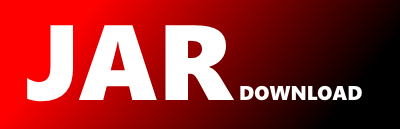
com.sun.messaging.jmq.util.RuntimeFaultInjection Maven / Gradle / Ivy
/*
* Copyright (c) 2000, 2017 Oracle and/or its affiliates. All rights reserved.
* Copyright (c) 2020 Payara Services Ltd.
* Copyright (c) 2021 Contributors to the Eclipse Foundation
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package com.sun.messaging.jmq.util;
import java.io.*;
import java.util.*;
import java.lang.reflect.*;
import com.sun.messaging.jmq.util.selector.*;
public abstract class RuntimeFaultInjection {
private LoggerWrapper logger = null;
private java.util.logging.Logger jlogger = null;
private Set injections = null;
private Map injectionSelectors = null;
private Map injectionProps = null;
private String shutdownMsg = "SHUTING DOWN BECAUSE OF" + " FAULT ";
private String haltMsg = "HALT BECAUSE OF" + " FAULT ";
public boolean FAULT_INJECTION = false;
/**
* @param name the process name
*/
public void setProcessName(String name) {
shutdownMsg = "SHUTING DOWN " + name + " BECAUSE OF" + " FAULT ";
haltMsg = "HALT " + name + " BECAUSE OF" + " FAULT ";
}
public RuntimeFaultInjection() {
injections = Collections.synchronizedSet(new HashSet<>());
injectionSelectors = Collections.synchronizedMap(new HashMap<>());
injectionProps = Collections.synchronizedMap(new HashMap<>());
}
protected void setLogger(Object l) {
if (l instanceof com.sun.messaging.jmq.util.LoggerWrapper) {
logger = (LoggerWrapper) l;
} else if (l instanceof java.util.logging.Logger) {
jlogger = (java.util.logging.Logger) l;
}
}
public void setFault(String fault, String selector) throws SelectorFormatException {
setFault(fault, selector, null);
}
public void setFault(String fault, String selector, Map props) throws SelectorFormatException {
logInfo("Setting Fault " + fault + "[ selector=" + selector + "], [props=" + props + "]");
injections.add(fault);
if (selector != null && selector.length() != 0) {
// create a selector and insert
Selector s = Selector.compile(selector);
injectionSelectors.put(fault, s);
}
if (props != null) {
injectionProps.put(fault, props);
}
}
public void unsetFault(String fault) {
logInfo("Removing Fault " + fault);
injections.remove(fault);
injectionSelectors.remove(fault);
injectionProps.remove(fault);
}
static class FaultInjectionException extends Exception {
private static final long serialVersionUID = -4916449065238440067L;
@Override
public String toString() {
return "FaultInjectionTrace";
}
}
public void setFaultInjection(boolean inject) {
if (FAULT_INJECTION != inject) {
if (inject) {
logInfo("Turning on Fault Injection");
} else {
logInfo("Turning off Fault Injection");
}
FAULT_INJECTION = inject;
}
}
private void logInjection(String fault, Selector sel) {
String str = "Fault Injection: triggered " + fault;
if (sel != null) {
str += " selector [ " + sel + "]";
}
Exception ex = new FaultInjectionException();
ex.fillInStackTrace();
logInfo(str, ex);
}
private Map checkFaultGetProps(String fault, Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy