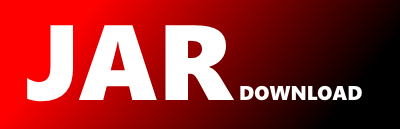
com.oracle.truffle.js.nodes.array.JSArrayNextElementIndexNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.array;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.profiles.InlinedExactClassProfile;
import com.oracle.truffle.js.nodes.access.JSHasPropertyNode;
import com.oracle.truffle.js.runtime.JSContext;
import com.oracle.truffle.js.runtime.array.ScriptArray;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
/**
* Debug Info:
* Specialization {@link JSArrayNextElementIndexNode#doWithoutHolesCached}
* Activation probability: 0.23929
* With/without class size: 8/4 bytes
* Specialization {@link JSArrayNextElementIndexNode#doWithoutHolesUncached}
* Activation probability: 0.20714
* With/without class size: 6/0 bytes
* Specialization {@link JSArrayNextElementIndexNode#nextWithHolesCached}
* Activation probability: 0.17500
* With/without class size: 8/4 bytes
* Specialization {@link JSArrayNextElementIndexNode#nextWithHolesUncached}
* Activation probability: 0.14286
* With/without class size: 7/5 bytes
* Specialization {@link JSArrayNextElementIndexNode#nextObjectViaEnumeration}
* Activation probability: 0.11071
* With/without class size: 5/0 bytes
* Specialization {@link JSArrayNextElementIndexNode#nextObjectViaFullEnumeration}
* Activation probability: 0.07857
* With/without class size: 4/0 bytes
* Specialization {@link JSArrayNextElementIndexNode#nextObjectViaPolling}
* Activation probability: 0.04643
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(JSArrayNextElementIndexNode.class)
@SuppressWarnings("javadoc")
public final class JSArrayNextElementIndexNodeGen extends JSArrayNextElementIndexNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
private static final StateField STATE_0_JSArrayNextElementIndexNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
static final ReferenceField WITHOUT_HOLES_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "withoutHolesCached_cache", WithoutHolesCachedData.class);
static final ReferenceField NEXT_WITH_HOLES_CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "nextWithHolesCached_cache", NextWithHolesCachedData.class);
/**
* Source Info:
* Specialization: {@link JSArrayNextElementIndexNode#nextWithHolesCached}
* Parameter: {@link InlinedConditionProfile} isPlusOne
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_IS_PLUS_ONE = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_UPDATER.subUpdater(7, 2)));
/**
* Source Info:
* Specialization: {@link JSArrayNextElementIndexNode#nextWithHolesUncached}
* Parameter: {@link InlinedExactClassProfile} arrayTypeProfile
* Inline method: {@link InlinedExactClassProfile#inline}
*/
private static final InlinedExactClassProfile INLINED_NEXT_WITH_HOLES_UNCACHED_ARRAY_TYPE_PROFILE_ = InlinedExactClassProfile.inline(InlineTarget.create(InlinedExactClassProfile.class, STATE_0_JSArrayNextElementIndexNode_UPDATER.subUpdater(9, 2), ReferenceField.create(MethodHandles.lookup(), "nextWithHolesUncached_arrayTypeProfile__field1_", Class.class)));
/**
* State Info:
* 0: SpecializationActive {@link JSArrayNextElementIndexNode#doWithoutHolesCached}
* 1: SpecializationActive {@link JSArrayNextElementIndexNode#doWithoutHolesUncached}
* 2: SpecializationActive {@link JSArrayNextElementIndexNode#nextWithHolesCached}
* 3: SpecializationActive {@link JSArrayNextElementIndexNode#nextWithHolesUncached}
* 4: SpecializationActive {@link JSArrayNextElementIndexNode#nextObjectViaEnumeration}
* 5: SpecializationActive {@link JSArrayNextElementIndexNode#nextObjectViaFullEnumeration}
* 6: SpecializationActive {@link JSArrayNextElementIndexNode#nextObjectViaPolling}
* 7-8: InlinedCache
* Specialization: {@link JSArrayNextElementIndexNode#nextWithHolesCached}
* Parameter: {@link InlinedConditionProfile} isPlusOne
* Inline method: {@link InlinedConditionProfile#inline}
* 9-10: InlinedCache
* Specialization: {@link JSArrayNextElementIndexNode#nextWithHolesUncached}
* Parameter: {@link InlinedExactClassProfile} arrayTypeProfile
* Inline method: {@link InlinedExactClassProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link JSArrayNextElementIndexNode#nextWithHolesCached}
* Parameter: {@link JSArrayNextElementIndexNode} nextElementIndexNode
*/
@Child private JSArrayNextElementIndexNode nextElementIndexNode;
/**
* Source Info:
* Specialization: {@link JSArrayNextElementIndexNode#nextObjectViaEnumeration}
* Parameter: {@link JSHasPropertyNode} hasPropertyNode
*/
@Child private JSHasPropertyNode hasPropertyNode;
@UnsafeAccessedField @CompilationFinal private WithoutHolesCachedData withoutHolesCached_cache;
@UnsafeAccessedField @Child private NextWithHolesCachedData nextWithHolesCached_cache;
/**
* Source Info:
* Specialization: {@link JSArrayNextElementIndexNode#nextWithHolesUncached}
* Parameter: {@link InlinedExactClassProfile} arrayTypeProfile
* Inline method: {@link InlinedExactClassProfile#inline}
* Inline field: {@link Class} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Class> nextWithHolesUncached_arrayTypeProfile__field1_;
private JSArrayNextElementIndexNodeGen(JSContext context) {
super(context);
}
@ExplodeLoop
@Override
public long executeLong(Object arg0Value, long arg1Value, long arg2Value, boolean arg3Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1111111) != 0 /* is SpecializationActive[JSArrayNextElementIndexNode.doWithoutHolesCached(JSDynamicObject, long, long, boolean, ScriptArray)] || SpecializationActive[JSArrayNextElementIndexNode.doWithoutHolesUncached(JSDynamicObject, long, long, boolean)] || SpecializationActive[JSArrayNextElementIndexNode.nextWithHolesCached(JSDynamicObject, long, long, boolean, ScriptArray, Node, JSArrayNextElementIndexNode, InlinedConditionProfile)] || SpecializationActive[JSArrayNextElementIndexNode.nextWithHolesUncached(JSDynamicObject, long, long, boolean, JSArrayNextElementIndexNode, InlinedConditionProfile, InlinedExactClassProfile)] || SpecializationActive[JSArrayNextElementIndexNode.nextObjectViaEnumeration(JSDynamicObject, long, long, boolean, JSHasPropertyNode)] || SpecializationActive[JSArrayNextElementIndexNode.nextObjectViaFullEnumeration(JSDynamicObject, long, long, boolean, JSHasPropertyNode)] || SpecializationActive[JSArrayNextElementIndexNode.nextObjectViaPolling(Object, long, long, boolean, JSHasPropertyNode)] */) {
if ((state_0 & 0b111111) != 0 /* is SpecializationActive[JSArrayNextElementIndexNode.doWithoutHolesCached(JSDynamicObject, long, long, boolean, ScriptArray)] || SpecializationActive[JSArrayNextElementIndexNode.doWithoutHolesUncached(JSDynamicObject, long, long, boolean)] || SpecializationActive[JSArrayNextElementIndexNode.nextWithHolesCached(JSDynamicObject, long, long, boolean, ScriptArray, Node, JSArrayNextElementIndexNode, InlinedConditionProfile)] || SpecializationActive[JSArrayNextElementIndexNode.nextWithHolesUncached(JSDynamicObject, long, long, boolean, JSArrayNextElementIndexNode, InlinedConditionProfile, InlinedExactClassProfile)] || SpecializationActive[JSArrayNextElementIndexNode.nextObjectViaEnumeration(JSDynamicObject, long, long, boolean, JSHasPropertyNode)] || SpecializationActive[JSArrayNextElementIndexNode.nextObjectViaFullEnumeration(JSDynamicObject, long, long, boolean, JSHasPropertyNode)] */ && arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSArrayNextElementIndexNode.doWithoutHolesCached(JSDynamicObject, long, long, boolean, ScriptArray)] */ && (arg3Value) && (!(hasPrototypeElements(arg0Value_)))) {
WithoutHolesCachedData s0_ = this.withoutHolesCached_cache;
while (s0_ != null) {
if ((JSArrayElementIndexNode.getArrayType(arg0Value_) == s0_.cachedArrayType_) && (!(s0_.cachedArrayType_.hasHoles(arg0Value_)))) {
return doWithoutHolesCached(arg0Value_, arg1Value, arg2Value, arg3Value, s0_.cachedArrayType_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSArrayNextElementIndexNode.doWithoutHolesUncached(JSDynamicObject, long, long, boolean)] */) {
if ((arg3Value) && (!(hasPrototypeElements(arg0Value_))) && (!(JSArrayElementIndexNode.hasHoles(arg0Value_)))) {
return doWithoutHolesUncached(arg0Value_, arg1Value, arg2Value, arg3Value);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSArrayNextElementIndexNode.nextWithHolesCached(JSDynamicObject, long, long, boolean, ScriptArray, Node, JSArrayNextElementIndexNode, InlinedConditionProfile)] */ && (arg3Value) && (!(hasPrototypeElements(arg0Value_)))) {
NextWithHolesCachedData s2_ = this.nextWithHolesCached_cache;
while (s2_ != null) {
{
JSArrayNextElementIndexNode nextElementIndexNode_ = this.nextElementIndexNode;
if (nextElementIndexNode_ != null) {
if ((JSArrayElementIndexNode.getArrayType(arg0Value_) == s2_.cachedArrayType_) && (s2_.cachedArrayType_.hasHoles(arg0Value_))) {
Node node__ = (this);
return nextWithHolesCached(arg0Value_, arg1Value, arg2Value, arg3Value, s2_.cachedArrayType_, node__, nextElementIndexNode_, INLINED_IS_PLUS_ONE);
}
}
}
s2_ = s2_.next_;
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSArrayNextElementIndexNode.nextWithHolesUncached(JSDynamicObject, long, long, boolean, JSArrayNextElementIndexNode, InlinedConditionProfile, InlinedExactClassProfile)] */) {
{
JSArrayNextElementIndexNode nextElementIndexNode_1 = this.nextElementIndexNode;
if (nextElementIndexNode_1 != null) {
if ((arg3Value) && (hasPrototypeElements(arg0Value_) || JSArrayElementIndexNode.hasHoles(arg0Value_))) {
return nextWithHolesUncached(arg0Value_, arg1Value, arg2Value, arg3Value, nextElementIndexNode_1, INLINED_IS_PLUS_ONE, INLINED_NEXT_WITH_HOLES_UNCACHED_ARRAY_TYPE_PROFILE_);
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSArrayNextElementIndexNode.nextObjectViaEnumeration(JSDynamicObject, long, long, boolean, JSHasPropertyNode)] */) {
{
JSHasPropertyNode hasPropertyNode_ = this.hasPropertyNode;
if (hasPropertyNode_ != null) {
if ((!(arg3Value)) && (isSuitableForEnumBasedProcessingUsingOwnKeys(arg0Value_, arg2Value))) {
return nextObjectViaEnumeration(arg0Value_, arg1Value, arg2Value, arg3Value, hasPropertyNode_);
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSArrayNextElementIndexNode.nextObjectViaFullEnumeration(JSDynamicObject, long, long, boolean, JSHasPropertyNode)] */) {
{
JSHasPropertyNode hasPropertyNode_1 = this.hasPropertyNode;
if (hasPropertyNode_1 != null) {
if ((!(arg3Value)) && (!(isSuitableForEnumBasedProcessingUsingOwnKeys(arg0Value_, arg2Value))) && (JSArrayElementIndexNode.isSuitableForEnumBasedProcessing(arg0Value_, arg2Value))) {
return nextObjectViaFullEnumeration(arg0Value_, arg1Value, arg2Value, arg3Value, hasPropertyNode_1);
}
}
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[JSArrayNextElementIndexNode.nextObjectViaPolling(Object, long, long, boolean, JSHasPropertyNode)] */) {
{
JSHasPropertyNode hasPropertyNode_2 = this.hasPropertyNode;
if (hasPropertyNode_2 != null) {
if ((!(arg3Value)) && (!(JSArrayElementIndexNode.isSuitableForEnumBasedProcessing(arg0Value, arg2Value)))) {
return nextObjectViaPolling(arg0Value, arg1Value, arg2Value, arg3Value, hasPropertyNode_2);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private long executeAndSpecialize(Object arg0Value, long arg1Value, long arg2Value, boolean arg3Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSArrayNextElementIndexNode.doWithoutHolesUncached(JSDynamicObject, long, long, boolean)] */ && (arg3Value) && (!(hasPrototypeElements(arg0Value_)))) {
while (true) {
int count0_ = 0;
WithoutHolesCachedData s0_ = WITHOUT_HOLES_CACHED_CACHE_UPDATER.getVolatile(this);
WithoutHolesCachedData s0_original = s0_;
while (s0_ != null) {
if ((JSArrayElementIndexNode.getArrayType(arg0Value_) == s0_.cachedArrayType_) && (!(s0_.cachedArrayType_.hasHoles(arg0Value_)))) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
ScriptArray cachedArrayType__ = (JSArrayElementIndexNode.getArrayTypeIfArray(arg0Value_, arg3Value));
if ((JSArrayElementIndexNode.getArrayType(arg0Value_) == cachedArrayType__) && (!(cachedArrayType__.hasHoles(arg0Value_))) && count0_ < (JSArrayElementIndexNode.MAX_CACHED_ARRAY_TYPES)) {
s0_ = new WithoutHolesCachedData(s0_original);
s0_.cachedArrayType_ = cachedArrayType__;
if (!WITHOUT_HOLES_CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[JSArrayNextElementIndexNode.doWithoutHolesCached(JSDynamicObject, long, long, boolean, ScriptArray)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return doWithoutHolesCached(arg0Value_, arg1Value, arg2Value, arg3Value, s0_.cachedArrayType_);
}
break;
}
}
if ((arg3Value) && (!(hasPrototypeElements(arg0Value_))) && (!(JSArrayElementIndexNode.hasHoles(arg0Value_)))) {
this.withoutHolesCached_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[JSArrayNextElementIndexNode.doWithoutHolesCached(JSDynamicObject, long, long, boolean, ScriptArray)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSArrayNextElementIndexNode.doWithoutHolesUncached(JSDynamicObject, long, long, boolean)] */;
this.state_0_ = state_0;
return doWithoutHolesUncached(arg0Value_, arg1Value, arg2Value, arg3Value);
}
{
Node node__ = null;
if (((state_0 & 0b1000)) == 0 /* is-not SpecializationActive[JSArrayNextElementIndexNode.nextWithHolesUncached(JSDynamicObject, long, long, boolean, JSArrayNextElementIndexNode, InlinedConditionProfile, InlinedExactClassProfile)] */ && (arg3Value) && (!(hasPrototypeElements(arg0Value_)))) {
while (true) {
int count2_ = 0;
NextWithHolesCachedData s2_ = NEXT_WITH_HOLES_CACHED_CACHE_UPDATER.getVolatile(this);
NextWithHolesCachedData s2_original = s2_;
while (s2_ != null) {
{
JSArrayNextElementIndexNode nextElementIndexNode_ = this.nextElementIndexNode;
if (nextElementIndexNode_ != null) {
if ((JSArrayElementIndexNode.getArrayType(arg0Value_) == s2_.cachedArrayType_) && (s2_.cachedArrayType_.hasHoles(arg0Value_))) {
node__ = (this);
break;
}
}
}
count2_++;
s2_ = s2_.next_;
}
if (s2_ == null) {
{
ScriptArray cachedArrayType__1 = (JSArrayElementIndexNode.getArrayTypeIfArray(arg0Value_, arg3Value));
if ((JSArrayElementIndexNode.getArrayType(arg0Value_) == cachedArrayType__1) && (cachedArrayType__1.hasHoles(arg0Value_)) && count2_ < (JSArrayElementIndexNode.MAX_CACHED_ARRAY_TYPES)) {
s2_ = this.insert(new NextWithHolesCachedData(s2_original));
s2_.cachedArrayType_ = cachedArrayType__1;
node__ = (this);
JSArrayNextElementIndexNode nextElementIndexNode_;
JSArrayNextElementIndexNode nextElementIndexNode__shared = this.nextElementIndexNode;
if (nextElementIndexNode__shared != null) {
nextElementIndexNode_ = nextElementIndexNode__shared;
} else {
nextElementIndexNode_ = s2_.insert((JSArrayNextElementIndexNode.create(context)));
if (nextElementIndexNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.nextElementIndexNode == null) {
this.nextElementIndexNode = nextElementIndexNode_;
}
if (!NEXT_WITH_HOLES_CACHED_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[JSArrayNextElementIndexNode.nextWithHolesCached(JSDynamicObject, long, long, boolean, ScriptArray, Node, JSArrayNextElementIndexNode, InlinedConditionProfile)] */;
this.state_0_ = state_0;
}
}
}
if (s2_ != null) {
return nextWithHolesCached(arg0Value_, arg1Value, arg2Value, arg3Value, s2_.cachedArrayType_, node__, this.nextElementIndexNode, INLINED_IS_PLUS_ONE);
}
break;
}
}
}
if ((arg3Value) && (hasPrototypeElements(arg0Value_) || JSArrayElementIndexNode.hasHoles(arg0Value_))) {
JSArrayNextElementIndexNode nextElementIndexNode_1;
JSArrayNextElementIndexNode nextElementIndexNode_1_shared = this.nextElementIndexNode;
if (nextElementIndexNode_1_shared != null) {
nextElementIndexNode_1 = nextElementIndexNode_1_shared;
} else {
nextElementIndexNode_1 = this.insert((JSArrayNextElementIndexNode.create(context)));
if (nextElementIndexNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.nextElementIndexNode == null) {
VarHandle.storeStoreFence();
this.nextElementIndexNode = nextElementIndexNode_1;
}
this.nextWithHolesCached_cache = null;
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[JSArrayNextElementIndexNode.nextWithHolesCached(JSDynamicObject, long, long, boolean, ScriptArray, Node, JSArrayNextElementIndexNode, InlinedConditionProfile)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSArrayNextElementIndexNode.nextWithHolesUncached(JSDynamicObject, long, long, boolean, JSArrayNextElementIndexNode, InlinedConditionProfile, InlinedExactClassProfile)] */;
this.state_0_ = state_0;
return nextWithHolesUncached(arg0Value_, arg1Value, arg2Value, arg3Value, nextElementIndexNode_1, INLINED_IS_PLUS_ONE, INLINED_NEXT_WITH_HOLES_UNCACHED_ARRAY_TYPE_PROFILE_);
}
if ((!(arg3Value)) && (isSuitableForEnumBasedProcessingUsingOwnKeys(arg0Value_, arg2Value))) {
JSHasPropertyNode hasPropertyNode_;
JSHasPropertyNode hasPropertyNode__shared = this.hasPropertyNode;
if (hasPropertyNode__shared != null) {
hasPropertyNode_ = hasPropertyNode__shared;
} else {
hasPropertyNode_ = this.insert((JSHasPropertyNode.create()));
if (hasPropertyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.hasPropertyNode == null) {
VarHandle.storeStoreFence();
this.hasPropertyNode = hasPropertyNode_;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSArrayNextElementIndexNode.nextObjectViaEnumeration(JSDynamicObject, long, long, boolean, JSHasPropertyNode)] */;
this.state_0_ = state_0;
return nextObjectViaEnumeration(arg0Value_, arg1Value, arg2Value, arg3Value, hasPropertyNode_);
}
if ((!(arg3Value)) && (!(isSuitableForEnumBasedProcessingUsingOwnKeys(arg0Value_, arg2Value))) && (JSArrayElementIndexNode.isSuitableForEnumBasedProcessing(arg0Value_, arg2Value))) {
JSHasPropertyNode hasPropertyNode_1;
JSHasPropertyNode hasPropertyNode_1_shared = this.hasPropertyNode;
if (hasPropertyNode_1_shared != null) {
hasPropertyNode_1 = hasPropertyNode_1_shared;
} else {
hasPropertyNode_1 = this.insert((JSHasPropertyNode.create()));
if (hasPropertyNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.hasPropertyNode == null) {
VarHandle.storeStoreFence();
this.hasPropertyNode = hasPropertyNode_1;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSArrayNextElementIndexNode.nextObjectViaFullEnumeration(JSDynamicObject, long, long, boolean, JSHasPropertyNode)] */;
this.state_0_ = state_0;
return nextObjectViaFullEnumeration(arg0Value_, arg1Value, arg2Value, arg3Value, hasPropertyNode_1);
}
}
if ((!(arg3Value)) && (!(JSArrayElementIndexNode.isSuitableForEnumBasedProcessing(arg0Value, arg2Value)))) {
JSHasPropertyNode hasPropertyNode_2;
JSHasPropertyNode hasPropertyNode_2_shared = this.hasPropertyNode;
if (hasPropertyNode_2_shared != null) {
hasPropertyNode_2 = hasPropertyNode_2_shared;
} else {
hasPropertyNode_2 = this.insert((JSHasPropertyNode.create()));
if (hasPropertyNode_2 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.hasPropertyNode == null) {
VarHandle.storeStoreFence();
this.hasPropertyNode = hasPropertyNode_2;
}
state_0 = state_0 | 0b1000000 /* add SpecializationActive[JSArrayNextElementIndexNode.nextObjectViaPolling(Object, long, long, boolean, JSHasPropertyNode)] */;
this.state_0_ = state_0;
return nextObjectViaPolling(arg0Value, arg1Value, arg2Value, arg3Value, hasPropertyNode_2);
}
throw new UnsupportedSpecializationException(this, null, arg0Value, arg1Value, arg2Value, arg3Value);
}
@NeverDefault
public static JSArrayNextElementIndexNode create(JSContext context) {
return new JSArrayNextElementIndexNodeGen(context);
}
@GeneratedBy(JSArrayNextElementIndexNode.class)
@DenyReplace
private static final class WithoutHolesCachedData implements SpecializationDataNode {
@CompilationFinal final WithoutHolesCachedData next_;
/**
* Source Info:
* Specialization: {@link JSArrayNextElementIndexNode#doWithoutHolesCached}
* Parameter: {@link ScriptArray} cachedArrayType
*/
@CompilationFinal ScriptArray cachedArrayType_;
WithoutHolesCachedData(WithoutHolesCachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(JSArrayNextElementIndexNode.class)
@DenyReplace
private static final class NextWithHolesCachedData extends Node implements SpecializationDataNode {
@Child NextWithHolesCachedData next_;
/**
* Source Info:
* Specialization: {@link JSArrayNextElementIndexNode#nextWithHolesCached}
* Parameter: {@link ScriptArray} cachedArrayType
*/
@CompilationFinal ScriptArray cachedArrayType_;
NextWithHolesCachedData(NextWithHolesCachedData next_) {
this.next_ = next_;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy