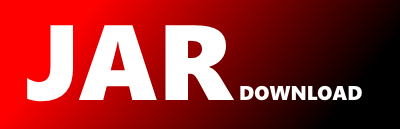
com.oracle.truffle.js.nodes.unary.IsCallableNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.nodes.unary;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.object.Shape;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.runtime.BigInt;
import com.oracle.truffle.js.runtime.JSConfig;
import com.oracle.truffle.js.runtime.Symbol;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import java.lang.invoke.MethodHandles;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link IsCallableNode#doJSFunctionShape}
* Activation probability: 0.15909
* With/without class size: 6/4 bytes
* Specialization {@link IsCallableNode#doJSFunction}
* Activation probability: 0.14545
* With/without class size: 5/0 bytes
* Specialization {@link IsCallableNode#doJSProxy}
* Activation probability: 0.13182
* With/without class size: 5/0 bytes
* Specialization {@link IsCallableNode#doJSTypeOther}
* Activation probability: 0.11818
* With/without class size: 5/0 bytes
* Specialization {@link IsCallableNode#doTruffleObject}
* Activation probability: 0.10455
* With/without class size: 6/4 bytes
* Specialization {@link IsCallableNode#doTruffleObject}
* Activation probability: 0.09091
* With/without class size: 5/0 bytes
* Specialization {@link IsCallableNode#doString}
* Activation probability: 0.07727
* With/without class size: 4/0 bytes
* Specialization {@link IsCallableNode#doNumber}
* Activation probability: 0.06364
* With/without class size: 4/0 bytes
* Specialization {@link IsCallableNode#doBoolean}
* Activation probability: 0.05000
* With/without class size: 4/0 bytes
* Specialization {@link IsCallableNode#doSymbol}
* Activation probability: 0.03636
* With/without class size: 4/0 bytes
* Specialization {@link IsCallableNode#doBigInt}
* Activation probability: 0.02273
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(IsCallableNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class IsCallableNodeGen extends IsCallableNode {
static final ReferenceField J_S_FUNCTION_SHAPE_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "jSFunctionShape_cache", JSFunctionShapeData.class);
static final ReferenceField TRUFFLE_OBJECT0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "truffleObject0_cache", TruffleObject0Data.class);
private static final Uncached UNCACHED = new Uncached();
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* State Info:
* 0: SpecializationActive {@link IsCallableNode#doJSFunctionShape}
* 1: SpecializationActive {@link IsCallableNode#doJSFunction}
* 2: SpecializationActive {@link IsCallableNode#doJSProxy}
* 3: SpecializationActive {@link IsCallableNode#doJSTypeOther}
* 4: SpecializationActive {@link IsCallableNode#doTruffleObject}
* 5: SpecializationActive {@link IsCallableNode#doTruffleObject}
* 6: SpecializationActive {@link IsCallableNode#doString}
* 7: SpecializationActive {@link IsCallableNode#doNumber}
* 8: SpecializationActive {@link IsCallableNode#doBoolean}
* 9: SpecializationActive {@link IsCallableNode#doSymbol}
* 10: SpecializationActive {@link IsCallableNode#doBigInt}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @CompilationFinal private JSFunctionShapeData jSFunctionShape_cache;
@UnsafeAccessedField @Child private TruffleObject0Data truffleObject0_cache;
private IsCallableNodeGen() {
}
@ExplodeLoop
@Override
public boolean executeBoolean(Object arg0Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[IsCallableNode.doJSFunctionShape(JSDynamicObject, Shape)] || SpecializationActive[IsCallableNode.doJSFunction(JSDynamicObject)] || SpecializationActive[IsCallableNode.doJSProxy(JSDynamicObject)] || SpecializationActive[IsCallableNode.doJSTypeOther(JSDynamicObject)] || SpecializationActive[IsCallableNode.doTruffleObject(Object, InteropLibrary)] || SpecializationActive[IsCallableNode.doTruffleObject(Object, InteropLibrary)] || SpecializationActive[IsCallableNode.doString(TruffleString)] || SpecializationActive[IsCallableNode.doNumber(Number)] || SpecializationActive[IsCallableNode.doBoolean(boolean)] || SpecializationActive[IsCallableNode.doSymbol(Symbol)] || SpecializationActive[IsCallableNode.doBigInt(BigInt)] */) {
if ((state_0 & 0b1111) != 0 /* is SpecializationActive[IsCallableNode.doJSFunctionShape(JSDynamicObject, Shape)] || SpecializationActive[IsCallableNode.doJSFunction(JSDynamicObject)] || SpecializationActive[IsCallableNode.doJSProxy(JSDynamicObject)] || SpecializationActive[IsCallableNode.doJSTypeOther(JSDynamicObject)] */ && arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[IsCallableNode.doJSFunctionShape(JSDynamicObject, Shape)] */) {
JSFunctionShapeData s0_ = this.jSFunctionShape_cache;
if (s0_ != null) {
if ((s0_.shape_.check(arg0Value_))) {
assert DSLSupport.assertIdempotence((JSGuards.isJSFunctionShape(s0_.shape_)));
return IsCallableNode.doJSFunctionShape(arg0Value_, s0_.shape_);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[IsCallableNode.doJSFunction(JSDynamicObject)] */) {
if ((JSGuards.isJSFunction(arg0Value_))) {
return IsCallableNode.doJSFunction(arg0Value_);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[IsCallableNode.doJSProxy(JSDynamicObject)] */) {
if ((JSGuards.isJSProxy(arg0Value_))) {
return IsCallableNode.doJSProxy(arg0Value_);
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[IsCallableNode.doJSTypeOther(JSDynamicObject)] */) {
if ((JSGuards.isJSDynamicObject(arg0Value_)) && (!(JSGuards.isJSFunction(arg0Value_))) && (!(JSGuards.isJSProxy(arg0Value_)))) {
return IsCallableNode.doJSTypeOther(arg0Value_);
}
}
}
if ((state_0 & 0b110000) != 0 /* is SpecializationActive[IsCallableNode.doTruffleObject(Object, InteropLibrary)] || SpecializationActive[IsCallableNode.doTruffleObject(Object, InteropLibrary)] */) {
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[IsCallableNode.doTruffleObject(Object, InteropLibrary)] */) {
TruffleObject0Data s4_ = this.truffleObject0_cache;
while (s4_ != null) {
if ((s4_.interop_.accepts(arg0Value)) && (JSGuards.isForeignObject(arg0Value))) {
return IsCallableNode.doTruffleObject(arg0Value, s4_.interop_);
}
s4_ = s4_.next_;
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[IsCallableNode.doTruffleObject(Object, InteropLibrary)] */) {
if ((JSGuards.isForeignObject(arg0Value))) {
return this.truffleObject1Boundary(state_0, arg0Value);
}
}
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[IsCallableNode.doString(TruffleString)] */ && arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
return IsCallableNode.doString(arg0Value_);
}
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[IsCallableNode.doNumber(Number)] */ && arg0Value instanceof Number) {
Number arg0Value_ = (Number) arg0Value;
return IsCallableNode.doNumber(arg0Value_);
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[IsCallableNode.doBoolean(boolean)] */ && arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
return IsCallableNode.doBoolean(arg0Value_);
}
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[IsCallableNode.doSymbol(Symbol)] */ && arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
return IsCallableNode.doSymbol(arg0Value_);
}
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[IsCallableNode.doBigInt(BigInt)] */ && arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
return IsCallableNode.doBigInt(arg0Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private boolean truffleObject1Boundary(int state_0, Object arg0Value) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(arg0Value));
return IsCallableNode.doTruffleObject(arg0Value, interop__);
}
} finally {
encapsulating_.set(prev_);
}
}
@SuppressWarnings("unused")
private boolean executeAndSpecialize(Object arg0Value) {
int state_0 = this.state_0_;
if (arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[IsCallableNode.doJSFunction(JSDynamicObject)] */) {
while (true) {
int count0_ = 0;
JSFunctionShapeData s0_ = J_S_FUNCTION_SHAPE_CACHE_UPDATER.getVolatile(this);
JSFunctionShapeData s0_original = s0_;
while (s0_ != null) {
if ((s0_.shape_.check(arg0Value_))) {
assert DSLSupport.assertIdempotence((JSGuards.isJSFunctionShape(s0_.shape_)));
break;
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
Shape shape__ = (arg0Value_.getShape());
if ((shape__.check(arg0Value_)) && (JSGuards.isJSFunctionShape(shape__))) {
s0_ = new JSFunctionShapeData();
Objects.requireNonNull(shape__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s0_.shape_ = shape__;
if (!J_S_FUNCTION_SHAPE_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[IsCallableNode.doJSFunctionShape(JSDynamicObject, Shape)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return IsCallableNode.doJSFunctionShape(arg0Value_, s0_.shape_);
}
break;
}
}
if ((JSGuards.isJSFunction(arg0Value_))) {
this.jSFunctionShape_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[IsCallableNode.doJSFunctionShape(JSDynamicObject, Shape)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[IsCallableNode.doJSFunction(JSDynamicObject)] */;
this.state_0_ = state_0;
return IsCallableNode.doJSFunction(arg0Value_);
}
if ((JSGuards.isJSProxy(arg0Value_))) {
state_0 = state_0 | 0b100 /* add SpecializationActive[IsCallableNode.doJSProxy(JSDynamicObject)] */;
this.state_0_ = state_0;
return IsCallableNode.doJSProxy(arg0Value_);
}
if ((JSGuards.isJSDynamicObject(arg0Value_)) && (!(JSGuards.isJSFunction(arg0Value_))) && (!(JSGuards.isJSProxy(arg0Value_)))) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[IsCallableNode.doJSTypeOther(JSDynamicObject)] */;
this.state_0_ = state_0;
return IsCallableNode.doJSTypeOther(arg0Value_);
}
}
if (((state_0 & 0b100000)) == 0 /* is-not SpecializationActive[IsCallableNode.doTruffleObject(Object, InteropLibrary)] */) {
while (true) {
int count4_ = 0;
TruffleObject0Data s4_ = TRUFFLE_OBJECT0_CACHE_UPDATER.getVolatile(this);
TruffleObject0Data s4_original = s4_;
while (s4_ != null) {
if ((s4_.interop_.accepts(arg0Value)) && (JSGuards.isForeignObject(arg0Value))) {
break;
}
count4_++;
s4_ = s4_.next_;
}
if (s4_ == null) {
if ((JSGuards.isForeignObject(arg0Value)) && count4_ < (JSConfig.InteropLibraryLimit)) {
// assert (s4_.interop_.accepts(arg0Value));
s4_ = this.insert(new TruffleObject0Data(s4_original));
InteropLibrary interop__ = s4_.insert((INTEROP_LIBRARY_.create(arg0Value)));
Objects.requireNonNull(interop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s4_.interop_ = interop__;
if (!TRUFFLE_OBJECT0_CACHE_UPDATER.compareAndSet(this, s4_original, s4_)) {
continue;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[IsCallableNode.doTruffleObject(Object, InteropLibrary)] */;
this.state_0_ = state_0;
}
}
if (s4_ != null) {
return IsCallableNode.doTruffleObject(arg0Value, s4_.interop_);
}
break;
}
}
{
InteropLibrary interop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
if ((JSGuards.isForeignObject(arg0Value))) {
interop__ = (INTEROP_LIBRARY_.getUncached(arg0Value));
this.truffleObject0_cache = null;
state_0 = state_0 & 0xffffffef /* remove SpecializationActive[IsCallableNode.doTruffleObject(Object, InteropLibrary)] */;
state_0 = state_0 | 0b100000 /* add SpecializationActive[IsCallableNode.doTruffleObject(Object, InteropLibrary)] */;
this.state_0_ = state_0;
return IsCallableNode.doTruffleObject(arg0Value, interop__);
}
} finally {
encapsulating_.set(prev_);
}
}
}
if (arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[IsCallableNode.doString(TruffleString)] */;
this.state_0_ = state_0;
return IsCallableNode.doString(arg0Value_);
}
if (arg0Value instanceof Number) {
Number arg0Value_ = (Number) arg0Value;
state_0 = state_0 | 0b10000000 /* add SpecializationActive[IsCallableNode.doNumber(Number)] */;
this.state_0_ = state_0;
return IsCallableNode.doNumber(arg0Value_);
}
if (arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[IsCallableNode.doBoolean(boolean)] */;
this.state_0_ = state_0;
return IsCallableNode.doBoolean(arg0Value_);
}
if (arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[IsCallableNode.doSymbol(Symbol)] */;
this.state_0_ = state_0;
return IsCallableNode.doSymbol(arg0Value_);
}
if (arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[IsCallableNode.doBigInt(BigInt)] */;
this.state_0_ = state_0;
return IsCallableNode.doBigInt(arg0Value_);
}
throw new UnsupportedSpecializationException(this, null, arg0Value);
}
@TruffleBoundary
private static UnsupportedSpecializationException newUnsupportedSpecializationException1(Node thisNode_, Object arg0Value) {
return new UnsupportedSpecializationException(thisNode_, null, arg0Value);
}
@NeverDefault
public static IsCallableNode create() {
return new IsCallableNodeGen();
}
@NeverDefault
public static IsCallableNode getUncached() {
return IsCallableNodeGen.UNCACHED;
}
@GeneratedBy(IsCallableNode.class)
@DenyReplace
private static final class JSFunctionShapeData implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link IsCallableNode#doJSFunctionShape}
* Parameter: {@link Shape} shape
*/
@CompilationFinal Shape shape_;
JSFunctionShapeData() {
}
}
@GeneratedBy(IsCallableNode.class)
@DenyReplace
private static final class TruffleObject0Data extends Node implements SpecializationDataNode {
@Child TruffleObject0Data next_;
/**
* Source Info:
* Specialization: {@link IsCallableNode#doTruffleObject}
* Parameter: {@link InteropLibrary} interop
*/
@Child InteropLibrary interop_;
TruffleObject0Data(TruffleObject0Data next_) {
this.next_ = next_;
}
}
@GeneratedBy(IsCallableNode.class)
@DenyReplace
private static final class Uncached extends IsCallableNode implements UnadoptableNode {
@TruffleBoundary
@Override
public boolean executeBoolean(Object arg0Value) {
if (arg0Value instanceof JSDynamicObject) {
JSDynamicObject arg0Value_ = (JSDynamicObject) arg0Value;
if ((JSGuards.isJSFunction(arg0Value_))) {
return IsCallableNode.doJSFunction(arg0Value_);
}
if ((JSGuards.isJSProxy(arg0Value_))) {
return IsCallableNode.doJSProxy(arg0Value_);
}
if ((JSGuards.isJSDynamicObject(arg0Value_)) && (!(JSGuards.isJSFunction(arg0Value_))) && (!(JSGuards.isJSProxy(arg0Value_)))) {
return IsCallableNode.doJSTypeOther(arg0Value_);
}
}
if ((JSGuards.isForeignObject(arg0Value))) {
return IsCallableNode.doTruffleObject(arg0Value, (INTEROP_LIBRARY_.getUncached(arg0Value)));
}
if (arg0Value instanceof TruffleString) {
TruffleString arg0Value_ = (TruffleString) arg0Value;
return IsCallableNode.doString(arg0Value_);
}
if (arg0Value instanceof Number) {
Number arg0Value_ = (Number) arg0Value;
return IsCallableNode.doNumber(arg0Value_);
}
if (arg0Value instanceof Boolean) {
boolean arg0Value_ = (boolean) arg0Value;
return IsCallableNode.doBoolean(arg0Value_);
}
if (arg0Value instanceof Symbol) {
Symbol arg0Value_ = (Symbol) arg0Value;
return IsCallableNode.doSymbol(arg0Value_);
}
if (arg0Value instanceof BigInt) {
BigInt arg0Value_ = (BigInt) arg0Value;
return IsCallableNode.doBigInt(arg0Value_);
}
throw newUnsupportedSpecializationException1(this, arg0Value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy