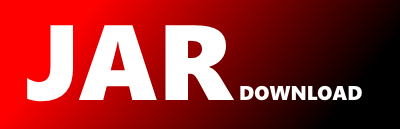
com.oracle.truffle.js.nodes.interop.JSInteropInvokeNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.interop;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.interop.UnknownIdentifierException;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.EqualNode;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.access.PropertyGetNode;
import com.oracle.truffle.js.nodes.access.ReadElementNode;
import com.oracle.truffle.js.nodes.function.JSFunctionCallNode;
import com.oracle.truffle.js.nodes.unary.IsCallableNode;
import com.oracle.truffle.js.nodes.unary.IsCallableNodeGen;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link JSInteropInvokeNode#doCached}
* Activation probability: 0.65000
* With/without class size: 22/12 bytes
* Specialization {@link JSInteropInvokeNode#doUncached}
* Activation probability: 0.35000
* With/without class size: 11/4 bytes
*
*/
@GeneratedBy(JSInteropInvokeNode.class)
@SuppressWarnings("javadoc")
public final class JSInteropInvokeNodeGen extends JSInteropInvokeNode {
static final ReferenceField CACHED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "cached_cache", CachedData.class);
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link JSInteropInvokeNode#doCached}
* 1: SpecializationActive {@link JSInteropInvokeNode#doUncached}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSInteropInvokeNode#doCached}
* Parameter: {@link IsCallableNode} isCallableNode
*/
@Child private IsCallableNode isCallableNode;
/**
* Source Info:
* Specialization: {@link JSInteropInvokeNode#doCached}
* Parameter: {@link JSFunctionCallNode} callNode
*/
@Child private JSFunctionCallNode callNode;
/**
* Source Info:
* Specialization: {@link JSInteropInvokeNode#doCached}
* Parameter: {@link ImportValueNode} importValueNode
*/
@Child private ImportValueNode importValueNode;
@UnsafeAccessedField @Child private CachedData cached_cache;
/**
* Source Info:
* Specialization: {@link JSInteropInvokeNode#doUncached}
* Parameter: {@link ReadElementNode} readNode
*/
@Child private ReadElementNode uncached_readNode_;
private JSInteropInvokeNodeGen() {
}
@Override
public Object execute(JSDynamicObject arg0Value, TruffleString arg1Value, Object[] arg2Value) throws UnknownIdentifierException, UnsupportedMessageException {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[JSInteropInvokeNode.doCached(JSDynamicObject, TruffleString, Object[], TruffleString, EqualNode, PropertyGetNode, IsCallableNode, JSFunctionCallNode, ImportValueNode)] || SpecializationActive[JSInteropInvokeNode.doUncached(JSDynamicObject, TruffleString, Object[], ReadElementNode, IsCallableNode, JSFunctionCallNode, ImportValueNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSInteropInvokeNode.doCached(JSDynamicObject, TruffleString, Object[], TruffleString, EqualNode, PropertyGetNode, IsCallableNode, JSFunctionCallNode, ImportValueNode)] */) {
CachedData s0_ = this.cached_cache;
if (s0_ != null) {
{
IsCallableNode isCallableNode_ = this.isCallableNode;
if (isCallableNode_ != null) {
JSFunctionCallNode callNode_ = this.callNode;
if (callNode_ != null) {
ImportValueNode importValueNode_ = this.importValueNode;
if (importValueNode_ != null) {
if ((JSGuards.stringEquals(s0_.equalNode_, s0_.cachedName_, arg1Value))) {
return doCached(arg0Value, arg1Value, arg2Value, s0_.cachedName_, s0_.equalNode_, s0_.functionPropertyGetNode_, isCallableNode_, callNode_, importValueNode_);
}
}
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSInteropInvokeNode.doUncached(JSDynamicObject, TruffleString, Object[], ReadElementNode, IsCallableNode, JSFunctionCallNode, ImportValueNode)] */) {
{
ReadElementNode readNode__ = this.uncached_readNode_;
if (readNode__ != null) {
IsCallableNode isCallableNode_1 = this.isCallableNode;
if (isCallableNode_1 != null) {
JSFunctionCallNode callNode_1 = this.callNode;
if (callNode_1 != null) {
ImportValueNode importValueNode_1 = this.importValueNode;
if (importValueNode_1 != null) {
return doUncached(arg0Value, arg1Value, arg2Value, readNode__, isCallableNode_1, callNode_1, importValueNode_1);
}
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
@SuppressWarnings("unused")
private Object executeAndSpecialize(JSDynamicObject arg0Value, TruffleString arg1Value, Object[] arg2Value) throws UnknownIdentifierException, UnsupportedMessageException {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[JSInteropInvokeNode.doUncached(JSDynamicObject, TruffleString, Object[], ReadElementNode, IsCallableNode, JSFunctionCallNode, ImportValueNode)] */) {
while (true) {
int count0_ = 0;
CachedData s0_ = CACHED_CACHE_UPDATER.getVolatile(this);
CachedData s0_original = s0_;
while (s0_ != null) {
{
IsCallableNode isCallableNode_ = this.isCallableNode;
if (isCallableNode_ != null) {
JSFunctionCallNode callNode_ = this.callNode;
if (callNode_ != null) {
ImportValueNode importValueNode_ = this.importValueNode;
if (importValueNode_ != null) {
if ((JSGuards.stringEquals(s0_.equalNode_, s0_.cachedName_, arg1Value))) {
break;
}
}
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
TruffleString cachedName__ = (arg1Value);
EqualNode equalNode__ = this.insert((EqualNode.create()));
if ((JSGuards.stringEquals(equalNode__, cachedName__, arg1Value))) {
s0_ = this.insert(new CachedData());
s0_.cachedName_ = cachedName__;
Objects.requireNonNull(s0_.insert(equalNode__), "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s0_.equalNode_ = equalNode__;
PropertyGetNode functionPropertyGetNode__ = s0_.insert((createGetProperty(cachedName__)));
Objects.requireNonNull(functionPropertyGetNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s0_.functionPropertyGetNode_ = functionPropertyGetNode__;
IsCallableNode isCallableNode_;
IsCallableNode isCallableNode__shared = this.isCallableNode;
if (isCallableNode__shared != null) {
isCallableNode_ = isCallableNode__shared;
} else {
isCallableNode_ = s0_.insert((IsCallableNode.create()));
if (isCallableNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.isCallableNode == null) {
this.isCallableNode = isCallableNode_;
}
JSFunctionCallNode callNode_;
JSFunctionCallNode callNode__shared = this.callNode;
if (callNode__shared != null) {
callNode_ = callNode__shared;
} else {
callNode_ = s0_.insert((JSFunctionCallNode.createCall()));
if (callNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callNode == null) {
this.callNode = callNode_;
}
ImportValueNode importValueNode_;
ImportValueNode importValueNode__shared = this.importValueNode;
if (importValueNode__shared != null) {
importValueNode_ = importValueNode__shared;
} else {
importValueNode_ = s0_.insert((ImportValueNode.create()));
if (importValueNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.importValueNode == null) {
this.importValueNode = importValueNode_;
}
if (!CACHED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[JSInteropInvokeNode.doCached(JSDynamicObject, TruffleString, Object[], TruffleString, EqualNode, PropertyGetNode, IsCallableNode, JSFunctionCallNode, ImportValueNode)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return doCached(arg0Value, arg1Value, arg2Value, s0_.cachedName_, s0_.equalNode_, s0_.functionPropertyGetNode_, this.isCallableNode, this.callNode, this.importValueNode);
}
break;
}
}
ReadElementNode readNode__ = this.insert((ReadElementNode.create(getLanguage().getJSContext())));
Objects.requireNonNull(readNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.uncached_readNode_ = readNode__;
IsCallableNode isCallableNode_1;
IsCallableNode isCallableNode_1_shared = this.isCallableNode;
if (isCallableNode_1_shared != null) {
isCallableNode_1 = isCallableNode_1_shared;
} else {
isCallableNode_1 = this.insert((IsCallableNode.create()));
if (isCallableNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.isCallableNode == null) {
VarHandle.storeStoreFence();
this.isCallableNode = isCallableNode_1;
}
JSFunctionCallNode callNode_1;
JSFunctionCallNode callNode_1_shared = this.callNode;
if (callNode_1_shared != null) {
callNode_1 = callNode_1_shared;
} else {
callNode_1 = this.insert((JSFunctionCallNode.createCall()));
if (callNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.callNode == null) {
VarHandle.storeStoreFence();
this.callNode = callNode_1;
}
ImportValueNode importValueNode_1;
ImportValueNode importValueNode_1_shared = this.importValueNode;
if (importValueNode_1_shared != null) {
importValueNode_1 = importValueNode_1_shared;
} else {
importValueNode_1 = this.insert((ImportValueNode.create()));
if (importValueNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.importValueNode == null) {
VarHandle.storeStoreFence();
this.importValueNode = importValueNode_1;
}
this.cached_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[JSInteropInvokeNode.doCached(JSDynamicObject, TruffleString, Object[], TruffleString, EqualNode, PropertyGetNode, IsCallableNode, JSFunctionCallNode, ImportValueNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[JSInteropInvokeNode.doUncached(JSDynamicObject, TruffleString, Object[], ReadElementNode, IsCallableNode, JSFunctionCallNode, ImportValueNode)] */;
this.state_0_ = state_0;
return doUncached(arg0Value, arg1Value, arg2Value, readNode__, isCallableNode_1, callNode_1, importValueNode_1);
}
@NeverDefault
public static JSInteropInvokeNode create() {
return new JSInteropInvokeNodeGen();
}
@NeverDefault
public static JSInteropInvokeNode getUncached() {
return JSInteropInvokeNodeGen.UNCACHED;
}
@GeneratedBy(JSInteropInvokeNode.class)
@DenyReplace
private static final class CachedData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link JSInteropInvokeNode#doCached}
* Parameter: {@link TruffleString} cachedName
*/
@CompilationFinal TruffleString cachedName_;
/**
* Source Info:
* Specialization: {@link JSInteropInvokeNode#doCached}
* Parameter: {@link EqualNode} equalNode
*/
@Child EqualNode equalNode_;
/**
* Source Info:
* Specialization: {@link JSInteropInvokeNode#doCached}
* Parameter: {@link PropertyGetNode} functionPropertyGetNode
*/
@Child PropertyGetNode functionPropertyGetNode_;
CachedData() {
}
}
@GeneratedBy(JSInteropInvokeNode.class)
@DenyReplace
private static final class Uncached extends JSInteropInvokeNode implements UnadoptableNode {
@TruffleBoundary
@Override
public Object execute(JSDynamicObject arg0Value, TruffleString arg1Value, Object[] arg2Value) throws UnknownIdentifierException, UnsupportedMessageException {
return doUncached(arg0Value, arg1Value, arg2Value, (JSInteropInvokeNode.getUncachedRead()), (IsCallableNodeGen.getUncached()), (JSFunctionCallNode.getUncachedCall()), (ImportValueNode.getUncached()));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy