String imqConnectionURL = "imqConnectionURL";
/**
* This property indicates whether the OpenMQ Client Application is prevented from changing the ClientID using the
* setClientID()
method in jakarta.jms.Connection
.
*
* The default value of this property is false
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqConfiguredClientID
* @see jakarta.jms.Connection#setClientID(java.lang.String) Connection.setClientID(clientID)
* @see jakarta.jms.Connection#getClientID() Connection.getClientID()
*/
public static final String imqDisableSetClientID = "imqDisableSetClientID";
/**
* This property holds the value of an administratively configured ClientID.
*
* The default value of this property is null
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqDisableSetClientID
* @see jakarta.jms.Connection#setClientID(java.lang.String) Connection.setClientID(clientID)
* @see jakarta.jms.Connection#getClientID() Connection.getClientID()
*/
public static final String imqConfiguredClientID = "imqConfiguredClientID";
/**
* This property indicates whether the client identifier requested and used by this connection is to be acquired in
* 'shared' mode.
*
* When a client identifier is used in 'shared' mode, other connections are also allowed to use the same client
* identifier.
*
* If a client identifier is already in use in 'unique' mode, then a request to set a client identifier in 'shared' mode
* will fail. Likewise, if a client identifier is already in use in 'shared' mode, then a request to set a client
* identifier in 'unique' mode will fail.
*
* The default value of this property is false
- i.e. a client identifier will be requested in
* 'unique' mode.
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqConfiguredClientID
* @see com.sun.messaging.ConnectionConfiguration#imqDisableSetClientID
* @see jakarta.jms.Connection#setClientID(java.lang.String) Connection.setClientID(clientID)
* @see jakarta.jms.Connection#getClientID() Connection.getClientID()
*/
public static final String imqEnableSharedClientID = "imqEnableSharedClientID";
/**
* This property is used to control the reliability of every message that is produced by a MessageProducer.
*
* The value of this property is used only when it is set. If the property is left unset, (the default behavior), the
* OpenMQ Client will ensure that every PERSISTENT message that is produced has been received by the OpenMQ Message
* Service before returning from the send()
and publish()
methods.
*
* If this property is set to true
, then the MQ Client will always wait until it ensures that
* the message has been reecived by the MQ Message Service before returning from the send()
and
* publish()
methods.
*
* If this property is set to false
, then the MQ Client will not wait until it ensures that
* the message has been reecived by the MQ Message Service before returning from the send()
and
* publish()
methods.
*
* When this property is used, it applies to all messages and not just the PERSISTENT messages.
*
* The value of this property is not set by default.
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqAckOnAcknowledge
*/
public static final String imqAckOnProduce = "imqAckOnProduce";
/**
* This property is used to control the reliability of message acknowledgement for every message that is consumed by a
* MessageConsumer.
*
* The value of this property is used only when it is set. If the property is left unset, (the default behavior), the
* OpenMQ Client will ensure that the acknowledgment for every PERSISTENT message that is consumed, has been received by
* the OpenMQ Message Service before the appropriate method returns - i.e. either the Message.acknowledge()
* method in the CLIENT_ACKNOWLEDGE
mode or the receive()
and/or onMessage()
* methods in the AUTO_ACKNOWLEDGE
and DUPS_OK_ACKNOWLEDGE
modes and transacted Sessions.
*
* If this property is set to true
, then the MQ Client will always wait until it ensures that
* the acknowledgement for the message has been reecived by the MQ Message Service before returning from the
* send()
and publish()
methods.
*
* If this property is set to false
, then the MQ Client will not wait until it ensures that
* the acknowledgement for the message has been reecived by the MQ Message Service before returning from the appropriate
* method that is responsible for sending the acknowledgement - i.e. either the Message.acknowledge()
* method in the CLIENT_ACKNOWLEDGE
mode or the receive()
and/or onMessage()
* methods in the AUTO_ACKNOWLEDGE
and DUPS_OK_ACKNOWLEDGE
modes and transacted Sessions.
*
* The value of this property is not set by default.
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqAckOnProduce
*/
public static final String imqAckOnAcknowledge = "imqAckOnAcknowledge";
/**
* This property specifies the upper limit of the number of messages per consumer that will be delivered and buffered in
* the MQ client. When the number of JMS messages delivered to a consumer reaches this limit, message delivery for that
* consumer stops. Message delivery is only resumed when the number of unconsumed messages for that consumer drops below
* the value determined using imqConsumerFlowThreshold
property.
*
* When a consumer is created, JMS messages for that consumer will begin to flow to the connection on which the consumer
* has been established. When the number of messages delivered to the consumer reaches the limit set by
* imqConsumerFlowLimit
, delivery of messages is stopped. As messages are consumed, the number of
* unconsumed messages buffered in the MQ client drops.
*
* When the number of unconsumed messages drops below the number determined by using
* imqConsumerFlowThreshold
, as a percentage of imqConsumerFlowLimit
, MQ resumes delivery of
* messages to that consumer until the number of messages delivered to that consumer reaches the limit set by
* imqConsumerFlowLimit
.
*
* Note that the actual value of the message flow limit used by MQ for each consumer is the lower of two values - the
* imqConsumerFlowLimit
set on the ConnectionFactory and the consumerFlowLimit
value set on
* the physical destination that the consumer is established on.
*
* The default value for this property is 1000
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqConsumerFlowThreshold
*/
public static final String imqConsumerFlowLimit = "imqConsumerFlowLimit";
/**
* This property controls when JMS message delivery will resume to consumers that have had their message delivery
* stopped due to the number of messages buffered in the MQ client exceeding the limit set by
* imqConsumerFlowLimit
and is expressed as a percentage of imqConsumerFlowLimit
.
*
* See the description for the imqConsumerFlowLimit
property for how the two properties are used together
* to meter the flow of JMS messages to individual consumers.
*
* The default value for this property is 50
(percent).
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqConsumerFlowLimit
*/
public static final String imqConsumerFlowThreshold = "imqConsumerFlowThreshold";
/**
* When this property is set to false, there will be no prefetch next message (except the first message) from broker to
* a consumer, and consumer flow control properties imqConsumerFlowLimit
and imqConsumerFlowThreshold
*
will be ignored. When it is set to true, the default, prefetch will take place as defined by consumer flow
* control properties.
*
* @see com.sun.messaging.ConnectionConfiguration#imqConsumerFlowLimit
* @see com.sun.messaging.ConnectionConfiguration#imqConsumerFlowThreshold
*/
public static final String imqConsumerFlowLimitPrefetch = "imqConsumerFlowLimitPrefetch";
/**
* This property manages the number of JMS messages that will flow from the OpenMQ Message Service to the MQ Client
* between each 'resume flow' notification from the Client to the Message Service to receive additional JMS messages.
*
* If the count is set to 0
then the Message Service will not restrict the number of JMS
* messages sent to the Client.
*
* After the Message Service has sent the number of JMS messages specified by this property, it will await a 'resume
* flow' notification from the Client before sending the next set of JMS messages. A non-zero value allows the Client to
* receive and process control messages from the Message Service even if there is a large number of JMS messages being
* held by the Message Service for delivery to this Client.
*
* The MQ Client further has the ability to limit this JMS message flow using the property
* imqConnectionFlowEnabled
*
* The default value for this property is 100
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqConnectionFlowLimitEnabled
* @see com.sun.messaging.ConnectionConfiguration#imqConnectionFlowLimit
*/
public static final String imqConnectionFlowCount = "imqConnectionFlowCount";
/**
* This property indicates whether the OpenMQ Client should limit the flow of JMS messages from the OpenMQ Message
* Service using the number of messages specified in imqConnectionFlowLimit
.
*
* This property will only be active if the property imqConnectionFlowCount
has been set to a non-zero
* value.
*
* If this property is set to true
the Client will use the property imqConnectionFlowLimit
as
* described below. Flow control will not be limited if this property is set to false
.
*
* The default value for this property is false
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqConnectionFlowCount
* @see com.sun.messaging.ConnectionConfiguration#imqConnectionFlowLimit
*/
public static final String imqConnectionFlowLimitEnabled = "imqConnectionFlowLimitEnabled";
/**
* This property specifies the number of uncomsumed JMS messages that a OpenMQ Client can hold, above which the MQ
* Client will refrain from sending a 'resume flow' notification to the OpenMQ Message Service. This property is active
* only when the imqFlowControlEnabled
property is set to true
and the
* imqFlowControlCount
property is set to a non-zero value.
*
* The MQ Client will notify the OpenMQ Message Service ('resume flow') and receive JMS message (in chunks determined by
* imqFlowControlCount
) until the number of received and uncomsumed JMS messages exceeds the value of this
* property. At this point, the Client will 'pause' until the JMS messages are consumed and when the unconsumed JMS
* message count drops below this value, the Client once again notifys the Message Service and the flow of JMS messages
* to the Client is resumed.
*
* The default value for this property is 1000
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqConnectionFlowLimitEnabled
* @see com.sun.messaging.ConnectionConfiguration#imqConnectionFlowCount
*/
public static final String imqConnectionFlowLimit = "imqConnectionFlowLimit";
/**
* For AUTO_ACKNOWLEDGE and DUPS_OK_ACKNOWLEDGE, maximum redelivery attempts (>= 1) when a
* jakarta.jms.MessageListener.onMessage() throws RuntimeException. The message will be sent to DMQ if this maximum
* atttempts (> 1) reached.
*
* This property is ignored if the MessageListener is Message Driven Bean
*
* The default value for this property is 1
.
*
*/
public static final String imqOnMessageExceptionRedeliveryAttempts = "imqOnMessageExceptionRedeliveryAttempts";
/**
* For AUTO_ACKNOWLEDGE and DUPS_OK_ACKNOWLEDGE, the interval in milliseconds for each subsequent redelivery attempt
* after first attempt when a jakarta.jms.MessageListener.onMessage() throws RuntimeException.
*
* This property is ignored if the MessageListener is Message Driven Bean
*
* The default value for this property is 500
milliseconds.
*
*/
public static final String imqOnMessageExceptionRedeliveryIntervals = "imqOnMessageExceptionRedeliveryIntervals";
/**
* This property holds the the maximum time, in milliseconds, that a OpenMQ Client Application will wait before throwing
* a NoSuchElementException when retrieving elements from a QueueBrowser Enumeration.
*
* The default value for this property is 60000
milliseconds.
*
*
* @see java.util.Enumeration
* @see java.util.Enumeration#nextElement()
* @see java.util.NoSuchElementException
* @see jakarta.jms.QueueBrowser jakarta.jms.QueueBrowser
*/
public static final String imqQueueBrowserRetrieveTimeout = "imqQueueBrowserRetrieveTimeout";
/**
* This property holds the the maximum number of messages that will be retrieved at one time when a OpenMQ Client
* Application is enumerating through the messages on a Queue using a QueueBrowser.
*
* The default value for this property is 1000
*
*
* @see java.util.Enumeration
* @see java.util.Enumeration#nextElement()
* @see jakarta.jms.QueueBrowser jakarta.jms.QueueBrowser
*/
public static final String imqQueueBrowserMaxMessagesPerRetrieve = "imqQueueBrowserMaxMessagesPerRetrieve";
/**
* This property indicates how the OpenMQ ConnectionConsumer should load messages into a ServerSession's JMS Session.
*
* When set to true
, OpenMQ will load as many messages as are available, upto the limit of the
* maxMessages
parameter used when creating the ConnectionConsumer, using the
* createConnectionConsumer()
API, into a ServerSession's JMS Session for processing.
*
* When set to false
, OpenMQ will only load a single message into a ServerSession's JMS Session for
* processing.
*
* The default value of this property is true
*
*
* @see jakarta.jms.ConnectionConsumer jakarta.jms.ConnectionConsumer
* @see jakarta.jms.QueueConnection#createConnectionConsumer(jakarta.jms.Queue, java.lang.String,
* jakarta.jms.ServerSessionPool, int) QueueConnection.createConnectionConsumer(Queue, messageSelector, ServerSessionPool,
* maxMessages)
* @see jakarta.jms.TopicConnection#createConnectionConsumer(jakarta.jms.Topic, java.lang.String,
* jakarta.jms.ServerSessionPool, int) TopicConnection.createConnectionConsumer(Topic, messageSelector, ServerSessionPool,
* maxMessages)
* @see jakarta.jms.TopicConnection#createDurableConnectionConsumer(jakarta.jms.Topic, java.lang.String, java.lang.String,
* jakarta.jms.ServerSessionPool, int) TopicConnection.createDurableConnectionConsumer(Topic, subscriptionName,
* messageSelector, ServerSessionPool, maxMessages)
*/
public static final String imqLoadMaxToServerSession = "imqLoadMaxToServerSession";
/**
* This property indicates whether OpenMQ should override the JMS Message Header JMSDeliveryMode
* which can be set using the JMS APIs.
*
* When this property is set to true
OpenMQ will set the Message Header JMSDeliveryMode
* to the value of the property imqJMSDeliveryMode
for all messages produced by the Connection
* created using this Administered Object. If imqJMSDeliveryMode
has an invalid value, then the
* default value of the JMS Message Header JMSDeliveryMode
will be used instead. value.
*
* The default value of this property is false
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqJMSDeliveryMode
* @see jakarta.jms.MessageProducer#setDeliveryMode(int) MessageProducer.setDeliveryMode(deliveryMode)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Message, int, int, long) QueueSender.send(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Queue, jakarta.jms.Message, int, int, long) QueueSender.send(Queue, Message, deliveryMode,
* priority, timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Message, int, int, long) QueueSender.publish(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Topic, jakarta.jms.Message, int, int, long) QueueSender.publish(Topic, Message,
* deliveryMode, priority, timeToLive)
*/
public static final String imqOverrideJMSDeliveryMode = "imqOverrideJMSDeliveryMode";
/**
* This property holds the administratively configured value of the JMS Message Header
* JMSDeliveryMode
.
*
* When imqOverrideJMSDeliveryMode
is set to true
, all messages produced to
* non-Temporary Destinations by the Connection created using this Administered Object will have their JMS
* Message Header JMSDeliveryMode
set to the value of this property.
*
* In addition, when imqOverrideJMSHeadersToTemporaryDestinations
is set to true
, all
* messages produced to Temporary Destinations will also contain the overridenvalue of this JMS Message
* Header.
*
* Setting an invalid value on this property will result in the Message Header being set to the default value of
* JMSDeliveryMode
as defined by the JMS Specification.
*
* The default value of this property is null
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqOverrideJMSDeliveryMode
* @see com.sun.messaging.ConnectionConfiguration#JMSDeliveryMode_PERSISTENT
* @see com.sun.messaging.ConnectionConfiguration#JMSDeliveryMode_NON_PERSISTENT
* @see jakarta.jms.Message#DEFAULT_DELIVERY_MODE
* @see jakarta.jms.DeliveryMode#PERSISTENT
* @see jakarta.jms.DeliveryMode#NON_PERSISTENT
* @see jakarta.jms.MessageProducer#setDeliveryMode(int) MessageProducer.setDeliveryMode(deliveryMode)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Message, int, int, long) QueueSender.send(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Queue, jakarta.jms.Message, int, int, long) QueueSender.send(Queue, Message, deliveryMode,
* priority, timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Message, int, int, long) QueueSender.publish(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Topic, jakarta.jms.Message, int, int, long) QueueSender.publish(Topic, Message,
* deliveryMode, priority, timeToLive)
*/
public static final String imqJMSDeliveryMode = "imqJMSDeliveryMode";
/**
* This constant is used to set the value of imqJMSDeliveryMode
to PERSISTENT when the
* JMSDeliveryMode JMS Message Header is administratively configured.
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqOverrideJMSDeliveryMode
* @see com.sun.messaging.ConnectionConfiguration#imqJMSDeliveryMode
*/
public static final String JMSDeliveryMode_PERSISTENT = "PERSISTENT";
/**
* This constant is used to set the value of imqJMSDeliveryMode
to NON_PERSISTENT when the
* JMSDeliveryMode JMS Message Header is administratively configured.
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqOverrideJMSDeliveryMode
* @see com.sun.messaging.ConnectionConfiguration#imqJMSDeliveryMode
*/
public static final String JMSDeliveryMode_NON_PERSISTENT = "NON_PERSISTENT";
/**
* This property indicates whether OpenMQ should override the JMS Message Header JMSExpiration
which
* can be set using the JMS APIs.
*
* When this property is set to true
OpenMQ will set the Message Header JMSExpiration
* to the value of the property imqJMSExpiration
for all messages produced by the Connection created
* using this Administered Object. If imqJMSExpiration
has an invalid value, then the default value
* of the JMS Message Header JMSExpiration
will be used instead. value.
*
* The default value of this property is false
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqJMSExpiration
* @see jakarta.jms.MessageProducer#setTimeToLive(long) MessageProducer.setTimeToLive(timeToLive)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Message, int, int, long) QueueSender.send(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Queue, jakarta.jms.Message, int, int, long) QueueSender.send(Queue, Message, deliveryMode,
* priority, timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Message, int, int, long) QueueSender.publish(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Topic, jakarta.jms.Message, int, int, long) QueueSender.publish(Topic, Message,
* deliveryMode, priority, timeToLive)
*/
public static final String imqOverrideJMSExpiration = "imqOverrideJMSExpiration";
/**
* This property holds the administratively configured value of the JMS Message Header
* JMSExpiration
.
*
* When imqOverrideJMSExpiration
is set to true
, all messages produced to
* non-Temporary Destinations by the Connection created using this Administered Object will have their JMS
* Message Header JMSExpiration
set to the value of this property.
*
* In addition, when imqOverrideJMSHeadersToTemporaryDestinations
is set to true
, all
* messages produced to Temporary Destinations will also contain the overridenvalue of this JMS Message
* Header.
*
* Setting an invalid value on this property will result in the Message Header being set to the default value of
* JMSExpiration
as defined by the JMS Specification.
*
* The JMS Specification defines JMSExpiration
as the time in milliseconds from its dispatch time
* that a produced message should be retained by the message service. An value of 0
indicates that
* the message is retained indefinitely. The default value of JMSExpiration
is
* 0
.
*
* The default value of this property is null
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqOverrideJMSExpiration
* @see jakarta.jms.Message#DEFAULT_TIME_TO_LIVE
* @see jakarta.jms.MessageProducer#setTimeToLive(long) MessageProducer.setTimeToLive(timeToLive)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Message, int, int, long) QueueSender.send(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Queue, jakarta.jms.Message, int, int, long) QueueSender.send(Queue, Message, deliveryMode,
* priority, timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Message, int, int, long) QueueSender.publish(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Topic, jakarta.jms.Message, int, int, long) QueueSender.publish(Topic, Message,
* deliveryMode, priority, timeToLive)
*/
public static final String imqJMSExpiration = "imqJMSExpiration";
/**
* This property indicates whether OpenMQ should override the JMS Message Header JMSPriority
which
* can be set using the JMS APIs.
*
* When this property is set to true
OpenMQ will set the Message Header JMSPriority
to
* the value of the property imqJMSPriority
for all messages produced by all messages produced to
* non-Temporary Destinations by the Connection created using this Administered Object. If
* imqJMSPriority
has an invalid value, then the default value of the JMS Message Header
* JMSPriority
will be used instead. value.
*
* The default value of this property is false
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqJMSPriority
* @see jakarta.jms.MessageProducer#setPriority(int) MessageProducer.setPriority(priority)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Message, int, int, long) QueueSender.send(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Queue, jakarta.jms.Message, int, int, long) QueueSender.send(Queue, Message, deliveryMode,
* priority, timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Message, int, int, long) QueueSender.publish(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Topic, jakarta.jms.Message, int, int, long) QueueSender.publish(Topic, Message,
* deliveryMode, priority, timeToLive)
* @see jakarta.jms.DeliveryMode#PERSISTENT
* @see jakarta.jms.DeliveryMode#NON_PERSISTENT
*/
public static final String imqOverrideJMSPriority = "imqOverrideJMSPriority";
/**
* This property holds the administratively configured value of the JMS Message Header JMSPriority
.
*
* When imqOverrideJMSPriority
is set to true
, all messages produced to
* non-Temporary Destinations by the Connection created using this Administered Object will have their JMS
* Message Header JMSPriority
set to the value of this property.
*
* In addition, when imqOverrideJMSHeadersToTemporaryDestinations
is set to true
, all
* messages produced to Temporary Destinations will also contain the overridenvalue of this JMS Message
* Header.
*
* Setting an invalid value on this property will result in the Message Header being set to the default value of
* JMSPriority
as defined by the JMS Specification.
*
* The JMS Specification defines a 10 level value for JMSPriority
, the priority with which produced
* messages are delivered by the message service, with 0 as the lowest and 9 as the highest. Clients should consider 0-4
* as gradients of normal priority and 5-9 as gradients of expedited priority. JMSPriority
is set to
* 4, by default.
*
* The default value of this property is null
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqOverrideJMSPriority
* @see jakarta.jms.Message#DEFAULT_PRIORITY
* @see jakarta.jms.MessageProducer#setPriority(int) MessageProducer.setPriority(priority)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Message, int, int, long) QueueSender.send(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Queue, jakarta.jms.Message, int, int, long) QueueSender.send(Queue, Message, deliveryMode,
* priority, timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Message, int, int, long) QueueSender.publish(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Topic, jakarta.jms.Message, int, int, long) QueueSender.publish(Topic, Message,
* deliveryMode, priority, timeToLive)
*/
public static final String imqJMSPriority = "imqJMSPriority";
/**
* This property indicates whether OpenMQ should override the JMS Message Headers on Messages that are sent to Temporary
* Destinations.
*
* When imqOverrideJMSHeadersToTemporaryDestinations
is set to true
, imq will override
* the JMS Message Headers listed below on Messages sent to Temporary Destinations providing the corresponding property
* enabling the JMS Message Header override on Messages sent to non-temporary Destinations is also set to
* true
.
*
* JMSPriority
*
* JMSExpiration
*
* JMSDeliveryMode
*
* The default value of this property is false
*
*
* @see com.sun.messaging.ConnectionConfiguration#imqOverrideJMSDeliveryMode
* @see com.sun.messaging.ConnectionConfiguration#imqJMSDeliveryMode
* @see com.sun.messaging.ConnectionConfiguration#imqOverrideJMSExpiration
* @see com.sun.messaging.ConnectionConfiguration#imqJMSExpiration
* @see com.sun.messaging.ConnectionConfiguration#imqOverrideJMSPriority
* @see com.sun.messaging.ConnectionConfiguration#imqJMSPriority
* @see jakarta.jms.MessageProducer#setDeliveryMode(int) MessageProducer.setDeliveryMode(deliveryMode)
* @see jakarta.jms.MessageProducer#setTimeToLive(long) MessageProducer.setTimeToLive(timeToLive)
* @see jakarta.jms.MessageProducer#setPriority(int) MessageProducer.setPriority(priority)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Message, int, int, long) QueueSender.send(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.QueueSender#send(jakarta.jms.Queue, jakarta.jms.Message, int, int, long) QueueSender.send(Queue, Message, deliveryMode,
* priority, timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Message, int, int, long) QueueSender.publish(Message, deliveryMode, priority,
* timeToLive)
* @see jakarta.jms.TopicPublisher#publish(jakarta.jms.Topic, jakarta.jms.Message, int, int, long) QueueSender.publish(Topic, Message,
* deliveryMode, priority, timeToLive)
* @see jakarta.jms.DeliveryMode#PERSISTENT
* @see jakarta.jms.DeliveryMode#NON_PERSISTENT
*/
public static final String imqOverrideJMSHeadersToTemporaryDestinations = "imqOverrideJMSHeadersToTemporaryDestinations";
}