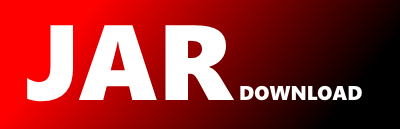
com.oracle.truffle.js.nodes.cast.JSToIntegerAsLongNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.cast;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.JSTypesGen;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.SafeInteger;
import com.oracle.truffle.js.runtime.Symbol;
import com.oracle.truffle.js.runtime.objects.JSObject;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSToIntegerAsLongNode#doInteger}
* Activation probability: 0.13626
* With/without class size: 5/0 bytes
* Specialization {@link JSToIntegerAsLongNode#doBoolean}
* Activation probability: 0.12637
* With/without class size: 5/0 bytes
* Specialization {@link JSToIntegerAsLongNode#doLong}
* Activation probability: 0.11648
* With/without class size: 5/0 bytes
* Specialization {@link JSToIntegerAsLongNode#doSafeInteger}
* Activation probability: 0.10659
* With/without class size: 5/0 bytes
* Specialization {@link JSToIntegerAsLongNode#doDouble}
* Activation probability: 0.09670
* With/without class size: 5/0 bytes
* Specialization {@link JSToIntegerAsLongNode#doDoubleInfinite}
* Activation probability: 0.08681
* With/without class size: 5/0 bytes
* Specialization {@link JSToIntegerAsLongNode#doUndefined}
* Activation probability: 0.07692
* With/without class size: 4/0 bytes
* Specialization {@link JSToIntegerAsLongNode#doNull}
* Activation probability: 0.06703
* With/without class size: 4/0 bytes
* Specialization {@link JSToIntegerAsLongNode#doSymbol}
* Activation probability: 0.05714
* With/without class size: 4/0 bytes
* Specialization {@link JSToIntegerAsLongNode#doBigInt}
* Activation probability: 0.04725
* With/without class size: 4/0 bytes
* Specialization {@link JSToIntegerAsLongNode#doString}
* Activation probability: 0.03736
* With/without class size: 4/8 bytes
* Specialization {@link JSToIntegerAsLongNode#doJSObject}
* Activation probability: 0.02747
* With/without class size: 4/0 bytes
* Specialization {@link JSToIntegerAsLongNode#doJSOrForeignObject}
* Activation probability: 0.01758
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(JSToIntegerAsLongNode.class)
@SuppressWarnings("javadoc")
public final class JSToIntegerAsLongNodeGen extends JSToIntegerAsLongNode {
/**
* State Info:
* 0: SpecializationActive {@link JSToIntegerAsLongNode#doInteger}
* 1: SpecializationActive {@link JSToIntegerAsLongNode#doBoolean}
* 2: SpecializationActive {@link JSToIntegerAsLongNode#doLong}
* 3: SpecializationActive {@link JSToIntegerAsLongNode#doSafeInteger}
* 4: SpecializationActive {@link JSToIntegerAsLongNode#doDouble}
* 5: SpecializationActive {@link JSToIntegerAsLongNode#doDoubleInfinite}
* 6: SpecializationActive {@link JSToIntegerAsLongNode#doUndefined}
* 7: SpecializationActive {@link JSToIntegerAsLongNode#doNull}
* 8: SpecializationActive {@link JSToIntegerAsLongNode#doSymbol}
* 9: SpecializationActive {@link JSToIntegerAsLongNode#doBigInt}
* 10: SpecializationActive {@link JSToIntegerAsLongNode#doString}
* 11: SpecializationActive {@link JSToIntegerAsLongNode#doJSObject}
* 12: SpecializationActive {@link JSToIntegerAsLongNode#doJSOrForeignObject}
* 13-15: ImplicitCast[type=double, index=0]
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSToIntegerAsLongNode#doJSObject}
* Parameter: {@link JSToNumberNode} toNumberNode
*/
@Child private JSToNumberNode toNumberNode;
@Child private StringData string_cache;
private JSToIntegerAsLongNodeGen() {
}
@Override
public long executeLong(Object arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b1111111111111) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doInteger(int)] || SpecializationActive[JSToIntegerAsLongNode.doBoolean(boolean)] || SpecializationActive[JSToIntegerAsLongNode.doLong(long)] || SpecializationActive[JSToIntegerAsLongNode.doSafeInteger(SafeInteger)] || SpecializationActive[JSToIntegerAsLongNode.doDouble(double)] || SpecializationActive[JSToIntegerAsLongNode.doDoubleInfinite(double)] || SpecializationActive[JSToIntegerAsLongNode.doUndefined(Object)] || SpecializationActive[JSToIntegerAsLongNode.doNull(Object)] || SpecializationActive[JSToIntegerAsLongNode.doSymbol(Symbol)] || SpecializationActive[JSToIntegerAsLongNode.doBigInt(BigInt)] || SpecializationActive[JSToIntegerAsLongNode.doString(TruffleString, JSToIntegerAsLongNode, JSStringToNumberNode)] || SpecializationActive[JSToIntegerAsLongNode.doJSObject(JSObject, JSToNumberNode)] || SpecializationActive[JSToIntegerAsLongNode.doJSOrForeignObject(Object, JSToNumberNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doInteger(int)] */ && arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
return JSToIntegerAsLongNode.doInteger(arg0Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doBoolean(boolean)] */ && arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
return JSToIntegerAsLongNode.doBoolean(arg0Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doLong(long)] */ && arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
return JSToIntegerAsLongNode.doLong(arg0Value_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doSafeInteger(SafeInteger)] */ && arg0Value instanceof SafeInteger) {
SafeInteger arg0Value_ = (SafeInteger) arg0Value;
return JSToIntegerAsLongNode.doSafeInteger(arg0Value_);
}
if ((state_0 & 0b110000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doDouble(double)] || SpecializationActive[JSToIntegerAsLongNode.doDoubleInfinite(double)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b1110000000000000) >>> 13 /* get-int ImplicitCast[type=double, index=0] */, arg0Value)) {
double arg0Value_ = JSTypesGen.asImplicitDouble((state_0 & 0b1110000000000000) >>> 13 /* get-int ImplicitCast[type=double, index=0] */, arg0Value);
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doDouble(double)] */) {
if ((!(Double.isInfinite(arg0Value_)))) {
return JSToIntegerAsLongNode.doDouble(arg0Value_);
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doDoubleInfinite(double)] */) {
if ((Double.isInfinite(arg0Value_))) {
return JSToIntegerAsLongNode.doDoubleInfinite(arg0Value_);
}
}
}
if ((state_0 & 0b11000000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doUndefined(Object)] || SpecializationActive[JSToIntegerAsLongNode.doNull(Object)] */) {
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doUndefined(Object)] */) {
if ((JSGuards.isUndefined(arg0Value))) {
return JSToIntegerAsLongNode.doUndefined(arg0Value);
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doNull(Object)] */) {
if ((JSGuards.isJSNull(arg0Value))) {
return JSToIntegerAsLongNode.doNull(arg0Value);
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doSymbol(Symbol)] */ && arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
return doSymbol(arg0Value_);
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doBigInt(BigInt)] */ && arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
return doBigInt(arg0Value_);
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doString(TruffleString, JSToIntegerAsLongNode, JSStringToNumberNode)] */ && arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
StringData s10_ = this.string_cache;
if (s10_ != null) {
return doString(arg0Value_, s10_.nestedToIntegerNode_, s10_.stringToNumberNode_);
}
}
if ((state_0 & 0b100000000000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doJSObject(JSObject, JSToNumberNode)] */ && arg0Value instanceof JSObject) {
JSObject arg0Value_ = (JSObject) arg0Value;
{
JSToNumberNode toNumberNode_ = this.toNumberNode;
if (toNumberNode_ != null) {
return doJSObject(arg0Value_, toNumberNode_);
}
}
}
if ((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[JSToIntegerAsLongNode.doJSOrForeignObject(Object, JSToNumberNode)] */) {
{
JSToNumberNode toNumberNode_1 = this.toNumberNode;
if (toNumberNode_1 != null) {
if ((JSGuards.isJSObject(arg0Value) || JSGuards.isForeignObject(arg0Value))) {
return doJSOrForeignObject(arg0Value, toNumberNode_1);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private long executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSToIntegerAsLongNode.doInteger(int)] */;
this.state_0_ = state_0;
return JSToIntegerAsLongNode.doInteger(arg0Value_);
}
if (arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSToIntegerAsLongNode.doBoolean(boolean)] */;
this.state_0_ = state_0;
return JSToIntegerAsLongNode.doBoolean(arg0Value_);
}
if (arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[JSToIntegerAsLongNode.doLong(long)] */;
this.state_0_ = state_0;
return JSToIntegerAsLongNode.doLong(arg0Value_);
}
if (arg0Value instanceof SafeInteger) {
SafeInteger arg0Value_ = (SafeInteger) arg0Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSToIntegerAsLongNode.doSafeInteger(SafeInteger)] */;
this.state_0_ = state_0;
return JSToIntegerAsLongNode.doSafeInteger(arg0Value_);
}
{
int doubleCast0;
if ((doubleCast0 = JSTypesGen.specializeImplicitDouble(arg0Value)) != 0) {
double arg0Value_ = JSTypesGen.asImplicitDouble(doubleCast0, arg0Value);
if ((!(Double.isInfinite(arg0Value_)))) {
state_0 = (state_0 | (doubleCast0 << 13) /* set-int ImplicitCast[type=double, index=0] */);
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSToIntegerAsLongNode.doDouble(double)] */;
this.state_0_ = state_0;
return JSToIntegerAsLongNode.doDouble(arg0Value_);
}
if ((Double.isInfinite(arg0Value_))) {
state_0 = (state_0 | (doubleCast0 << 13) /* set-int ImplicitCast[type=double, index=0] */);
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSToIntegerAsLongNode.doDoubleInfinite(double)] */;
this.state_0_ = state_0;
return JSToIntegerAsLongNode.doDoubleInfinite(arg0Value_);
}
}
}
if ((JSGuards.isUndefined(arg0Value))) {
state_0 = state_0 | 0b1000000 /* add SpecializationActive[JSToIntegerAsLongNode.doUndefined(Object)] */;
this.state_0_ = state_0;
return JSToIntegerAsLongNode.doUndefined(arg0Value);
}
if ((JSGuards.isJSNull(arg0Value))) {
state_0 = state_0 | 0b10000000 /* add SpecializationActive[JSToIntegerAsLongNode.doNull(Object)] */;
this.state_0_ = state_0;
return JSToIntegerAsLongNode.doNull(arg0Value);
}
if (arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[JSToIntegerAsLongNode.doSymbol(Symbol)] */;
this.state_0_ = state_0;
return doSymbol(arg0Value_);
}
if (arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[JSToIntegerAsLongNode.doBigInt(BigInt)] */;
this.state_0_ = state_0;
return doBigInt(arg0Value_);
}
if (arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
StringData s10_ = this.insert(new StringData());
JSToIntegerAsLongNode nestedToIntegerNode__ = s10_.insert((JSToIntegerAsLongNode.create()));
Objects.requireNonNull(nestedToIntegerNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s10_.nestedToIntegerNode_ = nestedToIntegerNode__;
JSStringToNumberNode stringToNumberNode__ = s10_.insert((JSStringToNumberNode.create()));
Objects.requireNonNull(stringToNumberNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s10_.stringToNumberNode_ = stringToNumberNode__;
VarHandle.storeStoreFence();
this.string_cache = s10_;
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[JSToIntegerAsLongNode.doString(TruffleString, JSToIntegerAsLongNode, JSStringToNumberNode)] */;
this.state_0_ = state_0;
return doString(arg0Value_, nestedToIntegerNode__, stringToNumberNode__);
}
if (((state_0 & 0b1000000000000)) == 0 /* is-not SpecializationActive[JSToIntegerAsLongNode.doJSOrForeignObject(Object, JSToNumberNode)] */ && arg0Value instanceof JSObject) {
JSObject arg0Value_ = (JSObject) arg0Value;
JSToNumberNode toNumberNode_;
JSToNumberNode toNumberNode__shared = this.toNumberNode;
if (toNumberNode__shared != null) {
toNumberNode_ = toNumberNode__shared;
} else {
toNumberNode_ = this.insert((JSToNumberNode.create()));
if (toNumberNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumberNode == null) {
VarHandle.storeStoreFence();
this.toNumberNode = toNumberNode_;
}
state_0 = state_0 | 0b100000000000 /* add SpecializationActive[JSToIntegerAsLongNode.doJSObject(JSObject, JSToNumberNode)] */;
this.state_0_ = state_0;
return doJSObject(arg0Value_, toNumberNode_);
}
if ((JSGuards.isJSObject(arg0Value) || JSGuards.isForeignObject(arg0Value))) {
JSToNumberNode toNumberNode_1;
JSToNumberNode toNumberNode_1_shared = this.toNumberNode;
if (toNumberNode_1_shared != null) {
toNumberNode_1 = toNumberNode_1_shared;
} else {
toNumberNode_1 = this.insert((JSToNumberNode.create()));
if (toNumberNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumberNode == null) {
VarHandle.storeStoreFence();
this.toNumberNode = toNumberNode_1;
}
state_0 = state_0 & 0xfffff7ff /* remove SpecializationActive[JSToIntegerAsLongNode.doJSObject(JSObject, JSToNumberNode)] */;
state_0 = state_0 | 0b1000000000000 /* add SpecializationActive[JSToIntegerAsLongNode.doJSOrForeignObject(Object, JSToNumberNode)] */;
this.state_0_ = state_0;
return doJSOrForeignObject(arg0Value, toNumberNode_1);
}
throw new UnsupportedSpecializationException(this, null, arg0Value);
}
@NeverDefault
public static JSToIntegerAsLongNode create() {
return new JSToIntegerAsLongNodeGen();
}
@GeneratedBy(JSToIntegerAsLongNode.class)
@DenyReplace
private static final class StringData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link JSToIntegerAsLongNode#doString}
* Parameter: {@link JSToIntegerAsLongNode} nestedToIntegerNode
*/
@Child JSToIntegerAsLongNode nestedToIntegerNode_;
/**
* Source Info:
* Specialization: {@link JSToIntegerAsLongNode#doString}
* Parameter: {@link JSStringToNumberNode} stringToNumberNode
*/
@Child JSStringToNumberNode stringToNumberNode_;
StringData() {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy