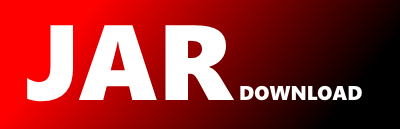
gnu.crypto.sasl.srp.CALG Maven / Gradle / Ivy
package gnu.crypto.sasl.srp;
// ----------------------------------------------------------------------------
// $Id: CALG.java,v 1.4 2003/11/21 09:33:34 raif Exp $
//
// Copyright (C) 2003 Free Software Foundation, Inc.
//
// This file is part of GNU Crypto.
//
// GNU Crypto is free software; you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation; either version 2, or (at your option)
// any later version.
//
// GNU Crypto is distributed in the hope that it will be useful, but
// WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
// General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program; see the file COPYING. If not, write to the
//
// Free Software Foundation Inc.,
// 59 Temple Place - Suite 330,
// Boston, MA 02111-1307
// USA
//
// Linking this library statically or dynamically with other modules is
// making a combined work based on this library. Thus, the terms and
// conditions of the GNU General Public License cover the whole
// combination.
//
// As a special exception, the copyright holders of this library give
// you permission to link this library with independent modules to
// produce an executable, regardless of the license terms of these
// independent modules, and to copy and distribute the resulting
// executable under terms of your choice, provided that you also meet,
// for each linked independent module, the terms and conditions of the
// license of that module. An independent module is a module which is
// not derived from or based on this library. If you modify this
// library, you may extend this exception to your version of the
// library, but you are not obligated to do so. If you do not wish to
// do so, delete this exception statement from your version.
// ----------------------------------------------------------------------------
import gnu.crypto.Registry;
import gnu.crypto.assembly.Assembly;
import gnu.crypto.assembly.Cascade;
import gnu.crypto.assembly.Direction;
import gnu.crypto.assembly.Stage;
import gnu.crypto.assembly.Transformer;
import gnu.crypto.assembly.TransformerException;
import gnu.crypto.cipher.CipherFactory;
import gnu.crypto.cipher.IBlockCipher;
import gnu.crypto.mode.IMode;
import gnu.crypto.mode.ModeFactory;
import gnu.crypto.pad.IPad;
import gnu.crypto.pad.PadFactory;
import gnu.crypto.sasl.ConfidentialityException;
import java.util.HashMap;
import javax.security.sasl.SaslException;
/**
* A Factory class that returns CALG (Confidentiality Algorithm) instances
* that operate as described in the draft-burdis-cat-sasl-srp-08.
*
* The designated CALG block cipher should be used in OFB (Output Feedback
* Block) mode in the ISO variant, as described in The Handbook of Applied
* Cryptography, algorithm 7.20.
*
* Let k
be the block size of the chosen symmetric key block
* cipher algorithm; e.g. for AES this is 128
bits or 16
* octets. The OFB mode used shall be of length/size k
.
*
* It is recommended that block ciphers operating in OFB mode be used with an
* Initial Vector (the mode's IV). In such a mode of operation - OFB with key
* re-use - the IV need not be secret. For the mechanism in question the IVs
* shall be a random octet sequence of k
bytes.
*
* The input data to the confidentiality protection algorithm shall be
* a multiple of the symmetric cipher block size k
. When the input
* length is not a multiple of k
octets, the data shall be padded
* according to the following scheme:
*
* Assuming the length of the input is l
octets,
* (k - (l mod k))
octets, all having the value
* (k - (l mod k))
, shall be appended to the original data. In
* other words, the input is padded at the trailing end with one of the
* following sequences:
*
*
*
* 01 -- if l mod k = k-1
* 02 02 -- if l mod k = k-2
* ...
* ...
* ...
* k k ... k k -- if l mod k = 0
*
*
* The padding can be removed unambiguously since all input is padded and no
* padding sequence is a suffix of another. This padding method is well-defined
* if and only if k < 256
octets, which is the case with
* symmetric key block ciphers today, and in the forseeable future.
*
* @version $Revision: 1.4 $
*/
public final class CALG {
// Constants and variables
// --------------------------------------------------------------------------
private Assembly assembly;
private Object modeNdx; // initialisation key of the cascade's attributes
private int blockSize; // the underlying cipher's blocksize == IV length
private int keySize; // the underlying cipher's key size (in bytes).
// Constructor(s)
// --------------------------------------------------------------------------
/** Private constructor to enforce instantiation through Factory method. */
private CALG(final int blockSize, final int keySize, final Object modeNdx,
final Assembly assembly) {
super();
this.blockSize = blockSize;
this.keySize = keySize;
this.modeNdx = modeNdx;
this.assembly = assembly;
}
// Class methods
// -------------------------------------------------------------------------
/**
* Returns an instance of a SASL-SRP CALG implementation.
*
* @param algorithm the name of the symmetric cipher algorithm.
* @return an instance of this object.
*/
static synchronized CALG getInstance(final String algorithm) {
final IBlockCipher cipher = CipherFactory.getInstance(algorithm);
final int blockSize = cipher.defaultBlockSize();
final int keySize = cipher.defaultKeySize();
final Cascade ofbCipher = new Cascade();
final Object modeNdx = ofbCipher.append(
Stage.getInstance(
ModeFactory.getInstance(Registry.OFB_MODE, cipher, blockSize),
Direction.FORWARD));
final IPad pkcs7 = PadFactory.getInstance(Registry.PKCS7_PAD);
// the passed IV may be longer that what we need. ensure correct length
// byte[] realIV = null;
// if (iv.length == blockSize) {
// realIV = iv;
// } else {
// realIV = new byte[blockSize];
// if (iv.length > blockSize) {
// System.arraycopy(iv, 0, realIV, 0, blockSize);
// } else { // shouldnt happen
// System.arraycopy(iv, 0, realIV, 0, iv.length);
// }
// }
// HashMap modeAttributes = new HashMap();
// modeAttributes.put(IBlockCipher.KEY_MATERIAL, K.clone());
// modeAttributes.put(IMode.IV, realIV);
final Assembly asm = new Assembly();
asm.addPreTransformer(Transformer.getCascadeTransformer(ofbCipher));
asm.addPreTransformer(Transformer.getPaddingTransformer(pkcs7));
// HashMap attributes = new HashMap();
// attributes.put(Assembly.DIRECTION, dir);
// attributes.put(modeNdx, modeAttributes);
// try {
// asm.init(attributes);
// } catch (TransformerException x) {
// throw new SaslException("getInstance()", x);
// }
return new CALG(blockSize, keySize, modeNdx, asm);
}
// Instance methods
// -------------------------------------------------------------------------
/**
* Initialises a SASL-SRP CALG implementation.
*
* @param kdf the key derivation function.
* @param iv the initial vector value to use.
* @param dir whether this CALG is used for encryption or decryption.
*/
// public void init(byte[] K, byte[] iv, Direction dir) throws SaslException {
public void init(final KDF kdf, final byte[] iv, final Direction dir)
throws SaslException {
// IBlockCipher cipher = CipherFactory.getInstance(algorithm);
// int blockSize = cipher.defaultBlockSize();
// Cascade ofbCipher = new Cascade();
// Object modeNdx = ofbCipher.append(
// Stage.getInstace(
// ModeFactory.getInstance(Registry.OFB_MODE, cipher, blockSize),
// Direction.FORWARD));
// IPad pkcs7 = PadFactory.getInstance(Registry.PKCS7_PAD);
// the passed IV may be longer that what we need. ensure correct length
final byte[] realIV;
if (iv.length == blockSize) {
realIV = iv;
} else {
realIV = new byte[blockSize];
if (iv.length > blockSize) {
System.arraycopy(iv, 0, realIV, 0, blockSize);
} else { // shouldnt happen
System.arraycopy(iv, 0, realIV, 0, iv.length);
}
}
final HashMap modeAttributes = new HashMap();
// modeAttributes.put(IBlockCipher.KEY_MATERIAL, K.clone());
final byte[] sk = kdf.derive(keySize);
modeAttributes.put(IBlockCipher.KEY_MATERIAL, sk);
//System.out.println("**** Initialised CALG with: "+gnu.crypto.util.Util.dumpString(sk));
modeAttributes.put(IMode.IV, realIV);
// Assembly asm = new Assembly();
// asm.addPreTransformer(Transformer.getCascadeTransformer(ofbCipher));
// asm.addPreTransformer(Transformer.getPaddingTransformer(pkcs7));
final HashMap attributes = new HashMap();
attributes.put(Assembly.DIRECTION, dir);
attributes.put(modeNdx, modeAttributes);
try {
// asm.init(attributes);
assembly.init(attributes);
} catch (TransformerException x) {
throw new SaslException("getInstance()", x);
}
// return new CALG(asm);
}
/**
* Encrypts or decrypts, depending on the mode already set, a designated
* array of bytes and returns the result.
*
* @param data the data to encrypt/decrypt.
* @return the decrypted/encrypted result.
* @throws ConfidentialityException if an exception occurs duirng the process.
*/
public byte[] doFinal(final byte[] data) throws ConfidentialityException {
return doFinal(data, 0, data.length);
}
/**
* Encrypts or decrypts, depending on the mode already set, a designated
* array of bytes and returns the result.
*
* @param data the data to encrypt/decrypt.
* @param offset where to start in data
.
* @param length how many bytes to consider in data
.
* @return the decrypted/encrypted result.
* @throws ConfidentialityException if an exception occurs duirng the process.
*/
public byte[] doFinal(final byte[] data, final int offset, final int length)
throws ConfidentialityException {
final byte[] result;
try {
result = assembly.lastUpdate(data, offset, length);
} catch (TransformerException x) {
throw new ConfidentialityException("doFinal()", x);
}
return result;
}
}