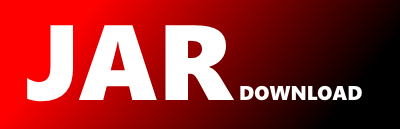
org.glowroot.config.Config Maven / Gradle / Ivy
package org.glowroot.config;
import org.glowroot.shaded.fasterxml.jackson.annotation.JsonCreator;
import org.glowroot.shaded.fasterxml.jackson.annotation.JsonProperty;
import org.glowroot.shaded.google.common.base.MoreObjects;
import org.glowroot.shaded.google.common.base.Preconditions;
import org.glowroot.shaded.google.common.collect.ImmutableList;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link ConfigBase}.
*
* Use builder to create immutable instances:
* {@code Config.builder()}.
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "ConfigBase"})
@Immutable
final class Config extends ConfigBase {
private final TransactionConfig transactionConfig;
private final UserInterfaceConfig userInterfaceConfig;
private final StorageConfig storageConfig;
private final SmtpConfig smtpConfig;
private final UserRecordingConfig userRecordingConfig;
private final AdvancedConfig advancedConfig;
private final ImmutableList pluginConfigs;
private final ImmutableList instrumentationConfigs;
private final ImmutableList gaugeConfigs;
private final ImmutableList alertConfigs;
private Config(Config.Builder builder) {
this.pluginConfigs = builder.pluginConfigsBuilder.build();
this.instrumentationConfigs = builder.instrumentationConfigsBuilder.build();
this.gaugeConfigs = builder.gaugeConfigsBuilder.build();
this.alertConfigs = builder.alertConfigsBuilder.build();
this.transactionConfig = builder.transactionConfig != null
? builder.transactionConfig
: Preconditions.checkNotNull(super.transactionConfig());
this.userInterfaceConfig = builder.userInterfaceConfig != null
? builder.userInterfaceConfig
: Preconditions.checkNotNull(super.userInterfaceConfig());
this.storageConfig = builder.storageConfig != null
? builder.storageConfig
: Preconditions.checkNotNull(super.storageConfig());
this.smtpConfig = builder.smtpConfig != null
? builder.smtpConfig
: Preconditions.checkNotNull(super.smtpConfig());
this.userRecordingConfig = builder.userRecordingConfig != null
? builder.userRecordingConfig
: Preconditions.checkNotNull(super.userRecordingConfig());
this.advancedConfig = builder.advancedConfig != null
? builder.advancedConfig
: Preconditions.checkNotNull(super.advancedConfig());
}
private Config(
TransactionConfig transactionConfig,
UserInterfaceConfig userInterfaceConfig,
StorageConfig storageConfig,
SmtpConfig smtpConfig,
UserRecordingConfig userRecordingConfig,
AdvancedConfig advancedConfig,
ImmutableList pluginConfigs,
ImmutableList instrumentationConfigs,
ImmutableList gaugeConfigs,
ImmutableList alertConfigs) {
this.transactionConfig = transactionConfig;
this.userInterfaceConfig = userInterfaceConfig;
this.storageConfig = storageConfig;
this.smtpConfig = smtpConfig;
this.userRecordingConfig = userRecordingConfig;
this.advancedConfig = advancedConfig;
this.pluginConfigs = pluginConfigs;
this.instrumentationConfigs = instrumentationConfigs;
this.gaugeConfigs = gaugeConfigs;
this.alertConfigs = alertConfigs;
}
/**
* {@inheritDoc}
* @return value of {@code transactionConfig} attribute
*/
@JsonProperty("transaction")
@Override
public TransactionConfig transactionConfig() {
return transactionConfig;
}
/**
* {@inheritDoc}
* @return value of {@code userInterfaceConfig} attribute
*/
@JsonProperty("ui")
@Override
public UserInterfaceConfig userInterfaceConfig() {
return userInterfaceConfig;
}
/**
* {@inheritDoc}
* @return value of {@code storageConfig} attribute
*/
@JsonProperty("storage")
@Override
public StorageConfig storageConfig() {
return storageConfig;
}
/**
* {@inheritDoc}
* @return value of {@code smtpConfig} attribute
*/
@JsonProperty("smtp")
@Override
public SmtpConfig smtpConfig() {
return smtpConfig;
}
/**
* {@inheritDoc}
* @return value of {@code userRecordingConfig} attribute
*/
@JsonProperty("userRecording")
@Override
public UserRecordingConfig userRecordingConfig() {
return userRecordingConfig;
}
/**
* {@inheritDoc}
* @return value of {@code advancedConfig} attribute
*/
@JsonProperty("advanced")
@Override
public AdvancedConfig advancedConfig() {
return advancedConfig;
}
/**
* {@inheritDoc}
* @return value of {@code pluginConfigs} attribute
*/
@JsonProperty("plugins")
@Override
public ImmutableList pluginConfigs() {
return pluginConfigs;
}
/**
* {@inheritDoc}
* @return value of {@code instrumentationConfigs} attribute
*/
@JsonProperty("instrumentation")
@Override
public ImmutableList instrumentationConfigs() {
return instrumentationConfigs;
}
/**
* {@inheritDoc}
* @return value of {@code gaugeConfigs} attribute
*/
@JsonProperty("gauges")
@Override
public ImmutableList gaugeConfigs() {
return gaugeConfigs;
}
/**
* {@inheritDoc}
* @return value of {@code alertConfigs} attribute
*/
@JsonProperty("alerts")
@Override
public ImmutableList alertConfigs() {
return alertConfigs;
}
/**
* Copy current immutable object by setting value for {@link ConfigBase#transactionConfig() transactionConfig}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for transactionConfig
* @return modified copy of the {@code this} object
*/
public final Config withTransactionConfig(TransactionConfig value) {
if (this.transactionConfig == value) {
return this;
}
TransactionConfig newValue = Preconditions.checkNotNull(value);
return new Config(
newValue,
this.userInterfaceConfig,
this.storageConfig,
this.smtpConfig,
this.userRecordingConfig,
this.advancedConfig,
this.pluginConfigs,
this.instrumentationConfigs,
this.gaugeConfigs,
this.alertConfigs);
}
/**
* Copy current immutable object by setting value for {@link ConfigBase#userInterfaceConfig() userInterfaceConfig}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for userInterfaceConfig
* @return modified copy of the {@code this} object
*/
public final Config withUserInterfaceConfig(UserInterfaceConfig value) {
if (this.userInterfaceConfig == value) {
return this;
}
UserInterfaceConfig newValue = Preconditions.checkNotNull(value);
return new Config(
this.transactionConfig,
newValue,
this.storageConfig,
this.smtpConfig,
this.userRecordingConfig,
this.advancedConfig,
this.pluginConfigs,
this.instrumentationConfigs,
this.gaugeConfigs,
this.alertConfigs);
}
/**
* Copy current immutable object by setting value for {@link ConfigBase#storageConfig() storageConfig}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for storageConfig
* @return modified copy of the {@code this} object
*/
public final Config withStorageConfig(StorageConfig value) {
if (this.storageConfig == value) {
return this;
}
StorageConfig newValue = Preconditions.checkNotNull(value);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
newValue,
this.smtpConfig,
this.userRecordingConfig,
this.advancedConfig,
this.pluginConfigs,
this.instrumentationConfigs,
this.gaugeConfigs,
this.alertConfigs);
}
/**
* Copy current immutable object by setting value for {@link ConfigBase#smtpConfig() smtpConfig}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for smtpConfig
* @return modified copy of the {@code this} object
*/
public final Config withSmtpConfig(SmtpConfig value) {
if (this.smtpConfig == value) {
return this;
}
SmtpConfig newValue = Preconditions.checkNotNull(value);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
this.storageConfig,
newValue,
this.userRecordingConfig,
this.advancedConfig,
this.pluginConfigs,
this.instrumentationConfigs,
this.gaugeConfigs,
this.alertConfigs);
}
/**
* Copy current immutable object by setting value for {@link ConfigBase#userRecordingConfig() userRecordingConfig}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for userRecordingConfig
* @return modified copy of the {@code this} object
*/
public final Config withUserRecordingConfig(UserRecordingConfig value) {
if (this.userRecordingConfig == value) {
return this;
}
UserRecordingConfig newValue = Preconditions.checkNotNull(value);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
this.storageConfig,
this.smtpConfig,
newValue,
this.advancedConfig,
this.pluginConfigs,
this.instrumentationConfigs,
this.gaugeConfigs,
this.alertConfigs);
}
/**
* Copy current immutable object by setting value for {@link ConfigBase#advancedConfig() advancedConfig}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for advancedConfig
* @return modified copy of the {@code this} object
*/
public final Config withAdvancedConfig(AdvancedConfig value) {
if (this.advancedConfig == value) {
return this;
}
AdvancedConfig newValue = Preconditions.checkNotNull(value);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
this.storageConfig,
this.smtpConfig,
this.userRecordingConfig,
newValue,
this.pluginConfigs,
this.instrumentationConfigs,
this.gaugeConfigs,
this.alertConfigs);
}
/**
* Copy current immutable object with elements that replace content of {@link ConfigBase#pluginConfigs() pluginConfigs}.
* @param elements elements to set
* @return modified copy of {@code this} object
*/
public final Config withPluginConfigs(PluginConfig... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
this.storageConfig,
this.smtpConfig,
this.userRecordingConfig,
this.advancedConfig,
newValue,
this.instrumentationConfigs,
this.gaugeConfigs,
this.alertConfigs);
}
/**
* Copy current immutable object with elements that replace content of {@link ConfigBase#pluginConfigs() pluginConfigs}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements iterable of pluginConfigs elements to set
* @return modified copy of {@code this} object
*/
public final Config withPluginConfigs(Iterable extends PluginConfig> elements) {
if (this.pluginConfigs == elements) {
return this;
}
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
this.storageConfig,
this.smtpConfig,
this.userRecordingConfig,
this.advancedConfig,
newValue,
this.instrumentationConfigs,
this.gaugeConfigs,
this.alertConfigs);
}
/**
* Copy current immutable object with elements that replace content of {@link ConfigBase#instrumentationConfigs() instrumentationConfigs}.
* @param elements elements to set
* @return modified copy of {@code this} object
*/
public final Config withInstrumentationConfigs(InstrumentationConfig... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
this.storageConfig,
this.smtpConfig,
this.userRecordingConfig,
this.advancedConfig,
this.pluginConfigs,
newValue,
this.gaugeConfigs,
this.alertConfigs);
}
/**
* Copy current immutable object with elements that replace content of {@link ConfigBase#instrumentationConfigs() instrumentationConfigs}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements iterable of instrumentationConfigs elements to set
* @return modified copy of {@code this} object
*/
public final Config withInstrumentationConfigs(Iterable extends InstrumentationConfig> elements) {
if (this.instrumentationConfigs == elements) {
return this;
}
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
this.storageConfig,
this.smtpConfig,
this.userRecordingConfig,
this.advancedConfig,
this.pluginConfigs,
newValue,
this.gaugeConfigs,
this.alertConfigs);
}
/**
* Copy current immutable object with elements that replace content of {@link ConfigBase#gaugeConfigs() gaugeConfigs}.
* @param elements elements to set
* @return modified copy of {@code this} object
*/
public final Config withGaugeConfigs(GaugeConfig... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
this.storageConfig,
this.smtpConfig,
this.userRecordingConfig,
this.advancedConfig,
this.pluginConfigs,
this.instrumentationConfigs,
newValue,
this.alertConfigs);
}
/**
* Copy current immutable object with elements that replace content of {@link ConfigBase#gaugeConfigs() gaugeConfigs}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements iterable of gaugeConfigs elements to set
* @return modified copy of {@code this} object
*/
public final Config withGaugeConfigs(Iterable extends GaugeConfig> elements) {
if (this.gaugeConfigs == elements) {
return this;
}
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
this.storageConfig,
this.smtpConfig,
this.userRecordingConfig,
this.advancedConfig,
this.pluginConfigs,
this.instrumentationConfigs,
newValue,
this.alertConfigs);
}
/**
* Copy current immutable object with elements that replace content of {@link ConfigBase#alertConfigs() alertConfigs}.
* @param elements elements to set
* @return modified copy of {@code this} object
*/
public final Config withAlertConfigs(AlertConfig... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
this.storageConfig,
this.smtpConfig,
this.userRecordingConfig,
this.advancedConfig,
this.pluginConfigs,
this.instrumentationConfigs,
this.gaugeConfigs,
newValue);
}
/**
* Copy current immutable object with elements that replace content of {@link ConfigBase#alertConfigs() alertConfigs}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements iterable of alertConfigs elements to set
* @return modified copy of {@code this} object
*/
public final Config withAlertConfigs(Iterable extends AlertConfig> elements) {
if (this.alertConfigs == elements) {
return this;
}
ImmutableList newValue = ImmutableList.copyOf(elements);
return new Config(
this.transactionConfig,
this.userInterfaceConfig,
this.storageConfig,
this.smtpConfig,
this.userRecordingConfig,
this.advancedConfig,
this.pluginConfigs,
this.instrumentationConfigs,
this.gaugeConfigs,
newValue);
}
/**
* This instance is equal to instances of {@code Config} with equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
return this == another
|| (another instanceof Config && equalTo((Config) another));
}
private boolean equalTo(Config another) {
return transactionConfig.equals(another.transactionConfig)
&& userInterfaceConfig.equals(another.userInterfaceConfig)
&& storageConfig.equals(another.storageConfig)
&& smtpConfig.equals(another.smtpConfig)
&& userRecordingConfig.equals(another.userRecordingConfig)
&& advancedConfig.equals(another.advancedConfig)
&& pluginConfigs.equals(another.pluginConfigs)
&& instrumentationConfigs.equals(another.instrumentationConfigs)
&& gaugeConfigs.equals(another.gaugeConfigs)
&& alertConfigs.equals(another.alertConfigs);
}
/**
* Computes hash code from attributes: {@code transactionConfig}, {@code userInterfaceConfig}, {@code storageConfig}, {@code smtpConfig}, {@code userRecordingConfig}, {@code advancedConfig}, {@code pluginConfigs}, {@code instrumentationConfigs}, {@code gaugeConfigs}, {@code alertConfigs}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + transactionConfig.hashCode();
h = h * 17 + userInterfaceConfig.hashCode();
h = h * 17 + storageConfig.hashCode();
h = h * 17 + smtpConfig.hashCode();
h = h * 17 + userRecordingConfig.hashCode();
h = h * 17 + advancedConfig.hashCode();
h = h * 17 + pluginConfigs.hashCode();
h = h * 17 + instrumentationConfigs.hashCode();
h = h * 17 + gaugeConfigs.hashCode();
h = h * 17 + alertConfigs.hashCode();
return h;
}
/**
* Prints immutable value {@code Config{...}} with attribute values,
* excluding any non-generated and auxiliary attributes.
* @return string representation of value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Config")
.add("transactionConfig", transactionConfig)
.add("userInterfaceConfig", userInterfaceConfig)
.add("storageConfig", storageConfig)
.add("smtpConfig", smtpConfig)
.add("userRecordingConfig", userRecordingConfig)
.add("advancedConfig", advancedConfig)
.add("pluginConfigs", pluginConfigs)
.add("instrumentationConfigs", instrumentationConfigs)
.add("gaugeConfigs", gaugeConfigs)
.add("alertConfigs", alertConfigs)
.toString();
}
@JsonCreator
public static Config fromAllAttributes(
@JsonProperty("transaction") @Nullable TransactionConfig transactionConfig,
@JsonProperty("ui") @Nullable UserInterfaceConfig userInterfaceConfig,
@JsonProperty("storage") @Nullable StorageConfig storageConfig,
@JsonProperty("smtp") @Nullable SmtpConfig smtpConfig,
@JsonProperty("userRecording") @Nullable UserRecordingConfig userRecordingConfig,
@JsonProperty("advanced") @Nullable AdvancedConfig advancedConfig,
@JsonProperty("plugins") @Nullable ImmutableList pluginConfigs,
@JsonProperty("instrumentation") @Nullable ImmutableList instrumentationConfigs,
@JsonProperty("gauges") @Nullable ImmutableList gaugeConfigs,
@JsonProperty("alerts") @Nullable ImmutableList alertConfigs) {
Config.Builder builder = Config.builder();
if (transactionConfig != null) {
builder.transactionConfig(transactionConfig);
}
if (userInterfaceConfig != null) {
builder.userInterfaceConfig(userInterfaceConfig);
}
if (storageConfig != null) {
builder.storageConfig(storageConfig);
}
if (smtpConfig != null) {
builder.smtpConfig(smtpConfig);
}
if (userRecordingConfig != null) {
builder.userRecordingConfig(userRecordingConfig);
}
if (advancedConfig != null) {
builder.advancedConfig(advancedConfig);
}
if (pluginConfigs != null) {
builder.addAllPluginConfigs(pluginConfigs);
}
if (instrumentationConfigs != null) {
builder.addAllInstrumentationConfigs(instrumentationConfigs);
}
if (gaugeConfigs != null) {
builder.addAllGaugeConfigs(gaugeConfigs);
}
if (alertConfigs != null) {
builder.addAllAlertConfigs(alertConfigs);
}
return builder.build();
}
/**
* Creates immutable copy of {@link ConfigBase}.
* Uses accessors to get values to initialize immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance instance to copy
* @return copied immutable Config instance
*/
static Config copyOf(ConfigBase instance) {
if (instance instanceof Config) {
return (Config) instance;
}
return Config.builder()
.transactionConfig(instance.transactionConfig())
.userInterfaceConfig(instance.userInterfaceConfig())
.storageConfig(instance.storageConfig())
.smtpConfig(instance.smtpConfig())
.userRecordingConfig(instance.userRecordingConfig())
.advancedConfig(instance.advancedConfig())
.addAllPluginConfigs(instance.pluginConfigs())
.addAllInstrumentationConfigs(instance.instrumentationConfigs())
.addAllGaugeConfigs(instance.gaugeConfigs())
.addAllAlertConfigs(instance.alertConfigs())
.build();
}
/**
* Creates builder for {@link org.glowroot.config.Config}.
* @return new Config builder
*/
static Config.Builder builder() {
return new Config.Builder();
}
/**
* Builds instances of {@link org.glowroot.config.Config}.
* Initialized attributes and then invoke {@link #build()} method to create
* immutable instance.
* Builder is not thread safe and generally should not be stored in field or collection,
* but used immediately to create instances.
*/
@NotThreadSafe
static final class Builder {
private static final long NONDEFAULT_BIT_TRANSACTION_CONFIG = 0x1L;
private static final long NONDEFAULT_BIT_USER_INTERFACE_CONFIG = 0x2L;
private static final long NONDEFAULT_BIT_STORAGE_CONFIG = 0x4L;
private static final long NONDEFAULT_BIT_SMTP_CONFIG = 0x8L;
private static final long NONDEFAULT_BIT_USER_RECORDING_CONFIG = 0x10L;
private static final long NONDEFAULT_BIT_ADVANCED_CONFIG = 0x20L;
private long nondefaultBitset;
private @Nullable TransactionConfig transactionConfig;
private @Nullable UserInterfaceConfig userInterfaceConfig;
private @Nullable StorageConfig storageConfig;
private @Nullable SmtpConfig smtpConfig;
private @Nullable UserRecordingConfig userRecordingConfig;
private @Nullable AdvancedConfig advancedConfig;
private ImmutableList.Builder pluginConfigsBuilder = ImmutableList.builder();
private ImmutableList.Builder instrumentationConfigsBuilder = ImmutableList.builder();
private ImmutableList.Builder gaugeConfigsBuilder = ImmutableList.builder();
private ImmutableList.Builder alertConfigsBuilder = ImmutableList.builder();
private Builder() {}
/**
* Initializes value for {@link ConfigBase#transactionConfig() transactionConfig}.
* If not set, this attribute will have default value returned by initializer of {@link ConfigBase#transactionConfig() transactionConfig}.
* @param transactionConfig value for transactionConfig
* @return {@code this} builder for chained invocation
*/
public final Builder transactionConfig(TransactionConfig transactionConfig) {
checkNotIsSet(transactionConfigIsSet(), "transactionConfig");
this.transactionConfig = Preconditions.checkNotNull(transactionConfig);
nondefaultBitset |= NONDEFAULT_BIT_TRANSACTION_CONFIG;
return this;
}
/**
* Initializes value for {@link ConfigBase#userInterfaceConfig() userInterfaceConfig}.
*
If not set, this attribute will have default value returned by initializer of {@link ConfigBase#userInterfaceConfig() userInterfaceConfig}.
* @param userInterfaceConfig value for userInterfaceConfig
* @return {@code this} builder for chained invocation
*/
public final Builder userInterfaceConfig(UserInterfaceConfig userInterfaceConfig) {
checkNotIsSet(userInterfaceConfigIsSet(), "userInterfaceConfig");
this.userInterfaceConfig = Preconditions.checkNotNull(userInterfaceConfig);
nondefaultBitset |= NONDEFAULT_BIT_USER_INTERFACE_CONFIG;
return this;
}
/**
* Initializes value for {@link ConfigBase#storageConfig() storageConfig}.
*
If not set, this attribute will have default value returned by initializer of {@link ConfigBase#storageConfig() storageConfig}.
* @param storageConfig value for storageConfig
* @return {@code this} builder for chained invocation
*/
public final Builder storageConfig(StorageConfig storageConfig) {
checkNotIsSet(storageConfigIsSet(), "storageConfig");
this.storageConfig = Preconditions.checkNotNull(storageConfig);
nondefaultBitset |= NONDEFAULT_BIT_STORAGE_CONFIG;
return this;
}
/**
* Initializes value for {@link ConfigBase#smtpConfig() smtpConfig}.
*
If not set, this attribute will have default value returned by initializer of {@link ConfigBase#smtpConfig() smtpConfig}.
* @param smtpConfig value for smtpConfig
* @return {@code this} builder for chained invocation
*/
public final Builder smtpConfig(SmtpConfig smtpConfig) {
checkNotIsSet(smtpConfigIsSet(), "smtpConfig");
this.smtpConfig = Preconditions.checkNotNull(smtpConfig);
nondefaultBitset |= NONDEFAULT_BIT_SMTP_CONFIG;
return this;
}
/**
* Initializes value for {@link ConfigBase#userRecordingConfig() userRecordingConfig}.
*
If not set, this attribute will have default value returned by initializer of {@link ConfigBase#userRecordingConfig() userRecordingConfig}.
* @param userRecordingConfig value for userRecordingConfig
* @return {@code this} builder for chained invocation
*/
public final Builder userRecordingConfig(UserRecordingConfig userRecordingConfig) {
checkNotIsSet(userRecordingConfigIsSet(), "userRecordingConfig");
this.userRecordingConfig = Preconditions.checkNotNull(userRecordingConfig);
nondefaultBitset |= NONDEFAULT_BIT_USER_RECORDING_CONFIG;
return this;
}
/**
* Initializes value for {@link ConfigBase#advancedConfig() advancedConfig}.
*
If not set, this attribute will have default value returned by initializer of {@link ConfigBase#advancedConfig() advancedConfig}.
* @param advancedConfig value for advancedConfig
* @return {@code this} builder for chained invocation
*/
public final Builder advancedConfig(AdvancedConfig advancedConfig) {
checkNotIsSet(advancedConfigIsSet(), "advancedConfig");
this.advancedConfig = Preconditions.checkNotNull(advancedConfig);
nondefaultBitset |= NONDEFAULT_BIT_ADVANCED_CONFIG;
return this;
}
/**
* Adds one element to {@link ConfigBase#pluginConfigs() pluginConfigs} list.
* @param element pluginConfigs element
* @return {@code this} builder for chained invocation
*/
public final Builder addPluginConfigs(PluginConfig element) {
pluginConfigsBuilder.add(element);
return this;
}
/**
* Adds elements to {@link ConfigBase#pluginConfigs() pluginConfigs} list.
* @param elements array of pluginConfigs elements
* @return {@code this} builder for chained invocation
*/
public final Builder addPluginConfigs(PluginConfig... elements) {
pluginConfigsBuilder.add(elements);
return this;
}
/**
* Adds elements to {@link ConfigBase#pluginConfigs() pluginConfigs} list.
* @param elements iterable of pluginConfigs elements
* @return {@code this} builder for chained invocation
*/
public final Builder addAllPluginConfigs(Iterable extends PluginConfig> elements) {
pluginConfigsBuilder.addAll(elements);
return this;
}
/**
* Adds one element to {@link ConfigBase#instrumentationConfigs() instrumentationConfigs} list.
* @param element instrumentationConfigs element
* @return {@code this} builder for chained invocation
*/
public final Builder addInstrumentationConfigs(InstrumentationConfig element) {
instrumentationConfigsBuilder.add(element);
return this;
}
/**
* Adds elements to {@link ConfigBase#instrumentationConfigs() instrumentationConfigs} list.
* @param elements array of instrumentationConfigs elements
* @return {@code this} builder for chained invocation
*/
public final Builder addInstrumentationConfigs(InstrumentationConfig... elements) {
instrumentationConfigsBuilder.add(elements);
return this;
}
/**
* Adds elements to {@link ConfigBase#instrumentationConfigs() instrumentationConfigs} list.
* @param elements iterable of instrumentationConfigs elements
* @return {@code this} builder for chained invocation
*/
public final Builder addAllInstrumentationConfigs(Iterable extends InstrumentationConfig> elements) {
instrumentationConfigsBuilder.addAll(elements);
return this;
}
/**
* Adds one element to {@link ConfigBase#gaugeConfigs() gaugeConfigs} list.
* @param element gaugeConfigs element
* @return {@code this} builder for chained invocation
*/
public final Builder addGaugeConfigs(GaugeConfig element) {
gaugeConfigsBuilder.add(element);
return this;
}
/**
* Adds elements to {@link ConfigBase#gaugeConfigs() gaugeConfigs} list.
* @param elements array of gaugeConfigs elements
* @return {@code this} builder for chained invocation
*/
public final Builder addGaugeConfigs(GaugeConfig... elements) {
gaugeConfigsBuilder.add(elements);
return this;
}
/**
* Adds elements to {@link ConfigBase#gaugeConfigs() gaugeConfigs} list.
* @param elements iterable of gaugeConfigs elements
* @return {@code this} builder for chained invocation
*/
public final Builder addAllGaugeConfigs(Iterable extends GaugeConfig> elements) {
gaugeConfigsBuilder.addAll(elements);
return this;
}
/**
* Adds one element to {@link ConfigBase#alertConfigs() alertConfigs} list.
* @param element alertConfigs element
* @return {@code this} builder for chained invocation
*/
public final Builder addAlertConfigs(AlertConfig element) {
alertConfigsBuilder.add(element);
return this;
}
/**
* Adds elements to {@link ConfigBase#alertConfigs() alertConfigs} list.
* @param elements array of alertConfigs elements
* @return {@code this} builder for chained invocation
*/
public final Builder addAlertConfigs(AlertConfig... elements) {
alertConfigsBuilder.add(elements);
return this;
}
/**
* Adds elements to {@link ConfigBase#alertConfigs() alertConfigs} list.
* @param elements iterable of alertConfigs elements
* @return {@code this} builder for chained invocation
*/
public final Builder addAllAlertConfigs(Iterable extends AlertConfig> elements) {
alertConfigsBuilder.addAll(elements);
return this;
}
/**
* Builds new {@link org.glowroot.config.Config}.
* @return immutable instance of Config
*/
public org.glowroot.config.Config build() {
return new Config(this);
}
private boolean transactionConfigIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_TRANSACTION_CONFIG) != 0;
}
private boolean userInterfaceConfigIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_USER_INTERFACE_CONFIG) != 0;
}
private boolean storageConfigIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_STORAGE_CONFIG) != 0;
}
private boolean smtpConfigIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_SMTP_CONFIG) != 0;
}
private boolean userRecordingConfigIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_USER_RECORDING_CONFIG) != 0;
}
private boolean advancedConfigIsSet() {
return (nondefaultBitset & NONDEFAULT_BIT_ADVANCED_CONFIG) != 0;
}
private void checkNotIsSet(boolean isSet, String name) {
if (isSet) {
throw new IllegalStateException("Builder of Config is strict, attribute is already set: ".concat(name));
}
}
}
}