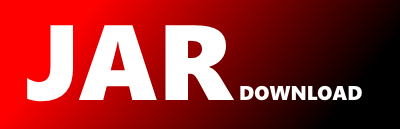
org.glowroot.instrumentation.engine.config.ImmutableAdviceConfig Maven / Gradle / Ivy
package org.glowroot.instrumentation.engine.config;
import org.glowroot.instrumentation.test.harness.shaded.com.google.common.base.MoreObjects;
import org.glowroot.instrumentation.test.harness.shaded.com.google.common.base.Objects;
import org.glowroot.instrumentation.test.harness.shaded.com.google.common.base.Preconditions;
import org.glowroot.instrumentation.test.harness.shaded.com.google.common.collect.ImmutableList;
import org.glowroot.instrumentation.test.harness.shaded.com.google.common.collect.ImmutableMap;
import org.glowroot.instrumentation.test.harness.shaded.com.google.common.primitives.Booleans;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.glowroot.instrumentation.api.Descriptor;
import org.glowroot.instrumentation.api.OptionalThreadContext;
import org.glowroot.instrumentation.api.weaving.Advice;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link AdviceConfig}.
*
* Use the builder to create immutable instances:
* {@code ImmutableAdviceConfig.builder()}.
*/
@Generated(from = "AdviceConfig", generator = "Immutables")
@SuppressWarnings({"all", "deprecation"})
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
public final class ImmutableAdviceConfig extends AdviceConfig {
private final String className;
private final String classAnnotation;
private final String subTypeRestriction;
private final String superTypeRestriction;
private final String methodDeclaringClassName;
private final String methodName;
private final String methodAnnotation;
private final ImmutableList methodParameterTypes;
private final String methodReturnType;
private final ImmutableList methodModifiers;
private final String nestingGroup;
private final int order;
private final Descriptor.CaptureKind captureKind;
private final String transactionType;
private final String transactionNameTemplate;
private final String transactionUserTemplate;
private final ImmutableMap transactionAttributeTemplates;
private final @Nullable Integer transactionSlowThresholdMillis;
private final @Nullable OptionalThreadContext.AlreadyInTransactionBehavior alreadyInTransactionBehavior;
private transient final @Nullable OptionalThreadContext.AlreadyInTransactionBehavior alreadyInTransactionBehaviorCorrected;
private final String spanMessageTemplate;
private final @Nullable Integer spanStackThresholdMillis;
private final boolean spanCaptureSelfNested;
private final String timerName;
private final String enabledProperty;
private final String localSpanEnabledProperty;
private transient final boolean isTimerOrGreater;
private transient final boolean isLocalSpanOrGreater;
private transient final boolean isTransaction;
private transient final ImmutableList validationErrors;
private ImmutableAdviceConfig(ImmutableAdviceConfig.Builder builder) {
this.methodParameterTypes = builder.methodParameterTypes.build();
this.methodModifiers = builder.methodModifiers.build();
this.captureKind = builder.captureKind;
this.transactionAttributeTemplates = builder.transactionAttributeTemplates.build();
this.transactionSlowThresholdMillis = builder.transactionSlowThresholdMillis;
this.alreadyInTransactionBehavior = builder.alreadyInTransactionBehavior;
this.spanStackThresholdMillis = builder.spanStackThresholdMillis;
if (builder.className != null) {
initShim.className(builder.className);
}
if (builder.classAnnotation != null) {
initShim.classAnnotation(builder.classAnnotation);
}
if (builder.subTypeRestriction != null) {
initShim.subTypeRestriction(builder.subTypeRestriction);
}
if (builder.superTypeRestriction != null) {
initShim.superTypeRestriction(builder.superTypeRestriction);
}
if (builder.methodDeclaringClassName != null) {
initShim.methodDeclaringClassName(builder.methodDeclaringClassName);
}
if (builder.methodName != null) {
initShim.methodName(builder.methodName);
}
if (builder.methodAnnotation != null) {
initShim.methodAnnotation(builder.methodAnnotation);
}
if (builder.methodReturnType != null) {
initShim.methodReturnType(builder.methodReturnType);
}
if (builder.nestingGroup != null) {
initShim.nestingGroup(builder.nestingGroup);
}
if (builder.orderIsSet()) {
initShim.order(builder.order);
}
if (builder.transactionType != null) {
initShim.transactionType(builder.transactionType);
}
if (builder.transactionNameTemplate != null) {
initShim.transactionNameTemplate(builder.transactionNameTemplate);
}
if (builder.transactionUserTemplate != null) {
initShim.transactionUserTemplate(builder.transactionUserTemplate);
}
if (builder.spanMessageTemplate != null) {
initShim.spanMessageTemplate(builder.spanMessageTemplate);
}
if (builder.spanCaptureSelfNestedIsSet()) {
initShim.spanCaptureSelfNested(builder.spanCaptureSelfNested);
}
if (builder.timerName != null) {
initShim.timerName(builder.timerName);
}
if (builder.enabledProperty != null) {
initShim.enabledProperty(builder.enabledProperty);
}
if (builder.localSpanEnabledProperty != null) {
initShim.localSpanEnabledProperty(builder.localSpanEnabledProperty);
}
this.className = initShim.className();
this.classAnnotation = initShim.classAnnotation();
this.subTypeRestriction = initShim.subTypeRestriction();
this.superTypeRestriction = initShim.superTypeRestriction();
this.methodDeclaringClassName = initShim.methodDeclaringClassName();
this.methodName = initShim.methodName();
this.methodAnnotation = initShim.methodAnnotation();
this.methodReturnType = initShim.methodReturnType();
this.nestingGroup = initShim.nestingGroup();
this.order = initShim.order();
this.transactionType = initShim.transactionType();
this.transactionNameTemplate = initShim.transactionNameTemplate();
this.transactionUserTemplate = initShim.transactionUserTemplate();
this.alreadyInTransactionBehaviorCorrected = initShim.alreadyInTransactionBehaviorCorrected();
this.spanMessageTemplate = initShim.spanMessageTemplate();
this.spanCaptureSelfNested = initShim.spanCaptureSelfNested();
this.timerName = initShim.timerName();
this.enabledProperty = initShim.enabledProperty();
this.localSpanEnabledProperty = initShim.localSpanEnabledProperty();
this.isTimerOrGreater = initShim.isTimerOrGreater();
this.isLocalSpanOrGreater = initShim.isLocalSpanOrGreater();
this.isTransaction = initShim.isTransaction();
this.validationErrors = initShim.validationErrors();
this.initShim = null;
}
private ImmutableAdviceConfig(
String className,
String classAnnotation,
String subTypeRestriction,
String superTypeRestriction,
String methodDeclaringClassName,
String methodName,
String methodAnnotation,
ImmutableList methodParameterTypes,
String methodReturnType,
ImmutableList methodModifiers,
String nestingGroup,
int order,
Descriptor.CaptureKind captureKind,
String transactionType,
String transactionNameTemplate,
String transactionUserTemplate,
ImmutableMap transactionAttributeTemplates,
@Nullable Integer transactionSlowThresholdMillis,
@Nullable OptionalThreadContext.AlreadyInTransactionBehavior alreadyInTransactionBehavior,
String spanMessageTemplate,
@Nullable Integer spanStackThresholdMillis,
boolean spanCaptureSelfNested,
String timerName,
String enabledProperty,
String localSpanEnabledProperty) {
initShim.className(className);
initShim.classAnnotation(classAnnotation);
initShim.subTypeRestriction(subTypeRestriction);
initShim.superTypeRestriction(superTypeRestriction);
initShim.methodDeclaringClassName(methodDeclaringClassName);
initShim.methodName(methodName);
initShim.methodAnnotation(methodAnnotation);
this.methodParameterTypes = methodParameterTypes;
initShim.methodReturnType(methodReturnType);
this.methodModifiers = methodModifiers;
initShim.nestingGroup(nestingGroup);
initShim.order(order);
this.captureKind = captureKind;
initShim.transactionType(transactionType);
initShim.transactionNameTemplate(transactionNameTemplate);
initShim.transactionUserTemplate(transactionUserTemplate);
this.transactionAttributeTemplates = transactionAttributeTemplates;
this.transactionSlowThresholdMillis = transactionSlowThresholdMillis;
this.alreadyInTransactionBehavior = alreadyInTransactionBehavior;
initShim.spanMessageTemplate(spanMessageTemplate);
this.spanStackThresholdMillis = spanStackThresholdMillis;
initShim.spanCaptureSelfNested(spanCaptureSelfNested);
initShim.timerName(timerName);
initShim.enabledProperty(enabledProperty);
initShim.localSpanEnabledProperty(localSpanEnabledProperty);
this.className = initShim.className();
this.classAnnotation = initShim.classAnnotation();
this.subTypeRestriction = initShim.subTypeRestriction();
this.superTypeRestriction = initShim.superTypeRestriction();
this.methodDeclaringClassName = initShim.methodDeclaringClassName();
this.methodName = initShim.methodName();
this.methodAnnotation = initShim.methodAnnotation();
this.methodReturnType = initShim.methodReturnType();
this.nestingGroup = initShim.nestingGroup();
this.order = initShim.order();
this.transactionType = initShim.transactionType();
this.transactionNameTemplate = initShim.transactionNameTemplate();
this.transactionUserTemplate = initShim.transactionUserTemplate();
this.alreadyInTransactionBehaviorCorrected = initShim.alreadyInTransactionBehaviorCorrected();
this.spanMessageTemplate = initShim.spanMessageTemplate();
this.spanCaptureSelfNested = initShim.spanCaptureSelfNested();
this.timerName = initShim.timerName();
this.enabledProperty = initShim.enabledProperty();
this.localSpanEnabledProperty = initShim.localSpanEnabledProperty();
this.isTimerOrGreater = initShim.isTimerOrGreater();
this.isLocalSpanOrGreater = initShim.isLocalSpanOrGreater();
this.isTransaction = initShim.isTransaction();
this.validationErrors = initShim.validationErrors();
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "AdviceConfig", generator = "Immutables")
private final class InitShim {
private byte classNameBuildStage = STAGE_UNINITIALIZED;
private String className;
String className() {
if (classNameBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (classNameBuildStage == STAGE_UNINITIALIZED) {
classNameBuildStage = STAGE_INITIALIZING;
this.className = Preconditions.checkNotNull(ImmutableAdviceConfig.super.className(), "className");
classNameBuildStage = STAGE_INITIALIZED;
}
return this.className;
}
void className(String className) {
this.className = className;
classNameBuildStage = STAGE_INITIALIZED;
}
private byte classAnnotationBuildStage = STAGE_UNINITIALIZED;
private String classAnnotation;
String classAnnotation() {
if (classAnnotationBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (classAnnotationBuildStage == STAGE_UNINITIALIZED) {
classAnnotationBuildStage = STAGE_INITIALIZING;
this.classAnnotation = Preconditions.checkNotNull(ImmutableAdviceConfig.super.classAnnotation(), "classAnnotation");
classAnnotationBuildStage = STAGE_INITIALIZED;
}
return this.classAnnotation;
}
void classAnnotation(String classAnnotation) {
this.classAnnotation = classAnnotation;
classAnnotationBuildStage = STAGE_INITIALIZED;
}
private byte subTypeRestrictionBuildStage = STAGE_UNINITIALIZED;
private String subTypeRestriction;
String subTypeRestriction() {
if (subTypeRestrictionBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (subTypeRestrictionBuildStage == STAGE_UNINITIALIZED) {
subTypeRestrictionBuildStage = STAGE_INITIALIZING;
this.subTypeRestriction = Preconditions.checkNotNull(ImmutableAdviceConfig.super.subTypeRestriction(), "subTypeRestriction");
subTypeRestrictionBuildStage = STAGE_INITIALIZED;
}
return this.subTypeRestriction;
}
void subTypeRestriction(String subTypeRestriction) {
this.subTypeRestriction = subTypeRestriction;
subTypeRestrictionBuildStage = STAGE_INITIALIZED;
}
private byte superTypeRestrictionBuildStage = STAGE_UNINITIALIZED;
private String superTypeRestriction;
String superTypeRestriction() {
if (superTypeRestrictionBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (superTypeRestrictionBuildStage == STAGE_UNINITIALIZED) {
superTypeRestrictionBuildStage = STAGE_INITIALIZING;
this.superTypeRestriction = Preconditions.checkNotNull(ImmutableAdviceConfig.super.superTypeRestriction(), "superTypeRestriction");
superTypeRestrictionBuildStage = STAGE_INITIALIZED;
}
return this.superTypeRestriction;
}
void superTypeRestriction(String superTypeRestriction) {
this.superTypeRestriction = superTypeRestriction;
superTypeRestrictionBuildStage = STAGE_INITIALIZED;
}
private byte methodDeclaringClassNameBuildStage = STAGE_UNINITIALIZED;
private String methodDeclaringClassName;
String methodDeclaringClassName() {
if (methodDeclaringClassNameBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (methodDeclaringClassNameBuildStage == STAGE_UNINITIALIZED) {
methodDeclaringClassNameBuildStage = STAGE_INITIALIZING;
this.methodDeclaringClassName = Preconditions.checkNotNull(ImmutableAdviceConfig.super.methodDeclaringClassName(), "methodDeclaringClassName");
methodDeclaringClassNameBuildStage = STAGE_INITIALIZED;
}
return this.methodDeclaringClassName;
}
void methodDeclaringClassName(String methodDeclaringClassName) {
this.methodDeclaringClassName = methodDeclaringClassName;
methodDeclaringClassNameBuildStage = STAGE_INITIALIZED;
}
private byte methodNameBuildStage = STAGE_UNINITIALIZED;
private String methodName;
String methodName() {
if (methodNameBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (methodNameBuildStage == STAGE_UNINITIALIZED) {
methodNameBuildStage = STAGE_INITIALIZING;
this.methodName = Preconditions.checkNotNull(ImmutableAdviceConfig.super.methodName(), "methodName");
methodNameBuildStage = STAGE_INITIALIZED;
}
return this.methodName;
}
void methodName(String methodName) {
this.methodName = methodName;
methodNameBuildStage = STAGE_INITIALIZED;
}
private byte methodAnnotationBuildStage = STAGE_UNINITIALIZED;
private String methodAnnotation;
String methodAnnotation() {
if (methodAnnotationBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (methodAnnotationBuildStage == STAGE_UNINITIALIZED) {
methodAnnotationBuildStage = STAGE_INITIALIZING;
this.methodAnnotation = Preconditions.checkNotNull(ImmutableAdviceConfig.super.methodAnnotation(), "methodAnnotation");
methodAnnotationBuildStage = STAGE_INITIALIZED;
}
return this.methodAnnotation;
}
void methodAnnotation(String methodAnnotation) {
this.methodAnnotation = methodAnnotation;
methodAnnotationBuildStage = STAGE_INITIALIZED;
}
private byte methodReturnTypeBuildStage = STAGE_UNINITIALIZED;
private String methodReturnType;
String methodReturnType() {
if (methodReturnTypeBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (methodReturnTypeBuildStage == STAGE_UNINITIALIZED) {
methodReturnTypeBuildStage = STAGE_INITIALIZING;
this.methodReturnType = Preconditions.checkNotNull(ImmutableAdviceConfig.super.methodReturnType(), "methodReturnType");
methodReturnTypeBuildStage = STAGE_INITIALIZED;
}
return this.methodReturnType;
}
void methodReturnType(String methodReturnType) {
this.methodReturnType = methodReturnType;
methodReturnTypeBuildStage = STAGE_INITIALIZED;
}
private byte nestingGroupBuildStage = STAGE_UNINITIALIZED;
private String nestingGroup;
String nestingGroup() {
if (nestingGroupBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (nestingGroupBuildStage == STAGE_UNINITIALIZED) {
nestingGroupBuildStage = STAGE_INITIALIZING;
this.nestingGroup = Preconditions.checkNotNull(ImmutableAdviceConfig.super.nestingGroup(), "nestingGroup");
nestingGroupBuildStage = STAGE_INITIALIZED;
}
return this.nestingGroup;
}
void nestingGroup(String nestingGroup) {
this.nestingGroup = nestingGroup;
nestingGroupBuildStage = STAGE_INITIALIZED;
}
private byte orderBuildStage = STAGE_UNINITIALIZED;
private int order;
int order() {
if (orderBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (orderBuildStage == STAGE_UNINITIALIZED) {
orderBuildStage = STAGE_INITIALIZING;
this.order = ImmutableAdviceConfig.super.order();
orderBuildStage = STAGE_INITIALIZED;
}
return this.order;
}
void order(int order) {
this.order = order;
orderBuildStage = STAGE_INITIALIZED;
}
private byte transactionTypeBuildStage = STAGE_UNINITIALIZED;
private String transactionType;
String transactionType() {
if (transactionTypeBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (transactionTypeBuildStage == STAGE_UNINITIALIZED) {
transactionTypeBuildStage = STAGE_INITIALIZING;
this.transactionType = Preconditions.checkNotNull(ImmutableAdviceConfig.super.transactionType(), "transactionType");
transactionTypeBuildStage = STAGE_INITIALIZED;
}
return this.transactionType;
}
void transactionType(String transactionType) {
this.transactionType = transactionType;
transactionTypeBuildStage = STAGE_INITIALIZED;
}
private byte transactionNameTemplateBuildStage = STAGE_UNINITIALIZED;
private String transactionNameTemplate;
String transactionNameTemplate() {
if (transactionNameTemplateBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (transactionNameTemplateBuildStage == STAGE_UNINITIALIZED) {
transactionNameTemplateBuildStage = STAGE_INITIALIZING;
this.transactionNameTemplate = Preconditions.checkNotNull(ImmutableAdviceConfig.super.transactionNameTemplate(), "transactionNameTemplate");
transactionNameTemplateBuildStage = STAGE_INITIALIZED;
}
return this.transactionNameTemplate;
}
void transactionNameTemplate(String transactionNameTemplate) {
this.transactionNameTemplate = transactionNameTemplate;
transactionNameTemplateBuildStage = STAGE_INITIALIZED;
}
private byte transactionUserTemplateBuildStage = STAGE_UNINITIALIZED;
private String transactionUserTemplate;
String transactionUserTemplate() {
if (transactionUserTemplateBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (transactionUserTemplateBuildStage == STAGE_UNINITIALIZED) {
transactionUserTemplateBuildStage = STAGE_INITIALIZING;
this.transactionUserTemplate = Preconditions.checkNotNull(ImmutableAdviceConfig.super.transactionUserTemplate(), "transactionUserTemplate");
transactionUserTemplateBuildStage = STAGE_INITIALIZED;
}
return this.transactionUserTemplate;
}
void transactionUserTemplate(String transactionUserTemplate) {
this.transactionUserTemplate = transactionUserTemplate;
transactionUserTemplateBuildStage = STAGE_INITIALIZED;
}
private byte alreadyInTransactionBehaviorCorrectedBuildStage = STAGE_UNINITIALIZED;
private OptionalThreadContext.AlreadyInTransactionBehavior alreadyInTransactionBehaviorCorrected;
OptionalThreadContext.AlreadyInTransactionBehavior alreadyInTransactionBehaviorCorrected() {
if (alreadyInTransactionBehaviorCorrectedBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (alreadyInTransactionBehaviorCorrectedBuildStage == STAGE_UNINITIALIZED) {
alreadyInTransactionBehaviorCorrectedBuildStage = STAGE_INITIALIZING;
this.alreadyInTransactionBehaviorCorrected = ImmutableAdviceConfig.super.alreadyInTransactionBehaviorCorrected();
alreadyInTransactionBehaviorCorrectedBuildStage = STAGE_INITIALIZED;
}
return this.alreadyInTransactionBehaviorCorrected;
}
private byte spanMessageTemplateBuildStage = STAGE_UNINITIALIZED;
private String spanMessageTemplate;
String spanMessageTemplate() {
if (spanMessageTemplateBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (spanMessageTemplateBuildStage == STAGE_UNINITIALIZED) {
spanMessageTemplateBuildStage = STAGE_INITIALIZING;
this.spanMessageTemplate = Preconditions.checkNotNull(ImmutableAdviceConfig.super.spanMessageTemplate(), "spanMessageTemplate");
spanMessageTemplateBuildStage = STAGE_INITIALIZED;
}
return this.spanMessageTemplate;
}
void spanMessageTemplate(String spanMessageTemplate) {
this.spanMessageTemplate = spanMessageTemplate;
spanMessageTemplateBuildStage = STAGE_INITIALIZED;
}
private byte spanCaptureSelfNestedBuildStage = STAGE_UNINITIALIZED;
private boolean spanCaptureSelfNested;
boolean spanCaptureSelfNested() {
if (spanCaptureSelfNestedBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (spanCaptureSelfNestedBuildStage == STAGE_UNINITIALIZED) {
spanCaptureSelfNestedBuildStage = STAGE_INITIALIZING;
this.spanCaptureSelfNested = ImmutableAdviceConfig.super.spanCaptureSelfNested();
spanCaptureSelfNestedBuildStage = STAGE_INITIALIZED;
}
return this.spanCaptureSelfNested;
}
void spanCaptureSelfNested(boolean spanCaptureSelfNested) {
this.spanCaptureSelfNested = spanCaptureSelfNested;
spanCaptureSelfNestedBuildStage = STAGE_INITIALIZED;
}
private byte timerNameBuildStage = STAGE_UNINITIALIZED;
private String timerName;
String timerName() {
if (timerNameBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (timerNameBuildStage == STAGE_UNINITIALIZED) {
timerNameBuildStage = STAGE_INITIALIZING;
this.timerName = Preconditions.checkNotNull(ImmutableAdviceConfig.super.timerName(), "timerName");
timerNameBuildStage = STAGE_INITIALIZED;
}
return this.timerName;
}
void timerName(String timerName) {
this.timerName = timerName;
timerNameBuildStage = STAGE_INITIALIZED;
}
private byte enabledPropertyBuildStage = STAGE_UNINITIALIZED;
private String enabledProperty;
String enabledProperty() {
if (enabledPropertyBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (enabledPropertyBuildStage == STAGE_UNINITIALIZED) {
enabledPropertyBuildStage = STAGE_INITIALIZING;
this.enabledProperty = Preconditions.checkNotNull(ImmutableAdviceConfig.super.enabledProperty(), "enabledProperty");
enabledPropertyBuildStage = STAGE_INITIALIZED;
}
return this.enabledProperty;
}
void enabledProperty(String enabledProperty) {
this.enabledProperty = enabledProperty;
enabledPropertyBuildStage = STAGE_INITIALIZED;
}
private byte localSpanEnabledPropertyBuildStage = STAGE_UNINITIALIZED;
private String localSpanEnabledProperty;
String localSpanEnabledProperty() {
if (localSpanEnabledPropertyBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (localSpanEnabledPropertyBuildStage == STAGE_UNINITIALIZED) {
localSpanEnabledPropertyBuildStage = STAGE_INITIALIZING;
this.localSpanEnabledProperty = Preconditions.checkNotNull(ImmutableAdviceConfig.super.localSpanEnabledProperty(), "localSpanEnabledProperty");
localSpanEnabledPropertyBuildStage = STAGE_INITIALIZED;
}
return this.localSpanEnabledProperty;
}
void localSpanEnabledProperty(String localSpanEnabledProperty) {
this.localSpanEnabledProperty = localSpanEnabledProperty;
localSpanEnabledPropertyBuildStage = STAGE_INITIALIZED;
}
private byte isTimerOrGreaterBuildStage = STAGE_UNINITIALIZED;
private boolean isTimerOrGreater;
boolean isTimerOrGreater() {
if (isTimerOrGreaterBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (isTimerOrGreaterBuildStage == STAGE_UNINITIALIZED) {
isTimerOrGreaterBuildStage = STAGE_INITIALIZING;
this.isTimerOrGreater = ImmutableAdviceConfig.super.isTimerOrGreater();
isTimerOrGreaterBuildStage = STAGE_INITIALIZED;
}
return this.isTimerOrGreater;
}
private byte isLocalSpanOrGreaterBuildStage = STAGE_UNINITIALIZED;
private boolean isLocalSpanOrGreater;
boolean isLocalSpanOrGreater() {
if (isLocalSpanOrGreaterBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (isLocalSpanOrGreaterBuildStage == STAGE_UNINITIALIZED) {
isLocalSpanOrGreaterBuildStage = STAGE_INITIALIZING;
this.isLocalSpanOrGreater = ImmutableAdviceConfig.super.isLocalSpanOrGreater();
isLocalSpanOrGreaterBuildStage = STAGE_INITIALIZED;
}
return this.isLocalSpanOrGreater;
}
private byte isTransactionBuildStage = STAGE_UNINITIALIZED;
private boolean isTransaction;
boolean isTransaction() {
if (isTransactionBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (isTransactionBuildStage == STAGE_UNINITIALIZED) {
isTransactionBuildStage = STAGE_INITIALIZING;
this.isTransaction = ImmutableAdviceConfig.super.isTransaction();
isTransactionBuildStage = STAGE_INITIALIZED;
}
return this.isTransaction;
}
private byte validationErrorsBuildStage = STAGE_UNINITIALIZED;
private ImmutableList validationErrors;
ImmutableList validationErrors() {
if (validationErrorsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (validationErrorsBuildStage == STAGE_UNINITIALIZED) {
validationErrorsBuildStage = STAGE_INITIALIZING;
this.validationErrors = Preconditions.checkNotNull(ImmutableAdviceConfig.super.validationErrors(), "validationErrors");
validationErrorsBuildStage = STAGE_INITIALIZED;
}
return this.validationErrors;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList();
if (classNameBuildStage == STAGE_INITIALIZING) attributes.add("className");
if (classAnnotationBuildStage == STAGE_INITIALIZING) attributes.add("classAnnotation");
if (subTypeRestrictionBuildStage == STAGE_INITIALIZING) attributes.add("subTypeRestriction");
if (superTypeRestrictionBuildStage == STAGE_INITIALIZING) attributes.add("superTypeRestriction");
if (methodDeclaringClassNameBuildStage == STAGE_INITIALIZING) attributes.add("methodDeclaringClassName");
if (methodNameBuildStage == STAGE_INITIALIZING) attributes.add("methodName");
if (methodAnnotationBuildStage == STAGE_INITIALIZING) attributes.add("methodAnnotation");
if (methodReturnTypeBuildStage == STAGE_INITIALIZING) attributes.add("methodReturnType");
if (nestingGroupBuildStage == STAGE_INITIALIZING) attributes.add("nestingGroup");
if (orderBuildStage == STAGE_INITIALIZING) attributes.add("order");
if (transactionTypeBuildStage == STAGE_INITIALIZING) attributes.add("transactionType");
if (transactionNameTemplateBuildStage == STAGE_INITIALIZING) attributes.add("transactionNameTemplate");
if (transactionUserTemplateBuildStage == STAGE_INITIALIZING) attributes.add("transactionUserTemplate");
if (alreadyInTransactionBehaviorCorrectedBuildStage == STAGE_INITIALIZING) attributes.add("alreadyInTransactionBehaviorCorrected");
if (spanMessageTemplateBuildStage == STAGE_INITIALIZING) attributes.add("spanMessageTemplate");
if (spanCaptureSelfNestedBuildStage == STAGE_INITIALIZING) attributes.add("spanCaptureSelfNested");
if (timerNameBuildStage == STAGE_INITIALIZING) attributes.add("timerName");
if (enabledPropertyBuildStage == STAGE_INITIALIZING) attributes.add("enabledProperty");
if (localSpanEnabledPropertyBuildStage == STAGE_INITIALIZING) attributes.add("localSpanEnabledProperty");
if (isTimerOrGreaterBuildStage == STAGE_INITIALIZING) attributes.add("isTimerOrGreater");
if (isLocalSpanOrGreaterBuildStage == STAGE_INITIALIZING) attributes.add("isLocalSpanOrGreater");
if (isTransactionBuildStage == STAGE_INITIALIZING) attributes.add("isTransaction");
if (validationErrorsBuildStage == STAGE_INITIALIZING) attributes.add("validationErrors");
return "Cannot build AdviceConfig, attribute initializers form cycle " + attributes;
}
}
/**
* @return The value of the {@code className} attribute
*/
@Override
public String className() {
InitShim shim = this.initShim;
return shim != null
? shim.className()
: this.className;
}
/**
* @return The value of the {@code classAnnotation} attribute
*/
@Override
public String classAnnotation() {
InitShim shim = this.initShim;
return shim != null
? shim.classAnnotation()
: this.classAnnotation;
}
/**
* @return The value of the {@code subTypeRestriction} attribute
*/
@Override
public String subTypeRestriction() {
InitShim shim = this.initShim;
return shim != null
? shim.subTypeRestriction()
: this.subTypeRestriction;
}
/**
* @return The value of the {@code superTypeRestriction} attribute
*/
@Override
public String superTypeRestriction() {
InitShim shim = this.initShim;
return shim != null
? shim.superTypeRestriction()
: this.superTypeRestriction;
}
/**
* @return The value of the {@code methodDeclaringClassName} attribute
*/
@Deprecated
@Override
public String methodDeclaringClassName() {
InitShim shim = this.initShim;
return shim != null
? shim.methodDeclaringClassName()
: this.methodDeclaringClassName;
}
/**
* @return The value of the {@code methodName} attribute
*/
@Override
public String methodName() {
InitShim shim = this.initShim;
return shim != null
? shim.methodName()
: this.methodName;
}
/**
* @return The value of the {@code methodAnnotation} attribute
*/
@Override
public String methodAnnotation() {
InitShim shim = this.initShim;
return shim != null
? shim.methodAnnotation()
: this.methodAnnotation;
}
/**
* @return The value of the {@code methodParameterTypes} attribute
*/
@Override
public ImmutableList methodParameterTypes() {
return methodParameterTypes;
}
/**
* @return The value of the {@code methodReturnType} attribute
*/
@Override
public String methodReturnType() {
InitShim shim = this.initShim;
return shim != null
? shim.methodReturnType()
: this.methodReturnType;
}
/**
* @return The value of the {@code methodModifiers} attribute
*/
@Override
public ImmutableList methodModifiers() {
return methodModifiers;
}
/**
* @return The value of the {@code nestingGroup} attribute
*/
@Override
public String nestingGroup() {
InitShim shim = this.initShim;
return shim != null
? shim.nestingGroup()
: this.nestingGroup;
}
/**
* @return The value of the {@code order} attribute
*/
@Override
public int order() {
InitShim shim = this.initShim;
return shim != null
? shim.order()
: this.order;
}
/**
* @return The value of the {@code captureKind} attribute
*/
@Override
public Descriptor.CaptureKind captureKind() {
return captureKind;
}
/**
* @return The value of the {@code transactionType} attribute
*/
@Override
public String transactionType() {
InitShim shim = this.initShim;
return shim != null
? shim.transactionType()
: this.transactionType;
}
/**
* @return The value of the {@code transactionNameTemplate} attribute
*/
@Override
public String transactionNameTemplate() {
InitShim shim = this.initShim;
return shim != null
? shim.transactionNameTemplate()
: this.transactionNameTemplate;
}
/**
* @return The value of the {@code transactionUserTemplate} attribute
*/
@Override
public String transactionUserTemplate() {
InitShim shim = this.initShim;
return shim != null
? shim.transactionUserTemplate()
: this.transactionUserTemplate;
}
/**
* @return The value of the {@code transactionAttributeTemplates} attribute
*/
@Override
public ImmutableMap transactionAttributeTemplates() {
return transactionAttributeTemplates;
}
/**
* @return The value of the {@code transactionSlowThresholdMillis} attribute
*/
@Override
public @Nullable Integer transactionSlowThresholdMillis() {
return transactionSlowThresholdMillis;
}
/**
* @return The value of the {@code alreadyInTransactionBehavior} attribute
*/
@Override
public @Nullable OptionalThreadContext.AlreadyInTransactionBehavior alreadyInTransactionBehavior() {
return alreadyInTransactionBehavior;
}
/**
* @return The computed-at-construction value of the {@code alreadyInTransactionBehaviorCorrected} attribute
*/
@Override
public @Nullable OptionalThreadContext.AlreadyInTransactionBehavior alreadyInTransactionBehaviorCorrected() {
InitShim shim = this.initShim;
return shim != null
? shim.alreadyInTransactionBehaviorCorrected()
: this.alreadyInTransactionBehaviorCorrected;
}
/**
* @return The value of the {@code spanMessageTemplate} attribute
*/
@Override
public String spanMessageTemplate() {
InitShim shim = this.initShim;
return shim != null
? shim.spanMessageTemplate()
: this.spanMessageTemplate;
}
/**
* @return The value of the {@code spanStackThresholdMillis} attribute
*/
@Override
public @Nullable Integer spanStackThresholdMillis() {
return spanStackThresholdMillis;
}
/**
* @return The value of the {@code spanCaptureSelfNested} attribute
*/
@Override
public boolean spanCaptureSelfNested() {
InitShim shim = this.initShim;
return shim != null
? shim.spanCaptureSelfNested()
: this.spanCaptureSelfNested;
}
/**
* @return The value of the {@code timerName} attribute
*/
@Override
public String timerName() {
InitShim shim = this.initShim;
return shim != null
? shim.timerName()
: this.timerName;
}
/**
* @return The value of the {@code enabledProperty} attribute
*/
@Override
public String enabledProperty() {
InitShim shim = this.initShim;
return shim != null
? shim.enabledProperty()
: this.enabledProperty;
}
/**
* @return The value of the {@code localSpanEnabledProperty} attribute
*/
@Override
public String localSpanEnabledProperty() {
InitShim shim = this.initShim;
return shim != null
? shim.localSpanEnabledProperty()
: this.localSpanEnabledProperty;
}
/**
* @return The computed-at-construction value of the {@code isTimerOrGreater} attribute
*/
@Override
public boolean isTimerOrGreater() {
InitShim shim = this.initShim;
return shim != null
? shim.isTimerOrGreater()
: this.isTimerOrGreater;
}
/**
* @return The computed-at-construction value of the {@code isLocalSpanOrGreater} attribute
*/
@Override
public boolean isLocalSpanOrGreater() {
InitShim shim = this.initShim;
return shim != null
? shim.isLocalSpanOrGreater()
: this.isLocalSpanOrGreater;
}
/**
* @return The computed-at-construction value of the {@code isTransaction} attribute
*/
@Override
public boolean isTransaction() {
InitShim shim = this.initShim;
return shim != null
? shim.isTransaction()
: this.isTransaction;
}
/**
* @return The computed-at-construction value of the {@code validationErrors} attribute
*/
@Override
public ImmutableList validationErrors() {
InitShim shim = this.initShim;
return shim != null
? shim.validationErrors()
: this.validationErrors;
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#className() className} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for className
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withClassName(String value) {
String newValue = Preconditions.checkNotNull(value, "className");
if (this.className.equals(newValue)) return this;
return new ImmutableAdviceConfig(
newValue,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#classAnnotation() classAnnotation} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for classAnnotation
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withClassAnnotation(String value) {
String newValue = Preconditions.checkNotNull(value, "classAnnotation");
if (this.classAnnotation.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
newValue,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#subTypeRestriction() subTypeRestriction} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for subTypeRestriction
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withSubTypeRestriction(String value) {
String newValue = Preconditions.checkNotNull(value, "subTypeRestriction");
if (this.subTypeRestriction.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
newValue,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#superTypeRestriction() superTypeRestriction} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for superTypeRestriction
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withSuperTypeRestriction(String value) {
String newValue = Preconditions.checkNotNull(value, "superTypeRestriction");
if (this.superTypeRestriction.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
newValue,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#methodDeclaringClassName() methodDeclaringClassName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for methodDeclaringClassName
* @return A modified copy of the {@code this} object
*/
@Deprecated
public final ImmutableAdviceConfig withMethodDeclaringClassName(String value) {
String newValue = Preconditions.checkNotNull(value, "methodDeclaringClassName");
if (this.methodDeclaringClassName.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
newValue,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#methodName() methodName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for methodName
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withMethodName(String value) {
String newValue = Preconditions.checkNotNull(value, "methodName");
if (this.methodName.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
newValue,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#methodAnnotation() methodAnnotation} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for methodAnnotation
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withMethodAnnotation(String value) {
String newValue = Preconditions.checkNotNull(value, "methodAnnotation");
if (this.methodAnnotation.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
newValue,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AdviceConfig#methodParameterTypes() methodParameterTypes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAdviceConfig withMethodParameterTypes(String... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
newValue,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AdviceConfig#methodParameterTypes() methodParameterTypes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of methodParameterTypes elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAdviceConfig withMethodParameterTypes(Iterable elements) {
if (this.methodParameterTypes == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
newValue,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#methodReturnType() methodReturnType} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for methodReturnType
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withMethodReturnType(String value) {
String newValue = Preconditions.checkNotNull(value, "methodReturnType");
if (this.methodReturnType.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
newValue,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AdviceConfig#methodModifiers() methodModifiers}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAdviceConfig withMethodModifiers(Advice.MethodModifier... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
newValue,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AdviceConfig#methodModifiers() methodModifiers}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of methodModifiers elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAdviceConfig withMethodModifiers(Iterable extends Advice.MethodModifier> elements) {
if (this.methodModifiers == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
newValue,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#nestingGroup() nestingGroup} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for nestingGroup
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withNestingGroup(String value) {
String newValue = Preconditions.checkNotNull(value, "nestingGroup");
if (this.nestingGroup.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
newValue,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#order() order} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for order
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withOrder(int value) {
if (this.order == value) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
value,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#captureKind() captureKind} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for captureKind
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withCaptureKind(Descriptor.CaptureKind value) {
if (this.captureKind == value) return this;
Descriptor.CaptureKind newValue = Preconditions.checkNotNull(value, "captureKind");
if (this.captureKind.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
newValue,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#transactionType() transactionType} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for transactionType
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withTransactionType(String value) {
String newValue = Preconditions.checkNotNull(value, "transactionType");
if (this.transactionType.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
newValue,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#transactionNameTemplate() transactionNameTemplate} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for transactionNameTemplate
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withTransactionNameTemplate(String value) {
String newValue = Preconditions.checkNotNull(value, "transactionNameTemplate");
if (this.transactionNameTemplate.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
newValue,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#transactionUserTemplate() transactionUserTemplate} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for transactionUserTemplate
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withTransactionUserTemplate(String value) {
String newValue = Preconditions.checkNotNull(value, "transactionUserTemplate");
if (this.transactionUserTemplate.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
newValue,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by replacing the {@link AdviceConfig#transactionAttributeTemplates() transactionAttributeTemplates} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the transactionAttributeTemplates map
* @return A modified copy of {@code this} object
*/
public final ImmutableAdviceConfig withTransactionAttributeTemplates(Map entries) {
if (this.transactionAttributeTemplates == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
newValue,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#transactionSlowThresholdMillis() transactionSlowThresholdMillis} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for transactionSlowThresholdMillis (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withTransactionSlowThresholdMillis(@Nullable Integer value) {
if (Objects.equal(this.transactionSlowThresholdMillis, value)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
value,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#alreadyInTransactionBehavior() alreadyInTransactionBehavior} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for alreadyInTransactionBehavior (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withAlreadyInTransactionBehavior(@Nullable OptionalThreadContext.AlreadyInTransactionBehavior value) {
if (this.alreadyInTransactionBehavior == value) return this;
if (Objects.equal(this.alreadyInTransactionBehavior, value)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
value,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#spanMessageTemplate() spanMessageTemplate} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for spanMessageTemplate
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withSpanMessageTemplate(String value) {
String newValue = Preconditions.checkNotNull(value, "spanMessageTemplate");
if (this.spanMessageTemplate.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
newValue,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#spanStackThresholdMillis() spanStackThresholdMillis} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for spanStackThresholdMillis (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withSpanStackThresholdMillis(@Nullable Integer value) {
if (Objects.equal(this.spanStackThresholdMillis, value)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
value,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#spanCaptureSelfNested() spanCaptureSelfNested} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for spanCaptureSelfNested
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withSpanCaptureSelfNested(boolean value) {
if (this.spanCaptureSelfNested == value) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
value,
this.timerName,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#timerName() timerName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for timerName
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withTimerName(String value) {
String newValue = Preconditions.checkNotNull(value, "timerName");
if (this.timerName.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
newValue,
this.enabledProperty,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#enabledProperty() enabledProperty} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for enabledProperty
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withEnabledProperty(String value) {
String newValue = Preconditions.checkNotNull(value, "enabledProperty");
if (this.enabledProperty.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
newValue,
this.localSpanEnabledProperty);
}
/**
* Copy the current immutable object by setting a value for the {@link AdviceConfig#localSpanEnabledProperty() localSpanEnabledProperty} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for localSpanEnabledProperty
* @return A modified copy of the {@code this} object
*/
public final ImmutableAdviceConfig withLocalSpanEnabledProperty(String value) {
String newValue = Preconditions.checkNotNull(value, "localSpanEnabledProperty");
if (this.localSpanEnabledProperty.equals(newValue)) return this;
return new ImmutableAdviceConfig(
this.className,
this.classAnnotation,
this.subTypeRestriction,
this.superTypeRestriction,
this.methodDeclaringClassName,
this.methodName,
this.methodAnnotation,
this.methodParameterTypes,
this.methodReturnType,
this.methodModifiers,
this.nestingGroup,
this.order,
this.captureKind,
this.transactionType,
this.transactionNameTemplate,
this.transactionUserTemplate,
this.transactionAttributeTemplates,
this.transactionSlowThresholdMillis,
this.alreadyInTransactionBehavior,
this.spanMessageTemplate,
this.spanStackThresholdMillis,
this.spanCaptureSelfNested,
this.timerName,
this.enabledProperty,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableAdviceConfig} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableAdviceConfig
&& equalTo((ImmutableAdviceConfig) another);
}
private boolean equalTo(ImmutableAdviceConfig another) {
return className.equals(another.className)
&& classAnnotation.equals(another.classAnnotation)
&& subTypeRestriction.equals(another.subTypeRestriction)
&& superTypeRestriction.equals(another.superTypeRestriction)
&& methodDeclaringClassName.equals(another.methodDeclaringClassName)
&& methodName.equals(another.methodName)
&& methodAnnotation.equals(another.methodAnnotation)
&& methodParameterTypes.equals(another.methodParameterTypes)
&& methodReturnType.equals(another.methodReturnType)
&& methodModifiers.equals(another.methodModifiers)
&& nestingGroup.equals(another.nestingGroup)
&& order == another.order
&& captureKind.equals(another.captureKind)
&& transactionType.equals(another.transactionType)
&& transactionNameTemplate.equals(another.transactionNameTemplate)
&& transactionUserTemplate.equals(another.transactionUserTemplate)
&& transactionAttributeTemplates.equals(another.transactionAttributeTemplates)
&& Objects.equal(transactionSlowThresholdMillis, another.transactionSlowThresholdMillis)
&& Objects.equal(alreadyInTransactionBehavior, another.alreadyInTransactionBehavior)
&& Objects.equal(alreadyInTransactionBehaviorCorrected, another.alreadyInTransactionBehaviorCorrected)
&& spanMessageTemplate.equals(another.spanMessageTemplate)
&& Objects.equal(spanStackThresholdMillis, another.spanStackThresholdMillis)
&& spanCaptureSelfNested == another.spanCaptureSelfNested
&& timerName.equals(another.timerName)
&& enabledProperty.equals(another.enabledProperty)
&& localSpanEnabledProperty.equals(another.localSpanEnabledProperty)
&& isTimerOrGreater == another.isTimerOrGreater
&& isLocalSpanOrGreater == another.isLocalSpanOrGreater
&& isTransaction == another.isTransaction
&& validationErrors.equals(another.validationErrors);
}
/**
* Computes a hash code from attributes: {@code className}, {@code classAnnotation}, {@code subTypeRestriction}, {@code superTypeRestriction}, {@code methodDeclaringClassName}, {@code methodName}, {@code methodAnnotation}, {@code methodParameterTypes}, {@code methodReturnType}, {@code methodModifiers}, {@code nestingGroup}, {@code order}, {@code captureKind}, {@code transactionType}, {@code transactionNameTemplate}, {@code transactionUserTemplate}, {@code transactionAttributeTemplates}, {@code transactionSlowThresholdMillis}, {@code alreadyInTransactionBehavior}, {@code alreadyInTransactionBehaviorCorrected}, {@code spanMessageTemplate}, {@code spanStackThresholdMillis}, {@code spanCaptureSelfNested}, {@code timerName}, {@code enabledProperty}, {@code localSpanEnabledProperty}, {@code isTimerOrGreater}, {@code isLocalSpanOrGreater}, {@code isTransaction}, {@code validationErrors}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + className.hashCode();
h += (h << 5) + classAnnotation.hashCode();
h += (h << 5) + subTypeRestriction.hashCode();
h += (h << 5) + superTypeRestriction.hashCode();
h += (h << 5) + methodDeclaringClassName.hashCode();
h += (h << 5) + methodName.hashCode();
h += (h << 5) + methodAnnotation.hashCode();
h += (h << 5) + methodParameterTypes.hashCode();
h += (h << 5) + methodReturnType.hashCode();
h += (h << 5) + methodModifiers.hashCode();
h += (h << 5) + nestingGroup.hashCode();
h += (h << 5) + order;
h += (h << 5) + captureKind.hashCode();
h += (h << 5) + transactionType.hashCode();
h += (h << 5) + transactionNameTemplate.hashCode();
h += (h << 5) + transactionUserTemplate.hashCode();
h += (h << 5) + transactionAttributeTemplates.hashCode();
h += (h << 5) + Objects.hashCode(transactionSlowThresholdMillis);
h += (h << 5) + Objects.hashCode(alreadyInTransactionBehavior);
h += (h << 5) + Objects.hashCode(alreadyInTransactionBehaviorCorrected);
h += (h << 5) + spanMessageTemplate.hashCode();
h += (h << 5) + Objects.hashCode(spanStackThresholdMillis);
h += (h << 5) + Booleans.hashCode(spanCaptureSelfNested);
h += (h << 5) + timerName.hashCode();
h += (h << 5) + enabledProperty.hashCode();
h += (h << 5) + localSpanEnabledProperty.hashCode();
h += (h << 5) + Booleans.hashCode(isTimerOrGreater);
h += (h << 5) + Booleans.hashCode(isLocalSpanOrGreater);
h += (h << 5) + Booleans.hashCode(isTransaction);
h += (h << 5) + validationErrors.hashCode();
return h;
}
/**
* Prints the immutable value {@code AdviceConfig} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("AdviceConfig")
.omitNullValues()
.add("className", className)
.add("classAnnotation", classAnnotation)
.add("subTypeRestriction", subTypeRestriction)
.add("superTypeRestriction", superTypeRestriction)
.add("methodDeclaringClassName", methodDeclaringClassName)
.add("methodName", methodName)
.add("methodAnnotation", methodAnnotation)
.add("methodParameterTypes", methodParameterTypes)
.add("methodReturnType", methodReturnType)
.add("methodModifiers", methodModifiers)
.add("nestingGroup", nestingGroup)
.add("order", order)
.add("captureKind", captureKind)
.add("transactionType", transactionType)
.add("transactionNameTemplate", transactionNameTemplate)
.add("transactionUserTemplate", transactionUserTemplate)
.add("transactionAttributeTemplates", transactionAttributeTemplates)
.add("transactionSlowThresholdMillis", transactionSlowThresholdMillis)
.add("alreadyInTransactionBehavior", alreadyInTransactionBehavior)
.add("alreadyInTransactionBehaviorCorrected", alreadyInTransactionBehaviorCorrected)
.add("spanMessageTemplate", spanMessageTemplate)
.add("spanStackThresholdMillis", spanStackThresholdMillis)
.add("spanCaptureSelfNested", spanCaptureSelfNested)
.add("timerName", timerName)
.add("enabledProperty", enabledProperty)
.add("localSpanEnabledProperty", localSpanEnabledProperty)
.add("isTimerOrGreater", isTimerOrGreater)
.add("isLocalSpanOrGreater", isLocalSpanOrGreater)
.add("isTransaction", isTransaction)
.add("validationErrors", validationErrors)
.toString();
}
/**
* Creates an immutable copy of a {@link AdviceConfig} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable AdviceConfig instance
*/
public static ImmutableAdviceConfig copyOf(AdviceConfig instance) {
if (instance instanceof ImmutableAdviceConfig) {
return (ImmutableAdviceConfig) instance;
}
return ImmutableAdviceConfig.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableAdviceConfig ImmutableAdviceConfig}.
*
* ImmutableAdviceConfig.builder()
* .className(String) // optional {@link AdviceConfig#className() className}
* .classAnnotation(String) // optional {@link AdviceConfig#classAnnotation() classAnnotation}
* .subTypeRestriction(String) // optional {@link AdviceConfig#subTypeRestriction() subTypeRestriction}
* .superTypeRestriction(String) // optional {@link AdviceConfig#superTypeRestriction() superTypeRestriction}
* .methodDeclaringClassName(String) // optional {@link AdviceConfig#methodDeclaringClassName() methodDeclaringClassName}
* .methodName(String) // optional {@link AdviceConfig#methodName() methodName}
* .methodAnnotation(String) // optional {@link AdviceConfig#methodAnnotation() methodAnnotation}
* .addMethodParameterTypes|addAllMethodParameterTypes(String) // {@link AdviceConfig#methodParameterTypes() methodParameterTypes} elements
* .methodReturnType(String) // optional {@link AdviceConfig#methodReturnType() methodReturnType}
* .addMethodModifiers|addAllMethodModifiers(org.glowroot.instrumentation.api.weaving.Advice.MethodModifier) // {@link AdviceConfig#methodModifiers() methodModifiers} elements
* .nestingGroup(String) // optional {@link AdviceConfig#nestingGroup() nestingGroup}
* .order(int) // optional {@link AdviceConfig#order() order}
* .captureKind(org.glowroot.instrumentation.api.Descriptor.CaptureKind) // required {@link AdviceConfig#captureKind() captureKind}
* .transactionType(String) // optional {@link AdviceConfig#transactionType() transactionType}
* .transactionNameTemplate(String) // optional {@link AdviceConfig#transactionNameTemplate() transactionNameTemplate}
* .transactionUserTemplate(String) // optional {@link AdviceConfig#transactionUserTemplate() transactionUserTemplate}
* .putTransactionAttributeTemplates|putAllTransactionAttributeTemplates(String => String) // {@link AdviceConfig#transactionAttributeTemplates() transactionAttributeTemplates} mappings
* .transactionSlowThresholdMillis(Integer | null) // nullable {@link AdviceConfig#transactionSlowThresholdMillis() transactionSlowThresholdMillis}
* .alreadyInTransactionBehavior(org.glowroot.instrumentation.api.OptionalThreadContext.AlreadyInTransactionBehavior | null) // nullable {@link AdviceConfig#alreadyInTransactionBehavior() alreadyInTransactionBehavior}
* .spanMessageTemplate(String) // optional {@link AdviceConfig#spanMessageTemplate() spanMessageTemplate}
* .spanStackThresholdMillis(Integer | null) // nullable {@link AdviceConfig#spanStackThresholdMillis() spanStackThresholdMillis}
* .spanCaptureSelfNested(boolean) // optional {@link AdviceConfig#spanCaptureSelfNested() spanCaptureSelfNested}
* .timerName(String) // optional {@link AdviceConfig#timerName() timerName}
* .enabledProperty(String) // optional {@link AdviceConfig#enabledProperty() enabledProperty}
* .localSpanEnabledProperty(String) // optional {@link AdviceConfig#localSpanEnabledProperty() localSpanEnabledProperty}
* .build();
*
* @return A new ImmutableAdviceConfig builder
*/
public static ImmutableAdviceConfig.Builder builder() {
return new ImmutableAdviceConfig.Builder();
}
/**
* Builds instances of type {@link ImmutableAdviceConfig ImmutableAdviceConfig}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "AdviceConfig", generator = "Immutables")
public static final class Builder {
private static final long INIT_BIT_CAPTURE_KIND = 0x1L;
private static final long OPT_BIT_ORDER = 0x1L;
private static final long OPT_BIT_SPAN_CAPTURE_SELF_NESTED = 0x2L;
private long initBits = 0x1L;
private long optBits;
private String className;
private String classAnnotation;
private String subTypeRestriction;
private String superTypeRestriction;
private String methodDeclaringClassName;
private String methodName;
private String methodAnnotation;
private ImmutableList.Builder methodParameterTypes = ImmutableList.builder();
private String methodReturnType;
private ImmutableList.Builder methodModifiers = ImmutableList.builder();
private String nestingGroup;
private int order;
private Descriptor.CaptureKind captureKind;
private String transactionType;
private String transactionNameTemplate;
private String transactionUserTemplate;
private ImmutableMap.Builder transactionAttributeTemplates = ImmutableMap.builder();
private Integer transactionSlowThresholdMillis;
private OptionalThreadContext.AlreadyInTransactionBehavior alreadyInTransactionBehavior;
private String spanMessageTemplate;
private Integer spanStackThresholdMillis;
private boolean spanCaptureSelfNested;
private String timerName;
private String enabledProperty;
private String localSpanEnabledProperty;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code AdviceConfig} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(AdviceConfig instance) {
Preconditions.checkNotNull(instance, "instance");
className(instance.className());
classAnnotation(instance.classAnnotation());
subTypeRestriction(instance.subTypeRestriction());
superTypeRestriction(instance.superTypeRestriction());
methodDeclaringClassName(instance.methodDeclaringClassName());
methodName(instance.methodName());
methodAnnotation(instance.methodAnnotation());
addAllMethodParameterTypes(instance.methodParameterTypes());
methodReturnType(instance.methodReturnType());
addAllMethodModifiers(instance.methodModifiers());
nestingGroup(instance.nestingGroup());
order(instance.order());
captureKind(instance.captureKind());
transactionType(instance.transactionType());
transactionNameTemplate(instance.transactionNameTemplate());
transactionUserTemplate(instance.transactionUserTemplate());
putAllTransactionAttributeTemplates(instance.transactionAttributeTemplates());
@Nullable Integer transactionSlowThresholdMillisValue = instance.transactionSlowThresholdMillis();
if (transactionSlowThresholdMillisValue != null) {
transactionSlowThresholdMillis(transactionSlowThresholdMillisValue);
}
@Nullable OptionalThreadContext.AlreadyInTransactionBehavior alreadyInTransactionBehaviorValue = instance.alreadyInTransactionBehavior();
if (alreadyInTransactionBehaviorValue != null) {
alreadyInTransactionBehavior(alreadyInTransactionBehaviorValue);
}
spanMessageTemplate(instance.spanMessageTemplate());
@Nullable Integer spanStackThresholdMillisValue = instance.spanStackThresholdMillis();
if (spanStackThresholdMillisValue != null) {
spanStackThresholdMillis(spanStackThresholdMillisValue);
}
spanCaptureSelfNested(instance.spanCaptureSelfNested());
timerName(instance.timerName());
enabledProperty(instance.enabledProperty());
localSpanEnabledProperty(instance.localSpanEnabledProperty());
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#className() className} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#className() className}.
* @param className The value for className
* @return {@code this} builder for use in a chained invocation
*/
public final Builder className(String className) {
this.className = Preconditions.checkNotNull(className, "className");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#classAnnotation() classAnnotation} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#classAnnotation() classAnnotation}.
* @param classAnnotation The value for classAnnotation
* @return {@code this} builder for use in a chained invocation
*/
public final Builder classAnnotation(String classAnnotation) {
this.classAnnotation = Preconditions.checkNotNull(classAnnotation, "classAnnotation");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#subTypeRestriction() subTypeRestriction} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#subTypeRestriction() subTypeRestriction}.
* @param subTypeRestriction The value for subTypeRestriction
* @return {@code this} builder for use in a chained invocation
*/
public final Builder subTypeRestriction(String subTypeRestriction) {
this.subTypeRestriction = Preconditions.checkNotNull(subTypeRestriction, "subTypeRestriction");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#superTypeRestriction() superTypeRestriction} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#superTypeRestriction() superTypeRestriction}.
* @param superTypeRestriction The value for superTypeRestriction
* @return {@code this} builder for use in a chained invocation
*/
public final Builder superTypeRestriction(String superTypeRestriction) {
this.superTypeRestriction = Preconditions.checkNotNull(superTypeRestriction, "superTypeRestriction");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#methodDeclaringClassName() methodDeclaringClassName} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#methodDeclaringClassName() methodDeclaringClassName}.
* @param methodDeclaringClassName The value for methodDeclaringClassName
* @return {@code this} builder for use in a chained invocation
*/
@Deprecated
public final Builder methodDeclaringClassName(String methodDeclaringClassName) {
this.methodDeclaringClassName = Preconditions.checkNotNull(methodDeclaringClassName, "methodDeclaringClassName");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#methodName() methodName} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#methodName() methodName}.
* @param methodName The value for methodName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder methodName(String methodName) {
this.methodName = Preconditions.checkNotNull(methodName, "methodName");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#methodAnnotation() methodAnnotation} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#methodAnnotation() methodAnnotation}.
* @param methodAnnotation The value for methodAnnotation
* @return {@code this} builder for use in a chained invocation
*/
public final Builder methodAnnotation(String methodAnnotation) {
this.methodAnnotation = Preconditions.checkNotNull(methodAnnotation, "methodAnnotation");
return this;
}
/**
* Adds one element to {@link AdviceConfig#methodParameterTypes() methodParameterTypes} list.
* @param element A methodParameterTypes element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMethodParameterTypes(String element) {
this.methodParameterTypes.add(element);
return this;
}
/**
* Adds elements to {@link AdviceConfig#methodParameterTypes() methodParameterTypes} list.
* @param elements An array of methodParameterTypes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMethodParameterTypes(String... elements) {
this.methodParameterTypes.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AdviceConfig#methodParameterTypes() methodParameterTypes} list.
* @param elements An iterable of methodParameterTypes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder methodParameterTypes(Iterable elements) {
this.methodParameterTypes = ImmutableList.builder();
return addAllMethodParameterTypes(elements);
}
/**
* Adds elements to {@link AdviceConfig#methodParameterTypes() methodParameterTypes} list.
* @param elements An iterable of methodParameterTypes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllMethodParameterTypes(Iterable elements) {
this.methodParameterTypes.addAll(elements);
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#methodReturnType() methodReturnType} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#methodReturnType() methodReturnType}.
* @param methodReturnType The value for methodReturnType
* @return {@code this} builder for use in a chained invocation
*/
public final Builder methodReturnType(String methodReturnType) {
this.methodReturnType = Preconditions.checkNotNull(methodReturnType, "methodReturnType");
return this;
}
/**
* Adds one element to {@link AdviceConfig#methodModifiers() methodModifiers} list.
* @param element A methodModifiers element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMethodModifiers(Advice.MethodModifier element) {
this.methodModifiers.add(element);
return this;
}
/**
* Adds elements to {@link AdviceConfig#methodModifiers() methodModifiers} list.
* @param elements An array of methodModifiers elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMethodModifiers(Advice.MethodModifier... elements) {
this.methodModifiers.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link AdviceConfig#methodModifiers() methodModifiers} list.
* @param elements An iterable of methodModifiers elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder methodModifiers(Iterable extends Advice.MethodModifier> elements) {
this.methodModifiers = ImmutableList.builder();
return addAllMethodModifiers(elements);
}
/**
* Adds elements to {@link AdviceConfig#methodModifiers() methodModifiers} list.
* @param elements An iterable of methodModifiers elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllMethodModifiers(Iterable extends Advice.MethodModifier> elements) {
this.methodModifiers.addAll(elements);
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#nestingGroup() nestingGroup} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#nestingGroup() nestingGroup}.
* @param nestingGroup The value for nestingGroup
* @return {@code this} builder for use in a chained invocation
*/
public final Builder nestingGroup(String nestingGroup) {
this.nestingGroup = Preconditions.checkNotNull(nestingGroup, "nestingGroup");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#order() order} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#order() order}.
* @param order The value for order
* @return {@code this} builder for use in a chained invocation
*/
public final Builder order(int order) {
this.order = order;
optBits |= OPT_BIT_ORDER;
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#captureKind() captureKind} attribute.
* @param captureKind The value for captureKind
* @return {@code this} builder for use in a chained invocation
*/
public final Builder captureKind(Descriptor.CaptureKind captureKind) {
this.captureKind = Preconditions.checkNotNull(captureKind, "captureKind");
initBits &= ~INIT_BIT_CAPTURE_KIND;
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#transactionType() transactionType} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#transactionType() transactionType}.
* @param transactionType The value for transactionType
* @return {@code this} builder for use in a chained invocation
*/
public final Builder transactionType(String transactionType) {
this.transactionType = Preconditions.checkNotNull(transactionType, "transactionType");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#transactionNameTemplate() transactionNameTemplate} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#transactionNameTemplate() transactionNameTemplate}.
* @param transactionNameTemplate The value for transactionNameTemplate
* @return {@code this} builder for use in a chained invocation
*/
public final Builder transactionNameTemplate(String transactionNameTemplate) {
this.transactionNameTemplate = Preconditions.checkNotNull(transactionNameTemplate, "transactionNameTemplate");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#transactionUserTemplate() transactionUserTemplate} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#transactionUserTemplate() transactionUserTemplate}.
* @param transactionUserTemplate The value for transactionUserTemplate
* @return {@code this} builder for use in a chained invocation
*/
public final Builder transactionUserTemplate(String transactionUserTemplate) {
this.transactionUserTemplate = Preconditions.checkNotNull(transactionUserTemplate, "transactionUserTemplate");
return this;
}
/**
* Put one entry to the {@link AdviceConfig#transactionAttributeTemplates() transactionAttributeTemplates} map.
* @param key The key in the transactionAttributeTemplates map
* @param value The associated value in the transactionAttributeTemplates map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putTransactionAttributeTemplates(String key, String value) {
this.transactionAttributeTemplates.put(key, value);
return this;
}
/**
* Put one entry to the {@link AdviceConfig#transactionAttributeTemplates() transactionAttributeTemplates} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putTransactionAttributeTemplates(Map.Entry entry) {
this.transactionAttributeTemplates.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link AdviceConfig#transactionAttributeTemplates() transactionAttributeTemplates} map. Nulls are not permitted
* @param entries The entries that will be added to the transactionAttributeTemplates map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder transactionAttributeTemplates(Map entries) {
this.transactionAttributeTemplates = ImmutableMap.builder();
return putAllTransactionAttributeTemplates(entries);
}
/**
* Put all mappings from the specified map as entries to {@link AdviceConfig#transactionAttributeTemplates() transactionAttributeTemplates} map. Nulls are not permitted
* @param entries The entries that will be added to the transactionAttributeTemplates map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putAllTransactionAttributeTemplates(Map entries) {
this.transactionAttributeTemplates.putAll(entries);
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#transactionSlowThresholdMillis() transactionSlowThresholdMillis} attribute.
* @param transactionSlowThresholdMillis The value for transactionSlowThresholdMillis (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder transactionSlowThresholdMillis(@Nullable Integer transactionSlowThresholdMillis) {
this.transactionSlowThresholdMillis = transactionSlowThresholdMillis;
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#alreadyInTransactionBehavior() alreadyInTransactionBehavior} attribute.
* @param alreadyInTransactionBehavior The value for alreadyInTransactionBehavior (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder alreadyInTransactionBehavior(@Nullable OptionalThreadContext.AlreadyInTransactionBehavior alreadyInTransactionBehavior) {
this.alreadyInTransactionBehavior = alreadyInTransactionBehavior;
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#spanMessageTemplate() spanMessageTemplate} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#spanMessageTemplate() spanMessageTemplate}.
* @param spanMessageTemplate The value for spanMessageTemplate
* @return {@code this} builder for use in a chained invocation
*/
public final Builder spanMessageTemplate(String spanMessageTemplate) {
this.spanMessageTemplate = Preconditions.checkNotNull(spanMessageTemplate, "spanMessageTemplate");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#spanStackThresholdMillis() spanStackThresholdMillis} attribute.
* @param spanStackThresholdMillis The value for spanStackThresholdMillis (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder spanStackThresholdMillis(@Nullable Integer spanStackThresholdMillis) {
this.spanStackThresholdMillis = spanStackThresholdMillis;
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#spanCaptureSelfNested() spanCaptureSelfNested} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#spanCaptureSelfNested() spanCaptureSelfNested}.
* @param spanCaptureSelfNested The value for spanCaptureSelfNested
* @return {@code this} builder for use in a chained invocation
*/
public final Builder spanCaptureSelfNested(boolean spanCaptureSelfNested) {
this.spanCaptureSelfNested = spanCaptureSelfNested;
optBits |= OPT_BIT_SPAN_CAPTURE_SELF_NESTED;
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#timerName() timerName} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#timerName() timerName}.
* @param timerName The value for timerName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder timerName(String timerName) {
this.timerName = Preconditions.checkNotNull(timerName, "timerName");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#enabledProperty() enabledProperty} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#enabledProperty() enabledProperty}.
* @param enabledProperty The value for enabledProperty
* @return {@code this} builder for use in a chained invocation
*/
public final Builder enabledProperty(String enabledProperty) {
this.enabledProperty = Preconditions.checkNotNull(enabledProperty, "enabledProperty");
return this;
}
/**
* Initializes the value for the {@link AdviceConfig#localSpanEnabledProperty() localSpanEnabledProperty} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AdviceConfig#localSpanEnabledProperty() localSpanEnabledProperty}.
* @param localSpanEnabledProperty The value for localSpanEnabledProperty
* @return {@code this} builder for use in a chained invocation
*/
public final Builder localSpanEnabledProperty(String localSpanEnabledProperty) {
this.localSpanEnabledProperty = Preconditions.checkNotNull(localSpanEnabledProperty, "localSpanEnabledProperty");
return this;
}
/**
* Builds a new {@link ImmutableAdviceConfig ImmutableAdviceConfig}.
* @return An immutable instance of AdviceConfig
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableAdviceConfig build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableAdviceConfig(this);
}
private boolean orderIsSet() {
return (optBits & OPT_BIT_ORDER) != 0;
}
private boolean spanCaptureSelfNestedIsSet() {
return (optBits & OPT_BIT_SPAN_CAPTURE_SELF_NESTED) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList();
if ((initBits & INIT_BIT_CAPTURE_KIND) != 0) attributes.add("captureKind");
return "Cannot build AdviceConfig, some of required attributes are not set " + attributes;
}
}
}