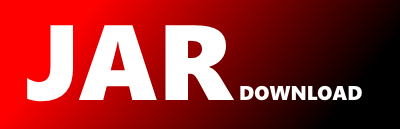
jdk.graal.compiler.nodes.java.PluginFactory_NewArrayNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compiler Show documentation
Show all versions of compiler Show documentation
The GraalVM compiler and the Graal-truffle optimizer.
// CheckStyle: stop header check
// CheckStyle: stop line length check
// GENERATED CONTENT - DO NOT EDIT
// GENERATORS: jdk.graal.compiler.replacements.processor.ReplacementsAnnotationProcessor, jdk.graal.compiler.replacements.processor.PluginGenerator
package jdk.graal.compiler.nodes.java;
import java.lang.annotation.Annotation;
import jdk.graal.compiler.core.common.type.Stamp;
import jdk.graal.compiler.graph.NodeInputList;
import jdk.graal.compiler.nodes.PluginReplacementNode;
import jdk.graal.compiler.nodes.ValueNode;
import jdk.graal.compiler.nodes.graphbuilderconf.GeneratedNodeIntrinsicInvocationPlugin;
import jdk.graal.compiler.nodes.graphbuilderconf.GeneratedPluginFactory;
import jdk.graal.compiler.nodes.graphbuilderconf.GeneratedPluginInjectionProvider;
import jdk.graal.compiler.nodes.graphbuilderconf.GraphBuilderContext;
import jdk.graal.compiler.nodes.graphbuilderconf.InvocationPlugin;
import jdk.graal.compiler.nodes.graphbuilderconf.InvocationPlugins;
import jdk.graal.compiler.options.ExcludeFromJacocoGeneratedReport;
import jdk.vm.ci.meta.JavaKind;
import jdk.vm.ci.meta.ResolvedJavaMethod;
// class: jdk.graal.compiler.nodes.java.NewArrayNode
// method: newArray(java.lang.Class>,int,boolean)
// generated-by: jdk.graal.compiler.replacements.processor.GeneratedNodeIntrinsicPlugin$ConstructorPlugin
final class Plugin_NewArrayNode_newArray extends GeneratedNodeIntrinsicInvocationPlugin {
@Override
public boolean execute(GraphBuilderContext b, ResolvedJavaMethod targetMethod, InvocationPlugin.Receiver receiver, ValueNode[] args) {
if (!b.isPluginEnabled(this)) {
return false;
}
jdk.vm.ci.meta.ResolvedJavaType arg0;
if (args[0].isConstant()) {
jdk.vm.ci.meta.JavaConstant cst = args[0].asJavaConstant();
arg0 = b.getConstantReflection()/* A CONSTANT_REFLECTION */.asJavaType(cst);
if (arg0 == null) {
arg0 = snippetReflection/* A SNIPPET_REFLECTION */.asObject(jdk.vm.ci.meta.ResolvedJavaType.class, cst);
}
} else {
if (b.shouldDeferPlugin(this)) {
b.replacePlugin(this, targetMethod, args, PluginReplacementNode_NewArrayNode_newArray.FUNCTION);
return true;
}
assert b.canDeferPlugin(this) : b.getClass().toString() + " " + args[0];
return false;
}
ValueNode arg1 = args[1];
boolean arg2;
if (args[2].isConstant()) {
arg2 = args[2].asJavaConstant().asInt() != 0;
} else {
if (b.shouldDeferPlugin(this)) {
b.replacePlugin(this, targetMethod, args, PluginReplacementNode_NewArrayNode_newArray.FUNCTION);
return true;
}
assert b.canDeferPlugin(this) : b.getClass().toString() + " " + args[2];
return false;
}
jdk.graal.compiler.nodes.java.NewArrayNode node = new jdk.graal.compiler.nodes.java.NewArrayNode(arg0, arg1, arg2);
b.addPush(JavaKind.Object, node);
return true;
}
@Override
public Class extends Annotation> getSource() {
return jdk.graal.compiler.graph.Node.NodeIntrinsic.class;
}
private final jdk.graal.compiler.api.replacements.SnippetReflectionProvider snippetReflection;
Plugin_NewArrayNode_newArray(GeneratedPluginInjectionProvider injection) {
super("newArray", java.lang.Class.class, int.class, boolean.class);
this.snippetReflection = injection.getInjectedArgument(jdk.graal.compiler.api.replacements.SnippetReflectionProvider.class);
}
}
// class: jdk.graal.compiler.nodes.java.NewArrayNode
// method: newArray(java.lang.Class>,int,boolean)
// generated-by: jdk.graal.compiler.replacements.processor.GeneratedNodeIntrinsicPlugin$ConstructorPlugin
@ExcludeFromJacocoGeneratedReport("deferred plugin support that is only called in libgraal")
final class PluginReplacementNode_NewArrayNode_newArray implements PluginReplacementNode.ReplacementFunction {
static PluginReplacementNode.ReplacementFunction FUNCTION = new PluginReplacementNode_NewArrayNode_newArray();
@Override
public boolean replace(GraphBuilderContext b, GeneratedPluginInjectionProvider injection, Stamp stamp, NodeInputList args) {
jdk.vm.ci.meta.ResolvedJavaType arg0;
if (args.get(0).isConstant()) {
jdk.vm.ci.meta.JavaConstant cst = args.get(0).asJavaConstant();
arg0 = b.getConstantReflection()/* B CONSTANT_REFLECTION */.asJavaType(cst);
if (arg0 == null) {
arg0 = injection.getInjectedArgument(jdk.graal.compiler.api.replacements.SnippetReflectionProvider.class)/* B SNIPPET_REFLECTION */.asObject(jdk.vm.ci.meta.ResolvedJavaType.class, cst);
}
} else {
return false;
}
ValueNode arg1 = args.get(1);
boolean arg2;
if (args.get(2).isConstant()) {
arg2 = args.get(2).asJavaConstant().asInt() != 0;
} else {
return false;
}
jdk.graal.compiler.nodes.java.NewArrayNode node = new jdk.graal.compiler.nodes.java.NewArrayNode(arg0, arg1, arg2);
b.addPush(JavaKind.Object, node);
return true;
}
}
public class PluginFactory_NewArrayNode implements GeneratedPluginFactory {
@Override
public void registerPlugins(InvocationPlugins plugins, GeneratedPluginInjectionProvider injection) {
plugins.register(jdk.graal.compiler.nodes.java.NewArrayNode.class, new Plugin_NewArrayNode_newArray(injection));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy