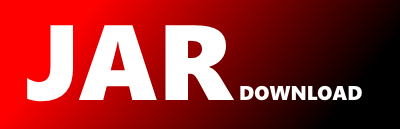
com.oracle.truffle.js.runtime.UserScriptExceptionGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.runtime;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.TruffleLanguage;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.interop.ExceptionType;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.Library;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.source.SourceSection;
import com.oracle.truffle.api.utilities.FinalBitSet;
import com.oracle.truffle.api.utilities.TriState;
import com.oracle.truffle.js.runtime.GraalJSException.IsIdenticalOrUndefined;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import java.lang.invoke.VarHandle;
@GeneratedBy(UserScriptException.class)
@SuppressWarnings("javadoc")
final class UserScriptExceptionGen {
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(UserScriptException.class, new InteropLibraryExports());
}
private UserScriptExceptionGen() {
}
@GeneratedBy(UserScriptException.class)
private static final class InteropLibraryExports extends LibraryExport {
static final FinalBitSet ENABLED_MESSAGES = createMessageBitSet(INTEROP_LIBRARY_, "isIdenticalOrUndefined", "hasSourceLocation", "getSourceLocation", "hasLanguage", "getLanguage", "toDisplayString", "identityHashCode", "isException", "throwException", "getExceptionType", "isExceptionIncompleteSource", "hasExceptionMessage", "getExceptionMessage");
private InteropLibraryExports() {
super(InteropLibrary.class, UserScriptException.class, false, false, 0);
}
@Override
protected InteropLibrary createUncached(Object receiver) {
assert receiver instanceof UserScriptException;
InteropLibrary uncached = createDelegate(INTEROP_LIBRARY_, new Uncached());
return uncached;
}
@Override
protected InteropLibrary createCached(Object receiver) {
assert receiver instanceof UserScriptException;
return createDelegate(INTEROP_LIBRARY_, new Cached(receiver));
}
@GeneratedBy(UserScriptException.class)
private static final class Cached extends InteropLibrary implements DelegateExport {
@Child private InteropLibrary receiverExceptionObjectInteropLibrary_;
/**
* State Info:
* 0: SpecializationActive {@link IsIdenticalOrUndefined#doException}
* 1: SpecializationActive {@link IsIdenticalOrUndefined#doJSObject}
* 2: SpecializationActive {@link IsIdenticalOrUndefined#doOther}
* 3: SpecializationActive {@link GraalJSException#identityHashCode(GraalJSException, InteropLibrary)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link IsIdenticalOrUndefined#doException}
* Parameter: {@link InteropLibrary} thisLib
*/
@Child private InteropLibrary thisLib;
/**
* Source Info:
* Specialization: {@link IsIdenticalOrUndefined#doException}
* Parameter: {@link InteropLibrary} otherLib
*/
@Child private InteropLibrary otherLib;
protected Cached(Object receiver) {
UserScriptException castReceiver = ((UserScriptException) receiver) ;
this.receiverExceptionObjectInteropLibrary_ = INTEROP_LIBRARY_.create((castReceiver.exceptionObject));
}
@Override
public FinalBitSet getDelegateExportMessages() {
return ENABLED_MESSAGES;
}
@Override
public Object readDelegateExport(Object receiver_) {
return ((UserScriptException) receiver_).exceptionObject;
}
@Override
public Library getDelegateExportLibrary(Object delegate) {
return this.receiverExceptionObjectInteropLibrary_;
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof UserScriptException) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
if (!(receiver instanceof UserScriptException)) {
return false;
} else if (!this.receiverExceptionObjectInteropLibrary_.accepts((((UserScriptException) receiver).exceptionObject))) {
return false;
} else {
return true;
}
}
/**
* Debug Info:
* Specialization {@link IsIdenticalOrUndefined#doException}
* Activation probability: 0.16111
* With/without class size: 5/0 bytes
* Specialization {@link IsIdenticalOrUndefined#doJSObject}
* Activation probability: 0.11111
* With/without class size: 5/0 bytes
* Specialization {@link IsIdenticalOrUndefined#doOther}
* Activation probability: 0.06111
* With/without class size: 4/0 bytes
*
*/
@Override
protected TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
assert arg0Value_ instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
UserScriptException arg0Value = ((UserScriptException) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b111) != 0 /* is SpecializationActive[GraalJSException.IsIdenticalOrUndefined.doException(GraalJSException, GraalJSException, InteropLibrary, InteropLibrary)] || SpecializationActive[GraalJSException.IsIdenticalOrUndefined.doJSObject(GraalJSException, JSDynamicObject)] || SpecializationActive[GraalJSException.IsIdenticalOrUndefined.doOther(GraalJSException, Object, InteropLibrary, InteropLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[GraalJSException.IsIdenticalOrUndefined.doException(GraalJSException, GraalJSException, InteropLibrary, InteropLibrary)] */ && arg1Value instanceof GraalJSException) {
GraalJSException arg1Value_ = (GraalJSException) arg1Value;
{
InteropLibrary thisLib_ = this.thisLib;
if (thisLib_ != null) {
InteropLibrary otherLib_ = this.otherLib;
if (otherLib_ != null) {
return IsIdenticalOrUndefined.doException(arg0Value, arg1Value_, thisLib_, otherLib_);
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[GraalJSException.IsIdenticalOrUndefined.doJSObject(GraalJSException, JSDynamicObject)] */ && arg1Value instanceof JSDynamicObject) {
JSDynamicObject arg1Value_ = (JSDynamicObject) arg1Value;
return IsIdenticalOrUndefined.doJSObject(arg0Value, arg1Value_);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[GraalJSException.IsIdenticalOrUndefined.doOther(GraalJSException, Object, InteropLibrary, InteropLibrary)] */) {
{
InteropLibrary thisLib_1 = this.thisLib;
if (thisLib_1 != null) {
InteropLibrary otherLib_1 = this.otherLib;
if (otherLib_1 != null) {
if ((!(IsIdenticalOrUndefined.isGraalJSException(arg1Value)))) {
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value, thisLib_1, otherLib_1);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isIdenticalOrUndefinedAndSpecialize(arg0Value, arg1Value);
}
private TriState isIdenticalOrUndefinedAndSpecialize(GraalJSException arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof GraalJSException) {
GraalJSException arg1Value_ = (GraalJSException) arg1Value;
InteropLibrary thisLib_;
InteropLibrary thisLib__shared = this.thisLib;
if (thisLib__shared != null) {
thisLib_ = thisLib__shared;
} else {
thisLib_ = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
if (thisLib_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.thisLib == null) {
VarHandle.storeStoreFence();
this.thisLib = thisLib_;
}
InteropLibrary otherLib_;
InteropLibrary otherLib__shared = this.otherLib;
if (otherLib__shared != null) {
otherLib_ = otherLib__shared;
} else {
otherLib_ = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
if (otherLib_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.otherLib == null) {
VarHandle.storeStoreFence();
this.otherLib = otherLib_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[GraalJSException.IsIdenticalOrUndefined.doException(GraalJSException, GraalJSException, InteropLibrary, InteropLibrary)] */;
this.state_0_ = state_0;
return IsIdenticalOrUndefined.doException(arg0Value, arg1Value_, thisLib_, otherLib_);
}
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[GraalJSException.IsIdenticalOrUndefined.doOther(GraalJSException, Object, InteropLibrary, InteropLibrary)] */ && arg1Value instanceof JSDynamicObject) {
JSDynamicObject arg1Value_ = (JSDynamicObject) arg1Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[GraalJSException.IsIdenticalOrUndefined.doJSObject(GraalJSException, JSDynamicObject)] */;
this.state_0_ = state_0;
return IsIdenticalOrUndefined.doJSObject(arg0Value, arg1Value_);
}
if ((!(IsIdenticalOrUndefined.isGraalJSException(arg1Value)))) {
InteropLibrary thisLib_1;
InteropLibrary thisLib_1_shared = this.thisLib;
if (thisLib_1_shared != null) {
thisLib_1 = thisLib_1_shared;
} else {
thisLib_1 = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
if (thisLib_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.thisLib == null) {
VarHandle.storeStoreFence();
this.thisLib = thisLib_1;
}
InteropLibrary otherLib_1;
InteropLibrary otherLib_1_shared = this.otherLib;
if (otherLib_1_shared != null) {
otherLib_1 = otherLib_1_shared;
} else {
otherLib_1 = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
if (otherLib_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.otherLib == null) {
VarHandle.storeStoreFence();
this.otherLib = otherLib_1;
}
state_0 = state_0 & 0xfffffffd /* remove SpecializationActive[GraalJSException.IsIdenticalOrUndefined.doJSObject(GraalJSException, JSDynamicObject)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[GraalJSException.IsIdenticalOrUndefined.doOther(GraalJSException, Object, InteropLibrary, InteropLibrary)] */;
this.state_0_ = state_0;
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value, thisLib_1, otherLib_1);
}
throw new UnsupportedSpecializationException(this, null, arg0Value, arg1Value);
}
@Override
public boolean hasSourceLocation(Object receiver) {
assert receiver instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((UserScriptException) receiver)).hasSourceLocation();
}
@Override
public SourceSection getSourceLocation(Object receiver) throws UnsupportedMessageException {
assert receiver instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((UserScriptException) receiver)).getSourceLocationInterop();
}
@Override
public boolean hasLanguage(Object receiver) {
assert receiver instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((UserScriptException) receiver)).hasLanguage();
}
@Override
public Class extends TruffleLanguage>> getLanguage(Object receiver) throws UnsupportedMessageException {
assert receiver instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((UserScriptException) receiver)).getLanguage();
}
@Override
public Object toDisplayString(Object receiver, boolean allowSideEffects) {
assert receiver instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((UserScriptException) receiver)).toDisplayString(allowSideEffects);
}
/**
* Debug Info:
* Specialization {@link GraalJSException#identityHashCode(GraalJSException, InteropLibrary)}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
*
*/
@Override
public int identityHashCode(Object arg0Value_) throws UnsupportedMessageException {
assert arg0Value_ instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
UserScriptException arg0Value = ((UserScriptException) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[GraalJSException.identityHashCode(GraalJSException, InteropLibrary)] */) {
{
InteropLibrary thisLib_ = this.thisLib;
if (thisLib_ != null) {
return arg0Value.identityHashCode(thisLib_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return identityHashCodeNode_AndSpecialize(arg0Value);
}
private int identityHashCodeNode_AndSpecialize(GraalJSException arg0Value) throws UnsupportedMessageException {
int state_0 = this.state_0_;
InteropLibrary thisLib_;
InteropLibrary thisLib__shared = this.thisLib;
if (thisLib__shared != null) {
thisLib_ = thisLib__shared;
} else {
thisLib_ = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
if (thisLib_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.thisLib == null) {
VarHandle.storeStoreFence();
this.thisLib = thisLib_;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[GraalJSException.identityHashCode(GraalJSException, InteropLibrary)] */;
this.state_0_ = state_0;
return arg0Value.identityHashCode(thisLib_);
}
@Override
public boolean isException(Object receiver) {
assert receiver instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((UserScriptException) receiver)).isException();
}
@Override
public RuntimeException throwException(Object receiver) throws UnsupportedMessageException {
assert receiver instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((UserScriptException) receiver)).throwException();
}
@Override
public ExceptionType getExceptionType(Object receiver) throws UnsupportedMessageException {
assert receiver instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((UserScriptException) receiver)).getExceptionType();
}
@Override
public boolean isExceptionIncompleteSource(Object receiver) throws UnsupportedMessageException {
assert receiver instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((UserScriptException) receiver)).isExceptionIncompleteSource();
}
@Override
public boolean hasExceptionMessage(Object receiver) {
assert receiver instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((UserScriptException) receiver)).hasExceptionMessage();
}
@Override
public Object getExceptionMessage(Object receiver) throws UnsupportedMessageException {
assert receiver instanceof UserScriptException : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((UserScriptException) receiver)).getExceptionMessage();
}
}
@GeneratedBy(UserScriptException.class)
@DenyReplace
private static final class Uncached extends InteropLibrary implements DelegateExport, UnadoptableNode {
protected Uncached() {
}
@Override
public FinalBitSet getDelegateExportMessages() {
return ENABLED_MESSAGES;
}
@Override
public Object readDelegateExport(Object receiver_) {
return (((UserScriptException) receiver_)).exceptionObject;
}
@Override
public Library getDelegateExportLibrary(Object delegate_) {
return INTEROP_LIBRARY_.getUncached(delegate_);
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert !(receiver instanceof UserScriptException) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof UserScriptException;
}
@TruffleBoundary
@Override
public TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
// declared: false
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
GraalJSException arg0Value = ((GraalJSException) arg0Value_);
if (arg1Value instanceof GraalJSException) {
GraalJSException arg1Value_ = (GraalJSException) arg1Value;
return IsIdenticalOrUndefined.doException(arg0Value, arg1Value_, (INTEROP_LIBRARY_.getUncached()), (INTEROP_LIBRARY_.getUncached()));
}
if ((!(IsIdenticalOrUndefined.isGraalJSException(arg1Value)))) {
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value, (INTEROP_LIBRARY_.getUncached()), (INTEROP_LIBRARY_.getUncached()));
}
throw newUnsupportedSpecializationException2(this, arg0Value, arg1Value);
}
@TruffleBoundary
@Override
public boolean hasSourceLocation(Object receiver) {
// declared: false
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((GraalJSException) receiver) .hasSourceLocation();
}
@TruffleBoundary
@Override
public SourceSection getSourceLocation(Object receiver) throws UnsupportedMessageException {
// declared: false
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((GraalJSException) receiver) .getSourceLocationInterop();
}
@TruffleBoundary
@Override
public boolean hasLanguage(Object receiver) {
// declared: false
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((GraalJSException) receiver) .hasLanguage();
}
@TruffleBoundary
@Override
public Class extends TruffleLanguage>> getLanguage(Object receiver) throws UnsupportedMessageException {
// declared: false
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((GraalJSException) receiver) .getLanguage();
}
@TruffleBoundary
@Override
public Object toDisplayString(Object receiver, boolean allowSideEffects) {
// declared: false
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((GraalJSException) receiver) .toDisplayString(allowSideEffects);
}
@TruffleBoundary
@Override
public int identityHashCode(Object arg0Value_) throws UnsupportedMessageException {
// declared: false
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
GraalJSException arg0Value = ((GraalJSException) arg0Value_);
return arg0Value.identityHashCode((INTEROP_LIBRARY_.getUncached()));
}
@TruffleBoundary
@Override
public boolean isException(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((UserScriptException) receiver) .isException();
}
@TruffleBoundary
@Override
public RuntimeException throwException(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((UserScriptException) receiver) .throwException();
}
@TruffleBoundary
@Override
public ExceptionType getExceptionType(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((UserScriptException) receiver) .getExceptionType();
}
@TruffleBoundary
@Override
public boolean isExceptionIncompleteSource(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((UserScriptException) receiver) .isExceptionIncompleteSource();
}
@TruffleBoundary
@Override
public boolean hasExceptionMessage(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((UserScriptException) receiver) .hasExceptionMessage();
}
@TruffleBoundary
@Override
public Object getExceptionMessage(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((UserScriptException) receiver) .getExceptionMessage();
}
@TruffleBoundary
private static UnsupportedSpecializationException newUnsupportedSpecializationException2(Node thisNode_, Object arg0Value, Object arg1Value) {
return new UnsupportedSpecializationException(thisNode_, null, arg0Value, arg1Value);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy