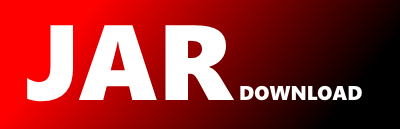
com.oracle.truffle.js.nodes.function.JSLoadNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.function;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.DirectCallNode;
import com.oracle.truffle.api.nodes.IndirectCallNode;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.source.Source;
import com.oracle.truffle.js.nodes.interop.ImportValueNode;
import com.oracle.truffle.js.runtime.JSRealm;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
/**
* Debug Info:
* Specialization {@link JSLoadNode#cachedLoad}
* Activation probability: 0.65000
* With/without class size: 19/8 bytes
* Specialization {@link JSLoadNode#uncachedLoad}
* Activation probability: 0.35000
* With/without class size: 11/4 bytes
*
*/
@GeneratedBy(JSLoadNode.class)
@SuppressWarnings("javadoc")
public final class JSLoadNodeGen extends JSLoadNode {
static final ReferenceField CACHED_LOAD_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "cachedLoad_cache", CachedLoadData.class);
/**
* State Info:
* 0: SpecializationActive {@link JSLoadNode#cachedLoad}
* 1: SpecializationActive {@link JSLoadNode#uncachedLoad}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSLoadNode#cachedLoad}
* Parameter: {@link ImportValueNode} importValue
*/
@Child private ImportValueNode importValue;
@UnsafeAccessedField @Child private CachedLoadData cachedLoad_cache;
/**
* Source Info:
* Specialization: {@link JSLoadNode#uncachedLoad}
* Parameter: {@link IndirectCallNode} callNode
*/
@Child private IndirectCallNode uncachedLoad_callNode_;
private JSLoadNodeGen() {
}
@Override
public Object executeLoad(Source arg0Value, JSRealm arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[JSLoadNode.cachedLoad(Source, JSRealm, ImportValueNode, Source, DirectCallNode)] || SpecializationActive[JSLoadNode.uncachedLoad(Source, JSRealm, ImportValueNode, IndirectCallNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSLoadNode.cachedLoad(Source, JSRealm, ImportValueNode, Source, DirectCallNode)] */) {
CachedLoadData s0_ = this.cachedLoad_cache;
if (s0_ != null) {
{
ImportValueNode importValue_ = this.importValue;
if (importValue_ != null) {
assert DSLSupport.assertIdempotence((JSLoadNode.isCached(s0_.cachedSource_)));
if ((JSLoadNode.equals(arg0Value, s0_.cachedSource_))) {
return JSLoadNode.cachedLoad(arg0Value, arg1Value, importValue_, s0_.cachedSource_, s0_.callNode_);
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSLoadNode.uncachedLoad(Source, JSRealm, ImportValueNode, IndirectCallNode)] */) {
{
ImportValueNode importValue_1 = this.importValue;
if (importValue_1 != null) {
IndirectCallNode callNode__ = this.uncachedLoad_callNode_;
if (callNode__ != null) {
return JSLoadNode.uncachedLoad(arg0Value, arg1Value, importValue_1, callNode__);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(Source arg0Value, JSRealm arg1Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSLoadNode.uncachedLoad(Source, JSRealm, ImportValueNode, IndirectCallNode)] */) {
while (true) {
int count0_ = 0;
CachedLoadData s0_ = CACHED_LOAD_CACHE_UPDATER.getVolatile(this);
CachedLoadData s0_original = s0_;
while (s0_ != null) {
{
ImportValueNode importValue_ = this.importValue;
if (importValue_ != null) {
assert DSLSupport.assertIdempotence((JSLoadNode.isCached(s0_.cachedSource_)));
if ((JSLoadNode.equals(arg0Value, s0_.cachedSource_))) {
break;
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
Source cachedSource__ = (arg0Value);
if ((JSLoadNode.isCached(cachedSource__)) && (JSLoadNode.equals(arg0Value, cachedSource__))) {
s0_ = this.insert(new CachedLoadData());
ImportValueNode importValue_;
ImportValueNode importValue__shared = this.importValue;
if (importValue__shared != null) {
importValue_ = importValue__shared;
} else {
importValue_ = s0_.insert((ImportValueNode.create()));
if (importValue_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.importValue == null) {
this.importValue = importValue_;
}
s0_.cachedSource_ = cachedSource__;
s0_.callNode_ = s0_.insert((DirectCallNode.create(JSLoadNode.loadScript(arg0Value, arg1Value))));
if (!CACHED_LOAD_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[JSLoadNode.cachedLoad(Source, JSRealm, ImportValueNode, Source, DirectCallNode)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return JSLoadNode.cachedLoad(arg0Value, arg1Value, this.importValue, s0_.cachedSource_, s0_.callNode_);
}
break;
}
}
ImportValueNode importValue_1;
ImportValueNode importValue_1_shared = this.importValue;
if (importValue_1_shared != null) {
importValue_1 = importValue_1_shared;
} else {
importValue_1 = this.insert((ImportValueNode.create()));
if (importValue_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.importValue == null) {
VarHandle.storeStoreFence();
this.importValue = importValue_1;
}
VarHandle.storeStoreFence();
this.uncachedLoad_callNode_ = this.insert((IndirectCallNode.create()));
this.cachedLoad_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[JSLoadNode.cachedLoad(Source, JSRealm, ImportValueNode, Source, DirectCallNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSLoadNode.uncachedLoad(Source, JSRealm, ImportValueNode, IndirectCallNode)] */;
this.state_0_ = state_0;
return JSLoadNode.uncachedLoad(arg0Value, arg1Value, importValue_1, this.uncachedLoad_callNode_);
}
@NeverDefault
public static JSLoadNode create() {
return new JSLoadNodeGen();
}
@GeneratedBy(JSLoadNode.class)
@DenyReplace
private static final class CachedLoadData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link JSLoadNode#cachedLoad}
* Parameter: {@link Source} cachedSource
*/
@CompilationFinal Source cachedSource_;
/**
* Source Info:
* Specialization: {@link JSLoadNode#cachedLoad}
* Parameter: {@link DirectCallNode} callNode
*/
@Child DirectCallNode callNode_;
CachedLoadData() {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy