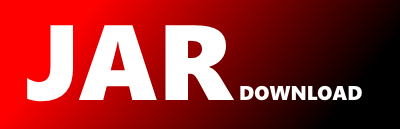
com.oracle.truffle.js.nodes.binary.JSLeftShiftConstantNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.binary;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.js.nodes.JSTypes;
import com.oracle.truffle.js.nodes.JSTypesGen;
import com.oracle.truffle.js.nodes.JavaScriptNode;
import com.oracle.truffle.js.nodes.cast.JSToInt32Node;
import com.oracle.truffle.js.nodes.cast.JSToNumericNode;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.SafeInteger;
import com.oracle.truffle.js.runtime.builtins.JSOverloadedOperatorsObject;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSLeftShiftConstantNode#doInteger}
* Activation probability: 0.27381
* With/without class size: 7/0 bytes
* Specialization {@link JSLeftShiftConstantNode#doSafeInteger}
* Activation probability: 0.23095
* With/without class size: 6/0 bytes
* Specialization {@link JSLeftShiftConstantNode#doDouble}
* Activation probability: 0.18810
* With/without class size: 7/4 bytes
* Specialization {@link JSLeftShiftConstantNode#doBigInt}
* Activation probability: 0.14524
* With/without class size: 5/0 bytes
* Specialization {@link JSLeftShiftConstantNode#doOverloaded}
* Activation probability: 0.10238
* With/without class size: 6/4 bytes
* Specialization {@link JSLeftShiftConstantNode#doGeneric}
* Activation probability: 0.05952
* With/without class size: 5/8 bytes
*
*/
@GeneratedBy(JSLeftShiftConstantNode.class)
@SuppressWarnings("javadoc")
public final class JSLeftShiftConstantNodeGen extends JSLeftShiftConstantNode {
/**
* State Info:
* 0: SpecializationActive {@link JSLeftShiftConstantNode#doInteger}
* 1: SpecializationActive {@link JSLeftShiftConstantNode#doGeneric}
* 2: SpecializationActive {@link JSLeftShiftConstantNode#doSafeInteger}
* 3: SpecializationActive {@link JSLeftShiftConstantNode#doDouble}
* 4: SpecializationActive {@link JSLeftShiftConstantNode#doBigInt}
* 5: SpecializationActive {@link JSLeftShiftConstantNode#doOverloaded}
* 6-8: ImplicitCast[type=double, index=0]
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSLeftShiftConstantNode#doDouble}
* Parameter: {@link JSToInt32Node} leftInt32Node
*/
@Child private JSToInt32Node double_leftInt32Node_;
/**
* Source Info:
* Specialization: {@link JSLeftShiftConstantNode#doOverloaded}
* Parameter: {@link JSOverloadedBinaryNode} overloadedOperatorNode
*/
@Child private JSOverloadedBinaryNode overloaded_overloadedOperatorNode_;
@Child private GenericData generic_cache;
private JSLeftShiftConstantNodeGen(JavaScriptNode operand, int shiftValue) {
super(operand, shiftValue);
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
if ((state_0 & 0b101110) == 0 /* only-active SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] */ && ((state_0 & 0b101111) != 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] && SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] && SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */)) {
return execute_int0(state_0, frameValue);
} else if ((state_0 & 0b100111) == 0 /* only-active SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */ && ((state_0 & 0b101111) != 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] && SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] && SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */)) {
return execute_double1(state_0, frameValue);
} else {
return execute_generic2(state_0, frameValue);
}
}
private Object execute_int0(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
int operandNodeValue_;
try {
operandNodeValue_ = super.operandNode.executeInt(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(ex.getResult());
}
assert (state_0 & 0b1) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] */;
return doInteger(operandNodeValue_);
}
private Object execute_double1(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
int operandNodeValue_int = 0;
double operandNodeValue_;
try {
if ((state_0 & 0b110000000) == 0 /* only-active ImplicitCast[type=double, index=0] */ && ((state_0 & 0b111111) != 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] && SpecializationActive[JSLeftShiftConstantNode.doBigInt(BigInt)] && SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] && SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */)) {
operandNodeValue_ = super.operandNode.executeDouble(frameValue);
} else if ((state_0 & 0b101000000) == 0 /* only-active ImplicitCast[type=double, index=0] */ && ((state_0 & 0b111111) != 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] && SpecializationActive[JSLeftShiftConstantNode.doBigInt(BigInt)] && SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] && SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */)) {
operandNodeValue_int = super.operandNode.executeInt(frameValue);
operandNodeValue_ = JSTypes.intToDouble(operandNodeValue_int);
} else {
Object operandNodeValue__ = super.operandNode.execute(frameValue);
operandNodeValue_ = JSTypesGen.expectImplicitDouble((state_0 & 0b111000000) >>> 6 /* get-int ImplicitCast[type=double, index=0] */, operandNodeValue__);
}
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(ex.getResult());
}
assert (state_0 & 0b1000) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */;
{
JSToInt32Node leftInt32Node__ = this.double_leftInt32Node_;
if (leftInt32Node__ != null) {
return doDouble(operandNodeValue_, leftInt32Node__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(((state_0 & 0b101000000) == 0 /* only-active ImplicitCast[type=double, index=0] */ && ((state_0 & 0b111111) != 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] && SpecializationActive[JSLeftShiftConstantNode.doBigInt(BigInt)] && SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] && SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */) ? (Object) operandNodeValue_int : (Object) operandNodeValue_));
}
private Object execute_generic2(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object operandNodeValue_ = super.operandNode.execute(frameValue);
if ((state_0 & 0b101111) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] || SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] || SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] || SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] || SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] */ && operandNodeValue_ instanceof Integer) {
int operandNodeValue__ = (int) operandNodeValue_;
return doInteger(operandNodeValue__);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] */ && operandNodeValue_ instanceof SafeInteger) {
SafeInteger operandNodeValue__ = (SafeInteger) operandNodeValue_;
return doSafeInteger(operandNodeValue__);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b111000000) >>> 6 /* get-int ImplicitCast[type=double, index=0] */, operandNodeValue_)) {
double operandNodeValue__ = JSTypesGen.asImplicitDouble((state_0 & 0b111000000) >>> 6 /* get-int ImplicitCast[type=double, index=0] */, operandNodeValue_);
{
JSToInt32Node leftInt32Node__ = this.double_leftInt32Node_;
if (leftInt32Node__ != null) {
return doDouble(operandNodeValue__, leftInt32Node__);
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] */ && operandNodeValue_ instanceof JSOverloadedOperatorsObject) {
JSOverloadedOperatorsObject operandNodeValue__ = (JSOverloadedOperatorsObject) operandNodeValue_;
{
JSOverloadedBinaryNode overloadedOperatorNode__ = this.overloaded_overloadedOperatorNode_;
if (overloadedOperatorNode__ != null) {
return doOverloaded(operandNodeValue__, overloadedOperatorNode__);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */) {
GenericData s5_ = this.generic_cache;
if (s5_ != null) {
if ((!(hasOverloadedOperators(operandNodeValue_)))) {
return doGeneric(operandNodeValue_, s5_.leftToNumericNode_, s5_.innerShiftNode_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(operandNodeValue_);
}
@Override
public int executeInt(VirtualFrame frameValue) throws UnexpectedResultException {
int state_0 = this.state_0_;
if ((state_0 & 0b100010) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] || SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */) {
return JSTypesGen.expectInteger(execute(frameValue));
}
if ((state_0 & 0b1100) == 0 /* only-active SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] */ && ((state_0 & 0b1101) != 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */)) {
return executeInt_int3(state_0, frameValue);
} else if ((state_0 & 0b101) == 0 /* only-active SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */ && ((state_0 & 0b1101) != 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */)) {
return executeInt_double4(state_0, frameValue);
} else {
return executeInt_generic5(state_0, frameValue);
}
}
private int executeInt_int3(int state_0__, VirtualFrame frameValue) throws UnexpectedResultException {
int state_0 = state_0__;
int operandNodeValue_;
try {
operandNodeValue_ = super.operandNode.executeInt(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return JSTypesGen.expectInteger(executeAndSpecialize(ex.getResult()));
}
assert (state_0 & 0b1) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] */;
return doInteger(operandNodeValue_);
}
private int executeInt_double4(int state_0__, VirtualFrame frameValue) throws UnexpectedResultException {
int state_0 = state_0__;
int operandNodeValue_int = 0;
double operandNodeValue_;
try {
if ((state_0 & 0b110000000) == 0 /* only-active ImplicitCast[type=double, index=0] */ && ((state_0 & 0b111111) != 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] && SpecializationActive[JSLeftShiftConstantNode.doBigInt(BigInt)] && SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] && SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */)) {
operandNodeValue_ = super.operandNode.executeDouble(frameValue);
} else if ((state_0 & 0b101000000) == 0 /* only-active ImplicitCast[type=double, index=0] */ && ((state_0 & 0b111111) != 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] && SpecializationActive[JSLeftShiftConstantNode.doBigInt(BigInt)] && SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] && SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */)) {
operandNodeValue_int = super.operandNode.executeInt(frameValue);
operandNodeValue_ = JSTypes.intToDouble(operandNodeValue_int);
} else {
Object operandNodeValue__ = super.operandNode.execute(frameValue);
operandNodeValue_ = JSTypesGen.expectImplicitDouble((state_0 & 0b111000000) >>> 6 /* get-int ImplicitCast[type=double, index=0] */, operandNodeValue__);
}
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return JSTypesGen.expectInteger(executeAndSpecialize(ex.getResult()));
}
assert (state_0 & 0b1000) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */;
{
JSToInt32Node leftInt32Node__ = this.double_leftInt32Node_;
if (leftInt32Node__ != null) {
return doDouble(operandNodeValue_, leftInt32Node__);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return JSTypesGen.expectInteger(executeAndSpecialize(((state_0 & 0b101000000) == 0 /* only-active ImplicitCast[type=double, index=0] */ && ((state_0 & 0b111111) != 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] && SpecializationActive[JSLeftShiftConstantNode.doBigInt(BigInt)] && SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] && SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */) ? (Object) operandNodeValue_int : (Object) operandNodeValue_)));
}
private int executeInt_generic5(int state_0__, VirtualFrame frameValue) throws UnexpectedResultException {
int state_0 = state_0__;
Object operandNodeValue_ = super.operandNode.execute(frameValue);
if ((state_0 & 0b1101) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] || SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] || SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] */ && operandNodeValue_ instanceof Integer) {
int operandNodeValue__ = (int) operandNodeValue_;
return doInteger(operandNodeValue__);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] */ && operandNodeValue_ instanceof SafeInteger) {
SafeInteger operandNodeValue__ = (SafeInteger) operandNodeValue_;
return doSafeInteger(operandNodeValue__);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b111000000) >>> 6 /* get-int ImplicitCast[type=double, index=0] */, operandNodeValue_)) {
double operandNodeValue__ = JSTypesGen.asImplicitDouble((state_0 & 0b111000000) >>> 6 /* get-int ImplicitCast[type=double, index=0] */, operandNodeValue_);
{
JSToInt32Node leftInt32Node__ = this.double_leftInt32Node_;
if (leftInt32Node__ != null) {
return doDouble(operandNodeValue__, leftInt32Node__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return JSTypesGen.expectInteger(executeAndSpecialize(operandNodeValue_));
}
@Override
public int executeInt(Object operandNodeValue) {
int state_0 = this.state_0_;
if ((state_0 & 0b1101) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] || SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] || SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] */ && operandNodeValue instanceof Integer) {
int operandNodeValue_ = (int) operandNodeValue;
return doInteger(operandNodeValue_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] */ && operandNodeValue instanceof SafeInteger) {
SafeInteger operandNodeValue_ = (SafeInteger) operandNodeValue;
return doSafeInteger(operandNodeValue_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b111000000) >>> 6 /* get-int ImplicitCast[type=double, index=0] */, operandNodeValue)) {
double operandNodeValue_ = JSTypesGen.asImplicitDouble((state_0 & 0b111000000) >>> 6 /* get-int ImplicitCast[type=double, index=0] */, operandNodeValue);
{
JSToInt32Node leftInt32Node__ = this.double_leftInt32Node_;
if (leftInt32Node__ != null) {
return doDouble(operandNodeValue_, leftInt32Node__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return (int) executeAndSpecialize(operandNodeValue);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
int state_0 = this.state_0_;
try {
if ((state_0 & 0b110010) == 0 /* only-active SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */ && ((state_0 & 0b111111) != 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] && SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] && SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] && SpecializationActive[JSLeftShiftConstantNode.doBigInt(BigInt)] && SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] && SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */)) {
executeInt(frameValue);
return;
} else if ((state_0 & 0b101111) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] || SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] || SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] || SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] || SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */) {
execute(frameValue);
return;
}
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return;
}
Object operandNodeValue_ = super.operandNode.execute(frameValue);
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSLeftShiftConstantNode.doBigInt(BigInt)] */ && operandNodeValue_ instanceof BigInt) {
BigInt operandNodeValue__ = (BigInt) operandNodeValue_;
doBigInt(operandNodeValue__);
return;
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(operandNodeValue_);
return;
}
private Object executeAndSpecialize(Object operandNodeValue) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */ && operandNodeValue instanceof Integer) {
int operandNodeValue_ = (int) operandNodeValue;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSLeftShiftConstantNode.doInteger(int)] */;
this.state_0_ = state_0;
return doInteger(operandNodeValue_);
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */ && operandNodeValue instanceof SafeInteger) {
SafeInteger operandNodeValue_ = (SafeInteger) operandNodeValue;
state_0 = state_0 | 0b100 /* add SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)] */;
this.state_0_ = state_0;
return doSafeInteger(operandNodeValue_);
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */) {
int doubleCast0;
if ((doubleCast0 = JSTypesGen.specializeImplicitDouble(operandNodeValue)) != 0) {
double operandNodeValue_ = JSTypesGen.asImplicitDouble(doubleCast0, operandNodeValue);
JSToInt32Node leftInt32Node__ = this.insert((JSToInt32Node.create()));
Objects.requireNonNull(leftInt32Node__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.double_leftInt32Node_ = leftInt32Node__;
state_0 = (state_0 | (doubleCast0 << 6) /* set-int ImplicitCast[type=double, index=0] */);
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)] */;
this.state_0_ = state_0;
return doDouble(operandNodeValue_, leftInt32Node__);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */ && operandNodeValue instanceof BigInt) {
BigInt operandNodeValue_ = (BigInt) operandNodeValue;
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSLeftShiftConstantNode.doBigInt(BigInt)] */;
this.state_0_ = state_0;
doBigInt(operandNodeValue_);
return null;
}
if (operandNodeValue instanceof JSOverloadedOperatorsObject) {
JSOverloadedOperatorsObject operandNodeValue_ = (JSOverloadedOperatorsObject) operandNodeValue;
JSOverloadedBinaryNode overloadedOperatorNode__ = this.insert((JSOverloadedBinaryNode.createNumeric(getOverloadedOperatorName())));
Objects.requireNonNull(overloadedOperatorNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.overloaded_overloadedOperatorNode_ = overloadedOperatorNode__;
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSLeftShiftConstantNode.doOverloaded(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] */;
this.state_0_ = state_0;
return doOverloaded(operandNodeValue_, overloadedOperatorNode__);
}
if ((!(hasOverloadedOperators(operandNodeValue)))) {
GenericData s5_ = this.insert(new GenericData());
JSToNumericNode leftToNumericNode__ = s5_.insert((JSToNumericNode.create()));
Objects.requireNonNull(leftToNumericNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s5_.leftToNumericNode_ = leftToNumericNode__;
s5_.innerShiftNode_ = s5_.insert((makeCopy()));
VarHandle.storeStoreFence();
this.generic_cache = s5_;
this.double_leftInt32Node_ = null;
state_0 = state_0 & 0xffffffe2 /* remove SpecializationActive[JSLeftShiftConstantNode.doInteger(int)], SpecializationActive[JSLeftShiftConstantNode.doSafeInteger(SafeInteger)], SpecializationActive[JSLeftShiftConstantNode.doDouble(double, JSToInt32Node)], SpecializationActive[JSLeftShiftConstantNode.doBigInt(BigInt)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSLeftShiftConstantNode.doGeneric(Object, JSToNumericNode, JSLeftShiftConstantNode)] */;
this.state_0_ = state_0;
return doGeneric(operandNodeValue, leftToNumericNode__, s5_.innerShiftNode_);
}
throw new UnsupportedSpecializationException(this, new Node[] {super.operandNode}, operandNodeValue);
}
@NeverDefault
public static JSLeftShiftConstantNode create(JavaScriptNode operand, int shiftValue) {
return new JSLeftShiftConstantNodeGen(operand, shiftValue);
}
@GeneratedBy(JSLeftShiftConstantNode.class)
@DenyReplace
private static final class GenericData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link JSLeftShiftConstantNode#doGeneric}
* Parameter: {@link JSToNumericNode} leftToNumericNode
*/
@Child JSToNumericNode leftToNumericNode_;
/**
* Source Info:
* Specialization: {@link JSLeftShiftConstantNode#doGeneric}
* Parameter: {@link JSLeftShiftConstantNode} innerShiftNode
*/
@Child JSLeftShiftConstantNode innerShiftNode_;
GenericData() {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy