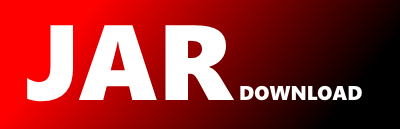
com.oracle.truffle.js.nodes.cast.JSToPropertyKeyNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.cast;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.JavaScriptNode;
import com.oracle.truffle.js.runtime.Symbol;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSToPropertyKeyNode#doTString}
* Activation probability: 0.48333
* With/without class size: 9/0 bytes
* Specialization {@link JSToPropertyKeyNode#doSymbol}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
* Specialization {@link JSToPropertyKeyNode#doOther}
* Activation probability: 0.18333
* With/without class size: 9/9 bytes
*
*/
@GeneratedBy(JSToPropertyKeyNode.class)
@SuppressWarnings("javadoc")
public final class JSToPropertyKeyNodeGen extends JSToPropertyKeyNode {
private static final StateField STATE_0_JSToPropertyKeyNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link JSToPropertyKeyNode#doOther}
* Parameter: {@link InlinedConditionProfile} isSymbol
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_OTHER_IS_SYMBOL_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_JSToPropertyKeyNode_UPDATER.subUpdater(3, 2)));
/**
* State Info:
* 0: SpecializationActive {@link JSToPropertyKeyNode#doTString}
* 1: SpecializationActive {@link JSToPropertyKeyNode#doSymbol}
* 2: SpecializationActive {@link JSToPropertyKeyNode#doOther}
* 3-4: InlinedCache
* Specialization: {@link JSToPropertyKeyNode#doOther}
* Parameter: {@link InlinedConditionProfile} isSymbol
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link JSToPropertyKeyNode#doOther}
* Parameter: {@link JSToPrimitiveNode} toPrimitiveNode
*/
@Child private JSToPrimitiveNode other_toPrimitiveNode_;
/**
* Source Info:
* Specialization: {@link JSToPropertyKeyNode#doOther}
* Parameter: {@link JSToStringNode} toStringNode
*/
@Child private JSToStringNode other_toStringNode_;
private JSToPropertyKeyNodeGen() {
}
@Override
public Object execute(Object arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111) != 0 /* is SpecializationActive[JSToPropertyKeyNode.doTString(TruffleString)] || SpecializationActive[JSToPropertyKeyNode.doSymbol(Symbol)] || SpecializationActive[JSToPropertyKeyNode.doOther(Object, JSToPrimitiveNode, JSToStringNode, InlinedConditionProfile)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSToPropertyKeyNode.doTString(TruffleString)] */ && arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
return doTString(arg0Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSToPropertyKeyNode.doSymbol(Symbol)] */ && arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
return doSymbol(arg0Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSToPropertyKeyNode.doOther(Object, JSToPrimitiveNode, JSToStringNode, InlinedConditionProfile)] */) {
{
JSToPrimitiveNode toPrimitiveNode__ = this.other_toPrimitiveNode_;
if (toPrimitiveNode__ != null) {
JSToStringNode toStringNode__ = this.other_toStringNode_;
if (toStringNode__ != null) {
if ((!(JSGuards.isSymbol(arg0Value)))) {
return doOther(arg0Value, toPrimitiveNode__, toStringNode__, INLINED_OTHER_IS_SYMBOL_);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private Object executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSToPropertyKeyNode.doTString(TruffleString)] */;
this.state_0_ = state_0;
return doTString(arg0Value_);
}
if (arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSToPropertyKeyNode.doSymbol(Symbol)] */;
this.state_0_ = state_0;
return doSymbol(arg0Value_);
}
if ((!(JSGuards.isSymbol(arg0Value)))) {
JSToPrimitiveNode toPrimitiveNode__ = this.insert((JSToPrimitiveNode.createHintString()));
Objects.requireNonNull(toPrimitiveNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.other_toPrimitiveNode_ = toPrimitiveNode__;
JSToStringNode toStringNode__ = this.insert((JSToStringNode.create()));
Objects.requireNonNull(toStringNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.other_toStringNode_ = toStringNode__;
state_0 = state_0 | 0b100 /* add SpecializationActive[JSToPropertyKeyNode.doOther(Object, JSToPrimitiveNode, JSToStringNode, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return doOther(arg0Value, toPrimitiveNode__, toStringNode__, INLINED_OTHER_IS_SYMBOL_);
}
throw new UnsupportedSpecializationException(this, null, arg0Value);
}
@NeverDefault
public static JSToPropertyKeyNode create() {
return new JSToPropertyKeyNodeGen();
}
/**
* Debug Info:
* Specialization {@link JSToPropertyKeyWrapperNode#doDefault}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(JSToPropertyKeyWrapperNode.class)
@SuppressWarnings("javadoc")
public static final class JSToPropertyKeyWrapperNodeGen extends JSToPropertyKeyWrapperNode {
/**
* State Info:
* 0: SpecializationActive {@link JSToPropertyKeyWrapperNode#doDefault}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSToPropertyKeyWrapperNode#doDefault}
* Parameter: {@link JSToPropertyKeyNode} toPropertyKeyNode
*/
@Child private JSToPropertyKeyNode toPropertyKeyNode_;
private JSToPropertyKeyWrapperNodeGen(JavaScriptNode operand) {
super(operand);
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object operandNodeValue_ = super.operandNode.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[JSToPropertyKeyNode.JSToPropertyKeyWrapperNode.doDefault(Object, JSToPropertyKeyNode)] */) {
{
JSToPropertyKeyNode toPropertyKeyNode__ = this.toPropertyKeyNode_;
if (toPropertyKeyNode__ != null) {
return JSToPropertyKeyWrapperNode.doDefault(operandNodeValue_, toPropertyKeyNode__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(operandNodeValue_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private Object executeAndSpecialize(Object operandNodeValue) {
int state_0 = this.state_0_;
JSToPropertyKeyNode toPropertyKeyNode__ = this.insert((JSToPropertyKeyNode.create()));
Objects.requireNonNull(toPropertyKeyNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toPropertyKeyNode_ = toPropertyKeyNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSToPropertyKeyNode.JSToPropertyKeyWrapperNode.doDefault(Object, JSToPropertyKeyNode)] */;
this.state_0_ = state_0;
return JSToPropertyKeyWrapperNode.doDefault(operandNodeValue, toPropertyKeyNode__);
}
@NeverDefault
public static JSToPropertyKeyWrapperNode create(JavaScriptNode operand) {
return new JSToPropertyKeyWrapperNodeGen(operand);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy