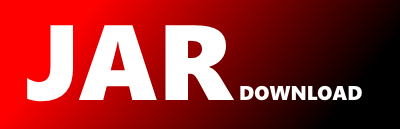
com.oracle.truffle.js.runtime.builtins.JSMapObjectGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.runtime.builtins;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.interop.UnknownKeyException;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.interop.UnsupportedTypeException;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.strings.TruffleString.FromJavaStringNode;
import com.oracle.truffle.js.builtins.helper.JSCollectionsNormalizeNode;
import com.oracle.truffle.js.builtins.helper.JSCollectionsNormalizeNodeGen;
import com.oracle.truffle.js.nodes.interop.ExportValueNode;
import com.oracle.truffle.js.nodes.interop.ImportValueNode;
import com.oracle.truffle.js.nodes.interop.JSInteropGetIteratorNode;
import com.oracle.truffle.js.nodes.interop.KeyInfoNode;
import com.oracle.truffle.js.runtime.objects.JSNonProxyObjectGen;
import com.oracle.truffle.js.runtime.objects.JSObject;
import java.lang.invoke.VarHandle;
import java.util.Objects;
@GeneratedBy(JSMapObject.class)
@SuppressWarnings("javadoc")
final class JSMapObjectGen {
static {
LibraryExport.register(JSMapObject.class, new InteropLibraryExports());
}
private JSMapObjectGen() {
}
@GeneratedBy(JSMapObject.class)
private static final class InteropLibraryExports extends LibraryExport {
private InteropLibraryExports() {
super(InteropLibrary.class, JSMapObject.class, false, false, 0);
}
@Override
protected InteropLibrary createUncached(Object receiver) {
assert receiver instanceof JSMapObject;
InteropLibrary uncached = new Uncached(receiver);
return uncached;
}
@Override
protected InteropLibrary createCached(Object receiver) {
assert receiver instanceof JSMapObject;
return new Cached(receiver);
}
@GeneratedBy(JSMapObject.class)
private static final class Cached extends JSNonProxyObjectGen.InteropLibraryExports.Cached {
/**
* State Info:
* 0: SpecializationActive {@link JSMapObject#isHashEntryReadable(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)}
* 1: SpecializationActive {@link JSMapObject#readHashValue(JSMapObject, Object, ExportValueNode, ImportValueNode, JSCollectionsNormalizeNode)}
* 2: SpecializationActive {@link JSMapObject#readHashValueOrDefault(JSMapObject, Object, Object, ExportValueNode, ImportValueNode, JSCollectionsNormalizeNode)}
* 3: SpecializationActive {@link JSMapObject#isHashEntryModifiable(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)}
* 4: SpecializationActive {@link JSMapObject#writeHashEntry(JSMapObject, Object, Object, ImportValueNode, ImportValueNode, JSCollectionsNormalizeNode)}
* 5: SpecializationActive {@link JSMapObject#removeHashEntry(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link JSObject#readMember}
* Parameter: {@link FromJavaStringNode} fromJavaString
*/
@Child private FromJavaStringNode fromJavaString;
/**
* Source Info:
* Specialization: {@link JSObject#isMemberReadable}
* Parameter: {@link KeyInfoNode} keyInfo
*/
@Child private KeyInfoNode keyInfo;
/**
* Source Info:
* Specialization: {@link JSObject#hasIterator}
* Parameter: {@link JSInteropGetIteratorNode} getIteratorNode
*/
@Child private JSInteropGetIteratorNode getIteratorNode;
/**
* Source Info:
* Specialization: {@link JSMapObject#isHashEntryReadable}
* Parameter: {@link ImportValueNode} importKeyNode
*/
@Child private ImportValueNode importKeyNode;
/**
* Source Info:
* Specialization: {@link JSMapObject#isHashEntryReadable}
* Parameter: {@link JSCollectionsNormalizeNode} normalizeKeyNode
*/
@Child private JSCollectionsNormalizeNode normalizeKeyNode;
/**
* Source Info:
* Specialization: {@link JSMapObject#readHashValue}
* Parameter: {@link ExportValueNode} exportValueNode
*/
@Child private ExportValueNode exportValueNode;
/**
* Source Info:
* Specialization: {@link JSMapObject#writeHashEntry(JSMapObject, Object, Object, ImportValueNode, ImportValueNode, JSCollectionsNormalizeNode)}
* Parameter: {@link ImportValueNode} importValueNode
*/
@Child private ImportValueNode writeHashEntryNode__writeHashEntry_importValueNode_;
protected Cached(Object receiver) {
super(receiver);
}
@Override
public boolean hasHashEntries(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((JSMapObject) receiver)).hasHashEntries();
}
@Override
public long getHashSize(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((JSMapObject) receiver)).getHashSize();
}
@Override
public Object getHashEntriesIterator(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((JSMapObject) receiver)).getHashEntriesIterator();
}
/**
* Debug Info:
* Specialization {@link JSMapObject#isHashEntryReadable(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)}
* Activation probability: 0.04348
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean isHashEntryReadable(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSMapObject.isHashEntryReadable(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)] */) {
{
ImportValueNode importKeyNode_ = this.importKeyNode;
if (importKeyNode_ != null) {
JSCollectionsNormalizeNode normalizeKeyNode_ = this.normalizeKeyNode;
if (normalizeKeyNode_ != null) {
return arg0Value.isHashEntryReadable(arg1Value, importKeyNode_, normalizeKeyNode_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isHashEntryReadableNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean isHashEntryReadableNode_AndSpecialize(JSMapObject arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
ImportValueNode importKeyNode_;
ImportValueNode importKeyNode__shared = this.importKeyNode;
if (importKeyNode__shared != null) {
importKeyNode_ = importKeyNode__shared;
} else {
importKeyNode_ = this.insert((ImportValueNode.create()));
if (importKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.importKeyNode == null) {
VarHandle.storeStoreFence();
this.importKeyNode = importKeyNode_;
}
JSCollectionsNormalizeNode normalizeKeyNode_;
JSCollectionsNormalizeNode normalizeKeyNode__shared = this.normalizeKeyNode;
if (normalizeKeyNode__shared != null) {
normalizeKeyNode_ = normalizeKeyNode__shared;
} else {
normalizeKeyNode_ = this.insert((JSCollectionsNormalizeNode.create()));
if (normalizeKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.normalizeKeyNode == null) {
VarHandle.storeStoreFence();
this.normalizeKeyNode = normalizeKeyNode_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[JSMapObject.isHashEntryReadable(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)] */;
this.state_0_ = state_0;
return arg0Value.isHashEntryReadable(arg1Value, importKeyNode_, normalizeKeyNode_);
}
/**
* Debug Info:
* Specialization {@link JSMapObject#readHashValue(JSMapObject, Object, ExportValueNode, ImportValueNode, JSCollectionsNormalizeNode)}
* Activation probability: 0.04348
* With/without class size: 4/0 bytes
*
*/
@Override
public Object readHashValue(Object arg0Value_, Object arg1Value) throws UnsupportedMessageException, UnknownKeyException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSMapObject.readHashValue(JSMapObject, Object, ExportValueNode, ImportValueNode, JSCollectionsNormalizeNode)] */) {
{
ExportValueNode exportValueNode_ = this.exportValueNode;
if (exportValueNode_ != null) {
ImportValueNode importKeyNode_ = this.importKeyNode;
if (importKeyNode_ != null) {
JSCollectionsNormalizeNode normalizeKeyNode_ = this.normalizeKeyNode;
if (normalizeKeyNode_ != null) {
return arg0Value.readHashValue(arg1Value, exportValueNode_, importKeyNode_, normalizeKeyNode_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readHashValueNode_AndSpecialize(arg0Value, arg1Value);
}
private Object readHashValueNode_AndSpecialize(JSMapObject arg0Value, Object arg1Value) throws UnknownKeyException {
int state_0 = this.state_0_;
ExportValueNode exportValueNode_;
ExportValueNode exportValueNode__shared = this.exportValueNode;
if (exportValueNode__shared != null) {
exportValueNode_ = exportValueNode__shared;
} else {
exportValueNode_ = this.insert((ExportValueNode.create()));
if (exportValueNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.exportValueNode == null) {
VarHandle.storeStoreFence();
this.exportValueNode = exportValueNode_;
}
ImportValueNode importKeyNode_;
ImportValueNode importKeyNode__shared = this.importKeyNode;
if (importKeyNode__shared != null) {
importKeyNode_ = importKeyNode__shared;
} else {
importKeyNode_ = this.insert((ImportValueNode.create()));
if (importKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.importKeyNode == null) {
VarHandle.storeStoreFence();
this.importKeyNode = importKeyNode_;
}
JSCollectionsNormalizeNode normalizeKeyNode_;
JSCollectionsNormalizeNode normalizeKeyNode__shared = this.normalizeKeyNode;
if (normalizeKeyNode__shared != null) {
normalizeKeyNode_ = normalizeKeyNode__shared;
} else {
normalizeKeyNode_ = this.insert((JSCollectionsNormalizeNode.create()));
if (normalizeKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.normalizeKeyNode == null) {
VarHandle.storeStoreFence();
this.normalizeKeyNode = normalizeKeyNode_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[JSMapObject.readHashValue(JSMapObject, Object, ExportValueNode, ImportValueNode, JSCollectionsNormalizeNode)] */;
this.state_0_ = state_0;
return arg0Value.readHashValue(arg1Value, exportValueNode_, importKeyNode_, normalizeKeyNode_);
}
/**
* Debug Info:
* Specialization {@link JSMapObject#readHashValueOrDefault(JSMapObject, Object, Object, ExportValueNode, ImportValueNode, JSCollectionsNormalizeNode)}
* Activation probability: 0.04348
* With/without class size: 4/0 bytes
*
*/
@Override
public Object readHashValueOrDefault(Object arg0Value_, Object arg1Value, Object arg2Value) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100) != 0 /* is SpecializationActive[JSMapObject.readHashValueOrDefault(JSMapObject, Object, Object, ExportValueNode, ImportValueNode, JSCollectionsNormalizeNode)] */) {
{
ExportValueNode exportValueNode_ = this.exportValueNode;
if (exportValueNode_ != null) {
ImportValueNode importKeyNode_ = this.importKeyNode;
if (importKeyNode_ != null) {
JSCollectionsNormalizeNode normalizeKeyNode_ = this.normalizeKeyNode;
if (normalizeKeyNode_ != null) {
return arg0Value.readHashValueOrDefault(arg1Value, arg2Value, exportValueNode_, importKeyNode_, normalizeKeyNode_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readHashValueOrDefaultNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object readHashValueOrDefaultNode_AndSpecialize(JSMapObject arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
ExportValueNode exportValueNode_;
ExportValueNode exportValueNode__shared = this.exportValueNode;
if (exportValueNode__shared != null) {
exportValueNode_ = exportValueNode__shared;
} else {
exportValueNode_ = this.insert((ExportValueNode.create()));
if (exportValueNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.exportValueNode == null) {
VarHandle.storeStoreFence();
this.exportValueNode = exportValueNode_;
}
ImportValueNode importKeyNode_;
ImportValueNode importKeyNode__shared = this.importKeyNode;
if (importKeyNode__shared != null) {
importKeyNode_ = importKeyNode__shared;
} else {
importKeyNode_ = this.insert((ImportValueNode.create()));
if (importKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.importKeyNode == null) {
VarHandle.storeStoreFence();
this.importKeyNode = importKeyNode_;
}
JSCollectionsNormalizeNode normalizeKeyNode_;
JSCollectionsNormalizeNode normalizeKeyNode__shared = this.normalizeKeyNode;
if (normalizeKeyNode__shared != null) {
normalizeKeyNode_ = normalizeKeyNode__shared;
} else {
normalizeKeyNode_ = this.insert((JSCollectionsNormalizeNode.create()));
if (normalizeKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.normalizeKeyNode == null) {
VarHandle.storeStoreFence();
this.normalizeKeyNode = normalizeKeyNode_;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[JSMapObject.readHashValueOrDefault(JSMapObject, Object, Object, ExportValueNode, ImportValueNode, JSCollectionsNormalizeNode)] */;
this.state_0_ = state_0;
return arg0Value.readHashValueOrDefault(arg1Value, arg2Value, exportValueNode_, importKeyNode_, normalizeKeyNode_);
}
/**
* Debug Info:
* Specialization {@link JSMapObject#isHashEntryModifiable(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)}
* Activation probability: 0.04348
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean isHashEntryModifiable(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSMapObject.isHashEntryModifiable(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)] */) {
{
ImportValueNode importKeyNode_ = this.importKeyNode;
if (importKeyNode_ != null) {
JSCollectionsNormalizeNode normalizeKeyNode_ = this.normalizeKeyNode;
if (normalizeKeyNode_ != null) {
return arg0Value.isHashEntryModifiable(arg1Value, importKeyNode_, normalizeKeyNode_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isHashEntryModifiableNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean isHashEntryModifiableNode_AndSpecialize(JSMapObject arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
ImportValueNode importKeyNode_;
ImportValueNode importKeyNode__shared = this.importKeyNode;
if (importKeyNode__shared != null) {
importKeyNode_ = importKeyNode__shared;
} else {
importKeyNode_ = this.insert((ImportValueNode.create()));
if (importKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.importKeyNode == null) {
VarHandle.storeStoreFence();
this.importKeyNode = importKeyNode_;
}
JSCollectionsNormalizeNode normalizeKeyNode_;
JSCollectionsNormalizeNode normalizeKeyNode__shared = this.normalizeKeyNode;
if (normalizeKeyNode__shared != null) {
normalizeKeyNode_ = normalizeKeyNode__shared;
} else {
normalizeKeyNode_ = this.insert((JSCollectionsNormalizeNode.create()));
if (normalizeKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.normalizeKeyNode == null) {
VarHandle.storeStoreFence();
this.normalizeKeyNode = normalizeKeyNode_;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[JSMapObject.isHashEntryModifiable(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)] */;
this.state_0_ = state_0;
return arg0Value.isHashEntryModifiable(arg1Value, importKeyNode_, normalizeKeyNode_);
}
/**
* Debug Info:
* Specialization {@link JSMapObject#isHashEntryModifiable(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)}
* Activation probability: 0.04348
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean isHashEntryRemovable(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[JSMapObject.isHashEntryModifiable(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)] */) {
{
ImportValueNode importKeyNode_ = this.importKeyNode;
if (importKeyNode_ != null) {
JSCollectionsNormalizeNode normalizeKeyNode_ = this.normalizeKeyNode;
if (normalizeKeyNode_ != null) {
return arg0Value.isHashEntryModifiable(arg1Value, importKeyNode_, normalizeKeyNode_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isHashEntryModifiableNode_AndSpecialize(arg0Value, arg1Value);
}
/**
* Debug Info:
* Specialization {@link JSMapObject#isHashEntryInsertable(JSMapObject, Object, InteropLibrary)}
* Activation probability: 0.04348
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean isHashEntryInsertable(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
{
InteropLibrary thisLibrary__ = (this);
return arg0Value.isHashEntryInsertable(arg1Value, thisLibrary__);
}
}
/**
* Debug Info:
* Specialization {@link JSMapObject#writeHashEntry(JSMapObject, Object, Object, ImportValueNode, ImportValueNode, JSCollectionsNormalizeNode)}
* Activation probability: 0.04348
* With/without class size: 4/4 bytes
*
*/
@Override
public void writeHashEntry(Object arg0Value_, Object arg1Value, Object arg2Value) throws UnsupportedMessageException, UnknownKeyException, UnsupportedTypeException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[JSMapObject.writeHashEntry(JSMapObject, Object, Object, ImportValueNode, ImportValueNode, JSCollectionsNormalizeNode)] */) {
{
ImportValueNode importKeyNode_ = this.importKeyNode;
if (importKeyNode_ != null) {
ImportValueNode importValueNode__ = this.writeHashEntryNode__writeHashEntry_importValueNode_;
if (importValueNode__ != null) {
JSCollectionsNormalizeNode normalizeKeyNode_ = this.normalizeKeyNode;
if (normalizeKeyNode_ != null) {
arg0Value.writeHashEntry(arg1Value, arg2Value, importKeyNode_, importValueNode__, normalizeKeyNode_);
return;
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
writeHashEntryNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void writeHashEntryNode_AndSpecialize(JSMapObject arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
ImportValueNode importKeyNode_;
ImportValueNode importKeyNode__shared = this.importKeyNode;
if (importKeyNode__shared != null) {
importKeyNode_ = importKeyNode__shared;
} else {
importKeyNode_ = this.insert((ImportValueNode.create()));
if (importKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.importKeyNode == null) {
VarHandle.storeStoreFence();
this.importKeyNode = importKeyNode_;
}
ImportValueNode importValueNode__ = this.insert((ImportValueNode.create()));
Objects.requireNonNull(importValueNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.writeHashEntryNode__writeHashEntry_importValueNode_ = importValueNode__;
JSCollectionsNormalizeNode normalizeKeyNode_;
JSCollectionsNormalizeNode normalizeKeyNode__shared = this.normalizeKeyNode;
if (normalizeKeyNode__shared != null) {
normalizeKeyNode_ = normalizeKeyNode__shared;
} else {
normalizeKeyNode_ = this.insert((JSCollectionsNormalizeNode.create()));
if (normalizeKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.normalizeKeyNode == null) {
VarHandle.storeStoreFence();
this.normalizeKeyNode = normalizeKeyNode_;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[JSMapObject.writeHashEntry(JSMapObject, Object, Object, ImportValueNode, ImportValueNode, JSCollectionsNormalizeNode)] */;
this.state_0_ = state_0;
arg0Value.writeHashEntry(arg1Value, arg2Value, importKeyNode_, importValueNode__, normalizeKeyNode_);
return;
}
/**
* Debug Info:
* Specialization {@link JSMapObject#removeHashEntry(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)}
* Activation probability: 0.04348
* With/without class size: 4/0 bytes
*
*/
@Override
public void removeHashEntry(Object arg0Value_, Object arg1Value) throws UnsupportedMessageException, UnknownKeyException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[JSMapObject.removeHashEntry(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)] */) {
{
ImportValueNode importKeyNode_ = this.importKeyNode;
if (importKeyNode_ != null) {
JSCollectionsNormalizeNode normalizeKeyNode_ = this.normalizeKeyNode;
if (normalizeKeyNode_ != null) {
arg0Value.removeHashEntry(arg1Value, importKeyNode_, normalizeKeyNode_);
return;
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
removeHashEntryNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void removeHashEntryNode_AndSpecialize(JSMapObject arg0Value, Object arg1Value) throws UnknownKeyException {
int state_0 = this.state_0_;
ImportValueNode importKeyNode_;
ImportValueNode importKeyNode__shared = this.importKeyNode;
if (importKeyNode__shared != null) {
importKeyNode_ = importKeyNode__shared;
} else {
importKeyNode_ = this.insert((ImportValueNode.create()));
if (importKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.importKeyNode == null) {
VarHandle.storeStoreFence();
this.importKeyNode = importKeyNode_;
}
JSCollectionsNormalizeNode normalizeKeyNode_;
JSCollectionsNormalizeNode normalizeKeyNode__shared = this.normalizeKeyNode;
if (normalizeKeyNode__shared != null) {
normalizeKeyNode_ = normalizeKeyNode__shared;
} else {
normalizeKeyNode_ = this.insert((JSCollectionsNormalizeNode.create()));
if (normalizeKeyNode_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.normalizeKeyNode == null) {
VarHandle.storeStoreFence();
this.normalizeKeyNode = normalizeKeyNode_;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[JSMapObject.removeHashEntry(JSMapObject, Object, ImportValueNode, JSCollectionsNormalizeNode)] */;
this.state_0_ = state_0;
arg0Value.removeHashEntry(arg1Value, importKeyNode_, normalizeKeyNode_);
return;
}
}
@GeneratedBy(JSMapObject.class)
@DenyReplace
private static final class Uncached extends JSNonProxyObjectGen.InteropLibraryExports.Uncached {
protected Uncached(Object receiver) {
super(receiver);
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
return super.accepts(receiver);
}
@TruffleBoundary
@Override
public boolean hasHashEntries(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((JSMapObject) receiver) .hasHashEntries();
}
@TruffleBoundary
@Override
public long getHashSize(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((JSMapObject) receiver) .getHashSize();
}
@TruffleBoundary
@Override
public Object getHashEntriesIterator(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((JSMapObject) receiver) .getHashEntriesIterator();
}
@TruffleBoundary
@Override
public boolean isHashEntryReadable(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
return arg0Value.isHashEntryReadable(arg1Value, (ImportValueNode.getUncached()), (JSCollectionsNormalizeNodeGen.getUncached()));
}
@TruffleBoundary
@Override
public Object readHashValue(Object arg0Value_, Object arg1Value) throws UnknownKeyException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
return arg0Value.readHashValue(arg1Value, (ExportValueNode.getUncached()), (ImportValueNode.getUncached()), (JSCollectionsNormalizeNodeGen.getUncached()));
}
@TruffleBoundary
@Override
public Object readHashValueOrDefault(Object arg0Value_, Object arg1Value, Object arg2Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
return arg0Value.readHashValueOrDefault(arg1Value, arg2Value, (ExportValueNode.getUncached()), (ImportValueNode.getUncached()), (JSCollectionsNormalizeNodeGen.getUncached()));
}
@TruffleBoundary
@Override
public boolean isHashEntryModifiable(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
return arg0Value.isHashEntryModifiable(arg1Value, (ImportValueNode.getUncached()), (JSCollectionsNormalizeNodeGen.getUncached()));
}
@TruffleBoundary
@Override
public boolean isHashEntryRemovable(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
return arg0Value.isHashEntryModifiable(arg1Value, (ImportValueNode.getUncached()), (JSCollectionsNormalizeNodeGen.getUncached()));
}
@TruffleBoundary
@Override
public boolean isHashEntryInsertable(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
return arg0Value.isHashEntryInsertable(arg1Value, (this));
}
@TruffleBoundary
@Override
public void writeHashEntry(Object arg0Value_, Object arg1Value, Object arg2Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
arg0Value.writeHashEntry(arg1Value, arg2Value, (ImportValueNode.getUncached()), (ImportValueNode.getUncached()), (JSCollectionsNormalizeNodeGen.getUncached()));
return;
}
@TruffleBoundary
@Override
public void removeHashEntry(Object arg0Value_, Object arg1Value) throws UnknownKeyException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSMapObject arg0Value = ((JSMapObject) arg0Value_);
arg0Value.removeHashEntry(arg1Value, (ImportValueNode.getUncached()), (JSCollectionsNormalizeNodeGen.getUncached()));
return;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy