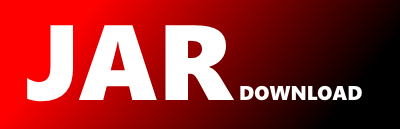
org.glowroot.local.ui.Layout Maven / Gradle / Ivy
package org.glowroot.local.ui;
import org.glowroot.shaded.fasterxml.jackson.annotation.JsonCreator;
import org.glowroot.shaded.fasterxml.jackson.annotation.JsonProperty;
import org.glowroot.shaded.google.common.base.MoreObjects;
import org.glowroot.shaded.google.common.base.Preconditions;
import org.glowroot.shaded.google.common.collect.ImmutableList;
import org.glowroot.shaded.google.common.collect.Lists;
import org.glowroot.shaded.google.common.primitives.Booleans;
import org.glowroot.shaded.google.common.primitives.Doubles;
import org.glowroot.shaded.google.common.primitives.Longs;
import java.util.Collection;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.glowroot.config.AnonymousAccess;
/**
* Immutable implementation of {@link LayoutBase}.
*
* Use builder to create immutable instances:
* {@code Layout.builder()}.
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "LayoutBase"})
@Immutable
final class Layout extends LayoutBase {
private final boolean jvmHeapDump;
private final String footerMessage;
private final boolean adminPasswordEnabled;
private final boolean readOnlyPasswordEnabled;
private final AnonymousAccess anonymousAccess;
private final ImmutableList transactionTypes;
private final String defaultTransactionType;
private final ImmutableList defaultPercentiles;
private final ImmutableList transactionCustomAttributes;
private final long fixedAggregateIntervalSeconds;
private final long fixedAggregateRollup1Seconds;
private final long fixedAggregateRollup2Seconds;
private final long fixedGaugeIntervalSeconds;
private final long fixedGaugeRollup1Seconds;
private final long fixedGaugeRollup2Seconds;
private final String version;
private Layout(
boolean jvmHeapDump,
String footerMessage,
boolean adminPasswordEnabled,
boolean readOnlyPasswordEnabled,
AnonymousAccess anonymousAccess,
ImmutableList transactionTypes,
String defaultTransactionType,
ImmutableList defaultPercentiles,
ImmutableList transactionCustomAttributes,
long fixedAggregateIntervalSeconds,
long fixedAggregateRollup1Seconds,
long fixedAggregateRollup2Seconds,
long fixedGaugeIntervalSeconds,
long fixedGaugeRollup1Seconds,
long fixedGaugeRollup2Seconds) {
this.jvmHeapDump = jvmHeapDump;
this.footerMessage = footerMessage;
this.adminPasswordEnabled = adminPasswordEnabled;
this.readOnlyPasswordEnabled = readOnlyPasswordEnabled;
this.anonymousAccess = anonymousAccess;
this.transactionTypes = transactionTypes;
this.defaultTransactionType = defaultTransactionType;
this.defaultPercentiles = defaultPercentiles;
this.transactionCustomAttributes = transactionCustomAttributes;
this.fixedAggregateIntervalSeconds = fixedAggregateIntervalSeconds;
this.fixedAggregateRollup1Seconds = fixedAggregateRollup1Seconds;
this.fixedAggregateRollup2Seconds = fixedAggregateRollup2Seconds;
this.fixedGaugeIntervalSeconds = fixedGaugeIntervalSeconds;
this.fixedGaugeRollup1Seconds = fixedGaugeRollup1Seconds;
this.fixedGaugeRollup2Seconds = fixedGaugeRollup2Seconds;
this.version = Preconditions.checkNotNull(super.version());
}
/**
* {@inheritDoc}
* @return value of {@code jvmHeapDump} attribute
*/
@JsonProperty("jvmHeapDump")
@Override
public boolean jvmHeapDump() {
return jvmHeapDump;
}
/**
* {@inheritDoc}
* @return value of {@code footerMessage} attribute
*/
@JsonProperty("footerMessage")
@Override
public String footerMessage() {
return footerMessage;
}
/**
* {@inheritDoc}
* @return value of {@code adminPasswordEnabled} attribute
*/
@JsonProperty("adminPasswordEnabled")
@Override
public boolean adminPasswordEnabled() {
return adminPasswordEnabled;
}
/**
* {@inheritDoc}
* @return value of {@code readOnlyPasswordEnabled} attribute
*/
@JsonProperty("readOnlyPasswordEnabled")
@Override
public boolean readOnlyPasswordEnabled() {
return readOnlyPasswordEnabled;
}
/**
* {@inheritDoc}
* @return value of {@code anonymousAccess} attribute
*/
@JsonProperty("anonymousAccess")
@Override
public AnonymousAccess anonymousAccess() {
return anonymousAccess;
}
/**
* {@inheritDoc}
* @return value of {@code transactionTypes} attribute
*/
@JsonProperty("transactionTypes")
@Override
public ImmutableList transactionTypes() {
return transactionTypes;
}
/**
* {@inheritDoc}
* @return value of {@code defaultTransactionType} attribute
*/
@JsonProperty("defaultTransactionType")
@Override
public String defaultTransactionType() {
return defaultTransactionType;
}
/**
* {@inheritDoc}
* @return value of {@code defaultPercentiles} attribute
*/
@JsonProperty("defaultPercentiles")
@Override
public ImmutableList defaultPercentiles() {
return defaultPercentiles;
}
/**
* {@inheritDoc}
* @return value of {@code transactionCustomAttributes} attribute
*/
@JsonProperty("transactionCustomAttributes")
@Override
public ImmutableList transactionCustomAttributes() {
return transactionCustomAttributes;
}
/**
* {@inheritDoc}
* @return value of {@code fixedAggregateIntervalSeconds} attribute
*/
@JsonProperty("fixedAggregateIntervalSeconds")
@Override
public long fixedAggregateIntervalSeconds() {
return fixedAggregateIntervalSeconds;
}
/**
* {@inheritDoc}
* @return value of {@code fixedAggregateRollup1Seconds} attribute
*/
@JsonProperty("fixedAggregateRollup1Seconds")
@Override
public long fixedAggregateRollup1Seconds() {
return fixedAggregateRollup1Seconds;
}
/**
* {@inheritDoc}
* @return value of {@code fixedAggregateRollup2Seconds} attribute
*/
@JsonProperty("fixedAggregateRollup2Seconds")
@Override
public long fixedAggregateRollup2Seconds() {
return fixedAggregateRollup2Seconds;
}
/**
* {@inheritDoc}
* @return value of {@code fixedGaugeIntervalSeconds} attribute
*/
@JsonProperty("fixedGaugeIntervalSeconds")
@Override
public long fixedGaugeIntervalSeconds() {
return fixedGaugeIntervalSeconds;
}
/**
* {@inheritDoc}
* @return value of {@code fixedGaugeRollup1Seconds} attribute
*/
@JsonProperty("fixedGaugeRollup1Seconds")
@Override
public long fixedGaugeRollup1Seconds() {
return fixedGaugeRollup1Seconds;
}
/**
* {@inheritDoc}
* @return value of {@code fixedGaugeRollup2Seconds} attribute
*/
@JsonProperty("fixedGaugeRollup2Seconds")
@Override
public long fixedGaugeRollup2Seconds() {
return fixedGaugeRollup2Seconds;
}
/**
* {@inheritDoc}
* @return computed at construction value of {@code version} attribute
*/
@JsonProperty("version")
@Override
public String version() {
return version;
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#jvmHeapDump() jvmHeapDump}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for jvmHeapDump
* @return modified copy of the {@code this} object
*/
public final Layout withJvmHeapDump(boolean value) {
if (this.jvmHeapDump == value) {
return this;
}
boolean newValue = value;
return new Layout(
newValue,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#footerMessage() footerMessage}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for footerMessage
* @return modified copy of the {@code this} object
*/
public final Layout withFooterMessage(String value) {
if (this.footerMessage == value) {
return this;
}
String newValue = Preconditions.checkNotNull(value);
return new Layout(
this.jvmHeapDump,
newValue,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#adminPasswordEnabled() adminPasswordEnabled}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for adminPasswordEnabled
* @return modified copy of the {@code this} object
*/
public final Layout withAdminPasswordEnabled(boolean value) {
if (this.adminPasswordEnabled == value) {
return this;
}
boolean newValue = value;
return new Layout(
this.jvmHeapDump,
this.footerMessage,
newValue,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#readOnlyPasswordEnabled() readOnlyPasswordEnabled}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for readOnlyPasswordEnabled
* @return modified copy of the {@code this} object
*/
public final Layout withReadOnlyPasswordEnabled(boolean value) {
if (this.readOnlyPasswordEnabled == value) {
return this;
}
boolean newValue = value;
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
newValue,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#anonymousAccess() anonymousAccess}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for anonymousAccess
* @return modified copy of the {@code this} object
*/
public final Layout withAnonymousAccess(AnonymousAccess value) {
if (this.anonymousAccess == value) {
return this;
}
AnonymousAccess newValue = Preconditions.checkNotNull(value);
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
newValue,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object with elements that replace content of {@link LayoutBase#transactionTypes() transactionTypes}.
* @param elements elements to set
* @return modified copy of {@code this} object
*/
public final Layout withTransactionTypes(String... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
newValue,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object with elements that replace content of {@link LayoutBase#transactionTypes() transactionTypes}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements iterable of transactionTypes elements to set
* @return modified copy of {@code this} object
*/
public final Layout withTransactionTypes(Iterable elements) {
if (this.transactionTypes == elements) {
return this;
}
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
newValue,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#defaultTransactionType() defaultTransactionType}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for defaultTransactionType
* @return modified copy of the {@code this} object
*/
public final Layout withDefaultTransactionType(String value) {
if (this.defaultTransactionType == value) {
return this;
}
String newValue = Preconditions.checkNotNull(value);
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
newValue,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object with elements that replace content of {@link LayoutBase#defaultPercentiles() defaultPercentiles}.
* @param elements elements to set
* @return modified copy of {@code this} object
*/
public final Layout withDefaultPercentiles(double... elements) {
ImmutableList newValue = ImmutableList.copyOf(Doubles.asList(elements));
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
newValue,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object with elements that replace content of {@link LayoutBase#defaultPercentiles() defaultPercentiles}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements iterable of defaultPercentiles elements to set
* @return modified copy of {@code this} object
*/
public final Layout withDefaultPercentiles(Iterable elements) {
if (this.defaultPercentiles == elements) {
return this;
}
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
newValue,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object with elements that replace content of {@link LayoutBase#transactionCustomAttributes() transactionCustomAttributes}.
* @param elements elements to set
* @return modified copy of {@code this} object
*/
public final Layout withTransactionCustomAttributes(String... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
newValue,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object with elements that replace content of {@link LayoutBase#transactionCustomAttributes() transactionCustomAttributes}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements iterable of transactionCustomAttributes elements to set
* @return modified copy of {@code this} object
*/
public final Layout withTransactionCustomAttributes(Iterable elements) {
if (this.transactionCustomAttributes == elements) {
return this;
}
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
newValue,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#fixedAggregateIntervalSeconds() fixedAggregateIntervalSeconds}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for fixedAggregateIntervalSeconds
* @return modified copy of the {@code this} object
*/
public final Layout withFixedAggregateIntervalSeconds(long value) {
if (this.fixedAggregateIntervalSeconds == value) {
return this;
}
long newValue = value;
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
newValue,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#fixedAggregateRollup1Seconds() fixedAggregateRollup1Seconds}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for fixedAggregateRollup1Seconds
* @return modified copy of the {@code this} object
*/
public final Layout withFixedAggregateRollup1Seconds(long value) {
if (this.fixedAggregateRollup1Seconds == value) {
return this;
}
long newValue = value;
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
newValue,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#fixedAggregateRollup2Seconds() fixedAggregateRollup2Seconds}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for fixedAggregateRollup2Seconds
* @return modified copy of the {@code this} object
*/
public final Layout withFixedAggregateRollup2Seconds(long value) {
if (this.fixedAggregateRollup2Seconds == value) {
return this;
}
long newValue = value;
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
newValue,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#fixedGaugeIntervalSeconds() fixedGaugeIntervalSeconds}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for fixedGaugeIntervalSeconds
* @return modified copy of the {@code this} object
*/
public final Layout withFixedGaugeIntervalSeconds(long value) {
if (this.fixedGaugeIntervalSeconds == value) {
return this;
}
long newValue = value;
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
newValue,
this.fixedGaugeRollup1Seconds,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#fixedGaugeRollup1Seconds() fixedGaugeRollup1Seconds}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for fixedGaugeRollup1Seconds
* @return modified copy of the {@code this} object
*/
public final Layout withFixedGaugeRollup1Seconds(long value) {
if (this.fixedGaugeRollup1Seconds == value) {
return this;
}
long newValue = value;
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
newValue,
this.fixedGaugeRollup2Seconds);
}
/**
* Copy current immutable object by setting value for {@link LayoutBase#fixedGaugeRollup2Seconds() fixedGaugeRollup2Seconds}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for fixedGaugeRollup2Seconds
* @return modified copy of the {@code this} object
*/
public final Layout withFixedGaugeRollup2Seconds(long value) {
if (this.fixedGaugeRollup2Seconds == value) {
return this;
}
long newValue = value;
return new Layout(
this.jvmHeapDump,
this.footerMessage,
this.adminPasswordEnabled,
this.readOnlyPasswordEnabled,
this.anonymousAccess,
this.transactionTypes,
this.defaultTransactionType,
this.defaultPercentiles,
this.transactionCustomAttributes,
this.fixedAggregateIntervalSeconds,
this.fixedAggregateRollup1Seconds,
this.fixedAggregateRollup2Seconds,
this.fixedGaugeIntervalSeconds,
this.fixedGaugeRollup1Seconds,
newValue);
}
/**
* This instance is equal to instances of {@code Layout} with equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
return this == another
|| (another instanceof Layout && equalTo((Layout) another));
}
private boolean equalTo(Layout another) {
return jvmHeapDump == another.jvmHeapDump
&& footerMessage.equals(another.footerMessage)
&& adminPasswordEnabled == another.adminPasswordEnabled
&& readOnlyPasswordEnabled == another.readOnlyPasswordEnabled
&& anonymousAccess.equals(another.anonymousAccess)
&& transactionTypes.equals(another.transactionTypes)
&& defaultTransactionType.equals(another.defaultTransactionType)
&& defaultPercentiles.equals(another.defaultPercentiles)
&& transactionCustomAttributes.equals(another.transactionCustomAttributes)
&& fixedAggregateIntervalSeconds == another.fixedAggregateIntervalSeconds
&& fixedAggregateRollup1Seconds == another.fixedAggregateRollup1Seconds
&& fixedAggregateRollup2Seconds == another.fixedAggregateRollup2Seconds
&& fixedGaugeIntervalSeconds == another.fixedGaugeIntervalSeconds
&& fixedGaugeRollup1Seconds == another.fixedGaugeRollup1Seconds
&& fixedGaugeRollup2Seconds == another.fixedGaugeRollup2Seconds
&& version.equals(another.version);
}
/**
* Computes hash code from attributes: {@code jvmHeapDump}, {@code footerMessage}, {@code adminPasswordEnabled}, {@code readOnlyPasswordEnabled}, {@code anonymousAccess}, {@code transactionTypes}, {@code defaultTransactionType}, {@code defaultPercentiles}, {@code transactionCustomAttributes}, {@code fixedAggregateIntervalSeconds}, {@code fixedAggregateRollup1Seconds}, {@code fixedAggregateRollup2Seconds}, {@code fixedGaugeIntervalSeconds}, {@code fixedGaugeRollup1Seconds}, {@code fixedGaugeRollup2Seconds}, {@code version}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + Booleans.hashCode(jvmHeapDump);
h = h * 17 + footerMessage.hashCode();
h = h * 17 + Booleans.hashCode(adminPasswordEnabled);
h = h * 17 + Booleans.hashCode(readOnlyPasswordEnabled);
h = h * 17 + anonymousAccess.hashCode();
h = h * 17 + transactionTypes.hashCode();
h = h * 17 + defaultTransactionType.hashCode();
h = h * 17 + defaultPercentiles.hashCode();
h = h * 17 + transactionCustomAttributes.hashCode();
h = h * 17 + Longs.hashCode(fixedAggregateIntervalSeconds);
h = h * 17 + Longs.hashCode(fixedAggregateRollup1Seconds);
h = h * 17 + Longs.hashCode(fixedAggregateRollup2Seconds);
h = h * 17 + Longs.hashCode(fixedGaugeIntervalSeconds);
h = h * 17 + Longs.hashCode(fixedGaugeRollup1Seconds);
h = h * 17 + Longs.hashCode(fixedGaugeRollup2Seconds);
h = h * 17 + version.hashCode();
return h;
}
/**
* Prints immutable value {@code Layout{...}} with attribute values,
* excluding any non-generated and auxiliary attributes.
* @return string representation of value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Layout")
.add("jvmHeapDump", jvmHeapDump)
.add("footerMessage", footerMessage)
.add("adminPasswordEnabled", adminPasswordEnabled)
.add("readOnlyPasswordEnabled", readOnlyPasswordEnabled)
.add("anonymousAccess", anonymousAccess)
.add("transactionTypes", transactionTypes)
.add("defaultTransactionType", defaultTransactionType)
.add("defaultPercentiles", defaultPercentiles)
.add("transactionCustomAttributes", transactionCustomAttributes)
.add("fixedAggregateIntervalSeconds", fixedAggregateIntervalSeconds)
.add("fixedAggregateRollup1Seconds", fixedAggregateRollup1Seconds)
.add("fixedAggregateRollup2Seconds", fixedAggregateRollup2Seconds)
.add("fixedGaugeIntervalSeconds", fixedGaugeIntervalSeconds)
.add("fixedGaugeRollup1Seconds", fixedGaugeRollup1Seconds)
.add("fixedGaugeRollup2Seconds", fixedGaugeRollup2Seconds)
.add("version", version)
.toString();
}
@JsonCreator
public static Layout fromAllAttributes(
@JsonProperty("jvmHeapDump") @Nullable Boolean jvmHeapDump,
@JsonProperty("footerMessage") @Nullable String footerMessage,
@JsonProperty("adminPasswordEnabled") @Nullable Boolean adminPasswordEnabled,
@JsonProperty("readOnlyPasswordEnabled") @Nullable Boolean readOnlyPasswordEnabled,
@JsonProperty("anonymousAccess") @Nullable AnonymousAccess anonymousAccess,
@JsonProperty("transactionTypes") @Nullable ImmutableList transactionTypes,
@JsonProperty("defaultTransactionType") @Nullable String defaultTransactionType,
@JsonProperty("defaultPercentiles") @Nullable ImmutableList defaultPercentiles,
@JsonProperty("transactionCustomAttributes") @Nullable ImmutableList transactionCustomAttributes,
@JsonProperty("fixedAggregateIntervalSeconds") @Nullable Long fixedAggregateIntervalSeconds,
@JsonProperty("fixedAggregateRollup1Seconds") @Nullable Long fixedAggregateRollup1Seconds,
@JsonProperty("fixedAggregateRollup2Seconds") @Nullable Long fixedAggregateRollup2Seconds,
@JsonProperty("fixedGaugeIntervalSeconds") @Nullable Long fixedGaugeIntervalSeconds,
@JsonProperty("fixedGaugeRollup1Seconds") @Nullable Long fixedGaugeRollup1Seconds,
@JsonProperty("fixedGaugeRollup2Seconds") @Nullable Long fixedGaugeRollup2Seconds) {
Layout.Builder builder = Layout.builder();
if (jvmHeapDump != null) {
builder.jvmHeapDump(jvmHeapDump);
}
if (footerMessage != null) {
builder.footerMessage(footerMessage);
}
if (adminPasswordEnabled != null) {
builder.adminPasswordEnabled(adminPasswordEnabled);
}
if (readOnlyPasswordEnabled != null) {
builder.readOnlyPasswordEnabled(readOnlyPasswordEnabled);
}
if (anonymousAccess != null) {
builder.anonymousAccess(anonymousAccess);
}
if (transactionTypes != null) {
builder.addAllTransactionTypes(transactionTypes);
}
if (defaultTransactionType != null) {
builder.defaultTransactionType(defaultTransactionType);
}
if (defaultPercentiles != null) {
builder.addAllDefaultPercentiles(defaultPercentiles);
}
if (transactionCustomAttributes != null) {
builder.addAllTransactionCustomAttributes(transactionCustomAttributes);
}
if (fixedAggregateIntervalSeconds != null) {
builder.fixedAggregateIntervalSeconds(fixedAggregateIntervalSeconds);
}
if (fixedAggregateRollup1Seconds != null) {
builder.fixedAggregateRollup1Seconds(fixedAggregateRollup1Seconds);
}
if (fixedAggregateRollup2Seconds != null) {
builder.fixedAggregateRollup2Seconds(fixedAggregateRollup2Seconds);
}
if (fixedGaugeIntervalSeconds != null) {
builder.fixedGaugeIntervalSeconds(fixedGaugeIntervalSeconds);
}
if (fixedGaugeRollup1Seconds != null) {
builder.fixedGaugeRollup1Seconds(fixedGaugeRollup1Seconds);
}
if (fixedGaugeRollup2Seconds != null) {
builder.fixedGaugeRollup2Seconds(fixedGaugeRollup2Seconds);
}
return builder.build();
}
/**
* Creates immutable copy of {@link LayoutBase}.
* Uses accessors to get values to initialize immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance instance to copy
* @return copied immutable Layout instance
*/
static Layout copyOf(LayoutBase instance) {
if (instance instanceof Layout) {
return (Layout) instance;
}
return Layout.builder()
.jvmHeapDump(instance.jvmHeapDump())
.footerMessage(instance.footerMessage())
.adminPasswordEnabled(instance.adminPasswordEnabled())
.readOnlyPasswordEnabled(instance.readOnlyPasswordEnabled())
.anonymousAccess(instance.anonymousAccess())
.addAllTransactionTypes(instance.transactionTypes())
.defaultTransactionType(instance.defaultTransactionType())
.addAllDefaultPercentiles(instance.defaultPercentiles())
.addAllTransactionCustomAttributes(instance.transactionCustomAttributes())
.fixedAggregateIntervalSeconds(instance.fixedAggregateIntervalSeconds())
.fixedAggregateRollup1Seconds(instance.fixedAggregateRollup1Seconds())
.fixedAggregateRollup2Seconds(instance.fixedAggregateRollup2Seconds())
.fixedGaugeIntervalSeconds(instance.fixedGaugeIntervalSeconds())
.fixedGaugeRollup1Seconds(instance.fixedGaugeRollup1Seconds())
.fixedGaugeRollup2Seconds(instance.fixedGaugeRollup2Seconds())
.build();
}
/**
* Creates builder for {@link org.glowroot.local.ui.Layout}.
* @return new Layout builder
*/
static Layout.Builder builder() {
return new Layout.Builder();
}
/**
* Builds instances of {@link org.glowroot.local.ui.Layout}.
* Initialized attributes and then invoke {@link #build()} method to create
* immutable instance.
* Builder is not thread safe and generally should not be stored in field or collection,
* but used immediately to create instances.
*/
@NotThreadSafe
static final class Builder {
private static final long INITIALIZED_BITSET_ALL = 0xfff;
private static final long INITIALIZED_BIT_JVM_HEAP_DUMP = 0x1L;
private static final long INITIALIZED_BIT_FOOTER_MESSAGE = 0x2L;
private static final long INITIALIZED_BIT_ADMIN_PASSWORD_ENABLED = 0x4L;
private static final long INITIALIZED_BIT_READ_ONLY_PASSWORD_ENABLED = 0x8L;
private static final long INITIALIZED_BIT_ANONYMOUS_ACCESS = 0x10L;
private static final long INITIALIZED_BIT_DEFAULT_TRANSACTION_TYPE = 0x20L;
private static final long INITIALIZED_BIT_FIXED_AGGREGATE_INTERVAL_SECONDS = 0x40L;
private static final long INITIALIZED_BIT_FIXED_AGGREGATE_ROLLUP1_SECONDS = 0x80L;
private static final long INITIALIZED_BIT_FIXED_AGGREGATE_ROLLUP2_SECONDS = 0x100L;
private static final long INITIALIZED_BIT_FIXED_GAUGE_INTERVAL_SECONDS = 0x200L;
private static final long INITIALIZED_BIT_FIXED_GAUGE_ROLLUP1_SECONDS = 0x400L;
private static final long INITIALIZED_BIT_FIXED_GAUGE_ROLLUP2_SECONDS = 0x800L;
private long initializedBitset;
private boolean jvmHeapDump;
private @Nullable String footerMessage;
private boolean adminPasswordEnabled;
private boolean readOnlyPasswordEnabled;
private @Nullable AnonymousAccess anonymousAccess;
private ImmutableList.Builder transactionTypesBuilder = ImmutableList.builder();
private @Nullable String defaultTransactionType;
private ImmutableList.Builder defaultPercentilesBuilder = ImmutableList.builder();
private ImmutableList.Builder transactionCustomAttributesBuilder = ImmutableList.builder();
private long fixedAggregateIntervalSeconds;
private long fixedAggregateRollup1Seconds;
private long fixedAggregateRollup2Seconds;
private long fixedGaugeIntervalSeconds;
private long fixedGaugeRollup1Seconds;
private long fixedGaugeRollup2Seconds;
private Builder() {}
/**
* Initializes value for {@link LayoutBase#jvmHeapDump() jvmHeapDump}.
* @param jvmHeapDump value for jvmHeapDump
* @return {@code this} builder for chained invocation
*/
public final Builder jvmHeapDump(boolean jvmHeapDump) {
checkNotIsSet(jvmHeapDumpIsSet(), "jvmHeapDump");
this.jvmHeapDump = jvmHeapDump;
initializedBitset |= INITIALIZED_BIT_JVM_HEAP_DUMP;
return this;
}
/**
* Initializes value for {@link LayoutBase#footerMessage() footerMessage}.
* @param footerMessage value for footerMessage
* @return {@code this} builder for chained invocation
*/
public final Builder footerMessage(String footerMessage) {
checkNotIsSet(footerMessageIsSet(), "footerMessage");
this.footerMessage = Preconditions.checkNotNull(footerMessage);
initializedBitset |= INITIALIZED_BIT_FOOTER_MESSAGE;
return this;
}
/**
* Initializes value for {@link LayoutBase#adminPasswordEnabled() adminPasswordEnabled}.
* @param adminPasswordEnabled value for adminPasswordEnabled
* @return {@code this} builder for chained invocation
*/
public final Builder adminPasswordEnabled(boolean adminPasswordEnabled) {
checkNotIsSet(adminPasswordEnabledIsSet(), "adminPasswordEnabled");
this.adminPasswordEnabled = adminPasswordEnabled;
initializedBitset |= INITIALIZED_BIT_ADMIN_PASSWORD_ENABLED;
return this;
}
/**
* Initializes value for {@link LayoutBase#readOnlyPasswordEnabled() readOnlyPasswordEnabled}.
* @param readOnlyPasswordEnabled value for readOnlyPasswordEnabled
* @return {@code this} builder for chained invocation
*/
public final Builder readOnlyPasswordEnabled(boolean readOnlyPasswordEnabled) {
checkNotIsSet(readOnlyPasswordEnabledIsSet(), "readOnlyPasswordEnabled");
this.readOnlyPasswordEnabled = readOnlyPasswordEnabled;
initializedBitset |= INITIALIZED_BIT_READ_ONLY_PASSWORD_ENABLED;
return this;
}
/**
* Initializes value for {@link LayoutBase#anonymousAccess() anonymousAccess}.
* @param anonymousAccess value for anonymousAccess
* @return {@code this} builder for chained invocation
*/
public final Builder anonymousAccess(AnonymousAccess anonymousAccess) {
checkNotIsSet(anonymousAccessIsSet(), "anonymousAccess");
this.anonymousAccess = Preconditions.checkNotNull(anonymousAccess);
initializedBitset |= INITIALIZED_BIT_ANONYMOUS_ACCESS;
return this;
}
/**
* Adds one element to {@link LayoutBase#transactionTypes() transactionTypes} list.
* @param element transactionTypes element
* @return {@code this} builder for chained invocation
*/
public final Builder addTransactionTypes(String element) {
transactionTypesBuilder.add(element);
return this;
}
/**
* Adds elements to {@link LayoutBase#transactionTypes() transactionTypes} list.
* @param elements array of transactionTypes elements
* @return {@code this} builder for chained invocation
*/
public final Builder addTransactionTypes(String... elements) {
transactionTypesBuilder.add(elements);
return this;
}
/**
* Adds elements to {@link LayoutBase#transactionTypes() transactionTypes} list.
* @param elements iterable of transactionTypes elements
* @return {@code this} builder for chained invocation
*/
public final Builder addAllTransactionTypes(Iterable elements) {
transactionTypesBuilder.addAll(elements);
return this;
}
/**
* Initializes value for {@link LayoutBase#defaultTransactionType() defaultTransactionType}.
* @param defaultTransactionType value for defaultTransactionType
* @return {@code this} builder for chained invocation
*/
public final Builder defaultTransactionType(String defaultTransactionType) {
checkNotIsSet(defaultTransactionTypeIsSet(), "defaultTransactionType");
this.defaultTransactionType = Preconditions.checkNotNull(defaultTransactionType);
initializedBitset |= INITIALIZED_BIT_DEFAULT_TRANSACTION_TYPE;
return this;
}
/**
* Adds one element to {@link LayoutBase#defaultPercentiles() defaultPercentiles} list.
* @param element defaultPercentiles element
* @return {@code this} builder for chained invocation
*/
public final Builder addDefaultPercentiles(double element) {
defaultPercentilesBuilder.add(element);
return this;
}
/**
* Adds elements to {@link LayoutBase#defaultPercentiles() defaultPercentiles} list.
* @param elements array of defaultPercentiles elements
* @return {@code this} builder for chained invocation
*/
public final Builder addDefaultPercentiles(double... elements) {
defaultPercentilesBuilder.addAll(Doubles.asList(elements));
return this;
}
/**
* Adds elements to {@link LayoutBase#defaultPercentiles() defaultPercentiles} list.
* @param elements iterable of defaultPercentiles elements
* @return {@code this} builder for chained invocation
*/
public final Builder addAllDefaultPercentiles(Iterable elements) {
defaultPercentilesBuilder.addAll(elements);
return this;
}
/**
* Adds one element to {@link LayoutBase#transactionCustomAttributes() transactionCustomAttributes} list.
* @param element transactionCustomAttributes element
* @return {@code this} builder for chained invocation
*/
public final Builder addTransactionCustomAttributes(String element) {
transactionCustomAttributesBuilder.add(element);
return this;
}
/**
* Adds elements to {@link LayoutBase#transactionCustomAttributes() transactionCustomAttributes} list.
* @param elements array of transactionCustomAttributes elements
* @return {@code this} builder for chained invocation
*/
public final Builder addTransactionCustomAttributes(String... elements) {
transactionCustomAttributesBuilder.add(elements);
return this;
}
/**
* Adds elements to {@link LayoutBase#transactionCustomAttributes() transactionCustomAttributes} list.
* @param elements iterable of transactionCustomAttributes elements
* @return {@code this} builder for chained invocation
*/
public final Builder addAllTransactionCustomAttributes(Iterable elements) {
transactionCustomAttributesBuilder.addAll(elements);
return this;
}
/**
* Initializes value for {@link LayoutBase#fixedAggregateIntervalSeconds() fixedAggregateIntervalSeconds}.
* @param fixedAggregateIntervalSeconds value for fixedAggregateIntervalSeconds
* @return {@code this} builder for chained invocation
*/
public final Builder fixedAggregateIntervalSeconds(long fixedAggregateIntervalSeconds) {
checkNotIsSet(fixedAggregateIntervalSecondsIsSet(), "fixedAggregateIntervalSeconds");
this.fixedAggregateIntervalSeconds = fixedAggregateIntervalSeconds;
initializedBitset |= INITIALIZED_BIT_FIXED_AGGREGATE_INTERVAL_SECONDS;
return this;
}
/**
* Initializes value for {@link LayoutBase#fixedAggregateRollup1Seconds() fixedAggregateRollup1Seconds}.
* @param fixedAggregateRollup1Seconds value for fixedAggregateRollup1Seconds
* @return {@code this} builder for chained invocation
*/
public final Builder fixedAggregateRollup1Seconds(long fixedAggregateRollup1Seconds) {
checkNotIsSet(fixedAggregateRollup1SecondsIsSet(), "fixedAggregateRollup1Seconds");
this.fixedAggregateRollup1Seconds = fixedAggregateRollup1Seconds;
initializedBitset |= INITIALIZED_BIT_FIXED_AGGREGATE_ROLLUP1_SECONDS;
return this;
}
/**
* Initializes value for {@link LayoutBase#fixedAggregateRollup2Seconds() fixedAggregateRollup2Seconds}.
* @param fixedAggregateRollup2Seconds value for fixedAggregateRollup2Seconds
* @return {@code this} builder for chained invocation
*/
public final Builder fixedAggregateRollup2Seconds(long fixedAggregateRollup2Seconds) {
checkNotIsSet(fixedAggregateRollup2SecondsIsSet(), "fixedAggregateRollup2Seconds");
this.fixedAggregateRollup2Seconds = fixedAggregateRollup2Seconds;
initializedBitset |= INITIALIZED_BIT_FIXED_AGGREGATE_ROLLUP2_SECONDS;
return this;
}
/**
* Initializes value for {@link LayoutBase#fixedGaugeIntervalSeconds() fixedGaugeIntervalSeconds}.
* @param fixedGaugeIntervalSeconds value for fixedGaugeIntervalSeconds
* @return {@code this} builder for chained invocation
*/
public final Builder fixedGaugeIntervalSeconds(long fixedGaugeIntervalSeconds) {
checkNotIsSet(fixedGaugeIntervalSecondsIsSet(), "fixedGaugeIntervalSeconds");
this.fixedGaugeIntervalSeconds = fixedGaugeIntervalSeconds;
initializedBitset |= INITIALIZED_BIT_FIXED_GAUGE_INTERVAL_SECONDS;
return this;
}
/**
* Initializes value for {@link LayoutBase#fixedGaugeRollup1Seconds() fixedGaugeRollup1Seconds}.
* @param fixedGaugeRollup1Seconds value for fixedGaugeRollup1Seconds
* @return {@code this} builder for chained invocation
*/
public final Builder fixedGaugeRollup1Seconds(long fixedGaugeRollup1Seconds) {
checkNotIsSet(fixedGaugeRollup1SecondsIsSet(), "fixedGaugeRollup1Seconds");
this.fixedGaugeRollup1Seconds = fixedGaugeRollup1Seconds;
initializedBitset |= INITIALIZED_BIT_FIXED_GAUGE_ROLLUP1_SECONDS;
return this;
}
/**
* Initializes value for {@link LayoutBase#fixedGaugeRollup2Seconds() fixedGaugeRollup2Seconds}.
* @param fixedGaugeRollup2Seconds value for fixedGaugeRollup2Seconds
* @return {@code this} builder for chained invocation
*/
public final Builder fixedGaugeRollup2Seconds(long fixedGaugeRollup2Seconds) {
checkNotIsSet(fixedGaugeRollup2SecondsIsSet(), "fixedGaugeRollup2Seconds");
this.fixedGaugeRollup2Seconds = fixedGaugeRollup2Seconds;
initializedBitset |= INITIALIZED_BIT_FIXED_GAUGE_ROLLUP2_SECONDS;
return this;
}
/**
* Builds new {@link org.glowroot.local.ui.Layout}.
* @return immutable instance of Layout
*/
public org.glowroot.local.ui.Layout build() {
checkRequiredAttributes();
return new Layout(
jvmHeapDump,
footerMessage,
adminPasswordEnabled,
readOnlyPasswordEnabled,
anonymousAccess,
transactionTypesBuilder.build(),
defaultTransactionType,
defaultPercentilesBuilder.build(),
transactionCustomAttributesBuilder.build(),
fixedAggregateIntervalSeconds,
fixedAggregateRollup1Seconds,
fixedAggregateRollup2Seconds,
fixedGaugeIntervalSeconds,
fixedGaugeRollup1Seconds,
fixedGaugeRollup2Seconds);
}
private boolean jvmHeapDumpIsSet() {
return (initializedBitset & INITIALIZED_BIT_JVM_HEAP_DUMP) != 0;
}
private boolean footerMessageIsSet() {
return (initializedBitset & INITIALIZED_BIT_FOOTER_MESSAGE) != 0;
}
private boolean adminPasswordEnabledIsSet() {
return (initializedBitset & INITIALIZED_BIT_ADMIN_PASSWORD_ENABLED) != 0;
}
private boolean readOnlyPasswordEnabledIsSet() {
return (initializedBitset & INITIALIZED_BIT_READ_ONLY_PASSWORD_ENABLED) != 0;
}
private boolean anonymousAccessIsSet() {
return (initializedBitset & INITIALIZED_BIT_ANONYMOUS_ACCESS) != 0;
}
private boolean defaultTransactionTypeIsSet() {
return (initializedBitset & INITIALIZED_BIT_DEFAULT_TRANSACTION_TYPE) != 0;
}
private boolean fixedAggregateIntervalSecondsIsSet() {
return (initializedBitset & INITIALIZED_BIT_FIXED_AGGREGATE_INTERVAL_SECONDS) != 0;
}
private boolean fixedAggregateRollup1SecondsIsSet() {
return (initializedBitset & INITIALIZED_BIT_FIXED_AGGREGATE_ROLLUP1_SECONDS) != 0;
}
private boolean fixedAggregateRollup2SecondsIsSet() {
return (initializedBitset & INITIALIZED_BIT_FIXED_AGGREGATE_ROLLUP2_SECONDS) != 0;
}
private boolean fixedGaugeIntervalSecondsIsSet() {
return (initializedBitset & INITIALIZED_BIT_FIXED_GAUGE_INTERVAL_SECONDS) != 0;
}
private boolean fixedGaugeRollup1SecondsIsSet() {
return (initializedBitset & INITIALIZED_BIT_FIXED_GAUGE_ROLLUP1_SECONDS) != 0;
}
private boolean fixedGaugeRollup2SecondsIsSet() {
return (initializedBitset & INITIALIZED_BIT_FIXED_GAUGE_ROLLUP2_SECONDS) != 0;
}
private void checkNotIsSet(boolean isSet, String name) {
if (isSet) {
throw new IllegalStateException("Builder of Layout is strict, attribute is already set: ".concat(name));
}
}
private void checkRequiredAttributes() {
if (initializedBitset != INITIALIZED_BITSET_ALL) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
Collection attributes = Lists.newArrayList();
if (!jvmHeapDumpIsSet()) {
attributes.add("jvmHeapDump");
}
if (!footerMessageIsSet()) {
attributes.add("footerMessage");
}
if (!adminPasswordEnabledIsSet()) {
attributes.add("adminPasswordEnabled");
}
if (!readOnlyPasswordEnabledIsSet()) {
attributes.add("readOnlyPasswordEnabled");
}
if (!anonymousAccessIsSet()) {
attributes.add("anonymousAccess");
}
if (!defaultTransactionTypeIsSet()) {
attributes.add("defaultTransactionType");
}
if (!fixedAggregateIntervalSecondsIsSet()) {
attributes.add("fixedAggregateIntervalSeconds");
}
if (!fixedAggregateRollup1SecondsIsSet()) {
attributes.add("fixedAggregateRollup1Seconds");
}
if (!fixedAggregateRollup2SecondsIsSet()) {
attributes.add("fixedAggregateRollup2Seconds");
}
if (!fixedGaugeIntervalSecondsIsSet()) {
attributes.add("fixedGaugeIntervalSeconds");
}
if (!fixedGaugeRollup1SecondsIsSet()) {
attributes.add("fixedGaugeRollup1Seconds");
}
if (!fixedGaugeRollup2SecondsIsSet()) {
attributes.add("fixedGaugeRollup2Seconds");
}
return "Cannot build Layout, some of required attributes are not set " + attributes;
}
}
}