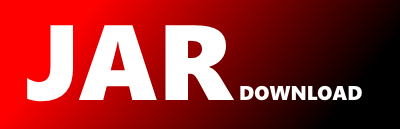
com.oracle.truffle.js.nodes.cast.ToArrayIndexNoToPropertyKeyNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.cast;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.InlineSupport;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.ReadCharUTF16Node;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.JSTypesGen;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.JSConfig;
import com.oracle.truffle.js.runtime.JSRuntime;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#doInteger}
* Activation probability: 0.13626
* With/without class size: 5/0 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#doIntegerNonArrayIndex}
* Activation probability: 0.12637
* With/without class size: 5/0 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#doLong}
* Activation probability: 0.11648
* With/without class size: 5/0 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#doLongNonArrayIndex}
* Activation probability: 0.10659
* With/without class size: 5/0 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#doDoubleAsIntIndex}
* Activation probability: 0.09670
* With/without class size: 5/0 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#doDoubleAsUintIndex}
* Activation probability: 0.08681
* With/without class size: 5/0 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#doDoubleNonArrayIndex}
* Activation probability: 0.07692
* With/without class size: 4/0 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#doBigInt}
* Activation probability: 0.06703
* With/without class size: 4/0 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#doBigIntNonArrayIndex}
* Activation probability: 0.05714
* With/without class size: 4/0 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#convertFromString}
* Activation probability: 0.04725
* With/without class size: 5/5 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#convertFromStringNotInRange}
* Activation probability: 0.03736
* With/without class size: 4/0 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#doNonArrayIndex}
* Activation probability: 0.02747
* With/without class size: 4/4 bytes
* Specialization {@link ToArrayIndexNoToPropertyKeyNode#doNonArrayIndex}
* Activation probability: 0.01758
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(ToArrayIndexNoToPropertyKeyNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class ToArrayIndexNoToPropertyKeyNodeGen {
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#convertFromString_stringReadNode_}
*
- {@link Inlined#nonArrayIndex0_cache}
*
*/
@NeverDefault
public static ToArrayIndexNoToPropertyKeyNode inline(@RequiredField(bits = 19, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new ToArrayIndexNoToPropertyKeyNodeGen.Inlined(target);
}
@GeneratedBy(ToArrayIndexNoToPropertyKeyNode.class)
@DenyReplace
private static final class Inlined extends ToArrayIndexNoToPropertyKeyNode implements UnadoptableNode {
/**
* State Info:
* 0: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#doInteger}
* 1: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#doIntegerNonArrayIndex}
* 2: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#doLong}
* 3: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#doLongNonArrayIndex}
* 4: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#doDoubleAsIntIndex}
* 5: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#doDoubleAsUintIndex}
* 6: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#doDoubleNonArrayIndex}
* 7: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#doBigInt}
* 8: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#doBigIntNonArrayIndex}
* 9: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#convertFromString}
* 10: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#convertFromStringNotInRange}
* 11: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#doNonArrayIndex}
* 12: SpecializationActive {@link ToArrayIndexNoToPropertyKeyNode#doNonArrayIndex}
* 13-15: ImplicitCast[type=double, index=1]
* 16: InlinedCache
* Specialization: {@link ToArrayIndexNoToPropertyKeyNode#convertFromString}
* Parameter: {@link InlinedBranchProfile} startsWithDigitBranch
* Inline method: {@link InlinedBranchProfile#inline}
* 17: InlinedCache
* Specialization: {@link ToArrayIndexNoToPropertyKeyNode#convertFromString}
* Parameter: {@link InlinedBranchProfile} isArrayIndexBranch
* Inline method: {@link InlinedBranchProfile#inline}
* 18: InlinedCache
* Specialization: {@link ToArrayIndexNoToPropertyKeyNode#convertFromString}
* Parameter: {@link InlinedBranchProfile} invalidArrayIndexBranch
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
private final StateField state_0_;
private final ReferenceField convertFromString_stringReadNode_;
private final ReferenceField nonArrayIndex0_cache;
/**
* Source Info:
* Specialization: {@link ToArrayIndexNoToPropertyKeyNode#convertFromString}
* Parameter: {@link InlinedBranchProfile} startsWithDigitBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private final InlinedBranchProfile convertFromString_startsWithDigitBranch_;
/**
* Source Info:
* Specialization: {@link ToArrayIndexNoToPropertyKeyNode#convertFromString}
* Parameter: {@link InlinedBranchProfile} isArrayIndexBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private final InlinedBranchProfile convertFromString_isArrayIndexBranch_;
/**
* Source Info:
* Specialization: {@link ToArrayIndexNoToPropertyKeyNode#convertFromString}
* Parameter: {@link InlinedBranchProfile} invalidArrayIndexBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private final InlinedBranchProfile convertFromString_invalidArrayIndexBranch_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(ToArrayIndexNoToPropertyKeyNode.class);
this.state_0_ = target.getState(0, 19);
this.convertFromString_stringReadNode_ = target.getReference(1, ReadCharUTF16Node.class);
this.nonArrayIndex0_cache = target.getReference(2, NonArrayIndex0Data.class);
this.convertFromString_startsWithDigitBranch_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, state_0_.subUpdater(16, 1)));
this.convertFromString_isArrayIndexBranch_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, state_0_.subUpdater(17, 1)));
this.convertFromString_invalidArrayIndexBranch_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, state_0_.subUpdater(18, 1)));
}
@ExplodeLoop
@Override
public long executeLong(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b1111111111111) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doInteger(int)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doIntegerNonArrayIndex(int)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doLong(long)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doLongNonArrayIndex(long)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleAsIntIndex(double)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleAsUintIndex(double)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleNonArrayIndex(double)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doBigInt(BigInt)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doBigIntNonArrayIndex(BigInt)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.convertFromString(Node, TruffleString, ReadCharUTF16Node, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.convertFromStringNotInRange(TruffleString)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(Object, InteropLibrary)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(Object, InteropLibrary)] */) {
if ((state_0 & 0b11) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doInteger(int)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doIntegerNonArrayIndex(int)] */ && arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doInteger(int)] */) {
if ((JSGuards.isIntArrayIndex(arg1Value_))) {
return ToArrayIndexNoToPropertyKeyNode.doInteger(arg1Value_);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doIntegerNonArrayIndex(int)] */) {
if ((!(JSGuards.isIntArrayIndex(arg1Value_)))) {
return ToArrayIndexNoToPropertyKeyNode.doIntegerNonArrayIndex(arg1Value_);
}
}
}
if ((state_0 & 0b1100) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doLong(long)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doLongNonArrayIndex(long)] */ && arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doLong(long)] */) {
if ((JSGuards.isLongArrayIndex(arg1Value_))) {
return ToArrayIndexNoToPropertyKeyNode.doLong(arg1Value_);
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doLongNonArrayIndex(long)] */) {
if ((!(JSGuards.isLongArrayIndex(arg1Value_)))) {
return ToArrayIndexNoToPropertyKeyNode.doLongNonArrayIndex(arg1Value_);
}
}
}
if ((state_0 & 0b1110000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleAsIntIndex(double)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleAsUintIndex(double)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleNonArrayIndex(double)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b1110000000000000) >>> 13 /* get-int ImplicitCast[type=double, index=1] */, arg1Value)) {
double arg1Value_ = JSTypesGen.asImplicitDouble((state_0 & 0b1110000000000000) >>> 13 /* get-int ImplicitCast[type=double, index=1] */, arg1Value);
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleAsIntIndex(double)] */) {
if ((ToArrayIndexNoToPropertyKeyNode.doubleIsIntIndex(arg1Value_))) {
return ToArrayIndexNoToPropertyKeyNode.doDoubleAsIntIndex(arg1Value_);
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleAsUintIndex(double)] */) {
if ((ToArrayIndexNoToPropertyKeyNode.doubleIsUintIndex(arg1Value_))) {
return ToArrayIndexNoToPropertyKeyNode.doDoubleAsUintIndex(arg1Value_);
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleNonArrayIndex(double)] */) {
if ((!(ToArrayIndexNoToPropertyKeyNode.doubleIsUintIndex(arg1Value_)))) {
return ToArrayIndexNoToPropertyKeyNode.doDoubleNonArrayIndex(arg1Value_);
}
}
}
if ((state_0 & 0b110000000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doBigInt(BigInt)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doBigIntNonArrayIndex(BigInt)] */ && arg1Value instanceof BigInt) {
BigInt arg1Value_ = (BigInt) arg1Value;
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doBigInt(BigInt)] */) {
if ((JSGuards.isBigIntArrayIndex(arg1Value_))) {
return ToArrayIndexNoToPropertyKeyNode.doBigInt(arg1Value_);
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doBigIntNonArrayIndex(BigInt)] */) {
return ToArrayIndexNoToPropertyKeyNode.doBigIntNonArrayIndex(arg1Value_);
}
}
if ((state_0 & 0b11000000000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.convertFromString(Node, TruffleString, ReadCharUTF16Node, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.convertFromStringNotInRange(TruffleString)] */ && arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.convertFromString(Node, TruffleString, ReadCharUTF16Node, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */) {
{
ReadCharUTF16Node stringReadNode__ = this.convertFromString_stringReadNode_.get(arg0Value);
if (stringReadNode__ != null) {
if ((JSRuntime.arrayIndexLengthInRange(arg1Value_))) {
assert InlineSupport.validate(arg0Value, this.state_0_, this.state_0_, this.state_0_);
return ToArrayIndexNoToPropertyKeyNode.convertFromString(arg0Value, arg1Value_, stringReadNode__, this.convertFromString_startsWithDigitBranch_, this.convertFromString_isArrayIndexBranch_, this.convertFromString_invalidArrayIndexBranch_);
}
}
}
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.convertFromStringNotInRange(TruffleString)] */) {
if ((!(JSRuntime.arrayIndexLengthInRange(arg1Value_)))) {
return ToArrayIndexNoToPropertyKeyNode.convertFromStringNotInRange(arg1Value_);
}
}
}
if ((state_0 & 0b1100000000000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(Object, InteropLibrary)] || SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(Object, InteropLibrary)] */) {
if ((state_0 & 0b100000000000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(Object, InteropLibrary)] */) {
NonArrayIndex0Data s11_ = this.nonArrayIndex0_cache.get(arg0Value);
while (s11_ != null) {
if ((s11_.interop_.accepts(arg1Value)) && (ToArrayIndexNoToPropertyKeyNode.notArrayIndex(arg1Value))) {
return ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(arg1Value, s11_.interop_);
}
s11_ = s11_.next_;
}
}
if ((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(Object, InteropLibrary)] */) {
if ((ToArrayIndexNoToPropertyKeyNode.notArrayIndex(arg1Value))) {
return this.nonArrayIndex1Boundary(state_0, arg0Value, arg1Value);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private long nonArrayIndex1Boundary(int state_0, Node arg0Value, Object arg1Value) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(arg0Value);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(arg1Value));
return ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(arg1Value, interop__);
}
} finally {
encapsulating_.set(prev_);
}
}
private long executeAndSpecialize(Node arg0Value, Object arg1Value) {
int state_0 = this.state_0_.get(arg0Value);
if (arg1Value instanceof Integer) {
int arg1Value_ = (int) arg1Value;
if ((JSGuards.isIntArrayIndex(arg1Value_))) {
state_0 = state_0 | 0b1 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doInteger(int)] */;
this.state_0_.set(arg0Value, state_0);
return ToArrayIndexNoToPropertyKeyNode.doInteger(arg1Value_);
}
if ((!(JSGuards.isIntArrayIndex(arg1Value_)))) {
state_0 = state_0 | 0b10 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doIntegerNonArrayIndex(int)] */;
this.state_0_.set(arg0Value, state_0);
return ToArrayIndexNoToPropertyKeyNode.doIntegerNonArrayIndex(arg1Value_);
}
}
if (arg1Value instanceof Long) {
long arg1Value_ = (long) arg1Value;
if ((JSGuards.isLongArrayIndex(arg1Value_))) {
state_0 = state_0 | 0b100 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doLong(long)] */;
this.state_0_.set(arg0Value, state_0);
return ToArrayIndexNoToPropertyKeyNode.doLong(arg1Value_);
}
if ((!(JSGuards.isLongArrayIndex(arg1Value_)))) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doLongNonArrayIndex(long)] */;
this.state_0_.set(arg0Value, state_0);
return ToArrayIndexNoToPropertyKeyNode.doLongNonArrayIndex(arg1Value_);
}
}
{
int doubleCast1;
if ((doubleCast1 = JSTypesGen.specializeImplicitDouble(arg1Value)) != 0) {
double arg1Value_ = JSTypesGen.asImplicitDouble(doubleCast1, arg1Value);
if (((state_0 & 0b100000)) == 0 /* is-not SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleAsUintIndex(double)] */) {
if ((ToArrayIndexNoToPropertyKeyNode.doubleIsIntIndex(arg1Value_))) {
state_0 = (state_0 | (doubleCast1 << 13) /* set-int ImplicitCast[type=double, index=1] */);
state_0 = state_0 | 0b10000 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleAsIntIndex(double)] */;
this.state_0_.set(arg0Value, state_0);
return ToArrayIndexNoToPropertyKeyNode.doDoubleAsIntIndex(arg1Value_);
}
}
if ((ToArrayIndexNoToPropertyKeyNode.doubleIsUintIndex(arg1Value_))) {
state_0 = state_0 & 0xffffffef /* remove SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleAsIntIndex(double)] */;
state_0 = (state_0 | (doubleCast1 << 13) /* set-int ImplicitCast[type=double, index=1] */);
state_0 = state_0 | 0b100000 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleAsUintIndex(double)] */;
this.state_0_.set(arg0Value, state_0);
return ToArrayIndexNoToPropertyKeyNode.doDoubleAsUintIndex(arg1Value_);
}
if ((!(ToArrayIndexNoToPropertyKeyNode.doubleIsUintIndex(arg1Value_)))) {
state_0 = (state_0 | (doubleCast1 << 13) /* set-int ImplicitCast[type=double, index=1] */);
state_0 = state_0 | 0b1000000 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doDoubleNonArrayIndex(double)] */;
this.state_0_.set(arg0Value, state_0);
return ToArrayIndexNoToPropertyKeyNode.doDoubleNonArrayIndex(arg1Value_);
}
}
}
if (arg1Value instanceof BigInt) {
BigInt arg1Value_ = (BigInt) arg1Value;
if ((JSGuards.isBigIntArrayIndex(arg1Value_))) {
state_0 = state_0 | 0b10000000 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doBigInt(BigInt)] */;
this.state_0_.set(arg0Value, state_0);
return ToArrayIndexNoToPropertyKeyNode.doBigInt(arg1Value_);
}
state_0 = state_0 | 0b100000000 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doBigIntNonArrayIndex(BigInt)] */;
this.state_0_.set(arg0Value, state_0);
return ToArrayIndexNoToPropertyKeyNode.doBigIntNonArrayIndex(arg1Value_);
}
if (arg1Value instanceof TruffleString) {
TruffleString arg1Value_ = (TruffleString) arg1Value;
if ((JSRuntime.arrayIndexLengthInRange(arg1Value_))) {
ReadCharUTF16Node stringReadNode__ = arg0Value.insert((ReadCharUTF16Node.create()));
Objects.requireNonNull(stringReadNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.convertFromString_stringReadNode_.set(arg0Value, stringReadNode__);
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.convertFromString(Node, TruffleString, ReadCharUTF16Node, InlinedBranchProfile, InlinedBranchProfile, InlinedBranchProfile)] */;
this.state_0_.set(arg0Value, state_0);
assert InlineSupport.validate(arg0Value, this.state_0_, this.state_0_, this.state_0_);
return ToArrayIndexNoToPropertyKeyNode.convertFromString(arg0Value, arg1Value_, stringReadNode__, this.convertFromString_startsWithDigitBranch_, this.convertFromString_isArrayIndexBranch_, this.convertFromString_invalidArrayIndexBranch_);
}
if ((!(JSRuntime.arrayIndexLengthInRange(arg1Value_)))) {
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.convertFromStringNotInRange(TruffleString)] */;
this.state_0_.set(arg0Value, state_0);
return ToArrayIndexNoToPropertyKeyNode.convertFromStringNotInRange(arg1Value_);
}
}
if (((state_0 & 0b1000000000000)) == 0 /* is-not SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(Object, InteropLibrary)] */) {
while (true) {
int count11_ = 0;
NonArrayIndex0Data s11_ = this.nonArrayIndex0_cache.getVolatile(arg0Value);
NonArrayIndex0Data s11_original = s11_;
while (s11_ != null) {
if ((s11_.interop_.accepts(arg1Value)) && (ToArrayIndexNoToPropertyKeyNode.notArrayIndex(arg1Value))) {
break;
}
count11_++;
s11_ = s11_.next_;
}
if (s11_ == null) {
if ((ToArrayIndexNoToPropertyKeyNode.notArrayIndex(arg1Value)) && count11_ < (JSConfig.InteropLibraryLimit)) {
// assert (s11_.interop_.accepts(arg1Value));
s11_ = arg0Value.insert(new NonArrayIndex0Data(s11_original));
InteropLibrary interop__ = s11_.insert((INTEROP_LIBRARY_.create(arg1Value)));
Objects.requireNonNull(interop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s11_.interop_ = interop__;
if (!this.nonArrayIndex0_cache.compareAndSet(arg0Value, s11_original, s11_)) {
continue;
}
state_0 = state_0 | 0b100000000000 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(Object, InteropLibrary)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s11_ != null) {
return ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(arg1Value, s11_.interop_);
}
break;
}
}
{
InteropLibrary interop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(arg0Value);
try {
if ((ToArrayIndexNoToPropertyKeyNode.notArrayIndex(arg1Value))) {
interop__ = (INTEROP_LIBRARY_.getUncached(arg1Value));
this.nonArrayIndex0_cache.set(arg0Value, null);
state_0 = state_0 & 0xfffff7ff /* remove SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(Object, InteropLibrary)] */;
state_0 = state_0 | 0b1000000000000 /* add SpecializationActive[ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(Object, InteropLibrary)] */;
this.state_0_.set(arg0Value, state_0);
return ToArrayIndexNoToPropertyKeyNode.doNonArrayIndex(arg1Value, interop__);
}
} finally {
encapsulating_.set(prev_);
}
}
}
throw new UnsupportedSpecializationException(this, null, arg0Value, arg1Value);
}
}
@GeneratedBy(ToArrayIndexNoToPropertyKeyNode.class)
@DenyReplace
private static final class NonArrayIndex0Data extends Node implements SpecializationDataNode {
@Child NonArrayIndex0Data next_;
/**
* Source Info:
* Specialization: {@link ToArrayIndexNoToPropertyKeyNode#doNonArrayIndex}
* Parameter: {@link InteropLibrary} interop
*/
@Child InteropLibrary interop_;
NonArrayIndex0Data(NonArrayIndex0Data next_) {
this.next_ = next_;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy