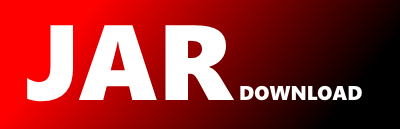
com.oracle.truffle.js.nodes.cast.JSToNumberNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.cast;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.JSTypesGen;
import com.oracle.truffle.js.nodes.JavaScriptNode;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.Symbol;
import com.oracle.truffle.js.runtime.objects.JSObject;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSToNumberNode#doInteger}
* Activation probability: 0.14679
* With/without class size: 5/0 bytes
* Specialization {@link JSToNumberNode#doBoolean}
* Activation probability: 0.13526
* With/without class size: 5/0 bytes
* Specialization {@link JSToNumberNode#doDouble}
* Activation probability: 0.12372
* With/without class size: 5/0 bytes
* Specialization {@link JSToNumberNode#doNull}
* Activation probability: 0.11218
* With/without class size: 5/0 bytes
* Specialization {@link JSToNumberNode#doUndefined}
* Activation probability: 0.10064
* With/without class size: 5/0 bytes
* Specialization {@link JSToNumberNode#doString}
* Activation probability: 0.08910
* With/without class size: 5/4 bytes
* Specialization {@link JSToNumberNode#doJSObject}
* Activation probability: 0.07756
* With/without class size: 4/0 bytes
* Specialization {@link JSToNumberNode#doSymbol}
* Activation probability: 0.06603
* With/without class size: 4/0 bytes
* Specialization {@link JSToNumberNode#doBigInt}
* Activation probability: 0.05449
* With/without class size: 4/0 bytes
* Specialization {@link JSToNumberNode#doForeignBigInt}
* Activation probability: 0.04295
* With/without class size: 4/0 bytes
* Specialization {@link JSToNumberNode#doLong}
* Activation probability: 0.03141
* With/without class size: 4/0 bytes
* Specialization {@link JSToNumberNode#doJSOrForeignObject}
* Activation probability: 0.01987
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(JSToNumberNode.class)
@SuppressWarnings("javadoc")
public final class JSToNumberNodeGen extends JSToNumberNode {
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link JSToNumberNode#doInteger}
* 1: SpecializationActive {@link JSToNumberNode#doBoolean}
* 2: SpecializationActive {@link JSToNumberNode#doDouble}
* 3: SpecializationActive {@link JSToNumberNode#doNull}
* 4: SpecializationActive {@link JSToNumberNode#doUndefined}
* 5: SpecializationActive {@link JSToNumberNode#doString}
* 6: SpecializationActive {@link JSToNumberNode#doJSObject}
* 7: SpecializationActive {@link JSToNumberNode#doJSOrForeignObject}
* 8: SpecializationActive {@link JSToNumberNode#doSymbol}
* 9: SpecializationActive {@link JSToNumberNode#doBigInt}
* 10: SpecializationActive {@link JSToNumberNode#doForeignBigInt}
* 11: SpecializationActive {@link JSToNumberNode#doLong}
* 12-14: ImplicitCast[type=double, index=0]
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSToNumberNode#doJSObject}
* Parameter: {@link JSToPrimitiveNode} toPrimitiveNode
*/
@Child private JSToPrimitiveNode toPrimitiveNode;
/**
* Source Info:
* Specialization: {@link JSToNumberNode#doJSObject}
* Parameter: {@link JSToNumberNode} toNumberNode
*/
@Child private JSToNumberNode toNumberNode;
/**
* Source Info:
* Specialization: {@link JSToNumberNode#doString}
* Parameter: {@link JSStringToNumberNode} stringToNumberNode
*/
@Child private JSStringToNumberNode string_stringToNumberNode_;
private JSToNumberNodeGen() {
}
@Override
public Object execute(Object arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111111111111) != 0 /* is SpecializationActive[JSToNumberNode.doInteger(int)] || SpecializationActive[JSToNumberNode.doBoolean(boolean)] || SpecializationActive[JSToNumberNode.doDouble(double)] || SpecializationActive[JSToNumberNode.doNull(Object)] || SpecializationActive[JSToNumberNode.doUndefined(Object)] || SpecializationActive[JSToNumberNode.doString(TruffleString, JSStringToNumberNode)] || SpecializationActive[JSToNumberNode.doJSObject(JSObject, JSToPrimitiveNode, JSToNumberNode)] || SpecializationActive[JSToNumberNode.doSymbol(Symbol)] || SpecializationActive[JSToNumberNode.doBigInt(BigInt)] || SpecializationActive[JSToNumberNode.doForeignBigInt(BigInt)] || SpecializationActive[JSToNumberNode.doLong(long)] || SpecializationActive[JSToNumberNode.doJSOrForeignObject(Object, JSToPrimitiveNode, JSToNumberNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSToNumberNode.doInteger(int)] */ && arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
return JSToNumberNode.doInteger(arg0Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSToNumberNode.doBoolean(boolean)] */ && arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
return JSToNumberNode.doBoolean(arg0Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSToNumberNode.doDouble(double)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b111000000000000) >>> 12 /* get-int ImplicitCast[type=double, index=0] */, arg0Value)) {
double arg0Value_ = JSTypesGen.asImplicitDouble((state_0 & 0b111000000000000) >>> 12 /* get-int ImplicitCast[type=double, index=0] */, arg0Value);
return JSToNumberNode.doDouble(arg0Value_);
}
if ((state_0 & 0b11000) != 0 /* is SpecializationActive[JSToNumberNode.doNull(Object)] || SpecializationActive[JSToNumberNode.doUndefined(Object)] */) {
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSToNumberNode.doNull(Object)] */) {
if ((JSGuards.isJSNull(arg0Value))) {
return JSToNumberNode.doNull(arg0Value);
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSToNumberNode.doUndefined(Object)] */) {
if ((JSGuards.isUndefined(arg0Value))) {
return JSToNumberNode.doUndefined(arg0Value);
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSToNumberNode.doString(TruffleString, JSStringToNumberNode)] */ && arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
{
JSStringToNumberNode stringToNumberNode__ = this.string_stringToNumberNode_;
if (stringToNumberNode__ != null) {
return doString(arg0Value_, stringToNumberNode__);
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[JSToNumberNode.doJSObject(JSObject, JSToPrimitiveNode, JSToNumberNode)] */ && arg0Value instanceof JSObject) {
JSObject arg0Value_ = (JSObject) arg0Value;
{
JSToPrimitiveNode toPrimitiveNode_ = this.toPrimitiveNode;
if (toPrimitiveNode_ != null) {
JSToNumberNode toNumberNode_ = this.toNumberNode;
if (toNumberNode_ != null) {
return doJSObject(arg0Value_, toPrimitiveNode_, toNumberNode_);
}
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[JSToNumberNode.doSymbol(Symbol)] */ && arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
return doSymbol(arg0Value_);
}
if ((state_0 & 0b11000000000) != 0 /* is SpecializationActive[JSToNumberNode.doBigInt(BigInt)] || SpecializationActive[JSToNumberNode.doForeignBigInt(BigInt)] */ && arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[JSToNumberNode.doBigInt(BigInt)] */) {
if ((!(arg0Value_.isForeign()))) {
return doBigInt(arg0Value_);
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[JSToNumberNode.doForeignBigInt(BigInt)] */) {
if ((arg0Value_.isForeign())) {
return JSToNumberNode.doForeignBigInt(arg0Value_);
}
}
}
if ((state_0 & 0b100000000000) != 0 /* is SpecializationActive[JSToNumberNode.doLong(long)] */ && arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
return JSToNumberNode.doLong(arg0Value_);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[JSToNumberNode.doJSOrForeignObject(Object, JSToPrimitiveNode, JSToNumberNode)] */) {
{
JSToPrimitiveNode toPrimitiveNode_1 = this.toPrimitiveNode;
if (toPrimitiveNode_1 != null) {
JSToNumberNode toNumberNode_1 = this.toNumberNode;
if (toNumberNode_1 != null) {
if ((JSGuards.isJSObject(arg0Value) || JSGuards.isForeignObject(arg0Value))) {
return doJSOrForeignObject(arg0Value, toPrimitiveNode_1, toNumberNode_1);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private Object executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSToNumberNode.doInteger(int)] */;
this.state_0_ = state_0;
return JSToNumberNode.doInteger(arg0Value_);
}
if (arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSToNumberNode.doBoolean(boolean)] */;
this.state_0_ = state_0;
return JSToNumberNode.doBoolean(arg0Value_);
}
{
int doubleCast0;
if ((doubleCast0 = JSTypesGen.specializeImplicitDouble(arg0Value)) != 0) {
double arg0Value_ = JSTypesGen.asImplicitDouble(doubleCast0, arg0Value);
state_0 = (state_0 | (doubleCast0 << 12) /* set-int ImplicitCast[type=double, index=0] */);
state_0 = state_0 | 0b100 /* add SpecializationActive[JSToNumberNode.doDouble(double)] */;
this.state_0_ = state_0;
return JSToNumberNode.doDouble(arg0Value_);
}
}
if ((JSGuards.isJSNull(arg0Value))) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSToNumberNode.doNull(Object)] */;
this.state_0_ = state_0;
return JSToNumberNode.doNull(arg0Value);
}
if ((JSGuards.isUndefined(arg0Value))) {
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSToNumberNode.doUndefined(Object)] */;
this.state_0_ = state_0;
return JSToNumberNode.doUndefined(arg0Value);
}
if (arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
JSStringToNumberNode stringToNumberNode__ = this.insert((JSStringToNumberNode.create()));
Objects.requireNonNull(stringToNumberNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.string_stringToNumberNode_ = stringToNumberNode__;
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSToNumberNode.doString(TruffleString, JSStringToNumberNode)] */;
this.state_0_ = state_0;
return doString(arg0Value_, stringToNumberNode__);
}
if (((state_0 & 0b10000000)) == 0 /* is-not SpecializationActive[JSToNumberNode.doJSOrForeignObject(Object, JSToPrimitiveNode, JSToNumberNode)] */ && arg0Value instanceof JSObject) {
JSObject arg0Value_ = (JSObject) arg0Value;
JSToPrimitiveNode toPrimitiveNode_;
JSToPrimitiveNode toPrimitiveNode__shared = this.toPrimitiveNode;
if (toPrimitiveNode__shared != null) {
toPrimitiveNode_ = toPrimitiveNode__shared;
} else {
toPrimitiveNode_ = this.insert((JSToPrimitiveNode.createHintNumber()));
if (toPrimitiveNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toPrimitiveNode == null) {
VarHandle.storeStoreFence();
this.toPrimitiveNode = toPrimitiveNode_;
}
JSToNumberNode toNumberNode_;
JSToNumberNode toNumberNode__shared = this.toNumberNode;
if (toNumberNode__shared != null) {
toNumberNode_ = toNumberNode__shared;
} else {
toNumberNode_ = this.insert((JSToNumberNode.create()));
if (toNumberNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumberNode == null) {
VarHandle.storeStoreFence();
this.toNumberNode = toNumberNode_;
}
state_0 = state_0 | 0b1000000 /* add SpecializationActive[JSToNumberNode.doJSObject(JSObject, JSToPrimitiveNode, JSToNumberNode)] */;
this.state_0_ = state_0;
return doJSObject(arg0Value_, toPrimitiveNode_, toNumberNode_);
}
if (arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[JSToNumberNode.doSymbol(Symbol)] */;
this.state_0_ = state_0;
return doSymbol(arg0Value_);
}
if (arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
if ((!(arg0Value_.isForeign()))) {
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[JSToNumberNode.doBigInt(BigInt)] */;
this.state_0_ = state_0;
return doBigInt(arg0Value_);
}
if ((arg0Value_.isForeign())) {
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[JSToNumberNode.doForeignBigInt(BigInt)] */;
this.state_0_ = state_0;
return JSToNumberNode.doForeignBigInt(arg0Value_);
}
}
if (arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
state_0 = state_0 | 0b100000000000 /* add SpecializationActive[JSToNumberNode.doLong(long)] */;
this.state_0_ = state_0;
return JSToNumberNode.doLong(arg0Value_);
}
if ((JSGuards.isJSObject(arg0Value) || JSGuards.isForeignObject(arg0Value))) {
JSToPrimitiveNode toPrimitiveNode_1;
JSToPrimitiveNode toPrimitiveNode_1_shared = this.toPrimitiveNode;
if (toPrimitiveNode_1_shared != null) {
toPrimitiveNode_1 = toPrimitiveNode_1_shared;
} else {
toPrimitiveNode_1 = this.insert((JSToPrimitiveNode.createHintNumber()));
if (toPrimitiveNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toPrimitiveNode == null) {
VarHandle.storeStoreFence();
this.toPrimitiveNode = toPrimitiveNode_1;
}
JSToNumberNode toNumberNode_1;
JSToNumberNode toNumberNode_1_shared = this.toNumberNode;
if (toNumberNode_1_shared != null) {
toNumberNode_1 = toNumberNode_1_shared;
} else {
toNumberNode_1 = this.insert((JSToNumberNode.create()));
if (toNumberNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toNumberNode == null) {
VarHandle.storeStoreFence();
this.toNumberNode = toNumberNode_1;
}
state_0 = state_0 & 0xffffffbf /* remove SpecializationActive[JSToNumberNode.doJSObject(JSObject, JSToPrimitiveNode, JSToNumberNode)] */;
state_0 = state_0 | 0b10000000 /* add SpecializationActive[JSToNumberNode.doJSOrForeignObject(Object, JSToPrimitiveNode, JSToNumberNode)] */;
this.state_0_ = state_0;
return doJSOrForeignObject(arg0Value, toPrimitiveNode_1, toNumberNode_1);
}
throw new UnsupportedSpecializationException(this, null, arg0Value);
}
@TruffleBoundary
private static UnsupportedSpecializationException newUnsupportedSpecializationException1(Node thisNode_, Object arg0Value) {
return new UnsupportedSpecializationException(thisNode_, null, arg0Value);
}
@NeverDefault
public static JSToNumberNode create() {
return new JSToNumberNodeGen();
}
@NeverDefault
public static JSToNumberNode getUncached() {
return JSToNumberNodeGen.UNCACHED;
}
@GeneratedBy(JSToNumberNode.class)
@DenyReplace
private static final class Uncached extends JSToNumberNode implements UnadoptableNode {
@TruffleBoundary
@Override
public Object execute(Object arg0Value) {
if (arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
return JSToNumberNode.doInteger(arg0Value_);
}
if (arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
return JSToNumberNode.doBoolean(arg0Value_);
}
if (JSTypesGen.isImplicitDouble(arg0Value)) {
double arg0Value_ = JSTypesGen.asImplicitDouble(arg0Value);
return JSToNumberNode.doDouble(arg0Value_);
}
if ((JSGuards.isJSNull(arg0Value))) {
return JSToNumberNode.doNull(arg0Value);
}
if ((JSGuards.isUndefined(arg0Value))) {
return JSToNumberNode.doUndefined(arg0Value);
}
if (arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
return doString(arg0Value_, (JSStringToNumberNodeGen.getUncached()));
}
if (arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
return doSymbol(arg0Value_);
}
if (arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
if ((!(arg0Value_.isForeign()))) {
return doBigInt(arg0Value_);
}
if ((arg0Value_.isForeign())) {
return JSToNumberNode.doForeignBigInt(arg0Value_);
}
}
if (arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
return JSToNumberNode.doLong(arg0Value_);
}
if ((JSGuards.isJSObject(arg0Value) || JSGuards.isForeignObject(arg0Value))) {
return doJSOrForeignObject(arg0Value, (JSToPrimitiveNode.getUncachedHintNumber()), (JSToNumberNodeGen.getUncached()));
}
throw newUnsupportedSpecializationException1(this, arg0Value);
}
}
/**
* Debug Info:
* Specialization {@link JSToNumberUnaryNode#doDefault}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(JSToNumberUnaryNode.class)
@SuppressWarnings("javadoc")
public static final class JSToNumberUnaryNodeGen extends JSToNumberUnaryNode {
/**
* State Info:
* 0: SpecializationActive {@link JSToNumberUnaryNode#doDefault}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSToNumberUnaryNode#doDefault}
* Parameter: {@link JSToNumberNode} toNumberNode
*/
@Child private JSToNumberNode toNumberNode_;
private JSToNumberUnaryNodeGen(JavaScriptNode operand) {
super(operand);
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object operandNodeValue_ = super.operandNode.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[JSToNumberNode.JSToNumberUnaryNode.doDefault(Object, JSToNumberNode)] */) {
{
JSToNumberNode toNumberNode__ = this.toNumberNode_;
if (toNumberNode__ != null) {
return JSToNumberUnaryNode.doDefault(operandNodeValue_, toNumberNode__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(operandNodeValue_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private Object executeAndSpecialize(Object operandNodeValue) {
int state_0 = this.state_0_;
JSToNumberNode toNumberNode__ = this.insert((JSToNumberNode.create()));
Objects.requireNonNull(toNumberNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toNumberNode_ = toNumberNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSToNumberNode.JSToNumberUnaryNode.doDefault(Object, JSToNumberNode)] */;
this.state_0_ = state_0;
return JSToNumberUnaryNode.doDefault(operandNodeValue, toNumberNode__);
}
@NeverDefault
public static JSToNumberUnaryNode create(JavaScriptNode operand) {
return new JSToNumberUnaryNodeGen(operand);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy