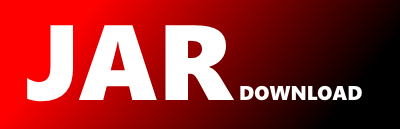
com.oracle.truffle.js.nodes.interop.KeyInfoNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.interop;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.object.DynamicObjectLibrary;
import com.oracle.truffle.api.object.Property;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.FromJavaStringNode;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.access.GetPrototypeNode;
import com.oracle.truffle.js.nodes.access.GetPrototypeNodeGen;
import com.oracle.truffle.js.nodes.access.IsExtensibleNode;
import com.oracle.truffle.js.nodes.access.IsExtensibleNodeGen;
import com.oracle.truffle.js.nodes.unary.IsCallableNode;
import com.oracle.truffle.js.nodes.unary.IsCallableNodeGen;
import com.oracle.truffle.js.runtime.Strings;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link KeyInfoNode#cachedOwnProperty}
* Activation probability: 0.65000
* With/without class size: 22/5 bytes
* Specialization {@link KeyInfoNode#member}
* Activation probability: 0.35000
* With/without class size: 12/8 bytes
*
*/
@GeneratedBy(KeyInfoNode.class)
@SuppressWarnings("javadoc")
public final class KeyInfoNodeGen extends KeyInfoNode {
private static final StateField CACHED_OWN_PROPERTY__KEY_INFO_NODE_CACHED_OWN_PROPERTY_STATE_0_UPDATER = StateField.create(CachedOwnPropertyData.lookup_(), "cachedOwnProperty_state_0_");
static final ReferenceField CACHED_OWN_PROPERTY_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "cachedOwnProperty_cache", CachedOwnPropertyData.class);
/**
* Source Info:
* Specialization: {@link KeyInfoNode#cachedOwnProperty}
* Parameter: {@link InlinedBranchProfile} proxyBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_CACHED_OWN_PROPERTY_PROXY_BRANCH_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, CACHED_OWN_PROPERTY__KEY_INFO_NODE_CACHED_OWN_PROPERTY_STATE_0_UPDATER.subUpdater(0, 1)));
/**
* Source Info:
* Specialization: {@link KeyInfoNode#cachedOwnProperty}
* Parameter: {@link InlinedBranchProfile} moduleNamespaceBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_CACHED_OWN_PROPERTY_MODULE_NAMESPACE_BRANCH_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, CACHED_OWN_PROPERTY__KEY_INFO_NODE_CACHED_OWN_PROPERTY_STATE_0_UPDATER.subUpdater(1, 1)));
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory DYNAMIC_OBJECT_LIBRARY_ = LibraryFactory.resolve(DynamicObjectLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link KeyInfoNode#cachedOwnProperty}
* 1: SpecializationActive {@link KeyInfoNode#member}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link KeyInfoNode#cachedOwnProperty}
* Parameter: {@link FromJavaStringNode} fromJavaStringNode
*/
@Child private FromJavaStringNode fromJavaStringNode;
/**
* Source Info:
* Specialization: {@link KeyInfoNode#cachedOwnProperty}
* Parameter: {@link IsCallableNode} isCallable
*/
@Child private IsCallableNode isCallable;
@UnsafeAccessedField @Child private CachedOwnPropertyData cachedOwnProperty_cache;
/**
* Source Info:
* Specialization: {@link KeyInfoNode#member}
* Parameter: {@link GetPrototypeNode} getPrototype
*/
@Child private GetPrototypeNode member_getPrototype_;
/**
* Source Info:
* Specialization: {@link KeyInfoNode#member}
* Parameter: {@link IsExtensibleNode} isExtensible
*/
@Child private IsExtensibleNode member_isExtensible_;
private KeyInfoNodeGen() {
}
@ExplodeLoop
@Override
public boolean execute(JSDynamicObject arg0Value, String arg1Value, int arg2Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[KeyInfoNode.cachedOwnProperty(JSDynamicObject, String, int, Node, DynamicObjectLibrary, FromJavaStringNode, TruffleString, Property, IsCallableNode, InlinedBranchProfile, InlinedBranchProfile)] || SpecializationActive[KeyInfoNode.member(JSDynamicObject, String, int, GetPrototypeNode, IsCallableNode, IsExtensibleNode, FromJavaStringNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[KeyInfoNode.cachedOwnProperty(JSDynamicObject, String, int, Node, DynamicObjectLibrary, FromJavaStringNode, TruffleString, Property, IsCallableNode, InlinedBranchProfile, InlinedBranchProfile)] */) {
CachedOwnPropertyData s0_ = this.cachedOwnProperty_cache;
while (s0_ != null) {
{
FromJavaStringNode fromJavaStringNode_ = this.fromJavaStringNode;
if (fromJavaStringNode_ != null) {
IsCallableNode isCallable_ = this.isCallable;
if (isCallable_ != null) {
if ((s0_.objectLibrary_.accepts(arg0Value)) && (!(JSGuards.isJSProxy(arg0Value)))) {
TruffleString tStringKey__ = (Strings.fromJavaString(fromJavaStringNode_, arg1Value));
Property property__ = (s0_.objectLibrary_.getProperty(arg0Value, tStringKey__));
if ((property__ != null)) {
Node node__ = (s0_);
return KeyInfoNode.cachedOwnProperty(arg0Value, arg1Value, arg2Value, node__, s0_.objectLibrary_, fromJavaStringNode_, tStringKey__, property__, isCallable_, INLINED_CACHED_OWN_PROPERTY_PROXY_BRANCH_, INLINED_CACHED_OWN_PROPERTY_MODULE_NAMESPACE_BRANCH_);
}
}
}
}
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[KeyInfoNode.member(JSDynamicObject, String, int, GetPrototypeNode, IsCallableNode, IsExtensibleNode, FromJavaStringNode)] */) {
{
GetPrototypeNode getPrototype__ = this.member_getPrototype_;
if (getPrototype__ != null) {
IsCallableNode isCallable_1 = this.isCallable;
if (isCallable_1 != null) {
IsExtensibleNode isExtensible__ = this.member_isExtensible_;
if (isExtensible__ != null) {
FromJavaStringNode fromJavaStringNode_1 = this.fromJavaStringNode;
if (fromJavaStringNode_1 != null) {
return KeyInfoNode.member(arg0Value, arg1Value, arg2Value, getPrototype__, isCallable_1, isExtensible__, fromJavaStringNode_1);
}
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
@SuppressWarnings("unused")
private boolean executeAndSpecialize(JSDynamicObject arg0Value, String arg1Value, int arg2Value) {
int state_0 = this.state_0_;
{
Property property__ = null;
TruffleString tStringKey__ = null;
Node node__ = null;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[KeyInfoNode.member(JSDynamicObject, String, int, GetPrototypeNode, IsCallableNode, IsExtensibleNode, FromJavaStringNode)] */) {
while (true) {
int count0_ = 0;
CachedOwnPropertyData s0_ = CACHED_OWN_PROPERTY_CACHE_UPDATER.getVolatile(this);
CachedOwnPropertyData s0_original = s0_;
while (s0_ != null) {
{
FromJavaStringNode fromJavaStringNode_ = this.fromJavaStringNode;
if (fromJavaStringNode_ != null) {
IsCallableNode isCallable_ = this.isCallable;
if (isCallable_ != null) {
if ((s0_.objectLibrary_.accepts(arg0Value)) && (!(JSGuards.isJSProxy(arg0Value)))) {
tStringKey__ = (Strings.fromJavaString(fromJavaStringNode_, arg1Value));
property__ = (s0_.objectLibrary_.getProperty(arg0Value, tStringKey__));
if ((property__ != null)) {
node__ = (s0_);
break;
}
}
}
}
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
if ((!(JSGuards.isJSProxy(arg0Value)))) {
// assert (s0_.objectLibrary_.accepts(arg0Value));
DynamicObjectLibrary objectLibrary__ = this.insert((DYNAMIC_OBJECT_LIBRARY_.create(arg0Value)));
FromJavaStringNode fromJavaStringNode_;
FromJavaStringNode fromJavaStringNode__shared = this.fromJavaStringNode;
if (fromJavaStringNode__shared != null) {
fromJavaStringNode_ = fromJavaStringNode__shared;
} else {
fromJavaStringNode_ = this.insert((FromJavaStringNode.create()));
if (fromJavaStringNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
tStringKey__ = (Strings.fromJavaString(fromJavaStringNode_, arg1Value));
property__ = (objectLibrary__.getProperty(arg0Value, tStringKey__));
if ((property__ != null) && count0_ < (2)) {
s0_ = this.insert(new CachedOwnPropertyData(s0_original));
node__ = (s0_);
Objects.requireNonNull(s0_.insert(objectLibrary__), "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s0_.objectLibrary_ = objectLibrary__;
if (this.fromJavaStringNode == null) {
this.fromJavaStringNode = fromJavaStringNode_;
}
IsCallableNode isCallable_;
IsCallableNode isCallable__shared = this.isCallable;
if (isCallable__shared != null) {
isCallable_ = isCallable__shared;
} else {
isCallable_ = s0_.insert((IsCallableNode.create()));
if (isCallable_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.isCallable == null) {
this.isCallable = isCallable_;
}
if (!CACHED_OWN_PROPERTY_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[KeyInfoNode.cachedOwnProperty(JSDynamicObject, String, int, Node, DynamicObjectLibrary, FromJavaStringNode, TruffleString, Property, IsCallableNode, InlinedBranchProfile, InlinedBranchProfile)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return KeyInfoNode.cachedOwnProperty(arg0Value, arg1Value, arg2Value, node__, s0_.objectLibrary_, this.fromJavaStringNode, tStringKey__, property__, this.isCallable, INLINED_CACHED_OWN_PROPERTY_PROXY_BRANCH_, INLINED_CACHED_OWN_PROPERTY_MODULE_NAMESPACE_BRANCH_);
}
break;
}
}
}
GetPrototypeNode getPrototype__ = this.insert((GetPrototypeNode.create()));
Objects.requireNonNull(getPrototype__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.member_getPrototype_ = getPrototype__;
IsCallableNode isCallable_1;
IsCallableNode isCallable_1_shared = this.isCallable;
if (isCallable_1_shared != null) {
isCallable_1 = isCallable_1_shared;
} else {
isCallable_1 = this.insert((IsCallableNode.create()));
if (isCallable_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.isCallable == null) {
VarHandle.storeStoreFence();
this.isCallable = isCallable_1;
}
IsExtensibleNode isExtensible__ = this.insert((IsExtensibleNode.create()));
Objects.requireNonNull(isExtensible__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.member_isExtensible_ = isExtensible__;
FromJavaStringNode fromJavaStringNode_1;
FromJavaStringNode fromJavaStringNode_1_shared = this.fromJavaStringNode;
if (fromJavaStringNode_1_shared != null) {
fromJavaStringNode_1 = fromJavaStringNode_1_shared;
} else {
fromJavaStringNode_1 = this.insert((FromJavaStringNode.create()));
if (fromJavaStringNode_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromJavaStringNode == null) {
VarHandle.storeStoreFence();
this.fromJavaStringNode = fromJavaStringNode_1;
}
this.cachedOwnProperty_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[KeyInfoNode.cachedOwnProperty(JSDynamicObject, String, int, Node, DynamicObjectLibrary, FromJavaStringNode, TruffleString, Property, IsCallableNode, InlinedBranchProfile, InlinedBranchProfile)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[KeyInfoNode.member(JSDynamicObject, String, int, GetPrototypeNode, IsCallableNode, IsExtensibleNode, FromJavaStringNode)] */;
this.state_0_ = state_0;
return KeyInfoNode.member(arg0Value, arg1Value, arg2Value, getPrototype__, isCallable_1, isExtensible__, fromJavaStringNode_1);
}
@NeverDefault
public static KeyInfoNode create() {
return new KeyInfoNodeGen();
}
@NeverDefault
public static KeyInfoNode getUncached() {
return KeyInfoNodeGen.UNCACHED;
}
@GeneratedBy(KeyInfoNode.class)
@DenyReplace
private static final class CachedOwnPropertyData extends Node implements SpecializationDataNode {
@Child CachedOwnPropertyData next_;
/**
* State Info:
* 0: InlinedCache
* Specialization: {@link KeyInfoNode#cachedOwnProperty}
* Parameter: {@link InlinedBranchProfile} proxyBranch
* Inline method: {@link InlinedBranchProfile#inline}
* 1: InlinedCache
* Specialization: {@link KeyInfoNode#cachedOwnProperty}
* Parameter: {@link InlinedBranchProfile} moduleNamespaceBranch
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int cachedOwnProperty_state_0_;
/**
* Source Info:
* Specialization: {@link KeyInfoNode#cachedOwnProperty}
* Parameter: {@link DynamicObjectLibrary} objectLibrary
*/
@Child DynamicObjectLibrary objectLibrary_;
CachedOwnPropertyData(CachedOwnPropertyData next_) {
this.next_ = next_;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(KeyInfoNode.class)
@DenyReplace
private static final class Uncached extends KeyInfoNode implements UnadoptableNode {
@TruffleBoundary
@Override
public boolean execute(JSDynamicObject arg0Value, String arg1Value, int arg2Value) {
return KeyInfoNode.member(arg0Value, arg1Value, arg2Value, (GetPrototypeNodeGen.getUncached()), (IsCallableNodeGen.getUncached()), (IsExtensibleNodeGen.getUncached()), (FromJavaStringNode.getUncached()));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy