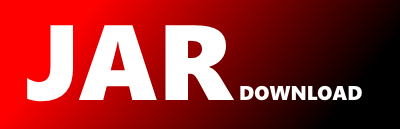
com.oracle.truffle.js.nodes.cast.JSNumberToBigIntNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.cast;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.js.nodes.JSTypesGen;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.SafeInteger;
/**
* Debug Info:
* Specialization {@link JSNumberToBigIntNode#doInteger}
* Activation probability: 0.32000
* With/without class size: 7/0 bytes
* Specialization {@link JSNumberToBigIntNode#doSafeInteger}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link JSNumberToBigIntNode#doDoubleAsLong}
* Activation probability: 0.20000
* With/without class size: 6/0 bytes
* Specialization {@link JSNumberToBigIntNode#doDoubleOther}
* Activation probability: 0.14000
* With/without class size: 5/0 bytes
* Specialization {@link JSNumberToBigIntNode#doOtherType}
* Activation probability: 0.08000
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(JSNumberToBigIntNode.class)
@SuppressWarnings("javadoc")
public final class JSNumberToBigIntNodeGen extends JSNumberToBigIntNode {
/**
* State Info:
* 0: SpecializationActive {@link JSNumberToBigIntNode#doInteger}
* 1: SpecializationActive {@link JSNumberToBigIntNode#doSafeInteger}
* 2: SpecializationActive {@link JSNumberToBigIntNode#doDoubleAsLong}
* 3: SpecializationActive {@link JSNumberToBigIntNode#doDoubleOther}
* 4: SpecializationActive {@link JSNumberToBigIntNode#doOtherType}
* 5-7: ImplicitCast[type=double, index=0]
*
*/
@CompilationFinal private int state_0_;
private JSNumberToBigIntNodeGen() {
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Object arg0Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[JSNumberToBigIntNode.doInteger(int)] */) && arg0Value instanceof Integer) {
return false;
}
if (!((state_0 & 0b10) != 0 /* is SpecializationActive[JSNumberToBigIntNode.doSafeInteger(SafeInteger)] */) && arg0Value instanceof SafeInteger) {
return false;
}
if (JSTypesGen.isImplicitDouble(arg0Value)) {
{
double arg0Value_ = JSTypesGen.asImplicitDouble(arg0Value);
if ((JSNumberToBigIntNode.doubleRepresentsSameValueAsLong(arg0Value_))) {
return false;
}
}
{
double arg0Value_ = JSTypesGen.asImplicitDouble(arg0Value);
if ((!(JSNumberToBigIntNode.doubleRepresentsSameValueAsLong(arg0Value_)))) {
return false;
}
}
}
return true;
}
@Override
protected BigInt execute(Object arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111) != 0 /* is SpecializationActive[JSNumberToBigIntNode.doInteger(int)] || SpecializationActive[JSNumberToBigIntNode.doSafeInteger(SafeInteger)] || SpecializationActive[JSNumberToBigIntNode.doDoubleAsLong(double)] || SpecializationActive[JSNumberToBigIntNode.doDoubleOther(double)] || SpecializationActive[JSNumberToBigIntNode.doOtherType(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSNumberToBigIntNode.doInteger(int)] */ && arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
return JSNumberToBigIntNode.doInteger(arg0Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSNumberToBigIntNode.doSafeInteger(SafeInteger)] */ && arg0Value instanceof SafeInteger) {
SafeInteger arg0Value_ = (SafeInteger) arg0Value;
return JSNumberToBigIntNode.doSafeInteger(arg0Value_);
}
if ((state_0 & 0b1100) != 0 /* is SpecializationActive[JSNumberToBigIntNode.doDoubleAsLong(double)] || SpecializationActive[JSNumberToBigIntNode.doDoubleOther(double)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b11100000) >>> 5 /* get-int ImplicitCast[type=double, index=0] */, arg0Value)) {
double arg0Value_ = JSTypesGen.asImplicitDouble((state_0 & 0b11100000) >>> 5 /* get-int ImplicitCast[type=double, index=0] */, arg0Value);
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSNumberToBigIntNode.doDoubleAsLong(double)] */) {
if ((JSNumberToBigIntNode.doubleRepresentsSameValueAsLong(arg0Value_))) {
return JSNumberToBigIntNode.doDoubleAsLong(arg0Value_);
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSNumberToBigIntNode.doDoubleOther(double)] */) {
if ((!(JSNumberToBigIntNode.doubleRepresentsSameValueAsLong(arg0Value_)))) {
return JSNumberToBigIntNode.doDoubleOther(arg0Value_);
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSNumberToBigIntNode.doOtherType(Object)] */) {
if (fallbackGuard_(state_0, arg0Value)) {
return JSNumberToBigIntNode.doOtherType(arg0Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private BigInt executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSNumberToBigIntNode.doInteger(int)] */;
this.state_0_ = state_0;
return JSNumberToBigIntNode.doInteger(arg0Value_);
}
if (arg0Value instanceof SafeInteger) {
SafeInteger arg0Value_ = (SafeInteger) arg0Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSNumberToBigIntNode.doSafeInteger(SafeInteger)] */;
this.state_0_ = state_0;
return JSNumberToBigIntNode.doSafeInteger(arg0Value_);
}
{
int doubleCast0;
if ((doubleCast0 = JSTypesGen.specializeImplicitDouble(arg0Value)) != 0) {
double arg0Value_ = JSTypesGen.asImplicitDouble(doubleCast0, arg0Value);
if ((JSNumberToBigIntNode.doubleRepresentsSameValueAsLong(arg0Value_))) {
state_0 = (state_0 | (doubleCast0 << 5) /* set-int ImplicitCast[type=double, index=0] */);
state_0 = state_0 | 0b100 /* add SpecializationActive[JSNumberToBigIntNode.doDoubleAsLong(double)] */;
this.state_0_ = state_0;
return JSNumberToBigIntNode.doDoubleAsLong(arg0Value_);
}
if ((!(JSNumberToBigIntNode.doubleRepresentsSameValueAsLong(arg0Value_)))) {
state_0 = (state_0 | (doubleCast0 << 5) /* set-int ImplicitCast[type=double, index=0] */);
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSNumberToBigIntNode.doDoubleOther(double)] */;
this.state_0_ = state_0;
return JSNumberToBigIntNode.doDoubleOther(arg0Value_);
}
}
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSNumberToBigIntNode.doOtherType(Object)] */;
this.state_0_ = state_0;
return JSNumberToBigIntNode.doOtherType(arg0Value);
}
@NeverDefault
public static JSNumberToBigIntNode create() {
return new JSNumberToBigIntNodeGen();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy