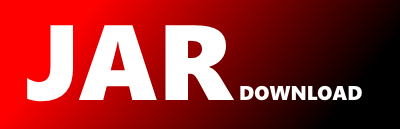
com.oracle.truffle.js.builtins.JavaBuiltinsFactory Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.builtins;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaAddToClasspathNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaExtendNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaFromNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaIsJavaFunctionNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaIsJavaMethodNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaIsJavaObject;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaIsScriptFunctionNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaIsScriptObjectNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaIsTypeNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaSuperNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaSynchronizedNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaToNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaTypeNameNode;
import com.oracle.truffle.js.builtins.JavaBuiltins.JavaTypeNode;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.JavaScriptNode;
import com.oracle.truffle.js.nodes.access.WriteElementNode;
import com.oracle.truffle.js.nodes.cast.JSToStringNode;
import com.oracle.truffle.js.nodes.function.JSBuiltin;
import com.oracle.truffle.js.nodes.interop.ImportValueNode;
import com.oracle.truffle.js.runtime.JSConfig;
import com.oracle.truffle.js.runtime.JSContext;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
@GeneratedBy(JavaBuiltins.class)
@SuppressWarnings({"javadoc", "unused"})
public final class JavaBuiltinsFactory {
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* Debug Info:
* Specialization {@link JavaTypeNode#type}
* Activation probability: 0.65000
* With/without class size: 11/0 bytes
* Specialization {@link JavaTypeNode#typeNoString}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(JavaTypeNode.class)
@SuppressWarnings("javadoc")
static final class JavaTypeNodeGen extends JavaTypeNode {
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link JavaTypeNode#type}
* 1: SpecializationActive {@link JavaTypeNode#typeNoString}
*
*/
@CompilationFinal private int state_0_;
private JavaTypeNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[JavaBuiltins.JavaTypeNode.type(TruffleString)] || SpecializationActive[JavaBuiltins.JavaTypeNode.typeNoString(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JavaBuiltins.JavaTypeNode.type(TruffleString)] */ && arguments0Value_ instanceof TruffleString) {
TruffleString arguments0Value__ = (TruffleString) arguments0Value_;
return type(arguments0Value__);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JavaBuiltins.JavaTypeNode.typeNoString(Object)] */) {
if ((!(JSGuards.isString(arguments0Value_)))) {
return typeNoString(arguments0Value_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private Object executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
if (arguments0Value instanceof TruffleString) {
TruffleString arguments0Value_ = (TruffleString) arguments0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JavaBuiltins.JavaTypeNode.type(TruffleString)] */;
this.state_0_ = state_0;
return type(arguments0Value_);
}
if ((!(JSGuards.isString(arguments0Value)))) {
state_0 = state_0 | 0b10 /* add SpecializationActive[JavaBuiltins.JavaTypeNode.typeNoString(Object)] */;
this.state_0_ = state_0;
return typeNoString(arguments0Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_}, arguments0Value);
}
@NeverDefault
public static JavaTypeNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaTypeNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaTypeNameNode#typeNameJavaInteropClass}
* Activation probability: 0.65000
* With/without class size: 19/8 bytes
* Specialization {@link JavaTypeNameNode#nonType}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(JavaTypeNameNode.class)
@SuppressWarnings("javadoc")
static final class JavaTypeNameNodeGen extends JavaTypeNameNode {
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link JavaTypeNameNode#typeNameJavaInteropClass}
* 1: GuardActive[guardIndex=0] {@link JavaTypeNameNode#typeNameJavaInteropClass}
* 2: SpecializationActive {@link JavaTypeNameNode#nonType}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JavaTypeNameNode#typeNameJavaInteropClass}
* Parameter: {@link InteropLibrary} typeInterop
*/
@Child private InteropLibrary typeNameJavaInteropClass_typeInterop_;
/**
* Source Info:
* Specialization: {@link JavaTypeNameNode#typeNameJavaInteropClass}
* Parameter: {@link ImportValueNode} importValue
*/
@Child private ImportValueNode typeNameJavaInteropClass_importValue_;
private JavaTypeNameNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Object arguments0Value) {
{
InteropLibrary typeInterop__ = this.typeNameJavaInteropClass_typeInterop_;
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[JavaBuiltins.JavaTypeNameNode.typeNameJavaInteropClass(Object, InteropLibrary, ImportValueNode)] */) && (((state_0 & 0b10)) == 0 /* is-not GuardActive[specialization=JavaBuiltins.JavaTypeNameNode.typeNameJavaInteropClass(Object, InteropLibrary, ImportValueNode), guardIndex=0] */ || typeInterop__ == null || (isJavaInteropClass(arguments0Value, typeInterop__)))) {
return false;
}
}
return true;
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if ((state_0 & 0b101) != 0 /* is SpecializationActive[JavaBuiltins.JavaTypeNameNode.typeNameJavaInteropClass(Object, InteropLibrary, ImportValueNode)] || SpecializationActive[JavaBuiltins.JavaTypeNameNode.nonType(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JavaBuiltins.JavaTypeNameNode.typeNameJavaInteropClass(Object, InteropLibrary, ImportValueNode)] */) {
{
InteropLibrary typeInterop__ = this.typeNameJavaInteropClass_typeInterop_;
if (typeInterop__ != null) {
ImportValueNode importValue__ = this.typeNameJavaInteropClass_importValue_;
if (importValue__ != null) {
if ((isJavaInteropClass(arguments0Value_, typeInterop__))) {
return typeNameJavaInteropClass(arguments0Value_, typeInterop__, importValue__);
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JavaBuiltins.JavaTypeNameNode.nonType(Object)] */) {
if (fallbackGuard_(state_0, arguments0Value_)) {
return nonType(arguments0Value_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
@SuppressWarnings("unused")
private Object executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
{
InteropLibrary typeInterop__ = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
if (((state_0 & 0b10)) == 0 /* is-not GuardActive[specialization=JavaBuiltins.JavaTypeNameNode.typeNameJavaInteropClass(Object, InteropLibrary, ImportValueNode), guardIndex=0] */) {
Objects.requireNonNull(this.insert(typeInterop__), "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.typeNameJavaInteropClass_typeInterop_ = typeInterop__;
state_0 = state_0 | 0b10 /* add GuardActive[specialization=JavaBuiltins.JavaTypeNameNode.typeNameJavaInteropClass(Object, InteropLibrary, ImportValueNode), guardIndex=0] */;
}
if ((isJavaInteropClass(arguments0Value, typeInterop__))) {
Objects.requireNonNull(this.insert(typeInterop__), "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.typeNameJavaInteropClass_typeInterop_ = typeInterop__;
ImportValueNode importValue__ = this.insert((ImportValueNode.create()));
Objects.requireNonNull(importValue__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.typeNameJavaInteropClass_importValue_ = importValue__;
state_0 = state_0 | 0b1 /* add SpecializationActive[JavaBuiltins.JavaTypeNameNode.typeNameJavaInteropClass(Object, InteropLibrary, ImportValueNode)] */;
this.state_0_ = state_0;
return typeNameJavaInteropClass(arguments0Value, typeInterop__, importValue__);
}
}
state_0 = state_0 | 0b100 /* add SpecializationActive[JavaBuiltins.JavaTypeNameNode.nonType(Object)] */;
this.state_0_ = state_0;
return nonType(arguments0Value);
}
@NeverDefault
public static JavaTypeNameNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaTypeNameNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaExtendNode#extend}
* Activation probability: 1.00000
* With/without class size: 24/1 bytes
*
*/
@GeneratedBy(JavaExtendNode.class)
@SuppressWarnings("javadoc")
static final class JavaExtendNodeGen extends JavaExtendNode {
private static final StateField STATE_0_JavaExtendNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link JavaExtendNode#extend}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_ERROR_BRANCH_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_JavaExtendNode_UPDATER.subUpdater(1, 1)));
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link JavaExtendNode#extend}
* 1: InlinedCache
* Specialization: {@link JavaExtendNode#extend}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
private JavaExtendNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JavaBuiltins.JavaExtendNode.extend(Object[], InlinedBranchProfile)] */ && arguments0Value_ instanceof Object[]) {
Object[] arguments0Value__ = (Object[]) arguments0Value_;
return extend(arguments0Value__, INLINED_ERROR_BRANCH_);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private Object executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
if (arguments0Value instanceof Object[]) {
Object[] arguments0Value_ = (Object[]) arguments0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JavaBuiltins.JavaExtendNode.extend(Object[], InlinedBranchProfile)] */;
this.state_0_ = state_0;
return extend(arguments0Value_, INLINED_ERROR_BRANCH_);
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_}, arguments0Value);
}
@NeverDefault
public static JavaExtendNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaExtendNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaFromNode#from}
* Activation probability: 1.00000
* With/without class size: 36/13 bytes
*
*/
@GeneratedBy(JavaFromNode.class)
@SuppressWarnings("javadoc")
static final class JavaFromNodeGen extends JavaFromNode {
private static final StateField STATE_0_JavaFromNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link JavaFromNode#from}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_ERROR_BRANCH_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_JavaFromNode_UPDATER.subUpdater(1, 1)));
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link JavaFromNode#from}
* 1: InlinedCache
* Specialization: {@link JavaFromNode#from}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link JavaFromNode#from}
* Parameter: {@link InteropLibrary} interop
*/
@Child private InteropLibrary interop_;
/**
* Source Info:
* Specialization: {@link JavaFromNode#from}
* Parameter: {@link ImportValueNode} importValueNode
*/
@Child private ImportValueNode importValueNode_;
/**
* Source Info:
* Specialization: {@link JavaFromNode#from}
* Parameter: {@link WriteElementNode} writeNode
*/
@Child private WriteElementNode writeNode_;
private JavaFromNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JavaBuiltins.JavaFromNode.from(Object, InteropLibrary, ImportValueNode, WriteElementNode, InlinedBranchProfile)] */) {
{
InteropLibrary interop__ = this.interop_;
if (interop__ != null) {
ImportValueNode importValueNode__ = this.importValueNode_;
if (importValueNode__ != null) {
WriteElementNode writeNode__ = this.writeNode_;
if (writeNode__ != null) {
return from(arguments0Value_, interop__, importValueNode__, writeNode__, INLINED_ERROR_BRANCH_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private JSDynamicObject executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
InteropLibrary interop__ = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
Objects.requireNonNull(interop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.interop_ = interop__;
ImportValueNode importValueNode__ = this.insert((ImportValueNode.create()));
Objects.requireNonNull(importValueNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.importValueNode_ = importValueNode__;
WriteElementNode writeNode__ = this.insert((WriteElementNode.createCachedInterop()));
Objects.requireNonNull(writeNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.writeNode_ = writeNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[JavaBuiltins.JavaFromNode.from(Object, InteropLibrary, ImportValueNode, WriteElementNode, InlinedBranchProfile)] */;
this.state_0_ = state_0;
return from(arguments0Value, interop__, importValueNode__, writeNode__, INLINED_ERROR_BRANCH_);
}
@NeverDefault
public static JavaFromNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaFromNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaToNode#to}
* Activation probability: 0.48333
* With/without class size: 9/0 bytes
* Specialization {@link JavaToNode#toNonObject}
* Activation probability: 0.33333
* With/without class size: 12/4 bytes
* Specialization {@link JavaToNode#toNonObject}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(JavaToNode.class)
@SuppressWarnings("javadoc")
static final class JavaToNodeGen extends JavaToNode {
static final ReferenceField TO_NON_OBJECT0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "toNonObject0_cache", ToNonObject0Data.class);
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
/**
* State Info:
* 0: SpecializationActive {@link JavaToNode#to}
* 1: SpecializationActive {@link JavaToNode#toNonObject}
* 2: SpecializationActive {@link JavaToNode#toNonObject}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JavaToNode#to}
* Parameter: {@link InteropLibrary} typeInterop
*/
@Child private InteropLibrary typeInterop;
@UnsafeAccessedField @Child private ToNonObject0Data toNonObject0_cache;
private JavaToNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_};
}
@ExplodeLoop
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[JavaBuiltins.JavaToNode.to(Object, Object, InteropLibrary)] || SpecializationActive[JavaBuiltins.JavaToNode.toNonObject(Object, Object, InteropLibrary, InteropLibrary)] || SpecializationActive[JavaBuiltins.JavaToNode.toNonObject(Object, Object, InteropLibrary, InteropLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JavaBuiltins.JavaToNode.to(Object, Object, InteropLibrary)] */) {
{
InteropLibrary typeInterop_ = this.typeInterop;
if (typeInterop_ != null) {
if ((JSGuards.isJSObject(arguments0Value_))) {
return to(arguments0Value_, arguments1Value_, typeInterop_);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JavaBuiltins.JavaToNode.toNonObject(Object, Object, InteropLibrary, InteropLibrary)] */) {
ToNonObject0Data s1_ = this.toNonObject0_cache;
while (s1_ != null) {
{
InteropLibrary typeInterop_1 = this.typeInterop;
if (typeInterop_1 != null) {
if ((s1_.objInterop_.accepts(arguments0Value_)) && (!(JSGuards.isJSObject(arguments0Value_)))) {
return toNonObject(arguments0Value_, arguments1Value_, s1_.objInterop_, typeInterop_1);
}
}
}
s1_ = s1_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JavaBuiltins.JavaToNode.toNonObject(Object, Object, InteropLibrary, InteropLibrary)] */) {
{
InteropLibrary typeInterop_1 = this.typeInterop;
if (typeInterop_1 != null) {
if ((!(JSGuards.isJSObject(arguments0Value_)))) {
return this.toNonObject1Boundary(state_0, arguments0Value_, arguments1Value_, typeInterop_1);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_, arguments1Value_);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object toNonObject1Boundary(int state_0, Object arguments0Value_, Object arguments1Value_, InteropLibrary typeInterop_1) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary objInterop__ = (INTEROP_LIBRARY_.getUncached(arguments0Value_));
return toNonObject(arguments0Value_, arguments1Value_, objInterop__, typeInterop_1);
}
} finally {
encapsulating_.set(prev_);
}
}
private Object executeAndSpecialize(Object arguments0Value, Object arguments1Value) {
int state_0 = this.state_0_;
if ((JSGuards.isJSObject(arguments0Value))) {
InteropLibrary typeInterop_;
InteropLibrary typeInterop__shared = this.typeInterop;
if (typeInterop__shared != null) {
typeInterop_ = typeInterop__shared;
} else {
typeInterop_ = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
if (typeInterop_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.typeInterop == null) {
VarHandle.storeStoreFence();
this.typeInterop = typeInterop_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[JavaBuiltins.JavaToNode.to(Object, Object, InteropLibrary)] */;
this.state_0_ = state_0;
return to(arguments0Value, arguments1Value, typeInterop_);
}
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[JavaBuiltins.JavaToNode.toNonObject(Object, Object, InteropLibrary, InteropLibrary)] */) {
while (true) {
int count1_ = 0;
ToNonObject0Data s1_ = TO_NON_OBJECT0_CACHE_UPDATER.getVolatile(this);
ToNonObject0Data s1_original = s1_;
while (s1_ != null) {
{
InteropLibrary typeInterop_1 = this.typeInterop;
if (typeInterop_1 != null) {
if ((s1_.objInterop_.accepts(arguments0Value)) && (!(JSGuards.isJSObject(arguments0Value)))) {
break;
}
}
}
count1_++;
s1_ = s1_.next_;
}
if (s1_ == null) {
if ((!(JSGuards.isJSObject(arguments0Value))) && count1_ < (JSConfig.InteropLibraryLimit)) {
// assert (s1_.objInterop_.accepts(arguments0Value));
s1_ = this.insert(new ToNonObject0Data(s1_original));
InteropLibrary objInterop__ = s1_.insert((INTEROP_LIBRARY_.create(arguments0Value)));
Objects.requireNonNull(objInterop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.objInterop_ = objInterop__;
InteropLibrary typeInterop_1;
InteropLibrary typeInterop_1_shared = this.typeInterop;
if (typeInterop_1_shared != null) {
typeInterop_1 = typeInterop_1_shared;
} else {
typeInterop_1 = s1_.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
if (typeInterop_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.typeInterop == null) {
this.typeInterop = typeInterop_1;
}
if (!TO_NON_OBJECT0_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[JavaBuiltins.JavaToNode.toNonObject(Object, Object, InteropLibrary, InteropLibrary)] */;
this.state_0_ = state_0;
}
}
if (s1_ != null) {
return toNonObject(arguments0Value, arguments1Value, s1_.objInterop_, this.typeInterop);
}
break;
}
}
{
InteropLibrary objInterop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
if ((!(JSGuards.isJSObject(arguments0Value)))) {
objInterop__ = (INTEROP_LIBRARY_.getUncached(arguments0Value));
InteropLibrary typeInterop_1;
InteropLibrary typeInterop_1_shared = this.typeInterop;
if (typeInterop_1_shared != null) {
typeInterop_1 = typeInterop_1_shared;
} else {
typeInterop_1 = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
if (typeInterop_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.typeInterop == null) {
VarHandle.storeStoreFence();
this.typeInterop = typeInterop_1;
}
this.toNonObject0_cache = null;
state_0 = state_0 & 0xfffffffd /* remove SpecializationActive[JavaBuiltins.JavaToNode.toNonObject(Object, Object, InteropLibrary, InteropLibrary)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[JavaBuiltins.JavaToNode.toNonObject(Object, Object, InteropLibrary, InteropLibrary)] */;
this.state_0_ = state_0;
return toNonObject(arguments0Value, arguments1Value, objInterop__, typeInterop_1);
}
} finally {
encapsulating_.set(prev_);
}
}
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_, this.arguments1_}, arguments0Value, arguments1Value);
}
@NeverDefault
public static JavaToNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaToNodeGen(context, builtin, arguments);
}
@GeneratedBy(JavaToNode.class)
@DenyReplace
private static final class ToNonObject0Data extends Node implements SpecializationDataNode {
@Child ToNonObject0Data next_;
/**
* Source Info:
* Specialization: {@link JavaToNode#toNonObject}
* Parameter: {@link InteropLibrary} objInterop
*/
@Child InteropLibrary objInterop_;
ToNonObject0Data(ToNonObject0Data next_) {
this.next_ = next_;
}
}
}
/**
* Debug Info:
* Specialization {@link JavaSuperNode#superAdapter}
* Activation probability: 1.00000
* With/without class size: 28/8 bytes
*
*/
@GeneratedBy(JavaSuperNode.class)
@SuppressWarnings("javadoc")
static final class JavaSuperNodeGen extends JavaSuperNode {
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link JavaSuperNode#superAdapter}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JavaSuperNode#superAdapter}
* Parameter: {@link InteropLibrary} interop
*/
@Child private InteropLibrary interop_;
/**
* Source Info:
* Specialization: {@link JavaSuperNode#superAdapter}
* Parameter: {@link ImportValueNode} toJSType
*/
@Child private ImportValueNode toJSType_;
private JavaSuperNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[JavaBuiltins.JavaSuperNode.superAdapter(Object, InteropLibrary, ImportValueNode)] */) {
{
InteropLibrary interop__ = this.interop_;
if (interop__ != null) {
ImportValueNode toJSType__ = this.toJSType_;
if (toJSType__ != null) {
return superAdapter(arguments0Value_, interop__, toJSType__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private Object executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
InteropLibrary interop__ = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
Objects.requireNonNull(interop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.interop_ = interop__;
ImportValueNode toJSType__ = this.insert((ImportValueNode.create()));
Objects.requireNonNull(toJSType__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toJSType_ = toJSType__;
state_0 = state_0 | 0b1 /* add SpecializationActive[JavaBuiltins.JavaSuperNode.superAdapter(Object, InteropLibrary, ImportValueNode)] */;
this.state_0_ = state_0;
return superAdapter(arguments0Value, interop__, toJSType__);
}
@NeverDefault
public static JavaSuperNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaSuperNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaIsTypeNode#isType}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(JavaIsTypeNode.class)
@SuppressWarnings("javadoc")
static final class JavaIsTypeNodeGen extends JavaIsTypeNode {
@Child private JavaScriptNode arguments0_;
private JavaIsTypeNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return isType(arguments0Value_);
}
@NeverDefault
public static JavaIsTypeNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaIsTypeNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaIsJavaObject#isJavaObject}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(JavaIsJavaObject.class)
@SuppressWarnings("javadoc")
static final class JavaIsJavaObjectNodeGen extends JavaIsJavaObject {
@Child private JavaScriptNode arguments0_;
private JavaIsJavaObjectNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return isJavaObject(arguments0Value_);
}
@NeverDefault
public static JavaIsJavaObject create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaIsJavaObjectNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaIsJavaMethodNode#isJavaMethod}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(JavaIsJavaMethodNode.class)
@SuppressWarnings("javadoc")
static final class JavaIsJavaMethodNodeGen extends JavaIsJavaMethodNode {
@Child private JavaScriptNode arguments0_;
private JavaIsJavaMethodNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return isJavaMethod(arguments0Value_);
}
@NeverDefault
public static JavaIsJavaMethodNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaIsJavaMethodNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaIsJavaFunctionNode#isJavaFunction}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(JavaIsJavaFunctionNode.class)
@SuppressWarnings("javadoc")
static final class JavaIsJavaFunctionNodeGen extends JavaIsJavaFunctionNode {
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link JavaIsJavaFunctionNode#isJavaFunction}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JavaIsJavaFunctionNode#isJavaFunction}
* Parameter: {@link InteropLibrary} interop
*/
@Child private InteropLibrary interop_;
private JavaIsJavaFunctionNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[JavaBuiltins.JavaIsJavaFunctionNode.isJavaFunction(Object, InteropLibrary)] */) {
{
InteropLibrary interop__ = this.interop_;
if (interop__ != null) {
return isJavaFunction(arguments0Value_, interop__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private boolean executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
InteropLibrary interop__ = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
Objects.requireNonNull(interop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.interop_ = interop__;
state_0 = state_0 | 0b1 /* add SpecializationActive[JavaBuiltins.JavaIsJavaFunctionNode.isJavaFunction(Object, InteropLibrary)] */;
this.state_0_ = state_0;
return isJavaFunction(arguments0Value, interop__);
}
@NeverDefault
public static JavaIsJavaFunctionNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaIsJavaFunctionNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaIsScriptFunctionNode#isScriptFunction}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(JavaIsScriptFunctionNode.class)
@SuppressWarnings("javadoc")
static final class JavaIsScriptFunctionNodeGen extends JavaIsScriptFunctionNode {
@Child private JavaScriptNode arguments0_;
private JavaIsScriptFunctionNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return JavaIsScriptFunctionNode.isScriptFunction(arguments0Value_);
}
@NeverDefault
public static JavaIsScriptFunctionNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaIsScriptFunctionNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaIsScriptObjectNode#isScriptObject}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(JavaIsScriptObjectNode.class)
@SuppressWarnings("javadoc")
static final class JavaIsScriptObjectNodeGen extends JavaIsScriptObjectNode {
@Child private JavaScriptNode arguments0_;
private JavaIsScriptObjectNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return JavaIsScriptObjectNode.isScriptObject(arguments0Value_);
}
@NeverDefault
public static JavaIsScriptObjectNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaIsScriptObjectNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaSynchronizedNode#doSynchronize}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(JavaSynchronizedNode.class)
@SuppressWarnings("javadoc")
static final class JavaSynchronizedNodeGen extends JavaSynchronizedNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
private JavaSynchronizedNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
return doSynchronize(arguments0Value_, arguments1Value_);
}
@NeverDefault
public static JavaSynchronizedNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaSynchronizedNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link JavaAddToClasspathNode#doString}
* Activation probability: 0.65000
* With/without class size: 11/0 bytes
* Specialization {@link JavaAddToClasspathNode#doObject}
* Activation probability: 0.35000
* With/without class size: 11/4 bytes
*
*/
@GeneratedBy(JavaAddToClasspathNode.class)
@SuppressWarnings("javadoc")
static final class JavaAddToClasspathNodeGen extends JavaAddToClasspathNode {
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link JavaAddToClasspathNode#doString}
* 1: SpecializationActive {@link JavaAddToClasspathNode#doObject}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JavaAddToClasspathNode#doObject}
* Parameter: {@link JSToStringNode} toStringNode
*/
@Child private JSToStringNode object_toStringNode_;
private JavaAddToClasspathNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[JavaBuiltins.JavaAddToClasspathNode.doString(TruffleString)] || SpecializationActive[JavaBuiltins.JavaAddToClasspathNode.doObject(Object, JSToStringNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JavaBuiltins.JavaAddToClasspathNode.doString(TruffleString)] */ && arguments0Value_ instanceof TruffleString) {
TruffleString arguments0Value__ = (TruffleString) arguments0Value_;
return doString(arguments0Value__);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JavaBuiltins.JavaAddToClasspathNode.doObject(Object, JSToStringNode)] */) {
{
JSToStringNode toStringNode__ = this.object_toStringNode_;
if (toStringNode__ != null) {
return doObject(arguments0Value_, toStringNode__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private Object executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JavaBuiltins.JavaAddToClasspathNode.doObject(Object, JSToStringNode)] */ && arguments0Value instanceof TruffleString) {
TruffleString arguments0Value_ = (TruffleString) arguments0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JavaBuiltins.JavaAddToClasspathNode.doString(TruffleString)] */;
this.state_0_ = state_0;
return doString(arguments0Value_);
}
JSToStringNode toStringNode__ = this.insert((JSToStringNode.create()));
Objects.requireNonNull(toStringNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.object_toStringNode_ = toStringNode__;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[JavaBuiltins.JavaAddToClasspathNode.doString(TruffleString)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[JavaBuiltins.JavaAddToClasspathNode.doObject(Object, JSToStringNode)] */;
this.state_0_ = state_0;
return doObject(arguments0Value, toStringNode__);
}
@NeverDefault
public static JavaAddToClasspathNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new JavaAddToClasspathNodeGen(context, builtin, arguments);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy