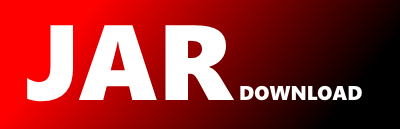
com.oracle.truffle.js.nodes.cast.JSToBigIntNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.cast;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.JSTypesGen;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.SafeInteger;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSToBigIntNode#doBigInt}
* Activation probability: 0.65000
* With/without class size: 11/0 bytes
* Specialization {@link JSToBigIntNode#doOther}
* Activation probability: 0.35000
* With/without class size: 12/5 bytes
*
*/
@GeneratedBy(JSToBigIntNode.class)
@SuppressWarnings("javadoc")
public final class JSToBigIntNodeGen extends JSToBigIntNode {
private static final StateField STATE_0_JSToBigIntNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link JSToBigIntNode#doOther}
* Parameter: {@link JSPrimitiveToBigIntNode} primitiveToBigInt
* Inline method: {@link JSPrimitiveToBigIntNodeGen#inline}
*/
private static final JSPrimitiveToBigIntNode INLINED_OTHER_PRIMITIVE_TO_BIG_INT_ = JSPrimitiveToBigIntNodeGen.inline(InlineTarget.create(JSPrimitiveToBigIntNode.class, STATE_0_JSToBigIntNode_UPDATER.subUpdater(2, 6)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link JSToBigIntNode#doBigInt}
* 1: SpecializationActive {@link JSToBigIntNode#doOther}
* 2-7: InlinedCache
* Specialization: {@link JSToBigIntNode#doOther}
* Parameter: {@link JSPrimitiveToBigIntNode} primitiveToBigInt
* Inline method: {@link JSPrimitiveToBigIntNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link JSToBigIntNode#doOther}
* Parameter: {@link JSToPrimitiveNode} toPrimitiveNode
*/
@Child private JSToPrimitiveNode other_toPrimitiveNode_;
private JSToBigIntNodeGen() {
}
@Override
public BigInt execute(Object arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11) != 0 /* is SpecializationActive[JSToBigIntNode.doBigInt(BigInt)] || SpecializationActive[JSToBigIntNode.doOther(Object, JSToPrimitiveNode, JSPrimitiveToBigIntNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSToBigIntNode.doBigInt(BigInt)] */ && arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
return JSToBigIntNode.doBigInt(arg0Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSToBigIntNode.doOther(Object, JSToPrimitiveNode, JSPrimitiveToBigIntNode)] */) {
{
JSToPrimitiveNode toPrimitiveNode__ = this.other_toPrimitiveNode_;
if (toPrimitiveNode__ != null) {
if ((!(JSGuards.isBigInt(arg0Value)))) {
return doOther(arg0Value, toPrimitiveNode__, INLINED_OTHER_PRIMITIVE_TO_BIG_INT_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private BigInt executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSToBigIntNode.doBigInt(BigInt)] */;
this.state_0_ = state_0;
return JSToBigIntNode.doBigInt(arg0Value_);
}
if ((!(JSGuards.isBigInt(arg0Value)))) {
JSToPrimitiveNode toPrimitiveNode__ = this.insert((JSToPrimitiveNode.createHintNumber()));
Objects.requireNonNull(toPrimitiveNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.other_toPrimitiveNode_ = toPrimitiveNode__;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSToBigIntNode.doOther(Object, JSToPrimitiveNode, JSPrimitiveToBigIntNode)] */;
this.state_0_ = state_0;
return doOther(arg0Value, toPrimitiveNode__, INLINED_OTHER_PRIMITIVE_TO_BIG_INT_);
}
throw new UnsupportedSpecializationException(this, null, arg0Value);
}
@TruffleBoundary
private static UnsupportedSpecializationException newUnsupportedSpecializationException1(Node thisNode_, Object arg0Value) {
return new UnsupportedSpecializationException(thisNode_, null, arg0Value);
}
@NeverDefault
public static JSToBigIntNode create() {
return new JSToBigIntNodeGen();
}
@NeverDefault
public static JSToBigIntNode getUncached() {
return JSToBigIntNodeGen.UNCACHED;
}
@GeneratedBy(JSToBigIntNode.class)
@DenyReplace
private static final class Uncached extends JSToBigIntNode implements UnadoptableNode {
@TruffleBoundary
@Override
public BigInt execute(Object arg0Value) {
if (arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
return JSToBigIntNode.doBigInt(arg0Value_);
}
if ((!(JSGuards.isBigInt(arg0Value)))) {
return doOther(arg0Value, (JSToPrimitiveNode.getUncachedHintNumber()), (JSPrimitiveToBigIntNodeGen.getUncached()));
}
throw newUnsupportedSpecializationException1(this, arg0Value);
}
}
/**
* Debug Info:
* Specialization {@link JSPrimitiveToBigIntNode#doBoolean}
* Activation probability: 0.27381
* With/without class size: 7/0 bytes
* Specialization {@link JSPrimitiveToBigIntNode#doBigInt}
* Activation probability: 0.23095
* With/without class size: 6/0 bytes
* Specialization {@link JSPrimitiveToBigIntNode#doForeignBigInt}
* Activation probability: 0.18810
* With/without class size: 6/0 bytes
* Specialization {@link JSPrimitiveToBigIntNode#doNumber}
* Activation probability: 0.14524
* With/without class size: 5/0 bytes
* Specialization {@link JSPrimitiveToBigIntNode#doSymbolNullOrUndefined}
* Activation probability: 0.10238
* With/without class size: 5/0 bytes
* Specialization {@link JSPrimitiveToBigIntNode#doString}
* Activation probability: 0.05952
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(JSPrimitiveToBigIntNode.class)
@SuppressWarnings("javadoc")
protected static final class JSPrimitiveToBigIntNodeGen {
private static final Uncached UNCACHED = new Uncached();
@TruffleBoundary
private static UnsupportedSpecializationException newUnsupportedSpecializationException2(Node thisNode_, Object arg0Value, Object arg1Value) {
return new UnsupportedSpecializationException(thisNode_, null, arg0Value, arg1Value);
}
@NeverDefault
public static JSPrimitiveToBigIntNode getUncached() {
return JSPrimitiveToBigIntNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static JSPrimitiveToBigIntNode inline(@RequiredField(bits = 6, value = StateField.class) InlineTarget target) {
return new JSPrimitiveToBigIntNodeGen.Inlined(target);
}
@GeneratedBy(JSPrimitiveToBigIntNode.class)
@DenyReplace
private static final class Inlined extends JSPrimitiveToBigIntNode implements UnadoptableNode {
/**
* State Info:
* 0: SpecializationActive {@link JSPrimitiveToBigIntNode#doBoolean}
* 1: SpecializationActive {@link JSPrimitiveToBigIntNode#doBigInt}
* 2: SpecializationActive {@link JSPrimitiveToBigIntNode#doForeignBigInt}
* 3: SpecializationActive {@link JSPrimitiveToBigIntNode#doNumber}
* 4: SpecializationActive {@link JSPrimitiveToBigIntNode#doSymbolNullOrUndefined}
* 5: SpecializationActive {@link JSPrimitiveToBigIntNode#doString}
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(JSPrimitiveToBigIntNode.class);
this.state_0_ = target.getState(0, 6);
}
@Override
public BigInt executeBigInt(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doBoolean(boolean)] || SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doBigInt(BigInt)] || SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doForeignBigInt(Node, BigInt)] || SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doNumber(Node, Object)] || SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doSymbolNullOrUndefined(Node, Object)] || SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doString(Node, TruffleString)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doBoolean(boolean)] */ && arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
return JSPrimitiveToBigIntNode.doBoolean(arg1Value_);
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doBigInt(BigInt)] || SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doForeignBigInt(Node, BigInt)] */ && arg1Value instanceof BigInt) {
BigInt arg1Value_ = (BigInt) arg1Value;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doBigInt(BigInt)] */) {
if ((!(arg1Value_.isForeign()))) {
return JSPrimitiveToBigIntNode.doBigInt(arg1Value_);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doForeignBigInt(Node, BigInt)] */) {
if ((arg1Value_.isForeign())) {
return JSPrimitiveToBigIntNode.doForeignBigInt(arg0Value, arg1Value_);
}
}
}
if ((state_0 & 0b11000) != 0 /* is SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doNumber(Node, Object)] || SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doSymbolNullOrUndefined(Node, Object)] */) {
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doNumber(Node, Object)] */) {
if ((JSGuards.isNumber(arg1Value) || JSGuards.isNumberLong(arg1Value))) {
return JSPrimitiveToBigIntNode.doNumber(arg0Value, arg1Value);
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doSymbolNullOrUndefined(Node, Object)] */) {
if ((JSGuards.isSymbol(arg1Value) || JSGuards.isNullOrUndefined(arg1Value))) {
return JSPrimitiveToBigIntNode.doSymbolNullOrUndefined(arg0Value, arg1Value);
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doString(Node, TruffleString)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return JSPrimitiveToBigIntNode.doString(arg0Value, arg1Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private BigInt executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doBoolean(boolean)] */;
this.state_0_.set(arg0Value, state_0);
return JSPrimitiveToBigIntNode.doBoolean(arg1Value_);
}
if (arg1Value instanceof BigInt) {
BigInt arg1Value_ = (BigInt) arg1Value;
if ((!(arg1Value_.isForeign()))) {
state_0 = state_0 | 0b10 /* add SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doBigInt(BigInt)] */;
this.state_0_.set(arg0Value, state_0);
return JSPrimitiveToBigIntNode.doBigInt(arg1Value_);
}
if ((arg1Value_.isForeign())) {
state_0 = state_0 | 0b100 /* add SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doForeignBigInt(Node, BigInt)] */;
this.state_0_.set(arg0Value, state_0);
return JSPrimitiveToBigIntNode.doForeignBigInt(arg0Value, arg1Value_);
}
}
if ((JSGuards.isNumber(arg1Value) || JSGuards.isNumberLong(arg1Value))) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doNumber(Node, Object)] */;
this.state_0_.set(arg0Value, state_0);
return JSPrimitiveToBigIntNode.doNumber(arg0Value, arg1Value);
}
if ((JSGuards.isSymbol(arg1Value) || JSGuards.isNullOrUndefined(arg1Value))) {
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doSymbolNullOrUndefined(Node, Object)] */;
this.state_0_.set(arg0Value, state_0);
return JSPrimitiveToBigIntNode.doSymbolNullOrUndefined(arg0Value, arg1Value);
}
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSToBigIntNode.JSPrimitiveToBigIntNode.doString(Node, TruffleString)] */;
this.state_0_.set(arg0Value, state_0);
return JSPrimitiveToBigIntNode.doString(arg0Value, arg1Value_);
}
throw newUnsupportedSpecializationException2(this, arg0Value, arg1Value);
}
}
@GeneratedBy(JSPrimitiveToBigIntNode.class)
@DenyReplace
private static final class Uncached extends JSPrimitiveToBigIntNode implements UnadoptableNode {
@TruffleBoundary
@Override
public BigInt executeBigInt(Node arg0Value, Object arg1Value) {
if (arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
return JSPrimitiveToBigIntNode.doBoolean(arg1Value_);
}
if (arg1Value instanceof BigInt) {
BigInt arg1Value_ = (BigInt) arg1Value;
if ((!(arg1Value_.isForeign()))) {
return JSPrimitiveToBigIntNode.doBigInt(arg1Value_);
}
if ((arg1Value_.isForeign())) {
return JSPrimitiveToBigIntNode.doForeignBigInt(arg0Value, arg1Value_);
}
}
if ((JSGuards.isNumber(arg1Value) || JSGuards.isNumberLong(arg1Value))) {
return JSPrimitiveToBigIntNode.doNumber(arg0Value, arg1Value);
}
if ((JSGuards.isSymbol(arg1Value) || JSGuards.isNullOrUndefined(arg1Value))) {
return JSPrimitiveToBigIntNode.doSymbolNullOrUndefined(arg0Value, arg1Value);
}
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return JSPrimitiveToBigIntNode.doString(arg0Value, arg1Value_);
}
throw newUnsupportedSpecializationException2(this, arg0Value, arg1Value);
}
}
}
/**
* Debug Info:
* Specialization {@link CoercePrimitiveToBigIntNode#doBoolean}
* Activation probability: 0.19111
* With/without class size: 6/0 bytes
* Specialization {@link CoercePrimitiveToBigIntNode#doBigInt}
* Activation probability: 0.17111
* With/without class size: 6/0 bytes
* Specialization {@link CoercePrimitiveToBigIntNode#doForeignBigInt}
* Activation probability: 0.15111
* With/without class size: 5/0 bytes
* Specialization {@link CoercePrimitiveToBigIntNode#doInteger}
* Activation probability: 0.13111
* With/without class size: 5/0 bytes
* Specialization {@link CoercePrimitiveToBigIntNode#doSafeInteger}
* Activation probability: 0.11111
* With/without class size: 5/0 bytes
* Specialization {@link CoercePrimitiveToBigIntNode#doLong}
* Activation probability: 0.09111
* With/without class size: 5/0 bytes
* Specialization {@link CoercePrimitiveToBigIntNode#doDouble}
* Activation probability: 0.07111
* With/without class size: 5/4 bytes
* Specialization {@link CoercePrimitiveToBigIntNode#doSymbolNullOrUndefined}
* Activation probability: 0.05111
* With/without class size: 4/0 bytes
* Specialization {@link CoercePrimitiveToBigIntNode#doString}
* Activation probability: 0.03111
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(CoercePrimitiveToBigIntNode.class)
@SuppressWarnings("javadoc")
public static final class CoercePrimitiveToBigIntNodeGen {
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#double_numberToBigInt_}
*
*/
@NeverDefault
public static CoercePrimitiveToBigIntNode inline(@RequiredField(bits = 12, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new CoercePrimitiveToBigIntNodeGen.Inlined(target);
}
@GeneratedBy(CoercePrimitiveToBigIntNode.class)
@DenyReplace
private static final class Inlined extends CoercePrimitiveToBigIntNode implements UnadoptableNode {
/**
* State Info:
* 0: SpecializationActive {@link CoercePrimitiveToBigIntNode#doBoolean}
* 1: SpecializationActive {@link CoercePrimitiveToBigIntNode#doBigInt}
* 2: SpecializationActive {@link CoercePrimitiveToBigIntNode#doForeignBigInt}
* 3: SpecializationActive {@link CoercePrimitiveToBigIntNode#doInteger}
* 4: SpecializationActive {@link CoercePrimitiveToBigIntNode#doSafeInteger}
* 5: SpecializationActive {@link CoercePrimitiveToBigIntNode#doLong}
* 6: SpecializationActive {@link CoercePrimitiveToBigIntNode#doDouble}
* 7: SpecializationActive {@link CoercePrimitiveToBigIntNode#doSymbolNullOrUndefined}
* 8: SpecializationActive {@link CoercePrimitiveToBigIntNode#doString}
* 9-11: ImplicitCast[type=double, index=1]
*
*/
private final StateField state_0_;
private final ReferenceField double_numberToBigInt_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(CoercePrimitiveToBigIntNode.class);
this.state_0_ = target.getState(0, 12);
this.double_numberToBigInt_ = target.getReference(1, JSNumberToBigIntNode.class);
}
@Override
public BigInt executeBigInt(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b111111111) != 0 /* is SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doBoolean(boolean)] || SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doBigInt(BigInt)] || SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doForeignBigInt(BigInt)] || SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doInteger(int)] || SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doSafeInteger(SafeInteger)] || SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doLong(long)] || SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doDouble(double, JSNumberToBigIntNode)] || SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doSymbolNullOrUndefined(Node, Object)] || SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doString(Node, TruffleString)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doBoolean(boolean)] */ && arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
return CoercePrimitiveToBigIntNode.doBoolean(arg1Value_);
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doBigInt(BigInt)] || SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doForeignBigInt(BigInt)] */ && arg1Value instanceof BigInt) {
BigInt arg1Value_ = (BigInt) arg1Value;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doBigInt(BigInt)] */) {
if ((!(arg1Value_.isForeign()))) {
return CoercePrimitiveToBigIntNode.doBigInt(arg1Value_);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doForeignBigInt(BigInt)] */) {
if ((arg1Value_.isForeign())) {
return CoercePrimitiveToBigIntNode.doForeignBigInt(arg1Value_);
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doInteger(int)] */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
return CoercePrimitiveToBigIntNode.doInteger(arg1Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doSafeInteger(SafeInteger)] */ && arg1Value instanceof SafeInteger) {
SafeInteger arg1Value_ = (SafeInteger) arg1Value;
return CoercePrimitiveToBigIntNode.doSafeInteger(arg1Value_);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doLong(long)] */ && arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
return CoercePrimitiveToBigIntNode.doLong(arg1Value_);
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doDouble(double, JSNumberToBigIntNode)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b111000000000) >>> 9 /* get-int ImplicitCast[type=double, index=1] */, arg1Value)) {
double arg1Value_ = JSTypesGen.asImplicitDouble((state_0 & 0b111000000000) >>> 9 /* get-int ImplicitCast[type=double, index=1] */, arg1Value);
{
JSNumberToBigIntNode numberToBigInt__ = this.double_numberToBigInt_.get(arg0Value);
if (numberToBigInt__ != null) {
return CoercePrimitiveToBigIntNode.doDouble(arg1Value_, numberToBigInt__);
}
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doSymbolNullOrUndefined(Node, Object)] */) {
if ((JSGuards.isSymbol(arg1Value) || JSGuards.isNullOrUndefined(arg1Value))) {
return CoercePrimitiveToBigIntNode.doSymbolNullOrUndefined(arg0Value, arg1Value);
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doString(Node, TruffleString)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
return CoercePrimitiveToBigIntNode.doString(arg0Value, arg1Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private BigInt executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof Boolean) {
boolean arg1Value_ = (boolean) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doBoolean(boolean)] */;
this.state_0_.set(arg0Value, state_0);
return CoercePrimitiveToBigIntNode.doBoolean(arg1Value_);
}
if (arg1Value instanceof BigInt) {
BigInt arg1Value_ = (BigInt) arg1Value;
if ((!(arg1Value_.isForeign()))) {
state_0 = state_0 | 0b10 /* add SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doBigInt(BigInt)] */;
this.state_0_.set(arg0Value, state_0);
return CoercePrimitiveToBigIntNode.doBigInt(arg1Value_);
}
if ((arg1Value_.isForeign())) {
state_0 = state_0 | 0b100 /* add SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doForeignBigInt(BigInt)] */;
this.state_0_.set(arg0Value, state_0);
return CoercePrimitiveToBigIntNode.doForeignBigInt(arg1Value_);
}
}
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doInteger(int)] */;
this.state_0_.set(arg0Value, state_0);
return CoercePrimitiveToBigIntNode.doInteger(arg1Value_);
}
if (arg1Value instanceof SafeInteger) {
SafeInteger arg1Value_ = (SafeInteger) arg1Value;
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doSafeInteger(SafeInteger)] */;
this.state_0_.set(arg0Value, state_0);
return CoercePrimitiveToBigIntNode.doSafeInteger(arg1Value_);
}
if (arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doLong(long)] */;
this.state_0_.set(arg0Value, state_0);
return CoercePrimitiveToBigIntNode.doLong(arg1Value_);
}
{
int doubleCast1;
if ((doubleCast1 = JSTypesGen.specializeImplicitDouble(arg1Value)) != 0) {
double arg1Value_ = JSTypesGen.asImplicitDouble(doubleCast1, arg1Value);
JSNumberToBigIntNode numberToBigInt__ = arg0Value.insert((JSNumberToBigIntNodeGen.create()));
Objects.requireNonNull(numberToBigInt__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.double_numberToBigInt_.set(arg0Value, numberToBigInt__);
state_0 = (state_0 | (doubleCast1 << 9) /* set-int ImplicitCast[type=double, index=1] */);
state_0 = state_0 | 0b1000000 /* add SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doDouble(double, JSNumberToBigIntNode)] */;
this.state_0_.set(arg0Value, state_0);
return CoercePrimitiveToBigIntNode.doDouble(arg1Value_, numberToBigInt__);
}
}
if ((JSGuards.isSymbol(arg1Value) || JSGuards.isNullOrUndefined(arg1Value))) {
state_0 = state_0 | 0b10000000 /* add SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doSymbolNullOrUndefined(Node, Object)] */;
this.state_0_.set(arg0Value, state_0);
return CoercePrimitiveToBigIntNode.doSymbolNullOrUndefined(arg0Value, arg1Value);
}
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[JSToBigIntNode.CoercePrimitiveToBigIntNode.doString(Node, TruffleString)] */;
this.state_0_.set(arg0Value, state_0);
return CoercePrimitiveToBigIntNode.doString(arg0Value, arg1Value_);
}
throw new UnsupportedSpecializationException(this, null, arg0Value, arg1Value);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy