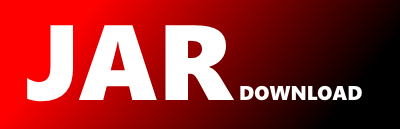
com.oracle.truffle.js.nodes.binary.InstanceofNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.binary;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.JSTypes;
import com.oracle.truffle.js.nodes.JSTypesGen;
import com.oracle.truffle.js.nodes.JavaScriptNode;
import com.oracle.truffle.js.nodes.access.GetMethodNode;
import com.oracle.truffle.js.nodes.access.GetPrototypeNode;
import com.oracle.truffle.js.nodes.access.IsJSObjectNode;
import com.oracle.truffle.js.nodes.access.IsObjectNode;
import com.oracle.truffle.js.nodes.cast.JSToBooleanNode;
import com.oracle.truffle.js.nodes.cast.JSToBooleanNodeGen;
import com.oracle.truffle.js.nodes.function.JSFunctionCallNode;
import com.oracle.truffle.js.nodes.interop.ForeignObjectPrototypeNode;
import com.oracle.truffle.js.nodes.unary.IsCallableNode;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.JSConfig;
import com.oracle.truffle.js.runtime.JSContext;
import com.oracle.truffle.js.runtime.Symbol;
import com.oracle.truffle.js.runtime.builtins.JSFunction;
import com.oracle.truffle.js.runtime.builtins.JSFunctionObject;
import com.oracle.truffle.js.runtime.builtins.JSProxyObject;
import com.oracle.truffle.js.runtime.builtins.JSFunctionObject.Bound;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link InstanceofNode#doJSObject}
* Activation probability: 0.15909
* With/without class size: 10/23 bytes
* Specialization {@link InstanceofNode#doNullOrUndefinedTarget}
* Activation probability: 0.14545
* With/without class size: 5/0 bytes
* Specialization {@link InstanceofNode#doStringTarget}
* Activation probability: 0.13182
* With/without class size: 5/0 bytes
* Specialization {@link InstanceofNode#doDoubleTarget}
* Activation probability: 0.11818
* With/without class size: 5/0 bytes
* Specialization {@link InstanceofNode#doLongTarget}
* Activation probability: 0.10455
* With/without class size: 5/0 bytes
* Specialization {@link InstanceofNode#doBooleanTarget}
* Activation probability: 0.09091
* With/without class size: 5/0 bytes
* Specialization {@link InstanceofNode#doBigIntTarget}
* Activation probability: 0.07727
* With/without class size: 4/0 bytes
* Specialization {@link InstanceofNode#doSymbolTarget}
* Activation probability: 0.06364
* With/without class size: 4/0 bytes
* Specialization {@link InstanceofNode#doForeignTargetJSType}
* Activation probability: 0.05000
* With/without class size: 4/0 bytes
* Specialization {@link InstanceofNode#doForeignTargetOther}
* Activation probability: 0.03636
* With/without class size: 4/4 bytes
* Specialization {@link InstanceofNode#doForeignTargetOther}
* Activation probability: 0.02273
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(InstanceofNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class InstanceofNodeGen extends InstanceofNode {
private static final StateField J_S_OBJECT__INSTANCEOF_NODE_J_S_OBJECT_STATE_0_UPDATER = StateField.create(JSObjectData.lookup_(), "jSObject_state_0_");
static final ReferenceField J_S_OBJECT_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "jSObject_cache", JSObjectData.class);
static final ReferenceField FOREIGN_TARGET_OTHER0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "foreignTargetOther0_cache", ForeignTargetOther0Data.class);
/**
* Source Info:
* Specialization: {@link InstanceofNode#doJSObject}
* Parameter: {@link JSToBooleanNode} toBooleanNode
* Inline method: {@link JSToBooleanNodeGen#inline}
*/
private static final JSToBooleanNode INLINED_J_S_OBJECT_TO_BOOLEAN_NODE_ = JSToBooleanNodeGen.inline(InlineTarget.create(JSToBooleanNode.class, J_S_OBJECT__INSTANCEOF_NODE_J_S_OBJECT_STATE_0_UPDATER.subUpdater(0, 15), ReferenceField.create(JSObjectData.lookup_(), "jSObject_toBooleanNode__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link InstanceofNode#doJSObject}
* Parameter: {@link InlinedConditionProfile} hasInstanceProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_J_S_OBJECT_HAS_INSTANCE_PROFILE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, J_S_OBJECT__INSTANCEOF_NODE_J_S_OBJECT_STATE_0_UPDATER.subUpdater(15, 2)));
/**
* Source Info:
* Specialization: {@link InstanceofNode#doJSObject}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_J_S_OBJECT_ERROR_BRANCH_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, J_S_OBJECT__INSTANCEOF_NODE_J_S_OBJECT_STATE_0_UPDATER.subUpdater(17, 1)));
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link InstanceofNode#doJSObject}
* 1: SpecializationActive {@link InstanceofNode#doNullOrUndefinedTarget}
* 2: SpecializationActive {@link InstanceofNode#doStringTarget}
* 3: SpecializationActive {@link InstanceofNode#doDoubleTarget}
* 4: SpecializationActive {@link InstanceofNode#doLongTarget}
* 5: SpecializationActive {@link InstanceofNode#doBooleanTarget}
* 6: SpecializationActive {@link InstanceofNode#doBigIntTarget}
* 7: SpecializationActive {@link InstanceofNode#doSymbolTarget}
* 8: SpecializationActive {@link InstanceofNode#doForeignTargetJSType}
* 9: SpecializationActive {@link InstanceofNode#doForeignTargetOther}
* 10: SpecializationActive {@link InstanceofNode#doForeignTargetOther}
* 11-13: ImplicitCast[type=double, index=1]
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @Child private JSObjectData jSObject_cache;
@UnsafeAccessedField @Child private ForeignTargetOther0Data foreignTargetOther0_cache;
private InstanceofNodeGen(JSContext context, JavaScriptNode left, JavaScriptNode right) {
super(context, left, right);
}
@ExplodeLoop
@Override
public boolean executeBoolean(Object leftNodeValue, Object rightNodeValue) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111111111) != 0 /* is SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] || SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] || SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] || SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] || SpecializationActive[InstanceofNode.doLongTarget(Object, long)] || SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] || SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] || SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] || SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((state_0 & 0b11111111) != 0 /* is SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] || SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] || SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] || SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] || SpecializationActive[InstanceofNode.doLongTarget(Object, long)] || SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] || SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] || SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] */ && rightNodeValue instanceof JSDynamicObject) {
JSDynamicObject rightNodeValue_ = (JSDynamicObject) rightNodeValue;
JSObjectData s0_ = this.jSObject_cache;
if (s0_ != null) {
if ((s0_.isObjectNode_.executeBoolean(rightNodeValue_))) {
Node node__ = (s0_);
return doJSObject(leftNodeValue, rightNodeValue_, node__, s0_.isObjectNode_, s0_.getMethodHasInstanceNode_, INLINED_J_S_OBJECT_TO_BOOLEAN_NODE_, s0_.callHasInstanceNode_, s0_.isCallableNode_, INLINED_J_S_OBJECT_HAS_INSTANCE_PROFILE_, INLINED_J_S_OBJECT_ERROR_BRANCH_);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] */) {
if ((JSGuards.isNullOrUndefined(rightNodeValue))) {
return doNullOrUndefinedTarget(leftNodeValue, rightNodeValue);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] */ && rightNodeValue instanceof TruffleString) {
TruffleString rightNodeValue_ = (TruffleString) rightNodeValue;
return doStringTarget(leftNodeValue, rightNodeValue_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b11100000000000) >>> 11 /* get-int ImplicitCast[type=double, index=1] */, rightNodeValue)) {
double rightNodeValue_ = JSTypesGen.asImplicitDouble((state_0 & 0b11100000000000) >>> 11 /* get-int ImplicitCast[type=double, index=1] */, rightNodeValue);
return doDoubleTarget(leftNodeValue, rightNodeValue_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[InstanceofNode.doLongTarget(Object, long)] */ && rightNodeValue instanceof Long) {
long rightNodeValue_ = (long) rightNodeValue;
return doLongTarget(leftNodeValue, rightNodeValue_);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] */ && rightNodeValue instanceof Boolean) {
boolean rightNodeValue_ = (boolean) rightNodeValue;
return doBooleanTarget(leftNodeValue, rightNodeValue_);
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] */ && rightNodeValue instanceof BigInt) {
BigInt rightNodeValue_ = (BigInt) rightNodeValue;
return doBigIntTarget(leftNodeValue, rightNodeValue_);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] */ && rightNodeValue instanceof Symbol) {
Symbol rightNodeValue_ = (Symbol) rightNodeValue;
return doSymbolTarget(leftNodeValue, rightNodeValue_);
}
}
if ((state_0 & 0b11100000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] */ && leftNodeValue instanceof JSDynamicObject) {
JSDynamicObject leftNodeValue_ = (JSDynamicObject) leftNodeValue;
if ((JSGuards.isForeignObject(rightNodeValue))) {
return doForeignTargetJSType(leftNodeValue_, rightNodeValue);
}
}
if ((state_0 & 0b11000000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
ForeignTargetOther0Data s9_ = this.foreignTargetOther0_cache;
while (s9_ != null) {
if ((s9_.interop_.accepts(rightNodeValue)) && (JSGuards.isForeignObject(rightNodeValue)) && (!(JSGuards.isJSDynamicObject(leftNodeValue)))) {
return doForeignTargetOther(leftNodeValue, rightNodeValue, s9_.interop_);
}
s9_ = s9_.next_;
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((JSGuards.isForeignObject(rightNodeValue)) && (!(JSGuards.isJSDynamicObject(leftNodeValue)))) {
return this.foreignTargetOther1Boundary(state_0, leftNodeValue, rightNodeValue);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue, rightNodeValue);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private boolean foreignTargetOther1Boundary(int state_0, Object leftNodeValue, Object rightNodeValue) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(rightNodeValue));
return doForeignTargetOther(leftNodeValue, rightNodeValue, interop__);
}
} finally {
encapsulating_.set(prev_);
}
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111110111) == 0 /* only-active SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] */ && ((state_0 & 0b11111111111) != 0 /* is-not SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] && SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] && SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] && SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] && SpecializationActive[InstanceofNode.doLongTarget(Object, long)] && SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] && SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] && SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] && SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */)) {
return execute_double0(state_0, frameValue);
} else if ((state_0 & 0b11111011111) == 0 /* only-active SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] */ && ((state_0 & 0b11111111111) != 0 /* is-not SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] && SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] && SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] && SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] && SpecializationActive[InstanceofNode.doLongTarget(Object, long)] && SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] && SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] && SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] && SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */)) {
return execute_boolean1(state_0, frameValue);
} else {
return execute_generic2(state_0, frameValue);
}
}
private Object execute_double0(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object leftNodeValue_ = super.leftNode.execute(frameValue);
int rightNodeValue_int = 0;
double rightNodeValue_;
try {
if ((state_0 & 0b11000000000000) == 0 /* only-active ImplicitCast[type=double, index=1] */ && ((state_0 & 0b11111111111) != 0 /* is-not SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] && SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] && SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] && SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] && SpecializationActive[InstanceofNode.doLongTarget(Object, long)] && SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] && SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] && SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] && SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */)) {
rightNodeValue_ = super.rightNode.executeDouble(frameValue);
} else if ((state_0 & 0b10100000000000) == 0 /* only-active ImplicitCast[type=double, index=1] */ && ((state_0 & 0b11111111111) != 0 /* is-not SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] && SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] && SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] && SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] && SpecializationActive[InstanceofNode.doLongTarget(Object, long)] && SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] && SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] && SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] && SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */)) {
rightNodeValue_int = super.rightNode.executeInt(frameValue);
rightNodeValue_ = JSTypes.intToDouble(rightNodeValue_int);
} else {
Object rightNodeValue__ = super.rightNode.execute(frameValue);
rightNodeValue_ = JSTypesGen.expectImplicitDouble((state_0 & 0b11100000000000) >>> 11 /* get-int ImplicitCast[type=double, index=1] */, rightNodeValue__);
}
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, ex.getResult());
}
assert (state_0 & 0b1000) != 0 /* is SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] */;
return doDoubleTarget(leftNodeValue_, rightNodeValue_);
}
private Object execute_boolean1(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object leftNodeValue_ = super.leftNode.execute(frameValue);
boolean rightNodeValue_;
try {
rightNodeValue_ = super.rightNode.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, ex.getResult());
}
assert (state_0 & 0b100000) != 0 /* is SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] */;
return doBooleanTarget(leftNodeValue_, rightNodeValue_);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object foreignTargetOther1Boundary0(int state_0, Object leftNodeValue_, Object rightNodeValue_) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(rightNodeValue_));
return doForeignTargetOther(leftNodeValue_, rightNodeValue_, interop__);
}
} finally {
encapsulating_.set(prev_);
}
}
@ExplodeLoop
private Object execute_generic2(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object leftNodeValue_ = super.leftNode.execute(frameValue);
Object rightNodeValue_ = super.rightNode.execute(frameValue);
if ((state_0 & 0b11111111111) != 0 /* is SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] || SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] || SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] || SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] || SpecializationActive[InstanceofNode.doLongTarget(Object, long)] || SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] || SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] || SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] || SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((state_0 & 0b11111111) != 0 /* is SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] || SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] || SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] || SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] || SpecializationActive[InstanceofNode.doLongTarget(Object, long)] || SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] || SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] || SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] */ && rightNodeValue_ instanceof JSDynamicObject) {
JSDynamicObject rightNodeValue__ = (JSDynamicObject) rightNodeValue_;
JSObjectData s0_ = this.jSObject_cache;
if (s0_ != null) {
if ((s0_.isObjectNode_.executeBoolean(rightNodeValue__))) {
Node node__ = (s0_);
return doJSObject(leftNodeValue_, rightNodeValue__, node__, s0_.isObjectNode_, s0_.getMethodHasInstanceNode_, INLINED_J_S_OBJECT_TO_BOOLEAN_NODE_, s0_.callHasInstanceNode_, s0_.isCallableNode_, INLINED_J_S_OBJECT_HAS_INSTANCE_PROFILE_, INLINED_J_S_OBJECT_ERROR_BRANCH_);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] */) {
if ((JSGuards.isNullOrUndefined(rightNodeValue_))) {
return doNullOrUndefinedTarget(leftNodeValue_, rightNodeValue_);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] */ && rightNodeValue_ instanceof TruffleString) {
TruffleString rightNodeValue__ = (TruffleString) rightNodeValue_;
return doStringTarget(leftNodeValue_, rightNodeValue__);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b11100000000000) >>> 11 /* get-int ImplicitCast[type=double, index=1] */, rightNodeValue_)) {
double rightNodeValue__ = JSTypesGen.asImplicitDouble((state_0 & 0b11100000000000) >>> 11 /* get-int ImplicitCast[type=double, index=1] */, rightNodeValue_);
return doDoubleTarget(leftNodeValue_, rightNodeValue__);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[InstanceofNode.doLongTarget(Object, long)] */ && rightNodeValue_ instanceof Long) {
long rightNodeValue__ = (long) rightNodeValue_;
return doLongTarget(leftNodeValue_, rightNodeValue__);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] */ && rightNodeValue_ instanceof Boolean) {
boolean rightNodeValue__ = (boolean) rightNodeValue_;
return doBooleanTarget(leftNodeValue_, rightNodeValue__);
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] */ && rightNodeValue_ instanceof BigInt) {
BigInt rightNodeValue__ = (BigInt) rightNodeValue_;
return doBigIntTarget(leftNodeValue_, rightNodeValue__);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] */ && rightNodeValue_ instanceof Symbol) {
Symbol rightNodeValue__ = (Symbol) rightNodeValue_;
return doSymbolTarget(leftNodeValue_, rightNodeValue__);
}
}
if ((state_0 & 0b11100000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] */ && leftNodeValue_ instanceof JSDynamicObject) {
JSDynamicObject leftNodeValue__ = (JSDynamicObject) leftNodeValue_;
if ((JSGuards.isForeignObject(rightNodeValue_))) {
return doForeignTargetJSType(leftNodeValue__, rightNodeValue_);
}
}
if ((state_0 & 0b11000000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
ForeignTargetOther0Data s9_ = this.foreignTargetOther0_cache;
while (s9_ != null) {
if ((s9_.interop_.accepts(rightNodeValue_)) && (JSGuards.isForeignObject(rightNodeValue_)) && (!(JSGuards.isJSDynamicObject(leftNodeValue_)))) {
return doForeignTargetOther(leftNodeValue_, rightNodeValue_, s9_.interop_);
}
s9_ = s9_.next_;
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((JSGuards.isForeignObject(rightNodeValue_)) && (!(JSGuards.isJSDynamicObject(leftNodeValue_)))) {
return this.foreignTargetOther1Boundary0(state_0, leftNodeValue_, rightNodeValue_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, rightNodeValue_);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111110111) == 0 /* only-active SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] */ && ((state_0 & 0b11111111111) != 0 /* is-not SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] && SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] && SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] && SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] && SpecializationActive[InstanceofNode.doLongTarget(Object, long)] && SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] && SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] && SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] && SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */)) {
return executeBoolean_double3(state_0, frameValue);
} else if ((state_0 & 0b11111011111) == 0 /* only-active SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] */ && ((state_0 & 0b11111111111) != 0 /* is-not SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] && SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] && SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] && SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] && SpecializationActive[InstanceofNode.doLongTarget(Object, long)] && SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] && SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] && SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] && SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */)) {
return executeBoolean_boolean4(state_0, frameValue);
} else {
return executeBoolean_generic5(state_0, frameValue);
}
}
private boolean executeBoolean_double3(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object leftNodeValue_ = super.leftNode.execute(frameValue);
int rightNodeValue_int = 0;
double rightNodeValue_;
try {
if ((state_0 & 0b11000000000000) == 0 /* only-active ImplicitCast[type=double, index=1] */ && ((state_0 & 0b11111111111) != 0 /* is-not SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] && SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] && SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] && SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] && SpecializationActive[InstanceofNode.doLongTarget(Object, long)] && SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] && SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] && SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] && SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */)) {
rightNodeValue_ = super.rightNode.executeDouble(frameValue);
} else if ((state_0 & 0b10100000000000) == 0 /* only-active ImplicitCast[type=double, index=1] */ && ((state_0 & 0b11111111111) != 0 /* is-not SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] && SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] && SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] && SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] && SpecializationActive[InstanceofNode.doLongTarget(Object, long)] && SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] && SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] && SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] && SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] && SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */)) {
rightNodeValue_int = super.rightNode.executeInt(frameValue);
rightNodeValue_ = JSTypes.intToDouble(rightNodeValue_int);
} else {
Object rightNodeValue__ = super.rightNode.execute(frameValue);
rightNodeValue_ = JSTypesGen.expectImplicitDouble((state_0 & 0b11100000000000) >>> 11 /* get-int ImplicitCast[type=double, index=1] */, rightNodeValue__);
}
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, ex.getResult());
}
assert (state_0 & 0b1000) != 0 /* is SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] */;
return doDoubleTarget(leftNodeValue_, rightNodeValue_);
}
private boolean executeBoolean_boolean4(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object leftNodeValue_ = super.leftNode.execute(frameValue);
boolean rightNodeValue_;
try {
rightNodeValue_ = super.rightNode.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, ex.getResult());
}
assert (state_0 & 0b100000) != 0 /* is SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] */;
return doBooleanTarget(leftNodeValue_, rightNodeValue_);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private boolean foreignTargetOther1Boundary1(int state_0, Object leftNodeValue_, Object rightNodeValue_) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(rightNodeValue_));
return doForeignTargetOther(leftNodeValue_, rightNodeValue_, interop__);
}
} finally {
encapsulating_.set(prev_);
}
}
@ExplodeLoop
private boolean executeBoolean_generic5(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object leftNodeValue_ = super.leftNode.execute(frameValue);
Object rightNodeValue_ = super.rightNode.execute(frameValue);
if ((state_0 & 0b11111111111) != 0 /* is SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] || SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] || SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] || SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] || SpecializationActive[InstanceofNode.doLongTarget(Object, long)] || SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] || SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] || SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] || SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((state_0 & 0b11111111) != 0 /* is SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] || SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] || SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] || SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] || SpecializationActive[InstanceofNode.doLongTarget(Object, long)] || SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] || SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] || SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] */ && rightNodeValue_ instanceof JSDynamicObject) {
JSDynamicObject rightNodeValue__ = (JSDynamicObject) rightNodeValue_;
JSObjectData s0_ = this.jSObject_cache;
if (s0_ != null) {
if ((s0_.isObjectNode_.executeBoolean(rightNodeValue__))) {
Node node__ = (s0_);
return doJSObject(leftNodeValue_, rightNodeValue__, node__, s0_.isObjectNode_, s0_.getMethodHasInstanceNode_, INLINED_J_S_OBJECT_TO_BOOLEAN_NODE_, s0_.callHasInstanceNode_, s0_.isCallableNode_, INLINED_J_S_OBJECT_HAS_INSTANCE_PROFILE_, INLINED_J_S_OBJECT_ERROR_BRANCH_);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] */) {
if ((JSGuards.isNullOrUndefined(rightNodeValue_))) {
return doNullOrUndefinedTarget(leftNodeValue_, rightNodeValue_);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] */ && rightNodeValue_ instanceof TruffleString) {
TruffleString rightNodeValue__ = (TruffleString) rightNodeValue_;
return doStringTarget(leftNodeValue_, rightNodeValue__);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b11100000000000) >>> 11 /* get-int ImplicitCast[type=double, index=1] */, rightNodeValue_)) {
double rightNodeValue__ = JSTypesGen.asImplicitDouble((state_0 & 0b11100000000000) >>> 11 /* get-int ImplicitCast[type=double, index=1] */, rightNodeValue_);
return doDoubleTarget(leftNodeValue_, rightNodeValue__);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[InstanceofNode.doLongTarget(Object, long)] */ && rightNodeValue_ instanceof Long) {
long rightNodeValue__ = (long) rightNodeValue_;
return doLongTarget(leftNodeValue_, rightNodeValue__);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] */ && rightNodeValue_ instanceof Boolean) {
boolean rightNodeValue__ = (boolean) rightNodeValue_;
return doBooleanTarget(leftNodeValue_, rightNodeValue__);
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] */ && rightNodeValue_ instanceof BigInt) {
BigInt rightNodeValue__ = (BigInt) rightNodeValue_;
return doBigIntTarget(leftNodeValue_, rightNodeValue__);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] */ && rightNodeValue_ instanceof Symbol) {
Symbol rightNodeValue__ = (Symbol) rightNodeValue_;
return doSymbolTarget(leftNodeValue_, rightNodeValue__);
}
}
if ((state_0 & 0b11100000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] */ && leftNodeValue_ instanceof JSDynamicObject) {
JSDynamicObject leftNodeValue__ = (JSDynamicObject) leftNodeValue_;
if ((JSGuards.isForeignObject(rightNodeValue_))) {
return doForeignTargetJSType(leftNodeValue__, rightNodeValue_);
}
}
if ((state_0 & 0b11000000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] || SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
ForeignTargetOther0Data s9_ = this.foreignTargetOther0_cache;
while (s9_ != null) {
if ((s9_.interop_.accepts(rightNodeValue_)) && (JSGuards.isForeignObject(rightNodeValue_)) && (!(JSGuards.isJSDynamicObject(leftNodeValue_)))) {
return doForeignTargetOther(leftNodeValue_, rightNodeValue_, s9_.interop_);
}
s9_ = s9_.next_;
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
if ((JSGuards.isForeignObject(rightNodeValue_)) && (!(JSGuards.isJSDynamicObject(leftNodeValue_)))) {
return this.foreignTargetOther1Boundary1(state_0, leftNodeValue_, rightNodeValue_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, rightNodeValue_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeBoolean(frameValue);
return;
}
@SuppressWarnings("unused")
private boolean executeAndSpecialize(Object leftNodeValue, Object rightNodeValue) {
int state_0 = this.state_0_;
{
Node node__ = null;
if (rightNodeValue instanceof JSDynamicObject) {
JSDynamicObject rightNodeValue_ = (JSDynamicObject) rightNodeValue;
while (true) {
int count0_ = 0;
JSObjectData s0_ = J_S_OBJECT_CACHE_UPDATER.getVolatile(this);
JSObjectData s0_original = s0_;
while (s0_ != null) {
if ((s0_.isObjectNode_.executeBoolean(rightNodeValue_))) {
node__ = (s0_);
break;
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
IsJSObjectNode isObjectNode__ = this.insert((IsJSObjectNode.create()));
if ((isObjectNode__.executeBoolean(rightNodeValue_))) {
s0_ = this.insert(new JSObjectData());
node__ = (s0_);
Objects.requireNonNull(s0_.insert(isObjectNode__), "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s0_.isObjectNode_ = isObjectNode__;
GetMethodNode getMethodHasInstanceNode__ = s0_.insert((createGetMethodHasInstance()));
Objects.requireNonNull(getMethodHasInstanceNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s0_.getMethodHasInstanceNode_ = getMethodHasInstanceNode__;
JSFunctionCallNode callHasInstanceNode__ = s0_.insert((JSFunctionCallNode.createCall()));
Objects.requireNonNull(callHasInstanceNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s0_.callHasInstanceNode_ = callHasInstanceNode__;
IsCallableNode isCallableNode__ = s0_.insert((IsCallableNode.create()));
Objects.requireNonNull(isCallableNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s0_.isCallableNode_ = isCallableNode__;
if (!J_S_OBJECT_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[InstanceofNode.doJSObject(Object, JSDynamicObject, Node, IsJSObjectNode, GetMethodNode, JSToBooleanNode, JSFunctionCallNode, IsCallableNode, InlinedConditionProfile, InlinedBranchProfile)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return doJSObject(leftNodeValue, rightNodeValue_, node__, s0_.isObjectNode_, s0_.getMethodHasInstanceNode_, INLINED_J_S_OBJECT_TO_BOOLEAN_NODE_, s0_.callHasInstanceNode_, s0_.isCallableNode_, INLINED_J_S_OBJECT_HAS_INSTANCE_PROFILE_, INLINED_J_S_OBJECT_ERROR_BRANCH_);
}
break;
}
}
}
if ((JSGuards.isNullOrUndefined(rightNodeValue))) {
state_0 = state_0 | 0b10 /* add SpecializationActive[InstanceofNode.doNullOrUndefinedTarget(Object, Object)] */;
this.state_0_ = state_0;
return doNullOrUndefinedTarget(leftNodeValue, rightNodeValue);
}
if (rightNodeValue instanceof TruffleString) {
TruffleString rightNodeValue_ = (TruffleString) rightNodeValue;
state_0 = state_0 | 0b100 /* add SpecializationActive[InstanceofNode.doStringTarget(Object, TruffleString)] */;
this.state_0_ = state_0;
return doStringTarget(leftNodeValue, rightNodeValue_);
}
{
int doubleCast1;
if ((doubleCast1 = JSTypesGen.specializeImplicitDouble(rightNodeValue)) != 0) {
double rightNodeValue_ = JSTypesGen.asImplicitDouble(doubleCast1, rightNodeValue);
state_0 = (state_0 | (doubleCast1 << 11) /* set-int ImplicitCast[type=double, index=1] */);
state_0 = state_0 | 0b1000 /* add SpecializationActive[InstanceofNode.doDoubleTarget(Object, double)] */;
this.state_0_ = state_0;
return doDoubleTarget(leftNodeValue, rightNodeValue_);
}
}
if (rightNodeValue instanceof Long) {
long rightNodeValue_ = (long) rightNodeValue;
state_0 = state_0 | 0b10000 /* add SpecializationActive[InstanceofNode.doLongTarget(Object, long)] */;
this.state_0_ = state_0;
return doLongTarget(leftNodeValue, rightNodeValue_);
}
if (rightNodeValue instanceof Boolean) {
boolean rightNodeValue_ = (boolean) rightNodeValue;
state_0 = state_0 | 0b100000 /* add SpecializationActive[InstanceofNode.doBooleanTarget(Object, boolean)] */;
this.state_0_ = state_0;
return doBooleanTarget(leftNodeValue, rightNodeValue_);
}
if (rightNodeValue instanceof BigInt) {
BigInt rightNodeValue_ = (BigInt) rightNodeValue;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[InstanceofNode.doBigIntTarget(Object, BigInt)] */;
this.state_0_ = state_0;
return doBigIntTarget(leftNodeValue, rightNodeValue_);
}
if (rightNodeValue instanceof Symbol) {
Symbol rightNodeValue_ = (Symbol) rightNodeValue;
state_0 = state_0 | 0b10000000 /* add SpecializationActive[InstanceofNode.doSymbolTarget(Object, Symbol)] */;
this.state_0_ = state_0;
return doSymbolTarget(leftNodeValue, rightNodeValue_);
}
if (leftNodeValue instanceof JSDynamicObject) {
JSDynamicObject leftNodeValue_ = (JSDynamicObject) leftNodeValue;
if ((JSGuards.isForeignObject(rightNodeValue))) {
state_0 = state_0 | 0b100000000 /* add SpecializationActive[InstanceofNode.doForeignTargetJSType(JSDynamicObject, Object)] */;
this.state_0_ = state_0;
return doForeignTargetJSType(leftNodeValue_, rightNodeValue);
}
}
if (((state_0 & 0b10000000000)) == 0 /* is-not SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */) {
while (true) {
int count9_ = 0;
ForeignTargetOther0Data s9_ = FOREIGN_TARGET_OTHER0_CACHE_UPDATER.getVolatile(this);
ForeignTargetOther0Data s9_original = s9_;
while (s9_ != null) {
if ((s9_.interop_.accepts(rightNodeValue)) && (JSGuards.isForeignObject(rightNodeValue)) && (!(JSGuards.isJSDynamicObject(leftNodeValue)))) {
break;
}
count9_++;
s9_ = s9_.next_;
}
if (s9_ == null) {
if ((JSGuards.isForeignObject(rightNodeValue)) && (!(JSGuards.isJSDynamicObject(leftNodeValue))) && count9_ < (JSConfig.InteropLibraryLimit)) {
// assert (s9_.interop_.accepts(rightNodeValue));
s9_ = this.insert(new ForeignTargetOther0Data(s9_original));
InteropLibrary interop__ = s9_.insert((INTEROP_LIBRARY_.create(rightNodeValue)));
Objects.requireNonNull(interop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s9_.interop_ = interop__;
if (!FOREIGN_TARGET_OTHER0_CACHE_UPDATER.compareAndSet(this, s9_original, s9_)) {
continue;
}
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */;
this.state_0_ = state_0;
}
}
if (s9_ != null) {
return doForeignTargetOther(leftNodeValue, rightNodeValue, s9_.interop_);
}
break;
}
}
{
InteropLibrary interop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
if ((JSGuards.isForeignObject(rightNodeValue)) && (!(JSGuards.isJSDynamicObject(leftNodeValue)))) {
interop__ = (INTEROP_LIBRARY_.getUncached(rightNodeValue));
this.foreignTargetOther0_cache = null;
state_0 = state_0 & 0xfffffdff /* remove SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */;
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[InstanceofNode.doForeignTargetOther(Object, Object, InteropLibrary)] */;
this.state_0_ = state_0;
return doForeignTargetOther(leftNodeValue, rightNodeValue, interop__);
}
} finally {
encapsulating_.set(prev_);
}
}
}
throw new UnsupportedSpecializationException(this, new Node[] {super.leftNode, super.rightNode}, leftNodeValue, rightNodeValue);
}
@NeverDefault
public static InstanceofNode create(JSContext context, JavaScriptNode left, JavaScriptNode right) {
return new InstanceofNodeGen(context, left, right);
}
@GeneratedBy(InstanceofNode.class)
@DenyReplace
private static final class JSObjectData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-14: InlinedCache
* Specialization: {@link InstanceofNode#doJSObject}
* Parameter: {@link JSToBooleanNode} toBooleanNode
* Inline method: {@link JSToBooleanNodeGen#inline}
* 15-16: InlinedCache
* Specialization: {@link InstanceofNode#doJSObject}
* Parameter: {@link InlinedConditionProfile} hasInstanceProfile
* Inline method: {@link InlinedConditionProfile#inline}
* 17: InlinedCache
* Specialization: {@link InstanceofNode#doJSObject}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int jSObject_state_0_;
/**
* Source Info:
* Specialization: {@link InstanceofNode#doJSObject}
* Parameter: {@link IsJSObjectNode} isObjectNode
*/
@Child IsJSObjectNode isObjectNode_;
/**
* Source Info:
* Specialization: {@link InstanceofNode#doJSObject}
* Parameter: {@link GetMethodNode} getMethodHasInstanceNode
*/
@Child GetMethodNode getMethodHasInstanceNode_;
/**
* Source Info:
* Specialization: {@link InstanceofNode#doJSObject}
* Parameter: {@link JSToBooleanNode} toBooleanNode
* Inline method: {@link JSToBooleanNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node jSObject_toBooleanNode__field1_;
/**
* Source Info:
* Specialization: {@link InstanceofNode#doJSObject}
* Parameter: {@link JSFunctionCallNode} callHasInstanceNode
*/
@Child JSFunctionCallNode callHasInstanceNode_;
/**
* Source Info:
* Specialization: {@link InstanceofNode#doJSObject}
* Parameter: {@link IsCallableNode} isCallableNode
*/
@Child IsCallableNode isCallableNode_;
JSObjectData() {
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(InstanceofNode.class)
@DenyReplace
private static final class ForeignTargetOther0Data extends Node implements SpecializationDataNode {
@Child ForeignTargetOther0Data next_;
/**
* Source Info:
* Specialization: {@link InstanceofNode#doForeignTargetOther}
* Parameter: {@link InteropLibrary} interop
*/
@Child InteropLibrary interop_;
ForeignTargetOther0Data(ForeignTargetOther0Data next_) {
this.next_ = next_;
}
}
/**
* Debug Info:
* Specialization {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Activation probability: 0.19111
* With/without class size: 7/0 bytes
* Specialization {@link OrdinaryHasInstanceNode#doJSObjectProxy}
* Activation probability: 0.17111
* With/without class size: 6/0 bytes
* Specialization {@link OrdinaryHasInstanceNode#doBound}
* Activation probability: 0.15111
* With/without class size: 7/4 bytes
* Specialization {@link OrdinaryHasInstanceNode#doForeignObjectUnbound}
* Activation probability: 0.13111
* With/without class size: 6/0 bytes
* Specialization {@link OrdinaryHasInstanceNode#doForeignObjectProxy}
* Activation probability: 0.11111
* With/without class size: 5/0 bytes
* Specialization {@link OrdinaryHasInstanceNode#doNotAnObjectUnbound}
* Activation probability: 0.09111
* With/without class size: 5/0 bytes
* Specialization {@link OrdinaryHasInstanceNode#doNotAnObjectProxy}
* Activation probability: 0.07111
* With/without class size: 4/0 bytes
* Specialization {@link OrdinaryHasInstanceNode#doNotCallable}
* Activation probability: 0.05111
* With/without class size: 4/0 bytes
* Specialization {@link OrdinaryHasInstanceNode#doForeignTarget}
* Activation probability: 0.03111
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(OrdinaryHasInstanceNode.class)
@SuppressWarnings("javadoc")
public static final class OrdinaryHasInstanceNodeGen extends OrdinaryHasInstanceNode {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} firstTrue
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_FIRST_TRUE = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(9, 1)));
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} firstFalse
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_FIRST_FALSE = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(10, 1)));
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} need2Hops
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_NEED2_HOPS = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(11, 1)));
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} need3Hops
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_NEED3_HOPS = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(12, 1)));
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_ERROR_BRANCH = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(13, 1)));
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} invalidPrototypeBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_INVALID_PROTOTYPE_BRANCH = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(14, 1)));
/**
* State Info:
* 0: SpecializationActive {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* 1: SpecializationActive {@link OrdinaryHasInstanceNode#doJSObjectProxy}
* 2: SpecializationActive {@link OrdinaryHasInstanceNode#doBound}
* 3: SpecializationActive {@link OrdinaryHasInstanceNode#doForeignObjectUnbound}
* 4: SpecializationActive {@link OrdinaryHasInstanceNode#doForeignObjectProxy}
* 5: SpecializationActive {@link OrdinaryHasInstanceNode#doNotAnObjectUnbound}
* 6: SpecializationActive {@link OrdinaryHasInstanceNode#doNotAnObjectProxy}
* 7: SpecializationActive {@link OrdinaryHasInstanceNode#doNotCallable}
* 8: SpecializationActive {@link OrdinaryHasInstanceNode#doForeignTarget}
* 9: InlinedCache
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} firstTrue
* Inline method: {@link InlinedBranchProfile#inline}
* 10: InlinedCache
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} firstFalse
* Inline method: {@link InlinedBranchProfile#inline}
* 11: InlinedCache
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} need2Hops
* Inline method: {@link InlinedBranchProfile#inline}
* 12: InlinedCache
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} need3Hops
* Inline method: {@link InlinedBranchProfile#inline}
* 13: InlinedCache
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
* 14: InlinedCache
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link InlinedBranchProfile} invalidPrototypeBranch
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link IsJSObjectNode} isObjectNode
*/
@Child private IsJSObjectNode isObjectNode;
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link GetPrototypeNode} getPrototype1Node
*/
@Child private GetPrototypeNode getPrototype1Node;
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link GetPrototypeNode} getPrototype2Node
*/
@Child private GetPrototypeNode getPrototype2Node;
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doJSObjectFunction}
* Parameter: {@link GetPrototypeNode} getPrototype3Node
*/
@Child private GetPrototypeNode getPrototype3Node;
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doForeignObjectUnbound}
* Parameter: {@link IsObjectNode} isAnyObjectNode
*/
@Child private IsObjectNode isAnyObjectNode;
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doForeignObjectUnbound}
* Parameter: {@link ForeignObjectPrototypeNode} getForeignPrototypeNode
*/
@Child private ForeignObjectPrototypeNode getForeignPrototypeNode;
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doForeignObjectUnbound}
* Parameter: {@link OrdinaryHasInstanceNode} ordinaryHasInstanceNode
*/
@Child private OrdinaryHasInstanceNode ordinaryHasInstanceNode;
/**
* Source Info:
* Specialization: {@link OrdinaryHasInstanceNode#doBound}
* Parameter: {@link InstanceofNode} instanceofNode
*/
@Child private InstanceofNode bound_instanceofNode_;
private OrdinaryHasInstanceNodeGen(JSContext context) {
super(context);
}
@Override
public boolean executeBoolean(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111111111) != 0 /* is SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doJSObjectFunction(Object, JSFunctionObject, IsJSObjectNode, GetPrototypeNode, GetPrototypeNode, GetPrototypeNode, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] || SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doJSObjectProxy(Object, JSProxyObject, IsJSObjectNode, GetPrototypeNode, GetPrototypeNode, GetPrototypeNode, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] || SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doBound(Object, Bound, InstanceofNode)] || SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doForeignObjectUnbound(Object, JSFunctionObject, IsObjectNode, ForeignObjectPrototypeNode, InlinedBranchProfile, OrdinaryHasInstanceNode)] || SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doForeignObjectProxy(Object, JSProxyObject, IsObjectNode, ForeignObjectPrototypeNode, InlinedBranchProfile, OrdinaryHasInstanceNode)] || SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doNotAnObjectUnbound(Object, JSFunctionObject)] || SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doNotAnObjectProxy(Object, JSProxyObject)] || SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doNotCallable(Object, Object)] || SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doForeignTarget(Object, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doJSObjectFunction(Object, JSFunctionObject, IsJSObjectNode, GetPrototypeNode, GetPrototypeNode, GetPrototypeNode, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */ && arg1Value instanceof JSFunctionObject) {
JSFunctionObject arg1Value_ = (JSFunctionObject) arg1Value;
{
IsJSObjectNode isObjectNode_ = this.isObjectNode;
if (isObjectNode_ != null) {
GetPrototypeNode getPrototype1Node_ = this.getPrototype1Node;
if (getPrototype1Node_ != null) {
GetPrototypeNode getPrototype2Node_ = this.getPrototype2Node;
if (getPrototype2Node_ != null) {
GetPrototypeNode getPrototype3Node_ = this.getPrototype3Node;
if (getPrototype3Node_ != null) {
if ((isObjectNode_.executeBoolean(arg0Value)) && (!(JSFunction.isBoundFunction(arg1Value_)))) {
return doJSObjectFunction(arg0Value, arg1Value_, isObjectNode_, getPrototype1Node_, getPrototype2Node_, getPrototype3Node_, INLINED_FIRST_TRUE, INLINED_FIRST_FALSE, INLINED_NEED2_HOPS, INLINED_NEED3_HOPS, INLINED_ERROR_BRANCH, INLINED_INVALID_PROTOTYPE_BRANCH);
}
}
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doJSObjectProxy(Object, JSProxyObject, IsJSObjectNode, GetPrototypeNode, GetPrototypeNode, GetPrototypeNode, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */ && arg1Value instanceof JSProxyObject) {
JSProxyObject arg1Value_ = (JSProxyObject) arg1Value;
{
IsJSObjectNode isObjectNode_1 = this.isObjectNode;
if (isObjectNode_1 != null) {
GetPrototypeNode getPrototype1Node_1 = this.getPrototype1Node;
if (getPrototype1Node_1 != null) {
GetPrototypeNode getPrototype2Node_1 = this.getPrototype2Node;
if (getPrototype2Node_1 != null) {
GetPrototypeNode getPrototype3Node_1 = this.getPrototype3Node;
if (getPrototype3Node_1 != null) {
if ((isObjectNode_1.executeBoolean(arg0Value)) && (JSGuards.isCallableProxy(arg1Value_))) {
return doJSObjectProxy(arg0Value, arg1Value_, isObjectNode_1, getPrototype1Node_1, getPrototype2Node_1, getPrototype3Node_1, INLINED_FIRST_TRUE, INLINED_FIRST_FALSE, INLINED_NEED2_HOPS, INLINED_NEED3_HOPS, INLINED_ERROR_BRANCH, INLINED_INVALID_PROTOTYPE_BRANCH);
}
}
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doBound(Object, Bound, InstanceofNode)] */ && arg1Value instanceof Bound) {
Bound arg1Value_ = (Bound) arg1Value;
{
InstanceofNode instanceofNode__ = this.bound_instanceofNode_;
if (instanceofNode__ != null) {
return doBound(arg0Value, arg1Value_, instanceofNode__);
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doForeignObjectUnbound(Object, JSFunctionObject, IsObjectNode, ForeignObjectPrototypeNode, InlinedBranchProfile, OrdinaryHasInstanceNode)] */ && arg1Value instanceof JSFunctionObject) {
JSFunctionObject arg1Value_ = (JSFunctionObject) arg1Value;
{
IsObjectNode isAnyObjectNode_ = this.isAnyObjectNode;
if (isAnyObjectNode_ != null) {
ForeignObjectPrototypeNode getForeignPrototypeNode_ = this.getForeignPrototypeNode;
if (getForeignPrototypeNode_ != null) {
OrdinaryHasInstanceNode ordinaryHasInstanceNode_ = this.ordinaryHasInstanceNode;
if (ordinaryHasInstanceNode_ != null) {
if ((!(JSGuards.isJSObject(arg0Value))) && (JSGuards.isForeignObject(arg0Value)) && (!(JSFunction.isBoundFunction(arg1Value_)))) {
return doForeignObjectUnbound(arg0Value, arg1Value_, isAnyObjectNode_, getForeignPrototypeNode_, INLINED_INVALID_PROTOTYPE_BRANCH, ordinaryHasInstanceNode_);
}
}
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doForeignObjectProxy(Object, JSProxyObject, IsObjectNode, ForeignObjectPrototypeNode, InlinedBranchProfile, OrdinaryHasInstanceNode)] */ && arg1Value instanceof JSProxyObject) {
JSProxyObject arg1Value_ = (JSProxyObject) arg1Value;
{
IsObjectNode isAnyObjectNode_1 = this.isAnyObjectNode;
if (isAnyObjectNode_1 != null) {
ForeignObjectPrototypeNode getForeignPrototypeNode_1 = this.getForeignPrototypeNode;
if (getForeignPrototypeNode_1 != null) {
OrdinaryHasInstanceNode ordinaryHasInstanceNode_1 = this.ordinaryHasInstanceNode;
if (ordinaryHasInstanceNode_1 != null) {
if ((!(JSGuards.isJSObject(arg0Value))) && (JSGuards.isForeignObject(arg0Value)) && (JSGuards.isCallableProxy(arg1Value_))) {
return doForeignObjectProxy(arg0Value, arg1Value_, isAnyObjectNode_1, getForeignPrototypeNode_1, INLINED_INVALID_PROTOTYPE_BRANCH, ordinaryHasInstanceNode_1);
}
}
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doNotAnObjectUnbound(Object, JSFunctionObject)] */ && arg1Value instanceof JSFunctionObject) {
JSFunctionObject arg1Value_ = (JSFunctionObject) arg1Value;
if ((!(JSGuards.isJSObject(arg0Value))) && (!(JSGuards.isForeignObject(arg0Value))) && (!(JSFunction.isBoundFunction(arg1Value_)))) {
return OrdinaryHasInstanceNode.doNotAnObjectUnbound(arg0Value, arg1Value_);
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doNotAnObjectProxy(Object, JSProxyObject)] */ && arg1Value instanceof JSProxyObject) {
JSProxyObject arg1Value_ = (JSProxyObject) arg1Value;
if ((!(JSGuards.isJSObject(arg0Value))) && (!(JSGuards.isForeignObject(arg0Value))) && (JSGuards.isCallableProxy(arg1Value_))) {
return OrdinaryHasInstanceNode.doNotAnObjectProxy(arg0Value, arg1Value_);
}
}
if ((state_0 & 0b110000000) != 0 /* is SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doNotCallable(Object, Object)] || SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doForeignTarget(Object, Object)] */) {
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doNotCallable(Object, Object)] */) {
if ((!(isCallableNode.executeBoolean(arg1Value)))) {
return OrdinaryHasInstanceNode.doNotCallable(arg0Value, arg1Value);
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doForeignTarget(Object, Object)] */) {
if ((JSGuards.isForeignObject(arg1Value))) {
return OrdinaryHasInstanceNode.doForeignTarget(arg0Value, arg1Value);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private boolean executeAndSpecialize(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof JSFunctionObject) {
JSFunctionObject arg1Value_ = (JSFunctionObject) arg1Value;
{
IsJSObjectNode isObjectNode_;
IsJSObjectNode isObjectNode__shared = this.isObjectNode;
if (isObjectNode__shared != null) {
isObjectNode_ = isObjectNode__shared;
} else {
isObjectNode_ = this.insert((IsJSObjectNode.create()));
if (isObjectNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((isObjectNode_.executeBoolean(arg0Value)) && (!(JSFunction.isBoundFunction(arg1Value_)))) {
if (this.isObjectNode == null) {
VarHandle.storeStoreFence();
this.isObjectNode = isObjectNode_;
}
GetPrototypeNode getPrototype1Node_;
GetPrototypeNode getPrototype1Node__shared = this.getPrototype1Node;
if (getPrototype1Node__shared != null) {
getPrototype1Node_ = getPrototype1Node__shared;
} else {
getPrototype1Node_ = this.insert((GetPrototypeNode.create()));
if (getPrototype1Node_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.getPrototype1Node == null) {
VarHandle.storeStoreFence();
this.getPrototype1Node = getPrototype1Node_;
}
GetPrototypeNode getPrototype2Node_;
GetPrototypeNode getPrototype2Node__shared = this.getPrototype2Node;
if (getPrototype2Node__shared != null) {
getPrototype2Node_ = getPrototype2Node__shared;
} else {
getPrototype2Node_ = this.insert((GetPrototypeNode.create()));
if (getPrototype2Node_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.getPrototype2Node == null) {
VarHandle.storeStoreFence();
this.getPrototype2Node = getPrototype2Node_;
}
GetPrototypeNode getPrototype3Node_;
GetPrototypeNode getPrototype3Node__shared = this.getPrototype3Node;
if (getPrototype3Node__shared != null) {
getPrototype3Node_ = getPrototype3Node__shared;
} else {
getPrototype3Node_ = this.insert((GetPrototypeNode.create()));
if (getPrototype3Node_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.getPrototype3Node == null) {
VarHandle.storeStoreFence();
this.getPrototype3Node = getPrototype3Node_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doJSObjectFunction(Object, JSFunctionObject, IsJSObjectNode, GetPrototypeNode, GetPrototypeNode, GetPrototypeNode, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */;
this.state_0_ = state_0;
return doJSObjectFunction(arg0Value, arg1Value_, isObjectNode_, getPrototype1Node_, getPrototype2Node_, getPrototype3Node_, INLINED_FIRST_TRUE, INLINED_FIRST_FALSE, INLINED_NEED2_HOPS, INLINED_NEED3_HOPS, INLINED_ERROR_BRANCH, INLINED_INVALID_PROTOTYPE_BRANCH);
}
}
}
if (arg1Value instanceof JSProxyObject) {
JSProxyObject arg1Value_ = (JSProxyObject) arg1Value;
{
IsJSObjectNode isObjectNode_1;
IsJSObjectNode isObjectNode_1_shared = this.isObjectNode;
if (isObjectNode_1_shared != null) {
isObjectNode_1 = isObjectNode_1_shared;
} else {
isObjectNode_1 = this.insert((IsJSObjectNode.create()));
if (isObjectNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((isObjectNode_1.executeBoolean(arg0Value)) && (JSGuards.isCallableProxy(arg1Value_))) {
if (this.isObjectNode == null) {
VarHandle.storeStoreFence();
this.isObjectNode = isObjectNode_1;
}
GetPrototypeNode getPrototype1Node_1;
GetPrototypeNode getPrototype1Node_1_shared = this.getPrototype1Node;
if (getPrototype1Node_1_shared != null) {
getPrototype1Node_1 = getPrototype1Node_1_shared;
} else {
getPrototype1Node_1 = this.insert((GetPrototypeNode.create()));
if (getPrototype1Node_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.getPrototype1Node == null) {
VarHandle.storeStoreFence();
this.getPrototype1Node = getPrototype1Node_1;
}
GetPrototypeNode getPrototype2Node_1;
GetPrototypeNode getPrototype2Node_1_shared = this.getPrototype2Node;
if (getPrototype2Node_1_shared != null) {
getPrototype2Node_1 = getPrototype2Node_1_shared;
} else {
getPrototype2Node_1 = this.insert((GetPrototypeNode.create()));
if (getPrototype2Node_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.getPrototype2Node == null) {
VarHandle.storeStoreFence();
this.getPrototype2Node = getPrototype2Node_1;
}
GetPrototypeNode getPrototype3Node_1;
GetPrototypeNode getPrototype3Node_1_shared = this.getPrototype3Node;
if (getPrototype3Node_1_shared != null) {
getPrototype3Node_1 = getPrototype3Node_1_shared;
} else {
getPrototype3Node_1 = this.insert((GetPrototypeNode.create()));
if (getPrototype3Node_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.getPrototype3Node == null) {
VarHandle.storeStoreFence();
this.getPrototype3Node = getPrototype3Node_1;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doJSObjectProxy(Object, JSProxyObject, IsJSObjectNode, GetPrototypeNode, GetPrototypeNode, GetPrototypeNode, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */;
this.state_0_ = state_0;
return doJSObjectProxy(arg0Value, arg1Value_, isObjectNode_1, getPrototype1Node_1, getPrototype2Node_1, getPrototype3Node_1, INLINED_FIRST_TRUE, INLINED_FIRST_FALSE, INLINED_NEED2_HOPS, INLINED_NEED3_HOPS, INLINED_ERROR_BRANCH, INLINED_INVALID_PROTOTYPE_BRANCH);
}
}
}
if (arg1Value instanceof Bound) {
Bound arg1Value_ = (Bound) arg1Value;
InstanceofNode instanceofNode__ = this.insert((InstanceofNode.create(context)));
Objects.requireNonNull(instanceofNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.bound_instanceofNode_ = instanceofNode__;
state_0 = state_0 | 0b100 /* add SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doBound(Object, Bound, InstanceofNode)] */;
this.state_0_ = state_0;
return doBound(arg0Value, arg1Value_, instanceofNode__);
}
if (arg1Value instanceof JSFunctionObject) {
JSFunctionObject arg1Value_ = (JSFunctionObject) arg1Value;
if ((!(JSGuards.isJSObject(arg0Value))) && (JSGuards.isForeignObject(arg0Value)) && (!(JSFunction.isBoundFunction(arg1Value_)))) {
IsObjectNode isAnyObjectNode_;
IsObjectNode isAnyObjectNode__shared = this.isAnyObjectNode;
if (isAnyObjectNode__shared != null) {
isAnyObjectNode_ = isAnyObjectNode__shared;
} else {
isAnyObjectNode_ = this.insert((IsObjectNode.create()));
if (isAnyObjectNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.isAnyObjectNode == null) {
VarHandle.storeStoreFence();
this.isAnyObjectNode = isAnyObjectNode_;
}
ForeignObjectPrototypeNode getForeignPrototypeNode_;
ForeignObjectPrototypeNode getForeignPrototypeNode__shared = this.getForeignPrototypeNode;
if (getForeignPrototypeNode__shared != null) {
getForeignPrototypeNode_ = getForeignPrototypeNode__shared;
} else {
getForeignPrototypeNode_ = this.insert((ForeignObjectPrototypeNode.create()));
if (getForeignPrototypeNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.getForeignPrototypeNode == null) {
VarHandle.storeStoreFence();
this.getForeignPrototypeNode = getForeignPrototypeNode_;
}
OrdinaryHasInstanceNode ordinaryHasInstanceNode_;
OrdinaryHasInstanceNode ordinaryHasInstanceNode__shared = this.ordinaryHasInstanceNode;
if (ordinaryHasInstanceNode__shared != null) {
ordinaryHasInstanceNode_ = ordinaryHasInstanceNode__shared;
} else {
ordinaryHasInstanceNode_ = this.insert((OrdinaryHasInstanceNode.create(context)));
if (ordinaryHasInstanceNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ordinaryHasInstanceNode == null) {
VarHandle.storeStoreFence();
this.ordinaryHasInstanceNode = ordinaryHasInstanceNode_;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doForeignObjectUnbound(Object, JSFunctionObject, IsObjectNode, ForeignObjectPrototypeNode, InlinedBranchProfile, OrdinaryHasInstanceNode)] */;
this.state_0_ = state_0;
return doForeignObjectUnbound(arg0Value, arg1Value_, isAnyObjectNode_, getForeignPrototypeNode_, INLINED_INVALID_PROTOTYPE_BRANCH, ordinaryHasInstanceNode_);
}
}
if (arg1Value instanceof JSProxyObject) {
JSProxyObject arg1Value_ = (JSProxyObject) arg1Value;
if ((!(JSGuards.isJSObject(arg0Value))) && (JSGuards.isForeignObject(arg0Value)) && (JSGuards.isCallableProxy(arg1Value_))) {
IsObjectNode isAnyObjectNode_1;
IsObjectNode isAnyObjectNode_1_shared = this.isAnyObjectNode;
if (isAnyObjectNode_1_shared != null) {
isAnyObjectNode_1 = isAnyObjectNode_1_shared;
} else {
isAnyObjectNode_1 = this.insert((IsObjectNode.create()));
if (isAnyObjectNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.isAnyObjectNode == null) {
VarHandle.storeStoreFence();
this.isAnyObjectNode = isAnyObjectNode_1;
}
ForeignObjectPrototypeNode getForeignPrototypeNode_1;
ForeignObjectPrototypeNode getForeignPrototypeNode_1_shared = this.getForeignPrototypeNode;
if (getForeignPrototypeNode_1_shared != null) {
getForeignPrototypeNode_1 = getForeignPrototypeNode_1_shared;
} else {
getForeignPrototypeNode_1 = this.insert((ForeignObjectPrototypeNode.create()));
if (getForeignPrototypeNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.getForeignPrototypeNode == null) {
VarHandle.storeStoreFence();
this.getForeignPrototypeNode = getForeignPrototypeNode_1;
}
OrdinaryHasInstanceNode ordinaryHasInstanceNode_1;
OrdinaryHasInstanceNode ordinaryHasInstanceNode_1_shared = this.ordinaryHasInstanceNode;
if (ordinaryHasInstanceNode_1_shared != null) {
ordinaryHasInstanceNode_1 = ordinaryHasInstanceNode_1_shared;
} else {
ordinaryHasInstanceNode_1 = this.insert((OrdinaryHasInstanceNode.create(context)));
if (ordinaryHasInstanceNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ordinaryHasInstanceNode == null) {
VarHandle.storeStoreFence();
this.ordinaryHasInstanceNode = ordinaryHasInstanceNode_1;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doForeignObjectProxy(Object, JSProxyObject, IsObjectNode, ForeignObjectPrototypeNode, InlinedBranchProfile, OrdinaryHasInstanceNode)] */;
this.state_0_ = state_0;
return doForeignObjectProxy(arg0Value, arg1Value_, isAnyObjectNode_1, getForeignPrototypeNode_1, INLINED_INVALID_PROTOTYPE_BRANCH, ordinaryHasInstanceNode_1);
}
}
if (arg1Value instanceof JSFunctionObject) {
JSFunctionObject arg1Value_ = (JSFunctionObject) arg1Value;
if ((!(JSGuards.isJSObject(arg0Value))) && (!(JSGuards.isForeignObject(arg0Value))) && (!(JSFunction.isBoundFunction(arg1Value_)))) {
state_0 = state_0 | 0b100000 /* add SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doNotAnObjectUnbound(Object, JSFunctionObject)] */;
this.state_0_ = state_0;
return OrdinaryHasInstanceNode.doNotAnObjectUnbound(arg0Value, arg1Value_);
}
}
if (arg1Value instanceof JSProxyObject) {
JSProxyObject arg1Value_ = (JSProxyObject) arg1Value;
if ((!(JSGuards.isJSObject(arg0Value))) && (!(JSGuards.isForeignObject(arg0Value))) && (JSGuards.isCallableProxy(arg1Value_))) {
state_0 = state_0 | 0b1000000 /* add SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doNotAnObjectProxy(Object, JSProxyObject)] */;
this.state_0_ = state_0;
return OrdinaryHasInstanceNode.doNotAnObjectProxy(arg0Value, arg1Value_);
}
}
if ((!(isCallableNode.executeBoolean(arg1Value)))) {
state_0 = state_0 | 0b10000000 /* add SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doNotCallable(Object, Object)] */;
this.state_0_ = state_0;
return OrdinaryHasInstanceNode.doNotCallable(arg0Value, arg1Value);
}
if ((JSGuards.isForeignObject(arg1Value))) {
state_0 = state_0 | 0b100000000 /* add SpecializationActive[InstanceofNode.OrdinaryHasInstanceNode.doForeignTarget(Object, Object)] */;
this.state_0_ = state_0;
return OrdinaryHasInstanceNode.doForeignTarget(arg0Value, arg1Value);
}
throw new UnsupportedSpecializationException(this, null, arg0Value, arg1Value);
}
@NeverDefault
public static OrdinaryHasInstanceNode create(JSContext context) {
return new OrdinaryHasInstanceNodeGen(context);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy