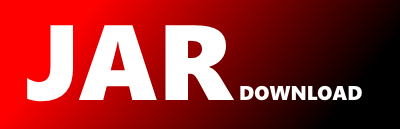
org.gosulang.gradle.tasks.compile.GosuCompile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-gosu-plugin Show documentation
Show all versions of gradle-gosu-plugin Show documentation
Gosu language compiler for Gradle. Built with Gradle 5.1.1.
This has also been tested extensively with Gradle version(s): 5.1.1
** Gradle versions below 2.12 are no longer supported **
Projects applying this plugin require a compile-time dependency on the gosu-core-api library.
This version requires Gosu 1.13.9 or greater, or 1.14.2 or greater.
Please include this in the depencencies closure:
dependencies {
compile group: 'org.gosu-lang.gosu', name: 'gosu-core-api', version: '1.14.12'
}
package org.gosulang.gradle.tasks.compile;
import groovy.lang.Closure;
import org.gosulang.gradle.tasks.InfersGosuRuntime;
import org.gradle.api.GradleException;
import org.gradle.api.Project;
import org.gradle.api.file.FileCollection;
import org.gradle.api.file.FileTree;
import org.gradle.api.file.SourceDirectorySet;
import org.gradle.api.logging.Logger;
import org.gradle.api.model.ObjectFactory;
import org.gradle.api.plugins.JavaPlugin;
import org.gradle.api.tasks.*;
import org.gradle.api.tasks.compile.AbstractCompile;
import org.gradle.api.tasks.compile.CompileOptions;
import org.gradle.util.VersionNumber;
import javax.inject.Inject;
import java.io.File;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import static org.gradle.api.tasks.PathSensitivity.NAME_ONLY;
@CacheableTask
public class GosuCompile extends AbstractCompile implements InfersGosuRuntime {
private GosuCompiler _compiler;
private Closure _gosuClasspath;
private Closure _orderClasspath;
private final CompileOptions _compileOptions;
private final GosuCompileOptions _gosuCompileOptions = new GosuCompileOptions();
@Inject
public GosuCompile() {
VersionNumber gradleVersion = VersionNumber.parse(getProject().getGradle().getGradleVersion());
if(gradleVersion.compareTo(VersionNumber.parse("4.2")) >= 0) {
_compileOptions = getServices().get(ObjectFactory.class).newInstance(CompileOptions.class);
} else {
try {
Constructor ctor = CompileOptions.class.getConstructor();
_compileOptions = (CompileOptions) ctor.newInstance();
} catch (ReflectiveOperationException e) {
throw new GradleException("Unable to apply Gosu plugin", e);
}
}
}
@Override
@TaskAction
protected void compile() {
DefaultGosuCompileSpec spec = createSpec();
_compiler = getCompiler(spec);
_compiler.execute(spec);
}
/**
* {@inheritDoc}
*/
@Override
@PathSensitive(NAME_ONLY)
public FileTree getSource() {
return super.getSource();
}
/**
* @return Gosu-specific compilation options.
*/
@Nested
public GosuCompileOptions getGosuOptions() {
return _gosuCompileOptions;
}
@Nested
public CompileOptions getOptions() {
return _compileOptions;
}
/**
* We override in order to apply the {@link org.gradle.api.tasks.CompileClasspath}, in order to ignore changes in JAR'd resources.
*/
@CompileClasspath
public FileCollection getClasspath() {
return super.getClasspath();
}
/**
* @return the classpath to use to load the Gosu compiler.
*/
@Override
@Classpath
@InputFiles
public Closure getGosuClasspath() {
return _gosuClasspath;
}
@Override
public void setGosuClasspath(Closure gosuClasspathClosure) {
_gosuClasspath = gosuClasspathClosure;
}
/**
* Annotating as @Input or @InputFiles causes errors in Guidewire applications, even when paired with @Optional.
* Marking as @Internal instead to skip warning thrown by :validateTaskProperties (org.gradle.plugin.devel.tasks.ValidateTaskProperties)
* @return a Closure returning a classpath to be passed to the GosuCompile task
*/
@Internal
public Closure getOrderClasspath() {
return _orderClasspath;
}
/**
* Normally setting this value is not required.
* Certain projects relying on depth-first resolution of module dependencies can use this
* Closure to reorder the classpath as needed.
*
* @param orderClasspath a Closure returning a classpath to be passed to the GosuCompile task
*/
public void setOrderClasspath(Closure orderClasspath) {
_orderClasspath = orderClasspath;
}
@Internal
@Optional
public FileCollection getSourceRoots() {
Set returnValues = new HashSet<>();
//noinspection Convert2streamapi
for(Object obj : getSourceReflectively()) {
if(obj instanceof SourceDirectorySet) {
returnValues.addAll(((SourceDirectorySet) obj).getSrcDirs());
}
}
return getProject().files(returnValues);
}
//!! todo: find a better way to iterate the FileTree
private Iterable getSourceReflectively() {
try {
Field field = SourceTask.class.getDeclaredField("source");
field.setAccessible(true);
return (Iterable)field.get(this);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
private DefaultGosuCompileSpec createSpec() {
DefaultGosuCompileSpec spec = new DefaultGosuCompileSpec();
spec.setCompileOptions(_compileOptions);
Project project = getProject();
spec.setSource(getSource());
spec.setSourceRoots(getSourceRoots());
spec.setDestinationDir(getDestinationDir());
spec.setTempDir(getTemporaryDir());
spec.setGosuClasspath(getGosuClasspath());
spec.setCompileOptions(_compileOptions);
spec.setGosuCompileOptions(_gosuCompileOptions);
if (_orderClasspath == null) {
spec.setClasspath(asList(getClasspath()));
} else {
spec.setClasspath(asList(_orderClasspath.call(project, project.getConfigurations().getByName(JavaPlugin.COMPILE_CONFIGURATION_NAME))));
}
Logger logger = project.getLogger();
if(logger.isInfoEnabled()) {
logger.info("Gosu Compiler source roots for {} are:", project.getName());
if(spec.getSourceRoots().isEmpty()) {
logger.info("");
} else {
for(File file : spec.getSourceRoots()) {
logger.info(file.getAbsolutePath());
}
}
logger.info("Gosu Compiler Spec classpath for {} is:", project.getName());
if(!spec.getClasspath().iterator().hasNext()) {
logger.info("");
} else {
for(File file : spec.getClasspath()) {
logger.info(file.getAbsolutePath());
}
}
logger.info("Gosu Compile Spec gosuClasspath for {} is:", project.getName());
FileCollection gosuClasspath = spec.getGosuClasspath().call();
if(gosuClasspath.isEmpty()) {
logger.info("");
} else {
for(File file : gosuClasspath) {
logger.info(file.getAbsolutePath());
}
}
}
return spec;
}
private GosuCompiler getCompiler(GosuCompileSpec spec) {
if(_compiler == null) {
GosuCompilerFactory gosuCompilerFactory = new GosuCompilerFactory(getProject(), this.getPath());
_compiler = gosuCompilerFactory.newCompiler(spec);
}
return _compiler;
}
private List asList(final FileCollection files) {
List list = new ArrayList<>();
files.forEach(list::add);
return list;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy