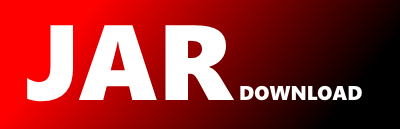
com.oracle.truffle.js.nodes.cast.JSStringToNumberNoTrimNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.cast;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.SlowPathException;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.ReadCharUTF16Node;
import com.oracle.truffle.api.strings.TruffleString.RegionEqualByteIndexNode;
import com.oracle.truffle.js.nodes.JSGuards;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSStringToNumberNoTrimNode#doLengthIsZero}
* Activation probability: 0.14679
* With/without class size: 5/0 bytes
* Specialization {@link JSStringToNumberNoTrimNode#doInfinity}
* Activation probability: 0.13526
* With/without class size: 7/5 bytes
* Specialization {@link JSStringToNumberNoTrimNode#doNaN}
* Activation probability: 0.12372
* With/without class size: 5/0 bytes
* Specialization {@link JSStringToNumberNoTrimNode#doHexSafe}
* Activation probability: 0.11218
* With/without class size: 5/0 bytes
* Specialization {@link JSStringToNumberNoTrimNode#doHex}
* Activation probability: 0.10064
* With/without class size: 5/0 bytes
* Specialization {@link JSStringToNumberNoTrimNode#doOctalSafe}
* Activation probability: 0.08910
* With/without class size: 5/0 bytes
* Specialization {@link JSStringToNumberNoTrimNode#doOctal}
* Activation probability: 0.07756
* With/without class size: 4/0 bytes
* Specialization {@link JSStringToNumberNoTrimNode#doBinarySafe}
* Activation probability: 0.06603
* With/without class size: 4/0 bytes
* Specialization {@link JSStringToNumberNoTrimNode#doBinary}
* Activation probability: 0.05449
* With/without class size: 4/0 bytes
* Specialization {@link JSStringToNumberNoTrimNode#doSmallPosInt}
* Activation probability: 0.04295
* With/without class size: 4/0 bytes
* Specialization {@link JSStringToNumberNoTrimNode#doInteger}
* Activation probability: 0.03141
* With/without class size: 4/0 bytes
* Specialization {@link JSStringToNumberNoTrimNode#doDouble}
* Activation probability: 0.01987
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(JSStringToNumberNoTrimNode.class)
@SuppressWarnings("javadoc")
final class JSStringToNumberNoTrimNodeGen extends JSStringToNumberNoTrimNode {
private static final StateField STATE_0_JSStringToNumberNoTrimNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link JSStringToNumberNoTrimNode#doInfinity}
* Parameter: {@link InlinedConditionProfile} endsWithInfinity
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_INFINITY_ENDS_WITH_INFINITY_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_JSStringToNumberNoTrimNode_UPDATER.subUpdater(13, 2)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link JSStringToNumberNoTrimNode#doLengthIsZero}
* 1: SpecializationActive {@link JSStringToNumberNoTrimNode#doInfinity}
* 2: SpecializationActive {@link JSStringToNumberNoTrimNode#doNaN}
* 3: SpecializationActive {@link JSStringToNumberNoTrimNode#doHexSafe}
* 4: SpecializationActive {@link JSStringToNumberNoTrimNode#doHex}
* 5: SpecializationActive {@link JSStringToNumberNoTrimNode#doOctalSafe}
* 6: SpecializationActive {@link JSStringToNumberNoTrimNode#doOctal}
* 7: SpecializationActive {@link JSStringToNumberNoTrimNode#doBinarySafe}
* 8: SpecializationActive {@link JSStringToNumberNoTrimNode#doBinary}
* 9: SpecializationActive {@link JSStringToNumberNoTrimNode#doSmallPosInt}
* 10: SpecializationActive {@link JSStringToNumberNoTrimNode#doInteger}
* 11: SpecializationExcluded {@link JSStringToNumberNoTrimNode#doInteger}
* 12: SpecializationActive {@link JSStringToNumberNoTrimNode#doDouble}
* 13-14: InlinedCache
* Specialization: {@link JSStringToNumberNoTrimNode#doInfinity}
* Parameter: {@link InlinedConditionProfile} endsWithInfinity
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link JSStringToNumberNoTrimNode#doInfinity}
* Parameter: {@link ReadCharUTF16Node} readChar
*/
@Child private ReadCharUTF16Node readChar;
/**
* Source Info:
* Specialization: {@link JSStringToNumberNoTrimNode#doInfinity}
* Parameter: {@link RegionEqualByteIndexNode} regionEqualsNode
*/
@Child private RegionEqualByteIndexNode infinity_regionEqualsNode_;
private JSStringToNumberNoTrimNodeGen() {
}
@Override
double executeNoTrim(TruffleString arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1011111111111) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doLengthIsZero(TruffleString)] || SpecializationActive[JSStringToNumberNoTrimNode.doInfinity(TruffleString, Node, InlinedConditionProfile, RegionEqualByteIndexNode, ReadCharUTF16Node)] || SpecializationActive[JSStringToNumberNoTrimNode.doNaN(TruffleString, ReadCharUTF16Node)] || SpecializationActive[JSStringToNumberNoTrimNode.doHexSafe(TruffleString, ReadCharUTF16Node)] || SpecializationActive[JSStringToNumberNoTrimNode.doHex(TruffleString, ReadCharUTF16Node)] || SpecializationActive[JSStringToNumberNoTrimNode.doOctalSafe(TruffleString, ReadCharUTF16Node)] || SpecializationActive[JSStringToNumberNoTrimNode.doOctal(TruffleString, ReadCharUTF16Node)] || SpecializationActive[JSStringToNumberNoTrimNode.doBinarySafe(TruffleString, ReadCharUTF16Node)] || SpecializationActive[JSStringToNumberNoTrimNode.doBinary(TruffleString, ReadCharUTF16Node)] || SpecializationActive[JSStringToNumberNoTrimNode.doSmallPosInt(TruffleString, ReadCharUTF16Node)] || SpecializationActive[JSStringToNumberNoTrimNode.doInteger(TruffleString, ReadCharUTF16Node)] || SpecializationActive[JSStringToNumberNoTrimNode.doDouble(TruffleString, ReadCharUTF16Node)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doLengthIsZero(TruffleString)] */) {
if ((JSGuards.stringLength(arg0Value) == 0)) {
return JSStringToNumberNoTrimNode.doLengthIsZero(arg0Value);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doInfinity(TruffleString, Node, InlinedConditionProfile, RegionEqualByteIndexNode, ReadCharUTF16Node)] */) {
{
RegionEqualByteIndexNode regionEqualsNode__ = this.infinity_regionEqualsNode_;
if (regionEqualsNode__ != null) {
ReadCharUTF16Node readChar_ = this.readChar;
if (readChar_ != null) {
if ((JSStringToNumberNoTrimNode.startsWithI(arg0Value, readChar_))) {
Node node__ = (this);
return JSStringToNumberNoTrimNode.doInfinity(arg0Value, node__, INLINED_INFINITY_ENDS_WITH_INFINITY_, regionEqualsNode__, readChar_);
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doNaN(TruffleString, ReadCharUTF16Node)] */) {
{
ReadCharUTF16Node readChar_1 = this.readChar;
if (readChar_1 != null) {
if ((JSGuards.stringLength(arg0Value) > 0) && (!(JSStringToNumberNoTrimNode.startsWithI(arg0Value, readChar_1))) && (!(JSStringToNumberNoTrimNode.startsWithValidDouble(arg0Value, readChar_1))) && (!(JSStringToNumberNoTrimNode.isHex(arg0Value, readChar_1))) && (!(JSStringToNumberNoTrimNode.isOctal(arg0Value, readChar_1))) && (!(JSStringToNumberNoTrimNode.isBinary(arg0Value, readChar_1)))) {
return JSStringToNumberNoTrimNode.doNaN(arg0Value, readChar_1);
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doHexSafe(TruffleString, ReadCharUTF16Node)] */) {
{
ReadCharUTF16Node readChar_2 = this.readChar;
if (readChar_2 != null) {
if ((JSStringToNumberNoTrimNode.isHex(arg0Value, readChar_2)) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.SAFE_HEX_DIGITS)) {
return JSStringToNumberNoTrimNode.doHexSafe(arg0Value, readChar_2);
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doHex(TruffleString, ReadCharUTF16Node)] */) {
{
ReadCharUTF16Node readChar_3 = this.readChar;
if (readChar_3 != null) {
if ((JSStringToNumberNoTrimNode.isHex(arg0Value, readChar_3)) && (JSGuards.stringLength(arg0Value) > JSStringToNumberNoTrimNode.SAFE_HEX_DIGITS)) {
return JSStringToNumberNoTrimNode.doHex(arg0Value, readChar_3);
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doOctalSafe(TruffleString, ReadCharUTF16Node)] */) {
{
ReadCharUTF16Node readChar_4 = this.readChar;
if (readChar_4 != null) {
if ((JSStringToNumberNoTrimNode.isOctal(arg0Value, readChar_4)) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.SAFE_OCTAL_DIGITS)) {
return JSStringToNumberNoTrimNode.doOctalSafe(arg0Value, readChar_4);
}
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doOctal(TruffleString, ReadCharUTF16Node)] */) {
{
ReadCharUTF16Node readChar_5 = this.readChar;
if (readChar_5 != null) {
if ((JSStringToNumberNoTrimNode.isOctal(arg0Value, readChar_5)) && (JSGuards.stringLength(arg0Value) > JSStringToNumberNoTrimNode.SAFE_OCTAL_DIGITS)) {
return JSStringToNumberNoTrimNode.doOctal(arg0Value, readChar_5);
}
}
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doBinarySafe(TruffleString, ReadCharUTF16Node)] */) {
{
ReadCharUTF16Node readChar_6 = this.readChar;
if (readChar_6 != null) {
if ((JSStringToNumberNoTrimNode.isBinary(arg0Value, readChar_6)) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.SAFE_BINARY_DIGITS)) {
return JSStringToNumberNoTrimNode.doBinarySafe(arg0Value, readChar_6);
}
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doBinary(TruffleString, ReadCharUTF16Node)] */) {
{
ReadCharUTF16Node readChar_7 = this.readChar;
if (readChar_7 != null) {
if ((JSStringToNumberNoTrimNode.isBinary(arg0Value, readChar_7)) && (JSGuards.stringLength(arg0Value) > JSStringToNumberNoTrimNode.SAFE_BINARY_DIGITS)) {
return JSStringToNumberNoTrimNode.doBinary(arg0Value, readChar_7);
}
}
}
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doSmallPosInt(TruffleString, ReadCharUTF16Node)] */) {
{
ReadCharUTF16Node readChar_8 = this.readChar;
if (readChar_8 != null) {
if ((JSGuards.stringLength(arg0Value) > 0) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.SMALL_INT_LENGTH) && (JSStringToNumberNoTrimNode.allDigits(arg0Value, JSStringToNumberNoTrimNode.SMALL_INT_LENGTH, readChar_8))) {
return JSStringToNumberNoTrimNode.doSmallPosInt(arg0Value, readChar_8);
}
}
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doInteger(TruffleString, ReadCharUTF16Node)] */) {
{
ReadCharUTF16Node readChar_9 = this.readChar;
if (readChar_9 != null) {
if ((JSGuards.stringLength(arg0Value) > 0) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.MAX_SAFE_INTEGER_LENGTH) && (JSStringToNumberNoTrimNode.startsWithValidInt(arg0Value, readChar_9))) {
try {
return JSStringToNumberNoTrimNode.doInteger(arg0Value, readChar_9);
} catch (SlowPathException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_;
state_0 = state_0 & 0xfffffbff /* remove SpecializationActive[JSStringToNumberNoTrimNode.doInteger(TruffleString, ReadCharUTF16Node)] */;
state_0 = state_0 | 0b100000000000 /* add SpecializationExcluded */;
this.state_0_ = state_0;
return executeAndSpecialize(arg0Value);
}
}
}
}
}
if ((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[JSStringToNumberNoTrimNode.doDouble(TruffleString, ReadCharUTF16Node)] */) {
{
ReadCharUTF16Node readChar_10 = this.readChar;
if (readChar_10 != null) {
if ((JSGuards.stringLength(arg0Value) > 0) && (JSStringToNumberNoTrimNode.startsWithValidDouble(arg0Value, readChar_10))) {
return JSStringToNumberNoTrimNode.doDouble(arg0Value, readChar_10);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private double executeAndSpecialize(TruffleString arg0Value) {
int state_0 = this.state_0_;
if ((JSGuards.stringLength(arg0Value) == 0)) {
state_0 = state_0 | 0b1 /* add SpecializationActive[JSStringToNumberNoTrimNode.doLengthIsZero(TruffleString)] */;
this.state_0_ = state_0;
return JSStringToNumberNoTrimNode.doLengthIsZero(arg0Value);
}
{
Node node__ = null;
{
ReadCharUTF16Node readChar_;
ReadCharUTF16Node readChar__shared = this.readChar;
if (readChar__shared != null) {
readChar_ = readChar__shared;
} else {
readChar_ = this.insert((ReadCharUTF16Node.create()));
if (readChar_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((JSStringToNumberNoTrimNode.startsWithI(arg0Value, readChar_))) {
node__ = (this);
RegionEqualByteIndexNode regionEqualsNode__ = this.insert((RegionEqualByteIndexNode.create()));
Objects.requireNonNull(regionEqualsNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.infinity_regionEqualsNode_ = regionEqualsNode__;
if (this.readChar == null) {
VarHandle.storeStoreFence();
this.readChar = readChar_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[JSStringToNumberNoTrimNode.doInfinity(TruffleString, Node, InlinedConditionProfile, RegionEqualByteIndexNode, ReadCharUTF16Node)] */;
this.state_0_ = state_0;
return JSStringToNumberNoTrimNode.doInfinity(arg0Value, node__, INLINED_INFINITY_ENDS_WITH_INFINITY_, regionEqualsNode__, readChar_);
}
}
}
if ((JSGuards.stringLength(arg0Value) > 0)) {
ReadCharUTF16Node readChar_1;
ReadCharUTF16Node readChar_1_shared = this.readChar;
if (readChar_1_shared != null) {
readChar_1 = readChar_1_shared;
} else {
readChar_1 = this.insert((ReadCharUTF16Node.create()));
if (readChar_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((!(JSStringToNumberNoTrimNode.startsWithI(arg0Value, readChar_1))) && (!(JSStringToNumberNoTrimNode.startsWithValidDouble(arg0Value, readChar_1))) && (!(JSStringToNumberNoTrimNode.isHex(arg0Value, readChar_1))) && (!(JSStringToNumberNoTrimNode.isOctal(arg0Value, readChar_1))) && (!(JSStringToNumberNoTrimNode.isBinary(arg0Value, readChar_1)))) {
if (this.readChar == null) {
VarHandle.storeStoreFence();
this.readChar = readChar_1;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[JSStringToNumberNoTrimNode.doNaN(TruffleString, ReadCharUTF16Node)] */;
this.state_0_ = state_0;
return JSStringToNumberNoTrimNode.doNaN(arg0Value, readChar_1);
}
}
{
ReadCharUTF16Node readChar_2;
ReadCharUTF16Node readChar_2_shared = this.readChar;
if (readChar_2_shared != null) {
readChar_2 = readChar_2_shared;
} else {
readChar_2 = this.insert((ReadCharUTF16Node.create()));
if (readChar_2 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((JSStringToNumberNoTrimNode.isHex(arg0Value, readChar_2)) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.SAFE_HEX_DIGITS)) {
if (this.readChar == null) {
VarHandle.storeStoreFence();
this.readChar = readChar_2;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSStringToNumberNoTrimNode.doHexSafe(TruffleString, ReadCharUTF16Node)] */;
this.state_0_ = state_0;
return JSStringToNumberNoTrimNode.doHexSafe(arg0Value, readChar_2);
}
}
{
ReadCharUTF16Node readChar_3;
ReadCharUTF16Node readChar_3_shared = this.readChar;
if (readChar_3_shared != null) {
readChar_3 = readChar_3_shared;
} else {
readChar_3 = this.insert((ReadCharUTF16Node.create()));
if (readChar_3 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((JSStringToNumberNoTrimNode.isHex(arg0Value, readChar_3)) && (JSGuards.stringLength(arg0Value) > JSStringToNumberNoTrimNode.SAFE_HEX_DIGITS)) {
if (this.readChar == null) {
VarHandle.storeStoreFence();
this.readChar = readChar_3;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSStringToNumberNoTrimNode.doHex(TruffleString, ReadCharUTF16Node)] */;
this.state_0_ = state_0;
return JSStringToNumberNoTrimNode.doHex(arg0Value, readChar_3);
}
}
{
ReadCharUTF16Node readChar_4;
ReadCharUTF16Node readChar_4_shared = this.readChar;
if (readChar_4_shared != null) {
readChar_4 = readChar_4_shared;
} else {
readChar_4 = this.insert((ReadCharUTF16Node.create()));
if (readChar_4 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((JSStringToNumberNoTrimNode.isOctal(arg0Value, readChar_4)) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.SAFE_OCTAL_DIGITS)) {
if (this.readChar == null) {
VarHandle.storeStoreFence();
this.readChar = readChar_4;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSStringToNumberNoTrimNode.doOctalSafe(TruffleString, ReadCharUTF16Node)] */;
this.state_0_ = state_0;
return JSStringToNumberNoTrimNode.doOctalSafe(arg0Value, readChar_4);
}
}
{
ReadCharUTF16Node readChar_5;
ReadCharUTF16Node readChar_5_shared = this.readChar;
if (readChar_5_shared != null) {
readChar_5 = readChar_5_shared;
} else {
readChar_5 = this.insert((ReadCharUTF16Node.create()));
if (readChar_5 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((JSStringToNumberNoTrimNode.isOctal(arg0Value, readChar_5)) && (JSGuards.stringLength(arg0Value) > JSStringToNumberNoTrimNode.SAFE_OCTAL_DIGITS)) {
if (this.readChar == null) {
VarHandle.storeStoreFence();
this.readChar = readChar_5;
}
state_0 = state_0 | 0b1000000 /* add SpecializationActive[JSStringToNumberNoTrimNode.doOctal(TruffleString, ReadCharUTF16Node)] */;
this.state_0_ = state_0;
return JSStringToNumberNoTrimNode.doOctal(arg0Value, readChar_5);
}
}
{
ReadCharUTF16Node readChar_6;
ReadCharUTF16Node readChar_6_shared = this.readChar;
if (readChar_6_shared != null) {
readChar_6 = readChar_6_shared;
} else {
readChar_6 = this.insert((ReadCharUTF16Node.create()));
if (readChar_6 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((JSStringToNumberNoTrimNode.isBinary(arg0Value, readChar_6)) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.SAFE_BINARY_DIGITS)) {
if (this.readChar == null) {
VarHandle.storeStoreFence();
this.readChar = readChar_6;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[JSStringToNumberNoTrimNode.doBinarySafe(TruffleString, ReadCharUTF16Node)] */;
this.state_0_ = state_0;
return JSStringToNumberNoTrimNode.doBinarySafe(arg0Value, readChar_6);
}
}
{
ReadCharUTF16Node readChar_7;
ReadCharUTF16Node readChar_7_shared = this.readChar;
if (readChar_7_shared != null) {
readChar_7 = readChar_7_shared;
} else {
readChar_7 = this.insert((ReadCharUTF16Node.create()));
if (readChar_7 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((JSStringToNumberNoTrimNode.isBinary(arg0Value, readChar_7)) && (JSGuards.stringLength(arg0Value) > JSStringToNumberNoTrimNode.SAFE_BINARY_DIGITS)) {
if (this.readChar == null) {
VarHandle.storeStoreFence();
this.readChar = readChar_7;
}
state_0 = state_0 | 0b100000000 /* add SpecializationActive[JSStringToNumberNoTrimNode.doBinary(TruffleString, ReadCharUTF16Node)] */;
this.state_0_ = state_0;
return JSStringToNumberNoTrimNode.doBinary(arg0Value, readChar_7);
}
}
if (((state_0 & 0b1010000000000)) == 0 /* is-not SpecializationActive[JSStringToNumberNoTrimNode.doInteger(TruffleString, ReadCharUTF16Node)] && SpecializationActive[JSStringToNumberNoTrimNode.doDouble(TruffleString, ReadCharUTF16Node)] */) {
if ((JSGuards.stringLength(arg0Value) > 0) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.SMALL_INT_LENGTH)) {
ReadCharUTF16Node readChar_8;
ReadCharUTF16Node readChar_8_shared = this.readChar;
if (readChar_8_shared != null) {
readChar_8 = readChar_8_shared;
} else {
readChar_8 = this.insert((ReadCharUTF16Node.create()));
if (readChar_8 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((JSStringToNumberNoTrimNode.allDigits(arg0Value, JSStringToNumberNoTrimNode.SMALL_INT_LENGTH, readChar_8))) {
if (this.readChar == null) {
VarHandle.storeStoreFence();
this.readChar = readChar_8;
}
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[JSStringToNumberNoTrimNode.doSmallPosInt(TruffleString, ReadCharUTF16Node)] */;
this.state_0_ = state_0;
return JSStringToNumberNoTrimNode.doSmallPosInt(arg0Value, readChar_8);
}
}
}
if (((state_0 & 0b1000000000000)) == 0 /* is-not SpecializationActive[JSStringToNumberNoTrimNode.doDouble(TruffleString, ReadCharUTF16Node)] */ && ((state_0 & 0b100000000000)) == 0 /* is-not SpecializationExcluded */) {
if ((JSGuards.stringLength(arg0Value) > 0) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.MAX_SAFE_INTEGER_LENGTH)) {
ReadCharUTF16Node readChar_9;
ReadCharUTF16Node readChar_9_shared = this.readChar;
if (readChar_9_shared != null) {
readChar_9 = readChar_9_shared;
} else {
readChar_9 = this.insert((ReadCharUTF16Node.create()));
if (readChar_9 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((JSStringToNumberNoTrimNode.startsWithValidInt(arg0Value, readChar_9))) {
if (this.readChar == null) {
VarHandle.storeStoreFence();
this.readChar = readChar_9;
}
state_0 = state_0 & 0xfffffdff /* remove SpecializationActive[JSStringToNumberNoTrimNode.doSmallPosInt(TruffleString, ReadCharUTF16Node)] */;
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[JSStringToNumberNoTrimNode.doInteger(TruffleString, ReadCharUTF16Node)] */;
this.state_0_ = state_0;
try {
return JSStringToNumberNoTrimNode.doInteger(arg0Value, readChar_9);
} catch (SlowPathException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_;
state_0 = state_0 & 0xfffffbff /* remove SpecializationActive[JSStringToNumberNoTrimNode.doInteger(TruffleString, ReadCharUTF16Node)] */;
state_0 = state_0 | 0b100000000000 /* add SpecializationExcluded */;
this.state_0_ = state_0;
return executeAndSpecialize(arg0Value);
}
}
}
}
if ((JSGuards.stringLength(arg0Value) > 0)) {
ReadCharUTF16Node readChar_10;
ReadCharUTF16Node readChar_10_shared = this.readChar;
if (readChar_10_shared != null) {
readChar_10 = readChar_10_shared;
} else {
readChar_10 = this.insert((ReadCharUTF16Node.create()));
if (readChar_10 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((JSStringToNumberNoTrimNode.startsWithValidDouble(arg0Value, readChar_10))) {
if (this.readChar == null) {
VarHandle.storeStoreFence();
this.readChar = readChar_10;
}
state_0 = state_0 & 0xfffff9ff /* remove SpecializationActive[JSStringToNumberNoTrimNode.doSmallPosInt(TruffleString, ReadCharUTF16Node)], SpecializationActive[JSStringToNumberNoTrimNode.doInteger(TruffleString, ReadCharUTF16Node)] */;
state_0 = state_0 | 0b1000000000000 /* add SpecializationActive[JSStringToNumberNoTrimNode.doDouble(TruffleString, ReadCharUTF16Node)] */;
this.state_0_ = state_0;
return JSStringToNumberNoTrimNode.doDouble(arg0Value, readChar_10);
}
}
throw new UnsupportedSpecializationException(this, null, arg0Value);
}
@TruffleBoundary
private static UnsupportedSpecializationException newUnsupportedSpecializationException1(Node thisNode_, Object arg0Value) {
return new UnsupportedSpecializationException(thisNode_, null, arg0Value);
}
@NeverDefault
public static JSStringToNumberNoTrimNode create() {
return new JSStringToNumberNoTrimNodeGen();
}
@NeverDefault
public static JSStringToNumberNoTrimNode getUncached() {
return JSStringToNumberNoTrimNodeGen.UNCACHED;
}
@GeneratedBy(JSStringToNumberNoTrimNode.class)
@DenyReplace
private static final class Uncached extends JSStringToNumberNoTrimNode implements UnadoptableNode {
@TruffleBoundary
@Override
double executeNoTrim(TruffleString arg0Value) {
if ((JSGuards.stringLength(arg0Value) == 0)) {
return JSStringToNumberNoTrimNode.doLengthIsZero(arg0Value);
}
if ((JSStringToNumberNoTrimNode.startsWithI(arg0Value, (ReadCharUTF16Node.getUncached())))) {
return JSStringToNumberNoTrimNode.doInfinity(arg0Value, (this), (InlinedConditionProfile.getUncached()), (RegionEqualByteIndexNode.getUncached()), (ReadCharUTF16Node.getUncached()));
}
if ((JSGuards.stringLength(arg0Value) > 0) && (!(JSStringToNumberNoTrimNode.startsWithI(arg0Value, (ReadCharUTF16Node.getUncached())))) && (!(JSStringToNumberNoTrimNode.startsWithValidDouble(arg0Value, (ReadCharUTF16Node.getUncached())))) && (!(JSStringToNumberNoTrimNode.isHex(arg0Value, (ReadCharUTF16Node.getUncached())))) && (!(JSStringToNumberNoTrimNode.isOctal(arg0Value, (ReadCharUTF16Node.getUncached())))) && (!(JSStringToNumberNoTrimNode.isBinary(arg0Value, (ReadCharUTF16Node.getUncached()))))) {
return JSStringToNumberNoTrimNode.doNaN(arg0Value, (ReadCharUTF16Node.getUncached()));
}
if ((JSStringToNumberNoTrimNode.isHex(arg0Value, (ReadCharUTF16Node.getUncached()))) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.SAFE_HEX_DIGITS)) {
return JSStringToNumberNoTrimNode.doHexSafe(arg0Value, (ReadCharUTF16Node.getUncached()));
}
if ((JSStringToNumberNoTrimNode.isHex(arg0Value, (ReadCharUTF16Node.getUncached()))) && (JSGuards.stringLength(arg0Value) > JSStringToNumberNoTrimNode.SAFE_HEX_DIGITS)) {
return JSStringToNumberNoTrimNode.doHex(arg0Value, (ReadCharUTF16Node.getUncached()));
}
if ((JSStringToNumberNoTrimNode.isOctal(arg0Value, (ReadCharUTF16Node.getUncached()))) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.SAFE_OCTAL_DIGITS)) {
return JSStringToNumberNoTrimNode.doOctalSafe(arg0Value, (ReadCharUTF16Node.getUncached()));
}
if ((JSStringToNumberNoTrimNode.isOctal(arg0Value, (ReadCharUTF16Node.getUncached()))) && (JSGuards.stringLength(arg0Value) > JSStringToNumberNoTrimNode.SAFE_OCTAL_DIGITS)) {
return JSStringToNumberNoTrimNode.doOctal(arg0Value, (ReadCharUTF16Node.getUncached()));
}
if ((JSStringToNumberNoTrimNode.isBinary(arg0Value, (ReadCharUTF16Node.getUncached()))) && (JSGuards.stringLength(arg0Value) <= JSStringToNumberNoTrimNode.SAFE_BINARY_DIGITS)) {
return JSStringToNumberNoTrimNode.doBinarySafe(arg0Value, (ReadCharUTF16Node.getUncached()));
}
if ((JSStringToNumberNoTrimNode.isBinary(arg0Value, (ReadCharUTF16Node.getUncached()))) && (JSGuards.stringLength(arg0Value) > JSStringToNumberNoTrimNode.SAFE_BINARY_DIGITS)) {
return JSStringToNumberNoTrimNode.doBinary(arg0Value, (ReadCharUTF16Node.getUncached()));
}
if ((JSGuards.stringLength(arg0Value) > 0) && (JSStringToNumberNoTrimNode.startsWithValidDouble(arg0Value, (ReadCharUTF16Node.getUncached())))) {
return JSStringToNumberNoTrimNode.doDouble(arg0Value, (ReadCharUTF16Node.getUncached()));
}
throw newUnsupportedSpecializationException1(this, arg0Value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy