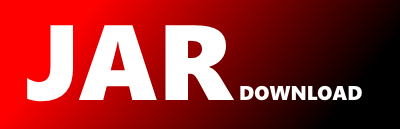
com.oracle.truffle.js.nodes.cast.JSToUInt32NodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.cast;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.JSTypesGen;
import com.oracle.truffle.js.nodes.JavaScriptNode;
import com.oracle.truffle.js.nodes.binary.JSOverloadedBinaryNode;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.JSRuntime;
import com.oracle.truffle.js.runtime.SafeInteger;
import com.oracle.truffle.js.runtime.Symbol;
import com.oracle.truffle.js.runtime.builtins.JSOverloadedOperatorsObject;
import com.oracle.truffle.js.runtime.objects.JSObject;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSToUInt32Node#doInteger}
* Activation probability: 0.10588
* With/without class size: 5/0 bytes
* Specialization {@link JSToUInt32Node#doIntegerNegative}
* Activation probability: 0.10000
* With/without class size: 5/0 bytes
* Specialization {@link JSToUInt32Node#doSafeInteger}
* Activation probability: 0.09412
* With/without class size: 5/0 bytes
* Specialization {@link JSToUInt32Node#doBoolean}
* Activation probability: 0.08824
* With/without class size: 5/0 bytes
* Specialization {@link JSToUInt32Node#doLong}
* Activation probability: 0.08235
* With/without class size: 4/0 bytes
* Specialization {@link JSToUInt32Node#doLongNotSafeInteger}
* Activation probability: 0.07647
* With/without class size: 4/0 bytes
* Specialization {@link JSToUInt32Node#doDoubleFitsInt32Negative}
* Activation probability: 0.07059
* With/without class size: 4/0 bytes
* Specialization {@link JSToUInt32Node#doDoubleRepresentableAsLong}
* Activation probability: 0.06471
* With/without class size: 4/0 bytes
* Specialization {@link JSToUInt32Node#doDouble}
* Activation probability: 0.05882
* With/without class size: 4/0 bytes
* Specialization {@link JSToUInt32Node#doNull}
* Activation probability: 0.05294
* With/without class size: 4/0 bytes
* Specialization {@link JSToUInt32Node#doUndefined}
* Activation probability: 0.04706
* With/without class size: 4/0 bytes
* Specialization {@link JSToUInt32Node#doString}
* Activation probability: 0.04118
* With/without class size: 4/4 bytes
* Specialization {@link JSToUInt32Node#doSymbol}
* Activation probability: 0.03529
* With/without class size: 4/0 bytes
* Specialization {@link JSToUInt32Node#doBigInt}
* Activation probability: 0.02941
* With/without class size: 4/0 bytes
* Specialization {@link JSToUInt32Node#doOverloadedOperator}
* Activation probability: 0.02353
* With/without class size: 4/4 bytes
* Specialization {@link JSToUInt32Node#doJSObject}
* Activation probability: 0.01765
* With/without class size: 4/4 bytes
* Specialization {@link JSToUInt32Node#doForeignObject}
* Activation probability: 0.01176
* With/without class size: 4/8 bytes
*
*/
@GeneratedBy(JSToUInt32Node.class)
@SuppressWarnings("javadoc")
public final class JSToUInt32NodeGen extends JSToUInt32Node {
/**
* State Info:
* 0: SpecializationActive {@link JSToUInt32Node#doInteger}
* 1: SpecializationActive {@link JSToUInt32Node#doIntegerNegative}
* 2: SpecializationActive {@link JSToUInt32Node#doSafeInteger}
* 3: SpecializationActive {@link JSToUInt32Node#doBoolean}
* 4: SpecializationActive {@link JSToUInt32Node#doLong}
* 5: SpecializationActive {@link JSToUInt32Node#doLongNotSafeInteger}
* 6: SpecializationActive {@link JSToUInt32Node#doDoubleFitsInt32Negative}
* 7: SpecializationActive {@link JSToUInt32Node#doDoubleRepresentableAsLong}
* 8: SpecializationActive {@link JSToUInt32Node#doDouble}
* 9: SpecializationActive {@link JSToUInt32Node#doNull}
* 10: SpecializationActive {@link JSToUInt32Node#doUndefined}
* 11: SpecializationActive {@link JSToUInt32Node#doString}
* 12: SpecializationActive {@link JSToUInt32Node#doSymbol}
* 13: SpecializationActive {@link JSToUInt32Node#doBigInt}
* 14: SpecializationActive {@link JSToUInt32Node#doOverloadedOperator}
* 15: SpecializationActive {@link JSToUInt32Node#doJSObject}
* 16: SpecializationActive {@link JSToUInt32Node#doForeignObject}
* 17-19: ImplicitCast[type=double, index=0]
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSToUInt32Node#doString}
* Parameter: {@link JSStringToNumberNode} stringToNumberNode
*/
@Child private JSStringToNumberNode string_stringToNumberNode_;
/**
* Source Info:
* Specialization: {@link JSToUInt32Node#doOverloadedOperator}
* Parameter: {@link JSOverloadedBinaryNode} overloadedOperatorNode
*/
@Child private JSOverloadedBinaryNode overloadedOperator_overloadedOperatorNode_;
/**
* Source Info:
* Specialization: {@link JSToUInt32Node#doJSObject}
* Parameter: {@link JSToNumberNode} toNumberNode
*/
@Child private JSToNumberNode jSObject_toNumberNode_;
@Child private ForeignObjectData foreignObject_cache;
private JSToUInt32NodeGen(boolean unsignedRightShift, int shiftValue) {
super(unsignedRightShift, shiftValue);
}
@Override
public Object execute(Object arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0x1ffff) != 0 /* is SpecializationActive[JSToUInt32Node.doInteger(int)] || SpecializationActive[JSToUInt32Node.doIntegerNegative(int)] || SpecializationActive[JSToUInt32Node.doSafeInteger(SafeInteger)] || SpecializationActive[JSToUInt32Node.doBoolean(boolean)] || SpecializationActive[JSToUInt32Node.doLong(long)] || SpecializationActive[JSToUInt32Node.doLongNotSafeInteger(long)] || SpecializationActive[JSToUInt32Node.doDoubleFitsInt32Negative(double)] || SpecializationActive[JSToUInt32Node.doDoubleRepresentableAsLong(double)] || SpecializationActive[JSToUInt32Node.doDouble(double)] || SpecializationActive[JSToUInt32Node.doNull(Object)] || SpecializationActive[JSToUInt32Node.doUndefined(Object)] || SpecializationActive[JSToUInt32Node.doString(TruffleString, JSStringToNumberNode)] || SpecializationActive[JSToUInt32Node.doSymbol(Symbol)] || SpecializationActive[JSToUInt32Node.doBigInt(BigInt)] || SpecializationActive[JSToUInt32Node.doOverloadedOperator(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] || SpecializationActive[JSToUInt32Node.doJSObject(JSObject, JSToNumberNode)] || SpecializationActive[JSToUInt32Node.doForeignObject(Object, JSToPrimitiveNode, JSToUInt32Node)] */) {
if ((state_0 & 0b11) != 0 /* is SpecializationActive[JSToUInt32Node.doInteger(int)] || SpecializationActive[JSToUInt32Node.doIntegerNegative(int)] */ && arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSToUInt32Node.doInteger(int)] */) {
if ((arg0Value_ >= 0)) {
return doInteger(arg0Value_);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSToUInt32Node.doIntegerNegative(int)] */) {
if ((arg0Value_ < 0)) {
return doIntegerNegative(arg0Value_);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSToUInt32Node.doSafeInteger(SafeInteger)] */ && arg0Value instanceof SafeInteger) {
SafeInteger arg0Value_ = (SafeInteger) arg0Value;
return doSafeInteger(arg0Value_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSToUInt32Node.doBoolean(boolean)] */ && arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
return doBoolean(arg0Value_);
}
if ((state_0 & 0b110000) != 0 /* is SpecializationActive[JSToUInt32Node.doLong(long)] || SpecializationActive[JSToUInt32Node.doLongNotSafeInteger(long)] */ && arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSToUInt32Node.doLong(long)] */) {
if ((JSRuntime.isSafeInteger(arg0Value_))) {
return JSToUInt32Node.doLong(arg0Value_);
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSToUInt32Node.doLongNotSafeInteger(long)] */) {
if ((!(JSRuntime.isSafeInteger(arg0Value_)))) {
return JSToUInt32Node.doLongNotSafeInteger(arg0Value_);
}
}
}
if ((state_0 & 0b111000000) != 0 /* is SpecializationActive[JSToUInt32Node.doDoubleFitsInt32Negative(double)] || SpecializationActive[JSToUInt32Node.doDoubleRepresentableAsLong(double)] || SpecializationActive[JSToUInt32Node.doDouble(double)] */ && JSTypesGen.isImplicitDouble((state_0 & 0xe0000) >>> 17 /* get-int ImplicitCast[type=double, index=0] */, arg0Value)) {
double arg0Value_ = JSTypesGen.asImplicitDouble((state_0 & 0xe0000) >>> 17 /* get-int ImplicitCast[type=double, index=0] */, arg0Value);
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[JSToUInt32Node.doDoubleFitsInt32Negative(double)] */) {
if ((!(JSGuards.isDoubleLargerThan2e32(arg0Value_)))) {
return doDoubleFitsInt32Negative(arg0Value_);
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[JSToUInt32Node.doDoubleRepresentableAsLong(double)] */) {
if ((JSGuards.isDoubleLargerThan2e32(arg0Value_)) && (JSGuards.isDoubleRepresentableAsLong(arg0Value_))) {
return doDoubleRepresentableAsLong(arg0Value_);
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[JSToUInt32Node.doDouble(double)] */) {
if ((JSGuards.isDoubleLargerThan2e32(arg0Value_)) && (!(JSGuards.isDoubleRepresentableAsLong(arg0Value_)))) {
return doDouble(arg0Value_);
}
}
}
if ((state_0 & 0b11000000000) != 0 /* is SpecializationActive[JSToUInt32Node.doNull(Object)] || SpecializationActive[JSToUInt32Node.doUndefined(Object)] */) {
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[JSToUInt32Node.doNull(Object)] */) {
if ((JSGuards.isJSNull(arg0Value))) {
return doNull(arg0Value);
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[JSToUInt32Node.doUndefined(Object)] */) {
if ((JSGuards.isUndefined(arg0Value))) {
return doUndefined(arg0Value);
}
}
}
if ((state_0 & 0b100000000000) != 0 /* is SpecializationActive[JSToUInt32Node.doString(TruffleString, JSStringToNumberNode)] */ && arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
{
JSStringToNumberNode stringToNumberNode__ = this.string_stringToNumberNode_;
if (stringToNumberNode__ != null) {
return doString(arg0Value_, stringToNumberNode__);
}
}
}
if ((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[JSToUInt32Node.doSymbol(Symbol)] */ && arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
return doSymbol(arg0Value_);
}
if ((state_0 & 0b10000000000000) != 0 /* is SpecializationActive[JSToUInt32Node.doBigInt(BigInt)] */ && arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
return doBigInt(arg0Value_);
}
if ((state_0 & 0b100000000000000) != 0 /* is SpecializationActive[JSToUInt32Node.doOverloadedOperator(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] */ && arg0Value instanceof JSOverloadedOperatorsObject) {
JSOverloadedOperatorsObject arg0Value_ = (JSOverloadedOperatorsObject) arg0Value;
{
JSOverloadedBinaryNode overloadedOperatorNode__ = this.overloadedOperator_overloadedOperatorNode_;
if (overloadedOperatorNode__ != null) {
assert DSLSupport.assertIdempotence((isUnsignedRightShift()));
return doOverloadedOperator(arg0Value_, overloadedOperatorNode__);
}
}
}
if ((state_0 & 0b1000000000000000) != 0 /* is SpecializationActive[JSToUInt32Node.doJSObject(JSObject, JSToNumberNode)] */ && arg0Value instanceof JSObject) {
JSObject arg0Value_ = (JSObject) arg0Value;
{
JSToNumberNode toNumberNode__ = this.jSObject_toNumberNode_;
if (toNumberNode__ != null) {
if ((!(isUnsignedRightShift()) || !(hasOverloadedOperators(arg0Value_)))) {
return doJSObject(arg0Value_, toNumberNode__);
}
}
}
}
if ((state_0 & 0x10000) != 0 /* is SpecializationActive[JSToUInt32Node.doForeignObject(Object, JSToPrimitiveNode, JSToUInt32Node)] */) {
ForeignObjectData s16_ = this.foreignObject_cache;
if (s16_ != null) {
if ((JSRuntime.isForeignObject(arg0Value))) {
return JSToUInt32Node.doForeignObject(arg0Value, s16_.toPrimitiveNode_, s16_.toUInt32Node_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private Object executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof Integer) {
int arg0Value_ = (int) arg0Value;
if ((arg0Value_ >= 0)) {
state_0 = state_0 | 0b1 /* add SpecializationActive[JSToUInt32Node.doInteger(int)] */;
this.state_0_ = state_0;
return doInteger(arg0Value_);
}
if ((arg0Value_ < 0)) {
state_0 = state_0 | 0b10 /* add SpecializationActive[JSToUInt32Node.doIntegerNegative(int)] */;
this.state_0_ = state_0;
return doIntegerNegative(arg0Value_);
}
}
if (arg0Value instanceof SafeInteger) {
SafeInteger arg0Value_ = (SafeInteger) arg0Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[JSToUInt32Node.doSafeInteger(SafeInteger)] */;
this.state_0_ = state_0;
return doSafeInteger(arg0Value_);
}
if (arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSToUInt32Node.doBoolean(boolean)] */;
this.state_0_ = state_0;
return doBoolean(arg0Value_);
}
if (arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
if ((JSRuntime.isSafeInteger(arg0Value_))) {
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSToUInt32Node.doLong(long)] */;
this.state_0_ = state_0;
return JSToUInt32Node.doLong(arg0Value_);
}
if ((!(JSRuntime.isSafeInteger(arg0Value_)))) {
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSToUInt32Node.doLongNotSafeInteger(long)] */;
this.state_0_ = state_0;
return JSToUInt32Node.doLongNotSafeInteger(arg0Value_);
}
}
{
int doubleCast0;
if ((doubleCast0 = JSTypesGen.specializeImplicitDouble(arg0Value)) != 0) {
double arg0Value_ = JSTypesGen.asImplicitDouble(doubleCast0, arg0Value);
if ((!(JSGuards.isDoubleLargerThan2e32(arg0Value_)))) {
state_0 = (state_0 | (doubleCast0 << 17) /* set-int ImplicitCast[type=double, index=0] */);
state_0 = state_0 | 0b1000000 /* add SpecializationActive[JSToUInt32Node.doDoubleFitsInt32Negative(double)] */;
this.state_0_ = state_0;
return doDoubleFitsInt32Negative(arg0Value_);
}
if ((JSGuards.isDoubleLargerThan2e32(arg0Value_)) && (JSGuards.isDoubleRepresentableAsLong(arg0Value_))) {
state_0 = (state_0 | (doubleCast0 << 17) /* set-int ImplicitCast[type=double, index=0] */);
state_0 = state_0 | 0b10000000 /* add SpecializationActive[JSToUInt32Node.doDoubleRepresentableAsLong(double)] */;
this.state_0_ = state_0;
return doDoubleRepresentableAsLong(arg0Value_);
}
if ((JSGuards.isDoubleLargerThan2e32(arg0Value_)) && (!(JSGuards.isDoubleRepresentableAsLong(arg0Value_)))) {
state_0 = (state_0 | (doubleCast0 << 17) /* set-int ImplicitCast[type=double, index=0] */);
state_0 = state_0 | 0b100000000 /* add SpecializationActive[JSToUInt32Node.doDouble(double)] */;
this.state_0_ = state_0;
return doDouble(arg0Value_);
}
}
}
if ((JSGuards.isJSNull(arg0Value))) {
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[JSToUInt32Node.doNull(Object)] */;
this.state_0_ = state_0;
return doNull(arg0Value);
}
if ((JSGuards.isUndefined(arg0Value))) {
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[JSToUInt32Node.doUndefined(Object)] */;
this.state_0_ = state_0;
return doUndefined(arg0Value);
}
if (arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
JSStringToNumberNode stringToNumberNode__ = this.insert((JSStringToNumberNode.create()));
Objects.requireNonNull(stringToNumberNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.string_stringToNumberNode_ = stringToNumberNode__;
state_0 = state_0 | 0b100000000000 /* add SpecializationActive[JSToUInt32Node.doString(TruffleString, JSStringToNumberNode)] */;
this.state_0_ = state_0;
return doString(arg0Value_, stringToNumberNode__);
}
if (arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
state_0 = state_0 | 0b1000000000000 /* add SpecializationActive[JSToUInt32Node.doSymbol(Symbol)] */;
this.state_0_ = state_0;
return doSymbol(arg0Value_);
}
if (arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
state_0 = state_0 | 0b10000000000000 /* add SpecializationActive[JSToUInt32Node.doBigInt(BigInt)] */;
this.state_0_ = state_0;
return doBigInt(arg0Value_);
}
if (arg0Value instanceof JSOverloadedOperatorsObject) {
JSOverloadedOperatorsObject arg0Value_ = (JSOverloadedOperatorsObject) arg0Value;
if ((isUnsignedRightShift())) {
JSOverloadedBinaryNode overloadedOperatorNode__ = this.insert((JSOverloadedBinaryNode.createNumeric(getOverloadedOperatorName())));
Objects.requireNonNull(overloadedOperatorNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.overloadedOperator_overloadedOperatorNode_ = overloadedOperatorNode__;
state_0 = state_0 | 0b100000000000000 /* add SpecializationActive[JSToUInt32Node.doOverloadedOperator(JSOverloadedOperatorsObject, JSOverloadedBinaryNode)] */;
this.state_0_ = state_0;
return doOverloadedOperator(arg0Value_, overloadedOperatorNode__);
}
}
if (arg0Value instanceof JSObject) {
JSObject arg0Value_ = (JSObject) arg0Value;
if ((!(isUnsignedRightShift()) || !(hasOverloadedOperators(arg0Value_)))) {
JSToNumberNode toNumberNode__ = this.insert((JSToNumberNode.create()));
Objects.requireNonNull(toNumberNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.jSObject_toNumberNode_ = toNumberNode__;
state_0 = state_0 | 0b1000000000000000 /* add SpecializationActive[JSToUInt32Node.doJSObject(JSObject, JSToNumberNode)] */;
this.state_0_ = state_0;
return doJSObject(arg0Value_, toNumberNode__);
}
}
if ((JSRuntime.isForeignObject(arg0Value))) {
ForeignObjectData s16_ = this.insert(new ForeignObjectData());
JSToPrimitiveNode toPrimitiveNode__ = s16_.insert((JSToPrimitiveNode.createHintNumber()));
Objects.requireNonNull(toPrimitiveNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s16_.toPrimitiveNode_ = toPrimitiveNode__;
JSToUInt32Node toUInt32Node__ = s16_.insert((JSToUInt32Node.create()));
Objects.requireNonNull(toUInt32Node__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s16_.toUInt32Node_ = toUInt32Node__;
VarHandle.storeStoreFence();
this.foreignObject_cache = s16_;
state_0 = state_0 | 0x10000 /* add SpecializationActive[JSToUInt32Node.doForeignObject(Object, JSToPrimitiveNode, JSToUInt32Node)] */;
this.state_0_ = state_0;
return JSToUInt32Node.doForeignObject(arg0Value, toPrimitiveNode__, toUInt32Node__);
}
throw new UnsupportedSpecializationException(this, null, arg0Value);
}
@NeverDefault
public static JSToUInt32Node create(boolean unsignedRightShift, int shiftValue) {
return new JSToUInt32NodeGen(unsignedRightShift, shiftValue);
}
@GeneratedBy(JSToUInt32Node.class)
@DenyReplace
private static final class ForeignObjectData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link JSToUInt32Node#doForeignObject}
* Parameter: {@link JSToPrimitiveNode} toPrimitiveNode
*/
@Child JSToPrimitiveNode toPrimitiveNode_;
/**
* Source Info:
* Specialization: {@link JSToUInt32Node#doForeignObject}
* Parameter: {@link JSToUInt32Node} toUInt32Node
*/
@Child JSToUInt32Node toUInt32Node_;
ForeignObjectData() {
}
}
/**
* Debug Info:
* Specialization {@link JSToUInt32WrapperNode#doDefault}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(JSToUInt32WrapperNode.class)
@SuppressWarnings("javadoc")
public static final class JSToUInt32WrapperNodeGen extends JSToUInt32WrapperNode {
/**
* State Info:
* 0: SpecializationActive {@link JSToUInt32WrapperNode#doDefault}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSToUInt32WrapperNode#doDefault}
* Parameter: {@link JSToUInt32Node} toUInt32Node
*/
@Child private JSToUInt32Node toUInt32Node_;
private JSToUInt32WrapperNodeGen(JavaScriptNode operand, boolean unsignedRightShift, int shiftValue) {
super(operand, unsignedRightShift, shiftValue);
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object operandNodeValue_ = super.operandNode.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[JSToUInt32Node.JSToUInt32WrapperNode.doDefault(Object, JSToUInt32Node)] */) {
{
JSToUInt32Node toUInt32Node__ = this.toUInt32Node_;
if (toUInt32Node__ != null) {
return JSToUInt32WrapperNode.doDefault(operandNodeValue_, toUInt32Node__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(operandNodeValue_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
execute(frameValue);
return;
}
private Object executeAndSpecialize(Object operandNodeValue) {
int state_0 = this.state_0_;
JSToUInt32Node toUInt32Node__ = this.insert((JSToUInt32Node.create(unsignedRightShift, shiftValue)));
Objects.requireNonNull(toUInt32Node__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toUInt32Node_ = toUInt32Node__;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSToUInt32Node.JSToUInt32WrapperNode.doDefault(Object, JSToUInt32Node)] */;
this.state_0_ = state_0;
return JSToUInt32WrapperNode.doDefault(operandNodeValue, toUInt32Node__);
}
@NeverDefault
public static JSToUInt32WrapperNode create(JavaScriptNode operand, boolean unsignedRightShift, int shiftValue) {
return new JSToUInt32WrapperNodeGen(operand, unsignedRightShift, shiftValue);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy