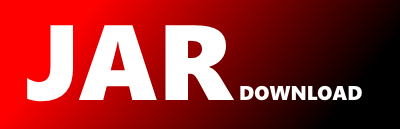
org.glowroot.MainEntryPoint Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2011-2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.glowroot;
import java.io.File;
import java.lang.instrument.Instrumentation;
import java.lang.management.ManagementFactory;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import javax.annotation.Nullable;
import org.glowroot.shaded.google.common.annotations.VisibleForTesting;
import org.glowroot.shaded.google.common.base.Joiner;
import org.glowroot.shaded.google.common.base.Objects;
import org.glowroot.shaded.google.common.base.Strings;
import org.glowroot.shaded.google.common.collect.ImmutableMap;
import org.glowroot.shaded.google.common.collect.Lists;
import org.checkerframework.checker.nullness.qual.MonotonicNonNull;
import org.glowroot.shaded.slf4j.Logger;
import org.glowroot.shaded.slf4j.LoggerFactory;
import org.glowroot.GlowrootModule.BaseDirLockedException;
import org.glowroot.common.JavaVersion;
import org.glowroot.config.PluginDescriptor;
import org.glowroot.markers.OnlyUsedByTests;
public class MainEntryPoint {
// log startup messages using logger name "org.glowroot"
private static final Logger startupLogger = LoggerFactory.getLogger("org.glowroot");
// this static field is only present for tests
private static volatile @MonotonicNonNull GlowrootModule glowrootModule;
private MainEntryPoint() {}
public static void premain(Instrumentation instrumentation, @Nullable File glowrootJarFile) {
boolean jbossModules = isJBossModules();
if (jbossModules) {
String jbossModulesSystemPkgs = System.getProperty("jboss.modules.system.pkgs");
if (Strings.isNullOrEmpty(jbossModulesSystemPkgs)) {
jbossModulesSystemPkgs = "org.glowroot";
} else {
jbossModulesSystemPkgs += ",org.glowroot";
}
System.setProperty("jboss.modules.system.pkgs", jbossModulesSystemPkgs);
}
ImmutableMap properties = getGlowrootProperties();
File baseDir = BaseDir.getBaseDir(properties, glowrootJarFile);
try {
start(baseDir, properties, instrumentation, glowrootJarFile, jbossModules);
} catch (BaseDirLockedException e) {
logBaseDirLockedException(baseDir);
} catch (Throwable t) {
// log error but don't re-throw which would prevent monitored app from starting
startupLogger.error("Glowroot not started: {}", t.getMessage(), t);
}
}
static void runViewer(@Nullable File glowrootJarFile) throws InterruptedException {
ImmutableMap properties = getGlowrootProperties();
File baseDir = BaseDir.getBaseDir(properties, glowrootJarFile);
String version = Version.getVersion();
try {
glowrootModule = new GlowrootModule(baseDir, properties, null, glowrootJarFile, version,
true, false);
} catch (BaseDirLockedException e) {
logBaseDirLockedException(baseDir);
return;
} catch (Throwable t) {
startupLogger.error("Viewer cannot start: {}", t.getMessage(), t);
return;
}
startupLogger.info("Viewer started (version {})", version);
startupLogger.info("Viewer listening at http://localhost:{}",
glowrootModule.getUiModule().getPort());
// Glowroot does not create any non-daemon threads, so need to block jvm from exiting when
// running the viewer
Thread.sleep(Long.MAX_VALUE);
}
private static void start(File baseDir, Map properties,
@Nullable Instrumentation instrumentation, @Nullable File glowrootJarFile,
boolean jbossModules) throws Exception {
ManagementFactory.getThreadMXBean().setThreadCpuTimeEnabled(true);
ManagementFactory.getThreadMXBean().setThreadContentionMonitoringEnabled(true);
String version = Version.getVersion();
glowrootModule = new GlowrootModule(baseDir, properties, instrumentation, glowrootJarFile,
version, false, jbossModules);
startupLogger.info("Glowroot started (version {})", version);
List pluginDescriptors =
glowrootModule.getConfigModule().getPluginDescriptors();
List pluginNames = Lists.newArrayList();
for (PluginDescriptor pluginDescriptor : pluginDescriptors) {
pluginNames.add(pluginDescriptor.name());
}
if (!pluginNames.isEmpty()) {
startupLogger.info("Glowroot plugins loaded: {}", Joiner.on(", ").join(pluginNames));
}
if (instrumentation == null || JavaVersion.isJava6()) {
// otherwise http server is lazy instantiated, see LocalUiModule
startupLogger.info("Glowroot listening at http://localhost:{}",
glowrootModule.getUiModule().getNonLazyPort());
}
}
private static ImmutableMap getGlowrootProperties() {
ImmutableMap.Builder builder = ImmutableMap.builder();
for (Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy