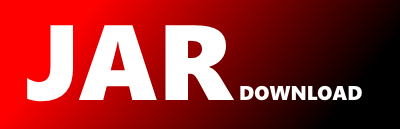
com.oracle.truffle.js.runtime.objects.JSDynamicObjectGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.js.runtime.objects;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.utilities.TriState;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject.IsIdenticalOrUndefined;
@GeneratedBy(JSDynamicObject.class)
@SuppressWarnings({"javadoc", "unused"})
public final class JSDynamicObjectGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(JSDynamicObject.class, new InteropLibraryExports());
}
private JSDynamicObjectGen() {
}
@GeneratedBy(JSDynamicObject.class)
public static class InteropLibraryExports extends LibraryExport {
private InteropLibraryExports() {
super(InteropLibrary.class, JSDynamicObject.class, false, false, 0);
}
@Override
protected InteropLibrary createUncached(Object receiver) {
assert receiver instanceof JSDynamicObject;
InteropLibrary uncached = new Uncached(receiver);
return uncached;
}
@Override
protected InteropLibrary createCached(Object receiver) {
assert receiver instanceof JSDynamicObject;
return new Cached(receiver);
}
@GeneratedBy(JSDynamicObject.class)
public static class Cached extends InteropLibrary {
private final Class extends JSDynamicObject> receiverClass_;
/**
* State Info:
* 0: SpecializationActive {@link IsIdenticalOrUndefined#doHostObject}
* 1: SpecializationActive {@link IsIdenticalOrUndefined#doOther}
*
*/
@CompilationFinal private int state_0_;
protected Cached(Object receiver) {
JSDynamicObject castReceiver = ((JSDynamicObject) receiver) ;
this.receiverClass_ = castReceiver.getClass();
}
@Override
public boolean accepts(Object receiver) {
assert receiver.getClass() != this.receiverClass_ || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return CompilerDirectives.isExact(receiver, this.receiverClass_);
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, JSDynamicObject arg0Value, Object arg1Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[JSDynamicObject.IsIdenticalOrUndefined.doHostObject(JSDynamicObject, JSDynamicObject)] */) && arg1Value instanceof JSDynamicObject) {
return false;
}
return true;
}
/**
* Debug Info:
* Specialization {@link IsIdenticalOrUndefined#doHostObject}
* Activation probability: 0.65000
* With/without class size: 11/0 bytes
* Specialization {@link IsIdenticalOrUndefined#doOther}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@Override
protected TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
JSDynamicObject arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[JSDynamicObject.IsIdenticalOrUndefined.doHostObject(JSDynamicObject, JSDynamicObject)] || SpecializationActive[JSDynamicObject.IsIdenticalOrUndefined.doOther(JSDynamicObject, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[JSDynamicObject.IsIdenticalOrUndefined.doHostObject(JSDynamicObject, JSDynamicObject)] */ && arg1Value instanceof JSDynamicObject) {
JSDynamicObject arg1Value_ = (JSDynamicObject) arg1Value;
return IsIdenticalOrUndefined.doHostObject(arg0Value, arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[JSDynamicObject.IsIdenticalOrUndefined.doOther(JSDynamicObject, Object)] */) {
if (fallbackGuard_(state_0, arg0Value, arg1Value)) {
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private TriState executeAndSpecialize(JSDynamicObject arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof JSDynamicObject) {
JSDynamicObject arg1Value_ = (JSDynamicObject) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[JSDynamicObject.IsIdenticalOrUndefined.doHostObject(JSDynamicObject, JSDynamicObject)] */;
this.state_0_ = state_0;
return IsIdenticalOrUndefined.doHostObject(arg0Value, arg1Value_);
}
state_0 = state_0 | 0b10 /* add SpecializationActive[JSDynamicObject.IsIdenticalOrUndefined.doOther(JSDynamicObject, Object)] */;
this.state_0_ = state_0;
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
@TruffleBoundary
@Override
public int identityHashCode(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (CompilerDirectives.castExact(receiver, receiverClass_)).identityHashCode();
}
}
@GeneratedBy(JSDynamicObject.class)
public static class Uncached extends InteropLibrary implements UnadoptableNode {
private final Class extends JSDynamicObject> receiverClass_;
protected Uncached(Object receiver) {
this.receiverClass_ = ((JSDynamicObject) receiver).getClass();
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert receiver.getClass() != this.receiverClass_ || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return CompilerDirectives.isExact(receiver, this.receiverClass_);
}
@TruffleBoundary
@Override
public TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
JSDynamicObject arg0Value = ((JSDynamicObject) arg0Value_);
if (arg1Value instanceof JSDynamicObject) {
JSDynamicObject arg1Value_ = (JSDynamicObject) arg1Value;
return IsIdenticalOrUndefined.doHostObject(arg0Value, arg1Value_);
}
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
@TruffleBoundary
@Override
public int identityHashCode(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((JSDynamicObject) receiver) .identityHashCode();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy