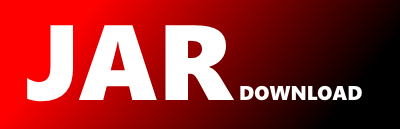
com.oracle.truffle.js.runtime.interop.InteropBoundFunctionGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.runtime.interop;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.interop.ArityException;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.interop.UnsupportedTypeException;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.Library;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.utilities.FinalBitSet;
import com.oracle.truffle.api.utilities.TriState;
import com.oracle.truffle.js.nodes.interop.ExportValueNode;
import com.oracle.truffle.js.nodes.interop.JSInteropExecuteNode;
import com.oracle.truffle.js.nodes.interop.JSInteropExecuteNodeGen;
import com.oracle.truffle.js.runtime.JSConfig;
import java.lang.invoke.VarHandle;
import java.util.Objects;
@GeneratedBy(InteropBoundFunction.class)
@SuppressWarnings("javadoc")
final class InteropBoundFunctionGen {
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(InteropBoundFunction.class, new InteropLibraryExports());
}
private InteropBoundFunctionGen() {
}
@GeneratedBy(InteropBoundFunction.class)
private static final class InteropLibraryExports extends LibraryExport {
static final FinalBitSet ENABLED_MESSAGES = createMessageBitSet(INTEROP_LIBRARY_, "isIdenticalOrUndefined", "identityHashCode", "isExecutable", "execute");
private InteropLibraryExports() {
super(InteropLibrary.class, InteropBoundFunction.class, false, false, 0);
}
@Override
protected InteropLibrary createUncached(Object receiver) {
assert receiver instanceof InteropBoundFunction;
InteropLibrary uncached = createDelegate(INTEROP_LIBRARY_, new Uncached());
return uncached;
}
@Override
protected InteropLibrary createCached(Object receiver) {
assert receiver instanceof InteropBoundFunction;
return createDelegate(INTEROP_LIBRARY_, new Cached(receiver));
}
@GeneratedBy(InteropBoundFunction.class)
private static final class Cached extends InteropLibrary implements DelegateExport {
@Child private InteropLibrary receiverFunctionInteropLibrary_;
/**
* State Info:
* 0: SpecializationActive {@link InteropFunction#isIdenticalOrUndefined(InteropFunction, Object, InteropLibrary, InteropLibrary)}
* 1: SpecializationActive {@link InteropBoundFunction#execute(InteropBoundFunction, Object[], InteropLibrary, JSInteropExecuteNode, ExportValueNode)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link InteropFunction#isIdenticalOrUndefined(InteropFunction, Object, InteropLibrary, InteropLibrary)}
* Parameter: {@link InteropLibrary} thisLib
*/
@Child private InteropLibrary isIdenticalOrUndefinedNode__isIdenticalOrUndefined_thisLib_;
/**
* Source Info:
* Specialization: {@link InteropFunction#isIdenticalOrUndefined(InteropFunction, Object, InteropLibrary, InteropLibrary)}
* Parameter: {@link InteropLibrary} otherLib
*/
@Child private InteropLibrary isIdenticalOrUndefinedNode__isIdenticalOrUndefined_otherLib_;
/**
* Source Info:
* Specialization: {@link InteropBoundFunction#execute(InteropBoundFunction, Object[], InteropLibrary, JSInteropExecuteNode, ExportValueNode)}
* Parameter: {@link JSInteropExecuteNode} callNode
*/
@Child private JSInteropExecuteNode executeNode__execute_callNode_;
/**
* Source Info:
* Specialization: {@link InteropBoundFunction#execute(InteropBoundFunction, Object[], InteropLibrary, JSInteropExecuteNode, ExportValueNode)}
* Parameter: {@link ExportValueNode} exportNode
*/
@Child private ExportValueNode executeNode__execute_exportNode_;
protected Cached(Object receiver) {
InteropBoundFunction castReceiver = ((InteropBoundFunction) receiver) ;
this.receiverFunctionInteropLibrary_ = INTEROP_LIBRARY_.create((castReceiver.function));
}
@Override
public FinalBitSet getDelegateExportMessages() {
return ENABLED_MESSAGES;
}
@Override
public Object readDelegateExport(Object receiver_) {
return ((InteropBoundFunction) receiver_).function;
}
@Override
public Library getDelegateExportLibrary(Object delegate) {
return this.receiverFunctionInteropLibrary_;
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof InteropBoundFunction) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
if (!(receiver instanceof InteropBoundFunction)) {
return false;
} else if (!this.receiverFunctionInteropLibrary_.accepts((((InteropBoundFunction) receiver).function))) {
return false;
} else {
return true;
}
}
/**
* Debug Info:
* Specialization {@link InteropFunction#isIdenticalOrUndefined(InteropFunction, Object, InteropLibrary, InteropLibrary)}
* Activation probability: 0.33333
* With/without class size: 12/8 bytes
*
*/
@Override
protected TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
assert arg0Value_ instanceof InteropBoundFunction : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
InteropBoundFunction arg0Value = ((InteropBoundFunction) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[InteropFunction.isIdenticalOrUndefined(InteropFunction, Object, InteropLibrary, InteropLibrary)] */) {
{
InteropLibrary thisLib__ = this.isIdenticalOrUndefinedNode__isIdenticalOrUndefined_thisLib_;
if (thisLib__ != null) {
InteropLibrary otherLib__ = this.isIdenticalOrUndefinedNode__isIdenticalOrUndefined_otherLib_;
if (otherLib__ != null) {
return arg0Value.isIdenticalOrUndefined(arg1Value, thisLib__, otherLib__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isIdenticalOrUndefinedNode_AndSpecialize(arg0Value, arg1Value);
}
private TriState isIdenticalOrUndefinedNode_AndSpecialize(InteropFunction arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
InteropLibrary thisLib__ = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
Objects.requireNonNull(thisLib__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.isIdenticalOrUndefinedNode__isIdenticalOrUndefined_thisLib_ = thisLib__;
InteropLibrary otherLib__ = this.insert((INTEROP_LIBRARY_.createDispatched(JSConfig.InteropLibraryLimit)));
Objects.requireNonNull(otherLib__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.isIdenticalOrUndefinedNode__isIdenticalOrUndefined_otherLib_ = otherLib__;
state_0 = state_0 | 0b1 /* add SpecializationActive[InteropFunction.isIdenticalOrUndefined(InteropFunction, Object, InteropLibrary, InteropLibrary)] */;
this.state_0_ = state_0;
return arg0Value.isIdenticalOrUndefined(arg1Value, thisLib__, otherLib__);
}
@TruffleBoundary
@Override
public int identityHashCode(Object receiver) throws UnsupportedMessageException {
assert receiver instanceof InteropBoundFunction : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((InteropBoundFunction) receiver)).identityHashCode();
}
@Override
public boolean isExecutable(Object receiver) {
assert receiver instanceof InteropBoundFunction : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((InteropBoundFunction) receiver)).isExecutable();
}
/**
* Debug Info:
* Specialization {@link InteropBoundFunction#execute(InteropBoundFunction, Object[], InteropLibrary, JSInteropExecuteNode, ExportValueNode)}
* Activation probability: 0.33333
* With/without class size: 12/8 bytes
*
*/
@Override
public Object execute(Object arg0Value_, Object... arg1Value) throws UnsupportedTypeException, ArityException, UnsupportedMessageException {
assert arg0Value_ instanceof InteropBoundFunction : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
InteropBoundFunction arg0Value = ((InteropBoundFunction) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[InteropBoundFunction.execute(InteropBoundFunction, Object[], InteropLibrary, JSInteropExecuteNode, ExportValueNode)] */) {
{
JSInteropExecuteNode callNode__ = this.executeNode__execute_callNode_;
if (callNode__ != null) {
ExportValueNode exportNode__ = this.executeNode__execute_exportNode_;
if (exportNode__ != null) {
InteropLibrary self__ = (((InteropLibrary) this.getParent()));
return arg0Value.execute(arg1Value, self__, callNode__, exportNode__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeNode_AndSpecialize(arg0Value, arg1Value);
}
private Object executeNode_AndSpecialize(InteropBoundFunction arg0Value, Object[] arg1Value) throws UnsupportedMessageException {
int state_0 = this.state_0_;
{
InteropLibrary self__ = null;
self__ = (((InteropLibrary) this.getParent()));
JSInteropExecuteNode callNode__ = this.insert((JSInteropExecuteNodeGen.create()));
Objects.requireNonNull(callNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.executeNode__execute_callNode_ = callNode__;
ExportValueNode exportNode__ = this.insert((ExportValueNode.create()));
Objects.requireNonNull(exportNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.executeNode__execute_exportNode_ = exportNode__;
state_0 = state_0 | 0b10 /* add SpecializationActive[InteropBoundFunction.execute(InteropBoundFunction, Object[], InteropLibrary, JSInteropExecuteNode, ExportValueNode)] */;
this.state_0_ = state_0;
return arg0Value.execute(arg1Value, self__, callNode__, exportNode__);
}
}
}
@GeneratedBy(InteropBoundFunction.class)
@DenyReplace
private static final class Uncached extends InteropLibrary implements DelegateExport, UnadoptableNode {
protected Uncached() {
}
@Override
public FinalBitSet getDelegateExportMessages() {
return ENABLED_MESSAGES;
}
@Override
public Object readDelegateExport(Object receiver_) {
return (((InteropBoundFunction) receiver_)).function;
}
@Override
public Library getDelegateExportLibrary(Object delegate_) {
return INTEROP_LIBRARY_.getUncached(delegate_);
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert !(receiver instanceof InteropBoundFunction) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof InteropBoundFunction;
}
@TruffleBoundary
@Override
public TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
// declared: false
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
InteropFunction arg0Value = ((InteropFunction) arg0Value_);
return arg0Value.isIdenticalOrUndefined(arg1Value, (INTEROP_LIBRARY_.getUncached()), (INTEROP_LIBRARY_.getUncached()));
}
@TruffleBoundary
@Override
public int identityHashCode(Object receiver) throws UnsupportedMessageException {
// declared: false
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((InteropFunction) receiver) .identityHashCode();
}
@TruffleBoundary
@Override
public boolean isExecutable(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((InteropBoundFunction) receiver) .isExecutable();
}
@TruffleBoundary
@Override
public Object execute(Object arg0Value_, Object... arg1Value) throws UnsupportedMessageException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
InteropBoundFunction arg0Value = ((InteropBoundFunction) arg0Value_);
return arg0Value.execute(arg1Value, (((InteropLibrary) this.getParent())), (JSInteropExecuteNodeGen.getUncached()), (ExportValueNode.getUncached()));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy