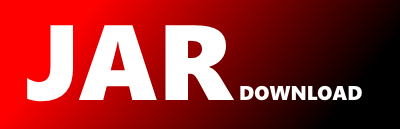
com.oracle.truffle.js.nodes.access.IsRegExpNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.access;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.js.nodes.cast.JSToBooleanNode;
import com.oracle.truffle.js.nodes.cast.JSToBooleanNodeGen;
import com.oracle.truffle.js.runtime.JSContext;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link IsRegExpNode#doIsObject}
* Activation probability: 0.65000
* With/without class size: 24/15 bytes
* Specialization {@link IsRegExpNode#doNonObject}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(IsRegExpNode.class)
@SuppressWarnings("javadoc")
public final class IsRegExpNodeGen extends IsRegExpNode {
private static final StateField STATE_0_IsRegExpNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link IsRegExpNode#doIsObject}
* Parameter: {@link JSToBooleanNode} toBooleanNode
* Inline method: {@link JSToBooleanNodeGen#inline}
*/
private static final JSToBooleanNode INLINED_IS_OBJECT_TO_BOOLEAN_NODE_ = JSToBooleanNodeGen.inline(InlineTarget.create(JSToBooleanNode.class, STATE_0_IsRegExpNode_UPDATER.subUpdater(2, 15), ReferenceField.create(MethodHandles.lookup(), "isObject_toBooleanNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link IsRegExpNode#doIsObject}
* Parameter: {@link InlinedConditionProfile} hasMatchSymbol
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_IS_OBJECT_HAS_MATCH_SYMBOL_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_IsRegExpNode_UPDATER.subUpdater(17, 2)));
/**
* State Info:
* 0: SpecializationActive {@link IsRegExpNode#doIsObject}
* 1: SpecializationActive {@link IsRegExpNode#doNonObject}
* 2-16: InlinedCache
* Specialization: {@link IsRegExpNode#doIsObject}
* Parameter: {@link JSToBooleanNode} toBooleanNode
* Inline method: {@link JSToBooleanNodeGen#inline}
* 17-18: InlinedCache
* Specialization: {@link IsRegExpNode#doIsObject}
* Parameter: {@link InlinedConditionProfile} hasMatchSymbol
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link IsRegExpNode#doIsObject}
* Parameter: {@link IsJSObjectNode} isObjectNode
*/
@Child private IsJSObjectNode isObject_isObjectNode_;
/**
* Source Info:
* Specialization: {@link IsRegExpNode#doIsObject}
* Parameter: {@link JSToBooleanNode} toBooleanNode
* Inline method: {@link JSToBooleanNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node isObject_toBooleanNode__field1_;
/**
* Source Info:
* Specialization: {@link IsRegExpNode#doIsObject}
* Parameter: {@link IsJSClassNode} isJSRegExpNode
*/
@Child private IsJSClassNode isObject_isJSRegExpNode_;
private IsRegExpNodeGen(JSContext context) {
super(context);
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Object arg0Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[IsRegExpNode.doIsObject(JSDynamicObject, IsJSObjectNode, JSToBooleanNode, IsJSClassNode, InlinedConditionProfile)] */) && arg0Value instanceof JSDynamicObject) {
return false;
}
return true;
}
@Override
public boolean executeBoolean(Object arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11) != 0 /* is SpecializationActive[IsRegExpNode.doIsObject(JSDynamicObject, IsJSObjectNode, JSToBooleanNode, IsJSClassNode, InlinedConditionProfile)] || SpecializationActive[IsRegExpNode.doNonObject(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[IsRegExpNode.doIsObject(JSDynamicObject, IsJSObjectNode, JSToBooleanNode, IsJSClassNode, InlinedConditionProfile)] */ && arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
{
IsJSObjectNode isObjectNode__ = this.isObject_isObjectNode_;
if (isObjectNode__ != null) {
IsJSClassNode isJSRegExpNode__ = this.isObject_isJSRegExpNode_;
if (isJSRegExpNode__ != null) {
return doIsObject(arg0Value_, isObjectNode__, INLINED_IS_OBJECT_TO_BOOLEAN_NODE_, isJSRegExpNode__, INLINED_IS_OBJECT_HAS_MATCH_SYMBOL_);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[IsRegExpNode.doNonObject(Object)] */) {
if (fallbackGuard_(state_0, arg0Value)) {
return doNonObject(arg0Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private boolean executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
IsJSObjectNode isObjectNode__ = this.insert((IsJSObjectNode.create()));
Objects.requireNonNull(isObjectNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.isObject_isObjectNode_ = isObjectNode__;
IsJSClassNode isJSRegExpNode__ = this.insert((IsRegExpNode.createIsJSRegExpNode()));
Objects.requireNonNull(isJSRegExpNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.isObject_isJSRegExpNode_ = isJSRegExpNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[IsRegExpNode.doIsObject(JSDynamicObject, IsJSObjectNode, JSToBooleanNode, IsJSClassNode, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return doIsObject(arg0Value_, isObjectNode__, INLINED_IS_OBJECT_TO_BOOLEAN_NODE_, isJSRegExpNode__, INLINED_IS_OBJECT_HAS_MATCH_SYMBOL_);
}
state_0 = state_0 | 0b10 /* add SpecializationActive[IsRegExpNode.doNonObject(Object)] */;
this.state_0_ = state_0;
return doNonObject(arg0Value);
}
@NeverDefault
public static IsRegExpNode create(JSContext context) {
return new IsRegExpNodeGen(context);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy