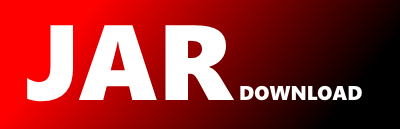
com.oracle.truffle.js.builtins.DebugBuiltinsFactory Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.builtins;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.CompareCharsUTF16Node;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugArrayTypeNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugAssertIntNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugClassNameNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugClassNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugContinueInInterpreter;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugCreateSafeInteger;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugDumpCountersNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugDumpFunctionTreeNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugHeapDumpNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugIsHolesArrayNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugJSStackNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugLoadModuleNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugNeverPartOfCompilationNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugPrintObjectNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugPrintSourceAttribution;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugShapeNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugStringCompareNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugSystemProperties;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugSystemProperty;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugToJavaStringNode;
import com.oracle.truffle.js.builtins.DebugBuiltins.DebugTypedArrayDetachBufferNode;
import com.oracle.truffle.js.nodes.JavaScriptNode;
import com.oracle.truffle.js.nodes.function.JSBuiltin;
import com.oracle.truffle.js.runtime.JSContext;
import com.oracle.truffle.js.runtime.SafeInteger;
import com.oracle.truffle.js.runtime.builtins.JSFunctionObject;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import java.lang.invoke.VarHandle;
import java.util.Objects;
@GeneratedBy(DebugBuiltins.class)
@SuppressWarnings({"javadoc", "unused"})
public final class DebugBuiltinsFactory {
/**
* Debug Info:
* Specialization {@link DebugContinueInInterpreter#continueInInterpreter}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugContinueInInterpreter.class)
@SuppressWarnings("javadoc")
public static final class DebugContinueInInterpreterNodeGen extends DebugContinueInInterpreter {
private DebugContinueInInterpreterNodeGen(JSContext context, JSBuiltin builtin, boolean invalidate, JavaScriptNode[] arguments) {
super(context, builtin, invalidate);
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {};
}
@Override
public Object execute(VirtualFrame frameValue) {
return continueInInterpreter();
}
@NeverDefault
public static DebugContinueInInterpreter create(JSContext context, JSBuiltin builtin, boolean invalidate, JavaScriptNode[] arguments) {
return new DebugContinueInInterpreterNodeGen(context, builtin, invalidate, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugClassNode#clazz}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugClassNode.class)
@SuppressWarnings("javadoc")
public static final class DebugClassNodeGen extends DebugClassNode {
@Child private JavaScriptNode arguments0_;
private DebugClassNodeGen(JSContext context, JSBuiltin builtin, boolean getName, JavaScriptNode[] arguments) {
super(context, builtin, getName);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return clazz(arguments0Value_);
}
@NeverDefault
public static DebugClassNode create(JSContext context, JSBuiltin builtin, boolean getName, JavaScriptNode[] arguments) {
return new DebugClassNodeGen(context, builtin, getName, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugClassNameNode#clazz}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugClassNameNode.class)
@SuppressWarnings("javadoc")
public static final class DebugClassNameNodeGen extends DebugClassNameNode {
@Child private JavaScriptNode arguments0_;
private DebugClassNameNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return DebugClassNameNode.clazz(arguments0Value_);
}
@NeverDefault
public static DebugClassNameNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugClassNameNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugShapeNode#shape}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugShapeNode.class)
@SuppressWarnings("javadoc")
public static final class DebugShapeNodeGen extends DebugShapeNode {
@Child private JavaScriptNode arguments0_;
private DebugShapeNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return DebugShapeNode.shape(arguments0Value_);
}
@NeverDefault
public static DebugShapeNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugShapeNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugDumpCountersNode#dumpCounters}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugDumpCountersNode.class)
@SuppressWarnings("javadoc")
public static final class DebugDumpCountersNodeGen extends DebugDumpCountersNode {
private DebugDumpCountersNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {};
}
@Override
public Object execute(VirtualFrame frameValue) {
return DebugDumpCountersNode.dumpCounters();
}
@NeverDefault
public static DebugDumpCountersNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugDumpCountersNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugDumpFunctionTreeNode#dumpFunctionTree}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugDumpFunctionTreeNode.class)
@SuppressWarnings("javadoc")
public static final class DebugDumpFunctionTreeNodeGen extends DebugDumpFunctionTreeNode {
@Child private JavaScriptNode arguments0_;
private DebugDumpFunctionTreeNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return dumpFunctionTree(arguments0Value_);
}
@NeverDefault
public static DebugDumpFunctionTreeNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugDumpFunctionTreeNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugPrintObjectNode#printObject}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugPrintObjectNode.class)
@SuppressWarnings("javadoc")
public static final class DebugPrintObjectNodeGen extends DebugPrintObjectNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
/**
* State Info:
* 0: SpecializationActive {@link DebugPrintObjectNode#printObject}
*
*/
@CompilationFinal private int state_0_;
private DebugPrintObjectNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[DebugBuiltins.DebugPrintObjectNode.printObject(JSDynamicObject, Object)] */ && arguments0Value_ instanceof JSDynamicObject) {
JSDynamicObject arguments0Value__ = (JSDynamicObject) arguments0Value_;
return printObject(arguments0Value__, arguments1Value_);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_, arguments1Value_);
}
private Object executeAndSpecialize(Object arguments0Value, Object arguments1Value) {
int state_0 = this.state_0_;
if (arguments0Value instanceof JSDynamicObject) {
JSDynamicObject arguments0Value_ = (JSDynamicObject) arguments0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[DebugBuiltins.DebugPrintObjectNode.printObject(JSDynamicObject, Object)] */;
this.state_0_ = state_0;
return printObject(arguments0Value_, arguments1Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_, this.arguments1_}, arguments0Value, arguments1Value);
}
@NeverDefault
public static DebugPrintObjectNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugPrintObjectNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugToJavaStringNode#toJavaString}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugToJavaStringNode.class)
@SuppressWarnings("javadoc")
public static final class DebugToJavaStringNodeGen extends DebugToJavaStringNode {
@Child private JavaScriptNode arguments0_;
private DebugToJavaStringNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return DebugToJavaStringNode.toJavaString(arguments0Value_);
}
@NeverDefault
public static DebugToJavaStringNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugToJavaStringNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugPrintSourceAttribution#printSourceAttribution(JSFunctionObject)}
* Activation probability: 0.48333
* With/without class size: 9/0 bytes
* Specialization {@link DebugPrintSourceAttribution#printSourceAttribution(TruffleString)}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
* Specialization {@link DebugPrintSourceAttribution#illegalArgument}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(DebugPrintSourceAttribution.class)
@SuppressWarnings("javadoc")
public static final class DebugPrintSourceAttributionNodeGen extends DebugPrintSourceAttribution {
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link DebugPrintSourceAttribution#printSourceAttribution(JSFunctionObject)}
* 1: SpecializationActive {@link DebugPrintSourceAttribution#printSourceAttribution(TruffleString)}
* 2: SpecializationActive {@link DebugPrintSourceAttribution#illegalArgument}
*
*/
@CompilationFinal private int state_0_;
private DebugPrintSourceAttributionNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Object arguments0Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[DebugBuiltins.DebugPrintSourceAttribution.printSourceAttribution(JSFunctionObject)] */) && arguments0Value instanceof JSFunctionObject) {
return false;
}
if (!((state_0 & 0b10) != 0 /* is SpecializationActive[DebugBuiltins.DebugPrintSourceAttribution.printSourceAttribution(TruffleString)] */) && arguments0Value instanceof TruffleString) {
return false;
}
return true;
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[DebugBuiltins.DebugPrintSourceAttribution.printSourceAttribution(JSFunctionObject)] || SpecializationActive[DebugBuiltins.DebugPrintSourceAttribution.printSourceAttribution(TruffleString)] || SpecializationActive[DebugBuiltins.DebugPrintSourceAttribution.illegalArgument(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[DebugBuiltins.DebugPrintSourceAttribution.printSourceAttribution(JSFunctionObject)] */ && arguments0Value_ instanceof JSFunctionObject) {
JSFunctionObject arguments0Value__ = (JSFunctionObject) arguments0Value_;
return printSourceAttribution(arguments0Value__);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[DebugBuiltins.DebugPrintSourceAttribution.printSourceAttribution(TruffleString)] */ && arguments0Value_ instanceof TruffleString) {
TruffleString arguments0Value__ = (TruffleString) arguments0Value_;
return printSourceAttribution(arguments0Value__);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[DebugBuiltins.DebugPrintSourceAttribution.illegalArgument(Object)] */) {
if (fallbackGuard_(state_0, arguments0Value_)) {
return illegalArgument(arguments0Value_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private Object executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
if (arguments0Value instanceof JSFunctionObject) {
JSFunctionObject arguments0Value_ = (JSFunctionObject) arguments0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[DebugBuiltins.DebugPrintSourceAttribution.printSourceAttribution(JSFunctionObject)] */;
this.state_0_ = state_0;
return printSourceAttribution(arguments0Value_);
}
if (arguments0Value instanceof TruffleString) {
TruffleString arguments0Value_ = (TruffleString) arguments0Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[DebugBuiltins.DebugPrintSourceAttribution.printSourceAttribution(TruffleString)] */;
this.state_0_ = state_0;
return printSourceAttribution(arguments0Value_);
}
state_0 = state_0 | 0b100 /* add SpecializationActive[DebugBuiltins.DebugPrintSourceAttribution.illegalArgument(Object)] */;
this.state_0_ = state_0;
return illegalArgument(arguments0Value);
}
@NeverDefault
public static DebugPrintSourceAttribution create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugPrintSourceAttributionNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugArrayTypeNode#arraytype}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugArrayTypeNode.class)
@SuppressWarnings("javadoc")
public static final class DebugArrayTypeNodeGen extends DebugArrayTypeNode {
@Child private JavaScriptNode arguments0_;
private DebugArrayTypeNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return arraytype(arguments0Value_);
}
@NeverDefault
public static DebugArrayTypeNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugArrayTypeNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugAssertIntNode#assertInt}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugAssertIntNode.class)
@SuppressWarnings("javadoc")
public static final class DebugAssertIntNodeGen extends DebugAssertIntNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
private DebugAssertIntNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
return assertInt(arguments0Value_, arguments1Value_);
}
@NeverDefault
public static DebugAssertIntNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugAssertIntNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugHeapDumpNode#heapDump}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugHeapDumpNode.class)
@SuppressWarnings("javadoc")
public static final class DebugHeapDumpNodeGen extends DebugHeapDumpNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
private DebugHeapDumpNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
return heapDump(arguments0Value_, arguments1Value_);
}
@NeverDefault
public static DebugHeapDumpNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugHeapDumpNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugStringCompareNode#stringCompare}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(DebugStringCompareNode.class)
@SuppressWarnings("javadoc")
public static final class DebugStringCompareNodeGen extends DebugStringCompareNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
/**
* State Info:
* 0: SpecializationActive {@link DebugStringCompareNode#stringCompare}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link DebugStringCompareNode#stringCompare}
* Parameter: {@link CompareCharsUTF16Node} compareNode
*/
@Child private CompareCharsUTF16Node compareNode_;
private DebugStringCompareNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[DebugBuiltins.DebugStringCompareNode.stringCompare(Object, Object, CompareCharsUTF16Node)] */) {
{
CompareCharsUTF16Node compareNode__ = this.compareNode_;
if (compareNode__ != null) {
return stringCompare(arguments0Value_, arguments1Value_, compareNode__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_, arguments1Value_);
}
private int executeAndSpecialize(Object arguments0Value, Object arguments1Value) {
int state_0 = this.state_0_;
CompareCharsUTF16Node compareNode__ = this.insert((CompareCharsUTF16Node.create()));
Objects.requireNonNull(compareNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.compareNode_ = compareNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[DebugBuiltins.DebugStringCompareNode.stringCompare(Object, Object, CompareCharsUTF16Node)] */;
this.state_0_ = state_0;
return stringCompare(arguments0Value, arguments1Value, compareNode__);
}
@NeverDefault
public static DebugStringCompareNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugStringCompareNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugIsHolesArrayNode#isHolesArray}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugIsHolesArrayNode.class)
@SuppressWarnings("javadoc")
public static final class DebugIsHolesArrayNodeGen extends DebugIsHolesArrayNode {
@Child private JavaScriptNode arguments0_;
private DebugIsHolesArrayNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return isHolesArray(arguments0Value_);
}
@NeverDefault
public static DebugIsHolesArrayNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugIsHolesArrayNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugJSStackNode#printJSStack}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugJSStackNode.class)
@SuppressWarnings("javadoc")
public static final class DebugJSStackNodeGen extends DebugJSStackNode {
private DebugJSStackNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {};
}
@Override
public Object execute(VirtualFrame frameValue) {
return printJSStack();
}
@NeverDefault
public static DebugJSStackNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugJSStackNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugLoadModuleNode#loadModule}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugLoadModuleNode.class)
@SuppressWarnings("javadoc")
public static final class DebugLoadModuleNodeGen extends DebugLoadModuleNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
private DebugLoadModuleNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
return loadModule(arguments0Value_, arguments1Value_);
}
@NeverDefault
public static DebugLoadModuleNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugLoadModuleNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugSystemProperties#systemProperties}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugSystemProperties.class)
@SuppressWarnings("javadoc")
public static final class DebugSystemPropertiesNodeGen extends DebugSystemProperties {
private DebugSystemPropertiesNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {};
}
@Override
public Object execute(VirtualFrame frameValue) {
return systemProperties();
}
@NeverDefault
public static DebugSystemProperties create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugSystemPropertiesNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugSystemProperty#systemProperty}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugSystemProperty.class)
@SuppressWarnings("javadoc")
public static final class DebugSystemPropertyNodeGen extends DebugSystemProperty {
@Child private JavaScriptNode arguments0_;
private DebugSystemPropertyNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return DebugSystemProperty.systemProperty(arguments0Value_);
}
@NeverDefault
public static DebugSystemProperty create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugSystemPropertyNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugTypedArrayDetachBufferNode#detachBuffer}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugTypedArrayDetachBufferNode.class)
@SuppressWarnings("javadoc")
public static final class DebugTypedArrayDetachBufferNodeGen extends DebugTypedArrayDetachBufferNode {
@Child private JavaScriptNode arguments0_;
private DebugTypedArrayDetachBufferNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return DebugTypedArrayDetachBufferNode.detachBuffer(arguments0Value_);
}
@NeverDefault
public static DebugTypedArrayDetachBufferNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugTypedArrayDetachBufferNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugCreateSafeInteger#createSafeInteger(int)}
* Activation probability: 0.48333
* With/without class size: 9/0 bytes
* Specialization {@link DebugCreateSafeInteger#createSafeInteger(SafeInteger)}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
* Specialization {@link DebugCreateSafeInteger#createSafeInteger(Object)}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(DebugCreateSafeInteger.class)
@SuppressWarnings("javadoc")
public static final class DebugCreateSafeIntegerNodeGen extends DebugCreateSafeInteger {
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link DebugCreateSafeInteger#createSafeInteger(int)}
* 1: SpecializationActive {@link DebugCreateSafeInteger#createSafeInteger(SafeInteger)}
* 2: SpecializationActive {@link DebugCreateSafeInteger#createSafeInteger(Object)}
*
*/
@CompilationFinal private int state_0_;
private DebugCreateSafeIntegerNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
if ((state_0 & 0b110) == 0 /* only-active SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(int)] */ && (state_0 != 0 /* is-not SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(int)] && SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(SafeInteger)] && SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(Object)] */)) {
return execute_int0(state_0, frameValue);
} else {
return execute_generic1(state_0, frameValue);
}
}
private Object execute_int0(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
int arguments0Value_;
try {
arguments0Value_ = this.arguments0_.executeInt(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(ex.getResult());
}
assert (state_0 & 0b1) != 0 /* is SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(int)] */;
return DebugCreateSafeInteger.createSafeInteger(arguments0Value_);
}
private Object execute_generic1(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(int)] || SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(SafeInteger)] || SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(int)] */ && arguments0Value_ instanceof Integer) {
int arguments0Value__ = (int) arguments0Value_;
return DebugCreateSafeInteger.createSafeInteger(arguments0Value__);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(SafeInteger)] */ && arguments0Value_ instanceof SafeInteger) {
SafeInteger arguments0Value__ = (SafeInteger) arguments0Value_;
return DebugCreateSafeInteger.createSafeInteger(arguments0Value__);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(Object)] */) {
return DebugCreateSafeInteger.createSafeInteger(arguments0Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private SafeInteger executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
if (arguments0Value instanceof Integer) {
int arguments0Value_ = (int) arguments0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(int)] */;
this.state_0_ = state_0;
return DebugCreateSafeInteger.createSafeInteger(arguments0Value_);
}
if (arguments0Value instanceof SafeInteger) {
SafeInteger arguments0Value_ = (SafeInteger) arguments0Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(SafeInteger)] */;
this.state_0_ = state_0;
return DebugCreateSafeInteger.createSafeInteger(arguments0Value_);
}
state_0 = state_0 | 0b100 /* add SpecializationActive[DebugBuiltins.DebugCreateSafeInteger.createSafeInteger(Object)] */;
this.state_0_ = state_0;
return DebugCreateSafeInteger.createSafeInteger(arguments0Value);
}
@NeverDefault
public static DebugCreateSafeInteger create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugCreateSafeIntegerNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link DebugNeverPartOfCompilationNode#neverPartOfCompilation}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(DebugNeverPartOfCompilationNode.class)
@SuppressWarnings("javadoc")
public static final class DebugNeverPartOfCompilationNodeGen extends DebugNeverPartOfCompilationNode {
private DebugNeverPartOfCompilationNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {};
}
@Override
public Object execute(VirtualFrame frameValue) {
return DebugNeverPartOfCompilationNode.neverPartOfCompilation();
}
@NeverDefault
public static DebugNeverPartOfCompilationNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new DebugNeverPartOfCompilationNodeGen(context, builtin, arguments);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy