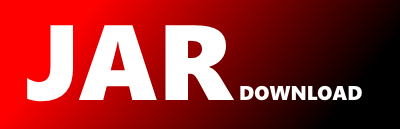
com.oracle.truffle.js.nodes.cast.JSTrimWhitespaceNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.cast;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.ReadCharUTF16Node;
import com.oracle.truffle.api.strings.TruffleString.SubstringByteIndexNode;
import com.oracle.truffle.js.nodes.JSGuards;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSTrimWhitespaceNode#doStringZero}
* Activation probability: 0.48333
* With/without class size: 9/0 bytes
* Specialization {@link JSTrimWhitespaceNode#doStringNoWhitespace}
* Activation probability: 0.33333
* With/without class size: 9/0 bytes
* Specialization {@link JSTrimWhitespaceNode#doString}
* Activation probability: 0.18333
* With/without class size: 8/5 bytes
*
*/
@GeneratedBy(JSTrimWhitespaceNode.class)
@SuppressWarnings("javadoc")
public final class JSTrimWhitespaceNodeGen extends JSTrimWhitespaceNode {
private static final StateField STATE_1_UPDATER = StateField.create(MethodHandles.lookup(), "state_1_");
private static final StateField STATE_0_JSTrimWhitespaceNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link JSTrimWhitespaceNode#doStringNoWhitespace}
* Parameter: {@link InlinedConditionProfile} isFastNonWhitespace
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_IS_FAST_NON_WHITESPACE = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_1_UPDATER.subUpdater(0, 2)));
/**
* Source Info:
* Specialization: {@link JSTrimWhitespaceNode#doStringNoWhitespace}
* Parameter: {@link InlinedConditionProfile} isFastWhitespace
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_IS_FAST_WHITESPACE = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_1_UPDATER.subUpdater(2, 2)));
/**
* Source Info:
* Specialization: {@link JSTrimWhitespaceNode#doString}
* Parameter: {@link InlinedBranchProfile} startsWithWhitespaceBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_STRING_STARTS_WITH_WHITESPACE_BRANCH_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_JSTrimWhitespaceNode_UPDATER.subUpdater(3, 1)));
/**
* Source Info:
* Specialization: {@link JSTrimWhitespaceNode#doString}
* Parameter: {@link InlinedBranchProfile} endsWithWhitespaceBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_STRING_ENDS_WITH_WHITESPACE_BRANCH_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_JSTrimWhitespaceNode_UPDATER.subUpdater(4, 1)));
/**
* Source Info:
* Specialization: {@link JSTrimWhitespaceNode#doString}
* Parameter: {@link InlinedConditionProfile} isEmpty
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_STRING_IS_EMPTY_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_JSTrimWhitespaceNode_UPDATER.subUpdater(5, 2)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link JSTrimWhitespaceNode#doStringZero}
* 1: SpecializationActive {@link JSTrimWhitespaceNode#doStringNoWhitespace}
* 2: SpecializationActive {@link JSTrimWhitespaceNode#doString}
* 3: InlinedCache
* Specialization: {@link JSTrimWhitespaceNode#doString}
* Parameter: {@link InlinedBranchProfile} startsWithWhitespaceBranch
* Inline method: {@link InlinedBranchProfile#inline}
* 4: InlinedCache
* Specialization: {@link JSTrimWhitespaceNode#doString}
* Parameter: {@link InlinedBranchProfile} endsWithWhitespaceBranch
* Inline method: {@link InlinedBranchProfile#inline}
* 5-6: InlinedCache
* Specialization: {@link JSTrimWhitespaceNode#doString}
* Parameter: {@link InlinedConditionProfile} isEmpty
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link JSTrimWhitespaceNode#doStringNoWhitespace}
* Parameter: {@link InlinedConditionProfile} isFastNonWhitespace
* Inline method: {@link InlinedConditionProfile#inline}
* 2-3: InlinedCache
* Specialization: {@link JSTrimWhitespaceNode#doStringNoWhitespace}
* Parameter: {@link InlinedConditionProfile} isFastWhitespace
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_1_;
/**
* Source Info:
* Specialization: {@link JSTrimWhitespaceNode#doStringNoWhitespace}
* Parameter: {@link ReadCharUTF16Node} readRawNode
*/
@Child private ReadCharUTF16Node readRawNode;
/**
* Source Info:
* Specialization: {@link JSTrimWhitespaceNode#doString}
* Parameter: {@link SubstringByteIndexNode} substringNode
*/
@Child private SubstringByteIndexNode string_substringNode_;
private JSTrimWhitespaceNodeGen() {
}
@Override
public TruffleString executeString(TruffleString arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111) != 0 /* is SpecializationActive[JSTrimWhitespaceNode.doStringZero(TruffleString)] || SpecializationActive[JSTrimWhitespaceNode.doStringNoWhitespace(TruffleString, ReadCharUTF16Node, InlinedConditionProfile, InlinedConditionProfile)] || SpecializationActive[JSTrimWhitespaceNode.doString(TruffleString, ReadCharUTF16Node, InlinedConditionProfile, InlinedConditionProfile, SubstringByteIndexNode, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSTrimWhitespaceNode.doStringZero(TruffleString)] */) {
if ((JSGuards.stringLength(arg0Value) == 0)) {
return JSTrimWhitespaceNode.doStringZero(arg0Value);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSTrimWhitespaceNode.doStringNoWhitespace(TruffleString, ReadCharUTF16Node, InlinedConditionProfile, InlinedConditionProfile)] */) {
{
ReadCharUTF16Node readRawNode_ = this.readRawNode;
if (readRawNode_ != null) {
if ((JSGuards.stringLength(arg0Value) > 0) && (!(startsOrEndsWithWhitespace(readRawNode_, arg0Value, INLINED_IS_FAST_NON_WHITESPACE, INLINED_IS_FAST_WHITESPACE)))) {
return JSTrimWhitespaceNode.doStringNoWhitespace(arg0Value, readRawNode_, INLINED_IS_FAST_NON_WHITESPACE, INLINED_IS_FAST_WHITESPACE);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSTrimWhitespaceNode.doString(TruffleString, ReadCharUTF16Node, InlinedConditionProfile, InlinedConditionProfile, SubstringByteIndexNode, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile)] */) {
{
ReadCharUTF16Node readRawNode_1 = this.readRawNode;
if (readRawNode_1 != null) {
SubstringByteIndexNode substringNode__ = this.string_substringNode_;
if (substringNode__ != null) {
if ((JSGuards.stringLength(arg0Value) > 0) && (startsOrEndsWithWhitespace(readRawNode_1, arg0Value, INLINED_IS_FAST_NON_WHITESPACE, INLINED_IS_FAST_WHITESPACE))) {
return doString(arg0Value, readRawNode_1, INLINED_IS_FAST_NON_WHITESPACE, INLINED_IS_FAST_WHITESPACE, substringNode__, INLINED_STRING_STARTS_WITH_WHITESPACE_BRANCH_, INLINED_STRING_ENDS_WITH_WHITESPACE_BRANCH_, INLINED_STRING_IS_EMPTY_);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private TruffleString executeAndSpecialize(TruffleString arg0Value) {
int state_0 = this.state_0_;
if ((JSGuards.stringLength(arg0Value) == 0)) {
state_0 = state_0 | 0b1 /* add SpecializationActive[JSTrimWhitespaceNode.doStringZero(TruffleString)] */;
this.state_0_ = state_0;
return JSTrimWhitespaceNode.doStringZero(arg0Value);
}
if ((JSGuards.stringLength(arg0Value) > 0)) {
ReadCharUTF16Node readRawNode_;
ReadCharUTF16Node readRawNode__shared = this.readRawNode;
if (readRawNode__shared != null) {
readRawNode_ = readRawNode__shared;
} else {
readRawNode_ = this.insert((ReadCharUTF16Node.create()));
if (readRawNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((!(startsOrEndsWithWhitespace(readRawNode_, arg0Value, INLINED_IS_FAST_NON_WHITESPACE, INLINED_IS_FAST_WHITESPACE)))) {
if (this.readRawNode == null) {
VarHandle.storeStoreFence();
this.readRawNode = readRawNode_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[JSTrimWhitespaceNode.doStringNoWhitespace(TruffleString, ReadCharUTF16Node, InlinedConditionProfile, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return JSTrimWhitespaceNode.doStringNoWhitespace(arg0Value, readRawNode_, INLINED_IS_FAST_NON_WHITESPACE, INLINED_IS_FAST_WHITESPACE);
}
}
if ((JSGuards.stringLength(arg0Value) > 0)) {
ReadCharUTF16Node readRawNode_1;
ReadCharUTF16Node readRawNode_1_shared = this.readRawNode;
if (readRawNode_1_shared != null) {
readRawNode_1 = readRawNode_1_shared;
} else {
readRawNode_1 = this.insert((ReadCharUTF16Node.create()));
if (readRawNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((startsOrEndsWithWhitespace(readRawNode_1, arg0Value, INLINED_IS_FAST_NON_WHITESPACE, INLINED_IS_FAST_WHITESPACE))) {
if (this.readRawNode == null) {
VarHandle.storeStoreFence();
this.readRawNode = readRawNode_1;
}
SubstringByteIndexNode substringNode__ = this.insert((SubstringByteIndexNode.create()));
Objects.requireNonNull(substringNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.string_substringNode_ = substringNode__;
state_0 = state_0 | 0b100 /* add SpecializationActive[JSTrimWhitespaceNode.doString(TruffleString, ReadCharUTF16Node, InlinedConditionProfile, InlinedConditionProfile, SubstringByteIndexNode, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return doString(arg0Value, readRawNode_1, INLINED_IS_FAST_NON_WHITESPACE, INLINED_IS_FAST_WHITESPACE, substringNode__, INLINED_STRING_STARTS_WITH_WHITESPACE_BRANCH_, INLINED_STRING_ENDS_WITH_WHITESPACE_BRANCH_, INLINED_STRING_IS_EMPTY_);
}
}
throw new UnsupportedSpecializationException(this, null, arg0Value);
}
@TruffleBoundary
private static UnsupportedSpecializationException newUnsupportedSpecializationException1(Node thisNode_, Object arg0Value) {
return new UnsupportedSpecializationException(thisNode_, null, arg0Value);
}
@NeverDefault
public static JSTrimWhitespaceNode create() {
return new JSTrimWhitespaceNodeGen();
}
@NeverDefault
public static JSTrimWhitespaceNode getUncached() {
return JSTrimWhitespaceNodeGen.UNCACHED;
}
@GeneratedBy(JSTrimWhitespaceNode.class)
@DenyReplace
private static final class Uncached extends JSTrimWhitespaceNode implements UnadoptableNode {
@TruffleBoundary
@Override
public TruffleString executeString(TruffleString arg0Value) {
if ((JSGuards.stringLength(arg0Value) == 0)) {
return JSTrimWhitespaceNode.doStringZero(arg0Value);
}
if ((JSGuards.stringLength(arg0Value) > 0) && (!(startsOrEndsWithWhitespace((ReadCharUTF16Node.getUncached()), arg0Value, (InlinedConditionProfile.getUncached()), (InlinedConditionProfile.getUncached()))))) {
return JSTrimWhitespaceNode.doStringNoWhitespace(arg0Value, (ReadCharUTF16Node.getUncached()), (InlinedConditionProfile.getUncached()), (InlinedConditionProfile.getUncached()));
}
if ((JSGuards.stringLength(arg0Value) > 0) && (startsOrEndsWithWhitespace((ReadCharUTF16Node.getUncached()), arg0Value, (InlinedConditionProfile.getUncached()), (InlinedConditionProfile.getUncached())))) {
return doString(arg0Value, (ReadCharUTF16Node.getUncached()), (InlinedConditionProfile.getUncached()), (InlinedConditionProfile.getUncached()), (SubstringByteIndexNode.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedConditionProfile.getUncached()));
}
throw newUnsupportedSpecializationException1(this, arg0Value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy