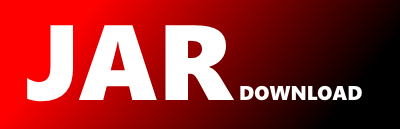
com.oracle.truffle.js.builtins.ReflectBuiltinsFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of js-language Show documentation
Show all versions of js-language Show documentation
Graal JavaScript implementation
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.js.builtins;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.strings.TruffleString.ToJavaStringNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectApplyNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectConstructNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectDefinePropertyNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectDeletePropertyNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectGetNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectGetOwnPropertyDescriptorNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectGetPrototypeOfNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectHasNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectIsExtensibleNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectOwnKeysNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectPreventExtensionsNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectSetNode;
import com.oracle.truffle.js.builtins.ReflectBuiltins.ReflectSetPrototypeOfNode;
import com.oracle.truffle.js.builtins.helper.ListSizeNode;
import com.oracle.truffle.js.builtins.helper.ListSizeNodeGen;
import com.oracle.truffle.js.nodes.JSGuards;
import com.oracle.truffle.js.nodes.JavaScriptNode;
import com.oracle.truffle.js.nodes.access.FromPropertyDescriptorNode;
import com.oracle.truffle.js.nodes.access.FromPropertyDescriptorNodeGen;
import com.oracle.truffle.js.nodes.access.IsExtensibleNode;
import com.oracle.truffle.js.nodes.access.IsObjectNode;
import com.oracle.truffle.js.nodes.access.JSGetOwnPropertyNode;
import com.oracle.truffle.js.nodes.access.ReadElementNode;
import com.oracle.truffle.js.nodes.access.ToPropertyDescriptorNode;
import com.oracle.truffle.js.nodes.cast.JSToPropertyKeyNode;
import com.oracle.truffle.js.nodes.control.DeletePropertyNode;
import com.oracle.truffle.js.nodes.function.JSBuiltin;
import com.oracle.truffle.js.nodes.interop.ForeignObjectPrototypeNode;
import com.oracle.truffle.js.nodes.unary.IsCallableNode;
import com.oracle.truffle.js.nodes.unary.IsConstructorNode;
import com.oracle.truffle.js.runtime.JSConfig;
import com.oracle.truffle.js.runtime.JSContext;
import com.oracle.truffle.js.runtime.objects.JSDynamicObject;
import com.oracle.truffle.js.runtime.objects.JSObject;
import com.oracle.truffle.js.runtime.util.JSClassProfile;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
@GeneratedBy(ReflectBuiltins.class)
@SuppressWarnings({"javadoc", "unused"})
public final class ReflectBuiltinsFactory {
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
/**
* Debug Info:
* Specialization {@link ReflectApplyNode#applyFunction}
* Activation probability: 0.48333
* With/without class size: 9/0 bytes
* Specialization {@link ReflectApplyNode#applyCallable}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
* Specialization {@link ReflectApplyNode#error}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(ReflectApplyNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectApplyNodeGen extends ReflectApplyNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
@Child private JavaScriptNode arguments2_;
/**
* State Info:
* 0: SpecializationActive {@link ReflectApplyNode#applyFunction}
* 1: SpecializationActive {@link ReflectApplyNode#applyCallable}
* 2: SpecializationActive {@link ReflectApplyNode#error}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link ReflectApplyNode#applyCallable}
* Parameter: {@link IsCallableNode} isCallable
*/
@Child private IsCallableNode isCallable;
private ReflectApplyNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_, this.arguments2_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
Object arguments2Value_ = this.arguments2_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[ReflectBuiltins.ReflectApplyNode.applyFunction(JSDynamicObject, Object, Object)] || SpecializationActive[ReflectBuiltins.ReflectApplyNode.applyCallable(Object, Object, Object, IsCallableNode)] || SpecializationActive[ReflectBuiltins.ReflectApplyNode.error(Object, Object, Object, IsCallableNode)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectApplyNode.applyFunction(JSDynamicObject, Object, Object)] */ && arguments0Value_ instanceof JSDynamicObject) {
JSDynamicObject arguments0Value__ = (JSDynamicObject) arguments0Value_;
if ((JSGuards.isJSFunction(arguments0Value__))) {
return applyFunction(arguments0Value__, arguments1Value_, arguments2Value_);
}
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectApplyNode.applyCallable(Object, Object, Object, IsCallableNode)] || SpecializationActive[ReflectBuiltins.ReflectApplyNode.error(Object, Object, Object, IsCallableNode)] */) {
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectApplyNode.applyCallable(Object, Object, Object, IsCallableNode)] */) {
{
IsCallableNode isCallable_ = this.isCallable;
if (isCallable_ != null) {
if ((isCallable_.executeBoolean(arguments0Value_))) {
return applyCallable(arguments0Value_, arguments1Value_, arguments2Value_, isCallable_);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectApplyNode.error(Object, Object, Object, IsCallableNode)] */) {
{
IsCallableNode isCallable_1 = this.isCallable;
if (isCallable_1 != null) {
if ((!(isCallable_1.executeBoolean(arguments0Value_)))) {
return ReflectApplyNode.error(arguments0Value_, arguments1Value_, arguments2Value_, isCallable_1);
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_, arguments1Value_, arguments2Value_);
}
private Object executeAndSpecialize(Object arguments0Value, Object arguments1Value, Object arguments2Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[ReflectBuiltins.ReflectApplyNode.applyCallable(Object, Object, Object, IsCallableNode)] */ && arguments0Value instanceof JSDynamicObject) {
JSDynamicObject arguments0Value_ = (JSDynamicObject) arguments0Value;
if ((JSGuards.isJSFunction(arguments0Value_))) {
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectBuiltins.ReflectApplyNode.applyFunction(JSDynamicObject, Object, Object)] */;
this.state_0_ = state_0;
return applyFunction(arguments0Value_, arguments1Value, arguments2Value);
}
}
{
IsCallableNode isCallable_;
IsCallableNode isCallable__shared = this.isCallable;
if (isCallable__shared != null) {
isCallable_ = isCallable__shared;
} else {
isCallable_ = this.insert((IsCallableNode.create()));
if (isCallable_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((isCallable_.executeBoolean(arguments0Value))) {
if (this.isCallable == null) {
VarHandle.storeStoreFence();
this.isCallable = isCallable_;
}
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[ReflectBuiltins.ReflectApplyNode.applyFunction(JSDynamicObject, Object, Object)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[ReflectBuiltins.ReflectApplyNode.applyCallable(Object, Object, Object, IsCallableNode)] */;
this.state_0_ = state_0;
return applyCallable(arguments0Value, arguments1Value, arguments2Value, isCallable_);
}
}
{
IsCallableNode isCallable_1;
IsCallableNode isCallable_1_shared = this.isCallable;
if (isCallable_1_shared != null) {
isCallable_1 = isCallable_1_shared;
} else {
isCallable_1 = this.insert((IsCallableNode.create()));
if (isCallable_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if ((!(isCallable_1.executeBoolean(arguments0Value)))) {
if (this.isCallable == null) {
VarHandle.storeStoreFence();
this.isCallable = isCallable_1;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[ReflectBuiltins.ReflectApplyNode.error(Object, Object, Object, IsCallableNode)] */;
this.state_0_ = state_0;
return ReflectApplyNode.error(arguments0Value, arguments1Value, arguments2Value, isCallable_1);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_, this.arguments1_, this.arguments2_}, arguments0Value, arguments1Value, arguments2Value);
}
@NeverDefault
public static ReflectApplyNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectApplyNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link ReflectConstructNode#reflectConstruct}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(ReflectConstructNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectConstructNodeGen extends ReflectConstructNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
@Child private JavaScriptNode arguments2_;
/**
* State Info:
* 0: SpecializationActive {@link ReflectConstructNode#reflectConstruct}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link ReflectConstructNode#reflectConstruct}
* Parameter: {@link IsConstructorNode} isConstructorNode
*/
@Child private IsConstructorNode isConstructorNode_;
private ReflectConstructNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_, this.arguments2_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
Object arguments2Value_ = this.arguments2_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[ReflectBuiltins.ReflectConstructNode.reflectConstruct(Object, Object, Object[], IsConstructorNode)] */ && arguments2Value_ instanceof Object[]) {
Object[] arguments2Value__ = (Object[]) arguments2Value_;
{
IsConstructorNode isConstructorNode__ = this.isConstructorNode_;
if (isConstructorNode__ != null) {
return reflectConstruct(arguments0Value_, arguments1Value_, arguments2Value__, isConstructorNode__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_, arguments1Value_, arguments2Value_);
}
private Object executeAndSpecialize(Object arguments0Value, Object arguments1Value, Object arguments2Value) {
int state_0 = this.state_0_;
if (arguments2Value instanceof Object[]) {
Object[] arguments2Value_ = (Object[]) arguments2Value;
IsConstructorNode isConstructorNode__ = this.insert((IsConstructorNode.create()));
Objects.requireNonNull(isConstructorNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.isConstructorNode_ = isConstructorNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectBuiltins.ReflectConstructNode.reflectConstruct(Object, Object, Object[], IsConstructorNode)] */;
this.state_0_ = state_0;
return reflectConstruct(arguments0Value, arguments1Value, arguments2Value_, isConstructorNode__);
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_, this.arguments1_, this.arguments2_}, arguments0Value, arguments1Value, arguments2Value);
}
@NeverDefault
public static ReflectConstructNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectConstructNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link ReflectDefinePropertyNode#reflectDefineProperty}
* Activation probability: 1.00000
* With/without class size: 28/8 bytes
*
*/
@GeneratedBy(ReflectDefinePropertyNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectDefinePropertyNodeGen extends ReflectDefinePropertyNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
@Child private JavaScriptNode arguments2_;
/**
* State Info:
* 0: SpecializationActive {@link ReflectDefinePropertyNode#reflectDefineProperty}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link ReflectDefinePropertyNode#reflectDefineProperty}
* Parameter: {@link JSToPropertyKeyNode} toPropertyKeyNode
*/
@Child private JSToPropertyKeyNode toPropertyKeyNode_;
/**
* Source Info:
* Specialization: {@link ReflectDefinePropertyNode#reflectDefineProperty}
* Parameter: {@link ToPropertyDescriptorNode} toPropertyDescriptorNode
*/
@Child private ToPropertyDescriptorNode toPropertyDescriptorNode_;
private ReflectDefinePropertyNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_, this.arguments2_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
Object arguments2Value_ = this.arguments2_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[ReflectBuiltins.ReflectDefinePropertyNode.reflectDefineProperty(Object, Object, Object, JSToPropertyKeyNode, ToPropertyDescriptorNode)] */) {
{
JSToPropertyKeyNode toPropertyKeyNode__ = this.toPropertyKeyNode_;
if (toPropertyKeyNode__ != null) {
ToPropertyDescriptorNode toPropertyDescriptorNode__ = this.toPropertyDescriptorNode_;
if (toPropertyDescriptorNode__ != null) {
return reflectDefineProperty(arguments0Value_, arguments1Value_, arguments2Value_, toPropertyKeyNode__, toPropertyDescriptorNode__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_, arguments1Value_, arguments2Value_);
}
private boolean executeAndSpecialize(Object arguments0Value, Object arguments1Value, Object arguments2Value) {
int state_0 = this.state_0_;
JSToPropertyKeyNode toPropertyKeyNode__ = this.insert((JSToPropertyKeyNode.create()));
Objects.requireNonNull(toPropertyKeyNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toPropertyKeyNode_ = toPropertyKeyNode__;
ToPropertyDescriptorNode toPropertyDescriptorNode__ = this.insert((ToPropertyDescriptorNode.create(getContext())));
Objects.requireNonNull(toPropertyDescriptorNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toPropertyDescriptorNode_ = toPropertyDescriptorNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectBuiltins.ReflectDefinePropertyNode.reflectDefineProperty(Object, Object, Object, JSToPropertyKeyNode, ToPropertyDescriptorNode)] */;
this.state_0_ = state_0;
return reflectDefineProperty(arguments0Value, arguments1Value, arguments2Value, toPropertyKeyNode__, toPropertyDescriptorNode__);
}
@NeverDefault
public static ReflectDefinePropertyNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectDefinePropertyNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link ReflectDeletePropertyNode#delete}
* Activation probability: 1.00000
* With/without class size: 32/12 bytes
*
*/
@GeneratedBy(ReflectDeletePropertyNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectDeletePropertyNodeGen extends ReflectDeletePropertyNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
/**
* State Info:
* 0: SpecializationActive {@link ReflectDeletePropertyNode#delete}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link ReflectDeletePropertyNode#delete}
* Parameter: {@link IsObjectNode} isObjectNode
*/
@Child private IsObjectNode isObjectNode_;
/**
* Source Info:
* Specialization: {@link ReflectDeletePropertyNode#delete}
* Parameter: {@link JSToPropertyKeyNode} toPropertyKeyNode
*/
@Child private JSToPropertyKeyNode toPropertyKeyNode_;
/**
* Source Info:
* Specialization: {@link ReflectDeletePropertyNode#delete}
* Parameter: {@link DeletePropertyNode} deletePropertyNode
*/
@Child private DeletePropertyNode deletePropertyNode_;
private ReflectDeletePropertyNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[ReflectBuiltins.ReflectDeletePropertyNode.delete(Object, Object, IsObjectNode, JSToPropertyKeyNode, DeletePropertyNode)] */) {
{
IsObjectNode isObjectNode__ = this.isObjectNode_;
if (isObjectNode__ != null) {
JSToPropertyKeyNode toPropertyKeyNode__ = this.toPropertyKeyNode_;
if (toPropertyKeyNode__ != null) {
DeletePropertyNode deletePropertyNode__ = this.deletePropertyNode_;
if (deletePropertyNode__ != null) {
return delete(arguments0Value_, arguments1Value_, isObjectNode__, toPropertyKeyNode__, deletePropertyNode__);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_, arguments1Value_);
}
private boolean executeAndSpecialize(Object arguments0Value, Object arguments1Value) {
int state_0 = this.state_0_;
IsObjectNode isObjectNode__ = this.insert((IsObjectNode.create()));
Objects.requireNonNull(isObjectNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.isObjectNode_ = isObjectNode__;
JSToPropertyKeyNode toPropertyKeyNode__ = this.insert((JSToPropertyKeyNode.create()));
Objects.requireNonNull(toPropertyKeyNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toPropertyKeyNode_ = toPropertyKeyNode__;
DeletePropertyNode deletePropertyNode__ = this.insert((DeletePropertyNode.create(false)));
Objects.requireNonNull(deletePropertyNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.deletePropertyNode_ = deletePropertyNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectBuiltins.ReflectDeletePropertyNode.delete(Object, Object, IsObjectNode, JSToPropertyKeyNode, DeletePropertyNode)] */;
this.state_0_ = state_0;
return delete(arguments0Value, arguments1Value, isObjectNode__, toPropertyKeyNode__, deletePropertyNode__);
}
@NeverDefault
public static ReflectDeletePropertyNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectDeletePropertyNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link ReflectGetNode#get}
* Activation probability: 1.00000
* With/without class size: 32/12 bytes
*
*/
@GeneratedBy(ReflectGetNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectGetNodeGen extends ReflectGetNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
@Child private JavaScriptNode arguments2_;
/**
* State Info:
* 0: SpecializationActive {@link ReflectGetNode#get}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link ReflectGetNode#get}
* Parameter: {@link IsObjectNode} isObjectNode
*/
@Child private IsObjectNode isObjectNode_;
/**
* Source Info:
* Specialization: {@link ReflectGetNode#get}
* Parameter: {@link JSToPropertyKeyNode} toPropertyKeyNode
*/
@Child private JSToPropertyKeyNode toPropertyKeyNode_;
/**
* Source Info:
* Specialization: {@link ReflectGetNode#get}
* Parameter: {@link ReadElementNode} readElementNode
*/
@Child private ReadElementNode readElementNode_;
private ReflectGetNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_, this.arguments2_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
Object arguments2Value_ = this.arguments2_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[ReflectBuiltins.ReflectGetNode.get(Object, Object, Object[], IsObjectNode, JSToPropertyKeyNode, ReadElementNode)] */ && arguments2Value_ instanceof Object[]) {
Object[] arguments2Value__ = (Object[]) arguments2Value_;
{
IsObjectNode isObjectNode__ = this.isObjectNode_;
if (isObjectNode__ != null) {
JSToPropertyKeyNode toPropertyKeyNode__ = this.toPropertyKeyNode_;
if (toPropertyKeyNode__ != null) {
ReadElementNode readElementNode__ = this.readElementNode_;
if (readElementNode__ != null) {
return get(arguments0Value_, arguments1Value_, arguments2Value__, isObjectNode__, toPropertyKeyNode__, readElementNode__);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_, arguments1Value_, arguments2Value_);
}
private Object executeAndSpecialize(Object arguments0Value, Object arguments1Value, Object arguments2Value) {
int state_0 = this.state_0_;
if (arguments2Value instanceof Object[]) {
Object[] arguments2Value_ = (Object[]) arguments2Value;
IsObjectNode isObjectNode__ = this.insert((IsObjectNode.create()));
Objects.requireNonNull(isObjectNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.isObjectNode_ = isObjectNode__;
JSToPropertyKeyNode toPropertyKeyNode__ = this.insert((JSToPropertyKeyNode.create()));
Objects.requireNonNull(toPropertyKeyNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toPropertyKeyNode_ = toPropertyKeyNode__;
ReadElementNode readElementNode__ = this.insert((ReadElementNode.create(getContext())));
Objects.requireNonNull(readElementNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.readElementNode_ = readElementNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectBuiltins.ReflectGetNode.get(Object, Object, Object[], IsObjectNode, JSToPropertyKeyNode, ReadElementNode)] */;
this.state_0_ = state_0;
return get(arguments0Value, arguments1Value, arguments2Value_, isObjectNode__, toPropertyKeyNode__, readElementNode__);
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_, this.arguments1_, this.arguments2_}, arguments0Value, arguments1Value, arguments2Value);
}
@NeverDefault
public static ReflectGetNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectGetNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link ReflectGetOwnPropertyDescriptorNode#reflectGetOwnPropertyDescriptor}
* Activation probability: 1.00000
* With/without class size: 32/12 bytes
*
*/
@GeneratedBy(ReflectGetOwnPropertyDescriptorNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectGetOwnPropertyDescriptorNodeGen extends ReflectGetOwnPropertyDescriptorNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
/**
* State Info:
* 0: SpecializationActive {@link ReflectGetOwnPropertyDescriptorNode#reflectGetOwnPropertyDescriptor}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link ReflectGetOwnPropertyDescriptorNode#reflectGetOwnPropertyDescriptor}
* Parameter: {@link JSToPropertyKeyNode} toPropertyKeyNode
*/
@Child private JSToPropertyKeyNode toPropertyKeyNode_;
/**
* Source Info:
* Specialization: {@link ReflectGetOwnPropertyDescriptorNode#reflectGetOwnPropertyDescriptor}
* Parameter: {@link JSGetOwnPropertyNode} getOwnPropertyNode
*/
@Child private JSGetOwnPropertyNode getOwnPropertyNode_;
/**
* Source Info:
* Specialization: {@link ReflectGetOwnPropertyDescriptorNode#reflectGetOwnPropertyDescriptor}
* Parameter: {@link FromPropertyDescriptorNode} fromPropertyDescriptorNode
*/
@Child private FromPropertyDescriptorNode fromPropertyDescriptorNode_;
private ReflectGetOwnPropertyDescriptorNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[ReflectBuiltins.ReflectGetOwnPropertyDescriptorNode.reflectGetOwnPropertyDescriptor(Object, Object, JSToPropertyKeyNode, JSGetOwnPropertyNode, FromPropertyDescriptorNode)] */) {
{
JSToPropertyKeyNode toPropertyKeyNode__ = this.toPropertyKeyNode_;
if (toPropertyKeyNode__ != null) {
JSGetOwnPropertyNode getOwnPropertyNode__ = this.getOwnPropertyNode_;
if (getOwnPropertyNode__ != null) {
FromPropertyDescriptorNode fromPropertyDescriptorNode__ = this.fromPropertyDescriptorNode_;
if (fromPropertyDescriptorNode__ != null) {
return reflectGetOwnPropertyDescriptor(arguments0Value_, arguments1Value_, toPropertyKeyNode__, getOwnPropertyNode__, fromPropertyDescriptorNode__);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_, arguments1Value_);
}
private JSDynamicObject executeAndSpecialize(Object arguments0Value, Object arguments1Value) {
int state_0 = this.state_0_;
JSToPropertyKeyNode toPropertyKeyNode__ = this.insert((JSToPropertyKeyNode.create()));
Objects.requireNonNull(toPropertyKeyNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toPropertyKeyNode_ = toPropertyKeyNode__;
JSGetOwnPropertyNode getOwnPropertyNode__ = this.insert((JSGetOwnPropertyNode.create()));
Objects.requireNonNull(getOwnPropertyNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.getOwnPropertyNode_ = getOwnPropertyNode__;
FromPropertyDescriptorNode fromPropertyDescriptorNode__ = this.insert((FromPropertyDescriptorNodeGen.create()));
Objects.requireNonNull(fromPropertyDescriptorNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.fromPropertyDescriptorNode_ = fromPropertyDescriptorNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectBuiltins.ReflectGetOwnPropertyDescriptorNode.reflectGetOwnPropertyDescriptor(Object, Object, JSToPropertyKeyNode, JSGetOwnPropertyNode, FromPropertyDescriptorNode)] */;
this.state_0_ = state_0;
return reflectGetOwnPropertyDescriptor(arguments0Value, arguments1Value, toPropertyKeyNode__, getOwnPropertyNode__, fromPropertyDescriptorNode__);
}
@NeverDefault
public static ReflectGetOwnPropertyDescriptorNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectGetOwnPropertyDescriptorNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link ReflectGetPrototypeOfNode#reflectGetPrototypeOf}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(ReflectGetPrototypeOfNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectGetPrototypeOfNodeGen extends ReflectGetPrototypeOfNode {
@Child private JavaScriptNode arguments0_;
private ReflectGetPrototypeOfNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
return reflectGetPrototypeOf(arguments0Value_);
}
@NeverDefault
public static ReflectGetPrototypeOfNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectGetPrototypeOfNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link ReflectHasNode#doObject}
* Activation probability: 0.38500
* With/without class size: 8/0 bytes
* Specialization {@link ReflectHasNode#doForeignObject}
* Activation probability: 0.29500
* With/without class size: 13/12 bytes
* Specialization {@link ReflectHasNode#doForeignObject}
* Activation probability: 0.20500
* With/without class size: 8/8 bytes
* Specialization {@link ReflectHasNode#doNonObject}
* Activation probability: 0.11500
* With/without class size: 5/0 bytes
*
*/
@GeneratedBy(ReflectHasNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectHasNodeGen extends ReflectHasNode {
static final ReferenceField FOREIGN_OBJECT0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "foreignObject0_cache", ForeignObject0Data.class);
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
/**
* State Info:
* 0: SpecializationActive {@link ReflectHasNode#doObject}
* 1: SpecializationActive {@link ReflectHasNode#doForeignObject}
* 2: SpecializationActive {@link ReflectHasNode#doForeignObject}
* 3: SpecializationActive {@link ReflectHasNode#doNonObject}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link ReflectHasNode#doObject}
* Parameter: {@link JSClassProfile} jsclassProfile
*/
@CompilationFinal private JSClassProfile jsclassProfile;
@UnsafeAccessedField @Child private ForeignObject0Data foreignObject0_cache;
/**
* Source Info:
* Specialization: {@link ReflectHasNode#doForeignObject}
* Parameter: {@link ToJavaStringNode} toJavaStringNode
*/
@Child private ToJavaStringNode foreignObject1_toJavaStringNode_;
/**
* Source Info:
* Specialization: {@link ReflectHasNode#doForeignObject}
* Parameter: {@link ForeignObjectPrototypeNode} foreignObjectPrototypeNode
*/
@Child private ForeignObjectPrototypeNode foreignObject1_foreignObjectPrototypeNode_;
private ReflectHasNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_};
}
@ExplodeLoop
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[ReflectBuiltins.ReflectHasNode.doObject(JSObject, Object, JSClassProfile)] || SpecializationActive[ReflectBuiltins.ReflectHasNode.doForeignObject(Object, Object, InteropLibrary, ToJavaStringNode, ForeignObjectPrototypeNode, JSClassProfile)] || SpecializationActive[ReflectBuiltins.ReflectHasNode.doForeignObject(Object, Object, InteropLibrary, ToJavaStringNode, ForeignObjectPrototypeNode, JSClassProfile)] || SpecializationActive[ReflectBuiltins.ReflectHasNode.doNonObject(Object, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectHasNode.doObject(JSObject, Object, JSClassProfile)] */ && arguments0Value_ instanceof JSObject) {
JSObject arguments0Value__ = (JSObject) arguments0Value_;
{
JSClassProfile jsclassProfile_ = this.jsclassProfile;
if (jsclassProfile_ != null) {
return doObject(arguments0Value__, arguments1Value_, jsclassProfile_);
}
}
}
if ((state_0 & 0b1110) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectHasNode.doForeignObject(Object, Object, InteropLibrary, ToJavaStringNode, ForeignObjectPrototypeNode, JSClassProfile)] || SpecializationActive[ReflectBuiltins.ReflectHasNode.doForeignObject(Object, Object, InteropLibrary, ToJavaStringNode, ForeignObjectPrototypeNode, JSClassProfile)] || SpecializationActive[ReflectBuiltins.ReflectHasNode.doNonObject(Object, Object)] */) {
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectHasNode.doForeignObject(Object, Object, InteropLibrary, ToJavaStringNode, ForeignObjectPrototypeNode, JSClassProfile)] */) {
ForeignObject0Data s1_ = this.foreignObject0_cache;
while (s1_ != null) {
{
JSClassProfile jsclassProfile_1 = this.jsclassProfile;
if (jsclassProfile_1 != null) {
if ((s1_.interop_.accepts(arguments0Value_)) && (JSGuards.isForeignObject(arguments0Value_))) {
return doForeignObject(arguments0Value_, arguments1Value_, s1_.interop_, s1_.toJavaStringNode_, s1_.foreignObjectPrototypeNode_, jsclassProfile_1);
}
}
}
s1_ = s1_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectHasNode.doForeignObject(Object, Object, InteropLibrary, ToJavaStringNode, ForeignObjectPrototypeNode, JSClassProfile)] */) {
{
ToJavaStringNode toJavaStringNode__ = this.foreignObject1_toJavaStringNode_;
if (toJavaStringNode__ != null) {
ForeignObjectPrototypeNode foreignObjectPrototypeNode__ = this.foreignObject1_foreignObjectPrototypeNode_;
if (foreignObjectPrototypeNode__ != null) {
JSClassProfile jsclassProfile_1 = this.jsclassProfile;
if (jsclassProfile_1 != null) {
if ((JSGuards.isForeignObject(arguments0Value_))) {
return this.foreignObject1Boundary(state_0, arguments0Value_, arguments1Value_, toJavaStringNode__, foreignObjectPrototypeNode__, jsclassProfile_1);
}
}
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectHasNode.doNonObject(Object, Object)] */) {
if ((!(JSGuards.isJSObject(arguments0Value_))) && (!(JSGuards.isForeignObject(arguments0Value_)))) {
return doNonObject(arguments0Value_, arguments1Value_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_, arguments1Value_);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object foreignObject1Boundary(int state_0, Object arguments0Value_, Object arguments1Value_, ToJavaStringNode toJavaStringNode__, ForeignObjectPrototypeNode foreignObjectPrototypeNode__, JSClassProfile jsclassProfile_1) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(arguments0Value_));
return doForeignObject(arguments0Value_, arguments1Value_, interop__, toJavaStringNode__, foreignObjectPrototypeNode__, jsclassProfile_1);
}
} finally {
encapsulating_.set(prev_);
}
}
private Object executeAndSpecialize(Object arguments0Value, Object arguments1Value) {
int state_0 = this.state_0_;
if (arguments0Value instanceof JSObject) {
JSObject arguments0Value_ = (JSObject) arguments0Value;
JSClassProfile jsclassProfile_;
JSClassProfile jsclassProfile__shared = this.jsclassProfile;
if (jsclassProfile__shared != null) {
jsclassProfile_ = jsclassProfile__shared;
} else {
jsclassProfile_ = (JSClassProfile.create());
if (jsclassProfile_ == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.jsclassProfile == null) {
VarHandle.storeStoreFence();
this.jsclassProfile = jsclassProfile_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectBuiltins.ReflectHasNode.doObject(JSObject, Object, JSClassProfile)] */;
this.state_0_ = state_0;
return doObject(arguments0Value_, arguments1Value, jsclassProfile_);
}
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[ReflectBuiltins.ReflectHasNode.doForeignObject(Object, Object, InteropLibrary, ToJavaStringNode, ForeignObjectPrototypeNode, JSClassProfile)] */) {
while (true) {
int count1_ = 0;
ForeignObject0Data s1_ = FOREIGN_OBJECT0_CACHE_UPDATER.getVolatile(this);
ForeignObject0Data s1_original = s1_;
while (s1_ != null) {
{
JSClassProfile jsclassProfile_1 = this.jsclassProfile;
if (jsclassProfile_1 != null) {
if ((s1_.interop_.accepts(arguments0Value)) && (JSGuards.isForeignObject(arguments0Value))) {
break;
}
}
}
count1_++;
s1_ = s1_.next_;
}
if (s1_ == null) {
if ((JSGuards.isForeignObject(arguments0Value)) && count1_ < (JSConfig.InteropLibraryLimit)) {
// assert (s1_.interop_.accepts(arguments0Value));
s1_ = this.insert(new ForeignObject0Data(s1_original));
InteropLibrary interop__ = s1_.insert((INTEROP_LIBRARY_.create(arguments0Value)));
Objects.requireNonNull(interop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.interop_ = interop__;
ToJavaStringNode toJavaStringNode__ = s1_.insert((ToJavaStringNode.create()));
Objects.requireNonNull(toJavaStringNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.toJavaStringNode_ = toJavaStringNode__;
ForeignObjectPrototypeNode foreignObjectPrototypeNode__ = s1_.insert((ForeignObjectPrototypeNode.create()));
Objects.requireNonNull(foreignObjectPrototypeNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.foreignObjectPrototypeNode_ = foreignObjectPrototypeNode__;
JSClassProfile jsclassProfile_1;
JSClassProfile jsclassProfile_1_shared = this.jsclassProfile;
if (jsclassProfile_1_shared != null) {
jsclassProfile_1 = jsclassProfile_1_shared;
} else {
jsclassProfile_1 = (JSClassProfile.create());
if (jsclassProfile_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.jsclassProfile == null) {
this.jsclassProfile = jsclassProfile_1;
}
if (!FOREIGN_OBJECT0_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[ReflectBuiltins.ReflectHasNode.doForeignObject(Object, Object, InteropLibrary, ToJavaStringNode, ForeignObjectPrototypeNode, JSClassProfile)] */;
this.state_0_ = state_0;
}
}
if (s1_ != null) {
return doForeignObject(arguments0Value, arguments1Value, s1_.interop_, s1_.toJavaStringNode_, s1_.foreignObjectPrototypeNode_, this.jsclassProfile);
}
break;
}
}
{
InteropLibrary interop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
if ((JSGuards.isForeignObject(arguments0Value))) {
interop__ = (INTEROP_LIBRARY_.getUncached(arguments0Value));
ToJavaStringNode toJavaStringNode__ = this.insert((ToJavaStringNode.create()));
Objects.requireNonNull(toJavaStringNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.foreignObject1_toJavaStringNode_ = toJavaStringNode__;
ForeignObjectPrototypeNode foreignObjectPrototypeNode__ = this.insert((ForeignObjectPrototypeNode.create()));
Objects.requireNonNull(foreignObjectPrototypeNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.foreignObject1_foreignObjectPrototypeNode_ = foreignObjectPrototypeNode__;
JSClassProfile jsclassProfile_1;
JSClassProfile jsclassProfile_1_shared = this.jsclassProfile;
if (jsclassProfile_1_shared != null) {
jsclassProfile_1 = jsclassProfile_1_shared;
} else {
jsclassProfile_1 = (JSClassProfile.create());
if (jsclassProfile_1 == null) {
throw new IllegalStateException("A specialization returned a default value for a cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.jsclassProfile == null) {
VarHandle.storeStoreFence();
this.jsclassProfile = jsclassProfile_1;
}
this.foreignObject0_cache = null;
state_0 = state_0 & 0xfffffffd /* remove SpecializationActive[ReflectBuiltins.ReflectHasNode.doForeignObject(Object, Object, InteropLibrary, ToJavaStringNode, ForeignObjectPrototypeNode, JSClassProfile)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[ReflectBuiltins.ReflectHasNode.doForeignObject(Object, Object, InteropLibrary, ToJavaStringNode, ForeignObjectPrototypeNode, JSClassProfile)] */;
this.state_0_ = state_0;
return doForeignObject(arguments0Value, arguments1Value, interop__, toJavaStringNode__, foreignObjectPrototypeNode__, jsclassProfile_1);
}
} finally {
encapsulating_.set(prev_);
}
}
}
if ((!(JSGuards.isJSObject(arguments0Value))) && (!(JSGuards.isForeignObject(arguments0Value)))) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[ReflectBuiltins.ReflectHasNode.doNonObject(Object, Object)] */;
this.state_0_ = state_0;
return doNonObject(arguments0Value, arguments1Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_, this.arguments1_}, arguments0Value, arguments1Value);
}
@NeverDefault
public static ReflectHasNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectHasNodeGen(context, builtin, arguments);
}
@GeneratedBy(ReflectHasNode.class)
@DenyReplace
private static final class ForeignObject0Data extends Node implements SpecializationDataNode {
@Child ForeignObject0Data next_;
/**
* Source Info:
* Specialization: {@link ReflectHasNode#doForeignObject}
* Parameter: {@link InteropLibrary} interop
*/
@Child InteropLibrary interop_;
/**
* Source Info:
* Specialization: {@link ReflectHasNode#doForeignObject}
* Parameter: {@link ToJavaStringNode} toJavaStringNode
*/
@Child ToJavaStringNode toJavaStringNode_;
/**
* Source Info:
* Specialization: {@link ReflectHasNode#doForeignObject}
* Parameter: {@link ForeignObjectPrototypeNode} foreignObjectPrototypeNode
*/
@Child ForeignObjectPrototypeNode foreignObjectPrototypeNode_;
ForeignObject0Data(ForeignObject0Data next_) {
this.next_ = next_;
}
}
}
/**
* Debug Info:
* Specialization {@link ReflectIsExtensibleNode#reflectIsExtensible}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(ReflectIsExtensibleNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectIsExtensibleNodeGen extends ReflectIsExtensibleNode {
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link ReflectIsExtensibleNode#reflectIsExtensible}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link ReflectIsExtensibleNode#reflectIsExtensible}
* Parameter: {@link IsExtensibleNode} isExtensibleNode
*/
@Child private IsExtensibleNode isExtensibleNode_;
private ReflectIsExtensibleNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[ReflectBuiltins.ReflectIsExtensibleNode.reflectIsExtensible(Object, IsExtensibleNode)] */) {
{
IsExtensibleNode isExtensibleNode__ = this.isExtensibleNode_;
if (isExtensibleNode__ != null) {
return reflectIsExtensible(arguments0Value_, isExtensibleNode__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private boolean executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
IsExtensibleNode isExtensibleNode__ = this.insert((IsExtensibleNode.create()));
Objects.requireNonNull(isExtensibleNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.isExtensibleNode_ = isExtensibleNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectBuiltins.ReflectIsExtensibleNode.reflectIsExtensible(Object, IsExtensibleNode)] */;
this.state_0_ = state_0;
return reflectIsExtensible(arguments0Value, isExtensibleNode__);
}
@NeverDefault
public static ReflectIsExtensibleNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectIsExtensibleNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link ReflectOwnKeysNode#reflectOwnKeys}
* Activation probability: 0.38500
* With/without class size: 13/8 bytes
* Specialization {@link ReflectOwnKeysNode#doForeignObject}
* Activation probability: 0.29500
* With/without class size: 11/4 bytes
* Specialization {@link ReflectOwnKeysNode#doForeignObject}
* Activation probability: 0.20500
* With/without class size: 6/0 bytes
* Specialization {@link ReflectOwnKeysNode#doNonObject}
* Activation probability: 0.11500
* With/without class size: 5/0 bytes
*
*/
@GeneratedBy(ReflectOwnKeysNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectOwnKeysNodeGen extends ReflectOwnKeysNode {
static final ReferenceField FOREIGN_OBJECT0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "foreignObject0_cache", ForeignObject0Data.class);
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link ReflectOwnKeysNode#reflectOwnKeys}
* 1: SpecializationActive {@link ReflectOwnKeysNode#doForeignObject}
* 2: SpecializationActive {@link ReflectOwnKeysNode#doForeignObject}
* 3: SpecializationActive {@link ReflectOwnKeysNode#doNonObject}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link ReflectOwnKeysNode#reflectOwnKeys}
* Parameter: {@link JSClassProfile} jsclassProfile
*/
@CompilationFinal private JSClassProfile reflectOwnKeys_jsclassProfile_;
/**
* Source Info:
* Specialization: {@link ReflectOwnKeysNode#reflectOwnKeys}
* Parameter: {@link ListSizeNode} listSize
*/
@Child private ListSizeNode reflectOwnKeys_listSize_;
@UnsafeAccessedField @Child private ForeignObject0Data foreignObject0_cache;
private ReflectOwnKeysNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@ExplodeLoop
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.reflectOwnKeys(JSObject, JSClassProfile, ListSizeNode)] || SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doForeignObject(Object, InteropLibrary)] || SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doForeignObject(Object, InteropLibrary)] || SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doNonObject(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.reflectOwnKeys(JSObject, JSClassProfile, ListSizeNode)] */ && arguments0Value_ instanceof JSObject) {
JSObject arguments0Value__ = (JSObject) arguments0Value_;
{
JSClassProfile jsclassProfile__ = this.reflectOwnKeys_jsclassProfile_;
if (jsclassProfile__ != null) {
ListSizeNode listSize__ = this.reflectOwnKeys_listSize_;
if (listSize__ != null) {
return reflectOwnKeys(arguments0Value__, jsclassProfile__, listSize__);
}
}
}
}
if ((state_0 & 0b1110) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doForeignObject(Object, InteropLibrary)] || SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doForeignObject(Object, InteropLibrary)] || SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doNonObject(Object)] */) {
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doForeignObject(Object, InteropLibrary)] */) {
ForeignObject0Data s1_ = this.foreignObject0_cache;
while (s1_ != null) {
if ((s1_.interop_.accepts(arguments0Value_)) && (JSGuards.isForeignObject(arguments0Value_))) {
return doForeignObject(arguments0Value_, s1_.interop_);
}
s1_ = s1_.next_;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doForeignObject(Object, InteropLibrary)] */) {
if ((JSGuards.isForeignObject(arguments0Value_))) {
return this.foreignObject1Boundary(state_0, arguments0Value_);
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doNonObject(Object)] */) {
if ((!(JSGuards.isJSObject(arguments0Value_))) && (!(JSGuards.isForeignObject(arguments0Value_)))) {
return doNonObject(arguments0Value_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object foreignObject1Boundary(int state_0, Object arguments0Value_) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(arguments0Value_));
return doForeignObject(arguments0Value_, interop__);
}
} finally {
encapsulating_.set(prev_);
}
}
private Object executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
if (arguments0Value instanceof JSObject) {
JSObject arguments0Value_ = (JSObject) arguments0Value;
JSClassProfile jsclassProfile__ = (JSClassProfile.create());
Objects.requireNonNull(jsclassProfile__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.reflectOwnKeys_jsclassProfile_ = jsclassProfile__;
ListSizeNode listSize__ = this.insert((ListSizeNodeGen.create()));
Objects.requireNonNull(listSize__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.reflectOwnKeys_listSize_ = listSize__;
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.reflectOwnKeys(JSObject, JSClassProfile, ListSizeNode)] */;
this.state_0_ = state_0;
return reflectOwnKeys(arguments0Value_, jsclassProfile__, listSize__);
}
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doForeignObject(Object, InteropLibrary)] */) {
while (true) {
int count1_ = 0;
ForeignObject0Data s1_ = FOREIGN_OBJECT0_CACHE_UPDATER.getVolatile(this);
ForeignObject0Data s1_original = s1_;
while (s1_ != null) {
if ((s1_.interop_.accepts(arguments0Value)) && (JSGuards.isForeignObject(arguments0Value))) {
break;
}
count1_++;
s1_ = s1_.next_;
}
if (s1_ == null) {
if ((JSGuards.isForeignObject(arguments0Value)) && count1_ < (JSConfig.InteropLibraryLimit)) {
// assert (s1_.interop_.accepts(arguments0Value));
s1_ = this.insert(new ForeignObject0Data(s1_original));
InteropLibrary interop__ = s1_.insert((INTEROP_LIBRARY_.create(arguments0Value)));
Objects.requireNonNull(interop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s1_.interop_ = interop__;
if (!FOREIGN_OBJECT0_CACHE_UPDATER.compareAndSet(this, s1_original, s1_)) {
continue;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doForeignObject(Object, InteropLibrary)] */;
this.state_0_ = state_0;
}
}
if (s1_ != null) {
return doForeignObject(arguments0Value, s1_.interop_);
}
break;
}
}
{
InteropLibrary interop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
if ((JSGuards.isForeignObject(arguments0Value))) {
interop__ = (INTEROP_LIBRARY_.getUncached(arguments0Value));
this.foreignObject0_cache = null;
state_0 = state_0 & 0xfffffffd /* remove SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doForeignObject(Object, InteropLibrary)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doForeignObject(Object, InteropLibrary)] */;
this.state_0_ = state_0;
return doForeignObject(arguments0Value, interop__);
}
} finally {
encapsulating_.set(prev_);
}
}
}
if ((!(JSGuards.isJSObject(arguments0Value))) && (!(JSGuards.isForeignObject(arguments0Value)))) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[ReflectBuiltins.ReflectOwnKeysNode.doNonObject(Object)] */;
this.state_0_ = state_0;
return doNonObject(arguments0Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_}, arguments0Value);
}
@NeverDefault
public static ReflectOwnKeysNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectOwnKeysNodeGen(context, builtin, arguments);
}
@GeneratedBy(ReflectOwnKeysNode.class)
@DenyReplace
private static final class ForeignObject0Data extends Node implements SpecializationDataNode {
@Child ForeignObject0Data next_;
/**
* Source Info:
* Specialization: {@link ReflectOwnKeysNode#doForeignObject}
* Parameter: {@link InteropLibrary} interop
*/
@Child InteropLibrary interop_;
ForeignObject0Data(ForeignObject0Data next_) {
this.next_ = next_;
}
}
}
/**
* Debug Info:
* Specialization {@link ReflectPreventExtensionsNode#reflectPreventExtensions}
* Activation probability: 0.65000
* With/without class size: 11/0 bytes
* Specialization {@link ReflectPreventExtensionsNode#doNonObject}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@GeneratedBy(ReflectPreventExtensionsNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectPreventExtensionsNodeGen extends ReflectPreventExtensionsNode {
@Child private JavaScriptNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link ReflectPreventExtensionsNode#reflectPreventExtensions}
* 1: SpecializationActive {@link ReflectPreventExtensionsNode#doNonObject}
*
*/
@CompilationFinal private int state_0_;
private ReflectPreventExtensionsNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[ReflectBuiltins.ReflectPreventExtensionsNode.reflectPreventExtensions(JSObject)] || SpecializationActive[ReflectBuiltins.ReflectPreventExtensionsNode.doNonObject(Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectPreventExtensionsNode.reflectPreventExtensions(JSObject)] */ && arguments0Value_ instanceof JSObject) {
JSObject arguments0Value__ = (JSObject) arguments0Value_;
return ReflectPreventExtensionsNode.reflectPreventExtensions(arguments0Value__);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectPreventExtensionsNode.doNonObject(Object)] */) {
if ((!(JSGuards.isJSObject(arguments0Value_)))) {
return doNonObject(arguments0Value_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private boolean executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
if (arguments0Value instanceof JSObject) {
JSObject arguments0Value_ = (JSObject) arguments0Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectBuiltins.ReflectPreventExtensionsNode.reflectPreventExtensions(JSObject)] */;
this.state_0_ = state_0;
return ReflectPreventExtensionsNode.reflectPreventExtensions(arguments0Value_);
}
if ((!(JSGuards.isJSObject(arguments0Value)))) {
state_0 = state_0 | 0b10 /* add SpecializationActive[ReflectBuiltins.ReflectPreventExtensionsNode.doNonObject(Object)] */;
this.state_0_ = state_0;
return doNonObject(arguments0Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_}, arguments0Value);
}
@NeverDefault
public static ReflectPreventExtensionsNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectPreventExtensionsNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link ReflectSetNode#reflectSet}
* Activation probability: 0.48333
* With/without class size: 11/4 bytes
* Specialization {@link ReflectSetNode#doForeignObject}
* Activation probability: 0.33333
* With/without class size: 10/4 bytes
* Specialization {@link ReflectSetNode#doNonObject}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(ReflectSetNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectSetNodeGen extends ReflectSetNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
@Child private JavaScriptNode arguments2_;
@Child private JavaScriptNode arguments3_;
/**
* State Info:
* 0: SpecializationActive {@link ReflectSetNode#reflectSet}
* 1: SpecializationActive {@link ReflectSetNode#doForeignObject}
* 2: SpecializationActive {@link ReflectSetNode#doNonObject}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link ReflectSetNode#reflectSet}
* Parameter: {@link JSClassProfile} jsclassProfile
*/
@CompilationFinal private JSClassProfile reflectSet_jsclassProfile_;
/**
* Source Info:
* Specialization: {@link ReflectSetNode#doForeignObject}
* Parameter: {@link IsObjectNode} isObjectNode
*/
@Child private IsObjectNode foreignObject_isObjectNode_;
private ReflectSetNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
this.arguments2_ = arguments != null && 2 < arguments.length ? arguments[2] : null;
this.arguments3_ = arguments != null && 3 < arguments.length ? arguments[3] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_, this.arguments2_, this.arguments3_};
}
@Override
public Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
Object arguments2Value_ = this.arguments2_.execute(frameValue);
Object arguments3Value_ = this.arguments3_.execute(frameValue);
if (state_0 != 0 /* is SpecializationActive[ReflectBuiltins.ReflectSetNode.reflectSet(JSDynamicObject, Object, Object, Object[], JSClassProfile)] || SpecializationActive[ReflectBuiltins.ReflectSetNode.doForeignObject(Object, Object, Object, Object[], IsObjectNode)] || SpecializationActive[ReflectBuiltins.ReflectSetNode.doNonObject(Object, Object, Object, Object[])] */ && arguments3Value_ instanceof Object[]) {
Object[] arguments3Value__ = (Object[]) arguments3Value_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectSetNode.reflectSet(JSDynamicObject, Object, Object, Object[], JSClassProfile)] */ && arguments0Value_ instanceof JSDynamicObject) {
JSDynamicObject arguments0Value__ = (JSDynamicObject) arguments0Value_;
{
JSClassProfile jsclassProfile__ = this.reflectSet_jsclassProfile_;
if (jsclassProfile__ != null) {
if ((JSGuards.isJSObject(arguments0Value__))) {
return reflectSet(arguments0Value__, arguments1Value_, arguments2Value_, arguments3Value__, jsclassProfile__);
}
}
}
}
if ((state_0 & 0b110) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectSetNode.doForeignObject(Object, Object, Object, Object[], IsObjectNode)] || SpecializationActive[ReflectBuiltins.ReflectSetNode.doNonObject(Object, Object, Object, Object[])] */) {
if ((state_0 & 0b10) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectSetNode.doForeignObject(Object, Object, Object, Object[], IsObjectNode)] */) {
{
IsObjectNode isObjectNode__ = this.foreignObject_isObjectNode_;
if (isObjectNode__ != null) {
if ((JSGuards.isForeignObject(arguments0Value_))) {
return doForeignObject(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value__, isObjectNode__);
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[ReflectBuiltins.ReflectSetNode.doNonObject(Object, Object, Object, Object[])] */) {
if ((!(JSGuards.isJSObject(arguments0Value_))) && (!(JSGuards.isForeignObject(arguments0Value_)))) {
return doNonObject(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_, arguments1Value_, arguments2Value_, arguments3Value_);
}
private boolean executeAndSpecialize(Object arguments0Value, Object arguments1Value, Object arguments2Value, Object arguments3Value) {
int state_0 = this.state_0_;
if (arguments3Value instanceof Object[]) {
Object[] arguments3Value_ = (Object[]) arguments3Value;
if (arguments0Value instanceof JSDynamicObject) {
JSDynamicObject arguments0Value_ = (JSDynamicObject) arguments0Value;
if ((JSGuards.isJSObject(arguments0Value_))) {
JSClassProfile jsclassProfile__ = (JSClassProfile.create());
Objects.requireNonNull(jsclassProfile__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.reflectSet_jsclassProfile_ = jsclassProfile__;
state_0 = state_0 | 0b1 /* add SpecializationActive[ReflectBuiltins.ReflectSetNode.reflectSet(JSDynamicObject, Object, Object, Object[], JSClassProfile)] */;
this.state_0_ = state_0;
return reflectSet(arguments0Value_, arguments1Value, arguments2Value, arguments3Value_, jsclassProfile__);
}
}
if ((JSGuards.isForeignObject(arguments0Value))) {
IsObjectNode isObjectNode__ = this.insert((IsObjectNode.create()));
Objects.requireNonNull(isObjectNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.foreignObject_isObjectNode_ = isObjectNode__;
state_0 = state_0 | 0b10 /* add SpecializationActive[ReflectBuiltins.ReflectSetNode.doForeignObject(Object, Object, Object, Object[], IsObjectNode)] */;
this.state_0_ = state_0;
return doForeignObject(arguments0Value, arguments1Value, arguments2Value, arguments3Value_, isObjectNode__);
}
if ((!(JSGuards.isJSObject(arguments0Value))) && (!(JSGuards.isForeignObject(arguments0Value)))) {
state_0 = state_0 | 0b100 /* add SpecializationActive[ReflectBuiltins.ReflectSetNode.doNonObject(Object, Object, Object, Object[])] */;
this.state_0_ = state_0;
return doNonObject(arguments0Value, arguments1Value, arguments2Value, arguments3Value_);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_, this.arguments1_, this.arguments2_, this.arguments3_}, arguments0Value, arguments1Value, arguments2Value, arguments3Value);
}
@NeverDefault
public static ReflectSetNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectSetNodeGen(context, builtin, arguments);
}
}
/**
* Debug Info:
* Specialization {@link ReflectSetPrototypeOfNode#reflectSetPrototypeOf}
* Activation probability: 1.00000
* With/without class size: 16/0 bytes
*
*/
@GeneratedBy(ReflectSetPrototypeOfNode.class)
@SuppressWarnings("javadoc")
public static final class ReflectSetPrototypeOfNodeGen extends ReflectSetPrototypeOfNode {
@Child private JavaScriptNode arguments0_;
@Child private JavaScriptNode arguments1_;
private ReflectSetPrototypeOfNodeGen(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
super(context, builtin);
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
this.arguments1_ = arguments != null && 1 < arguments.length ? arguments[1] : null;
}
@Override
public JavaScriptNode[] getArguments() {
return new JavaScriptNode[] {this.arguments0_, this.arguments1_};
}
@Override
public Object execute(VirtualFrame frameValue) {
Object arguments0Value_ = this.arguments0_.execute(frameValue);
Object arguments1Value_ = this.arguments1_.execute(frameValue);
return reflectSetPrototypeOf(arguments0Value_, arguments1Value_);
}
@NeverDefault
public static ReflectSetPrototypeOfNode create(JSContext context, JSBuiltin builtin, JavaScriptNode[] arguments) {
return new ReflectSetPrototypeOfNodeGen(context, builtin, arguments);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy