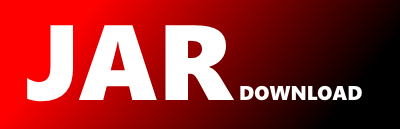
org.glowroot.collector.Trace Maven / Gradle / Ivy
package org.glowroot.collector;
import org.glowroot.shaded.fasterxml.jackson.annotation.JsonCreator;
import org.glowroot.shaded.fasterxml.jackson.annotation.JsonIgnore;
import org.glowroot.shaded.fasterxml.jackson.annotation.JsonProperty;
import org.glowroot.shaded.fasterxml.jackson.annotation.JsonRawValue;
import org.glowroot.shaded.google.common.base.MoreObjects;
import org.glowroot.shaded.google.common.base.Objects;
import org.glowroot.shaded.google.common.base.Preconditions;
import org.glowroot.shaded.google.common.collect.ImmutableSetMultimap;
import org.glowroot.shaded.google.common.collect.Lists;
import org.glowroot.shaded.google.common.collect.Multimap;
import org.glowroot.shaded.google.common.primitives.Booleans;
import org.glowroot.shaded.google.common.primitives.Longs;
import java.util.Arrays;
import java.util.Collection;
import java.util.Map;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link TraceBase}.
*
* Use builder to create immutable instances:
* {@code Trace.builder()}.
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "TraceBase"})
@Immutable
public final class Trace extends TraceBase {
private final String id;
private final boolean active;
private final boolean partial;
private final boolean error;
private final long startTime;
private final long captureTime;
private final long duration;
private final String transactionType;
private final String transactionName;
private final String headline;
private final @Nullable String user;
private final @Nullable String customAttributes;
private final @Nullable String customDetail;
private final @Nullable String errorMessage;
private final @Nullable String errorThrowable;
private final @Nullable String timers;
private final @Nullable Long threadCpuTime;
private final @Nullable Long threadBlockedTime;
private final @Nullable Long threadWaitedTime;
private final @Nullable Long threadAllocatedBytes;
private final @Nullable String gcInfos;
private final int entryCount;
private final long profileSampleCount;
private final Existence entriesExistence;
private final Existence profileExistence;
private final ImmutableSetMultimap customAttributesForIndexing;
private Trace(
String id,
boolean active,
boolean partial,
boolean error,
long startTime,
long captureTime,
long duration,
String transactionType,
String transactionName,
String headline,
@Nullable String user,
@Nullable String customAttributes,
@Nullable String customDetail,
@Nullable String errorMessage,
@Nullable String errorThrowable,
@Nullable String timers,
@Nullable Long threadCpuTime,
@Nullable Long threadBlockedTime,
@Nullable Long threadWaitedTime,
@Nullable Long threadAllocatedBytes,
@Nullable String gcInfos,
int entryCount,
long profileSampleCount,
Existence entriesExistence,
Existence profileExistence,
ImmutableSetMultimap customAttributesForIndexing) {
this.id = id;
this.active = active;
this.partial = partial;
this.error = error;
this.startTime = startTime;
this.captureTime = captureTime;
this.duration = duration;
this.transactionType = transactionType;
this.transactionName = transactionName;
this.headline = headline;
this.user = user;
this.customAttributes = customAttributes;
this.customDetail = customDetail;
this.errorMessage = errorMessage;
this.errorThrowable = errorThrowable;
this.timers = timers;
this.threadCpuTime = threadCpuTime;
this.threadBlockedTime = threadBlockedTime;
this.threadWaitedTime = threadWaitedTime;
this.threadAllocatedBytes = threadAllocatedBytes;
this.gcInfos = gcInfos;
this.entryCount = entryCount;
this.profileSampleCount = profileSampleCount;
this.entriesExistence = entriesExistence;
this.profileExistence = profileExistence;
this.customAttributesForIndexing = customAttributesForIndexing;
}
/**
* {@inheritDoc}
* @return value of {@code id} attribute
*/
@JsonProperty("id")
@Override
public String id() {
return id;
}
/**
* {@inheritDoc}
* @return value of {@code active} attribute
*/
@JsonProperty("active")
@Override
public boolean active() {
return active;
}
/**
* {@inheritDoc}
* @return value of {@code partial} attribute
*/
@JsonProperty("partial")
@Override
public boolean partial() {
return partial;
}
/**
* {@inheritDoc}
* @return value of {@code error} attribute
*/
@JsonProperty("error")
@Override
public boolean error() {
return error;
}
/**
* {@inheritDoc}
* @return value of {@code startTime} attribute
*/
@JsonProperty("startTime")
@Override
public long startTime() {
return startTime;
}
/**
* {@inheritDoc}
* @return value of {@code captureTime} attribute
*/
@JsonProperty("captureTime")
@Override
public long captureTime() {
return captureTime;
}
/**
* {@inheritDoc}
* @return value of {@code duration} attribute
*/
@JsonProperty("duration")
@Override
public long duration() {
return duration;
}
/**
* {@inheritDoc}
* @return value of {@code transactionType} attribute
*/
@JsonProperty("transactionType")
@Override
public String transactionType() {
return transactionType;
}
/**
* {@inheritDoc}
* @return value of {@code transactionName} attribute
*/
@JsonProperty("transactionName")
@Override
public String transactionName() {
return transactionName;
}
/**
* {@inheritDoc}
* @return value of {@code headline} attribute
*/
@JsonProperty("headline")
@Override
public String headline() {
return headline;
}
/**
* {@inheritDoc}
* @return value of {@code user} attribute
*/
@JsonProperty("user")
@Override
public String user() {
return user;
}
/**
* {@inheritDoc}
* @return value of {@code customAttributes} attribute
*/
@JsonRawValue
@JsonProperty("customAttributes")
@Override
public String customAttributes() {
return customAttributes;
}
/**
* {@inheritDoc}
* @return value of {@code customDetail} attribute
*/
@JsonRawValue
@JsonProperty("customDetail")
@Override
public String customDetail() {
return customDetail;
}
/**
* {@inheritDoc}
* @return value of {@code errorMessage} attribute
*/
@JsonProperty("errorMessage")
@Override
public String errorMessage() {
return errorMessage;
}
/**
* {@inheritDoc}
* @return value of {@code errorThrowable} attribute
*/
@JsonRawValue
@JsonProperty("errorThrowable")
@Override
public String errorThrowable() {
return errorThrowable;
}
/**
* {@inheritDoc}
* @return value of {@code timers} attribute
*/
@JsonRawValue
@JsonProperty("timers")
@Override
public String timers() {
return timers;
}
/**
* {@inheritDoc}
* @return value of {@code threadCpuTime} attribute
*/
@JsonProperty("threadCpuTime")
@Override
public Long threadCpuTime() {
return threadCpuTime;
}
/**
* {@inheritDoc}
* @return value of {@code threadBlockedTime} attribute
*/
@JsonProperty("threadBlockedTime")
@Override
public Long threadBlockedTime() {
return threadBlockedTime;
}
/**
* {@inheritDoc}
* @return value of {@code threadWaitedTime} attribute
*/
@JsonProperty("threadWaitedTime")
@Override
public Long threadWaitedTime() {
return threadWaitedTime;
}
/**
* {@inheritDoc}
* @return value of {@code threadAllocatedBytes} attribute
*/
@JsonProperty("threadAllocatedBytes")
@Override
public Long threadAllocatedBytes() {
return threadAllocatedBytes;
}
/**
* {@inheritDoc}
* @return value of {@code gcInfos} attribute
*/
@JsonRawValue
@JsonProperty("gcInfos")
@Override
public String gcInfos() {
return gcInfos;
}
/**
* {@inheritDoc}
* @return value of {@code entryCount} attribute
*/
@JsonProperty("entryCount")
@Override
public int entryCount() {
return entryCount;
}
/**
* {@inheritDoc}
* @return value of {@code profileSampleCount} attribute
*/
@JsonProperty("profileSampleCount")
@Override
public long profileSampleCount() {
return profileSampleCount;
}
/**
* {@inheritDoc}
* @return value of {@code entriesExistence} attribute
*/
@JsonProperty("entriesExistence")
@Override
public Existence entriesExistence() {
return entriesExistence;
}
/**
* {@inheritDoc}
* @return value of {@code profileExistence} attribute
*/
@JsonProperty("profileExistence")
@Override
public Existence profileExistence() {
return profileExistence;
}
/**
* {@inheritDoc}
* @return value of {@code customAttributesForIndexing} attribute
*/
@JsonIgnore
@JsonProperty("customAttributesForIndexing")
@Override
public ImmutableSetMultimap customAttributesForIndexing() {
return customAttributesForIndexing;
}
/**
* Copy current immutable object by setting value for {@link TraceBase#id() id}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for id
* @return modified copy of the {@code this} object
*/
public final Trace withId(String value) {
if (this.id == value) {
return this;
}
String newValue = Preconditions.checkNotNull(value);
return new Trace(
newValue,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#active() active}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for active
* @return modified copy of the {@code this} object
*/
public final Trace withActive(boolean value) {
if (this.active == value) {
return this;
}
boolean newValue = value;
return new Trace(
this.id,
newValue,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#partial() partial}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for partial
* @return modified copy of the {@code this} object
*/
public final Trace withPartial(boolean value) {
if (this.partial == value) {
return this;
}
boolean newValue = value;
return new Trace(
this.id,
this.active,
newValue,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#error() error}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for error
* @return modified copy of the {@code this} object
*/
public final Trace withError(boolean value) {
if (this.error == value) {
return this;
}
boolean newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
newValue,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#startTime() startTime}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for startTime
* @return modified copy of the {@code this} object
*/
public final Trace withStartTime(long value) {
if (this.startTime == value) {
return this;
}
long newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
newValue,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#captureTime() captureTime}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for captureTime
* @return modified copy of the {@code this} object
*/
public final Trace withCaptureTime(long value) {
if (this.captureTime == value) {
return this;
}
long newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
newValue,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#duration() duration}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for duration
* @return modified copy of the {@code this} object
*/
public final Trace withDuration(long value) {
if (this.duration == value) {
return this;
}
long newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
newValue,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#transactionType() transactionType}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for transactionType
* @return modified copy of the {@code this} object
*/
public final Trace withTransactionType(String value) {
if (this.transactionType == value) {
return this;
}
String newValue = Preconditions.checkNotNull(value);
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
newValue,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#transactionName() transactionName}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for transactionName
* @return modified copy of the {@code this} object
*/
public final Trace withTransactionName(String value) {
if (this.transactionName == value) {
return this;
}
String newValue = Preconditions.checkNotNull(value);
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
newValue,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#headline() headline}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for headline
* @return modified copy of the {@code this} object
*/
public final Trace withHeadline(String value) {
if (this.headline == value) {
return this;
}
String newValue = Preconditions.checkNotNull(value);
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
newValue,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#user() user}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for user, can be {@code null}
* @return modified copy of the {@code this} object
*/
public final Trace withUser(@Nullable String value) {
if (this.user == value) {
return this;
}
@Nullable String newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
newValue,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#customAttributes() customAttributes}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for customAttributes, can be {@code null}
* @return modified copy of the {@code this} object
*/
public final Trace withCustomAttributes(@Nullable String value) {
if (this.customAttributes == value) {
return this;
}
@Nullable String newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
newValue,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#customDetail() customDetail}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for customDetail, can be {@code null}
* @return modified copy of the {@code this} object
*/
public final Trace withCustomDetail(@Nullable String value) {
if (this.customDetail == value) {
return this;
}
@Nullable String newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
newValue,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#errorMessage() errorMessage}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for errorMessage, can be {@code null}
* @return modified copy of the {@code this} object
*/
public final Trace withErrorMessage(@Nullable String value) {
if (this.errorMessage == value) {
return this;
}
@Nullable String newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
newValue,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#errorThrowable() errorThrowable}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for errorThrowable, can be {@code null}
* @return modified copy of the {@code this} object
*/
public final Trace withErrorThrowable(@Nullable String value) {
if (this.errorThrowable == value) {
return this;
}
@Nullable String newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
newValue,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#timers() timers}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for timers, can be {@code null}
* @return modified copy of the {@code this} object
*/
public final Trace withTimers(@Nullable String value) {
if (this.timers == value) {
return this;
}
@Nullable String newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
newValue,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#threadCpuTime() threadCpuTime}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for threadCpuTime, can be {@code null}
* @return modified copy of the {@code this} object
*/
public final Trace withThreadCpuTime(@Nullable Long value) {
if (this.threadCpuTime == value) {
return this;
}
@Nullable Long newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
newValue,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#threadBlockedTime() threadBlockedTime}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for threadBlockedTime, can be {@code null}
* @return modified copy of the {@code this} object
*/
public final Trace withThreadBlockedTime(@Nullable Long value) {
if (this.threadBlockedTime == value) {
return this;
}
@Nullable Long newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
newValue,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#threadWaitedTime() threadWaitedTime}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for threadWaitedTime, can be {@code null}
* @return modified copy of the {@code this} object
*/
public final Trace withThreadWaitedTime(@Nullable Long value) {
if (this.threadWaitedTime == value) {
return this;
}
@Nullable Long newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
newValue,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#threadAllocatedBytes() threadAllocatedBytes}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for threadAllocatedBytes, can be {@code null}
* @return modified copy of the {@code this} object
*/
public final Trace withThreadAllocatedBytes(@Nullable Long value) {
if (this.threadAllocatedBytes == value) {
return this;
}
@Nullable Long newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
newValue,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#gcInfos() gcInfos}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for gcInfos, can be {@code null}
* @return modified copy of the {@code this} object
*/
public final Trace withGcInfos(@Nullable String value) {
if (this.gcInfos == value) {
return this;
}
@Nullable String newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
newValue,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#entryCount() entryCount}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for entryCount
* @return modified copy of the {@code this} object
*/
public final Trace withEntryCount(int value) {
if (this.entryCount == value) {
return this;
}
int newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
newValue,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#profileSampleCount() profileSampleCount}.
* Value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for profileSampleCount
* @return modified copy of the {@code this} object
*/
public final Trace withProfileSampleCount(long value) {
if (this.profileSampleCount == value) {
return this;
}
long newValue = value;
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
newValue,
this.entriesExistence,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#entriesExistence() entriesExistence}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for entriesExistence
* @return modified copy of the {@code this} object
*/
public final Trace withEntriesExistence(Existence value) {
if (this.entriesExistence == value) {
return this;
}
Existence newValue = Preconditions.checkNotNull(value);
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
newValue,
this.profileExistence,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by setting value for {@link TraceBase#profileExistence() profileExistence}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for profileExistence
* @return modified copy of the {@code this} object
*/
public final Trace withProfileExistence(Existence value) {
if (this.profileExistence == value) {
return this;
}
Existence newValue = Preconditions.checkNotNull(value);
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
newValue,
this.customAttributesForIndexing);
}
/**
* Copy current immutable object by replacing {@link TraceBase#customAttributesForIndexing() customAttributesForIndexing} map with specified map.
* Nulls are not permitted as keys or values.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries to be added to customAttributesForIndexing map
* @return modified copy of {@code this} object
*/
public final Trace withCustomAttributesForIndexing(Multimap entries) {
if (this.customAttributesForIndexing == entries) {
return this;
}
ImmutableSetMultimap newValue = ImmutableSetMultimap.copyOf(entries);
return new Trace(
this.id,
this.active,
this.partial,
this.error,
this.startTime,
this.captureTime,
this.duration,
this.transactionType,
this.transactionName,
this.headline,
this.user,
this.customAttributes,
this.customDetail,
this.errorMessage,
this.errorThrowable,
this.timers,
this.threadCpuTime,
this.threadBlockedTime,
this.threadWaitedTime,
this.threadAllocatedBytes,
this.gcInfos,
this.entryCount,
this.profileSampleCount,
this.entriesExistence,
this.profileExistence,
newValue);
}
/**
* This instance is equal to instances of {@code Trace} with equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
return this == another
|| (another instanceof Trace && equalTo((Trace) another));
}
private boolean equalTo(Trace another) {
return id.equals(another.id)
&& active == another.active
&& partial == another.partial
&& error == another.error
&& startTime == another.startTime
&& captureTime == another.captureTime
&& duration == another.duration
&& transactionType.equals(another.transactionType)
&& transactionName.equals(another.transactionName)
&& headline.equals(another.headline)
&& Objects.equal(user, another.user)
&& Objects.equal(customAttributes, another.customAttributes)
&& Objects.equal(customDetail, another.customDetail)
&& Objects.equal(errorMessage, another.errorMessage)
&& Objects.equal(errorThrowable, another.errorThrowable)
&& Objects.equal(timers, another.timers)
&& Objects.equal(threadCpuTime, another.threadCpuTime)
&& Objects.equal(threadBlockedTime, another.threadBlockedTime)
&& Objects.equal(threadWaitedTime, another.threadWaitedTime)
&& Objects.equal(threadAllocatedBytes, another.threadAllocatedBytes)
&& Objects.equal(gcInfos, another.gcInfos)
&& entryCount == another.entryCount
&& profileSampleCount == another.profileSampleCount
&& entriesExistence.equals(another.entriesExistence)
&& profileExistence.equals(another.profileExistence)
&& customAttributesForIndexing.equals(another.customAttributesForIndexing);
}
/**
* Computes hash code from attributes: {@code id}, {@code active}, {@code partial}, {@code error}, {@code startTime}, {@code captureTime}, {@code duration}, {@code transactionType}, {@code transactionName}, {@code headline}, {@code user}, {@code customAttributes}, {@code customDetail}, {@code errorMessage}, {@code errorThrowable}, {@code timers}, {@code threadCpuTime}, {@code threadBlockedTime}, {@code threadWaitedTime}, {@code threadAllocatedBytes}, {@code gcInfos}, {@code entryCount}, {@code profileSampleCount}, {@code entriesExistence}, {@code profileExistence}, {@code customAttributesForIndexing}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + id.hashCode();
h = h * 17 + Booleans.hashCode(active);
h = h * 17 + Booleans.hashCode(partial);
h = h * 17 + Booleans.hashCode(error);
h = h * 17 + Longs.hashCode(startTime);
h = h * 17 + Longs.hashCode(captureTime);
h = h * 17 + Longs.hashCode(duration);
h = h * 17 + transactionType.hashCode();
h = h * 17 + transactionName.hashCode();
h = h * 17 + headline.hashCode();
h = h * 17 + Objects.hashCode(user);
h = h * 17 + Objects.hashCode(customAttributes);
h = h * 17 + Objects.hashCode(customDetail);
h = h * 17 + Objects.hashCode(errorMessage);
h = h * 17 + Objects.hashCode(errorThrowable);
h = h * 17 + Objects.hashCode(timers);
h = h * 17 + Objects.hashCode(threadCpuTime);
h = h * 17 + Objects.hashCode(threadBlockedTime);
h = h * 17 + Objects.hashCode(threadWaitedTime);
h = h * 17 + Objects.hashCode(threadAllocatedBytes);
h = h * 17 + Objects.hashCode(gcInfos);
h = h * 17 + entryCount;
h = h * 17 + Longs.hashCode(profileSampleCount);
h = h * 17 + entriesExistence.hashCode();
h = h * 17 + profileExistence.hashCode();
h = h * 17 + customAttributesForIndexing.hashCode();
return h;
}
/**
* Prints immutable value {@code Trace{...}} with attribute values,
* excluding any non-generated and auxiliary attributes.
* @return string representation of value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Trace")
.add("id", id)
.add("active", active)
.add("partial", partial)
.add("error", error)
.add("startTime", startTime)
.add("captureTime", captureTime)
.add("duration", duration)
.add("transactionType", transactionType)
.add("transactionName", transactionName)
.add("headline", headline)
.add("user", user)
.add("customAttributes", customAttributes)
.add("customDetail", customDetail)
.add("errorMessage", errorMessage)
.add("errorThrowable", errorThrowable)
.add("timers", timers)
.add("threadCpuTime", threadCpuTime)
.add("threadBlockedTime", threadBlockedTime)
.add("threadWaitedTime", threadWaitedTime)
.add("threadAllocatedBytes", threadAllocatedBytes)
.add("gcInfos", gcInfos)
.add("entryCount", entryCount)
.add("profileSampleCount", profileSampleCount)
.add("entriesExistence", entriesExistence)
.add("profileExistence", profileExistence)
.add("customAttributesForIndexing", customAttributesForIndexing)
.toString();
}
@JsonCreator
public static Trace fromAllAttributes(
@JsonProperty("id") @Nullable String id,
@JsonProperty("active") @Nullable Boolean active,
@JsonProperty("partial") @Nullable Boolean partial,
@JsonProperty("error") @Nullable Boolean error,
@JsonProperty("startTime") @Nullable Long startTime,
@JsonProperty("captureTime") @Nullable Long captureTime,
@JsonProperty("duration") @Nullable Long duration,
@JsonProperty("transactionType") @Nullable String transactionType,
@JsonProperty("transactionName") @Nullable String transactionName,
@JsonProperty("headline") @Nullable String headline,
@JsonProperty("user") @Nullable String user,
@JsonProperty("customAttributes") @Nullable String customAttributes,
@JsonProperty("customDetail") @Nullable String customDetail,
@JsonProperty("errorMessage") @Nullable String errorMessage,
@JsonProperty("errorThrowable") @Nullable String errorThrowable,
@JsonProperty("timers") @Nullable String timers,
@JsonProperty("threadCpuTime") @Nullable Long threadCpuTime,
@JsonProperty("threadBlockedTime") @Nullable Long threadBlockedTime,
@JsonProperty("threadWaitedTime") @Nullable Long threadWaitedTime,
@JsonProperty("threadAllocatedBytes") @Nullable Long threadAllocatedBytes,
@JsonProperty("gcInfos") @Nullable String gcInfos,
@JsonProperty("entryCount") @Nullable Integer entryCount,
@JsonProperty("profileSampleCount") @Nullable Long profileSampleCount,
@JsonProperty("entriesExistence") @Nullable Existence entriesExistence,
@JsonProperty("profileExistence") @Nullable Existence profileExistence,
@JsonProperty("customAttributesForIndexing") @Nullable ImmutableSetMultimap customAttributesForIndexing) {
Trace.Builder builder = Trace.builder();
if (id != null) {
builder.id(id);
}
if (active != null) {
builder.active(active);
}
if (partial != null) {
builder.partial(partial);
}
if (error != null) {
builder.error(error);
}
if (startTime != null) {
builder.startTime(startTime);
}
if (captureTime != null) {
builder.captureTime(captureTime);
}
if (duration != null) {
builder.duration(duration);
}
if (transactionType != null) {
builder.transactionType(transactionType);
}
if (transactionName != null) {
builder.transactionName(transactionName);
}
if (headline != null) {
builder.headline(headline);
}
if (user != null) {
builder.user(user);
}
if (customAttributes != null) {
builder.customAttributes(customAttributes);
}
if (customDetail != null) {
builder.customDetail(customDetail);
}
if (errorMessage != null) {
builder.errorMessage(errorMessage);
}
if (errorThrowable != null) {
builder.errorThrowable(errorThrowable);
}
if (timers != null) {
builder.timers(timers);
}
if (threadCpuTime != null) {
builder.threadCpuTime(threadCpuTime);
}
if (threadBlockedTime != null) {
builder.threadBlockedTime(threadBlockedTime);
}
if (threadWaitedTime != null) {
builder.threadWaitedTime(threadWaitedTime);
}
if (threadAllocatedBytes != null) {
builder.threadAllocatedBytes(threadAllocatedBytes);
}
if (gcInfos != null) {
builder.gcInfos(gcInfos);
}
if (entryCount != null) {
builder.entryCount(entryCount);
}
if (profileSampleCount != null) {
builder.profileSampleCount(profileSampleCount);
}
if (entriesExistence != null) {
builder.entriesExistence(entriesExistence);
}
if (profileExistence != null) {
builder.profileExistence(profileExistence);
}
if (customAttributesForIndexing != null) {
builder.putAllCustomAttributesForIndexing(customAttributesForIndexing);
}
return builder.build();
}
/**
* Creates immutable copy of {@link TraceBase}.
* Uses accessors to get values to initialize immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance instance to copy
* @return copied immutable Trace instance
*/
public static Trace copyOf(TraceBase instance) {
if (instance instanceof Trace) {
return (Trace) instance;
}
return Trace.builder()
.id(instance.id())
.active(instance.active())
.partial(instance.partial())
.error(instance.error())
.startTime(instance.startTime())
.captureTime(instance.captureTime())
.duration(instance.duration())
.transactionType(instance.transactionType())
.transactionName(instance.transactionName())
.headline(instance.headline())
.user(instance.user())
.customAttributes(instance.customAttributes())
.customDetail(instance.customDetail())
.errorMessage(instance.errorMessage())
.errorThrowable(instance.errorThrowable())
.timers(instance.timers())
.threadCpuTime(instance.threadCpuTime())
.threadBlockedTime(instance.threadBlockedTime())
.threadWaitedTime(instance.threadWaitedTime())
.threadAllocatedBytes(instance.threadAllocatedBytes())
.gcInfos(instance.gcInfos())
.entryCount(instance.entryCount())
.profileSampleCount(instance.profileSampleCount())
.entriesExistence(instance.entriesExistence())
.profileExistence(instance.profileExistence())
.putAllCustomAttributesForIndexing(instance.customAttributesForIndexing())
.build();
}
/**
* Creates builder for {@link org.glowroot.collector.Trace}.
* @return new Trace builder
*/
public static Trace.Builder builder() {
return new Trace.Builder();
}
/**
* Builds instances of {@link org.glowroot.collector.Trace}.
* Initialized attributes and then invoke {@link #build()} method to create
* immutable instance.
* Builder is not thread safe and generally should not be stored in field or collection,
* but used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private static final long INITIALIZED_BITSET_ALL = 0x3fff;
private static final long INITIALIZED_BIT_ID = 0x1L;
private static final long INITIALIZED_BIT_ACTIVE = 0x2L;
private static final long INITIALIZED_BIT_PARTIAL = 0x4L;
private static final long INITIALIZED_BIT_ERROR = 0x8L;
private static final long INITIALIZED_BIT_START_TIME = 0x10L;
private static final long INITIALIZED_BIT_CAPTURE_TIME = 0x20L;
private static final long INITIALIZED_BIT_DURATION = 0x40L;
private static final long INITIALIZED_BIT_TRANSACTION_TYPE = 0x80L;
private static final long INITIALIZED_BIT_TRANSACTION_NAME = 0x100L;
private static final long INITIALIZED_BIT_HEADLINE = 0x200L;
private static final long INITIALIZED_BIT_ENTRY_COUNT = 0x400L;
private static final long INITIALIZED_BIT_PROFILE_SAMPLE_COUNT = 0x800L;
private static final long INITIALIZED_BIT_ENTRIES_EXISTENCE = 0x1000L;
private static final long INITIALIZED_BIT_PROFILE_EXISTENCE = 0x2000L;
private static final long NONDEFAULT_BIT_USER = 0x1L;
private static final long NONDEFAULT_BIT_CUSTOM_ATTRIBUTES = 0x2L;
private static final long NONDEFAULT_BIT_CUSTOM_DETAIL = 0x4L;
private static final long NONDEFAULT_BIT_ERROR_MESSAGE = 0x8L;
private static final long NONDEFAULT_BIT_ERROR_THROWABLE = 0x10L;
private static final long NONDEFAULT_BIT_TIMERS = 0x20L;
private static final long NONDEFAULT_BIT_THREAD_CPU_TIME = 0x40L;
private static final long NONDEFAULT_BIT_THREAD_BLOCKED_TIME = 0x80L;
private static final long NONDEFAULT_BIT_THREAD_WAITED_TIME = 0x100L;
private static final long NONDEFAULT_BIT_THREAD_ALLOCATED_BYTES = 0x200L;
private static final long NONDEFAULT_BIT_GC_INFOS = 0x400L;
private long initializedBitset;
private long nondefaultBitset;
private @Nullable String id;
private boolean active;
private boolean partial;
private boolean error;
private long startTime;
private long captureTime;
private long duration;
private @Nullable String transactionType;
private @Nullable String transactionName;
private @Nullable String headline;
private @Nullable String user;
private @Nullable String customAttributes;
private @Nullable String customDetail;
private @Nullable String errorMessage;
private @Nullable String errorThrowable;
private @Nullable String timers;
private @Nullable Long threadCpuTime;
private @Nullable Long threadBlockedTime;
private @Nullable Long threadWaitedTime;
private @Nullable Long threadAllocatedBytes;
private @Nullable String gcInfos;
private int entryCount;
private long profileSampleCount;
private @Nullable Existence entriesExistence;
private @Nullable Existence profileExistence;
private ImmutableSetMultimap.Builder customAttributesForIndexingBuilder = ImmutableSetMultimap.builder();
private Builder() {}
/**
* Initializes value for {@link TraceBase#id() id}.
* @param id value for id
* @return {@code this} builder for chained invocation
*/
public final Builder id(String id) {
checkNotIsSet(idIsSet(), "id");
this.id = Preconditions.checkNotNull(id);
initializedBitset |= INITIALIZED_BIT_ID;
return this;
}
/**
* Initializes value for {@link TraceBase#active() active}.
* @param active value for active
* @return {@code this} builder for chained invocation
*/
public final Builder active(boolean active) {
checkNotIsSet(activeIsSet(), "active");
this.active = active;
initializedBitset |= INITIALIZED_BIT_ACTIVE;
return this;
}
/**
* Initializes value for {@link TraceBase#partial() partial}.
* @param partial value for partial
* @return {@code this} builder for chained invocation
*/
public final Builder partial(boolean partial) {
checkNotIsSet(partialIsSet(), "partial");
this.partial = partial;
initializedBitset |= INITIALIZED_BIT_PARTIAL;
return this;
}
/**
* Initializes value for {@link TraceBase#error() error}.
* @param error value for error
* @return {@code this} builder for chained invocation
*/
public final Builder error(boolean error) {
checkNotIsSet(errorIsSet(), "error");
this.error = error;
initializedBitset |= INITIALIZED_BIT_ERROR;
return this;
}
/**
* Initializes value for {@link TraceBase#startTime() startTime}.
* @param startTime value for startTime
* @return {@code this} builder for chained invocation
*/
public final Builder startTime(long startTime) {
checkNotIsSet(startTimeIsSet(), "startTime");
this.startTime = startTime;
initializedBitset |= INITIALIZED_BIT_START_TIME;
return this;
}
/**
* Initializes value for {@link TraceBase#captureTime() captureTime}.
* @param captureTime value for captureTime
* @return {@code this} builder for chained invocation
*/
public final Builder captureTime(long captureTime) {
checkNotIsSet(captureTimeIsSet(), "captureTime");
this.captureTime = captureTime;
initializedBitset |= INITIALIZED_BIT_CAPTURE_TIME;
return this;
}
/**
* Initializes value for {@link TraceBase#duration() duration}.
* @param duration value for duration
* @return {@code this} builder for chained invocation
*/
public final Builder duration(long duration) {
checkNotIsSet(durationIsSet(), "duration");
this.duration = duration;
initializedBitset |= INITIALIZED_BIT_DURATION;
return this;
}
/**
* Initializes value for {@link TraceBase#transactionType() transactionType}.
* @param transactionType value for transactionType
* @return {@code this} builder for chained invocation
*/
public final Builder transactionType(String transactionType) {
checkNotIsSet(transactionTypeIsSet(), "transactionType");
this.transactionType = Preconditions.checkNotNull(transactionType);
initializedBitset |= INITIALIZED_BIT_TRANSACTION_TYPE;
return this;
}
/**
* Initializes value for {@link TraceBase#transactionName() transactionName}.
* @param transactionName value for transactionName
* @return {@code this} builder for chained invocation
*/
public final Builder transactionName(String transactionName) {
checkNotIsSet(transactionNameIsSet(), "transactionName");
this.transactionName = Preconditions.checkNotNull(transactionName);
initializedBitset |= INITIALIZED_BIT_TRANSACTION_NAME;
return this;
}
/**
* Initializes value for {@link TraceBase#headline() headline}.
* @param headline value for headline
* @return {@code this} builder for chained invocation
*/
public final Builder headline(String headline) {
checkNotIsSet(headlineIsSet(), "headline");
this.headline = Preconditions.checkNotNull(headline);
initializedBitset |= INITIALIZED_BIT_HEADLINE;
return this;
}
/**
* Initializes value for {@link TraceBase#user() user}.
* @param user value for user, can be {@code null}
* @return {@code this} builder for chained invocation
*/
public final Builder user(@Nullable String user) {
checkNotIsSet(userIsSet(), "user");
this.user = user;
nondefaultBitset |= NONDEFAULT_BIT_USER;
return this;
}
/**
* Initializes value for {@link TraceBase#customAttributes() customAttributes}.
* @param customAttributes value for customAttributes, can be {@code null}
* @return {@code this} builder for chained invocation
*/
public final Builder customAttributes(@Nullable String customAttributes) {
checkNotIsSet(customAttributesIsSet(), "customAttributes");
this.customAttributes = customAttributes;
nondefaultBitset |= NONDEFAULT_BIT_CUSTOM_ATTRIBUTES;
return this;
}
/**
* Initializes value for {@link TraceBase#customDetail() customDetail}.
* @param customDetail value for customDetail, can be {@code null}
* @return {@code this} builder for chained invocation
*/
public final Builder customDetail(@Nullable String customDetail) {
checkNotIsSet(customDetailIsSet(), "customDetail");
this.customDetail = customDetail;
nondefaultBitset |= NONDEFAULT_BIT_CUSTOM_DETAIL;
return this;
}
/**
* Initializes value for {@link TraceBase#errorMessage() errorMessage}.
* @param errorMessage value for errorMessage, can be {@code null}
* @return {@code this} builder for chained invocation
*/
public final Builder errorMessage(@Nullable String errorMessage) {
checkNotIsSet(errorMessageIsSet(), "errorMessage");
this.errorMessage = errorMessage;
nondefaultBitset |= NONDEFAULT_BIT_ERROR_MESSAGE;
return this;
}
/**
* Initializes value for {@link TraceBase#errorThrowable() errorThrowable}.
* @param errorThrowable value for errorThrowable, can be {@code null}
* @return {@code this} builder for chained invocation
*/
public final Builder errorThrowable(@Nullable String errorThrowable) {
checkNotIsSet(errorThrowableIsSet(), "errorThrowable");
this.errorThrowable = errorThrowable;
nondefaultBitset |= NONDEFAULT_BIT_ERROR_THROWABLE;
return this;
}
/**
* Initializes value for {@link TraceBase#timers() timers}.
* @param timers value for timers, can be {@code null}
* @return {@code this} builder for chained invocation
*/
public final Builder timers(@Nullable String timers) {
checkNotIsSet(timersIsSet(), "timers");
this.timers = timers;
nondefaultBitset |= NONDEFAULT_BIT_TIMERS;
return this;
}
/**
* Initializes value for {@link TraceBase#threadCpuTime() threadCpuTime}.
* @param threadCpuTime value for threadCpuTime, can be {@code null}
* @return {@code this} builder for chained invocation
*/
public final Builder threadCpuTime(@Nullable Long threadCpuTime) {
checkNotIsSet(threadCpuTimeIsSet(), "threadCpuTime");
this.threadCpuTime = threadCpuTime;
nondefaultBitset |= NONDEFAULT_BIT_THREAD_CPU_TIME;
return this;
}
/**
* Initializes value for {@link TraceBase#threadBlockedTime() threadBlockedTime}.
* @param threadBlockedTime value for threadBlockedTime, can be {@code null}
* @return {@code this} builder for chained invocation
*/
public final Builder threadBlockedTime(@Nullable Long threadBlockedTime) {
checkNotIsSet(threadBlockedTimeIsSet(), "threadBlockedTime");
this.threadBlockedTime = threadBlockedTime;
nondefaultBitset |= NONDEFAULT_BIT_THREAD_BLOCKED_TIME;
return this;
}
/**
* Initializes value for {@link TraceBase#threadWaitedTime() threadWaitedTime}.
* @param threadWaitedTime value for threadWaitedTime, can be {@code null}
* @return {@code this} builder for chained invocation
*/
public final Builder threadWaitedTime(@Nullable Long threadWaitedTime) {
checkNotIsSet(threadWaitedTimeIsSet(), "threadWaitedTime");
this.threadWaitedTime = threadWaitedTime;
nondefaultBitset |= NONDEFAULT_BIT_THREAD_WAITED_TIME;
return this;
}
/**
* Initializes value for {@link TraceBase#threadAllocatedBytes() threadAllocatedBytes}.
* @param threadAllocatedBytes value for threadAllocatedBytes, can be {@code null}
* @return {@code this} builder for chained invocation
*/
public final Builder threadAllocatedBytes(@Nullable Long threadAllocatedBytes) {
checkNotIsSet(threadAllocatedBytesIsSet(), "threadAllocatedBytes");
this.threadAllocatedBytes = threadAllocatedBytes;
nondefaultBitset |= NONDEFAULT_BIT_THREAD_ALLOCATED_BYTES;
return this;
}
/**
* Initializes value for {@link TraceBase#gcInfos() gcInfos}.
* @param gcInfos value for gcInfos, can be {@code null}
* @return {@code this} builder for chained invocation
*/
public final Builder gcInfos(@Nullable String gcInfos) {
checkNotIsSet(gcInfosIsSet(), "gcInfos");
this.gcInfos = gcInfos;
nondefaultBitset |= NONDEFAULT_BIT_GC_INFOS;
return this;
}
/**
* Initializes value for {@link TraceBase#entryCount() entryCount}.
* @param entryCount value for entryCount
* @return {@code this} builder for chained invocation
*/
public final Builder entryCount(int entryCount) {
checkNotIsSet(entryCountIsSet(), "entryCount");
this.entryCount = entryCount;
initializedBitset |= INITIALIZED_BIT_ENTRY_COUNT;
return this;
}
/**
* Initializes value for {@link TraceBase#profileSampleCount() profileSampleCount}.
* @param profileSampleCount value for profileSampleCount
* @return {@code this} builder for chained invocation
*/
public final Builder profileSampleCount(long profileSampleCount) {
checkNotIsSet(profileSampleCountIsSet(), "profileSampleCount");
this.profileSampleCount = profileSampleCount;
initializedBitset |= INITIALIZED_BIT_PROFILE_SAMPLE_COUNT;
return this;
}
/**
* Initializes value for {@link TraceBase#entriesExistence() entriesExistence}.
* @param entriesExistence value for entriesExistence
* @return {@code this} builder for chained invocation
*/
public final Builder entriesExistence(Existence entriesExistence) {
checkNotIsSet(entriesExistenceIsSet(), "entriesExistence");
this.entriesExistence = Preconditions.checkNotNull(entriesExistence);
initializedBitset |= INITIALIZED_BIT_ENTRIES_EXISTENCE;
return this;
}
/**
* Initializes value for {@link TraceBase#profileExistence() profileExistence}.
* @param profileExistence value for profileExistence
* @return {@code this} builder for chained invocation
*/
public final Builder profileExistence(Existence profileExistence) {
checkNotIsSet(profileExistenceIsSet(), "profileExistence");
this.profileExistence = Preconditions.checkNotNull(profileExistence);
initializedBitset |= INITIALIZED_BIT_PROFILE_EXISTENCE;
return this;
}
/**
* Put all mappings from specified key to values for {@link TraceBase#customAttributesForIndexing() customAttributesForIndexing} true. Nulls are not permitted
* @param key the key for customAttributesForIndexing
* @param values the values for customAttributesForIndexing
* @return {@code this} builder for chained invocation
*/
public final Builder putCustomAttributesForIndexing(String key, String... values) {
customAttributesForIndexingBuilder.putAll(key, Arrays.asList(values));
return this;
}
/**
* Put all mappings from specified key to values for {@link TraceBase#customAttributesForIndexing() customAttributesForIndexing} true. Nulls are not permitted
* @param key the key for customAttributesForIndexing
* @param values the values for customAttributesForIndexing
* @return {@code this} builder for chained invocation
*/
public final Builder putAllCustomAttributesForIndexing(String key, Iterable values) {
customAttributesForIndexingBuilder.putAll(key, values);
return this;
}
/**
* Put one entry to {@link TraceBase#customAttributesForIndexing() customAttributesForIndexing} map.
* @param key the key in customAttributesForIndexing map
* @param value the associated value in customAttributesForIndexing map
* @return {@code this} builder for chained invocation
*/
public final Builder putCustomAttributesForIndexing(String key, String value) {
customAttributesForIndexingBuilder.put(key, value);
return this;
}
/**
* Put one entry to {@link TraceBase#customAttributesForIndexing() customAttributesForIndexing} map. Nulls are not permitted
* @param entry the key and value entry
* @return {@code this} builder for chained invocation
*/
public final Builder putCustomAttributesForIndexing(Map.Entry entry) {
customAttributesForIndexingBuilder.put(entry);
return this;
}
/**
* Put all mappings from specified map as entries to {@link TraceBase#customAttributesForIndexing() customAttributesForIndexing} map. Nulls are not permitted
* @param entries to be added to customAttributesForIndexing map
* @return {@code this} builder for chained invocation
*/
public final Builder putAllCustomAttributesForIndexing(Multimap entries) {
customAttributesForIndexingBuilder.putAll(entries);
return this;
}
/**
* Builds new {@link org.glowroot.collector.Trace}.
* @return immutable instance of Trace
*/
public Trace build() {
checkRequiredAttributes();
return new Trace(
id,
active,
partial,
error,
startTime,
captureTime,
duration,
transactionType,
transactionName,
headline,
user,
customAttributes,
customDetail,
errorMessage,
errorThrowable,
timers,
threadCpuTime,
threadBlockedTime,
threadWaitedTime,
threadAllocatedBytes,
gcInfos,
entryCount,
profileSampleCount,
entriesExistence,
profileExistence,
customAttributesForIndexingBuilder.build());
}
private boolean userIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_USER) != 0;
}
private boolean customAttributesIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_CUSTOM_ATTRIBUTES) != 0;
}
private boolean customDetailIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_CUSTOM_DETAIL) != 0;
}
private boolean errorMessageIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_ERROR_MESSAGE) != 0;
}
private boolean errorThrowableIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_ERROR_THROWABLE) != 0;
}
private boolean timersIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_TIMERS) != 0;
}
private boolean threadCpuTimeIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_THREAD_CPU_TIME) != 0;
}
private boolean threadBlockedTimeIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_THREAD_BLOCKED_TIME) != 0;
}
private boolean threadWaitedTimeIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_THREAD_WAITED_TIME) != 0;
}
private boolean threadAllocatedBytesIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_THREAD_ALLOCATED_BYTES) != 0;
}
private boolean gcInfosIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_GC_INFOS) != 0;
}
private boolean idIsSet() {
return (initializedBitset & INITIALIZED_BIT_ID) != 0;
}
private boolean activeIsSet() {
return (initializedBitset & INITIALIZED_BIT_ACTIVE) != 0;
}
private boolean partialIsSet() {
return (initializedBitset & INITIALIZED_BIT_PARTIAL) != 0;
}
private boolean errorIsSet() {
return (initializedBitset & INITIALIZED_BIT_ERROR) != 0;
}
private boolean startTimeIsSet() {
return (initializedBitset & INITIALIZED_BIT_START_TIME) != 0;
}
private boolean captureTimeIsSet() {
return (initializedBitset & INITIALIZED_BIT_CAPTURE_TIME) != 0;
}
private boolean durationIsSet() {
return (initializedBitset & INITIALIZED_BIT_DURATION) != 0;
}
private boolean transactionTypeIsSet() {
return (initializedBitset & INITIALIZED_BIT_TRANSACTION_TYPE) != 0;
}
private boolean transactionNameIsSet() {
return (initializedBitset & INITIALIZED_BIT_TRANSACTION_NAME) != 0;
}
private boolean headlineIsSet() {
return (initializedBitset & INITIALIZED_BIT_HEADLINE) != 0;
}
private boolean entryCountIsSet() {
return (initializedBitset & INITIALIZED_BIT_ENTRY_COUNT) != 0;
}
private boolean profileSampleCountIsSet() {
return (initializedBitset & INITIALIZED_BIT_PROFILE_SAMPLE_COUNT) != 0;
}
private boolean entriesExistenceIsSet() {
return (initializedBitset & INITIALIZED_BIT_ENTRIES_EXISTENCE) != 0;
}
private boolean profileExistenceIsSet() {
return (initializedBitset & INITIALIZED_BIT_PROFILE_EXISTENCE) != 0;
}
private void checkNotIsSet(boolean isSet, String name) {
if (isSet) {
throw new IllegalStateException("Builder of Trace is strict, attribute is already set: ".concat(name));
}
}
private void checkRequiredAttributes() {
if (initializedBitset != INITIALIZED_BITSET_ALL) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
Collection attributes = Lists.newArrayList();
if (!idIsSet()) {
attributes.add("id");
}
if (!activeIsSet()) {
attributes.add("active");
}
if (!partialIsSet()) {
attributes.add("partial");
}
if (!errorIsSet()) {
attributes.add("error");
}
if (!startTimeIsSet()) {
attributes.add("startTime");
}
if (!captureTimeIsSet()) {
attributes.add("captureTime");
}
if (!durationIsSet()) {
attributes.add("duration");
}
if (!transactionTypeIsSet()) {
attributes.add("transactionType");
}
if (!transactionNameIsSet()) {
attributes.add("transactionName");
}
if (!headlineIsSet()) {
attributes.add("headline");
}
if (!entryCountIsSet()) {
attributes.add("entryCount");
}
if (!profileSampleCountIsSet()) {
attributes.add("profileSampleCount");
}
if (!entriesExistenceIsSet()) {
attributes.add("entriesExistence");
}
if (!profileExistenceIsSet()) {
attributes.add("profileExistence");
}
return "Cannot build Trace, some of required attributes are not set " + attributes;
}
}
}