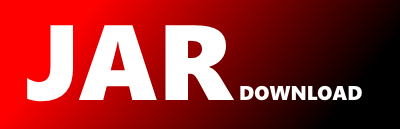
com.oracle.truffle.js.nodes.access.CopyDataPropertiesNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.access;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.strings.TruffleString.EqualNode;
import com.oracle.truffle.api.strings.TruffleString.SwitchEncodingNode;
import com.oracle.truffle.api.strings.TruffleString.ToJavaStringNode;
import com.oracle.truffle.js.builtins.helper.ListGetNode;
import com.oracle.truffle.js.builtins.helper.ListGetNodeGen;
import com.oracle.truffle.js.builtins.helper.ListSizeNode;
import com.oracle.truffle.js.builtins.helper.ListSizeNodeGen;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.binary.JSIdenticalNode;
import com.oracle.truffle.js.nodes.cast.JSToStringNode;
import com.oracle.truffle.js.nodes.interop.ImportValueNode;
import com.oracle.truffle.js.runtime.JSConfig;
import com.oracle.truffle.js.runtime.JSContext;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import com.oracle.truffle.js.runtime.objects.JSObject;
import com.oracle.truffle.js.runtime.util.JSClassProfile;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link CopyDataPropertiesNode#doNullOrUndefined}
* Activation probability: 0.38500
* With/without class size: 8/0 bytes
* Specialization {@link CopyDataPropertiesNode#copyDataProperties}
* Activation probability: 0.29500
* With/without class size: 14/20 bytes
* Specialization {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Activation probability: 0.20500
* With/without class size: 14/32 bytes
* Specialization {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Activation probability: 0.11500
* With/without class size: 9/28 bytes
*
*/
@GeneratedBy(CopyDataPropertiesNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class CopyDataPropertiesNodeGen extends CopyDataPropertiesNode {
static final ReferenceField COPY_DATA_PROPERTIES_FOREIGN0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "copyDataPropertiesForeign0_cache", CopyDataPropertiesForeign0Data.class);
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link CopyDataPropertiesNode#doNullOrUndefined}
* 1: SpecializationActive {@link CopyDataPropertiesNode#copyDataProperties}
* 2: SpecializationActive {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* 3: SpecializationActive {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataProperties}
* Parameter: {@link EqualNode} equalsNode
*/
@Child private EqualNode equalsNode;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataProperties}
* Parameter: {@link JSIdenticalNode} sameValueNode
*/
@Child private JSIdenticalNode sameValueNode;
@Child private CopyDataPropertiesData copyDataProperties_cache;
@UnsafeAccessedField @Child private CopyDataPropertiesForeign0Data copyDataPropertiesForeign0_cache;
@Child private CopyDataPropertiesForeign1Data copyDataPropertiesForeign1_cache;
private CopyDataPropertiesNodeGen(JSContext context) {
super(context);
}
@ExplodeLoop
@Override
protected Object executeImpl(Object arg0Value, Object arg1Value, Object[] arg2Value, boolean arg3Value, Object[] arg4Value, boolean arg5Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[CopyDataPropertiesNode.doNullOrUndefined(JSDynamicObject, Object, Object[], boolean, Object[], boolean)] || SpecializationActive[CopyDataPropertiesNode.copyDataProperties(JSDynamicObject, JSObject, Object[], boolean, Object[], boolean, ReadElementNode, JSGetOwnPropertyNode, ListSizeNode, ListGetNode, JSClassProfile, EqualNode, JSIdenticalNode)] || SpecializationActive[CopyDataPropertiesNode.copyDataPropertiesForeign(JSDynamicObject, Object, Object[], boolean, Object[], boolean, InteropLibrary, InteropLibrary, InteropLibrary, InteropLibrary, ImportValueNode, JSToStringNode, EqualNode, JSIdenticalNode, SwitchEncodingNode, ToJavaStringNode)] || SpecializationActive[CopyDataPropertiesNode.copyDataPropertiesForeign(JSDynamicObject, Object, Object[], boolean, Object[], boolean, InteropLibrary, InteropLibrary, InteropLibrary, InteropLibrary, ImportValueNode, JSToStringNode, EqualNode, JSIdenticalNode, SwitchEncodingNode, ToJavaStringNode)] */ && arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[CopyDataPropertiesNode.doNullOrUndefined(JSDynamicObject, Object, Object[], boolean, Object[], boolean)] */) {
if ((JSGuards.isNullOrUndefined(arg1Value))) {
return CopyDataPropertiesNode.doNullOrUndefined(arg0Value_, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[CopyDataPropertiesNode.copyDataProperties(JSDynamicObject, JSObject, Object[], boolean, Object[], boolean, ReadElementNode, JSGetOwnPropertyNode, ListSizeNode, ListGetNode, JSClassProfile, EqualNode, JSIdenticalNode)] */ && arg1Value instanceof JSObject) {
JSObject arg1Value_ = (JSObject) arg1Value;
CopyDataPropertiesData s1_ = this.copyDataProperties_cache;
if (s1_ != null) {
{
EqualNode equalsNode_ = this.equalsNode;
if (equalsNode_ != null) {
JSIdenticalNode sameValueNode_ = this.sameValueNode;
if (sameValueNode_ != null) {
return CopyDataPropertiesNode.copyDataProperties(arg0Value_, arg1Value_, arg2Value, arg3Value, arg4Value, arg5Value, s1_.getNode_, s1_.getOwnProperty_, s1_.listSize_, s1_.listGet_, s1_.classProfile_, equalsNode_, sameValueNode_);
}
}
}
}
}
if ((state_0 & 0b1100) != 0 /* is SpecializationActive[CopyDataPropertiesNode.copyDataPropertiesForeign(JSDynamicObject, Object, Object[], boolean, Object[], boolean, InteropLibrary, InteropLibrary, InteropLibrary, InteropLibrary, ImportValueNode, JSToStringNode, EqualNode, JSIdenticalNode, SwitchEncodingNode, ToJavaStringNode)] || SpecializationActive[CopyDataPropertiesNode.copyDataPropertiesForeign(JSDynamicObject, Object, Object[], boolean, Object[], boolean, InteropLibrary, InteropLibrary, InteropLibrary, InteropLibrary, ImportValueNode, JSToStringNode, EqualNode, JSIdenticalNode, SwitchEncodingNode, ToJavaStringNode)] */) {
if ((state_0 & 0b100) != 0 /* is SpecializationActive[CopyDataPropertiesNode.copyDataPropertiesForeign(JSDynamicObject, Object, Object[], boolean, Object[], boolean, InteropLibrary, InteropLibrary, InteropLibrary, InteropLibrary, ImportValueNode, JSToStringNode, EqualNode, JSIdenticalNode, SwitchEncodingNode, ToJavaStringNode)] */) {
CopyDataPropertiesForeign0Data s2_ = this.copyDataPropertiesForeign0_cache;
while (s2_ != null) {
{
EqualNode equalsNode_1 = this.equalsNode;
if (equalsNode_1 != null) {
JSIdenticalNode sameValueNode_1 = this.sameValueNode;
if (sameValueNode_1 != null) {
if ((s2_.objInterop_.accepts(arg1Value)) && (!(JSGuards.isJSDynamicObject(arg1Value)))) {
return copyDataPropertiesForeign(arg0Value_, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, s2_.objInterop_, s2_.iteratorInterop_, s2_.arrayInterop_, s2_.stringInterop_, s2_.importValue_, s2_.toString_, equalsNode_1, sameValueNode_1, s2_.switchEncodingNode_, s2_.toJavaStringNode_);
}
}
}
}
s2_ = s2_.next_;
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[CopyDataPropertiesNode.copyDataPropertiesForeign(JSDynamicObject, Object, Object[], boolean, Object[], boolean, InteropLibrary, InteropLibrary, InteropLibrary, InteropLibrary, ImportValueNode, JSToStringNode, EqualNode, JSIdenticalNode, SwitchEncodingNode, ToJavaStringNode)] */) {
CopyDataPropertiesForeign1Data s3_ = this.copyDataPropertiesForeign1_cache;
if (s3_ != null) {
{
EqualNode equalsNode_1 = this.equalsNode;
if (equalsNode_1 != null) {
JSIdenticalNode sameValueNode_1 = this.sameValueNode;
if (sameValueNode_1 != null) {
if ((!(JSGuards.isJSDynamicObject(arg1Value)))) {
return this.copyDataPropertiesForeign1Boundary(state_0, s3_, arg0Value_, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, equalsNode_1, sameValueNode_1);
}
}
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object copyDataPropertiesForeign1Boundary(int state_0, CopyDataPropertiesForeign1Data s3_, JSDynamicObject arg0Value_, Object arg1Value, Object[] arg2Value, boolean arg3Value, Object[] arg4Value, boolean arg5Value, EqualNode equalsNode_1, JSIdenticalNode sameValueNode_1) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary objInterop__ = (INTEROP_LIBRARY_.getUncached(arg1Value));
return copyDataPropertiesForeign(arg0Value_, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, objInterop__, s3_.iteratorInterop_, s3_.arrayInterop_, s3_.stringInterop_, s3_.importValue_, s3_.toString_, equalsNode_1, sameValueNode_1, s3_.switchEncodingNode_, s3_.toJavaStringNode_);
}
} finally {
encapsulating_.set(prev_);
}
}
private JSDynamicObject executeAndSpecialize(Object arg0Value, Object arg1Value, Object[] arg2Value, boolean arg3Value, Object[] arg4Value, boolean arg5Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if ((JSGuards.isNullOrUndefined(arg1Value))) {
state_0 = state_0 | 0b1 /* add SpecializationActive[CopyDataPropertiesNode.doNullOrUndefined(JSDynamicObject, Object, Object[], boolean, Object[], boolean)] */;
this.state_0_ = state_0;
return CopyDataPropertiesNode.doNullOrUndefined(arg0Value_, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if (arg1Value instanceof JSObject) {
JSObject arg1Value_ = (JSObject) arg1Value;
CopyDataPropertiesData s1_ = this.insert(new CopyDataPropertiesData());
ReadElementNode getNode__ = s1_.insert((ReadElementNode.create(context)));
Objects.requireNonNull(getNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.getNode_ = getNode__;
JSGetOwnPropertyNode getOwnProperty__ = s1_.insert((JSGetOwnPropertyNode.create(false)));
Objects.requireNonNull(getOwnProperty__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.getOwnProperty_ = getOwnProperty__;
ListSizeNode listSize__ = s1_.insert((ListSizeNodeGen.create()));
Objects.requireNonNull(listSize__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.listSize_ = listSize__;
ListGetNode listGet__ = s1_.insert((ListGetNodeGen.create()));
Objects.requireNonNull(listGet__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.listGet_ = listGet__;
JSClassProfile classProfile__ = (JSClassProfile.create());
Objects.requireNonNull(classProfile__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.classProfile_ = classProfile__;
EqualNode equalsNode_;
EqualNode equalsNode__shared = this.equalsNode;
if (equalsNode__shared != null) {
equalsNode_ = equalsNode__shared;
} else {
equalsNode_ = s1_.insert((EqualNode.create()));
if (equalsNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.equalsNode == null) {
this.equalsNode = equalsNode_;
}
JSIdenticalNode sameValueNode_;
JSIdenticalNode sameValueNode__shared = this.sameValueNode;
if (sameValueNode__shared != null) {
sameValueNode_ = sameValueNode__shared;
} else {
sameValueNode_ = s1_.insert((JSIdenticalNode.createSameValue()));
if (sameValueNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.sameValueNode == null) {
this.sameValueNode = sameValueNode_;
}
VarHandle.storeStoreFence();
this.copyDataProperties_cache = s1_;
state_0 = state_0 | 0b10 /* add SpecializationActive[CopyDataPropertiesNode.copyDataProperties(JSDynamicObject, JSObject, Object[], boolean, Object[], boolean, ReadElementNode, JSGetOwnPropertyNode, ListSizeNode, ListGetNode, JSClassProfile, EqualNode, JSIdenticalNode)] */;
this.state_0_ = state_0;
return CopyDataPropertiesNode.copyDataProperties(arg0Value_, arg1Value_, arg2Value, arg3Value, arg4Value, arg5Value, getNode__, getOwnProperty__, listSize__, listGet__, classProfile__, equalsNode_, sameValueNode_);
}
if (((state_0 & 0b1000)) == 0 /* is-not SpecializationActive[CopyDataPropertiesNode.copyDataPropertiesForeign(JSDynamicObject, Object, Object[], boolean, Object[], boolean, InteropLibrary, InteropLibrary, InteropLibrary, InteropLibrary, ImportValueNode, JSToStringNode, EqualNode, JSIdenticalNode, SwitchEncodingNode, ToJavaStringNode)] */) {
while (true) {
int count2_ = 0;
CopyDataPropertiesForeign0Data s2_ = COPY_DATA_PROPERTIES_FOREIGN0_CACHE_UPDATER.getVolatile(this);
CopyDataPropertiesForeign0Data s2_original = s2_;
while (s2_ != null) {
{
EqualNode equalsNode_1 = this.equalsNode;
if (equalsNode_1 != null) {
JSIdenticalNode sameValueNode_1 = this.sameValueNode;
if (sameValueNode_1 != null) {
if ((s2_.objInterop_.accepts(arg1Value)) && (!(JSGuards.isJSDynamicObject(arg1Value)))) {
break;
}
}
}
}
count2_++;
s2_ = s2_.next_;
}
if (s2_ == null) {
if ((!(JSGuards.isJSDynamicObject(arg1Value))) && count2_ < (JSConfig.InteropLibraryLimit)) {
// assert (s2_.objInterop_.accepts(arg1Value));
s2_ = this.insert(new CopyDataPropertiesForeign0Data(s2_original));
InteropLibrary objInterop__ = s2_.insert((INTEROP_LIBRARY_.create(arg1Value)));
Objects.requireNonNull(objInterop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s2_.objInterop_ = objInterop__;
InteropLibrary iteratorInterop__ = s2_.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
Objects.requireNonNull(iteratorInterop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s2_.iteratorInterop_ = iteratorInterop__;
InteropLibrary arrayInterop__ = s2_.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
Objects.requireNonNull(arrayInterop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s2_.arrayInterop_ = arrayInterop__;
InteropLibrary stringInterop__ = s2_.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
Objects.requireNonNull(stringInterop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s2_.stringInterop_ = stringInterop__;
ImportValueNode importValue__ = s2_.insert((ImportValueNode.create()));
Objects.requireNonNull(importValue__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s2_.importValue_ = importValue__;
JSToStringNode toString__ = s2_.insert((JSToStringNode.create()));
Objects.requireNonNull(toString__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s2_.toString_ = toString__;
EqualNode equalsNode_1;
EqualNode equalsNode_1_shared = this.equalsNode;
if (equalsNode_1_shared != null) {
equalsNode_1 = equalsNode_1_shared;
} else {
equalsNode_1 = s2_.insert((EqualNode.create()));
if (equalsNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.equalsNode == null) {
this.equalsNode = equalsNode_1;
}
JSIdenticalNode sameValueNode_1;
JSIdenticalNode sameValueNode_1_shared = this.sameValueNode;
if (sameValueNode_1_shared != null) {
sameValueNode_1 = sameValueNode_1_shared;
} else {
sameValueNode_1 = s2_.insert((JSIdenticalNode.createSameValue()));
if (sameValueNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.sameValueNode == null) {
this.sameValueNode = sameValueNode_1;
}
SwitchEncodingNode switchEncodingNode__ = s2_.insert((SwitchEncodingNode.create()));
Objects.requireNonNull(switchEncodingNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s2_.switchEncodingNode_ = switchEncodingNode__;
ToJavaStringNode toJavaStringNode__ = s2_.insert((ToJavaStringNode.create()));
Objects.requireNonNull(toJavaStringNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s2_.toJavaStringNode_ = toJavaStringNode__;
if (!COPY_DATA_PROPERTIES_FOREIGN0_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[CopyDataPropertiesNode.copyDataPropertiesForeign(JSDynamicObject, Object, Object[], boolean, Object[], boolean, InteropLibrary, InteropLibrary, InteropLibrary, InteropLibrary, ImportValueNode, JSToStringNode, EqualNode, JSIdenticalNode, SwitchEncodingNode, ToJavaStringNode)] */;
this.state_0_ = state_0;
}
}
if (s2_ != null) {
return copyDataPropertiesForeign(arg0Value_, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, s2_.objInterop_, s2_.iteratorInterop_, s2_.arrayInterop_, s2_.stringInterop_, s2_.importValue_, s2_.toString_, this.equalsNode, this.sameValueNode, s2_.switchEncodingNode_, s2_.toJavaStringNode_);
}
break;
}
}
{
InteropLibrary objInterop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
if ((!(JSGuards.isJSDynamicObject(arg1Value)))) {
CopyDataPropertiesForeign1Data s3_ = this.insert(new CopyDataPropertiesForeign1Data());
objInterop__ = (INTEROP_LIBRARY_.getUncached(arg1Value));
InteropLibrary iteratorInterop__ = s3_.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
Objects.requireNonNull(iteratorInterop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s3_.iteratorInterop_ = iteratorInterop__;
InteropLibrary arrayInterop__ = s3_.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
Objects.requireNonNull(arrayInterop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s3_.arrayInterop_ = arrayInterop__;
InteropLibrary stringInterop__ = s3_.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
Objects.requireNonNull(stringInterop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s3_.stringInterop_ = stringInterop__;
ImportValueNode importValue__ = s3_.insert((ImportValueNode.create()));
Objects.requireNonNull(importValue__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s3_.importValue_ = importValue__;
JSToStringNode toString__ = s3_.insert((JSToStringNode.create()));
Objects.requireNonNull(toString__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s3_.toString_ = toString__;
EqualNode equalsNode_1;
EqualNode equalsNode_1_shared = this.equalsNode;
if (equalsNode_1_shared != null) {
equalsNode_1 = equalsNode_1_shared;
} else {
equalsNode_1 = s3_.insert((EqualNode.create()));
if (equalsNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.equalsNode == null) {
this.equalsNode = equalsNode_1;
}
JSIdenticalNode sameValueNode_1;
JSIdenticalNode sameValueNode_1_shared = this.sameValueNode;
if (sameValueNode_1_shared != null) {
sameValueNode_1 = sameValueNode_1_shared;
} else {
sameValueNode_1 = s3_.insert((JSIdenticalNode.createSameValue()));
if (sameValueNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.sameValueNode == null) {
this.sameValueNode = sameValueNode_1;
}
SwitchEncodingNode switchEncodingNode__ = s3_.insert((SwitchEncodingNode.create()));
Objects.requireNonNull(switchEncodingNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s3_.switchEncodingNode_ = switchEncodingNode__;
ToJavaStringNode toJavaStringNode__ = s3_.insert((ToJavaStringNode.create()));
Objects.requireNonNull(toJavaStringNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s3_.toJavaStringNode_ = toJavaStringNode__;
VarHandle.storeStoreFence();
this.copyDataPropertiesForeign1_cache = s3_;
this.copyDataPropertiesForeign0_cache = null;
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[CopyDataPropertiesNode.copyDataPropertiesForeign(JSDynamicObject, Object, Object[], boolean, Object[], boolean, InteropLibrary, InteropLibrary, InteropLibrary, InteropLibrary, ImportValueNode, JSToStringNode, EqualNode, JSIdenticalNode, SwitchEncodingNode, ToJavaStringNode)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[CopyDataPropertiesNode.copyDataPropertiesForeign(JSDynamicObject, Object, Object[], boolean, Object[], boolean, InteropLibrary, InteropLibrary, InteropLibrary, InteropLibrary, ImportValueNode, JSToStringNode, EqualNode, JSIdenticalNode, SwitchEncodingNode, ToJavaStringNode)] */;
this.state_0_ = state_0;
return copyDataPropertiesForeign(arg0Value_, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, objInterop__, iteratorInterop__, arrayInterop__, stringInterop__, importValue__, toString__, equalsNode_1, sameValueNode_1, switchEncodingNode__, toJavaStringNode__);
}
} finally {
encapsulating_.set(prev_);
}
}
}
}
throw new UnsupportedSpecializationException(this, null, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@NeverDefault
public static CopyDataPropertiesNode create(JSContext context) {
return new CopyDataPropertiesNodeGen(context);
}
@GeneratedBy(CopyDataPropertiesNode.class)
@DenyReplace
private static final class CopyDataPropertiesData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataProperties}
* Parameter: {@link ReadElementNode} getNode
*/
@Child ReadElementNode getNode_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataProperties}
* Parameter: {@link JSGetOwnPropertyNode} getOwnProperty
*/
@Child JSGetOwnPropertyNode getOwnProperty_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataProperties}
* Parameter: {@link ListSizeNode} listSize
*/
@Child ListSizeNode listSize_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataProperties}
* Parameter: {@link ListGetNode} listGet
*/
@Child ListGetNode listGet_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataProperties}
* Parameter: {@link JSClassProfile} classProfile
*/
@CompilationFinal JSClassProfile classProfile_;
CopyDataPropertiesData() {
}
}
@GeneratedBy(CopyDataPropertiesNode.class)
@DenyReplace
private static final class CopyDataPropertiesForeign0Data extends Node implements SpecializationDataNode {
@Child CopyDataPropertiesForeign0Data next_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link InteropLibrary} objInterop
*/
@Child InteropLibrary objInterop_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link InteropLibrary} iteratorInterop
*/
@Child InteropLibrary iteratorInterop_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link InteropLibrary} arrayInterop
*/
@Child InteropLibrary arrayInterop_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link InteropLibrary} stringInterop
*/
@Child InteropLibrary stringInterop_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link ImportValueNode} importValue
*/
@Child ImportValueNode importValue_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link JSToStringNode} toString
*/
@Child JSToStringNode toString_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link SwitchEncodingNode} switchEncodingNode
*/
@Child SwitchEncodingNode switchEncodingNode_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link ToJavaStringNode} toJavaStringNode
*/
@Child ToJavaStringNode toJavaStringNode_;
CopyDataPropertiesForeign0Data(CopyDataPropertiesForeign0Data next_) {
this.next_ = next_;
}
}
@GeneratedBy(CopyDataPropertiesNode.class)
@DenyReplace
private static final class CopyDataPropertiesForeign1Data extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link InteropLibrary} iteratorInterop
*/
@Child InteropLibrary iteratorInterop_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link InteropLibrary} arrayInterop
*/
@Child InteropLibrary arrayInterop_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link InteropLibrary} stringInterop
*/
@Child InteropLibrary stringInterop_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link ImportValueNode} importValue
*/
@Child ImportValueNode importValue_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link JSToStringNode} toString
*/
@Child JSToStringNode toString_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link SwitchEncodingNode} switchEncodingNode
*/
@Child SwitchEncodingNode switchEncodingNode_;
/**
* Source Info:
* Specialization: {@link CopyDataPropertiesNode#copyDataPropertiesForeign}
* Parameter: {@link ToJavaStringNode} toJavaStringNode
*/
@Child ToJavaStringNode toJavaStringNode_;
CopyDataPropertiesForeign1Data() {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy