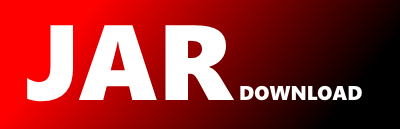
jdk.graal.compiler.truffle.TruffleCompilerOptionsOptionDescriptors Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compiler Show documentation
Show all versions of compiler Show documentation
The GraalVM compiler and the Graal-truffle optimizer.
// CheckStyle: stop header check
// CheckStyle: stop line length check
// GENERATED CONTENT - DO NOT EDIT
// Source: TruffleCompilerOptions.java
package jdk.graal.compiler.truffle;
import java.util.*;
import jdk.graal.compiler.options.*;
import jdk.graal.compiler.options.OptionType;
import jdk.graal.compiler.options.OptionStability;
public class TruffleCompilerOptionsOptionDescriptors implements Iterable {
static {
for (OptionDescriptor d : new TruffleCompilerOptionsOptionDescriptors()) {
// consume all options once to ensure that option key descriptors are set
// this is necessary if the option descriptors are not consumed as services
assert d.getOptionKey().getDescriptor() != null;
}
}
public OptionDescriptor get(String value) {
switch (value) {
// CheckStyle: stop line length check
case "compiler.DiagnoseFailure": {
return OptionDescriptor.create(
/*name*/ "compiler.DiagnoseFailure",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Forces diagnostics for compilation failures (default: false).",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "DiagnoseFailure",
/*option*/ TruffleCompilerOptions.DiagnoseFailure,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.EncodedGraphCache": {
return OptionDescriptor.create(
/*name*/ "compiler.EncodedGraphCache",
/*optionType*/ OptionType.Expert,
/*optionValueType*/ Boolean.class,
/*help*/ "Cache encoded graphs across Truffle compilations to speed up partial evaluation. (default: true).",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "EncodedGraphCache",
/*option*/ TruffleCompilerOptions.EncodedGraphCache,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.ExcludeAssertions": {
return OptionDescriptor.create(
/*name*/ "compiler.ExcludeAssertions",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Exclude assertion code from Truffle compilations (default: true)",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "ExcludeAssertions",
/*option*/ TruffleCompilerOptions.ExcludeAssertions,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.FirstTierInliningPolicy": {
return OptionDescriptor.create(
/*name*/ "compiler.FirstTierInliningPolicy",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ String.class,
/*help*/ "Explicitly pick a first tier inlining policy by name (None, TrivialOnly). If empty (default) the lowest priority policy (TrivialOnly) is chosen.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "FirstTierInliningPolicy",
/*option*/ TruffleCompilerOptions.FirstTierInliningPolicy,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.FirstTierUseEconomy": {
return OptionDescriptor.create(
/*name*/ "compiler.FirstTierUseEconomy",
/*optionType*/ OptionType.Expert,
/*optionValueType*/ Boolean.class,
/*help*/ "Whether to use the economy configuration in the first-tier compilations. (default: true, syntax: true|false)",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "FirstTierUseEconomy",
/*option*/ TruffleCompilerOptions.FirstTierUseEconomy,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InlineAcrossTruffleBoundary": {
return OptionDescriptor.create(
/*name*/ "compiler.InlineAcrossTruffleBoundary",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Enable inlining across Truffle boundary",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InlineAcrossTruffleBoundary",
/*option*/ TruffleCompilerOptions.InlineAcrossTruffleBoundary,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InlineOnly": {
return OptionDescriptor.create(
/*name*/ "compiler.InlineOnly",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ String.class,
/*help*/ "Restrict inlined methods to ','-separated list of includes (or excludes prefixed with '~'). No restriction by default. (usage: ,,...)",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InlineOnly",
/*option*/ TruffleCompilerOptions.InlineOnly,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.Inlining": {
return OptionDescriptor.create(
/*name*/ "compiler.Inlining",
/*optionType*/ OptionType.Expert,
/*optionValueType*/ Boolean.class,
/*help*/ "Enable automatic inlining of guest language call targets (default: true, usage: true|false).",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "Inlining",
/*option*/ TruffleCompilerOptions.Inlining,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InliningExpansionBudget": {
return OptionDescriptor.create(
/*name*/ "compiler.InliningExpansionBudget",
/*optionType*/ OptionType.Expert,
/*optionValueType*/ Integer.class,
/*help*/ "The base expansion budget for language-agnostic inlining (default: 12000). Syntax: [1, inf)",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InliningExpansionBudget",
/*option*/ TruffleCompilerOptions.InliningExpansionBudget,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InliningInliningBudget": {
return OptionDescriptor.create(
/*name*/ "compiler.InliningInliningBudget",
/*optionType*/ OptionType.Expert,
/*optionValueType*/ Integer.class,
/*help*/ "The base inlining budget for language-agnostic inlining (default: 12000). Syntax: [1, inf)",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InliningInliningBudget",
/*option*/ TruffleCompilerOptions.InliningInliningBudget,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InliningPolicy": {
return OptionDescriptor.create(
/*name*/ "compiler.InliningPolicy",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ String.class,
/*help*/ "Explicitly pick a inlining policy by name. If empty (default) the highest priority chosen by default.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InliningPolicy",
/*option*/ TruffleCompilerOptions.InliningPolicy,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InliningRecursionDepth": {
return OptionDescriptor.create(
/*name*/ "compiler.InliningRecursionDepth",
/*optionType*/ OptionType.Expert,
/*optionValueType*/ Integer.class,
/*help*/ "Maximum depth for recursive inlining (default: 2, usage: [0, inf)).",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InliningRecursionDepth",
/*option*/ TruffleCompilerOptions.InliningRecursionDepth,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InliningUseSize": {
return OptionDescriptor.create(
/*name*/ "compiler.InliningUseSize",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Use the graph size as a cost model during inlining (default: false).",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InliningUseSize",
/*option*/ TruffleCompilerOptions.InliningUseSize,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InstrumentBoundaries": {
return OptionDescriptor.create(
/*name*/ "compiler.InstrumentBoundaries",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Instrument Truffle boundaries and output profiling information to the standard output.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InstrumentBoundaries",
/*option*/ TruffleCompilerOptions.InstrumentBoundaries,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InstrumentBoundariesPerInlineSite": {
return OptionDescriptor.create(
/*name*/ "compiler.InstrumentBoundariesPerInlineSite",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Instrument Truffle boundaries by considering different inlining sites as different branches.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InstrumentBoundariesPerInlineSite",
/*option*/ TruffleCompilerOptions.InstrumentBoundariesPerInlineSite,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InstrumentBranches": {
return OptionDescriptor.create(
/*name*/ "compiler.InstrumentBranches",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Instrument branches and output profiling information to the standard output.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InstrumentBranches",
/*option*/ TruffleCompilerOptions.InstrumentBranches,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InstrumentBranchesPerInlineSite": {
return OptionDescriptor.create(
/*name*/ "compiler.InstrumentBranchesPerInlineSite",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Instrument branches by considering different inlining sites as different branches.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InstrumentBranchesPerInlineSite",
/*option*/ TruffleCompilerOptions.InstrumentBranchesPerInlineSite,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InstrumentFilter": {
return OptionDescriptor.create(
/*name*/ "compiler.InstrumentFilter",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ String.class,
/*help*/ "Method filter for host methods in which to add instrumentation (syntax: ,,....)",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InstrumentFilter",
/*option*/ TruffleCompilerOptions.InstrumentFilter,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.InstrumentationTableSize": {
return OptionDescriptor.create(
/*name*/ "compiler.InstrumentationTableSize",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Integer.class,
/*help*/ "Maximum number of instrumentation counters available (default: 10000, syntax: [1, inf))",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "InstrumentationTableSize",
/*option*/ TruffleCompilerOptions.InstrumentationTableSize,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.IterativePartialEscape": {
return OptionDescriptor.create(
/*name*/ "compiler.IterativePartialEscape",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Run the partial escape analysis iteratively in Truffle compilation.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "IterativePartialEscape",
/*option*/ TruffleCompilerOptions.IterativePartialEscape,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.LogInlinedTargets": {
return OptionDescriptor.create(
/*name*/ "compiler.LogInlinedTargets",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Logs inlined targets for statistical purposes (default: false).",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "LogInlinedTargets",
/*option*/ TruffleCompilerOptions.LogInlinedTargets,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.MaximumGraalGraphSize": {
return OptionDescriptor.create(
/*name*/ "compiler.MaximumGraalGraphSize",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Integer.class,
/*help*/ "Stop partial evaluation when the graph exceeded this size (default: 150000, syntax: [1, inf))",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "MaximumGraalGraphSize",
/*option*/ TruffleCompilerOptions.MaximumGraalGraphSize,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.MethodExpansionStatistics": {
return OptionDescriptor.create(
/*name*/ "compiler.MethodExpansionStatistics",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ jdk.graal.compiler.truffle.TruffleCompilerOptions.CompilationTiers.class,
/*help*/ "Print statistics on expanded Java methods during partial evaluation at the end of a run.(syntax: true|false|peTier|truffleTier|lowTier|,,...)%nAccepted values are:%n true - Collect data for the default tier 'truffleTier'.%n false - No data will be collected.%nOr one or multiple tiers separated by comma (e.g. truffleTier,lowTier):%n peTier - After partial evaluation without additional phases applied.%n truffleTier - After partial evaluation with additional phases applied.%n lowTier - After low tier phases were applied.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "MethodExpansionStatistics",
/*option*/ TruffleCompilerOptions.MethodExpansionStatistics,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.NodeExpansionStatistics": {
return OptionDescriptor.create(
/*name*/ "compiler.NodeExpansionStatistics",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ jdk.graal.compiler.truffle.TruffleCompilerOptions.CompilationTiers.class,
/*help*/ "Print statistics on expanded Truffle nodes during partial evaluation at the end of a run.(syntax: true|false|peTier|truffleTier|lowTier|,,...)%nAccepted values are:%n true - Collect data for the default tier 'truffleTier'.%n false - No data will be collected.%nOr one or multiple tiers separated by comma (e.g. truffleTier,lowTier):%n peTier - After partial evaluation without additional phases applied.%n truffleTier - After partial evaluation with additional phases applied.%n lowTier - After low tier phases were applied.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "NodeExpansionStatistics",
/*option*/ TruffleCompilerOptions.NodeExpansionStatistics,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.NodeSourcePositions": {
return OptionDescriptor.create(
/*name*/ "compiler.NodeSourcePositions",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Enable node source positions in truffle partial evaluations.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "NodeSourcePositions",
/*option*/ TruffleCompilerOptions.NodeSourcePositions,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.ParsePEGraphsWithAssumptions": {
return OptionDescriptor.create(
/*name*/ "compiler.ParsePEGraphsWithAssumptions",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Allow assumptions during parsing of seed graphs for partial evaluation. Disables the persistent encoded graph cache 'engine.EncodedGraphCache'. (default: false).",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "ParsePEGraphsWithAssumptions",
/*option*/ TruffleCompilerOptions.ParsePEGraphsWithAssumptions,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.TraceInlining": {
return OptionDescriptor.create(
/*name*/ "compiler.TraceInlining",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Print information for inlining decisions.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "TraceInlining",
/*option*/ TruffleCompilerOptions.TraceInlining,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.TraceInliningDetails": {
return OptionDescriptor.create(
/*name*/ "compiler.TraceInliningDetails",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Boolean.class,
/*help*/ "Print detailed information for inlining (i.e. the entire explored call tree).",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "TraceInliningDetails",
/*option*/ TruffleCompilerOptions.TraceInliningDetails,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.TraceMethodExpansion": {
return OptionDescriptor.create(
/*name*/ "compiler.TraceMethodExpansion",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ jdk.graal.compiler.truffle.TruffleCompilerOptions.CompilationTiers.class,
/*help*/ "Print a tree of all expanded Java methods with statistics after each compilation. (syntax: true|false|peTier|truffleTier|lowTier|,,...)%nAccepted values are:%n true - Collect data for the default tier 'truffleTier'.%n false - No data will be collected.%nOr one or multiple tiers separated by comma (e.g. truffleTier,lowTier):%n peTier - After partial evaluation without additional phases applied.%n truffleTier - After partial evaluation with additional phases applied.%n lowTier - After low tier phases were applied.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "TraceMethodExpansion",
/*option*/ TruffleCompilerOptions.TraceMethodExpansion,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.TraceNodeExpansion": {
return OptionDescriptor.create(
/*name*/ "compiler.TraceNodeExpansion",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ jdk.graal.compiler.truffle.TruffleCompilerOptions.CompilationTiers.class,
/*help*/ "Print a tree of all expanded Truffle nodes with statistics after each compilation. (syntax: true|false|peTier|truffleTier|lowTier|,,...)%nAccepted values are:%n true - Collect data for the default tier 'truffleTier'.%n false - No data will be collected.%nOr one or multiple tiers separated by comma (e.g. truffleTier,lowTier):%n peTier - After partial evaluation without additional phases applied.%n truffleTier - After partial evaluation with additional phases applied.%n lowTier - After low tier phases were applied.",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "TraceNodeExpansion",
/*option*/ TruffleCompilerOptions.TraceNodeExpansion,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.TracePerformanceWarnings": {
return OptionDescriptor.create(
/*name*/ "compiler.TracePerformanceWarnings",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ jdk.graal.compiler.truffle.TruffleCompilerOptions.PerformanceWarnings.class,
/*help*/ "Print potential performance problems, Performance warnings are: call, instanceof, store, frame_merge, trivial. (syntax: none|all|,,...)",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "TracePerformanceWarnings",
/*option*/ TruffleCompilerOptions.TracePerformanceWarnings,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.TraceStackTraceLimit": {
return OptionDescriptor.create(
/*name*/ "compiler.TraceStackTraceLimit",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ Integer.class,
/*help*/ "Number of stack trace elements printed by TraceTruffleTransferToInterpreter, TraceTruffleAssumptions and TraceDeoptimizeFrame (default: 20). Syntax: [1, inf).",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "TraceStackTraceLimit",
/*option*/ TruffleCompilerOptions.TraceStackTraceLimit,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
case "compiler.TreatPerformanceWarningsAsErrors": {
return OptionDescriptor.create(
/*name*/ "compiler.TreatPerformanceWarningsAsErrors",
/*optionType*/ OptionType.Debug,
/*optionValueType*/ jdk.graal.compiler.truffle.TruffleCompilerOptions.PerformanceWarnings.class,
/*help*/ "Treat performance warnings as error. Handling of the error depends on the CompilationFailureAction option value. Performance warnings are: call, instanceof, store, frame_merge, trivial. (syntax: none|all|,,...)",
/*declaringClass*/ TruffleCompilerOptions.class,
/*fieldName*/ "TreatPerformanceWarningsAsErrors",
/*option*/ TruffleCompilerOptions.TreatPerformanceWarningsAsErrors,
/*stability*/ OptionStability.EXPERIMENTAL,
/*deprecated*/ false,
/*deprecationMessage*/ "");
}
// CheckStyle: resume line length check
}
return null;
}
@Override
public Iterator iterator() {
return new Iterator<>() {
int i = 0;
@Override
public boolean hasNext() {
return i < 33;
}
@Override
public OptionDescriptor next() {
switch (i++) {
case 0: return get("compiler.DiagnoseFailure");
case 1: return get("compiler.EncodedGraphCache");
case 2: return get("compiler.ExcludeAssertions");
case 3: return get("compiler.FirstTierInliningPolicy");
case 4: return get("compiler.FirstTierUseEconomy");
case 5: return get("compiler.InlineAcrossTruffleBoundary");
case 6: return get("compiler.InlineOnly");
case 7: return get("compiler.Inlining");
case 8: return get("compiler.InliningExpansionBudget");
case 9: return get("compiler.InliningInliningBudget");
case 10: return get("compiler.InliningPolicy");
case 11: return get("compiler.InliningRecursionDepth");
case 12: return get("compiler.InliningUseSize");
case 13: return get("compiler.InstrumentBoundaries");
case 14: return get("compiler.InstrumentBoundariesPerInlineSite");
case 15: return get("compiler.InstrumentBranches");
case 16: return get("compiler.InstrumentBranchesPerInlineSite");
case 17: return get("compiler.InstrumentFilter");
case 18: return get("compiler.InstrumentationTableSize");
case 19: return get("compiler.IterativePartialEscape");
case 20: return get("compiler.LogInlinedTargets");
case 21: return get("compiler.MaximumGraalGraphSize");
case 22: return get("compiler.MethodExpansionStatistics");
case 23: return get("compiler.NodeExpansionStatistics");
case 24: return get("compiler.NodeSourcePositions");
case 25: return get("compiler.ParsePEGraphsWithAssumptions");
case 26: return get("compiler.TraceInlining");
case 27: return get("compiler.TraceInliningDetails");
case 28: return get("compiler.TraceMethodExpansion");
case 29: return get("compiler.TraceNodeExpansion");
case 30: return get("compiler.TracePerformanceWarnings");
case 31: return get("compiler.TraceStackTraceLimit");
case 32: return get("compiler.TreatPerformanceWarningsAsErrors");
}
throw new NoSuchElementException();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy