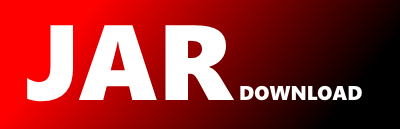
org.gnode.nix.SetDimension Maven / Gradle / Ivy
Show all versions of nix-linux-x86_64 Show documentation
package org.gnode.nix;
import org.bytedeco.javacpp.Loader;
import org.bytedeco.javacpp.annotation.*;
import org.gnode.nix.base.ImplContainer;
import org.gnode.nix.internal.None;
import org.gnode.nix.internal.VectorUtils;
import java.util.List;
/**
* SetDimension
* Dimension descriptor for a dimension that represents just a list or set of values.
*
* The SetDimension is used in cases where data is given as a set or list. This can be just a collection of values but
* also a list of recorded signals or a stack of images. Optionally an array of labels, one for each index of this
* dimension, can be specified.
*
* @see Dimension
* @see RangeDimension
* @see SampledDimension
*/
@Properties(value = {
@Platform(include = {""}, link = "nix", preload = "hdf5"),
@Platform(value = "linux"),
@Platform(value = "windows")})
@Namespace("nix")
public class SetDimension extends ImplContainer implements Comparable {
static {
Loader.load();
}
//--------------------------------------------------
// Constructors
//--------------------------------------------------
/**
* Constructor that creates an uninitialized SetDimension.
*
* Calling any method on an uninitialized dimension will throw a {@link java.lang.RuntimeException}.
*/
public SetDimension() {
allocate();
}
private native void allocate();
//--------------------------------------------------
// Base class methods
//--------------------------------------------------
public native
@Cast("bool")
boolean isNone();
//--------------------------------------------------
// Methods concerning SetDimension
//--------------------------------------------------
/**
* The actual dimension that is described by the dimension descriptor.
*
* The index of the dimension entity representing the dimension of the actual
* data that is defined by this descriptor.
*
* @return The dimension index of the dimension.
*/
public native
@Name("index")
@Cast("size_t")
long getIndex();
/**
* The type of the dimension.
*
* This field indicates whether the dimension is a SampledDimension, SetDimension or
* RangeDimension.
*
* @return The dimension type. Constants specified in {@link DataType}
* @see DataType
*/
public native
@Name("dimensionType")
@ByVal
@Cast("nix::DimensionType")
int getDimensionType();
private native
@ByVal
VectorUtils.StringVector labels();
/**
* Get the labels of the range dimension.
*
* The labels serve as names for each index of the data at the respective
* dimension.
*
* @return The labels of the dimension as a list of strings.
*/
public List getLabels() {
return labels().getStrings();
}
private native void labels(@Const @ByRef VectorUtils.StringVector labels);
private native void labels(@Const @ByVal None t);
/**
* Set the labels for the dimension.
*
* @param labels A list containing all new labels. If null removes the labels from the dimension.
*/
public void setLabels(List labels) {
if (labels != null) {
labels(new VectorUtils.StringVector(labels));
} else {
labels(new None());
}
}
//--------------------------------------------------
// Overrides
//--------------------------------------------------
@Override
public int compareTo(T dimension) {
if (this == dimension) {
return 0;
}
return (int) (this.getIndex() - dimension.getIndex());
}
}