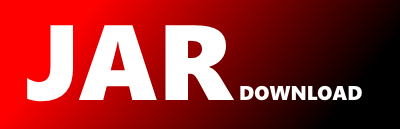
com.oracle.truffle.js.nodes.access.CachedGetPropertyNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.access;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString.EqualNode;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.access.FrequencyBasedPolymorphicAccessNode.FrequencyBasedPropertyGetNode;
import com.oracle.truffle.js.nodes.cast.ToArrayIndexNoToPropertyKeyNode;
import com.oracle.truffle.js.nodes.cast.ToArrayIndexNoToPropertyKeyNodeGen;
import com.oracle.truffle.js.nodes.cast.ToArrayIndexNode;
import com.oracle.truffle.js.runtime.JSContext;
import com.oracle.truffle.js.runtime.JSRuntime;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import com.oracle.truffle.js.runtime.util.JSClassProfile;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link CachedGetPropertyNode#doCachedKey}
* Activation probability: 0.32000
* With/without class size: 12/8 bytes
* Specialization {@link CachedGetPropertyNode#doIntIndex}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link CachedGetPropertyNode#doArrayIndex}
* Activation probability: 0.20000
* With/without class size: 9/11 bytes
* Specialization {@link CachedGetPropertyNode#doProxy}
* Activation probability: 0.14000
* With/without class size: 6/4 bytes
* Specialization {@link CachedGetPropertyNode#doGeneric}
* Activation probability: 0.08000
* With/without class size: 6/13 bytes
*
*/
@GeneratedBy(CachedGetPropertyNode.class)
@SuppressWarnings("javadoc")
final class CachedGetPropertyNodeGen extends CachedGetPropertyNode {
private static final StateField ARRAY_INDEX__CACHED_GET_PROPERTY_NODE_ARRAY_INDEX_STATE_0_UPDATER = StateField.create(ArrayIndexData.lookup_(), "arrayIndex_state_0_");
private static final StateField GENERIC__CACHED_GET_PROPERTY_NODE_GENERIC_STATE_0_UPDATER = StateField.create(GenericData.lookup_(), "generic_state_0_");
static final ReferenceField CACHED_KEY_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "cachedKey_cache", CachedKeyData.class);
static final ReferenceField ARRAY_INDEX_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "arrayIndex_cache", ArrayIndexData.class);
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doArrayIndex}
* Parameter: {@link ToArrayIndexNoToPropertyKeyNode} toArrayIndexNode
* Inline method: {@link ToArrayIndexNoToPropertyKeyNodeGen#inline}
*/
private static final ToArrayIndexNoToPropertyKeyNode INLINED_ARRAY_INDEX_TO_ARRAY_INDEX_NODE_ = ToArrayIndexNoToPropertyKeyNodeGen.inline(InlineTarget.create(ToArrayIndexNoToPropertyKeyNode.class, ARRAY_INDEX__CACHED_GET_PROPERTY_NODE_ARRAY_INDEX_STATE_0_UPDATER.subUpdater(0, 19), ReferenceField.create(ArrayIndexData.lookup_(), "arrayIndex_toArrayIndexNode__field1_", Node.class), ReferenceField.create(ArrayIndexData.lookup_(), "arrayIndex_toArrayIndexNode__field2_", Node.class)));
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doGeneric}
* Parameter: {@link InlinedConditionProfile} getType
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_GENERIC_GET_TYPE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, GENERIC__CACHED_GET_PROPERTY_NODE_GENERIC_STATE_0_UPDATER.subUpdater(0, 2)));
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doGeneric}
* Parameter: {@link InlinedConditionProfile} highFrequency
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_GENERIC_HIGH_FREQUENCY_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, GENERIC__CACHED_GET_PROPERTY_NODE_GENERIC_STATE_0_UPDATER.subUpdater(2, 2)));
/**
* State Info:
* 0: SpecializationActive {@link CachedGetPropertyNode#doCachedKey}
* 1: SpecializationActive {@link CachedGetPropertyNode#doGeneric}
* 2: SpecializationActive {@link CachedGetPropertyNode#doIntIndex}
* 3: SpecializationActive {@link CachedGetPropertyNode#doArrayIndex}
* 4: SpecializationActive {@link CachedGetPropertyNode#doProxy}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doCachedKey}
* Parameter: {@link EqualNode} equalsNode
*/
@Child private EqualNode equalsNode;
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doIntIndex}
* Parameter: {@link JSClassProfile} jsclassProfile
*/
@CompilationFinal private JSClassProfile jsclassProfile;
@UnsafeAccessedField @Child private CachedKeyData cachedKey_cache;
@UnsafeAccessedField @Child private ArrayIndexData arrayIndex_cache;
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doProxy}
* Parameter: {@link JSProxyPropertyGetNode} proxyGet
*/
@Child private JSProxyPropertyGetNode proxy_proxyGet_;
@Child private GenericData generic_cache;
private CachedGetPropertyNodeGen(JSContext context) {
super(context);
}
@ExplodeLoop
@Override
public Object execute(JSDynamicObject arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[CachedGetPropertyNode.doCachedKey(JSDynamicObject, Object, Object, Object, Object, PropertyGetNode, EqualNode)] || SpecializationActive[CachedGetPropertyNode.doIntIndex(JSDynamicObject, int, Object, Object, JSClassProfile)] || SpecializationActive[CachedGetPropertyNode.doArrayIndex(JSDynamicObject, Object, Object, Object, ToArrayIndexNoToPropertyKeyNode, long, JSClassProfile)] || SpecializationActive[CachedGetPropertyNode.doProxy(JSDynamicObject, Object, Object, Object, JSProxyPropertyGetNode)] || SpecializationActive[CachedGetPropertyNode.doGeneric(JSDynamicObject, Object, Object, Object, Node, RequireObjectCoercibleNode, ToArrayIndexNode, InlinedConditionProfile, JSClassProfile, InlinedConditionProfile, FrequencyBasedPropertyGetNode, EqualNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[CachedGetPropertyNode.doCachedKey(JSDynamicObject, Object, Object, Object, Object, PropertyGetNode, EqualNode)] */) {
CachedKeyData s0_ = this.cachedKey_cache;
while (s0_ != null) {
{
EqualNode equalsNode_ = this.equalsNode;
if (equalsNode_ != null) {
assert DSLSupport.assertIdempotence((s0_.cachedKey_ != null));
assert DSLSupport.assertIdempotence((!(JSRuntime.isArrayIndex(s0_.cachedKey_))));
if ((JSRuntime.propertyKeyEquals(equalsNode_, s0_.cachedKey_, arg1Value))) {
return doCachedKey(arg0Value, arg1Value, arg2Value, arg3Value, s0_.cachedKey_, s0_.propertyNode_, equalsNode_);
}
}
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[CachedGetPropertyNode.doIntIndex(JSDynamicObject, int, Object, Object, JSClassProfile)] */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
{
JSClassProfile jsclassProfile_ = this.jsclassProfile;
if (jsclassProfile_ != null) {
if ((JSRuntime.isArrayIndex(arg1Value_)) && (!(JSGuards.isJSProxy(arg0Value)))) {
return doIntIndex(arg0Value, arg1Value_, arg2Value, arg3Value, jsclassProfile_);
}
}
}
}
if ((state_0 & 0b11010) != 0 /* is SpecializationActive[CachedGetPropertyNode.doArrayIndex(JSDynamicObject, Object, Object, Object, ToArrayIndexNoToPropertyKeyNode, long, JSClassProfile)] || SpecializationActive[CachedGetPropertyNode.doProxy(JSDynamicObject, Object, Object, Object, JSProxyPropertyGetNode)] || SpecializationActive[CachedGetPropertyNode.doGeneric(JSDynamicObject, Object, Object, Object, Node, RequireObjectCoercibleNode, ToArrayIndexNode, InlinedConditionProfile, JSClassProfile, InlinedConditionProfile, FrequencyBasedPropertyGetNode, EqualNode)] */) {
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[CachedGetPropertyNode.doArrayIndex(JSDynamicObject, Object, Object, Object, ToArrayIndexNoToPropertyKeyNode, long, JSClassProfile)] */) {
ArrayIndexData s2_ = this.arrayIndex_cache;
if (s2_ != null) {
{
JSClassProfile jsclassProfile_1 = this.jsclassProfile;
if (jsclassProfile_1 != null) {
if ((!(JSGuards.isJSProxy(arg0Value)))) {
long index__ = (INLINED_ARRAY_INDEX_TO_ARRAY_INDEX_NODE_.executeLong(s2_, arg1Value));
if ((index__ >= 0)) {
return doArrayIndex(arg0Value, arg1Value, arg2Value, arg3Value, INLINED_ARRAY_INDEX_TO_ARRAY_INDEX_NODE_, index__, jsclassProfile_1);
}
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[CachedGetPropertyNode.doProxy(JSDynamicObject, Object, Object, Object, JSProxyPropertyGetNode)] */) {
{
JSProxyPropertyGetNode proxyGet__ = this.proxy_proxyGet_;
if (proxyGet__ != null) {
if ((JSGuards.isJSProxy(arg0Value))) {
return doProxy(arg0Value, arg1Value, arg2Value, arg3Value, proxyGet__);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[CachedGetPropertyNode.doGeneric(JSDynamicObject, Object, Object, Object, Node, RequireObjectCoercibleNode, ToArrayIndexNode, InlinedConditionProfile, JSClassProfile, InlinedConditionProfile, FrequencyBasedPropertyGetNode, EqualNode)] */) {
GenericData s4_ = this.generic_cache;
if (s4_ != null) {
{
JSClassProfile jsclassProfile_2 = this.jsclassProfile;
if (jsclassProfile_2 != null) {
EqualNode equalsNode_1 = this.equalsNode;
if (equalsNode_1 != null) {
Node node__ = (s4_);
return CachedGetPropertyNode.doGeneric(arg0Value, arg1Value, arg2Value, arg3Value, node__, s4_.requireObjectCoercibleNode_, s4_.toArrayIndexNode_, INLINED_GENERIC_GET_TYPE_, jsclassProfile_2, INLINED_GENERIC_HIGH_FREQUENCY_, s4_.hotKey_, equalsNode_1);
}
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private Object executeAndSpecialize(JSDynamicObject arg0Value, Object arg1Value, Object arg2Value, Object arg3Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[CachedGetPropertyNode.doGeneric(JSDynamicObject, Object, Object, Object, Node, RequireObjectCoercibleNode, ToArrayIndexNode, InlinedConditionProfile, JSClassProfile, InlinedConditionProfile, FrequencyBasedPropertyGetNode, EqualNode)] */) {
while (true) {
int count0_ = 0;
CachedKeyData s0_ = CACHED_KEY_CACHE_UPDATER.getVolatile(this);
CachedKeyData s0_original = s0_;
while (s0_ != null) {
{
EqualNode equalsNode_ = this.equalsNode;
if (equalsNode_ != null) {
assert DSLSupport.assertIdempotence((s0_.cachedKey_ != null));
assert DSLSupport.assertIdempotence((!(JSRuntime.isArrayIndex(s0_.cachedKey_))));
if ((JSRuntime.propertyKeyEquals(equalsNode_, s0_.cachedKey_, arg1Value))) {
break;
}
}
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
Object cachedKey__ = (CachedGetPropertyNode.propertyKeyOrNull(arg1Value));
if ((cachedKey__ != null) && (!(JSRuntime.isArrayIndex(cachedKey__)))) {
EqualNode equalsNode_;
EqualNode equalsNode__shared = this.equalsNode;
if (equalsNode__shared != null) {
equalsNode_ = equalsNode__shared;
} else {
equalsNode_ = this.insert((EqualNode.create()));
if (equalsNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((JSRuntime.propertyKeyEquals(equalsNode_, cachedKey__, arg1Value)) && count0_ < (CachedGetPropertyNode.MAX_DEPTH)) {
s0_ = this.insert(new CachedKeyData(s0_original));
s0_.cachedKey_ = cachedKey__;
PropertyGetNode propertyNode__ = s0_.insert((PropertyGetNode.create(cachedKey__, context)));
Objects.requireNonNull(propertyNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s0_.propertyNode_ = propertyNode__;
if (this.equalsNode == null) {
this.equalsNode = equalsNode_;
}
if (!CACHED_KEY_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[CachedGetPropertyNode.doCachedKey(JSDynamicObject, Object, Object, Object, Object, PropertyGetNode, EqualNode)] */;
this.state_0_ = state_0;
}
}
}
}
if (s0_ != null) {
return doCachedKey(arg0Value, arg1Value, arg2Value, arg3Value, s0_.cachedKey_, s0_.propertyNode_, this.equalsNode);
}
break;
}
}
if (((state_0 & 0b1010)) == 0 /* is-not SpecializationActive[CachedGetPropertyNode.doArrayIndex(JSDynamicObject, Object, Object, Object, ToArrayIndexNoToPropertyKeyNode, long, JSClassProfile)] && SpecializationActive[CachedGetPropertyNode.doGeneric(JSDynamicObject, Object, Object, Object, Node, RequireObjectCoercibleNode, ToArrayIndexNode, InlinedConditionProfile, JSClassProfile, InlinedConditionProfile, FrequencyBasedPropertyGetNode, EqualNode)] */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((JSRuntime.isArrayIndex(arg1Value_)) && (!(JSGuards.isJSProxy(arg0Value)))) {
JSClassProfile jsclassProfile_;
JSClassProfile jsclassProfile__shared = this.jsclassProfile;
if (jsclassProfile__shared != null) {
jsclassProfile_ = jsclassProfile__shared;
} else {
jsclassProfile_ = (JSClassProfile.create());
if (jsclassProfile_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.jsclassProfile == null) {
VarHandle.storeStoreFence();
this.jsclassProfile = jsclassProfile_;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[CachedGetPropertyNode.doIntIndex(JSDynamicObject, int, Object, Object, JSClassProfile)] */;
this.state_0_ = state_0;
return doIntIndex(arg0Value, arg1Value_, arg2Value, arg3Value, jsclassProfile_);
}
}
{
long index__ = 0L;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[CachedGetPropertyNode.doGeneric(JSDynamicObject, Object, Object, Object, Node, RequireObjectCoercibleNode, ToArrayIndexNode, InlinedConditionProfile, JSClassProfile, InlinedConditionProfile, FrequencyBasedPropertyGetNode, EqualNode)] */) {
while (true) {
int count2_ = 0;
ArrayIndexData s2_ = ARRAY_INDEX_CACHE_UPDATER.getVolatile(this);
ArrayIndexData s2_original = s2_;
while (s2_ != null) {
{
JSClassProfile jsclassProfile_1 = this.jsclassProfile;
if (jsclassProfile_1 != null) {
if ((!(JSGuards.isJSProxy(arg0Value)))) {
index__ = (INLINED_ARRAY_INDEX_TO_ARRAY_INDEX_NODE_.executeLong(s2_, arg1Value));
if ((index__ >= 0)) {
break;
}
}
}
}
count2_++;
s2_ = null;
break;
}
if (s2_ == null && count2_ < 1) {
if ((!(JSGuards.isJSProxy(arg0Value)))) {
s2_ = this.insert(new ArrayIndexData());
index__ = (INLINED_ARRAY_INDEX_TO_ARRAY_INDEX_NODE_.executeLong(s2_, arg1Value));
if ((index__ >= 0)) {
JSClassProfile jsclassProfile_1;
JSClassProfile jsclassProfile_1_shared = this.jsclassProfile;
if (jsclassProfile_1_shared != null) {
jsclassProfile_1 = jsclassProfile_1_shared;
} else {
jsclassProfile_1 = (JSClassProfile.create());
if (jsclassProfile_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.jsclassProfile == null) {
this.jsclassProfile = jsclassProfile_1;
}
if (!ARRAY_INDEX_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[CachedGetPropertyNode.doIntIndex(JSDynamicObject, int, Object, Object, JSClassProfile)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[CachedGetPropertyNode.doArrayIndex(JSDynamicObject, Object, Object, Object, ToArrayIndexNoToPropertyKeyNode, long, JSClassProfile)] */;
this.state_0_ = state_0;
} else {
s2_ = null;
}
} else {
s2_ = null;
}
}
if (s2_ != null) {
return doArrayIndex(arg0Value, arg1Value, arg2Value, arg3Value, INLINED_ARRAY_INDEX_TO_ARRAY_INDEX_NODE_, index__, this.jsclassProfile);
}
break;
}
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[CachedGetPropertyNode.doGeneric(JSDynamicObject, Object, Object, Object, Node, RequireObjectCoercibleNode, ToArrayIndexNode, InlinedConditionProfile, JSClassProfile, InlinedConditionProfile, FrequencyBasedPropertyGetNode, EqualNode)] */) {
if ((JSGuards.isJSProxy(arg0Value))) {
JSProxyPropertyGetNode proxyGet__ = this.insert((JSProxyPropertyGetNode.create(context)));
Objects.requireNonNull(proxyGet__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.proxy_proxyGet_ = proxyGet__;
state_0 = state_0 | 0b10000 /* add SpecializationActive[CachedGetPropertyNode.doProxy(JSDynamicObject, Object, Object, Object, JSProxyPropertyGetNode)] */;
this.state_0_ = state_0;
return doProxy(arg0Value, arg1Value, arg2Value, arg3Value, proxyGet__);
}
}
{
Node node__ = null;
GenericData s4_ = this.insert(new GenericData());
node__ = (s4_);
RequireObjectCoercibleNode requireObjectCoercibleNode__ = s4_.insert((RequireObjectCoercibleNode.create()));
Objects.requireNonNull(requireObjectCoercibleNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s4_.requireObjectCoercibleNode_ = requireObjectCoercibleNode__;
ToArrayIndexNode toArrayIndexNode__ = s4_.insert((ToArrayIndexNode.create()));
Objects.requireNonNull(toArrayIndexNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s4_.toArrayIndexNode_ = toArrayIndexNode__;
JSClassProfile jsclassProfile_2;
JSClassProfile jsclassProfile_2_shared = this.jsclassProfile;
if (jsclassProfile_2_shared != null) {
jsclassProfile_2 = jsclassProfile_2_shared;
} else {
jsclassProfile_2 = (JSClassProfile.create());
if (jsclassProfile_2 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.jsclassProfile == null) {
this.jsclassProfile = jsclassProfile_2;
}
FrequencyBasedPropertyGetNode hotKey__ = s4_.insert((FrequencyBasedPropertyGetNode.create(context)));
Objects.requireNonNull(hotKey__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s4_.hotKey_ = hotKey__;
EqualNode equalsNode_1;
EqualNode equalsNode_1_shared = this.equalsNode;
if (equalsNode_1_shared != null) {
equalsNode_1 = equalsNode_1_shared;
} else {
equalsNode_1 = s4_.insert((EqualNode.create()));
if (equalsNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.equalsNode == null) {
this.equalsNode = equalsNode_1;
}
VarHandle.storeStoreFence();
this.generic_cache = s4_;
this.cachedKey_cache = null;
this.arrayIndex_cache = null;
this.proxy_proxyGet_ = null;
state_0 = state_0 & 0xffffffe2 /* remove SpecializationActive[CachedGetPropertyNode.doCachedKey(JSDynamicObject, Object, Object, Object, Object, PropertyGetNode, EqualNode)], SpecializationActive[CachedGetPropertyNode.doIntIndex(JSDynamicObject, int, Object, Object, JSClassProfile)], SpecializationActive[CachedGetPropertyNode.doArrayIndex(JSDynamicObject, Object, Object, Object, ToArrayIndexNoToPropertyKeyNode, long, JSClassProfile)], SpecializationActive[CachedGetPropertyNode.doProxy(JSDynamicObject, Object, Object, Object, JSProxyPropertyGetNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[CachedGetPropertyNode.doGeneric(JSDynamicObject, Object, Object, Object, Node, RequireObjectCoercibleNode, ToArrayIndexNode, InlinedConditionProfile, JSClassProfile, InlinedConditionProfile, FrequencyBasedPropertyGetNode, EqualNode)] */;
this.state_0_ = state_0;
return CachedGetPropertyNode.doGeneric(arg0Value, arg1Value, arg2Value, arg3Value, node__, requireObjectCoercibleNode__, toArrayIndexNode__, INLINED_GENERIC_GET_TYPE_, jsclassProfile_2, INLINED_GENERIC_HIGH_FREQUENCY_, hotKey__, equalsNode_1);
}
}
@NeverDefault
public static CachedGetPropertyNode create(JSContext context) {
return new CachedGetPropertyNodeGen(context);
}
@GeneratedBy(CachedGetPropertyNode.class)
@DenyReplace
private static final class CachedKeyData extends Node implements SpecializationDataNode {
@Child CachedKeyData next_;
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doCachedKey}
* Parameter: {@link Object} cachedKey
*/
@CompilationFinal Object cachedKey_;
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doCachedKey}
* Parameter: {@link PropertyGetNode} propertyNode
*/
@Child PropertyGetNode propertyNode_;
CachedKeyData(CachedKeyData next_) {
this.next_ = next_;
}
}
@GeneratedBy(CachedGetPropertyNode.class)
@DenyReplace
private static final class ArrayIndexData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-18: InlinedCache
* Specialization: {@link CachedGetPropertyNode#doArrayIndex}
* Parameter: {@link ToArrayIndexNoToPropertyKeyNode} toArrayIndexNode
* Inline method: {@link ToArrayIndexNoToPropertyKeyNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int arrayIndex_state_0_;
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doArrayIndex}
* Parameter: {@link ToArrayIndexNoToPropertyKeyNode} toArrayIndexNode
* Inline method: {@link ToArrayIndexNoToPropertyKeyNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node arrayIndex_toArrayIndexNode__field1_;
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doArrayIndex}
* Parameter: {@link ToArrayIndexNoToPropertyKeyNode} toArrayIndexNode
* Inline method: {@link ToArrayIndexNoToPropertyKeyNodeGen#inline}
* Inline field: {@link Node} field2
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node arrayIndex_toArrayIndexNode__field2_;
ArrayIndexData() {
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(CachedGetPropertyNode.class)
@DenyReplace
private static final class GenericData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link CachedGetPropertyNode#doGeneric}
* Parameter: {@link InlinedConditionProfile} getType
* Inline method: {@link InlinedConditionProfile#inline}
* 2-3: InlinedCache
* Specialization: {@link CachedGetPropertyNode#doGeneric}
* Parameter: {@link InlinedConditionProfile} highFrequency
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int generic_state_0_;
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doGeneric}
* Parameter: {@link RequireObjectCoercibleNode} requireObjectCoercibleNode
*/
@Child RequireObjectCoercibleNode requireObjectCoercibleNode_;
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doGeneric}
* Parameter: {@link ToArrayIndexNode} toArrayIndexNode
*/
@Child ToArrayIndexNode toArrayIndexNode_;
/**
* Source Info:
* Specialization: {@link CachedGetPropertyNode#doGeneric}
* Parameter: {@link FrequencyBasedPropertyGetNode} hotKey
*/
@Child FrequencyBasedPropertyGetNode hotKey_;
GenericData() {
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy