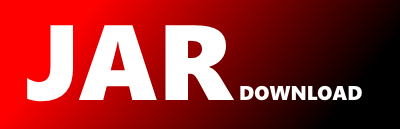
com.oracle.truffle.js.nodes.access.IsArrayNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.access;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.object.Shape;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.runtime.builtins.JSArgumentsObject;
import com.oracle.truffle.js.runtime.builtins.JSArrayObject;
import com.oracle.truffle.js.runtime.builtins.JSTypedArrayObject;
import java.lang.invoke.MethodHandles;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link IsArrayNode#doJSArray}
* Activation probability: 0.19111
* With/without class size: 6/0 bytes
* Specialization {@link IsArrayNode#doJSFastArrayShape}
* Activation probability: 0.17111
* With/without class size: 6/4 bytes
* Specialization {@link IsArrayNode#doJSFastArray}
* Activation probability: 0.15111
* With/without class size: 5/0 bytes
* Specialization {@link IsArrayNode#doJSTypedArray}
* Activation probability: 0.13111
* With/without class size: 5/0 bytes
* Specialization {@link IsArrayNode#doJSArgumentsObject}
* Activation probability: 0.11111
* With/without class size: 5/0 bytes
* Specialization {@link IsArrayNode#doJSObjectPrototype}
* Activation probability: 0.09111
* With/without class size: 5/0 bytes
* Specialization {@link IsArrayNode#doNotJSArray}
* Activation probability: 0.07111
* With/without class size: 4/0 bytes
* Specialization {@link IsArrayNode#doOtherCached}
* Activation probability: 0.05111
* With/without class size: 4/4 bytes
* Specialization {@link IsArrayNode#doOther}
* Activation probability: 0.03111
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(IsArrayNode.class)
@SuppressWarnings("javadoc")
public final class IsArrayNodeGen extends IsArrayNode {
static final ReferenceField J_S_FAST_ARRAY_SHAPE_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "jSFastArrayShape_cache", JSFastArrayShapeData.class);
static final ReferenceField OTHER_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "otherCached_cache", OtherCachedData.class);
/**
* State Info:
* 0: SpecializationActive {@link IsArrayNode#doJSArray}
* 1: SpecializationActive {@link IsArrayNode#doJSFastArrayShape}
* 2: SpecializationActive {@link IsArrayNode#doJSFastArray}
* 3: SpecializationActive {@link IsArrayNode#doJSTypedArray}
* 4: SpecializationActive {@link IsArrayNode#doJSArgumentsObject}
* 5: SpecializationActive {@link IsArrayNode#doJSObjectPrototype}
* 6: SpecializationActive {@link IsArrayNode#doNotJSArray}
* 7: SpecializationActive {@link IsArrayNode#doOtherCached}
* 8: SpecializationActive {@link IsArrayNode#doOther}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @CompilationFinal private JSFastArrayShapeData jSFastArrayShape_cache;
@UnsafeAccessedField @CompilationFinal private OtherCachedData otherCached_cache;
private IsArrayNodeGen(Kind kind) {
super(kind);
}
@Override
public boolean execute(Object arg0Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[IsArrayNode.doJSArray(JSArrayObject)] || SpecializationActive[IsArrayNode.doJSFastArrayShape(JSArrayObject, Shape)] || SpecializationActive[IsArrayNode.doJSFastArray(JSArrayObject)] || SpecializationActive[IsArrayNode.doJSTypedArray(JSTypedArrayObject)] || SpecializationActive[IsArrayNode.doJSArgumentsObject(JSArgumentsObject)] || SpecializationActive[IsArrayNode.doJSObjectPrototype(Object)] || SpecializationActive[IsArrayNode.doNotJSArray(Object)] || SpecializationActive[IsArrayNode.doOtherCached(Object, Class<>)] || SpecializationActive[IsArrayNode.doOther(Object)] */) {
if ((state_0 & 0b111) != 0 /* is SpecializationActive[IsArrayNode.doJSArray(JSArrayObject)] || SpecializationActive[IsArrayNode.doJSFastArrayShape(JSArrayObject, Shape)] || SpecializationActive[IsArrayNode.doJSFastArray(JSArrayObject)] */ && arg0Value instanceof JSArrayObject) {
JSArrayObject arg0Value_ = (JSArrayObject) arg0Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[IsArrayNode.doJSArray(JSArrayObject)] */) {
assert DSLSupport.assertIdempotence((kind == Kind.Array || kind == Kind.AnyArray));
return doJSArray(arg0Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[IsArrayNode.doJSFastArrayShape(JSArrayObject, Shape)] */) {
JSFastArrayShapeData s1_ = this.jSFastArrayShape_cache;
if (s1_ != null) {
assert DSLSupport.assertIdempotence((kind == Kind.FastArray || kind == Kind.FastOrTypedArray));
if ((arg0Value_.getShape() == s1_.cachedShape_)) {
return doJSFastArrayShape(arg0Value_, s1_.cachedShape_);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[IsArrayNode.doJSFastArray(JSArrayObject)] */) {
assert DSLSupport.assertIdempotence((kind == Kind.FastArray || kind == Kind.FastOrTypedArray));
return doJSFastArray(arg0Value_);
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[IsArrayNode.doJSTypedArray(JSTypedArrayObject)] */ && arg0Value instanceof JSTypedArrayObject) {
JSTypedArrayObject arg0Value_ = (JSTypedArrayObject) arg0Value;
assert DSLSupport.assertIdempotence((kind == Kind.AnyArray || kind == Kind.FastOrTypedArray));
return doJSTypedArray(arg0Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[IsArrayNode.doJSArgumentsObject(JSArgumentsObject)] */ && arg0Value instanceof JSArgumentsObject) {
JSArgumentsObject arg0Value_ = (JSArgumentsObject) arg0Value;
assert DSLSupport.assertIdempotence((kind == Kind.AnyArray || kind == Kind.FastOrTypedArray));
if ((JSGuards.isJSArgumentsObject(arg0Value_))) {
return doJSArgumentsObject(arg0Value_);
}
}
if ((state_0 & 0b111100000) != 0 /* is SpecializationActive[IsArrayNode.doJSObjectPrototype(Object)] || SpecializationActive[IsArrayNode.doNotJSArray(Object)] || SpecializationActive[IsArrayNode.doOtherCached(Object, Class<>)] || SpecializationActive[IsArrayNode.doOther(Object)] */) {
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[IsArrayNode.doJSObjectPrototype(Object)] */) {
assert DSLSupport.assertIdempotence((kind == Kind.AnyArray));
if ((JSGuards.isJSObjectPrototype(arg0Value))) {
return doJSObjectPrototype(arg0Value);
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[IsArrayNode.doNotJSArray(Object)] */) {
assert DSLSupport.assertIdempotence((kind == Kind.Array || kind == Kind.FastArray));
if ((!(JSGuards.isJSArray(arg0Value)))) {
return doNotJSArray(arg0Value);
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[IsArrayNode.doOtherCached(Object, Class<>)] */) {
OtherCachedData s7_ = this.otherCached_cache;
if (s7_ != null) {
if ((CompilerDirectives.isExact(arg0Value, s7_.cachedClass_))) {
return doOtherCached(arg0Value, s7_.cachedClass_);
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[IsArrayNode.doOther(Object)] */) {
return doOther(arg0Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
@SuppressWarnings("unused")
private boolean executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof JSArrayObject) {
JSArrayObject arg0Value_ = (JSArrayObject) arg0Value;
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[IsArrayNode.doOther(Object)] */) {
if ((kind == Kind.Array || kind == Kind.AnyArray)) {
state_0 = state_0 | 0b1 /* add SpecializationActive[IsArrayNode.doJSArray(JSArrayObject)] */;
this.state_0_ = state_0;
return doJSArray(arg0Value_);
}
}
if (((state_0 & 0b100000100)) == 0 /* is-not SpecializationActive[IsArrayNode.doJSFastArray(JSArrayObject)] && SpecializationActive[IsArrayNode.doOther(Object)] */) {
while (true) {
int count1_ = 0;
JSFastArrayShapeData s1_ = J_S_FAST_ARRAY_SHAPE_CACHE_UPDATER.getVolatile(this);
JSFastArrayShapeData s1_original = s1_;
while (s1_ != null) {
assert DSLSupport.assertIdempotence((kind == Kind.FastArray || kind == Kind.FastOrTypedArray));
if ((arg0Value_.getShape() == s1_.cachedShape_)) {
break;
}
count1_++;
s1_ = null;
break;
}
if (s1_ == null && count1_ < 1) {
if ((kind == Kind.FastArray || kind == Kind.FastOrTypedArray)) {
Shape cachedShape__ = (getInitialArrayShape());
if ((arg0Value_.getShape() == cachedShape__)) {
s1_ = new JSFastArrayShapeData();
Objects.requireNonNull(cachedShape__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.cachedShape_ = cachedShape__;
if (!J_S_FAST_ARRAY_SHAPE_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[IsArrayNode.doJSFastArrayShape(JSArrayObject, Shape)] */;
this.state_0_ = state_0;
}
}
}
if (s1_ != null) {
return doJSFastArrayShape(arg0Value_, s1_.cachedShape_);
}
break;
}
}
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[IsArrayNode.doOther(Object)] */) {
if ((kind == Kind.FastArray || kind == Kind.FastOrTypedArray)) {
this.jSFastArrayShape_cache = null;
state_0 = state_0 & 0xfffffffd /* remove SpecializationActive[IsArrayNode.doJSFastArrayShape(JSArrayObject, Shape)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[IsArrayNode.doJSFastArray(JSArrayObject)] */;
this.state_0_ = state_0;
return doJSFastArray(arg0Value_);
}
}
}
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[IsArrayNode.doOther(Object)] */ && arg0Value instanceof JSTypedArrayObject) {
JSTypedArrayObject arg0Value_ = (JSTypedArrayObject) arg0Value;
if ((kind == Kind.AnyArray || kind == Kind.FastOrTypedArray)) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[IsArrayNode.doJSTypedArray(JSTypedArrayObject)] */;
this.state_0_ = state_0;
return doJSTypedArray(arg0Value_);
}
}
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[IsArrayNode.doOther(Object)] */ && arg0Value instanceof JSArgumentsObject) {
JSArgumentsObject arg0Value_ = (JSArgumentsObject) arg0Value;
if ((kind == Kind.AnyArray || kind == Kind.FastOrTypedArray) && (JSGuards.isJSArgumentsObject(arg0Value_))) {
state_0 = state_0 | 0b10000 /* add SpecializationActive[IsArrayNode.doJSArgumentsObject(JSArgumentsObject)] */;
this.state_0_ = state_0;
return doJSArgumentsObject(arg0Value_);
}
}
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[IsArrayNode.doOther(Object)] */) {
if ((kind == Kind.AnyArray) && (JSGuards.isJSObjectPrototype(arg0Value))) {
state_0 = state_0 | 0b100000 /* add SpecializationActive[IsArrayNode.doJSObjectPrototype(Object)] */;
this.state_0_ = state_0;
return doJSObjectPrototype(arg0Value);
}
}
if ((kind == Kind.Array || kind == Kind.FastArray) && (!(JSGuards.isJSArray(arg0Value)))) {
state_0 = state_0 | 0b1000000 /* add SpecializationActive[IsArrayNode.doNotJSArray(Object)] */;
this.state_0_ = state_0;
return doNotJSArray(arg0Value);
}
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[IsArrayNode.doOther(Object)] */) {
while (true) {
int count7_ = 0;
OtherCachedData s7_ = OTHER_CACHED_CACHE_UPDATER.getVolatile(this);
OtherCachedData s7_original = s7_;
while (s7_ != null) {
if ((CompilerDirectives.isExact(arg0Value, s7_.cachedClass_))) {
break;
}
count7_++;
s7_ = null;
break;
}
if (s7_ == null && count7_ < 1) {
{
Class> cachedClass__ = (arg0Value.getClass());
if ((CompilerDirectives.isExact(arg0Value, cachedClass__))) {
s7_ = new OtherCachedData();
s7_.cachedClass_ = cachedClass__;
if (!OTHER_CACHED_CACHE_UPDATER.compareAndSet(this, s7_original, s7_)) {
continue;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[IsArrayNode.doOtherCached(Object, Class<>)] */;
this.state_0_ = state_0;
}
}
}
if (s7_ != null) {
return doOtherCached(arg0Value, s7_.cachedClass_);
}
break;
}
}
this.jSFastArrayShape_cache = null;
this.otherCached_cache = null;
state_0 = state_0 & 0xffffff40 /* remove SpecializationActive[IsArrayNode.doJSArray(JSArrayObject)], SpecializationActive[IsArrayNode.doJSFastArrayShape(JSArrayObject, Shape)], SpecializationActive[IsArrayNode.doJSFastArray(JSArrayObject)], SpecializationActive[IsArrayNode.doJSTypedArray(JSTypedArrayObject)], SpecializationActive[IsArrayNode.doJSArgumentsObject(JSArgumentsObject)], SpecializationActive[IsArrayNode.doJSObjectPrototype(Object)], SpecializationActive[IsArrayNode.doOtherCached(Object, Class<>)] */;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[IsArrayNode.doOther(Object)] */;
this.state_0_ = state_0;
return doOther(arg0Value);
}
@NeverDefault
public static IsArrayNode create(Kind kind) {
return new IsArrayNodeGen(kind);
}
@GeneratedBy(IsArrayNode.class)
@DenyReplace
private static final class JSFastArrayShapeData implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link IsArrayNode#doJSFastArrayShape}
* Parameter: {@link Shape} cachedShape
*/
@CompilationFinal Shape cachedShape_;
JSFastArrayShapeData() {
}
}
@GeneratedBy(IsArrayNode.class)
@DenyReplace
private static final class OtherCachedData implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link IsArrayNode#doOtherCached}
* Parameter: {@link Class} cachedClass
*/
@CompilationFinal Class> cachedClass_;
OtherCachedData() {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy