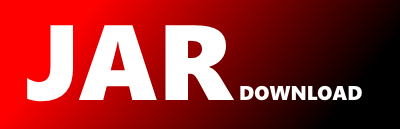
com.oracle.truffle.js.nodes.cast.JSToIntegerWithoutRoundingNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.cast;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.JSTypesGen;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.SafeInteger;
import com.oracle.truffle.js.runtime.Symbol;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSToIntegerWithoutRoundingNode#doInteger}
* Activation probability: 0.15909
* With/without class size: 5/0 bytes
* Specialization {@link JSToIntegerWithoutRoundingNode#doLong}
* Activation probability: 0.14545
* With/without class size: 5/0 bytes
* Specialization {@link JSToIntegerWithoutRoundingNode#doBoolean}
* Activation probability: 0.13182
* With/without class size: 5/0 bytes
* Specialization {@link JSToIntegerWithoutRoundingNode#doSafeInteger}
* Activation probability: 0.11818
* With/without class size: 5/0 bytes
* Specialization {@link JSToIntegerWithoutRoundingNode#doDoubleInfinite}
* Activation probability: 0.10455
* With/without class size: 6/1 bytes
* Specialization {@link JSToIntegerWithoutRoundingNode#doNull}
* Activation probability: 0.09091
* With/without class size: 5/0 bytes
* Specialization {@link JSToIntegerWithoutRoundingNode#doUndefined}
* Activation probability: 0.07727
* With/without class size: 4/0 bytes
* Specialization {@link JSToIntegerWithoutRoundingNode#doSymbol}
* Activation probability: 0.06364
* With/without class size: 4/0 bytes
* Specialization {@link JSToIntegerWithoutRoundingNode#doBigInt}
* Activation probability: 0.05000
* With/without class size: 4/0 bytes
* Specialization {@link JSToIntegerWithoutRoundingNode#doString}
* Activation probability: 0.03636
* With/without class size: 4/4 bytes
* Specialization {@link JSToIntegerWithoutRoundingNode#doJSOrForeignObject}
* Activation probability: 0.02273
* With/without class size: 4/4 bytes
*
*/
@GeneratedBy(JSToIntegerWithoutRoundingNode.class)
@SuppressWarnings("javadoc")
public final class JSToIntegerWithoutRoundingNodeGen extends JSToIntegerWithoutRoundingNode {
private static final StateField STATE_0_JSToIntegerWithoutRoundingNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link JSToIntegerWithoutRoundingNode#doDoubleInfinite}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_DOUBLE_INFINITE_ERROR_BRANCH_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_JSToIntegerWithoutRoundingNode_UPDATER.subUpdater(14, 1)));
/**
* State Info:
* 0: SpecializationActive {@link JSToIntegerWithoutRoundingNode#doInteger}
* 1: SpecializationActive {@link JSToIntegerWithoutRoundingNode#doLong}
* 2: SpecializationActive {@link JSToIntegerWithoutRoundingNode#doBoolean}
* 3: SpecializationActive {@link JSToIntegerWithoutRoundingNode#doSafeInteger}
* 4: SpecializationActive {@link JSToIntegerWithoutRoundingNode#doDoubleInfinite}
* 5: SpecializationActive {@link JSToIntegerWithoutRoundingNode#doNull}
* 6: SpecializationActive {@link JSToIntegerWithoutRoundingNode#doUndefined}
* 7: SpecializationActive {@link JSToIntegerWithoutRoundingNode#doSymbol}
* 8: SpecializationActive {@link JSToIntegerWithoutRoundingNode#doBigInt}
* 9: SpecializationActive {@link JSToIntegerWithoutRoundingNode#doString}
* 10: SpecializationActive {@link JSToIntegerWithoutRoundingNode#doJSOrForeignObject}
* 11-13: ImplicitCast[type=double, index=0]
* 14: InlinedCache
* Specialization: {@link JSToIntegerWithoutRoundingNode#doDoubleInfinite}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link JSToIntegerWithoutRoundingNode#doString}
* Parameter: {@link JSToIntegerWithoutRoundingNode} toIntOrInf
*/
@Child private JSToIntegerWithoutRoundingNode toIntOrInf;
/**
* Source Info:
* Specialization: {@link JSToIntegerWithoutRoundingNode#doString}
* Parameter: {@link JSStringToNumberNode} stringToNumberNode
*/
@Child private JSStringToNumberNode string_stringToNumberNode_;
/**
* Source Info:
* Specialization: {@link JSToIntegerWithoutRoundingNode#doJSOrForeignObject}
* Parameter: {@link JSToNumberNode} toNumberNode
*/
@Child private JSToNumberNode jSOrForeignObject_toNumberNode_;
private JSToIntegerWithoutRoundingNodeGen() {
}
@Override
public double executeDouble(Object arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111111111) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doInteger(int)] || SpecializationActive[JSToIntegerWithoutRoundingNode.doLong(long)] || SpecializationActive[JSToIntegerWithoutRoundingNode.doBoolean(boolean)] || SpecializationActive[JSToIntegerWithoutRoundingNode.doSafeInteger(SafeInteger)] || SpecializationActive[JSToIntegerWithoutRoundingNode.doDoubleInfinite(double, InlinedBranchProfile)] || SpecializationActive[JSToIntegerWithoutRoundingNode.doNull(Object)] || SpecializationActive[JSToIntegerWithoutRoundingNode.doUndefined(Object)] || SpecializationActive[JSToIntegerWithoutRoundingNode.doSymbol(Symbol)] || SpecializationActive[JSToIntegerWithoutRoundingNode.doBigInt(BigInt)] || SpecializationActive[JSToIntegerWithoutRoundingNode.doString(TruffleString, JSToIntegerWithoutRoundingNode, JSStringToNumberNode)] || SpecializationActive[JSToIntegerWithoutRoundingNode.doJSOrForeignObject(Object, JSToIntegerWithoutRoundingNode, JSToNumberNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doInteger(int)] */ && arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
return JSToIntegerWithoutRoundingNode.doInteger(arg0Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doLong(long)] */ && arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
return JSToIntegerWithoutRoundingNode.doLong(arg0Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doBoolean(boolean)] */ && arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
return JSToIntegerWithoutRoundingNode.doBoolean(arg0Value_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doSafeInteger(SafeInteger)] */ && arg0Value instanceof SafeInteger) {
SafeInteger arg0Value_ = (SafeInteger) arg0Value;
return JSToIntegerWithoutRoundingNode.doSafeInteger(arg0Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doDoubleInfinite(double, InlinedBranchProfile)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b11100000000000) >>> 11 /* get-int ImplicitCast[type=double, index=0] */, arg0Value)) {
double arg0Value_ = JSTypesGen.asImplicitDouble((state_0 & 0b11100000000000) >>> 11 /* get-int ImplicitCast[type=double, index=0] */, arg0Value);
return doDoubleInfinite(arg0Value_, INLINED_DOUBLE_INFINITE_ERROR_BRANCH_);
}
if ((state_0 & 0b1100000) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doNull(Object)] || SpecializationActive[JSToIntegerWithoutRoundingNode.doUndefined(Object)] */) {
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doNull(Object)] */) {
if ((JSGuards.isJSNull(arg0Value))) {
return JSToIntegerWithoutRoundingNode.doNull(arg0Value);
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doUndefined(Object)] */) {
if ((JSGuards.isUndefined(arg0Value))) {
return JSToIntegerWithoutRoundingNode.doUndefined(arg0Value);
}
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doSymbol(Symbol)] */ && arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
return doSymbol(arg0Value_);
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doBigInt(BigInt)] */ && arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
return doBigInt(arg0Value_);
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doString(TruffleString, JSToIntegerWithoutRoundingNode, JSStringToNumberNode)] */ && arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
{
JSToIntegerWithoutRoundingNode toIntOrInf_ = this.toIntOrInf;
if (toIntOrInf_ != null) {
JSStringToNumberNode stringToNumberNode__ = this.string_stringToNumberNode_;
if (stringToNumberNode__ != null) {
return doString(arg0Value_, toIntOrInf_, stringToNumberNode__);
}
}
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[JSToIntegerWithoutRoundingNode.doJSOrForeignObject(Object, JSToIntegerWithoutRoundingNode, JSToNumberNode)] */) {
{
JSToIntegerWithoutRoundingNode toIntOrInf_1 = this.toIntOrInf;
if (toIntOrInf_1 != null) {
JSToNumberNode toNumberNode__ = this.jSOrForeignObject_toNumberNode_;
if (toNumberNode__ != null) {
if ((JSGuards.isJSObject(arg0Value) || JSGuards.isForeignObject(arg0Value))) {
return doJSOrForeignObject(arg0Value, toIntOrInf_1, toNumberNode__);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private double executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSToIntegerWithoutRoundingNode.doInteger(int)] */;
this.state_0_ = state_0;
return JSToIntegerWithoutRoundingNode.doInteger(arg0Value_);
}
if (arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSToIntegerWithoutRoundingNode.doLong(long)] */;
this.state_0_ = state_0;
return JSToIntegerWithoutRoundingNode.doLong(arg0Value_);
}
if (arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[JSToIntegerWithoutRoundingNode.doBoolean(boolean)] */;
this.state_0_ = state_0;
return JSToIntegerWithoutRoundingNode.doBoolean(arg0Value_);
}
if (arg0Value instanceof SafeInteger) {
SafeInteger arg0Value_ = (SafeInteger) arg0Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSToIntegerWithoutRoundingNode.doSafeInteger(SafeInteger)] */;
this.state_0_ = state_0;
return JSToIntegerWithoutRoundingNode.doSafeInteger(arg0Value_);
}
{
int doubleCast0;
if ((doubleCast0 = JSTypesGen.specializeImplicitDouble(arg0Value)) != 0) {
double arg0Value_ = JSTypesGen.asImplicitDouble(doubleCast0, arg0Value);
state_0 = (state_0 | (doubleCast0 << 11) /* set-int ImplicitCast[type=double, index=0] */);
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSToIntegerWithoutRoundingNode.doDoubleInfinite(double, InlinedBranchProfile)] */;
this.state_0_ = state_0;
return doDoubleInfinite(arg0Value_, INLINED_DOUBLE_INFINITE_ERROR_BRANCH_);
}
}
if ((JSGuards.isJSNull(arg0Value))) {
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSToIntegerWithoutRoundingNode.doNull(Object)] */;
this.state_0_ = state_0;
return JSToIntegerWithoutRoundingNode.doNull(arg0Value);
}
if ((JSGuards.isUndefined(arg0Value))) {
state_0 = state_0 | 0b1000000 /* add SpecializationActive[JSToIntegerWithoutRoundingNode.doUndefined(Object)] */;
this.state_0_ = state_0;
return JSToIntegerWithoutRoundingNode.doUndefined(arg0Value);
}
if (arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
state_0 = state_0 | 0b10000000 /* add SpecializationActive[JSToIntegerWithoutRoundingNode.doSymbol(Symbol)] */;
this.state_0_ = state_0;
return doSymbol(arg0Value_);
}
if (arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[JSToIntegerWithoutRoundingNode.doBigInt(BigInt)] */;
this.state_0_ = state_0;
return doBigInt(arg0Value_);
}
if (arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
JSToIntegerWithoutRoundingNode toIntOrInf_;
JSToIntegerWithoutRoundingNode toIntOrInf__shared = this.toIntOrInf;
if (toIntOrInf__shared != null) {
toIntOrInf_ = toIntOrInf__shared;
} else {
toIntOrInf_ = this.insert((JSToIntegerWithoutRoundingNodeGen.create()));
if (toIntOrInf_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toIntOrInf == null) {
VarHandle.storeStoreFence();
this.toIntOrInf = toIntOrInf_;
}
JSStringToNumberNode stringToNumberNode__ = this.insert((JSStringToNumberNode.create()));
Objects.requireNonNull(stringToNumberNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.string_stringToNumberNode_ = stringToNumberNode__;
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[JSToIntegerWithoutRoundingNode.doString(TruffleString, JSToIntegerWithoutRoundingNode, JSStringToNumberNode)] */;
this.state_0_ = state_0;
return doString(arg0Value_, toIntOrInf_, stringToNumberNode__);
}
if ((JSGuards.isJSObject(arg0Value) || JSGuards.isForeignObject(arg0Value))) {
JSToIntegerWithoutRoundingNode toIntOrInf_1;
JSToIntegerWithoutRoundingNode toIntOrInf_1_shared = this.toIntOrInf;
if (toIntOrInf_1_shared != null) {
toIntOrInf_1 = toIntOrInf_1_shared;
} else {
toIntOrInf_1 = this.insert((JSToIntegerWithoutRoundingNodeGen.create()));
if (toIntOrInf_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toIntOrInf == null) {
VarHandle.storeStoreFence();
this.toIntOrInf = toIntOrInf_1;
}
JSToNumberNode toNumberNode__ = this.insert((JSToNumberNode.create()));
Objects.requireNonNull(toNumberNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.jSOrForeignObject_toNumberNode_ = toNumberNode__;
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[JSToIntegerWithoutRoundingNode.doJSOrForeignObject(Object, JSToIntegerWithoutRoundingNode, JSToNumberNode)] */;
this.state_0_ = state_0;
return doJSOrForeignObject(arg0Value, toIntOrInf_1, toNumberNode__);
}
throw new UnsupportedSpecializationException(this, null, arg0Value);
}
@NeverDefault
public static JSToIntegerWithoutRoundingNode create() {
return new JSToIntegerWithoutRoundingNodeGen();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy