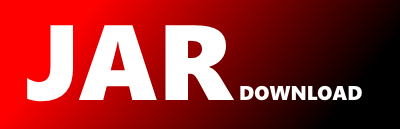
com.oracle.truffle.js.nodes.access.IsJSObjectNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.access;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.js.nodes.JSGuards;
import java.lang.invoke.MethodHandles;
/**
* Debug Info:
* Specialization {@link IsJSObjectNode#isObjectCached}
* Activation probability: 0.65000
* With/without class size: 15/5 bytes
* Specialization {@link IsJSObjectNode#isObject}
* Activation probability: 0.35000
* With/without class size: 11/1 bytes
*
*/
@GeneratedBy(IsJSObjectNode.class)
@SuppressWarnings("javadoc")
public final class IsJSObjectNodeGen extends IsJSObjectNode {
private static final StateField STATE_0_IsJSObjectNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
static final ReferenceField IS_OBJECT_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "isObjectCached_cache", IsObjectCachedData.class);
/**
* Source Info:
* Specialization: {@link IsJSObjectNode#isObject}
* Parameter: {@link InlinedConditionProfile} resultProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_IS_OBJECT_RESULT_PROFILE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_IsJSObjectNode_UPDATER.subUpdater(2, 2)));
/**
* State Info:
* 0: SpecializationActive {@link IsJSObjectNode#isObjectCached}
* 1: SpecializationActive {@link IsJSObjectNode#isObject}
* 2-3: InlinedCache
* Specialization: {@link IsJSObjectNode#isObject}
* Parameter: {@link InlinedConditionProfile} resultProfile
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
@UnsafeAccessedField @CompilationFinal private IsObjectCachedData isObjectCached_cache;
private IsJSObjectNodeGen() {
}
@Override
public boolean executeBoolean(Object arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11) != 0 /* is SpecializationActive[IsJSObjectNode.isObjectCached(Object, Class<>, boolean)] || SpecializationActive[IsJSObjectNode.isObject(Object, InlinedConditionProfile)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[IsJSObjectNode.isObjectCached(Object, Class<>, boolean)] */) {
IsObjectCachedData s0_ = this.isObjectCached_cache;
if (s0_ != null) {
assert DSLSupport.assertIdempotence((s0_.cachedClass_ != null));
if ((CompilerDirectives.isExact(arg0Value, s0_.cachedClass_))) {
return IsJSObjectNode.isObjectCached(arg0Value, s0_.cachedClass_, s0_.cachedResult_);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[IsJSObjectNode.isObject(Object, InlinedConditionProfile)] */) {
return isObject(arg0Value, INLINED_IS_OBJECT_RESULT_PROFILE_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private boolean executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[IsJSObjectNode.isObject(Object, InlinedConditionProfile)] */) {
while (true) {
int count0_ = 0;
IsObjectCachedData s0_ = IS_OBJECT_CACHED_CACHE_UPDATER.getVolatile(this);
IsObjectCachedData s0_original = s0_;
while (s0_ != null) {
assert DSLSupport.assertIdempotence((s0_.cachedClass_ != null));
if ((CompilerDirectives.isExact(arg0Value, s0_.cachedClass_))) {
break;
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
Class> cachedClass__ = (JSGuards.getClassIfJSDynamicObject(arg0Value));
if ((cachedClass__ != null) && (CompilerDirectives.isExact(arg0Value, cachedClass__))) {
s0_ = new IsObjectCachedData();
s0_.cachedClass_ = cachedClass__;
s0_.cachedResult_ = (JSGuards.isJSObject(arg0Value));
if (!IS_OBJECT_CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[IsJSObjectNode.isObjectCached(Object, Class<>, boolean)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return IsJSObjectNode.isObjectCached(arg0Value, s0_.cachedClass_, s0_.cachedResult_);
}
break;
}
}
this.isObjectCached_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[IsJSObjectNode.isObjectCached(Object, Class<>, boolean)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[IsJSObjectNode.isObject(Object, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return isObject(arg0Value, INLINED_IS_OBJECT_RESULT_PROFILE_);
}
@NeverDefault
public static IsJSObjectNode create() {
return new IsJSObjectNodeGen();
}
@GeneratedBy(IsJSObjectNode.class)
@DenyReplace
private static final class IsObjectCachedData implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link IsJSObjectNode#isObjectCached}
* Parameter: {@link Class} cachedClass
*/
@CompilationFinal Class> cachedClass_;
/**
* Source Info:
* Specialization: {@link IsJSObjectNode#isObjectCached}
* Parameter: boolean cachedResult
*/
@CompilationFinal boolean cachedResult_;
IsObjectCachedData() {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy