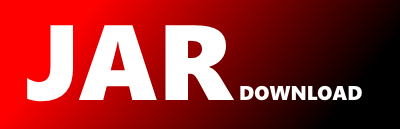
com.oracle.truffle.js.nodes.binary.JSOverloadedBinaryNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.binary;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.cast.JSToNumericNode;
import com.oracle.truffle.js.nodes.cast.JSToOperandNode;
import com.oracle.truffle.js.nodes.cast.JSToStringNode;
import com.oracle.truffle.js.nodes.cast.JSToPrimitiveNode.Hint;
import com.oracle.truffle.js.nodes.function.JSFunctionCallNode;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.builtins.JSOverloadedOperatorsObject;
import com.oracle.truffle.js.runtime.objects.OperatorSet;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSOverloadedBinaryNode#doToOperandGeneric}
* Activation probability: 0.48333
* With/without class size: 15/8 bytes
* Specialization {@link JSOverloadedBinaryNode#doToOperandAddition}
* Activation probability: 0.33333
* With/without class size: 17/21 bytes
* Specialization {@link JSOverloadedBinaryNode#doToNumericOperand}
* Activation probability: 0.18333
* With/without class size: 8/8 bytes
*
*/
@GeneratedBy(JSOverloadedBinaryNode.class)
@SuppressWarnings("javadoc")
public final class JSOverloadedBinaryNodeGen extends JSOverloadedBinaryNode {
private static final StateField TO_OPERAND_ADDITION__J_S_OVERLOADED_BINARY_NODE_TO_OPERAND_ADDITION_STATE_0_UPDATER = StateField.create(ToOperandAdditionData.lookup_(), "toOperandAddition_state_0_");
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToOperandAddition}
* Parameter: {@link InlinedConditionProfile} leftStringProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_TO_OPERAND_ADDITION_LEFT_STRING_PROFILE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, TO_OPERAND_ADDITION__J_S_OVERLOADED_BINARY_NODE_TO_OPERAND_ADDITION_STATE_0_UPDATER.subUpdater(0, 2)));
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToOperandAddition}
* Parameter: {@link InlinedConditionProfile} rightStringProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_TO_OPERAND_ADDITION_RIGHT_STRING_PROFILE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, TO_OPERAND_ADDITION__J_S_OVERLOADED_BINARY_NODE_TO_OPERAND_ADDITION_STATE_0_UPDATER.subUpdater(2, 2)));
/**
* State Info:
* 0: SpecializationActive {@link JSOverloadedBinaryNode#doToOperandGeneric}
* 1: SpecializationActive {@link JSOverloadedBinaryNode#doToOperandAddition}
* 2: SpecializationActive {@link JSOverloadedBinaryNode#doToNumericOperand}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToOperandGeneric}
* Parameter: {@link DispatchBinaryOperatorNode} dispatchBinaryOperatorNode
*/
@Child private DispatchBinaryOperatorNode dispatchBinaryOperatorNode;
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToOperandGeneric}
* Parameter: {@link JSToOperandNode} toOperandLeftNode
*/
@Child private JSToOperandNode toOperandGeneric_toOperandLeftNode_;
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToOperandGeneric}
* Parameter: {@link JSToOperandNode} toOperandRightNode
*/
@Child private JSToOperandNode toOperandGeneric_toOperandRightNode_;
@Child private ToOperandAdditionData toOperandAddition_cache;
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToNumericOperand}
* Parameter: {@link JSToNumericNode} toNumericOperandLeftNode
*/
@Child private JSToNumericNode toNumericOperand_toNumericOperandLeftNode_;
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToNumericOperand}
* Parameter: {@link JSToNumericNode} toNumericOperandRightNode
*/
@Child private JSToNumericNode toNumericOperand_toNumericOperandRightNode_;
private JSOverloadedBinaryNodeGen(TruffleString overloadedOperatorName, boolean numeric, Hint hint, boolean leftToRight) {
super(overloadedOperatorName, numeric, hint, leftToRight);
}
@Override
public Object execute(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[JSOverloadedBinaryNode.doToOperandGeneric(Object, Object, JSToOperandNode, JSToOperandNode, DispatchBinaryOperatorNode)] || SpecializationActive[JSOverloadedBinaryNode.doToOperandAddition(Object, Object, Node, JSToOperandNode, JSToOperandNode, DispatchBinaryOperatorNode, JSToStringNode, JSToStringNode, InlinedConditionProfile, InlinedConditionProfile, JSAddNode)] || SpecializationActive[JSOverloadedBinaryNode.doToNumericOperand(Object, Object, JSToNumericNode, JSToNumericNode, DispatchBinaryOperatorNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.doToOperandGeneric(Object, Object, JSToOperandNode, JSToOperandNode, DispatchBinaryOperatorNode)] */) {
{
JSToOperandNode toOperandLeftNode__ = this.toOperandGeneric_toOperandLeftNode_;
if (toOperandLeftNode__ != null) {
JSToOperandNode toOperandRightNode__ = this.toOperandGeneric_toOperandRightNode_;
if (toOperandRightNode__ != null) {
DispatchBinaryOperatorNode dispatchBinaryOperatorNode_ = this.dispatchBinaryOperatorNode;
if (dispatchBinaryOperatorNode_ != null) {
assert DSLSupport.assertIdempotence((!(isNumeric())));
assert DSLSupport.assertIdempotence((!(isAddition())));
return doToOperandGeneric(arg0Value, arg1Value, toOperandLeftNode__, toOperandRightNode__, dispatchBinaryOperatorNode_);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.doToOperandAddition(Object, Object, Node, JSToOperandNode, JSToOperandNode, DispatchBinaryOperatorNode, JSToStringNode, JSToStringNode, InlinedConditionProfile, InlinedConditionProfile, JSAddNode)] */) {
ToOperandAdditionData s1_ = this.toOperandAddition_cache;
if (s1_ != null) {
{
DispatchBinaryOperatorNode dispatchBinaryOperatorNode_1 = this.dispatchBinaryOperatorNode;
if (dispatchBinaryOperatorNode_1 != null) {
Node node__ = (s1_);
assert DSLSupport.assertIdempotence((!(isNumeric())));
assert DSLSupport.assertIdempotence((isAddition()));
return doToOperandAddition(arg0Value, arg1Value, node__, s1_.toOperandLeftNode_, s1_.toOperandRightNode_, dispatchBinaryOperatorNode_1, s1_.toStringLeftNode_, s1_.toStringRightNode_, INLINED_TO_OPERAND_ADDITION_LEFT_STRING_PROFILE_, INLINED_TO_OPERAND_ADDITION_RIGHT_STRING_PROFILE_, s1_.addNode_);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.doToNumericOperand(Object, Object, JSToNumericNode, JSToNumericNode, DispatchBinaryOperatorNode)] */) {
{
JSToNumericNode toNumericOperandLeftNode__ = this.toNumericOperand_toNumericOperandLeftNode_;
if (toNumericOperandLeftNode__ != null) {
JSToNumericNode toNumericOperandRightNode__ = this.toNumericOperand_toNumericOperandRightNode_;
if (toNumericOperandRightNode__ != null) {
DispatchBinaryOperatorNode dispatchBinaryOperatorNode_2 = this.dispatchBinaryOperatorNode;
if (dispatchBinaryOperatorNode_2 != null) {
assert DSLSupport.assertIdempotence((isNumeric()));
return doToNumericOperand(arg0Value, arg1Value, toNumericOperandLeftNode__, toNumericOperandRightNode__, dispatchBinaryOperatorNode_2);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if ((!(isNumeric())) && (!(isAddition()))) {
JSToOperandNode toOperandLeftNode__ = this.insert((JSToOperandNode.create(getHint(), !(isEquality()))));
Objects.requireNonNull(toOperandLeftNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toOperandGeneric_toOperandLeftNode_ = toOperandLeftNode__;
JSToOperandNode toOperandRightNode__ = this.insert((JSToOperandNode.create(getHint(), !(isEquality()))));
Objects.requireNonNull(toOperandRightNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toOperandGeneric_toOperandRightNode_ = toOperandRightNode__;
DispatchBinaryOperatorNode dispatchBinaryOperatorNode_;
DispatchBinaryOperatorNode dispatchBinaryOperatorNode__shared = this.dispatchBinaryOperatorNode;
if (dispatchBinaryOperatorNode__shared != null) {
dispatchBinaryOperatorNode_ = dispatchBinaryOperatorNode__shared;
} else {
dispatchBinaryOperatorNode_ = this.insert((DispatchBinaryOperatorNodeGen.create(getOverloadedOperatorName())));
if (dispatchBinaryOperatorNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dispatchBinaryOperatorNode == null) {
VarHandle.storeStoreFence();
this.dispatchBinaryOperatorNode = dispatchBinaryOperatorNode_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[JSOverloadedBinaryNode.doToOperandGeneric(Object, Object, JSToOperandNode, JSToOperandNode, DispatchBinaryOperatorNode)] */;
this.state_0_ = state_0;
return doToOperandGeneric(arg0Value, arg1Value, toOperandLeftNode__, toOperandRightNode__, dispatchBinaryOperatorNode_);
}
{
Node node__ = null;
if ((!(isNumeric())) && (isAddition())) {
ToOperandAdditionData s1_ = this.insert(new ToOperandAdditionData());
node__ = (s1_);
JSToOperandNode toOperandLeftNode__1 = s1_.insert((JSToOperandNode.create(getHint())));
Objects.requireNonNull(toOperandLeftNode__1, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.toOperandLeftNode_ = toOperandLeftNode__1;
JSToOperandNode toOperandRightNode__1 = s1_.insert((JSToOperandNode.create(getHint())));
Objects.requireNonNull(toOperandRightNode__1, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.toOperandRightNode_ = toOperandRightNode__1;
DispatchBinaryOperatorNode dispatchBinaryOperatorNode_1;
DispatchBinaryOperatorNode dispatchBinaryOperatorNode_1_shared = this.dispatchBinaryOperatorNode;
if (dispatchBinaryOperatorNode_1_shared != null) {
dispatchBinaryOperatorNode_1 = dispatchBinaryOperatorNode_1_shared;
} else {
dispatchBinaryOperatorNode_1 = s1_.insert((DispatchBinaryOperatorNodeGen.create(getOverloadedOperatorName())));
if (dispatchBinaryOperatorNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dispatchBinaryOperatorNode == null) {
this.dispatchBinaryOperatorNode = dispatchBinaryOperatorNode_1;
}
JSToStringNode toStringLeftNode__ = s1_.insert((JSToStringNode.create()));
Objects.requireNonNull(toStringLeftNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.toStringLeftNode_ = toStringLeftNode__;
JSToStringNode toStringRightNode__ = s1_.insert((JSToStringNode.create()));
Objects.requireNonNull(toStringRightNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.toStringRightNode_ = toStringRightNode__;
s1_.addNode_ = s1_.insert((JSAddNode.createUnoptimized()));
VarHandle.storeStoreFence();
this.toOperandAddition_cache = s1_;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSOverloadedBinaryNode.doToOperandAddition(Object, Object, Node, JSToOperandNode, JSToOperandNode, DispatchBinaryOperatorNode, JSToStringNode, JSToStringNode, InlinedConditionProfile, InlinedConditionProfile, JSAddNode)] */;
this.state_0_ = state_0;
return doToOperandAddition(arg0Value, arg1Value, node__, toOperandLeftNode__1, toOperandRightNode__1, dispatchBinaryOperatorNode_1, toStringLeftNode__, toStringRightNode__, INLINED_TO_OPERAND_ADDITION_LEFT_STRING_PROFILE_, INLINED_TO_OPERAND_ADDITION_RIGHT_STRING_PROFILE_, s1_.addNode_);
}
}
if ((isNumeric())) {
JSToNumericNode toNumericOperandLeftNode__ = this.insert((JSToNumericNode.create(true)));
Objects.requireNonNull(toNumericOperandLeftNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toNumericOperand_toNumericOperandLeftNode_ = toNumericOperandLeftNode__;
JSToNumericNode toNumericOperandRightNode__ = this.insert((JSToNumericNode.create(true)));
Objects.requireNonNull(toNumericOperandRightNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toNumericOperand_toNumericOperandRightNode_ = toNumericOperandRightNode__;
DispatchBinaryOperatorNode dispatchBinaryOperatorNode_2;
DispatchBinaryOperatorNode dispatchBinaryOperatorNode_2_shared = this.dispatchBinaryOperatorNode;
if (dispatchBinaryOperatorNode_2_shared != null) {
dispatchBinaryOperatorNode_2 = dispatchBinaryOperatorNode_2_shared;
} else {
dispatchBinaryOperatorNode_2 = this.insert((DispatchBinaryOperatorNodeGen.create(getOverloadedOperatorName())));
if (dispatchBinaryOperatorNode_2 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.dispatchBinaryOperatorNode == null) {
VarHandle.storeStoreFence();
this.dispatchBinaryOperatorNode = dispatchBinaryOperatorNode_2;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[JSOverloadedBinaryNode.doToNumericOperand(Object, Object, JSToNumericNode, JSToNumericNode, DispatchBinaryOperatorNode)] */;
this.state_0_ = state_0;
return doToNumericOperand(arg0Value, arg1Value, toNumericOperandLeftNode__, toNumericOperandRightNode__, dispatchBinaryOperatorNode_2);
}
throw new UnsupportedSpecializationException(this, null, arg0Value, arg1Value);
}
@NeverDefault
public static JSOverloadedBinaryNode create(TruffleString overloadedOperatorName, boolean numeric, Hint hint, boolean leftToRight) {
return new JSOverloadedBinaryNodeGen(overloadedOperatorName, numeric, hint, leftToRight);
}
@GeneratedBy(JSOverloadedBinaryNode.class)
@DenyReplace
private static final class ToOperandAdditionData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link JSOverloadedBinaryNode#doToOperandAddition}
* Parameter: {@link InlinedConditionProfile} leftStringProfile
* Inline method: {@link InlinedConditionProfile#inline}
* 2-3: InlinedCache
* Specialization: {@link JSOverloadedBinaryNode#doToOperandAddition}
* Parameter: {@link InlinedConditionProfile} rightStringProfile
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int toOperandAddition_state_0_;
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToOperandAddition}
* Parameter: {@link JSToOperandNode} toOperandLeftNode
*/
@Child JSToOperandNode toOperandLeftNode_;
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToOperandAddition}
* Parameter: {@link JSToOperandNode} toOperandRightNode
*/
@Child JSToOperandNode toOperandRightNode_;
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToOperandAddition}
* Parameter: {@link JSToStringNode} toStringLeftNode
*/
@Child JSToStringNode toStringLeftNode_;
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToOperandAddition}
* Parameter: {@link JSToStringNode} toStringRightNode
*/
@Child JSToStringNode toStringRightNode_;
/**
* Source Info:
* Specialization: {@link JSOverloadedBinaryNode#doToOperandAddition}
* Parameter: {@link JSAddNode} addNode
*/
@Child JSAddNode addNode_;
ToOperandAdditionData() {
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
/**
* Debug Info:
* Specialization {@link DispatchBinaryOperatorNode#doOverloadedOverloaded}
* Activation probability: 0.17364
* With/without class size: 10/16 bytes
* Specialization {@link DispatchBinaryOperatorNode#doOverloadedNumber}
* Activation probability: 0.15727
* With/without class size: 9/12 bytes
* Specialization {@link DispatchBinaryOperatorNode#doOverloadedBigInt}
* Activation probability: 0.14091
* With/without class size: 8/12 bytes
* Specialization {@link DispatchBinaryOperatorNode#doOverloadedString}
* Activation probability: 0.12455
* With/without class size: 7/12 bytes
* Specialization {@link DispatchBinaryOperatorNode#doOverloadedNullish}
* Activation probability: 0.10818
* With/without class size: 5/0 bytes
* Specialization {@link DispatchBinaryOperatorNode#doNumberOverloaded}
* Activation probability: 0.09182
* With/without class size: 6/12 bytes
* Specialization {@link DispatchBinaryOperatorNode#doBigIntOverloaded}
* Activation probability: 0.07545
* With/without class size: 6/12 bytes
* Specialization {@link DispatchBinaryOperatorNode#doStringOverloaded}
* Activation probability: 0.05909
* With/without class size: 5/12 bytes
* Specialization {@link DispatchBinaryOperatorNode#doNullishOverloaded}
* Activation probability: 0.04273
* With/without class size: 4/0 bytes
* Specialization {@link DispatchBinaryOperatorNode#doGeneric}
* Activation probability: 0.02636
* With/without class size: 4/4 bytes
*
*/
@GeneratedBy(DispatchBinaryOperatorNode.class)
@SuppressWarnings("javadoc")
public static final class DispatchBinaryOperatorNodeGen extends DispatchBinaryOperatorNode {
static final ReferenceField OVERLOADED_OVERLOADED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "overloadedOverloaded_cache", OverloadedOverloadedData.class);
static final ReferenceField OVERLOADED_NUMBER_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "overloadedNumber_cache", OverloadedNumberData.class);
static final ReferenceField OVERLOADED_BIG_INT_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "overloadedBigInt_cache", OverloadedBigIntData.class);
static final ReferenceField OVERLOADED_STRING_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "overloadedString_cache", OverloadedStringData.class);
static final ReferenceField NUMBER_OVERLOADED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "numberOverloaded_cache", NumberOverloadedData.class);
static final ReferenceField BIG_INT_OVERLOADED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "bigIntOverloaded_cache", BigIntOverloadedData.class);
static final ReferenceField STRING_OVERLOADED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "stringOverloaded_cache", StringOverloadedData.class);
/**
* State Info:
* 0: SpecializationActive {@link DispatchBinaryOperatorNode#doOverloadedOverloaded}
* 1: SpecializationActive {@link DispatchBinaryOperatorNode#doGeneric}
* 2: SpecializationActive {@link DispatchBinaryOperatorNode#doOverloadedNumber}
* 3: SpecializationActive {@link DispatchBinaryOperatorNode#doOverloadedBigInt}
* 4: SpecializationActive {@link DispatchBinaryOperatorNode#doOverloadedString}
* 5: SpecializationActive {@link DispatchBinaryOperatorNode#doOverloadedNullish}
* 6: SpecializationActive {@link DispatchBinaryOperatorNode#doNumberOverloaded}
* 7: SpecializationActive {@link DispatchBinaryOperatorNode#doBigIntOverloaded}
* 8: SpecializationActive {@link DispatchBinaryOperatorNode#doStringOverloaded}
* 9: SpecializationActive {@link DispatchBinaryOperatorNode#doNullishOverloaded}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @Child private OverloadedOverloadedData overloadedOverloaded_cache;
@UnsafeAccessedField @Child private OverloadedNumberData overloadedNumber_cache;
@UnsafeAccessedField @Child private OverloadedBigIntData overloadedBigInt_cache;
@UnsafeAccessedField @Child private OverloadedStringData overloadedString_cache;
@UnsafeAccessedField @Child private NumberOverloadedData numberOverloaded_cache;
@UnsafeAccessedField @Child private BigIntOverloadedData bigIntOverloaded_cache;
@UnsafeAccessedField @Child private StringOverloadedData stringOverloaded_cache;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doGeneric}
* Parameter: {@link JSFunctionCallNode} callNode
*/
@Child private JSFunctionCallNode generic_callNode_;
private DispatchBinaryOperatorNodeGen(TruffleString overloadedOperatorName) {
super(overloadedOperatorName);
}
@ExplodeLoop
@Override
protected Object execute(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedOverloaded(JSOverloadedOperatorsObject, JSOverloadedOperatorsObject, int, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedNumber(JSOverloadedOperatorsObject, Object, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedBigInt(JSOverloadedOperatorsObject, BigInt, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedString(JSOverloadedOperatorsObject, Object, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedNullish(JSOverloadedOperatorsObject, Object)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doNumberOverloaded(Object, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doBigIntOverloaded(BigInt, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doStringOverloaded(Object, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doNullishOverloaded(Object, JSOverloadedOperatorsObject)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doGeneric(Object, Object, JSFunctionCallNode)] */) {
if ((state_0 & 0b111101) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedOverloaded(JSOverloadedOperatorsObject, JSOverloadedOperatorsObject, int, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedNumber(JSOverloadedOperatorsObject, Object, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedBigInt(JSOverloadedOperatorsObject, BigInt, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedString(JSOverloadedOperatorsObject, Object, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedNullish(JSOverloadedOperatorsObject, Object)] */ && arg0Value instanceof JSOverloadedOperatorsObject) {
JSOverloadedOperatorsObject arg0Value_ = (JSOverloadedOperatorsObject) arg0Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedOverloaded(JSOverloadedOperatorsObject, JSOverloadedOperatorsObject, int, int, Object, JSFunctionCallNode)] */ && arg1Value instanceof JSOverloadedOperatorsObject) {
JSOverloadedOperatorsObject arg1Value_ = (JSOverloadedOperatorsObject) arg1Value;
OverloadedOverloadedData s0_ = this.overloadedOverloaded_cache;
while (s0_ != null) {
if ((arg0Value_.matchesOperatorCounter(s0_.leftOperatorCounter_)) && (arg1Value_.matchesOperatorCounter(s0_.rightOperatorCounter_))) {
return doOverloadedOverloaded(arg0Value_, arg1Value_, s0_.leftOperatorCounter_, s0_.rightOperatorCounter_, s0_.operatorImplementation_, s0_.callNode_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedNumber(JSOverloadedOperatorsObject, Object, int, Object, JSFunctionCallNode)] */) {
OverloadedNumberData s1_ = this.overloadedNumber_cache;
while (s1_ != null) {
if ((arg0Value_.matchesOperatorCounter(s1_.leftOperatorCounter_)) && (JSGuards.isNumber(arg1Value))) {
return doOverloadedNumber(arg0Value_, arg1Value, s1_.leftOperatorCounter_, s1_.operatorImplementation_, s1_.callNode_);
}
s1_ = s1_.next_;
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedBigInt(JSOverloadedOperatorsObject, BigInt, int, Object, JSFunctionCallNode)] */ && arg1Value instanceof BigInt) {
BigInt arg1Value_ = (BigInt) arg1Value;
OverloadedBigIntData s2_ = this.overloadedBigInt_cache;
while (s2_ != null) {
if ((arg0Value_.matchesOperatorCounter(s2_.leftOperatorCounter_))) {
return doOverloadedBigInt(arg0Value_, arg1Value_, s2_.leftOperatorCounter_, s2_.operatorImplementation_, s2_.callNode_);
}
s2_ = s2_.next_;
}
}
if ((state_0 & 0b110000) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedString(JSOverloadedOperatorsObject, Object, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedNullish(JSOverloadedOperatorsObject, Object)] */) {
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedString(JSOverloadedOperatorsObject, Object, int, Object, JSFunctionCallNode)] */) {
OverloadedStringData s3_ = this.overloadedString_cache;
while (s3_ != null) {
if ((arg0Value_.matchesOperatorCounter(s3_.leftOperatorCounter_)) && (JSGuards.isString(arg1Value))) {
assert DSLSupport.assertIdempotence((!(isAddition())));
return doOverloadedString(arg0Value_, arg1Value, s3_.leftOperatorCounter_, s3_.operatorImplementation_, s3_.callNode_);
}
s3_ = s3_.next_;
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedNullish(JSOverloadedOperatorsObject, Object)] */) {
if ((JSGuards.isNullOrUndefined(arg1Value))) {
return doOverloadedNullish(arg0Value_, arg1Value);
}
}
}
}
if ((state_0 & 0b1111000000) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doNumberOverloaded(Object, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doBigIntOverloaded(BigInt, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doStringOverloaded(Object, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doNullishOverloaded(Object, JSOverloadedOperatorsObject)] */ && arg1Value instanceof JSOverloadedOperatorsObject) {
JSOverloadedOperatorsObject arg1Value_ = (JSOverloadedOperatorsObject) arg1Value;
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doNumberOverloaded(Object, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] */) {
NumberOverloadedData s5_ = this.numberOverloaded_cache;
while (s5_ != null) {
if ((arg1Value_.matchesOperatorCounter(s5_.rightOperatorCounter_)) && (JSGuards.isNumber(arg0Value))) {
return doNumberOverloaded(arg0Value, arg1Value_, s5_.rightOperatorCounter_, s5_.operatorImplementation_, s5_.callNode_);
}
s5_ = s5_.next_;
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doBigIntOverloaded(BigInt, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] */ && arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
BigIntOverloadedData s6_ = this.bigIntOverloaded_cache;
while (s6_ != null) {
if ((arg1Value_.matchesOperatorCounter(s6_.rightOperatorCounter_))) {
return doBigIntOverloaded(arg0Value_, arg1Value_, s6_.rightOperatorCounter_, s6_.operatorImplementation_, s6_.callNode_);
}
s6_ = s6_.next_;
}
}
if ((state_0 & 0b1100000000) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doStringOverloaded(Object, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] || SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doNullishOverloaded(Object, JSOverloadedOperatorsObject)] */) {
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doStringOverloaded(Object, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] */) {
StringOverloadedData s7_ = this.stringOverloaded_cache;
while (s7_ != null) {
if ((arg1Value_.matchesOperatorCounter(s7_.rightOperatorCounter_)) && (JSGuards.isString(arg0Value))) {
assert DSLSupport.assertIdempotence((!(isAddition())));
return doStringOverloaded(arg0Value, arg1Value_, s7_.rightOperatorCounter_, s7_.operatorImplementation_, s7_.callNode_);
}
s7_ = s7_.next_;
}
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doNullishOverloaded(Object, JSOverloadedOperatorsObject)] */) {
if ((JSGuards.isNullOrUndefined(arg0Value))) {
return doNullishOverloaded(arg0Value, arg1Value_);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doGeneric(Object, Object, JSFunctionCallNode)] */) {
{
JSFunctionCallNode callNode__ = this.generic_callNode_;
if (callNode__ != null) {
return doGeneric(arg0Value, arg1Value, callNode__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Object arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
int oldState_0 = state_0;
try {
if (arg0Value instanceof JSOverloadedOperatorsObject) {
JSOverloadedOperatorsObject arg0Value_ = (JSOverloadedOperatorsObject) arg0Value;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doGeneric(Object, Object, JSFunctionCallNode)] */ && arg1Value instanceof JSOverloadedOperatorsObject) {
JSOverloadedOperatorsObject arg1Value_ = (JSOverloadedOperatorsObject) arg1Value;
while (true) {
int count0_ = 0;
OverloadedOverloadedData s0_ = OVERLOADED_OVERLOADED_CACHE_UPDATER.getVolatile(this);
OverloadedOverloadedData s0_original = s0_;
while (s0_ != null) {
if ((arg0Value_.matchesOperatorCounter(s0_.leftOperatorCounter_)) && (arg1Value_.matchesOperatorCounter(s0_.rightOperatorCounter_))) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
int leftOperatorCounter__ = (arg0Value_.getOperatorCounter());
if ((arg0Value_.matchesOperatorCounter(leftOperatorCounter__))) {
int rightOperatorCounter__ = (arg1Value_.getOperatorCounter());
if ((arg1Value_.matchesOperatorCounter(rightOperatorCounter__)) && count0_ < (DispatchBinaryOperatorNode.LIMIT)) {
s0_ = this.insert(new OverloadedOverloadedData(s0_original));
s0_.leftOperatorCounter_ = leftOperatorCounter__;
s0_.rightOperatorCounter_ = rightOperatorCounter__;
s0_.operatorImplementation_ = (OperatorSet.getOperatorImplementation(arg0Value_, arg1Value_, getOverloadedOperatorName()));
JSFunctionCallNode callNode__1 = s0_.insert((JSFunctionCallNode.createCall()));
Objects.requireNonNull(callNode__1, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s0_.callNode_ = callNode__1;
if (!OVERLOADED_OVERLOADED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedOverloaded(JSOverloadedOperatorsObject, JSOverloadedOperatorsObject, int, int, Object, JSFunctionCallNode)] */;
this.state_0_ = state_0;
}
}
}
}
if (s0_ != null) {
return doOverloadedOverloaded(arg0Value_, arg1Value_, s0_.leftOperatorCounter_, s0_.rightOperatorCounter_, s0_.operatorImplementation_, s0_.callNode_);
}
break;
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doGeneric(Object, Object, JSFunctionCallNode)] */) {
while (true) {
int count1_ = 0;
OverloadedNumberData s1_ = OVERLOADED_NUMBER_CACHE_UPDATER.getVolatile(this);
OverloadedNumberData s1_original = s1_;
while (s1_ != null) {
if ((arg0Value_.matchesOperatorCounter(s1_.leftOperatorCounter_)) && (JSGuards.isNumber(arg1Value))) {
break;
}
count1_++;
s1_ = s1_.next_;
}
if (s1_ == null) {
{
int leftOperatorCounter__1 = (arg0Value_.getOperatorCounter());
if ((arg0Value_.matchesOperatorCounter(leftOperatorCounter__1)) && (JSGuards.isNumber(arg1Value)) && count1_ < (DispatchBinaryOperatorNode.LIMIT)) {
s1_ = this.insert(new OverloadedNumberData(s1_original));
s1_.leftOperatorCounter_ = leftOperatorCounter__1;
s1_.operatorImplementation_ = (OperatorSet.getOperatorImplementation(arg0Value_, arg1Value, getOverloadedOperatorName()));
JSFunctionCallNode callNode__2 = s1_.insert((JSFunctionCallNode.createCall()));
Objects.requireNonNull(callNode__2, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.callNode_ = callNode__2;
if (!OVERLOADED_NUMBER_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedNumber(JSOverloadedOperatorsObject, Object, int, Object, JSFunctionCallNode)] */;
this.state_0_ = state_0;
}
}
}
if (s1_ != null) {
return doOverloadedNumber(arg0Value_, arg1Value, s1_.leftOperatorCounter_, s1_.operatorImplementation_, s1_.callNode_);
}
break;
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doGeneric(Object, Object, JSFunctionCallNode)] */ && arg1Value instanceof BigInt) {
BigInt arg1Value_ = (BigInt) arg1Value;
while (true) {
int count2_ = 0;
OverloadedBigIntData s2_ = OVERLOADED_BIG_INT_CACHE_UPDATER.getVolatile(this);
OverloadedBigIntData s2_original = s2_;
while (s2_ != null) {
if ((arg0Value_.matchesOperatorCounter(s2_.leftOperatorCounter_))) {
break;
}
count2_++;
s2_ = s2_.next_;
}
if (s2_ == null) {
{
int leftOperatorCounter__2 = (arg0Value_.getOperatorCounter());
if ((arg0Value_.matchesOperatorCounter(leftOperatorCounter__2)) && count2_ < (DispatchBinaryOperatorNode.LIMIT)) {
s2_ = this.insert(new OverloadedBigIntData(s2_original));
s2_.leftOperatorCounter_ = leftOperatorCounter__2;
s2_.operatorImplementation_ = (OperatorSet.getOperatorImplementation(arg0Value_, arg1Value_, getOverloadedOperatorName()));
JSFunctionCallNode callNode__3 = s2_.insert((JSFunctionCallNode.createCall()));
Objects.requireNonNull(callNode__3, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s2_.callNode_ = callNode__3;
if (!OVERLOADED_BIG_INT_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedBigInt(JSOverloadedOperatorsObject, BigInt, int, Object, JSFunctionCallNode)] */;
this.state_0_ = state_0;
}
}
}
if (s2_ != null) {
return doOverloadedBigInt(arg0Value_, arg1Value_, s2_.leftOperatorCounter_, s2_.operatorImplementation_, s2_.callNode_);
}
break;
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doGeneric(Object, Object, JSFunctionCallNode)] */) {
while (true) {
int count3_ = 0;
OverloadedStringData s3_ = OVERLOADED_STRING_CACHE_UPDATER.getVolatile(this);
OverloadedStringData s3_original = s3_;
while (s3_ != null) {
if ((arg0Value_.matchesOperatorCounter(s3_.leftOperatorCounter_)) && (JSGuards.isString(arg1Value))) {
assert DSLSupport.assertIdempotence((!(isAddition())));
break;
}
count3_++;
s3_ = s3_.next_;
}
if (s3_ == null) {
{
int leftOperatorCounter__3 = (arg0Value_.getOperatorCounter());
if ((arg0Value_.matchesOperatorCounter(leftOperatorCounter__3)) && (JSGuards.isString(arg1Value)) && (!(isAddition())) && count3_ < (DispatchBinaryOperatorNode.LIMIT)) {
s3_ = this.insert(new OverloadedStringData(s3_original));
s3_.leftOperatorCounter_ = leftOperatorCounter__3;
s3_.operatorImplementation_ = (OperatorSet.getOperatorImplementation(arg0Value_, arg1Value, getOverloadedOperatorName()));
JSFunctionCallNode callNode__4 = s3_.insert((JSFunctionCallNode.createCall()));
Objects.requireNonNull(callNode__4, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s3_.callNode_ = callNode__4;
if (!OVERLOADED_STRING_CACHE_UPDATER.compareAndSet(this, s3_original, s3_)) {
continue;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedString(JSOverloadedOperatorsObject, Object, int, Object, JSFunctionCallNode)] */;
this.state_0_ = state_0;
}
}
}
if (s3_ != null) {
return doOverloadedString(arg0Value_, arg1Value, s3_.leftOperatorCounter_, s3_.operatorImplementation_, s3_.callNode_);
}
break;
}
}
if ((JSGuards.isNullOrUndefined(arg1Value))) {
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedNullish(JSOverloadedOperatorsObject, Object)] */;
this.state_0_ = state_0;
return doOverloadedNullish(arg0Value_, arg1Value);
}
}
if (arg1Value instanceof JSOverloadedOperatorsObject) {
JSOverloadedOperatorsObject arg1Value_ = (JSOverloadedOperatorsObject) arg1Value;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doGeneric(Object, Object, JSFunctionCallNode)] */) {
while (true) {
int count5_ = 0;
NumberOverloadedData s5_ = NUMBER_OVERLOADED_CACHE_UPDATER.getVolatile(this);
NumberOverloadedData s5_original = s5_;
while (s5_ != null) {
if ((arg1Value_.matchesOperatorCounter(s5_.rightOperatorCounter_)) && (JSGuards.isNumber(arg0Value))) {
break;
}
count5_++;
s5_ = s5_.next_;
}
if (s5_ == null) {
{
int rightOperatorCounter__1 = (arg1Value_.getOperatorCounter());
if ((arg1Value_.matchesOperatorCounter(rightOperatorCounter__1)) && (JSGuards.isNumber(arg0Value)) && count5_ < (DispatchBinaryOperatorNode.LIMIT)) {
s5_ = this.insert(new NumberOverloadedData(s5_original));
s5_.rightOperatorCounter_ = rightOperatorCounter__1;
s5_.operatorImplementation_ = (OperatorSet.getOperatorImplementation(arg0Value, arg1Value_, getOverloadedOperatorName()));
JSFunctionCallNode callNode__5 = s5_.insert((JSFunctionCallNode.createCall()));
Objects.requireNonNull(callNode__5, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s5_.callNode_ = callNode__5;
if (!NUMBER_OVERLOADED_CACHE_UPDATER.compareAndSet(this, s5_original, s5_)) {
continue;
}
state_0 = state_0 | 0b1000000 /* add SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doNumberOverloaded(Object, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] */;
this.state_0_ = state_0;
}
}
}
if (s5_ != null) {
return doNumberOverloaded(arg0Value, arg1Value_, s5_.rightOperatorCounter_, s5_.operatorImplementation_, s5_.callNode_);
}
break;
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doGeneric(Object, Object, JSFunctionCallNode)] */ && arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
while (true) {
int count6_ = 0;
BigIntOverloadedData s6_ = BIG_INT_OVERLOADED_CACHE_UPDATER.getVolatile(this);
BigIntOverloadedData s6_original = s6_;
while (s6_ != null) {
if ((arg1Value_.matchesOperatorCounter(s6_.rightOperatorCounter_))) {
break;
}
count6_++;
s6_ = s6_.next_;
}
if (s6_ == null) {
{
int rightOperatorCounter__2 = (arg1Value_.getOperatorCounter());
if ((arg1Value_.matchesOperatorCounter(rightOperatorCounter__2)) && count6_ < (DispatchBinaryOperatorNode.LIMIT)) {
s6_ = this.insert(new BigIntOverloadedData(s6_original));
s6_.rightOperatorCounter_ = rightOperatorCounter__2;
s6_.operatorImplementation_ = (OperatorSet.getOperatorImplementation(arg0Value_, arg1Value_, getOverloadedOperatorName()));
JSFunctionCallNode callNode__6 = s6_.insert((JSFunctionCallNode.createCall()));
Objects.requireNonNull(callNode__6, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s6_.callNode_ = callNode__6;
if (!BIG_INT_OVERLOADED_CACHE_UPDATER.compareAndSet(this, s6_original, s6_)) {
continue;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doBigIntOverloaded(BigInt, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] */;
this.state_0_ = state_0;
}
}
}
if (s6_ != null) {
return doBigIntOverloaded(arg0Value_, arg1Value_, s6_.rightOperatorCounter_, s6_.operatorImplementation_, s6_.callNode_);
}
break;
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doGeneric(Object, Object, JSFunctionCallNode)] */) {
while (true) {
int count7_ = 0;
StringOverloadedData s7_ = STRING_OVERLOADED_CACHE_UPDATER.getVolatile(this);
StringOverloadedData s7_original = s7_;
while (s7_ != null) {
if ((arg1Value_.matchesOperatorCounter(s7_.rightOperatorCounter_)) && (JSGuards.isString(arg0Value))) {
assert DSLSupport.assertIdempotence((!(isAddition())));
break;
}
count7_++;
s7_ = s7_.next_;
}
if (s7_ == null) {
{
int rightOperatorCounter__3 = (arg1Value_.getOperatorCounter());
if ((arg1Value_.matchesOperatorCounter(rightOperatorCounter__3)) && (JSGuards.isString(arg0Value)) && (!(isAddition())) && count7_ < (DispatchBinaryOperatorNode.LIMIT)) {
s7_ = this.insert(new StringOverloadedData(s7_original));
s7_.rightOperatorCounter_ = rightOperatorCounter__3;
s7_.operatorImplementation_ = (OperatorSet.getOperatorImplementation(arg0Value, arg1Value_, getOverloadedOperatorName()));
JSFunctionCallNode callNode__7 = s7_.insert((JSFunctionCallNode.createCall()));
Objects.requireNonNull(callNode__7, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s7_.callNode_ = callNode__7;
if (!STRING_OVERLOADED_CACHE_UPDATER.compareAndSet(this, s7_original, s7_)) {
continue;
}
state_0 = state_0 | 0b100000000 /* add SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doStringOverloaded(Object, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] */;
this.state_0_ = state_0;
}
}
}
if (s7_ != null) {
return doStringOverloaded(arg0Value, arg1Value_, s7_.rightOperatorCounter_, s7_.operatorImplementation_, s7_.callNode_);
}
break;
}
}
if ((JSGuards.isNullOrUndefined(arg0Value))) {
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doNullishOverloaded(Object, JSOverloadedOperatorsObject)] */;
this.state_0_ = state_0;
return doNullishOverloaded(arg0Value, arg1Value_);
}
}
JSFunctionCallNode callNode__ = this.insert((JSFunctionCallNode.createCall()));
Objects.requireNonNull(callNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.generic_callNode_ = callNode__;
this.overloadedOverloaded_cache = null;
this.overloadedNumber_cache = null;
this.overloadedBigInt_cache = null;
this.overloadedString_cache = null;
this.numberOverloaded_cache = null;
this.bigIntOverloaded_cache = null;
this.stringOverloaded_cache = null;
state_0 = state_0 & 0xfffffe22 /* remove SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedOverloaded(JSOverloadedOperatorsObject, JSOverloadedOperatorsObject, int, int, Object, JSFunctionCallNode)], SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedNumber(JSOverloadedOperatorsObject, Object, int, Object, JSFunctionCallNode)], SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedBigInt(JSOverloadedOperatorsObject, BigInt, int, Object, JSFunctionCallNode)], SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doOverloadedString(JSOverloadedOperatorsObject, Object, int, Object, JSFunctionCallNode)], SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doNumberOverloaded(Object, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)], SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doBigIntOverloaded(BigInt, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)], SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doStringOverloaded(Object, JSOverloadedOperatorsObject, int, Object, JSFunctionCallNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSOverloadedBinaryNode.DispatchBinaryOperatorNode.doGeneric(Object, Object, JSFunctionCallNode)] */;
this.state_0_ = state_0;
return doGeneric(arg0Value, arg1Value, callNode__);
} finally {
if (oldState_0 != 0) {
checkForPolymorphicSpecialize(oldState_0);
}
}
}
private void checkForPolymorphicSpecialize(int oldState_0) {
if (((oldState_0 & 0b10) == 0 && (state_0_ & 0b10) != 0)) {
this.reportPolymorphicSpecialize();
}
}
@NeverDefault
public static DispatchBinaryOperatorNode create(TruffleString overloadedOperatorName) {
return new DispatchBinaryOperatorNodeGen(overloadedOperatorName);
}
@GeneratedBy(DispatchBinaryOperatorNode.class)
@DenyReplace
private static final class OverloadedOverloadedData extends Node implements SpecializationDataNode {
@Child OverloadedOverloadedData next_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedOverloaded}
* Parameter: int leftOperatorCounter
*/
@CompilationFinal int leftOperatorCounter_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedOverloaded}
* Parameter: int rightOperatorCounter
*/
@CompilationFinal int rightOperatorCounter_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedOverloaded}
* Parameter: {@link Object} operatorImplementation
*/
@CompilationFinal Object operatorImplementation_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedOverloaded}
* Parameter: {@link JSFunctionCallNode} callNode
*/
@Child JSFunctionCallNode callNode_;
OverloadedOverloadedData(OverloadedOverloadedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(DispatchBinaryOperatorNode.class)
@DenyReplace
private static final class OverloadedNumberData extends Node implements SpecializationDataNode {
@Child OverloadedNumberData next_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedNumber}
* Parameter: int leftOperatorCounter
*/
@CompilationFinal int leftOperatorCounter_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedNumber}
* Parameter: {@link Object} operatorImplementation
*/
@CompilationFinal Object operatorImplementation_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedNumber}
* Parameter: {@link JSFunctionCallNode} callNode
*/
@Child JSFunctionCallNode callNode_;
OverloadedNumberData(OverloadedNumberData next_) {
this.next_ = next_;
}
}
@GeneratedBy(DispatchBinaryOperatorNode.class)
@DenyReplace
private static final class OverloadedBigIntData extends Node implements SpecializationDataNode {
@Child OverloadedBigIntData next_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedBigInt}
* Parameter: int leftOperatorCounter
*/
@CompilationFinal int leftOperatorCounter_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedBigInt}
* Parameter: {@link Object} operatorImplementation
*/
@CompilationFinal Object operatorImplementation_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedBigInt}
* Parameter: {@link JSFunctionCallNode} callNode
*/
@Child JSFunctionCallNode callNode_;
OverloadedBigIntData(OverloadedBigIntData next_) {
this.next_ = next_;
}
}
@GeneratedBy(DispatchBinaryOperatorNode.class)
@DenyReplace
private static final class OverloadedStringData extends Node implements SpecializationDataNode {
@Child OverloadedStringData next_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedString}
* Parameter: int leftOperatorCounter
*/
@CompilationFinal int leftOperatorCounter_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedString}
* Parameter: {@link Object} operatorImplementation
*/
@CompilationFinal Object operatorImplementation_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doOverloadedString}
* Parameter: {@link JSFunctionCallNode} callNode
*/
@Child JSFunctionCallNode callNode_;
OverloadedStringData(OverloadedStringData next_) {
this.next_ = next_;
}
}
@GeneratedBy(DispatchBinaryOperatorNode.class)
@DenyReplace
private static final class NumberOverloadedData extends Node implements SpecializationDataNode {
@Child NumberOverloadedData next_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doNumberOverloaded}
* Parameter: int rightOperatorCounter
*/
@CompilationFinal int rightOperatorCounter_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doNumberOverloaded}
* Parameter: {@link Object} operatorImplementation
*/
@CompilationFinal Object operatorImplementation_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doNumberOverloaded}
* Parameter: {@link JSFunctionCallNode} callNode
*/
@Child JSFunctionCallNode callNode_;
NumberOverloadedData(NumberOverloadedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(DispatchBinaryOperatorNode.class)
@DenyReplace
private static final class BigIntOverloadedData extends Node implements SpecializationDataNode {
@Child BigIntOverloadedData next_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doBigIntOverloaded}
* Parameter: int rightOperatorCounter
*/
@CompilationFinal int rightOperatorCounter_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doBigIntOverloaded}
* Parameter: {@link Object} operatorImplementation
*/
@CompilationFinal Object operatorImplementation_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doBigIntOverloaded}
* Parameter: {@link JSFunctionCallNode} callNode
*/
@Child JSFunctionCallNode callNode_;
BigIntOverloadedData(BigIntOverloadedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(DispatchBinaryOperatorNode.class)
@DenyReplace
private static final class StringOverloadedData extends Node implements SpecializationDataNode {
@Child StringOverloadedData next_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doStringOverloaded}
* Parameter: int rightOperatorCounter
*/
@CompilationFinal int rightOperatorCounter_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doStringOverloaded}
* Parameter: {@link Object} operatorImplementation
*/
@CompilationFinal Object operatorImplementation_;
/**
* Source Info:
* Specialization: {@link DispatchBinaryOperatorNode#doStringOverloaded}
* Parameter: {@link JSFunctionCallNode} callNode
*/
@Child JSFunctionCallNode callNode_;
StringOverloadedData(StringOverloadedData next_) {
this.next_ = next_;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy