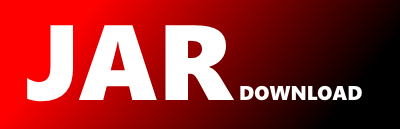
com.oracle.truffle.js.builtins.math.MaxNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.builtins.math;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.js.nodes.JavaScriptNode;
import com.oracle.truffle.js.nodes.cast.JSNumberToDoubleNode;
import com.oracle.truffle.js.nodes.cast.JSNumberToDoubleNodeGen;
import com.oracle.truffle.js.nodes.cast.JSToNumberNode;
import com.oracle.truffle.js.nodes.function.JSBuiltin;
import com.oracle.truffle.js.runtime.JSContext;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
/**
* Debug Info:
* Specialization {@link MinMaxNode#do1}
* Activation probability: 0.32000
* With/without class size: 7/0 bytes
* Specialization {@link MinMaxNode#do2}
* Activation probability: 0.26000
* With/without class size: 8/0 bytes
* Specialization {@link MinMaxNode#do3}
* Activation probability: 0.20000
* With/without class size: 7/0 bytes
* Specialization {@link MinMaxNode#do4OrMore}
* Activation probability: 0.14000
* With/without class size: 6/0 bytes
* Specialization {@link MaxNode#do0}
* Activation probability: 0.08000
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(MaxNode.class)
@SuppressWarnings("javadoc")
public final class MaxNodeGen extends MaxNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link MinMaxNode#do2}
* Parameter: {@link InlinedBranchProfile} isIntBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_IS_INT_BRANCH = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(5, 1)));
/**
* Source Info:
* Specialization: {@link MinMaxNode#do2}
* Parameter: {@link JSNumberToDoubleNode} numberToDouble
* Inline method: {@link JSNumberToDoubleNodeGen#inline}
*/
private static final JSNumberToDoubleNode INLINED_NUMBER_TO_DOUBLE = JSNumberToDoubleNodeGen.inline(InlineTarget.create(JSNumberToDoubleNode.class, STATE_0_UPDATER.subUpdater(6, 5)));
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link MinMaxNode#do1}
* 1: SpecializationActive {@link MinMaxNode#do2}
* 2: SpecializationActive {@link MinMaxNode#do3}
* 3: SpecializationActive {@link MinMaxNode#do4OrMore}
* 4: SpecializationActive {@link MaxNode#do0}
* 5: InlinedCache
* Specialization: {@link MinMaxNode#do2}
* Parameter: {@link InlinedBranchProfile} isIntBranch
* Inline method: {@link InlinedBranchProfile#inline}
* 6-10: InlinedCache
* Specialization: {@link MinMaxNode#do2}
* Parameter: {@link JSNumberToDoubleNode} numberToDouble
* Inline method: {@link JSNumberToDoubleNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link MinMaxNode#do1}
* Parameter: {@link JSToNumberNode} toNumber0Node
*/
@Child private JSToNumberNode toNumber0Node;
/**
* Source Info:
* Specialization: {@link MinMaxNode#do2}
* Parameter: {@link JSToNumberNode} toNumber1Node
*/
@Child private JSToNumberNode toNumber1Node;
/**
* Source Info:
* Specialization: {@link MinMaxNode#do3}
* Parameter: {@link JSToNumberNode} toNumber2Node
*/
@Child private JSToNumberNode toNumber2Node;
private MaxNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if ((state_0 & 0b11111) != 0 /* is SpecializationActive[MinMaxNode.do1(Object[], JSToNumberNode)] || SpecializationActive[MinMaxNode.do2(Object[], InlinedBranchProfile, JSNumberToDoubleNode, JSToNumberNode, JSToNumberNode)] || SpecializationActive[MinMaxNode.do3(Object[], InlinedBranchProfile, JSNumberToDoubleNode, JSToNumberNode, JSToNumberNode, JSToNumberNode)] || SpecializationActive[MinMaxNode.do4OrMore(Object[], InlinedBranchProfile, JSToNumberNode, JSToNumberNode, JSToNumberNode, JSNumberToDoubleNode)] || SpecializationActive[MaxNode.do0(Object[])] */ && arguments0Value_ instanceof Object[]) {
Object[] arguments0Value__ = (Object[]) arguments0Value_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[MinMaxNode.do1(Object[], JSToNumberNode)] */) {
{
JSToNumberNode toNumber0Node_ = this.toNumber0Node;
if (toNumber0Node_ != null) {
if ((arguments0Value__.length == 1)) {
return MinMaxNode.do1(arguments0Value__, toNumber0Node_);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[MinMaxNode.do2(Object[], InlinedBranchProfile, JSNumberToDoubleNode, JSToNumberNode, JSToNumberNode)] */) {
{
JSToNumberNode toNumber0Node_1 = this.toNumber0Node;
if (toNumber0Node_1 != null) {
JSToNumberNode toNumber1Node_ = this.toNumber1Node;
if (toNumber1Node_ != null) {
if ((arguments0Value__.length == 2)) {
return do2(arguments0Value__, INLINED_IS_INT_BRANCH, INLINED_NUMBER_TO_DOUBLE, toNumber0Node_1, toNumber1Node_);
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[MinMaxNode.do3(Object[], InlinedBranchProfile, JSNumberToDoubleNode, JSToNumberNode, JSToNumberNode, JSToNumberNode)] */) {
{
JSToNumberNode toNumber0Node_2 = this.toNumber0Node;
if (toNumber0Node_2 != null) {
JSToNumberNode toNumber1Node_1 = this.toNumber1Node;
if (toNumber1Node_1 != null) {
JSToNumberNode toNumber2Node_ = this.toNumber2Node;
if (toNumber2Node_ != null) {
if ((arguments0Value__.length == 3)) {
return do3(arguments0Value__, INLINED_IS_INT_BRANCH, INLINED_NUMBER_TO_DOUBLE, toNumber0Node_2, toNumber1Node_1, toNumber2Node_);
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[MinMaxNode.do4OrMore(Object[], InlinedBranchProfile, JSToNumberNode, JSToNumberNode, JSToNumberNode, JSNumberToDoubleNode)] */) {
{
JSToNumberNode toNumber0Node_3 = this.toNumber0Node;
if (toNumber0Node_3 != null) {
JSToNumberNode toNumber1Node_2 = this.toNumber1Node;
if (toNumber1Node_2 != null) {
JSToNumberNode toNumber2Node_1 = this.toNumber2Node;
if (toNumber2Node_1 != null) {
if ((arguments0Value__.length >= 4)) {
return do4OrMore(arguments0Value__, INLINED_IS_INT_BRANCH, toNumber0Node_3, toNumber1Node_2, toNumber2Node_1, INLINED_NUMBER_TO_DOUBLE);
}
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[MaxNode.do0(Object[])] */) {
if ((arguments0Value__.length == 0)) {
return MaxNode.do0(arguments0Value__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private Object executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
if (arguments0Value instanceof Object[]) {
Object[] arguments0Value_ = (Object[]) arguments0Value;
if ((arguments0Value_.length == 1)) {
JSToNumberNode toNumber0Node_;
JSToNumberNode toNumber0Node__shared = this.toNumber0Node;
if (toNumber0Node__shared != null) {
toNumber0Node_ = toNumber0Node__shared;
} else {
toNumber0Node_ = this.insert((JSToNumberNode.create()));
if (toNumber0Node_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumber0Node == null) {
VarHandle.storeStoreFence();
this.toNumber0Node = toNumber0Node_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[MinMaxNode.do1(Object[], JSToNumberNode)] */;
this.state_0_ = state_0;
return MinMaxNode.do1(arguments0Value_, toNumber0Node_);
}
if ((arguments0Value_.length == 2)) {
JSToNumberNode toNumber0Node_1;
JSToNumberNode toNumber0Node_1_shared = this.toNumber0Node;
if (toNumber0Node_1_shared != null) {
toNumber0Node_1 = toNumber0Node_1_shared;
} else {
toNumber0Node_1 = this.insert((JSToNumberNode.create()));
if (toNumber0Node_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumber0Node == null) {
VarHandle.storeStoreFence();
this.toNumber0Node = toNumber0Node_1;
}
JSToNumberNode toNumber1Node_;
JSToNumberNode toNumber1Node__shared = this.toNumber1Node;
if (toNumber1Node__shared != null) {
toNumber1Node_ = toNumber1Node__shared;
} else {
toNumber1Node_ = this.insert((JSToNumberNode.create()));
if (toNumber1Node_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumber1Node == null) {
VarHandle.storeStoreFence();
this.toNumber1Node = toNumber1Node_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[MinMaxNode.do2(Object[], InlinedBranchProfile, JSNumberToDoubleNode, JSToNumberNode, JSToNumberNode)] */;
this.state_0_ = state_0;
return do2(arguments0Value_, INLINED_IS_INT_BRANCH, INLINED_NUMBER_TO_DOUBLE, toNumber0Node_1, toNumber1Node_);
}
if ((arguments0Value_.length == 3)) {
JSToNumberNode toNumber0Node_2;
JSToNumberNode toNumber0Node_2_shared = this.toNumber0Node;
if (toNumber0Node_2_shared != null) {
toNumber0Node_2 = toNumber0Node_2_shared;
} else {
toNumber0Node_2 = this.insert((JSToNumberNode.create()));
if (toNumber0Node_2 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumber0Node == null) {
VarHandle.storeStoreFence();
this.toNumber0Node = toNumber0Node_2;
}
JSToNumberNode toNumber1Node_1;
JSToNumberNode toNumber1Node_1_shared = this.toNumber1Node;
if (toNumber1Node_1_shared != null) {
toNumber1Node_1 = toNumber1Node_1_shared;
} else {
toNumber1Node_1 = this.insert((JSToNumberNode.create()));
if (toNumber1Node_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumber1Node == null) {
VarHandle.storeStoreFence();
this.toNumber1Node = toNumber1Node_1;
}
JSToNumberNode toNumber2Node_;
JSToNumberNode toNumber2Node__shared = this.toNumber2Node;
if (toNumber2Node__shared != null) {
toNumber2Node_ = toNumber2Node__shared;
} else {
toNumber2Node_ = this.insert((JSToNumberNode.create()));
if (toNumber2Node_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumber2Node == null) {
VarHandle.storeStoreFence();
this.toNumber2Node = toNumber2Node_;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[MinMaxNode.do3(Object[], InlinedBranchProfile, JSNumberToDoubleNode, JSToNumberNode, JSToNumberNode, JSToNumberNode)] */;
this.state_0_ = state_0;
return do3(arguments0Value_, INLINED_IS_INT_BRANCH, INLINED_NUMBER_TO_DOUBLE, toNumber0Node_2, toNumber1Node_1, toNumber2Node_);
}
if ((arguments0Value_.length >= 4)) {
JSToNumberNode toNumber0Node_3;
JSToNumberNode toNumber0Node_3_shared = this.toNumber0Node;
if (toNumber0Node_3_shared != null) {
toNumber0Node_3 = toNumber0Node_3_shared;
} else {
toNumber0Node_3 = this.insert((JSToNumberNode.create()));
if (toNumber0Node_3 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumber0Node == null) {
VarHandle.storeStoreFence();
this.toNumber0Node = toNumber0Node_3;
}
JSToNumberNode toNumber1Node_2;
JSToNumberNode toNumber1Node_2_shared = this.toNumber1Node;
if (toNumber1Node_2_shared != null) {
toNumber1Node_2 = toNumber1Node_2_shared;
} else {
toNumber1Node_2 = this.insert((JSToNumberNode.create()));
if (toNumber1Node_2 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumber1Node == null) {
VarHandle.storeStoreFence();
this.toNumber1Node = toNumber1Node_2;
}
JSToNumberNode toNumber2Node_1;
JSToNumberNode toNumber2Node_1_shared = this.toNumber2Node;
if (toNumber2Node_1_shared != null) {
toNumber2Node_1 = toNumber2Node_1_shared;
} else {
toNumber2Node_1 = this.insert((JSToNumberNode.create()));
if (toNumber2Node_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumber2Node == null) {
VarHandle.storeStoreFence();
this.toNumber2Node = toNumber2Node_1;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[MinMaxNode.do4OrMore(Object[], InlinedBranchProfile, JSToNumberNode, JSToNumberNode, JSToNumberNode, JSNumberToDoubleNode)] */;
this.state_0_ = state_0;
return do4OrMore(arguments0Value_, INLINED_IS_INT_BRANCH, toNumber0Node_3, toNumber1Node_2, toNumber2Node_1, INLINED_NUMBER_TO_DOUBLE);
}
if ((arguments0Value_.length == 0)) {
state_0 = state_0 | 0b10000 /* add SpecializationActive[MaxNode.do0(Object[])] */;
this.state_0_ = state_0;
return MaxNode.do0(arguments0Value_);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_}, arguments0Value);
}
@NeverDefault
public static MaxNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new MaxNodeGen(context, builtin, arguments);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy