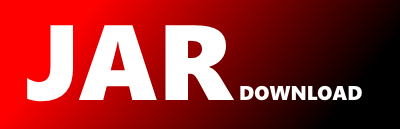
com.oracle.truffle.js.runtime.builtins.JSFunctionObjectGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.runtime.builtins;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.interop.ArityException;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.interop.UnsupportedTypeException;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.source.SourceSection;
import com.oracle.truffle.api.strings.TruffleString.FromJavaStringNode;
import com.oracle.truffle.js.nodes.interop.ExportValueNode;
import com.oracle.truffle.js.nodes.interop.JSInteropExecuteNode;
import com.oracle.truffle.js.nodes.interop.JSInteropExecuteNodeGen;
import com.oracle.truffle.js.nodes.interop.JSInteropGetIteratorNode;
import com.oracle.truffle.js.nodes.interop.JSInteropInstantiateNode;
import com.oracle.truffle.js.nodes.interop.JSInteropInstantiateNodeGen;
import com.oracle.truffle.js.nodes.interop.KeyInfoNode;
import com.oracle.truffle.js.nodes.unary.IsCallableNode;
import com.oracle.truffle.js.nodes.unary.IsCallableNodeGen;
import com.oracle.truffle.js.runtime.objects.JSNonProxyObjectGen;
import com.oracle.truffle.js.runtime.objects.JSObject;
import java.lang.invoke.VarHandle;
import java.util.Objects;
@GeneratedBy(JSFunctionObject.class)
@SuppressWarnings("javadoc")
public final class JSFunctionObjectGen {
static {
LibraryExport.register(JSFunctionObject.class, new InteropLibraryExports());
}
private JSFunctionObjectGen() {
}
@GeneratedBy(JSFunctionObject.class)
public static class InteropLibraryExports extends LibraryExport {
private InteropLibraryExports() {
super(InteropLibrary.class, JSFunctionObject.class, false, false, 0);
}
@Override
protected InteropLibrary createUncached(Object receiver) {
assert receiver instanceof JSFunctionObject;
InteropLibrary uncached = new Uncached(receiver);
return uncached;
}
@Override
protected InteropLibrary createCached(Object receiver) {
assert receiver instanceof JSFunctionObject;
return new Cached(receiver);
}
@GeneratedBy(JSFunctionObject.class)
public static class Cached extends JSNonProxyObjectGen.InteropLibraryExports.Cached {
/**
* State Info:
* 0: SpecializationActive {@link JSFunctionObject#isExecutable(JSFunctionObject, IsCallableNode)}
* 1: SpecializationActive {@link JSFunctionObject#execute(JSFunctionObject, Object[], InteropLibrary, JSInteropExecuteNode, ExportValueNode)}
* 2: SpecializationActive {@link JSFunctionObject#instantiate(JSFunctionObject, Object[], InteropLibrary, JSInteropInstantiateNode, ExportValueNode)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSObject#readMember}
* Parameter: {@link FromJavaStringNode} fromJavaString
*/
@Child private FromJavaStringNode fromJavaString;
/**
* Source Info:
* Specialization: {@link JSObject#isMemberReadable}
* Parameter: {@link KeyInfoNode} keyInfo
*/
@Child private KeyInfoNode keyInfo;
/**
* Source Info:
* Specialization: {@link JSObject#hasIterator}
* Parameter: {@link JSInteropGetIteratorNode} getIteratorNode
*/
@Child private JSInteropGetIteratorNode getIteratorNode;
/**
* Source Info:
* Specialization: {@link JSFunctionObject#execute}
* Parameter: {@link ExportValueNode} exportNode
*/
@Child private ExportValueNode exportNode;
/**
* Source Info:
* Specialization: {@link JSFunctionObject#isExecutable(JSFunctionObject, IsCallableNode)}
* Parameter: {@link IsCallableNode} isCallable
*/
@Child private IsCallableNode isExecutableNode__isExecutable_isCallable_;
/**
* Source Info:
* Specialization: {@link JSFunctionObject#execute(JSFunctionObject, Object[], InteropLibrary, JSInteropExecuteNode, ExportValueNode)}
* Parameter: {@link JSInteropExecuteNode} callNode
*/
@Child private JSInteropExecuteNode executeNode__execute_callNode_;
/**
* Source Info:
* Specialization: {@link JSFunctionObject#instantiate(JSFunctionObject, Object[], InteropLibrary, JSInteropInstantiateNode, ExportValueNode)}
* Parameter: {@link JSInteropInstantiateNode} callNode
*/
@Child private JSInteropInstantiateNode instantiateNode__instantiate_callNode_;
protected Cached(Object receiver) {
super(receiver);
}
/**
* Debug Info:
* Specialization {@link JSFunctionObject#isExecutable(JSFunctionObject, IsCallableNode)}
* Activation probability: 0.05556
* With/without class size: 5/4 bytes
*
*/
@Override
public boolean isExecutable(Object arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSFunctionObject arg0Value = ((JSFunctionObject) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSFunctionObject.isExecutable(JSFunctionObject, IsCallableNode)] */) {
{
IsCallableNode isCallable__ = this.isExecutableNode__isExecutable_isCallable_;
if (isCallable__ != null) {
return arg0Value.isExecutable(isCallable__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isExecutableNode_AndSpecialize(arg0Value);
}
private boolean isExecutableNode_AndSpecialize(JSFunctionObject arg0Value) {
int state_0 = this.state_0_;
IsCallableNode isCallable__ = this.insert((IsCallableNode.create()));
Objects.requireNonNull(isCallable__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.isExecutableNode__isExecutable_isCallable_ = isCallable__;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSFunctionObject.isExecutable(JSFunctionObject, IsCallableNode)] */;
this.state_0_ = state_0;
return arg0Value.isExecutable(isCallable__);
}
/**
* Debug Info:
* Specialization {@link JSFunctionObject#execute(JSFunctionObject, Object[], InteropLibrary, JSInteropExecuteNode, ExportValueNode)}
* Activation probability: 0.05556
* With/without class size: 5/4 bytes
*
*/
@Override
public Object execute(Object arg0Value_, Object... arg1Value) throws UnsupportedTypeException, ArityException, UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSFunctionObject arg0Value = ((JSFunctionObject) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSFunctionObject.execute(JSFunctionObject, Object[], InteropLibrary, JSInteropExecuteNode, ExportValueNode)] */) {
{
JSInteropExecuteNode callNode__ = this.executeNode__execute_callNode_;
if (callNode__ != null) {
ExportValueNode exportNode_ = this.exportNode;
if (exportNode_ != null) {
InteropLibrary self__ = (this);
return arg0Value.execute(arg1Value, self__, callNode__, exportNode_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeNode_AndSpecialize(arg0Value, arg1Value);
}
private Object executeNode_AndSpecialize(JSFunctionObject arg0Value, Object[] arg1Value) throws UnsupportedMessageException {
int state_0 = this.state_0_;
{
InteropLibrary self__ = null;
self__ = (this);
JSInteropExecuteNode callNode__ = this.insert((JSInteropExecuteNodeGen.create()));
Objects.requireNonNull(callNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.executeNode__execute_callNode_ = callNode__;
ExportValueNode exportNode_;
ExportValueNode exportNode__shared = this.exportNode;
if (exportNode__shared != null) {
exportNode_ = exportNode__shared;
} else {
exportNode_ = this.insert((ExportValueNode.create()));
if (exportNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.exportNode == null) {
VarHandle.storeStoreFence();
this.exportNode = exportNode_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[JSFunctionObject.execute(JSFunctionObject, Object[], InteropLibrary, JSInteropExecuteNode, ExportValueNode)] */;
this.state_0_ = state_0;
return arg0Value.execute(arg1Value, self__, callNode__, exportNode_);
}
}
@Override
public boolean isInstantiable(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((JSFunctionObject) receiver)).isInstantiable();
}
/**
* Debug Info:
* Specialization {@link JSFunctionObject#instantiate(JSFunctionObject, Object[], InteropLibrary, JSInteropInstantiateNode, ExportValueNode)}
* Activation probability: 0.05556
* With/without class size: 5/4 bytes
*
*/
@Override
public Object instantiate(Object arg0Value_, Object... arg1Value) throws UnsupportedTypeException, ArityException, UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSFunctionObject arg0Value = ((JSFunctionObject) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSFunctionObject.instantiate(JSFunctionObject, Object[], InteropLibrary, JSInteropInstantiateNode, ExportValueNode)] */) {
{
JSInteropInstantiateNode callNode__ = this.instantiateNode__instantiate_callNode_;
if (callNode__ != null) {
ExportValueNode exportNode_ = this.exportNode;
if (exportNode_ != null) {
InteropLibrary self__ = (this);
return arg0Value.instantiate(arg1Value, self__, callNode__, exportNode_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return instantiateNode_AndSpecialize(arg0Value, arg1Value);
}
private Object instantiateNode_AndSpecialize(JSFunctionObject arg0Value, Object[] arg1Value) throws UnsupportedMessageException {
int state_0 = this.state_0_;
{
InteropLibrary self__ = null;
self__ = (this);
JSInteropInstantiateNode callNode__ = this.insert((JSInteropInstantiateNodeGen.create()));
Objects.requireNonNull(callNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.instantiateNode__instantiate_callNode_ = callNode__;
ExportValueNode exportNode_;
ExportValueNode exportNode__shared = this.exportNode;
if (exportNode__shared != null) {
exportNode_ = exportNode__shared;
} else {
exportNode_ = this.insert((ExportValueNode.create()));
if (exportNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.exportNode == null) {
VarHandle.storeStoreFence();
this.exportNode = exportNode_;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[JSFunctionObject.instantiate(JSFunctionObject, Object[], InteropLibrary, JSInteropInstantiateNode, ExportValueNode)] */;
this.state_0_ = state_0;
return arg0Value.instantiate(arg1Value, self__, callNode__, exportNode_);
}
}
@Override
public boolean hasSourceLocation(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((JSFunctionObject) receiver)).hasSourceLocation();
}
@Override
public SourceSection getSourceLocation(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((JSFunctionObject) receiver)).getSourceLocation();
}
@Override
public boolean isMetaObject(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((JSFunctionObject) receiver)).isMetaObject();
}
@TruffleBoundary
@Override
public Object getMetaQualifiedName(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((JSFunctionObject) receiver)).getMetaObjectName();
}
@TruffleBoundary
@Override
public Object getMetaSimpleName(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((JSFunctionObject) receiver)).getMetaObjectName();
}
@TruffleBoundary
@Override
public boolean isMetaInstance(Object receiver, Object instance) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((JSFunctionObject) receiver)).isMetaInstance(instance);
}
}
@GeneratedBy(JSFunctionObject.class)
public static class Uncached extends JSNonProxyObjectGen.InteropLibraryExports.Uncached {
protected Uncached(Object receiver) {
super(receiver);
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
return super.accepts(receiver);
}
@TruffleBoundary
@Override
public boolean isExecutable(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSFunctionObject arg0Value = ((JSFunctionObject) arg0Value_);
return arg0Value.isExecutable((IsCallableNodeGen.getUncached()));
}
@TruffleBoundary
@Override
public Object execute(Object arg0Value_, Object... arg1Value) throws UnsupportedMessageException, UnsupportedTypeException, ArityException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSFunctionObject arg0Value = ((JSFunctionObject) arg0Value_);
return arg0Value.execute(arg1Value, (this), (JSInteropExecuteNodeGen.getUncached()), (ExportValueNode.getUncached()));
}
@TruffleBoundary
@Override
public boolean isInstantiable(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((JSFunctionObject) receiver) .isInstantiable();
}
@TruffleBoundary
@Override
public Object instantiate(Object arg0Value_, Object... arg1Value) throws UnsupportedMessageException, UnsupportedTypeException, ArityException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSFunctionObject arg0Value = ((JSFunctionObject) arg0Value_);
return arg0Value.instantiate(arg1Value, (this), (JSInteropInstantiateNodeGen.getUncached()), (ExportValueNode.getUncached()));
}
@TruffleBoundary
@Override
public boolean hasSourceLocation(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((JSFunctionObject) receiver) .hasSourceLocation();
}
@TruffleBoundary
@Override
public SourceSection getSourceLocation(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((JSFunctionObject) receiver) .getSourceLocation();
}
@TruffleBoundary
@Override
public boolean isMetaObject(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((JSFunctionObject) receiver) .isMetaObject();
}
@TruffleBoundary
@Override
public Object getMetaQualifiedName(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((JSFunctionObject) receiver) .getMetaObjectName();
}
@TruffleBoundary
@Override
public Object getMetaSimpleName(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((JSFunctionObject) receiver) .getMetaObjectName();
}
@TruffleBoundary
@Override
public boolean isMetaInstance(Object receiver, Object instance) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((JSFunctionObject) receiver) .isMetaInstance(instance);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy