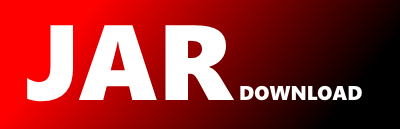
com.oracle.truffle.js.nodes.intl.ToIntlMathematicalValueNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.intl;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.JSTypesGen;
import com.oracle.truffle.js.nodes.cast.JSToPrimitiveNode;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.Symbol;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link ToIntlMathematicalValue#doDouble}
* Activation probability: 0.19111
* With/without class size: 6/0 bytes
* Specialization {@link ToIntlMathematicalValue#doBigInt}
* Activation probability: 0.17111
* With/without class size: 6/0 bytes
* Specialization {@link ToIntlMathematicalValue#doLong}
* Activation probability: 0.15111
* With/without class size: 5/0 bytes
* Specialization {@link ToIntlMathematicalValue#doString}
* Activation probability: 0.13111
* With/without class size: 5/0 bytes
* Specialization {@link ToIntlMathematicalValue#doBoolean}
* Activation probability: 0.11111
* With/without class size: 5/0 bytes
* Specialization {@link ToIntlMathematicalValue#doUndefined}
* Activation probability: 0.09111
* With/without class size: 5/0 bytes
* Specialization {@link ToIntlMathematicalValue#doNull}
* Activation probability: 0.07111
* With/without class size: 4/0 bytes
* Specialization {@link ToIntlMathematicalValue#doSymbol}
* Activation probability: 0.05111
* With/without class size: 4/0 bytes
* Specialization {@link ToIntlMathematicalValue#doGeneric}
* Activation probability: 0.03111
* With/without class size: 4/8 bytes
*
*/
@GeneratedBy(ToIntlMathematicalValue.class)
@SuppressWarnings("javadoc")
public final class ToIntlMathematicalValueNodeGen extends ToIntlMathematicalValue {
/**
* State Info:
* 0: SpecializationActive {@link ToIntlMathematicalValue#doDouble}
* 1: SpecializationActive {@link ToIntlMathematicalValue#doGeneric}
* 2: SpecializationActive {@link ToIntlMathematicalValue#doBigInt}
* 3: SpecializationActive {@link ToIntlMathematicalValue#doLong}
* 4: SpecializationActive {@link ToIntlMathematicalValue#doString}
* 5: SpecializationActive {@link ToIntlMathematicalValue#doBoolean}
* 6: SpecializationActive {@link ToIntlMathematicalValue#doUndefined}
* 7: SpecializationActive {@link ToIntlMathematicalValue#doNull}
* 8: SpecializationActive {@link ToIntlMathematicalValue#doSymbol}
* 9-11: ImplicitCast[type=double, index=0]
*
*/
@CompilationFinal private int state_0_;
@Child private GenericData generic_cache;
private ToIntlMathematicalValueNodeGen(boolean partOfRange) {
super(partOfRange);
}
@Override
public Number executeNumber(Object arg0Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b111111111) != 0 /* is SpecializationActive[ToIntlMathematicalValue.doDouble(double)] || SpecializationActive[ToIntlMathematicalValue.doBigInt(BigInt)] || SpecializationActive[ToIntlMathematicalValue.doLong(long)] || SpecializationActive[ToIntlMathematicalValue.doString(TruffleString)] || SpecializationActive[ToIntlMathematicalValue.doBoolean(boolean)] || SpecializationActive[ToIntlMathematicalValue.doUndefined(Object)] || SpecializationActive[ToIntlMathematicalValue.doNull(Object)] || SpecializationActive[ToIntlMathematicalValue.doSymbol(Symbol)] || SpecializationActive[ToIntlMathematicalValue.doGeneric(Object, JSToPrimitiveNode, ToIntlMathematicalValue)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ToIntlMathematicalValue.doDouble(double)] */ && JSTypesGen.isImplicitDouble((state_0 & 0b111000000000) >>> 9 /* get-int ImplicitCast[type=double, index=0] */, arg0Value)) {
double arg0Value_ = JSTypesGen.asImplicitDouble((state_0 & 0b111000000000) >>> 9 /* get-int ImplicitCast[type=double, index=0] */, arg0Value);
return doDouble(arg0Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ToIntlMathematicalValue.doBigInt(BigInt)] */ && arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
return doBigInt(arg0Value_);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[ToIntlMathematicalValue.doLong(long)] */ && arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
return doLong(arg0Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[ToIntlMathematicalValue.doString(TruffleString)] */ && arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
return doString(arg0Value_);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[ToIntlMathematicalValue.doBoolean(boolean)] */ && arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
return doBoolean(arg0Value_);
}
if ((state_0 & 0b11000000) != 0 /* is SpecializationActive[ToIntlMathematicalValue.doUndefined(Object)] || SpecializationActive[ToIntlMathematicalValue.doNull(Object)] */) {
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[ToIntlMathematicalValue.doUndefined(Object)] */) {
if ((JSGuards.isUndefined(arg0Value))) {
return doUndefined(arg0Value);
}
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[ToIntlMathematicalValue.doNull(Object)] */) {
if ((JSGuards.isJSNull(arg0Value))) {
return doNull(arg0Value);
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[ToIntlMathematicalValue.doSymbol(Symbol)] */ && arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
return doSymbol(arg0Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ToIntlMathematicalValue.doGeneric(Object, JSToPrimitiveNode, ToIntlMathematicalValue)] */) {
GenericData s8_ = this.generic_cache;
if (s8_ != null) {
return doGeneric(arg0Value, s8_.toPrimitiveNode_, s8_.nestedToIntlMVNode_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
private Number executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[ToIntlMathematicalValue.doGeneric(Object, JSToPrimitiveNode, ToIntlMathematicalValue)] */) {
int doubleCast0;
if ((doubleCast0 = JSTypesGen.specializeImplicitDouble(arg0Value)) != 0) {
double arg0Value_ = JSTypesGen.asImplicitDouble(doubleCast0, arg0Value);
state_0 = (state_0 | (doubleCast0 << 9) /* set-int ImplicitCast[type=double, index=0] */);
state_0 = state_0 | 0b1 /* add SpecializationActive[ToIntlMathematicalValue.doDouble(double)] */;
this.state_0_ = state_0;
return doDouble(arg0Value_);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[ToIntlMathematicalValue.doGeneric(Object, JSToPrimitiveNode, ToIntlMathematicalValue)] */ && arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[ToIntlMathematicalValue.doBigInt(BigInt)] */;
this.state_0_ = state_0;
return doBigInt(arg0Value_);
}
if (arg0Value instanceof Long) {
long arg0Value_ = (long) arg0Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[ToIntlMathematicalValue.doLong(long)] */;
this.state_0_ = state_0;
return doLong(arg0Value_);
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[ToIntlMathematicalValue.doGeneric(Object, JSToPrimitiveNode, ToIntlMathematicalValue)] */ && arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
state_0 = state_0 | 0b10000 /* add SpecializationActive[ToIntlMathematicalValue.doString(TruffleString)] */;
this.state_0_ = state_0;
return doString(arg0Value_);
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[ToIntlMathematicalValue.doGeneric(Object, JSToPrimitiveNode, ToIntlMathematicalValue)] */ && arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[ToIntlMathematicalValue.doBoolean(boolean)] */;
this.state_0_ = state_0;
return doBoolean(arg0Value_);
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[ToIntlMathematicalValue.doGeneric(Object, JSToPrimitiveNode, ToIntlMathematicalValue)] */) {
if ((JSGuards.isUndefined(arg0Value))) {
state_0 = state_0 | 0b1000000 /* add SpecializationActive[ToIntlMathematicalValue.doUndefined(Object)] */;
this.state_0_ = state_0;
return doUndefined(arg0Value);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[ToIntlMathematicalValue.doGeneric(Object, JSToPrimitiveNode, ToIntlMathematicalValue)] */) {
if ((JSGuards.isJSNull(arg0Value))) {
state_0 = state_0 | 0b10000000 /* add SpecializationActive[ToIntlMathematicalValue.doNull(Object)] */;
this.state_0_ = state_0;
return doNull(arg0Value);
}
}
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[ToIntlMathematicalValue.doGeneric(Object, JSToPrimitiveNode, ToIntlMathematicalValue)] */ && arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[ToIntlMathematicalValue.doSymbol(Symbol)] */;
this.state_0_ = state_0;
return doSymbol(arg0Value_);
}
GenericData s8_ = this.insert(new GenericData());
JSToPrimitiveNode toPrimitiveNode__ = s8_.insert((JSToPrimitiveNode.createHintNumber()));
Objects.requireNonNull(toPrimitiveNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s8_.toPrimitiveNode_ = toPrimitiveNode__;
ToIntlMathematicalValue nestedToIntlMVNode__ = s8_.insert((ToIntlMathematicalValue.create(partOfRange)));
Objects.requireNonNull(nestedToIntlMVNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s8_.nestedToIntlMVNode_ = nestedToIntlMVNode__;
VarHandle.storeStoreFence();
this.generic_cache = s8_;
state_0 = state_0 & 0xfffffe0a /* remove SpecializationActive[ToIntlMathematicalValue.doDouble(double)], SpecializationActive[ToIntlMathematicalValue.doBigInt(BigInt)], SpecializationActive[ToIntlMathematicalValue.doString(TruffleString)], SpecializationActive[ToIntlMathematicalValue.doBoolean(boolean)], SpecializationActive[ToIntlMathematicalValue.doUndefined(Object)], SpecializationActive[ToIntlMathematicalValue.doNull(Object)], SpecializationActive[ToIntlMathematicalValue.doSymbol(Symbol)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[ToIntlMathematicalValue.doGeneric(Object, JSToPrimitiveNode, ToIntlMathematicalValue)] */;
this.state_0_ = state_0;
return doGeneric(arg0Value, toPrimitiveNode__, nestedToIntlMVNode__);
}
@NeverDefault
public static ToIntlMathematicalValue create(boolean partOfRange) {
return new ToIntlMathematicalValueNodeGen(partOfRange);
}
@GeneratedBy(ToIntlMathematicalValue.class)
@DenyReplace
private static final class GenericData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link ToIntlMathematicalValue#doGeneric}
* Parameter: {@link JSToPrimitiveNode} toPrimitiveNode
*/
@Child JSToPrimitiveNode toPrimitiveNode_;
/**
* Source Info:
* Specialization: {@link ToIntlMathematicalValue#doGeneric}
* Parameter: {@link ToIntlMathematicalValue} nestedToIntlMVNode
*/
@Child ToIntlMathematicalValue nestedToIntlMVNode_;
GenericData() {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy