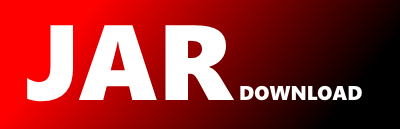
com.oracle.truffle.js.nodes.array.JSArrayToDenseObjectArrayNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.array;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.js.nodes.access.ReadElementNode;
import com.oracle.truffle.js.runtime.array.ScriptArray;
import com.oracle.truffle.js.runtime.builtins.JSArrayObject;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
/**
* Debug Info:
* Specialization {@link JSArrayToDenseObjectArrayNode#fromDenseArray}
* Activation probability: 0.48333
* With/without class size: 13/4 bytes
* Specialization {@link JSArrayToDenseObjectArrayNode#fromSparseArray}
* Activation probability: 0.33333
* With/without class size: 12/4 bytes
* Specialization {@link JSArrayToDenseObjectArrayNode#doUncached}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(JSArrayToDenseObjectArrayNode.class)
@SuppressWarnings("javadoc")
public final class JSArrayToDenseObjectArrayNodeGen extends JSArrayToDenseObjectArrayNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
static final ReferenceField FROM_DENSE_ARRAY_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "fromDenseArray_cache", FromDenseArrayData.class);
static final ReferenceField FROM_SPARSE_ARRAY_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "fromSparseArray_cache", FromSparseArrayData.class);
/**
* Source Info:
* Specialization: {@link JSArrayToDenseObjectArrayNode#fromSparseArray}
* Parameter: {@link InlinedBranchProfile} growProfile
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_GROW_PROFILE = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(3, 1)));
/**
* State Info:
* 0: SpecializationActive {@link JSArrayToDenseObjectArrayNode#fromDenseArray}
* 1: SpecializationActive {@link JSArrayToDenseObjectArrayNode#doUncached}
* 2: SpecializationActive {@link JSArrayToDenseObjectArrayNode#fromSparseArray}
* 3: InlinedCache
* Specialization: {@link JSArrayToDenseObjectArrayNode#fromSparseArray}
* Parameter: {@link InlinedBranchProfile} growProfile
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link JSArrayToDenseObjectArrayNode#fromDenseArray}
* Parameter: {@link ReadElementNode} readNode
*/
@Child private ReadElementNode readNode;
/**
* Source Info:
* Specialization: {@link JSArrayToDenseObjectArrayNode#fromSparseArray}
* Parameter: {@link JSArrayNextElementIndexNode} nextElementIndexNode
*/
@Child private JSArrayNextElementIndexNode nextElementIndexNode;
@UnsafeAccessedField @CompilationFinal private FromDenseArrayData fromDenseArray_cache;
@UnsafeAccessedField @Child private FromSparseArrayData fromSparseArray_cache;
private JSArrayToDenseObjectArrayNodeGen() {
}
@ExplodeLoop
@Override
public Object[] executeObjectArray(JSArrayObject arg0Value, ScriptArray arg1Value, long arg2Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111) != 0 /* is SpecializationActive[JSArrayToDenseObjectArrayNode.fromDenseArray(JSArrayObject, ScriptArray, long, ScriptArray, ReadElementNode)] || SpecializationActive[JSArrayToDenseObjectArrayNode.fromSparseArray(JSArrayObject, ScriptArray, long, ScriptArray, Node, JSArrayNextElementIndexNode, InlinedBranchProfile)] || SpecializationActive[JSArrayToDenseObjectArrayNode.doUncached(JSArrayObject, ScriptArray, long, JSArrayNextElementIndexNode, ReadElementNode, InlinedBranchProfile)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSArrayToDenseObjectArrayNode.fromDenseArray(JSArrayObject, ScriptArray, long, ScriptArray, ReadElementNode)] */) {
FromDenseArrayData s0_ = this.fromDenseArray_cache;
while (s0_ != null) {
{
ReadElementNode readNode_ = this.readNode;
if (readNode_ != null) {
if ((s0_.cachedArrayType_.isInstance(arg1Value))) {
assert DSLSupport.assertIdempotence((!(s0_.cachedArrayType_.isHolesType())));
return JSArrayToDenseObjectArrayNode.fromDenseArray(arg0Value, arg1Value, arg2Value, s0_.cachedArrayType_, readNode_);
}
}
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSArrayToDenseObjectArrayNode.fromSparseArray(JSArrayObject, ScriptArray, long, ScriptArray, Node, JSArrayNextElementIndexNode, InlinedBranchProfile)] */) {
FromSparseArrayData s1_ = this.fromSparseArray_cache;
while (s1_ != null) {
{
JSArrayNextElementIndexNode nextElementIndexNode_ = this.nextElementIndexNode;
if (nextElementIndexNode_ != null) {
if ((s1_.cachedArrayType_.isInstance(arg1Value))) {
Node node__ = (this);
assert DSLSupport.assertIdempotence((s1_.cachedArrayType_.isHolesType()));
return JSArrayToDenseObjectArrayNode.fromSparseArray(arg0Value, arg1Value, arg2Value, s1_.cachedArrayType_, node__, nextElementIndexNode_, INLINED_GROW_PROFILE);
}
}
}
s1_ = s1_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSArrayToDenseObjectArrayNode.doUncached(JSArrayObject, ScriptArray, long, JSArrayNextElementIndexNode, ReadElementNode, InlinedBranchProfile)] */) {
{
JSArrayNextElementIndexNode nextElementIndexNode_1 = this.nextElementIndexNode;
if (nextElementIndexNode_1 != null) {
ReadElementNode readNode_1 = this.readNode;
if (readNode_1 != null) {
return doUncached(arg0Value, arg1Value, arg2Value, nextElementIndexNode_1, readNode_1, INLINED_GROW_PROFILE);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object[] executeAndSpecialize(JSArrayObject arg0Value, ScriptArray arg1Value, long arg2Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSArrayToDenseObjectArrayNode.doUncached(JSArrayObject, ScriptArray, long, JSArrayNextElementIndexNode, ReadElementNode, InlinedBranchProfile)] */) {
while (true) {
int count0_ = 0;
FromDenseArrayData s0_ = FROM_DENSE_ARRAY_CACHE_UPDATER.getVolatile(this);
FromDenseArrayData s0_original = s0_;
while (s0_ != null) {
{
ReadElementNode readNode_ = this.readNode;
if (readNode_ != null) {
if ((s0_.cachedArrayType_.isInstance(arg1Value))) {
assert DSLSupport.assertIdempotence((!(s0_.cachedArrayType_.isHolesType())));
break;
}
}
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
ScriptArray cachedArrayType__ = (arg1Value);
if ((cachedArrayType__.isInstance(arg1Value)) && (!(cachedArrayType__.isHolesType())) && count0_ < (5)) {
s0_ = new FromDenseArrayData(s0_original);
s0_.cachedArrayType_ = cachedArrayType__;
ReadElementNode readNode_;
ReadElementNode readNode__shared = this.readNode;
if (readNode__shared != null) {
readNode_ = readNode__shared;
} else {
readNode_ = this.insert((ReadElementNode.create(getJSContext())));
if (readNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readNode == null) {
this.readNode = readNode_;
}
if (!FROM_DENSE_ARRAY_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[JSArrayToDenseObjectArrayNode.fromDenseArray(JSArrayObject, ScriptArray, long, ScriptArray, ReadElementNode)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return JSArrayToDenseObjectArrayNode.fromDenseArray(arg0Value, arg1Value, arg2Value, s0_.cachedArrayType_, this.readNode);
}
break;
}
}
{
Node node__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSArrayToDenseObjectArrayNode.doUncached(JSArrayObject, ScriptArray, long, JSArrayNextElementIndexNode, ReadElementNode, InlinedBranchProfile)] */) {
while (true) {
int count1_ = 0;
FromSparseArrayData s1_ = FROM_SPARSE_ARRAY_CACHE_UPDATER.getVolatile(this);
FromSparseArrayData s1_original = s1_;
while (s1_ != null) {
{
JSArrayNextElementIndexNode nextElementIndexNode_ = this.nextElementIndexNode;
if (nextElementIndexNode_ != null) {
if ((s1_.cachedArrayType_.isInstance(arg1Value))) {
assert DSLSupport.assertIdempotence((s1_.cachedArrayType_.isHolesType()));
node__ = (this);
break;
}
}
}
count1_++;
s1_ = s1_.next_;
}
if (s1_ == null) {
{
ScriptArray cachedArrayType__1 = (arg1Value);
if ((cachedArrayType__1.isInstance(arg1Value)) && (cachedArrayType__1.isHolesType()) && count1_ < (5)) {
s1_ = this.insert(new FromSparseArrayData(s1_original));
s1_.cachedArrayType_ = cachedArrayType__1;
node__ = (this);
JSArrayNextElementIndexNode nextElementIndexNode_;
JSArrayNextElementIndexNode nextElementIndexNode__shared = this.nextElementIndexNode;
if (nextElementIndexNode__shared != null) {
nextElementIndexNode_ = nextElementIndexNode__shared;
} else {
nextElementIndexNode_ = s1_.insert((JSArrayNextElementIndexNode.create(getJSContext())));
if (nextElementIndexNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.nextElementIndexNode == null) {
this.nextElementIndexNode = nextElementIndexNode_;
}
if (!FROM_SPARSE_ARRAY_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[JSArrayToDenseObjectArrayNode.fromSparseArray(JSArrayObject, ScriptArray, long, ScriptArray, Node, JSArrayNextElementIndexNode, InlinedBranchProfile)] */;
this.state_0_ = state_0;
}
}
}
if (s1_ != null) {
return JSArrayToDenseObjectArrayNode.fromSparseArray(arg0Value, arg1Value, arg2Value, s1_.cachedArrayType_, node__, this.nextElementIndexNode, INLINED_GROW_PROFILE);
}
break;
}
}
}
JSArrayNextElementIndexNode nextElementIndexNode_1;
JSArrayNextElementIndexNode nextElementIndexNode_1_shared = this.nextElementIndexNode;
if (nextElementIndexNode_1_shared != null) {
nextElementIndexNode_1 = nextElementIndexNode_1_shared;
} else {
nextElementIndexNode_1 = this.insert((JSArrayNextElementIndexNode.create(getJSContext())));
if (nextElementIndexNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.nextElementIndexNode == null) {
VarHandle.storeStoreFence();
this.nextElementIndexNode = nextElementIndexNode_1;
}
ReadElementNode readNode_1;
ReadElementNode readNode_1_shared = this.readNode;
if (readNode_1_shared != null) {
readNode_1 = readNode_1_shared;
} else {
readNode_1 = this.insert((ReadElementNode.create(getJSContext())));
if (readNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.readNode == null) {
VarHandle.storeStoreFence();
this.readNode = readNode_1;
}
this.fromDenseArray_cache = null;
this.fromSparseArray_cache = null;
state_0 = state_0 & 0xfffffffa /* remove SpecializationActive[JSArrayToDenseObjectArrayNode.fromDenseArray(JSArrayObject, ScriptArray, long, ScriptArray, ReadElementNode)], SpecializationActive[JSArrayToDenseObjectArrayNode.fromSparseArray(JSArrayObject, ScriptArray, long, ScriptArray, Node, JSArrayNextElementIndexNode, InlinedBranchProfile)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSArrayToDenseObjectArrayNode.doUncached(JSArrayObject, ScriptArray, long, JSArrayNextElementIndexNode, ReadElementNode, InlinedBranchProfile)] */;
this.state_0_ = state_0;
return doUncached(arg0Value, arg1Value, arg2Value, nextElementIndexNode_1, readNode_1, INLINED_GROW_PROFILE);
}
@NeverDefault
public static JSArrayToDenseObjectArrayNode create() {
return new JSArrayToDenseObjectArrayNodeGen();
}
@GeneratedBy(JSArrayToDenseObjectArrayNode.class)
@DenyReplace
private static final class FromDenseArrayData implements SpecializationDataNode {
@CompilationFinal final FromDenseArrayData next_;
/**
* Source Info:
* Specialization: {@link JSArrayToDenseObjectArrayNode#fromDenseArray}
* Parameter: {@link ScriptArray} cachedArrayType
*/
@CompilationFinal ScriptArray cachedArrayType_;
FromDenseArrayData(FromDenseArrayData next_) {
this.next_ = next_;
}
}
@GeneratedBy(JSArrayToDenseObjectArrayNode.class)
@DenyReplace
private static final class FromSparseArrayData extends Node implements SpecializationDataNode {
@Child FromSparseArrayData next_;
/**
* Source Info:
* Specialization: {@link JSArrayToDenseObjectArrayNode#fromSparseArray}
* Parameter: {@link ScriptArray} cachedArrayType
*/
@CompilationFinal ScriptArray cachedArrayType_;
FromSparseArrayData(FromSparseArrayData next_) {
this.next_ = next_;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy